Ruby Tricks, Idiomatic Ruby, Refactorings and Best Practices
Conditional assignment.
Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read.
There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
If you're just assigning based on a boolean, the ternary operator works best (this is not specific to ruby/lisp but still good and in the spirit of the following examples!).
If you need to perform more complex logic based on a conditional or allow for > 2 outcomes, if / elsif / else statements work well.
If you're assigning based on the value of a single variable, use case / when A good barometer is to think if you could represent the assignment in a hash. For example, in the following example you could look up the value of opinion in a hash that looks like {"ANGRY" => comfort, "MEH" => ignore ...}
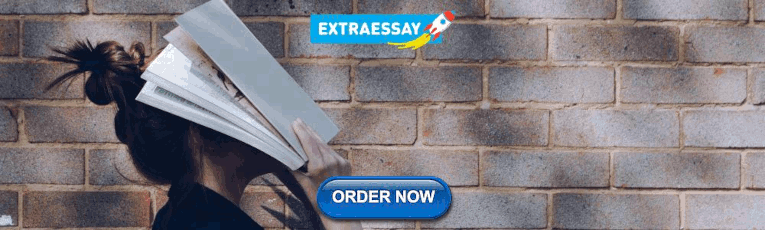
Conditionals
if statements allow you to take different actions depending on which conditions are met. For example:
- if the city is equal to ( == ) "Toronto" , then set drinking_age to 19.
- Otherwise ( else ) set drinking_age to 21.
true and false
Ruby has a notion of true and false. This is best illustrated through some example. Start irb and type the following:
The if statements evaluates whether the expression (e.g. ' city == "Toronto" ) is true or false and acts accordingly.
Most common conditionals
Here is a list of some of the most common conditionals:
String comparisons
How do these operators behave with strings? Well, == is string equality and > and friends are determined by ASCIIbetical order.
What is ASCIIbetical order? The ASCII character table contains all the characters in the keyboard. It lists them in this order:
Start irb and type these in:
elsif allows you to add more than one condition. Take this for example:
Let's go through this:
- If age is 60 or more, we give a senior fare.
- If that's not true, but age is 14 or more, we give the adult fare.
- If that's not true, but age is more than 2 we give the child fare.
- Otherwise we ride free.
Ruby goes through this sequence of conditions one by one. The first condition to hold gets executed. You can put as many elsif 's as you like.
Example - fare_finder.rb
To make things more clear, let's put this in a program. The program asks for your age and gives you the corresponding fare.
Type this in and run it. It should behave like this:
This will output:
Here age is both greater than 10 and greater than 20. Only the first statement that holds true gets executed.
The correct way to write this would be:
Re-arrange these characters in ASCIIbetical order:
The ASCII table contains all the characters in the keyboard. Use irb to find out wheter the characters "?" lies:
- After 9 but before A.
- After Z but before a.
Using your experience the previous question, make a program that accepts a character input and tells you if the character lines:
Then try the program with the following characters:
Sample answers:
- $ lies before 0
- < lies between 9 and A
- - lies between Z and a
- ~ lies after z
Ruby: If Statements
One of the most powerful features present in most programming and scripting languages is the ability to change the flow of the program as certain conditions change. The simplest form of flow control and logic in Ruby is called an "if statement" (or technically speaking in Ruby, since everything is an expression, an "if expression").
These 'if' experssions essentially check whether a condition is true or not. In Ruby they are created by writing the if keyword, followed by a condition, optionally followed by the then keyword, then the code you want to execute if the condition is true, and then finally the end keyword to end the structure. So they should look something like the following:
As mentioned though, the then is often dropped from the structure as it simply provides separation from the condition and the code to execute - one-line 'if' statements require the then to separate these, but multi-line ones like we'll be writing most of the time can use a new line to separate the condition and code.
From here the obvious question is: "How do I formulate a condition?". Well firstly, it's important to note that any expression that evaluates to a 'true' or 'false' value can be in the place of the condition there -- that includes boolean variables and method calls! So the following would always output "Hello" as 'some_variable' will, in this case, always evaluate to true:
"Real" conditions, for example comparing one variable to another, can be created by using 'conditional' or 'comparison' operators. These are operators that are made for comparing things, and when values are present at both sides of the operator, this will result in a 'true' or 'false' value. The basic conditional operators are:
- == - Is equal to
- != - Is not equal to
- > - Is greater than
- < - Is less than
- >= - Is greater than or equal to
- <= - Is less than or equal to
So to put these into actions, let's create a basic script which checks if the user is an administrator of our made-up system – it's not going to be very secure or really good for anything at the moment, but it'll be a good example to make sure we know this stuff.
The easiest way to authenticate a user is probably via a username and password, so the first thing our example script should do is ask for this data. Luckily we already know how to do this using puts and gets , however in this example it's more suitable to use print instead of puts - the two essentially do the same thing, however puts appends a newline to the output whereas print does not. So let's write this portion of the script!
From here, the 'if' statements need to do their magic. In this case we can just hard-code the administrator username and password into our script (not a good idea in a real system, but it'll work fine here). So first our comparison between the administrator username and what the user actually entered:
From here we want to check if the password is correct. If statements and other similar structures can actually be nested, so in this case it might be a nice idea to put another 'if' statement inside this one to check if the password is correct, like so:
You can see I've added a little puts message so that we can tell when the username and password were correct ("foo" and "bar"). If you take our code so far and put it into a ruby file and run it, you should see that our special message only displays when "foo" and "bar" are entered! But what do we do if we want something to happen when the username and password are entered wrongly? Perhaps we want to display a message telling the user that the details they entered were wrong. We could write a few 'if' statements that use the "is not equal to" ( != ) operator, however this seems like a waste of processing power given that we've already determined if the credentials are correct or not. This is where 'else' statements come in.
After an 'if' statement, just before 'end', you can introduce an 'else' clause. The code in this clause will execute if the 'if' statement was not true (and if none of the other 'if's in that chain were true, but we'll get on to this in a minute). These are accomplished using the else keyword where end would usually be, and moving end to the end. The syntax is as follows:
In our example we could easily utilise this functionality to output errors to the user in our example program:
As I previously touched on however, you can actually have more than one condition in an 'if' statement "chain", in which case the 'else' clause will only happen if none of the conditions are true. A nice way to show this in our example is if our system had multiple users. Perhaps we want to add a 'guest' user which doesn't require a password. We can add these "extra conditions" by using what is called an 'else if', written via the elsif keyword. Reading 'if' chains out-loud often makes some sense of them: "If this, do this, else if this, do this, else if this, do this, else, do this", this should also make obvious the fact that the next item in the chain will only be proceeded to if the previous is false – so elsif s are only checked if the primary condition is false, and else s are only checked if everything else is false. The syntax of an 'elsif' in a chain is as follows:
With this, it should be fairly easy to create a guest user:
You could argue that asking the 'guest' user for their password isn't necessary, and this could be fixed by moving the password prompt and gets into the administrator 'if' statement, and feel free to see if you can do this, however it's not important for this example.
The best way to learn about 'if' statements is really to play around with them. A good idea might be to try creating a script which takes the score for an exam out of 100 and then outputs a grade depending on the score entered (within certain grade boundaries – the greater than and less than comparisons should be useful here!). The only problem you might run into in creating this script is the comparison of different data types. If you try to compare a string variable (for example something received from gets ) with an integer (like 1), then you will run into problems. As such, you might want to explore to to_i and to_s methods. The former will likely want to be used in this example and is a method to convert strings to integers, while the latter is less useful in this example however may be more useful in others, and converts integers to strings.
You could use the to_i method on the gets.chomp itself (probably the better option here), or if you wanted you could use it in every condition with comparison to an integer. The former is shown, basically, below:
Moving away from this a bit, which hopefully you should now understand and be able to use, there is also some more flexibility and some more shorthand options in this 'if' functionality. Firstly, a basic 'if' can actually be after the line that should be executed if it's true, like so:
Similarly, a basic 'if' statement can be compressed to one line if the then keyword is used to replace the newline:
Ruby also has something called a "ternary operator" which provides a shortcut way of making basic comparisons. The syntax of this is a condition, followed by a question mark, followed by the expression that should be given if the condition is true, followed by a colon, followed by the expression that should be given if the condition is false, as can be seen below:
This is useful when a quick and compact decision between expressions is necessary, for example the following:

Conditional Statements in Ruby
Conditional statements are an essential part of any programming language, including Ruby. They give us the ability to make decisions and alter the flow of our code based on certain conditions. In this tutorial, we will delve into the basics of conditional statements in Ruby, exploring various types and their usage.
If Statements
The most basic form of a conditional statement is the if statement. It allows us to execute a block of code only if a certain condition is met.
Here is a simple example:
In this example, we first declare a variable age with a value of 25. The if statement checks whether age is greater than 18. If the condition evaluates to true, the code inside the if block is executed, and the message "You are an adult!" is printed.
We can also add an else block after the if block to handle cases when the condition is not met. Taking the previous example further:
In this case, since age is 16, the condition age > 18 is false, and thus the code in the else block is executed, printing "You are not yet an adult."
ElseIf Statements
Ruby provides the elsif statement as a way to add additional conditions to our if statements. This allows us to handle multiple possible outcomes in a more organized manner.
Consider the following example:
In this example, we have multiple conditions. If age is less than 18, the first if block is executed. If not, the program checks the next condition using the elsif keyword. If age is between 18 and 65, the second elsif block is executed. Finally, if none of the previous conditions are met, the code inside the else block is executed.
Case Statements
Another way to handle conditional statements in Ruby is by using case statements. These statements can be helpful when dealing with multiple possible values for a variable.
Here is an example:
In this case statement, we check the value of the variable day . Depending on its value, different code blocks are executed. In this example, since day is "Monday," the code inside the first when block is executed, printing "It's the beginning of the week."
Case statements also support ranges and multiple conditions for each when block. They are a flexible tool for handling complex conditional logic.
Conditional statements are a crucial aspect of programming in any language, and Ruby is no exception. They provide us with the ability to control the flow of our code based on specific conditions. In this tutorial, we explored the basics of conditional statements in Ruby, including if statements, elsif statements for multiple conditions, and case statements for handling various possible values.
With the knowledge gained from this tutorial, you are now equipped to use conditional statements effectively in your Ruby programs. Happy coding!
Remember to save this Markdown file and convert it to HTML using your preferred Markdown converter to properly format and present the content on your blog.

Hi, I'm Ada, your personal AI tutor. I can help you with any coding tutorial. Go ahead and ask me anything.
I have a question about this topic
Give more examples
Ruby Assignments in Conditional Expressions
For around two and a half years, I had been working in a Python 2.x environment at my old job . Since switching companies, Ruby and Rails has replaced Python and Flask. At first glance, the languages don’t seem super different, but there are definitely common Ruby idioms that I needed to learn.
While adding a new feature, I came across code that looked like this:
Intuitively, I guessed this meant “if some_func() returns something, do_something with it.” What took a second was understanding that you could do an assignment conditional expressions.
Coming from Python
In Python 2.x land (and any Python 3 before 3.8), assigning a variable in a conditional expression would return a syntax error:
Most likely, the assignment in a conditional expression is a typo where the programmer meant to do a comparison == instead.
This kind of typo is a big enough issue that there is a programming style called Yoda Conditionals , where the constant portion of a comparison is put on the left side of the operator. This would cause a syntax error if = were used instead of == , like this:
Back to Ruby
Now that I’ve come across this kind of assignment in Ruby for the first time, I searched whether assignments in conditional expressions were good style. Sure enough, the Ruby Style Guide has a section called “Safe Assignment in Condition” . The section and examples are short and straightforward. You should take a look if this Ruby idiom is new to you!
What is interesting about this idiom is that the assignment should be wrapped in parentheses. Take a look at the examples from the Ruby Style Guide:
Why is the first example bad? Well, the Style Guide also says that you shouldn’t put parentheses around conditional expressions . Parentheses around assignments in conditional expressions are specifically called out as an exception to this rule.
My guess is that by enforcing two different conventions, one for pure conditionals and one for assignments in conditionals, the onus is now on the programmer to decide what they want to do. This should prevent typos where an assignment = is used when a comparison == was intended, or vice versa.
The Python Equivalent
As called out above, assignments in conditional expressions are possible in Python 3.8 via PEP 572 . Instead of using an = for these kinds of assignments, the PEP introduced a new operator := . As parentheses are more common in Python expressions, introducing a new operator := (informally known as “the walrus operator”!!) was probably Python’s solution to the typo issue.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Ruby Programming Language
- Ruby For Beginners
- Ruby Programming Language (Introduction)
- Comparison of Java with other programming languages
- Similarities and Differences between Ruby and C language
- Similarities and Differences between Ruby and C++
- Environment Setup in Ruby
- How to install Ruby on Linux?
- How to install Ruby on Windows?
- Interesting facts about Ruby Programming Language
- Ruby | Keywords
- Ruby | Data Types
- Ruby Basic Syntax
- Hello World in Ruby
- Ruby | Types of Variables
- Global Variable in Ruby
- Comments in Ruby
- Ruby | Ranges
- Ruby Literals
- Ruby Directories
- Ruby | Operators
- Operator Precedence in Ruby
- Operator Overloading in Ruby
- Ruby | Pre-define Variables & Constants
- Ruby | unless Statement and unless Modifier
Control Statements
- Ruby | Decision Making (if, if-else, if-else-if, ternary) | Set - 1
- Ruby | Loops (for, while, do..while, until)
- Ruby | Case Statement
- Ruby | Control Flow Alteration
- Ruby Break and Next Statement
- Ruby redo and retry Statement
- BEGIN and END Blocks In Ruby
- File Handling in Ruby
- Ruby | Methods
- Method Visibility in Ruby
- Recursion in Ruby
- Ruby Hook Methods
- Ruby | Range Class Methods
- The Initialize Method in Ruby
- Ruby | Method overriding
- Ruby Date and Time
OOP Concepts
- Object-Oriented Programming in Ruby | Set 1
- Object Oriented Programming in Ruby | Set-2
- Ruby | Class & Object
- Private Classes in Ruby
- Freezing Objects | Ruby
- Ruby | Inheritance
- Polymorphism in Ruby
- Ruby | Constructors
- Ruby | Access Control
- Ruby | Encapsulation
- Ruby Mixins
- Instance Variables in Ruby
- Data Abstraction in Ruby
- Ruby Static Members
- Ruby | Exceptions
- Ruby | Exception handling
- Catch and Throw Exception In Ruby
- Raising Exceptions in Ruby
- Ruby | Exception Handling in Threads | Set - 1
- Ruby | Exception Class and its Methods
- Ruby | Regular Expressions
- Ruby Search and Replace
Ruby Classes
- Ruby | Float Class
- Ruby | Integer Class
- Ruby | Symbol Class
- Ruby | Struct Class
- Ruby | Dir Class and its methods
- Ruby | MatchData Class
Ruby Module
- Ruby | Module
- Ruby | Comparable Module
- Ruby | Math Module
- Include v/s Extend in Ruby
Collections
- Ruby | Arrays
- Ruby | String Basics
- Ruby | String Interpolation
- Ruby | Hashes Basics
- Ruby | Hash Class
- Ruby | Blocks
Ruby Threading
- Ruby | Introduction to Multi-threading
- Ruby | Thread Class-Public Class Methods
- Ruby | Thread Life Cycle & Its States
Miscellaneous
- Ruby | Types of Iterators
- Ruby getters and setters Method
Ruby | Decision Making (if, if-else, if-else-if, ternary) | Set – 1
Decision Making in programming is similar to decision making in real life. In programming too, a certain block of code needs to be executed when some condition is fulfilled. A programming language uses control statements to control the flow of execution of the program based on certain conditions. These are used to cause the flow of execution to advance and branch based on changes to the state of a program. Similarly, in Ruby, the if-else statement is used to test the specified condition.
Decision-Making Statements in Ruby:
if statement
- if-else statement
- if – elsif ladder
- Ternary statement
If statement in Ruby is used to decide whether a certain statement or block of statements will be executed or not i.e if a certain condition is true then a block of statement is executed otherwise not.
Flowchart:
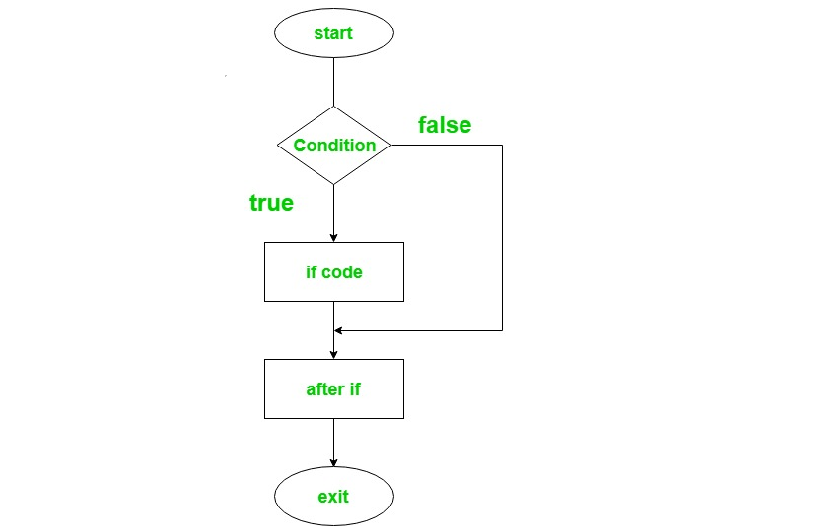
Output:
if – else Statement
In this ‘if’ statement used to execute block of code when the condition is true and ‘else’ statement is used to execute a block of code when the condition is false.
Syntax:
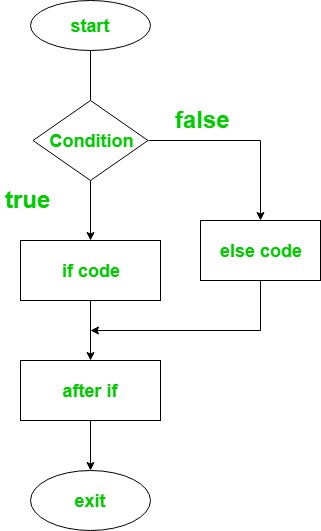
Example:
If – elsif – else ladder Statement
Here, a user can decide among multiple options. ‘if’ statements are executed from the top down. As soon as one of the conditions controlling the ‘if’ is true, the statement associated with that ‘if’ is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed.
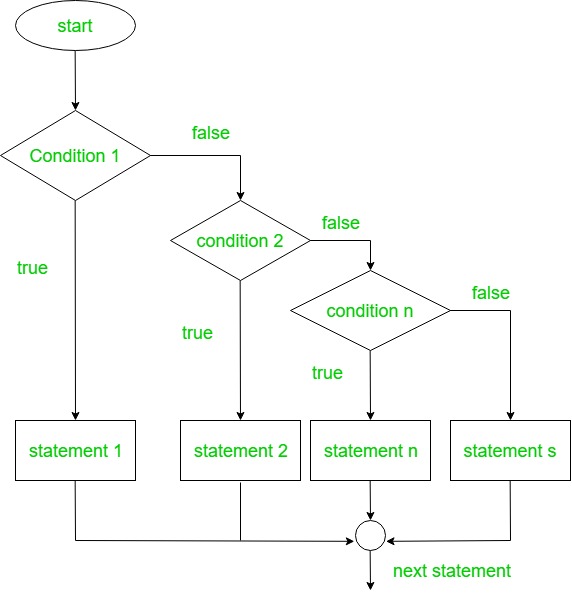
Ternary Statement
In Ruby ternary statement is also termed as the shortened if statement . It will first evaluate the expression for true or false value and then execute one of the statements. If the expression is true, then the true statement is executed else false statement will get executed.
Syntax:
Please Login to comment...
Similar reads.
- Ruby-Basics
- Ruby-Decision-Making
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
+123-456-7890
[email protected]
Mon - Fri: 7AM - 7PM
Conference on optimizing Ruby code execution
The Beginners Guide to Ruby If & Else Statements
Have you ever wondered how computers make decisions? How do they know what to do in different situations? Well, in the world of programming, this is done through conditional statements. And in the world of Ruby, this is done through if and else statements. In this article, we will dive deep into the world of Ruby if and else statements, learn about different types of conditions, and explore some special symbols that can be used in strings. So let’s get started!
How Do You Make Decisions in Ruby?
In simple terms, making decisions in Ruby means using conditional statements to perform certain actions based on specific conditions. These conditions can be anything from checking the value of a variable to comparing two values. Let’s take a look at some examples:
- If the room is too cold, turn on the heater;
- If we don’t have enough stock of a product, then send an order to buy more;
- If a customer has been with us for more than 3 years, then send them a thank you gift.
These are just a few examples of how we can use conditional statements to make decisions in Ruby. Now, let’s see how we can write these decisions in code using if statements.
Types Of Conditions
In Ruby, there are various types of conditions that we can use in our if statements. These conditions are represented by different symbols, each with its own meaning. Here’s a table that summarizes these symbols and their meanings:
One important thing to note here is that we use two equal signs (==) to check for equality, not just one. This is because a single equal sign (=) is used for assignment in Ruby. So if we want to check if two values are equal, we need to use two equal signs.
Ruby Unless Statement
Apart from the if statement, Ruby also has an unless statement that can be used to make decisions. The unless statement is the opposite of the if statement, meaning it will only execute the code inside its condition if the condition is false. Let’s take a look at an example:
In this example, the code inside the unless statement will only be executed if the value of stock is not greater than 0, which means the stock is equal to or less than 0. So in this case, the message “Sorry, we are out of stock!” will be printed.
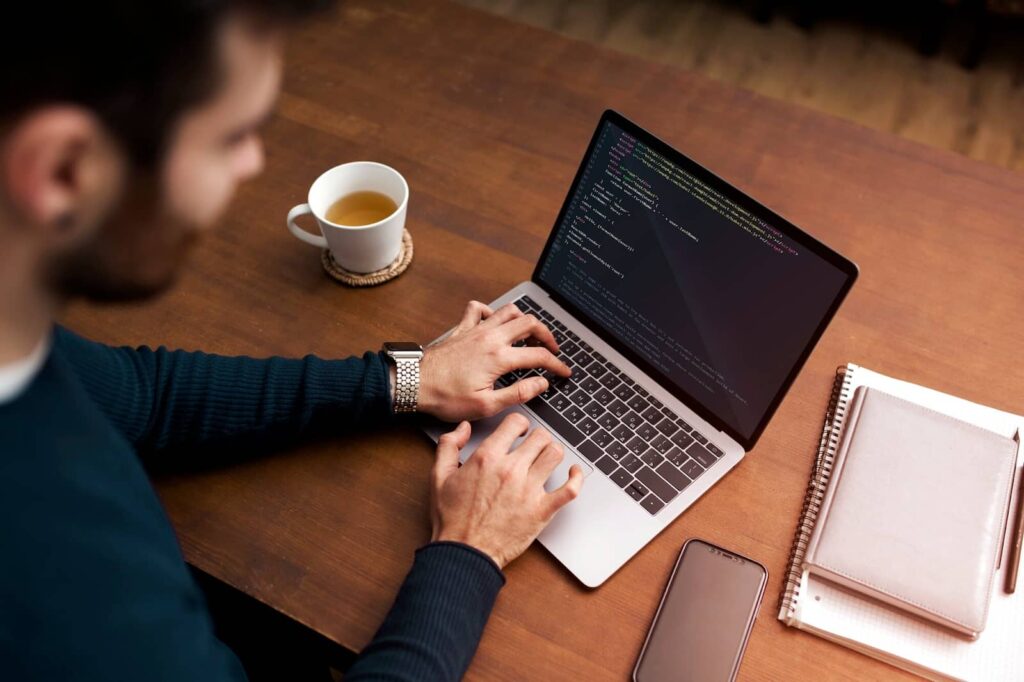
The If Else Statement
Now, let’s move on to the if else statement. This statement allows us to specify what should happen if the condition is true and what should happen if the condition is false. Here’s an example:
In this example, if the value of age is greater than or equal to 18, the first message will be printed. Otherwise, the second message will be printed.
How to Use Multiple Conditions
Sometimes, we may need to check multiple conditions before executing a certain piece of code. In such cases, we can use logical operators like && (and), || (or), and ! (not). These operators allow us to combine multiple conditions and create more complex conditions. Let’s take a look at an example:
In this example, the code inside the if statement will only be executed if both conditions are true, meaning the value of age is between 18 and 30.
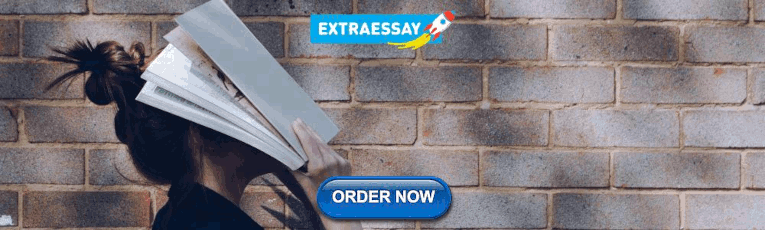
Things to Watch Out For
When using conditional statements in Ruby, there are a few things that we need to watch out for to avoid unexpected results. One common mistake is using a single equal sign (=) instead of two (==) when checking for equality. Another thing to keep in mind is the order of conditions. If we have multiple conditions, the order in which they are written can affect the outcome.
Special Symbols in Strings
Apart from the symbols we use in conditions, there are also some special symbols that we can use in strings to make our code more readable. These symbols are called escape sequences and they start with a backslash (). Here are some commonly used escape sequences:
For example, if we want to print a string that contains a double quote, we can use the escape sequence \” to tell Ruby that the double quote is part of the string and not the end of it. Let’s see an example:
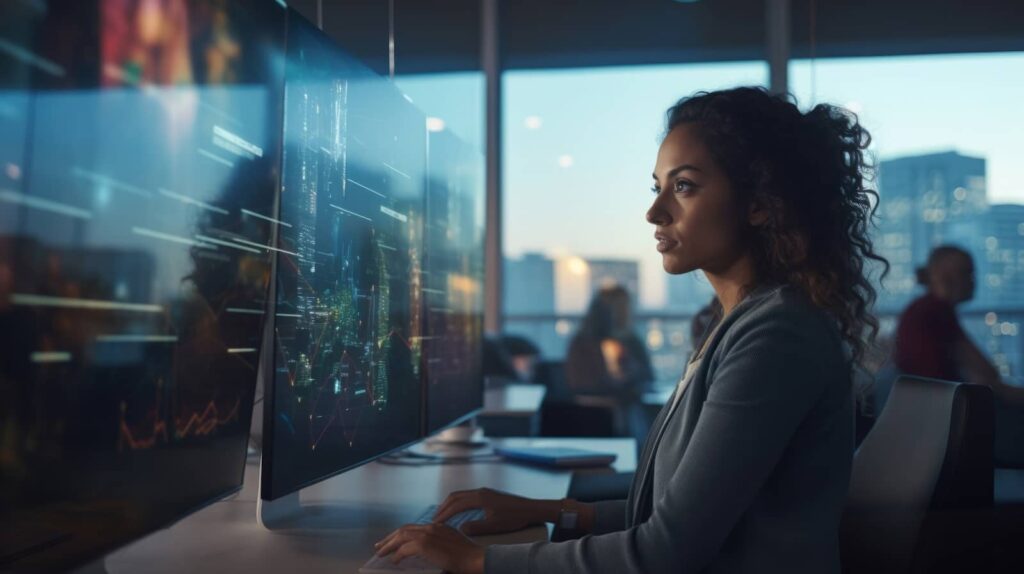
If Construct in One Line
In Ruby, we can also write if statements in one line using the if construct. This is useful when we have a simple condition and don’t want to write multiple lines of code. Here’s an example:
This will print the message only if the value of stock is less than 1.
Is There an Alternative?
While if and else statements are commonly used in Ruby, there is an alternative that can be used in some situations. This alternative is called the ternary operator and it allows us to write if statements in a more concise way. Let’s take a look at an example:
This is equivalent to writing:
As you can see, the ternary operator can save us some lines of code, but it’s important to use it wisely and only when it makes our code more readable.
In this article, we learned about conditional statements in Ruby, specifically if and else statements. We explored different types of conditions and how to use them in our code. We also saw some special symbols that can be used in strings and how to write if statements in one line using the if construct. Lastly, we briefly mentioned the ternary operator as an alternative to if and else statements.
Recommended Articles
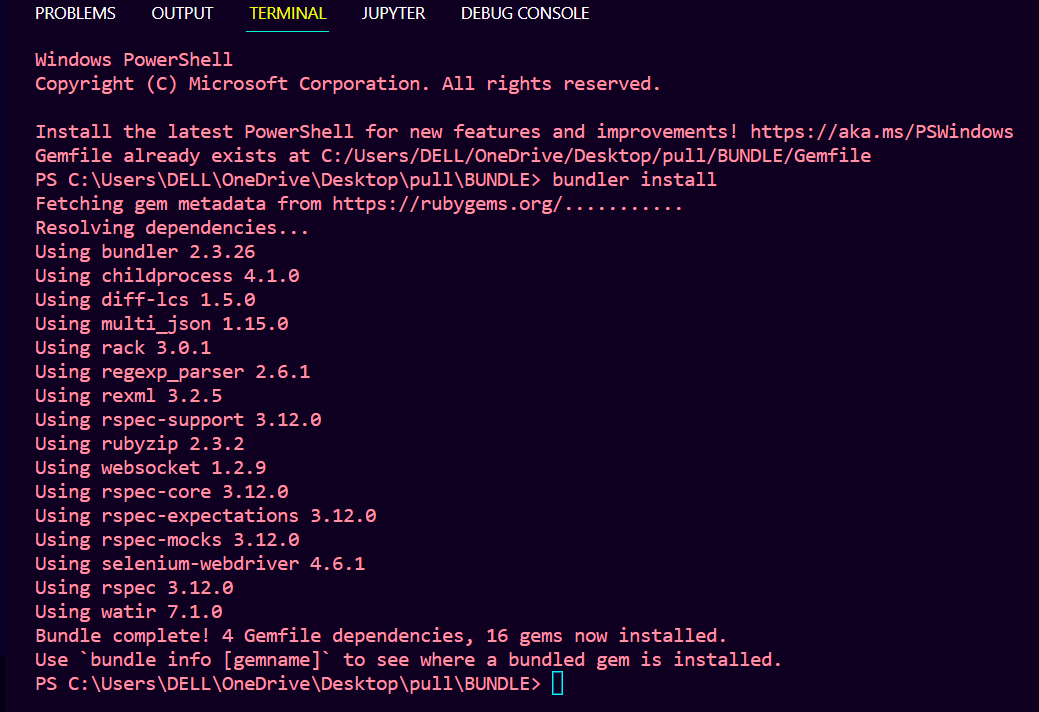
Ruby Gems & Bundler: Code Mastery
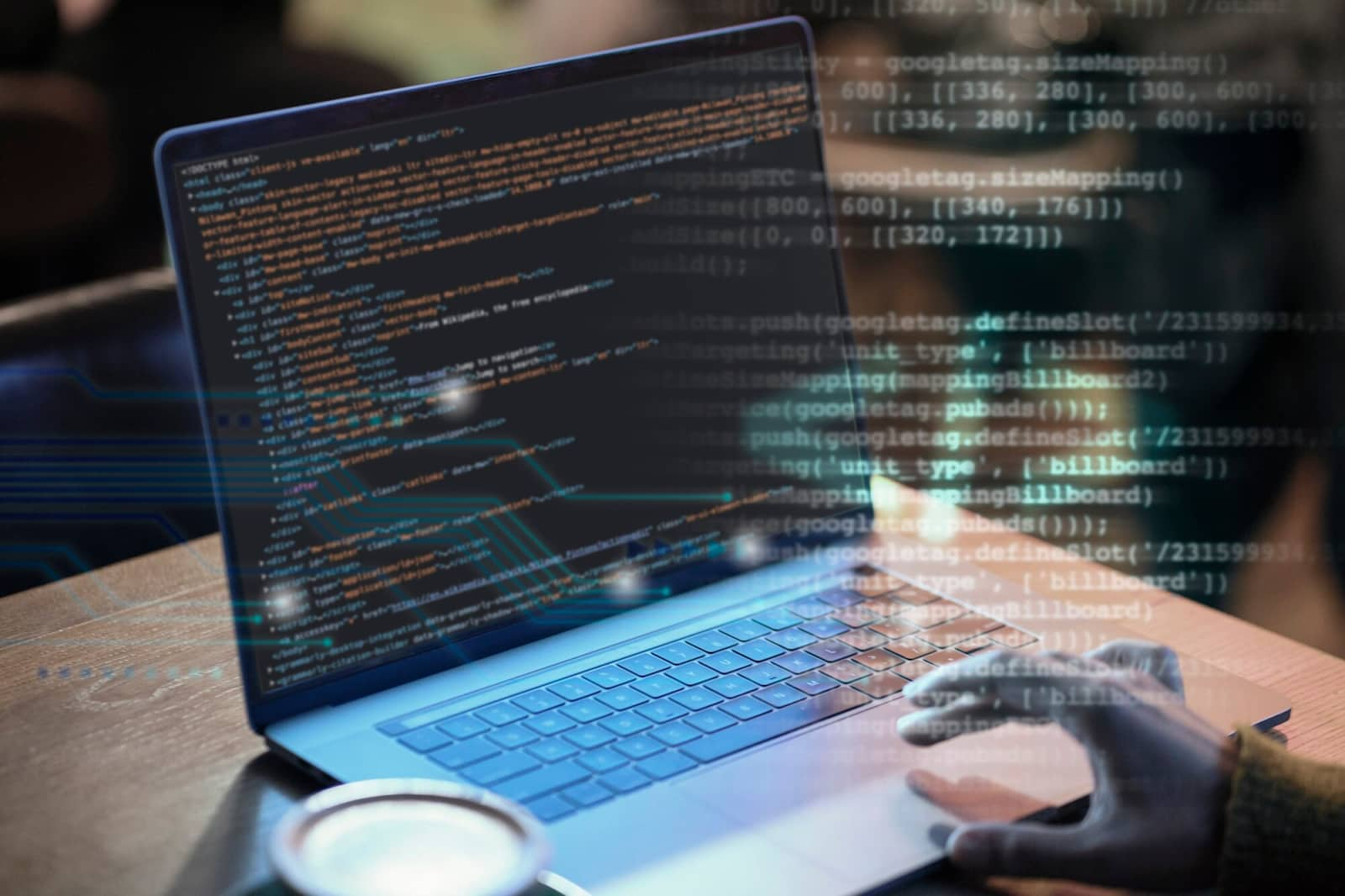
Using Redis in Ruby: Installation to Advanced Features
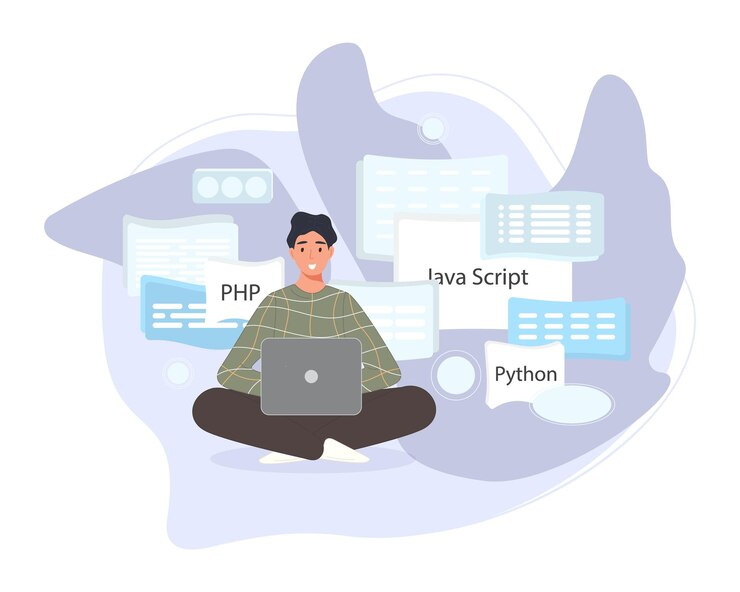
Ruby Array Select: Streamline Data Processing in Ruby
Leave a comment cancel reply.
You must be logged in to post a comment.

- Ruby Basics
- Ruby - Home
- Ruby - Overview
- Ruby - Environment Setup
- Ruby - Syntax
- Ruby - Classes and Objects
- Ruby - Variables
- Ruby - Operators
- Ruby - Comments
- Ruby - IF...ELSE
- Ruby - Loops
- Ruby - Methods
- Ruby - Blocks
- Ruby - Modules
- Ruby - Strings
- Ruby - Arrays
- Ruby - Hashes
- Ruby - Date & Time
- Ruby - Ranges
- Ruby - Iterators
- Ruby - File I/O
- Ruby - Exceptions
- Ruby Advanced
- Ruby - Object Oriented
- Ruby - Regular Expressions
- Ruby - Database Access
- Ruby - Web Applications
- Ruby - Sending Email
- Ruby - Socket Programming
- Ruby - Ruby/XML, XSLT
- Ruby - Web Services
- Ruby - Tk Guide
- Ruby - Ruby/LDAP Tutorial
- Ruby - Multithreading
- Ruby - Built-in Functions
- Ruby - Predefined Variables
- Ruby - Predefined Constants
- Ruby - Associated Tools
- Ruby Useful Resources
- Ruby - Quick Guide
- Ruby - Useful Resources
- Ruby - Discussion
- Ruby - Ruby on Rails Tutorial
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Ruby - if...else, case, unless
Ruby offers conditional structures that are pretty common to modern languages. Here, we will explain all the conditional statements and modifiers available in Ruby.
Ruby if...else Statement
if expressions are used for conditional execution. The values false and nil are false, and everything else are true. Notice Ruby uses elsif, not else if nor elif.
Executes code if the conditional is true. If the conditional is not true, code specified in the else clause is executed.
An if expression's conditional is separated from code by the reserved word then , a newline, or a semicolon.
Ruby if modifier
Executes code if the conditional is true.
This will produce the following result −
Ruby unless Statement
Executes code if conditional is false. If the conditional is true, code specified in the else clause is executed.
Ruby unless modifier
Executes code if conditional is false.
Ruby case Statement
Compares the expression specified by case and that specified by when using the === operator and executes the code of the when clause that matches.
The expression specified by the when clause is evaluated as the left operand. If no when clauses match, case executes the code of the else clause.
A when statement's expression is separated from code by the reserved word then, a newline, or a semicolon. Thus −
is basically similar to the following −
To Continue Learning Please Login
Ruby 2.7 Reference SAVE UKRAINE
In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v :
Assignment creates a local variable if the variable was not previously referenced.
Abbreviated Assignment
You can mix several of the operators and assignment. To add 1 to an object you can write:
This is equivalent to:
You can use the following operators this way: + , - , * , / , % , ** , & , | , ^ , << , >>
There are also ||= and &&= . The former makes an assignment if the value was nil or false while the latter makes an assignment if the value was not nil or false .
Here is an example:
Note that these two operators behave more like a || a = 0 than a = a || 0 .
Multiple Assignment
You can assign multiple values on the right-hand side to multiple variables:
In the following sections any place “variable” is used an assignment method, instance, class or global will also work:
You can use multiple assignment to swap two values in-place:
If you have more values on the right hand side of the assignment than variables on the left hand side, the extra values are ignored:
You can use * to gather extra values on the right-hand side of the assignment.
The * can appear anywhere on the left-hand side:
But you may only use one * in an assignment.
Array Decomposition
Like Array decomposition in method arguments you can decompose an Array during assignment using parenthesis:
You can decompose an Array as part of a larger multiple assignment:
Since each decomposition is considered its own multiple assignment you can use * to gather arguments in the decomposition:
Sandy Springs school issues statement after Hitler assignment controversy
ATLANTA, Ga. (Atlanta News First) - A metro Atlanta school is responding to backlash to a controversial assignment.
Parents are fuming and questioning the Mount Vernon School in Sandy Springs after their kids were asked about Hitler’s leadership.
The assignment asked students to rate the attributes of the former Nazi dictator. The school now acknowledges this caused distress and the assignment has now been removed from the curriculum.
Head of School Kristy Lundstrom sent the following statement to Mount Vernon parents:
Copyright 2024 WANF. All rights reserved.

3 teens killed, 2 others injured in Alpharetta crash, officials say
![ruby assignment in if statement [insert caption here]](https://gray-wgcl-prod.cdn.arcpublishing.com/resizer/v2/5PGALTGVHVHTVKNVQJX3KXIGMM.jpg?auth=1225ae9879961b13e33ced4472b86098e5a47effe99b965effdb1e85648deef0&width=800&height=450&smart=true)
Jimmy Carter doing OK, but nearing the end, grandson says
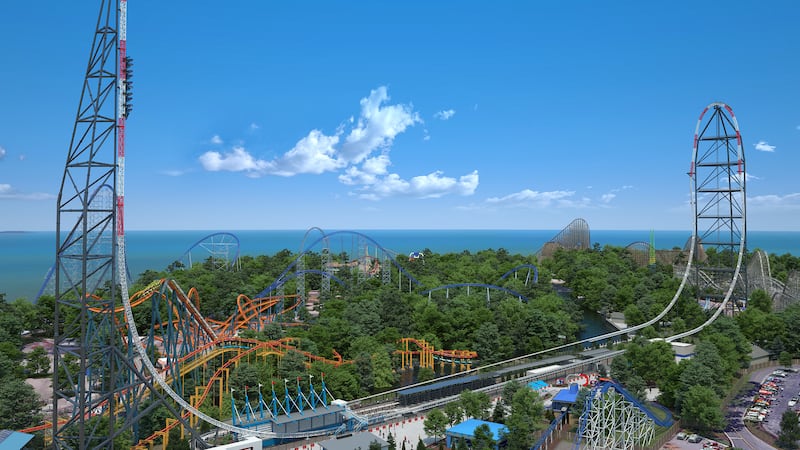
Cedar Point says new Top Thrill 2 coaster is closed indefinitely just days after opening

3 shot during filming of music video in northwest Atlanta, police say

‘I miss her so much’: Shooting victim’s sister wants city to shut down ‘not safe’ Buckhead nightclub
Latest news.
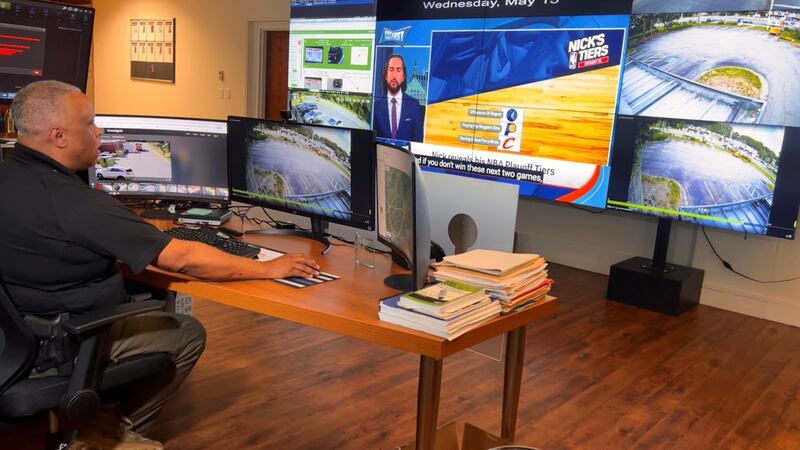
The future of fighting crime: AI helps City of South Fulton Police track and solve crimes

One of the 3 teens killed in Alpharetta crash identified as high school senior, officials say

Atlanta News First gives out ‘Books to Kids’ at College Park Elementary

Metro Atlanta woman raising money for Brazil flood victims, family members
Man charged with assaulting 2 females along Highway 39 in Angeles National Forest
M ay 15—A man was charged Wednesday, May 15, with sexually assaulting two females along Highway 39 in the Angeles National Forest, officials said.
Eduardo Sarabia, 40, allegedly assaulted one victim Sunday and the other on Monday, according to a statement from the Los Angeles County Sheriff's Department. The alleged crimes happened between 9:30 p.m. and 10 p.m. Sheriff's officials said both victims were females but provided no other description.
The Los Angeles County District Attorney's Office filed one count of forcible rape and one count of oral copulation by means of force or fear of bodily injury on the victim, court records show. Sarabia's arraignment Wednesday at Pomona Superior Court was continued to June 27.
Deputies checking a van parked on a turnout on Highway 39 arrested Sarabia on Monday night, Sheriff's Lt William Fillpot said. But the Sheriff's Department did not say what led to the arrest or confirm a media report that a victim was rescued from the van on Monday night. Neither did they say how investigators found out about the earlier victim and how the suspect met them.
A sergeant from the Sheriff's Special Victims Bureau couldn't be reached for comment on Wednesday.
"Based on the nature of the allegations, detectives believe there may be additional unidentified victims," the statement said.
Investigators asked for the public's help in finding more possible victims. The department released a mug shot of Sarabia and a picture of the van he drives.
Sarabia is being held without bail in a county jail.
Detectives asked that anyone with information call the sheriff's Special Victims Bureau toll free tip line at 877-710-5273 or email them at [email protected] . Anonymous tipsters can call Crime Stoppers at 800-222-8477 or go to lacrimestoppers.org
(c)2024 San Gabriel Valley Tribune, West Covina, Calif. Distributed by Tribune Content Agency, LLC.
Latter-day Saint missionary serving in Utah arrested on suspicion of rape
The 19-year-old is being held without bail..
(Saratoga Springs Police Department) Police in Saratoga Springs have arrested a 19-year-old Latter-day Saint missionary, who is suspected of rape.
A 19-year-old missionary with The Church of Jesus Christ of Latter-day Saints was arrested Saturday in Saratoga Springs on suspicion of rape.
The man is from California and was serving a Latter-day Saint mission in Utah. He is accused of rape, forcible sodomy and sexual battery, according to Utah County jail records.
He was arrested after police were called to a Saratoga Springs home Saturday, where a witness told investigators the man sexually assaulted the witness’s sister in a garage. The Salt Lake Tribune generally does not name defendants unless they have been formally charged with a crime.
The 19-year-old told police that he and the alleged victim, whose age was not released, were “making out” in the garage of her home and “eventually had sex,” according to a probable cause statement explaining his arrest. He declined to answer further questions, the document states.
The man was living across the street from the alleged victim’s home. She told police she was in the garage with the man when he grabbed her and began kissing her, to which she said she “originally consented.”
But when she told him to stop, he did not, proceeding to assault her, she told police. As he was allegedly assaulting her, friends came into the garage and told him to stop, a witness said.
According to a statement from the church, the 19-year-old was “immediately removed from his volunteer missionary service as soon as the church learned of these very serious and troubling allegations,” FOX 13 reported .
The church “is cooperating fully with law enforcement in this investigation,” the statement provided to FOX 13 added.
“Missionaries are expected to abide by the highest standards, and those who do not will be released and sent home,” the statement continued. “And in situations involving criminal allegations, also face loss of church membership.”
As of Tuesday afternoon, the man remained held in Utah County jail without the option of bail.

Donate to the newsroom now. The Salt Lake Tribune, Inc. is a 501(c)(3) public charity and contributions are tax deductible
RELATED STORIES
19-year-old lds missionary dies from ‘sudden medical emergency’, latest from mormon land: updated missionary rules; a pop quiz — how many kids did brigham have, ‘we are devastated’: 19-year-old collapses, dies after reading aloud lds missionary assignment, lds missionary is killed in the south pacific, becomes the fifth to die this year, utah goalkeeper has one of the nhl’s best stories and the award to prove it, why mitt romney says president biden should have pardoned donald trump, utah’s 2024 debate dreams dashed as biden and trump opt for independent presidential showdowns, pucker pass lives up to its name for one man’s misadventure, utah county clerk initially rejects alpine school district split proposal; leaders to try again, proponen incremento de un 20% en los impuestos a la propiedad en draper, featured local savings.
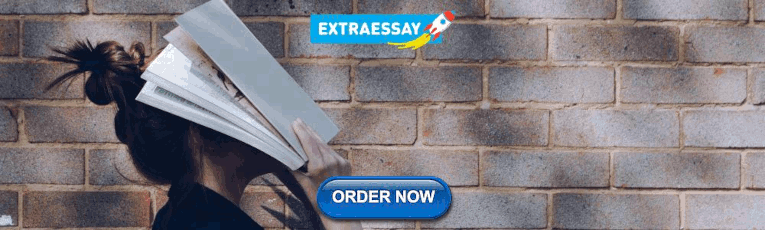
COMMENTS
Yes, it will. It's an instance variable. In ruby, if you prefix your variable with @, it makes the variable an instance variable that will be available outside the block. In addition to that, Ruby does not have block scope (block in the traditional sense, if/then/else in this case).
If something is true (the condition) then you can do something. In Ruby, you do this using if statements: stock = 10. if stock < 1. puts "Sorry we are out of stock!" end. Notice the syntax. It's important to get it right. The stock < 1 part is what we call a "condition".
Since Ruby parses the bare a left of the if first and has not yet seen an assignment to a it assumes you wish to call a method. Ruby then sees the assignment to a and will assume you are referencing a local method. The confusion comes from the out-of-order execution of the expression. First the local variable is assigned-to then you attempt to ...
The if. The if statement is how we create a branch in our program flow. The if statement includes a true-or-false expression: if 4 == 2 + 2. puts "The laws of arithmetic work today" end. If that expression evaluates to true, then the Ruby interpreter will execute the puts statement that follows the if statement.
Conditional assignment. Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read. There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
Ruby has a notion of true and false. This is best illustrated through some example. Start irb and type the following: The if statements evaluates whether the expression (e.g. 'city == "Toronto") is true or false and acts accordingly. Warning: Notice the difference between '=' and '=='. '=' is an assignment operator. '==' is a comparison ...
If - Elseif - Else Statements. If the if statement is not true, the block of code in the elseif statement will be executed if the condition is true. There may me multiple elseif statements. Finally, if none of the conditions are true, the block of code in the else statement will be executed.
Control Expressions. Ruby has a variety of ways to control execution. All the expressions described here return a value. For the tests in these control expressions, nil and false are false-values and true and any other object are true-values. In this document "true" will mean "true-value" and "false" will mean "false-value".
Ruby: If Statements. One of the most powerful features present in most programming and scripting languages is the ability to change the flow of the program as certain conditions change. The simplest form of flow control and logic in Ruby is called an "if statement" (or technically speaking in Ruby, since everything is an expression, an "if ...
If Statements. The most basic form of a conditional statement is the if statement. It allows us to execute a block of code only if a certain condition is met. Here is a simple example: age = 25. if age > 18. puts "You are an adult!" end. In this example, we first declare a variable age with a value of 25.
SyntaxError: invalid syntax. Most likely, the assignment in a conditional expression is a typo where the programmer meant to do a comparison == instead. This kind of typo is a big enough issue that there is a programming style called Yoda Conditionals , where the constant portion of a comparison is put on the left side of the operator.
Assignment. In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. An assignment expression result is always the assigned value, including assignment methods.
Ternary Statement. In Ruby ternary statement is also termed as the shortened if statement. It will first evaluate the expression for true or false value and then execute one of the statements. If the expression is true, then the true statement is executed else false statement will get executed. Syntax:
Well, in the world of programming, this is done through conditional statements. And in the world of Ruby, this is done through if and else statements. In this article, we will dive deep into the world of Ruby if and else statements, learn about different types of conditions, and explore some special symbols that can be used in strings.
Ruby unless Statement Syntax unless conditional [then] code [else code ] end Executes code if conditional is false. If the conditional is true, code specified in the else clause is executed. Example. Live Demo #!/usr/bin/ruby x = 1 unless x>=2 puts "x is less than 2" else puts "x is greater than 2" end This will produce the following result − ...
In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. Abbreviated Assignment. You can mix several of the operators and assignment. To add 1 to an object you can write:
Ruby Case & Ranges. The case statement is more flexible than it might appear at first sight. Let's see an example where we want to print some message depending on what range a value falls in. case capacity. when 0. "You ran out of gas." when 1..20. "The tank is almost empty. Quickly, find a gas station!"
That's part of the syntax! It's how Ruby knows that you're writing a ternary operator. Next: We have whatever code you want to run if the condition turns out to be true, the first possible outcome. Then a colon (: ), another syntax element. Lastly, we have the code you want to run if the condition is false, the second possible outcome.
Parents are fuming and questioning the Mount Vernon School in Sandy Springs after their kids were asked about Hitler's leadership. The assignment asked students to rate the attributes of the former Nazi dictator. The school now acknowledges this caused distress and the assignment has now been removed from the curriculum.
This is equivalent to: value = key rescue nil if value .. end or. value = begin key rescue nil end if value .. end Remember nil and false are the only two objects that are falsey in ruby and since value here could be nil, that if statement could return false.
Story by Ruby Gonzales, San Gabriel Valley Tribune, West Covina, Calif. • 45m M ay 15—A man was charged Wednesday, May 15, with sexually assaulting two females along Highway 39 in the Angeles ...
According to a statement from the church, ... 'We are devastated': 19-year-old collapses, dies after reading aloud LDS missionary assignment. LDS missionary is killed in the South Pacific ...
m_x answer is perfect but you may be interested to know other ways to do it, which may look better in other circumstances: if params [:property] == nil then @item.property = true else @item.property = false end. or. @item.property = if params [:property] == nil then true else false end. answered Dec 8, 2012 at 12:30.
I am wondering if there is a way in Ruby to write a one-line if-then-else statement using words as operators, in a manner similar to python's a if b else c I am aware of the classic ternary conditional, but I have the feeling Ruby is more of a "wordsey" language than a "symboley" language, so I am trying to better encapsulate the spirit of the ...