Learn Python practically and Get Certified .
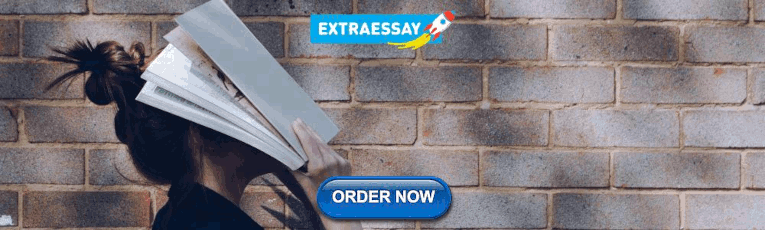
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
Python Data Types
- Python Type Conversion
- Python I/O and Import
- Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
- Python List
- Python Tuple
- Python String
Python Dictionary
Python files.
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Python Dictionary clear()
Python Dictionary items()
- Python Dictionary keys()
Python Dictionary fromkeys()
- Python Nested Dictionary
- Python Dictionary update()
A Python dictionary is a collection of items, similar to lists and tuples. However, unlike lists and tuples, each item in a dictionary is a key-value pair (consisting of a key and a value).
- Create a Dictionary
We create a dictionary by placing key: value pairs inside curly brackets {} , separated by commas. For example,
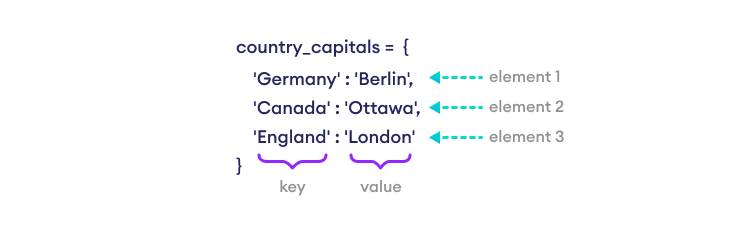
- Dictionary keys must be immutable, such as tuples, strings, integers, etc. We cannot use mutable (changeable) objects such as lists as keys.
- We can also create a dictionary using a Python built-in function dict() . To learn more, visit Python dict() .
Valid and Invalid Dictionaries
Immutable objects can't be changed once created. Some immutable objects in Python are integer, tuple and string.
In this example, we have used integers, tuples, and strings as keys for the dictionaries. When we used a list as a key, an error message occurred due to the list's mutable nature.
Note: Dictionary values can be of any data type, including mutable types like lists.
The keys of a dictionary must be unique. If there are duplicate keys, the later value of the key overwrites the previous value.
Here, the key Harry Potter is first assigned to Gryffindor . However, there is a second entry where Harry Potter is assigned to Slytherin .
As duplicate keys are not allowed in a dictionary, the last entry Slytherin overwrites the previous value Gryffindor .
- Access Dictionary Items
We can access the value of a dictionary item by placing the key inside square brackets.
Note: We can also use the get() method to access dictionary items.
- Add Items to a Dictionary
We can add an item to a dictionary by assigning a value to a new key. For example,
- Remove Dictionary Items
We can use the del statement to remove an element from a dictionary. For example,
Note : We can also use the pop() method to remove an item from a dictionary.
If we need to remove all items from a dictionary at once, we can use the clear() method.
- Change Dictionary Items
Python dictionaries are mutable (changeable). We can change the value of a dictionary element by referring to its key. For example,
Note : We can also use the update() method to add or change dictionary items.
- Iterate Through a Dictionary
A dictionary is an ordered collection of items (starting from Python 3.7), therefore it maintains the order of its items.
We can iterate through dictionary keys one by one using a for loop .
- Find Dictionary Length
We can find the length of a dictionary by using the len() function.
- Python Dictionary Methods
Here are some of the commonly used dictionary methods .
- Dictionary Membership Test
We can check whether a key exists in a dictionary by using the in and not in operators.
Note: The in operator checks whether a key exists; it doesn't check whether a value exists or not.
Table of Contents
Video: python dictionaries to store key/value pairs.
Sorry about that.
Related Tutorials
Python Library
Python Tutorial
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Dictionaries in Python
- How to create a Dictionary in Python
- Get length of dictionary in Python
- Python - Value length dictionary
- Python - Dictionary values String Length Summation
- Python - Dictionary value lists lengths product
- Access Dictionary Values | Python Tutorial
- Python - Dictionary items in value range
- Python | Ways to change keys in dictionary
- Python Program to Swap dictionary item's position
- Python | Merging two Dictionaries
- How to Compare Two Dictionaries in Python?
- Python Dictionary Comprehension
How to add values to dictionary in Python
- Python | Add new keys to a dictionary
- Python - Add item after given Key in dictionary
- Python | Ways to remove a key from dictionary
- Python | Removing dictionary from list of dictionaries
- Python | Remove item from dictionary when key is unknown
- Python - Test if all Values are Same in Dictionary
- Python | Test if element is dictionary value
- Why is iterating over a dictionary slow in Python?
- Iterate over a dictionary in Python
- Python - How to Iterate over nested dictionary ?
- Python | Delete items from dictionary while iterating
In this article, we will learn what are the different ways to add values in a dictionary in Python .
Assign values using unique keys
After defining a dictionary we can index through it using a key and assign a value to it to make a key-value pair.
{0: ‘Carrot’, 1: ‘Raddish’, 2: ‘Brinjal’, 3: ‘Potato’}
But what if a key already exists in it?
{0: ‘Tomato’, 1: ‘Raddish’, 2: ‘Brinjal’, 3: ‘Potato’}
We can observe that the value corresponding to key 0 has been updated to ‘Tomato’ from ‘Carrot’.
Merging two dictionaries using update()
We can merge two dictionaries by using the update() function.
{0: ‘Carrot’, 1: ‘Raddish’, 2: ‘Brinjal’, 3: ‘Potato’, 4: ‘Tomato’, 5: ‘Spinach’}
Add values to dictionary Using two lists of the same length
This method is used if we have two lists and we want to convert them into a dictionary with one being key and the other one as corresponding values.
{10: ‘Ramesh’, 20: ‘Mahesh’, 30: ‘Kamlesh’, 40: ‘Suresh’, 50: ‘Dinesh’}
Converting a list to the dictionary
This method is used to convert a python list into a python dictionary with indexes as keys.
There are other methods as well to add values to a dictionary but the above-shown methods are some of the most commonly used ones and highly efficient as well.
Add values to dictionary Using the merge( | ) operator
By using the merge operator we can combine two dictionaries.
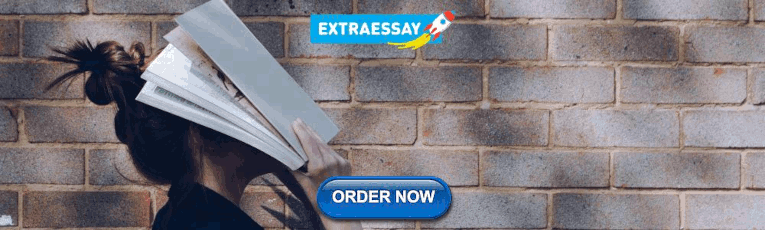
Add values to dictionary Using the in-place merge( |= ) operator.
By using the in place merge operator we can combine two dictionaries. In in place operator, all elements of one dictionary are added in the other but while using merge original state of the dictionaries remains the same and a new one is created.
Note: Methods 4 and 5 work in only Python 3.9+ versions.
Please Login to comment...
- 10 Best Tools to Convert DOC to DOCX
- How To Summarize PDF Documents Using Google Bard for Free
- Best free Android apps for Meditation and Mindfulness
- TikTok Is Paying Creators To Up Its Search Game
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Hiring? Flexiple helps you build your dream team of developers and designers .
How To Add Values To A Dictionary In Python

Harsh Pandey
Last updated on 23 Mar 2024
Python dictionaries are versatile and essential for managing key-value pairs in programming. Adding values to a dictionary is a fundamental skill in Python.
Assign Values Using Unique Keys
Assigning values to a dictionary in Python involves using unique keys. Each key in a dictionary is distinct and serves as an identifier for its corresponding value. When you add a value to a dictionary, you must specify a key that does not already exist in the dictionary. If the key is new, Python adds the key-value pair to the dictionary. If the key already exists, Python updates the existing key with the new value.
Here's how to add values using unique keys.
Direct Assignment
Assign a value to a dictionary by specifying a new key and its corresponding value.
Using the update() Method
This method allows adding multiple key-value pairs at once. It can also be used for adding a single key-value pair.
In summary, adding values to a dictionary in Python is straightforward and can be achieved through direct assignment or using the update() method. Unique keys are essential for correctly storing and retrieving values in a dictionary.
Merging Two Dictionaries Using update()
Merging two dictionaries in Python can be efficiently accomplished using the update() method. This method adds key-value pairs from one dictionary into another, effectively merging them. If there are overlapping keys, the values in the second dictionary will overwrite those in the first.
To use update(), you call it on the dictionary you want to update and pass the dictionary you want to merge into it as an argument.
After this operation, dict1 will contain the merged key-value pairs.
In this example, the value of the key 'b' in dict1 is updated to 3, the value from dict2, while the other key-value pairs are added to dict1. The update() method provides a straightforward way to combine dictionaries, enhancing the flexibility of dictionary management in Python.
Add Values To Dictionary Using Two Lists Of The Same Length
To add values to a dictionary in Python using two lists of the same length, you can utilize the zip() function combined with a dictionary comprehension. This method pairs elements from both lists and creates dictionary entries efficiently.
Firstly, create two lists: one for keys and another for values. Ensure both lists have the same length to maintain a one-to-one correspondence between keys and values.
Next, use the zip() function to pair elements from both lists. Then, apply a dictionary comprehension to construct the dictionary from these pairs.
This code creates a new dictionary named my_dict with keys and values paired from keys_list and values_list, respectively.
The output of this operation will be
In this dictionary, 'key1', 'key2', and 'key3' are mapped to 1, 2, and 3, respectively. This approach is straightforward and efficient for adding values to a dictionary from two parallel lists in Python.
Converting A List To The Dictionary
Converting a list to a dictionary is a common task in Python programming, especially when you need to add values to a dictionary. There are several methods to achieve this, each suited for different scenarios.
Using dict() With Zip
If you have two separate lists, one for keys and one for values, you can use the zip function along with dict() to combine them into a dictionary.
Using List Comprehension
For more complex scenarios or when you need to apply transformations, list comprehension offers a flexible approach.
Using fromkeys()
When you need to initialize a dictionary with the same value for each key, fromkeys() is an efficient method.
These methods provide a clear and concise way to convert a list to a dictionary in Python. Choosing the right one depends on your specific data and requirements. By using these techniques, you can efficiently add values to a dictionary in your Python projects.
Add Values To Dictionary Using The merge( | ) Operator
Adding values to a dictionary in Python can be efficiently done using the merge (|) operator. This operator allows you to combine two dictionaries into a new one, merging their key-value pairs. It is particularly useful when you want to update a dictionary with another dictionary's elements without modifying the original dictionaries.
To use the merge operator, simply place the | symbol between the two dictionaries you wish to combine. The resulting dictionary will contain all key-value pairs from both dictionaries. If there are overlapping keys, the values from the right-hand dictionary will be used in the new dictionary.
In this example, dict1 and dict2 are merged into merged_dict. The key 'b' appears in both dictionaries, but in merged_dict, it takes the value from dict2, which is 3.
Remember, the merge operator creates a new dictionary and does not modify the original dictionaries. This feature makes it a convenient and safe way to add values to dictionaries in Python.
Add Values To Dictionary Using The in-place merge( |= ) Operator
To add values to a dictionary in Python, one effective method is using the in-place merge (|=) operator. This operator was introduced in Python 3.9 and provides a convenient way to merge two dictionaries. The left-hand side dictionary gets updated with the key-value pairs from the right-hand side dictionary.
In this example, my_dict initially contains two key-value pairs. By using the |= operator with new_values, we directly add the key-value pairs from new_values to my_dict. The result is an updated my_dict that now includes the merged contents of both dictionaries.
This method is efficient and concise, making it ideal for situations where you need to combine dictionaries or add multiple key-value pairs to an existing dictionary in Python.
Work with top startups & companies. Get paid on time.
Try a top quality developer for 7 days. pay only if satisfied..
// Find jobs by category
You've got the vision, we help you create the best squad. Pick from our highly skilled lineup of the best independent engineers in the world.
- Ruby on Rails
- Elasticsearch
- Google Cloud
- React Native
- Contact Details
- 2093, Philadelphia Pike, DE 19703, Claymont
- [email protected]
- Explore jobs
- We are Hiring!
- Write for us
- 2093, Philadelphia Pike DE 19703, Claymont
Copyright @ 2024 Flexiple Inc
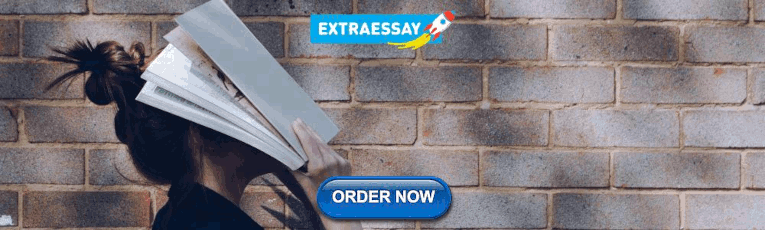
IMAGES
VIDEO
COMMENTS
Add values to dictionary Using the in-place merge ( |= ) operator. By using the in place merge operator we can combine two dictionaries. In in place operator, all elements of one dictionary are added in the other but while using merge original state of the dictionaries remains the same and a new one is created. Python3.
Python dictionaries are versatile and essential for managing key-value pairs in programming. Adding values to a dictionary is a fundamental skill in Python. Assign Values Using Unique Keys. Assigning values to a dictionary in Python involves using unique keys. Each key in a dictionary is distinct and serves as an identifier for its ...