Conditionals
if statements allow you to take different actions depending on which conditions are met. For example:
- if the city is equal to ( == ) "Toronto" , then set drinking_age to 19.
- Otherwise ( else ) set drinking_age to 21.
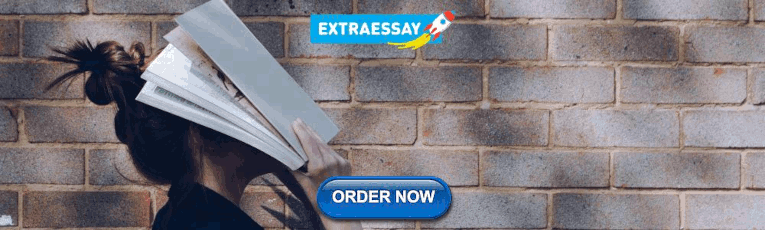
true and false
Ruby has a notion of true and false. This is best illustrated through some example. Start irb and type the following:
The if statements evaluates whether the expression (e.g. ' city == "Toronto" ) is true or false and acts accordingly.
Most common conditionals
Here is a list of some of the most common conditionals:
String comparisons
How do these operators behave with strings? Well, == is string equality and > and friends are determined by ASCIIbetical order.
What is ASCIIbetical order? The ASCII character table contains all the characters in the keyboard. It lists them in this order:
Start irb and type these in:
elsif allows you to add more than one condition. Take this for example:
Let's go through this:
- If age is 60 or more, we give a senior fare.
- If that's not true, but age is 14 or more, we give the adult fare.
- If that's not true, but age is more than 2 we give the child fare.
- Otherwise we ride free.
Ruby goes through this sequence of conditions one by one. The first condition to hold gets executed. You can put as many elsif 's as you like.
Example - fare_finder.rb
To make things more clear, let's put this in a program. The program asks for your age and gives you the corresponding fare.
Type this in and run it. It should behave like this:
This will output:
Here age is both greater than 10 and greater than 20. Only the first statement that holds true gets executed.
The correct way to write this would be:
Re-arrange these characters in ASCIIbetical order:
The ASCII table contains all the characters in the keyboard. Use irb to find out wheter the characters "?" lies:
- After 9 but before A.
- After Z but before a.
Using your experience the previous question, make a program that accepts a character input and tells you if the character lines:
Then try the program with the following characters:
Sample answers:
- $ lies before 0
- < lies between 9 and A
- - lies between Z and a
- ~ lies after z
Ruby Tricks, Idiomatic Ruby, Refactorings and Best Practices
Conditional assignment.
Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read.
There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
If you're just assigning based on a boolean, the ternary operator works best (this is not specific to ruby/lisp but still good and in the spirit of the following examples!).
If you need to perform more complex logic based on a conditional or allow for > 2 outcomes, if / elsif / else statements work well.
If you're assigning based on the value of a single variable, use case / when A good barometer is to think if you could represent the assignment in a hash. For example, in the following example you could look up the value of opinion in a hash that looks like {"ANGRY" => comfort, "MEH" => ignore ...}
Ruby Assignments in Conditional Expressions
For around two and a half years, I had been working in a Python 2.x environment at my old job . Since switching companies, Ruby and Rails has replaced Python and Flask. At first glance, the languages don’t seem super different, but there are definitely common Ruby idioms that I needed to learn.
While adding a new feature, I came across code that looked like this:
Intuitively, I guessed this meant “if some_func() returns something, do_something with it.” What took a second was understanding that you could do an assignment conditional expressions.
Coming from Python
In Python 2.x land (and any Python 3 before 3.8), assigning a variable in a conditional expression would return a syntax error:
Most likely, the assignment in a conditional expression is a typo where the programmer meant to do a comparison == instead.
This kind of typo is a big enough issue that there is a programming style called Yoda Conditionals , where the constant portion of a comparison is put on the left side of the operator. This would cause a syntax error if = were used instead of == , like this:
Back to Ruby
Now that I’ve come across this kind of assignment in Ruby for the first time, I searched whether assignments in conditional expressions were good style. Sure enough, the Ruby Style Guide has a section called “Safe Assignment in Condition” . The section and examples are short and straightforward. You should take a look if this Ruby idiom is new to you!
What is interesting about this idiom is that the assignment should be wrapped in parentheses. Take a look at the examples from the Ruby Style Guide:
Why is the first example bad? Well, the Style Guide also says that you shouldn’t put parentheses around conditional expressions . Parentheses around assignments in conditional expressions are specifically called out as an exception to this rule.
My guess is that by enforcing two different conventions, one for pure conditionals and one for assignments in conditionals, the onus is now on the programmer to decide what they want to do. This should prevent typos where an assignment = is used when a comparison == was intended, or vice versa.
The Python Equivalent
As called out above, assignments in conditional expressions are possible in Python 3.8 via PEP 572 . Instead of using an = for these kinds of assignments, the PEP introduced a new operator := . As parentheses are more common in Python expressions, introducing a new operator := (informally known as “the walrus operator”!!) was probably Python’s solution to the typo issue.
TheDeveloperBlog.com
Home | Contact Us
C-Sharp | Java | Python | Swift | GO | WPF | Ruby | Scala | F# | JavaScript | SQL | PHP | Angular | HTML
- Ruby if Examples: elsif, else and unless
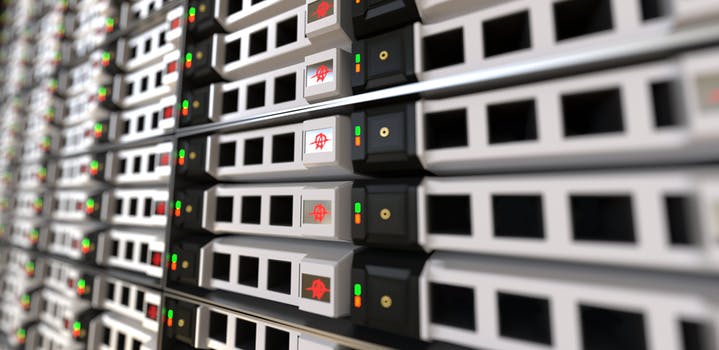
Related Links:
- Ruby Convert Types: Arrays and Strings
- Ruby File Handling: File and IO Classes
- Ruby Regexp Match Method
- Ruby String Array Examples
- Ruby include: Array Contains Method
- Ruby Class Examples: Self, Super and Module
- Ruby DateTime Examples: require date
- Ruby 2D Array Examples
- Ruby Number Examples: Integer, Float, zero and eql
- Ruby Exception Examples: Begin and Rescue
- Top 65 Ruby Interview Questions (2021)
- Ruby on Rails Interview Questions (2021)
- Ruby String Examples (each char, each line)
- Ruby Math Examples: floor, ceil, round and truncate
- Ruby Sub, gsub: Replace String
- Ruby Substring Examples
- Ruby Console: Puts, Print and stdin
- Ruby Remove Duplicates From Array
- Ruby Random Number Generator: rand, srand
- Ruby Recursion Example
- Ruby ROT13 Method
- Ruby Iterator: times, step Loops
- Ruby String Length, For Loop Over Chars
- Ruby Join Example (Convert Array to String)
- Ruby Format String Examples
- Ruby Copy Array Example
- Ruby Keywords
- Ruby Nil Value Examples: NoMethodError
- Learn Ruby Tutorial
- Ruby Method: Def, Arguments and Return Values
- Ruby Fibonacci Sequence Example
- Ruby Hash Examples
- Ruby While, Until and For Loop Examples
- Learn Ruby on Rails Tutorial
- Ruby Word Count: Split Method
- Ruby Sort Arrays (Use Block Syntax)
- Ruby Case Examples: Ranges, Strings and Regexp
- Ruby Array Examples
- Ruby Split String Examples
Related Links

Conditional Statements in Ruby
Conditional statements are an essential part of any programming language, including Ruby. They give us the ability to make decisions and alter the flow of our code based on certain conditions. In this tutorial, we will delve into the basics of conditional statements in Ruby, exploring various types and their usage.
If Statements
The most basic form of a conditional statement is the if statement. It allows us to execute a block of code only if a certain condition is met.
Here is a simple example:
In this example, we first declare a variable age with a value of 25. The if statement checks whether age is greater than 18. If the condition evaluates to true, the code inside the if block is executed, and the message "You are an adult!" is printed.
We can also add an else block after the if block to handle cases when the condition is not met. Taking the previous example further:
In this case, since age is 16, the condition age > 18 is false, and thus the code in the else block is executed, printing "You are not yet an adult."
ElseIf Statements
Ruby provides the elsif statement as a way to add additional conditions to our if statements. This allows us to handle multiple possible outcomes in a more organized manner.
Consider the following example:
In this example, we have multiple conditions. If age is less than 18, the first if block is executed. If not, the program checks the next condition using the elsif keyword. If age is between 18 and 65, the second elsif block is executed. Finally, if none of the previous conditions are met, the code inside the else block is executed.
Case Statements
Another way to handle conditional statements in Ruby is by using case statements. These statements can be helpful when dealing with multiple possible values for a variable.
Here is an example:
In this case statement, we check the value of the variable day . Depending on its value, different code blocks are executed. In this example, since day is "Monday," the code inside the first when block is executed, printing "It's the beginning of the week."
Case statements also support ranges and multiple conditions for each when block. They are a flexible tool for handling complex conditional logic.
Conditional statements are a crucial aspect of programming in any language, and Ruby is no exception. They provide us with the ability to control the flow of our code based on specific conditions. In this tutorial, we explored the basics of conditional statements in Ruby, including if statements, elsif statements for multiple conditions, and case statements for handling various possible values.
With the knowledge gained from this tutorial, you are now equipped to use conditional statements effectively in your Ruby programs. Happy coding!
Remember to save this Markdown file and convert it to HTML using your preferred Markdown converter to properly format and present the content on your blog.

Hi, I'm Ada, your personal AI tutor. I can help you with any coding tutorial. Go ahead and ask me anything.
I have a question about this topic
Give more examples
Ruby: If Statements
One of the most powerful features present in most programming and scripting languages is the ability to change the flow of the program as certain conditions change. The simplest form of flow control and logic in Ruby is called an "if statement" (or technically speaking in Ruby, since everything is an expression, an "if expression").
These 'if' experssions essentially check whether a condition is true or not. In Ruby they are created by writing the if keyword, followed by a condition, optionally followed by the then keyword, then the code you want to execute if the condition is true, and then finally the end keyword to end the structure. So they should look something like the following:
As mentioned though, the then is often dropped from the structure as it simply provides separation from the condition and the code to execute - one-line 'if' statements require the then to separate these, but multi-line ones like we'll be writing most of the time can use a new line to separate the condition and code.
From here the obvious question is: "How do I formulate a condition?". Well firstly, it's important to note that any expression that evaluates to a 'true' or 'false' value can be in the place of the condition there -- that includes boolean variables and method calls! So the following would always output "Hello" as 'some_variable' will, in this case, always evaluate to true:
"Real" conditions, for example comparing one variable to another, can be created by using 'conditional' or 'comparison' operators. These are operators that are made for comparing things, and when values are present at both sides of the operator, this will result in a 'true' or 'false' value. The basic conditional operators are:
- == - Is equal to
- != - Is not equal to
- > - Is greater than
- < - Is less than
- >= - Is greater than or equal to
- <= - Is less than or equal to
So to put these into actions, let's create a basic script which checks if the user is an administrator of our made-up system – it's not going to be very secure or really good for anything at the moment, but it'll be a good example to make sure we know this stuff.
The easiest way to authenticate a user is probably via a username and password, so the first thing our example script should do is ask for this data. Luckily we already know how to do this using puts and gets , however in this example it's more suitable to use print instead of puts - the two essentially do the same thing, however puts appends a newline to the output whereas print does not. So let's write this portion of the script!
From here, the 'if' statements need to do their magic. In this case we can just hard-code the administrator username and password into our script (not a good idea in a real system, but it'll work fine here). So first our comparison between the administrator username and what the user actually entered:
From here we want to check if the password is correct. If statements and other similar structures can actually be nested, so in this case it might be a nice idea to put another 'if' statement inside this one to check if the password is correct, like so:
You can see I've added a little puts message so that we can tell when the username and password were correct ("foo" and "bar"). If you take our code so far and put it into a ruby file and run it, you should see that our special message only displays when "foo" and "bar" are entered! But what do we do if we want something to happen when the username and password are entered wrongly? Perhaps we want to display a message telling the user that the details they entered were wrong. We could write a few 'if' statements that use the "is not equal to" ( != ) operator, however this seems like a waste of processing power given that we've already determined if the credentials are correct or not. This is where 'else' statements come in.
After an 'if' statement, just before 'end', you can introduce an 'else' clause. The code in this clause will execute if the 'if' statement was not true (and if none of the other 'if's in that chain were true, but we'll get on to this in a minute). These are accomplished using the else keyword where end would usually be, and moving end to the end. The syntax is as follows:
In our example we could easily utilise this functionality to output errors to the user in our example program:
As I previously touched on however, you can actually have more than one condition in an 'if' statement "chain", in which case the 'else' clause will only happen if none of the conditions are true. A nice way to show this in our example is if our system had multiple users. Perhaps we want to add a 'guest' user which doesn't require a password. We can add these "extra conditions" by using what is called an 'else if', written via the elsif keyword. Reading 'if' chains out-loud often makes some sense of them: "If this, do this, else if this, do this, else if this, do this, else, do this", this should also make obvious the fact that the next item in the chain will only be proceeded to if the previous is false – so elsif s are only checked if the primary condition is false, and else s are only checked if everything else is false. The syntax of an 'elsif' in a chain is as follows:
With this, it should be fairly easy to create a guest user:
You could argue that asking the 'guest' user for their password isn't necessary, and this could be fixed by moving the password prompt and gets into the administrator 'if' statement, and feel free to see if you can do this, however it's not important for this example.
The best way to learn about 'if' statements is really to play around with them. A good idea might be to try creating a script which takes the score for an exam out of 100 and then outputs a grade depending on the score entered (within certain grade boundaries – the greater than and less than comparisons should be useful here!). The only problem you might run into in creating this script is the comparison of different data types. If you try to compare a string variable (for example something received from gets ) with an integer (like 1), then you will run into problems. As such, you might want to explore to to_i and to_s methods. The former will likely want to be used in this example and is a method to convert strings to integers, while the latter is less useful in this example however may be more useful in others, and converts integers to strings.
You could use the to_i method on the gets.chomp itself (probably the better option here), or if you wanted you could use it in every condition with comparison to an integer. The former is shown, basically, below:
Moving away from this a bit, which hopefully you should now understand and be able to use, there is also some more flexibility and some more shorthand options in this 'if' functionality. Firstly, a basic 'if' can actually be after the line that should be executed if it's true, like so:
Similarly, a basic 'if' statement can be compressed to one line if the then keyword is used to replace the newline:
Ruby also has something called a "ternary operator" which provides a shortcut way of making basic comparisons. The syntax of this is a condition, followed by a question mark, followed by the expression that should be given if the condition is true, followed by a colon, followed by the expression that should be given if the condition is false, as can be seen below:
This is useful when a quick and compact decision between expressions is necessary, for example the following:
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Ruby Programming Language
- Ruby For Beginners
- Ruby Programming Language (Introduction)
- Comparison of Java with other programming languages
- Similarities and Differences between Ruby and C language
- Similarities and Differences between Ruby and C++
- Environment Setup in Ruby
- How to install Ruby on Linux?
- How to install Ruby on Windows?
- Interesting facts about Ruby Programming Language
- Ruby | Keywords
- Ruby | Data Types
- Ruby Basic Syntax
- Hello World in Ruby
- Ruby | Types of Variables
- Global Variable in Ruby
- Comments in Ruby
- Ruby | Ranges
- Ruby Literals
- Ruby Directories
- Ruby | Operators
- Operator Precedence in Ruby
- Operator Overloading in Ruby
- Ruby | Pre-define Variables & Constants
- Ruby | unless Statement and unless Modifier
Control Statements
- Ruby | Decision Making (if, if-else, if-else-if, ternary) | Set - 1
- Ruby | Loops (for, while, do..while, until)
- Ruby | Case Statement
- Ruby | Control Flow Alteration
- Ruby Break and Next Statement
- Ruby redo and retry Statement
- BEGIN and END Blocks In Ruby
- File Handling in Ruby
- Ruby | Methods
- Method Visibility in Ruby
- Recursion in Ruby
- Ruby Hook Methods
- Ruby | Range Class Methods
- The Initialize Method in Ruby
- Ruby | Method overriding
- Ruby Date and Time
OOP Concepts
- Object-Oriented Programming in Ruby | Set 1
- Object Oriented Programming in Ruby | Set-2
- Ruby | Class & Object
- Private Classes in Ruby
- Freezing Objects | Ruby
- Ruby | Inheritance
- Polymorphism in Ruby
- Ruby | Constructors
- Ruby | Access Control
- Ruby | Encapsulation
- Ruby Mixins
- Instance Variables in Ruby
- Data Abstraction in Ruby
- Ruby Static Members
- Ruby | Exceptions
- Ruby | Exception handling
- Catch and Throw Exception In Ruby
- Raising Exceptions in Ruby
- Ruby | Exception Handling in Threads | Set - 1
- Ruby | Exception Class and its Methods
- Ruby | Regular Expressions
- Ruby Search and Replace
Ruby Classes
- Ruby | Float Class
- Ruby | Integer Class
- Ruby | Symbol Class
- Ruby | Struct Class
- Ruby | Dir Class and its methods
- Ruby | MatchData Class
Ruby Module
- Ruby | Module
- Ruby | Comparable Module
- Ruby | Math Module
- Include v/s Extend in Ruby
Collections
- Ruby | Arrays
- Ruby | String Basics
- Ruby | String Interpolation
- Ruby | Hashes Basics
- Ruby | Hash Class
- Ruby | Blocks
Ruby Threading
- Ruby | Introduction to Multi-threading
- Ruby | Thread Class-Public Class Methods
- Ruby | Thread Life Cycle & Its States
Miscellaneous
- Ruby | Types of Iterators
- Ruby getters and setters Method
Ruby | Decision Making (if, if-else, if-else-if, ternary) | Set – 1
Decision Making in programming is similar to decision making in real life. In programming too, a certain block of code needs to be executed when some condition is fulfilled. A programming language uses control statements to control the flow of execution of the program based on certain conditions. These are used to cause the flow of execution to advance and branch based on changes to the state of a program. Similarly, in Ruby, the if-else statement is used to test the specified condition.
Decision-Making Statements in Ruby:
if statement
- if-else statement
- if – elsif ladder
- Ternary statement
If statement in Ruby is used to decide whether a certain statement or block of statements will be executed or not i.e if a certain condition is true then a block of statement is executed otherwise not.
Flowchart:
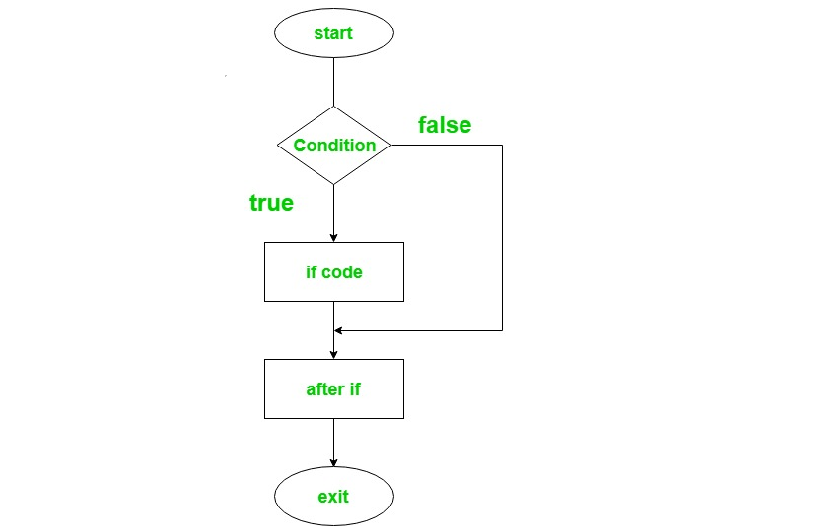
Output:
if – else Statement
In this ‘if’ statement used to execute block of code when the condition is true and ‘else’ statement is used to execute a block of code when the condition is false.
Syntax:
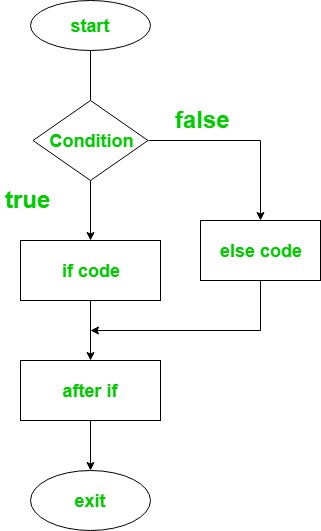
Example:
If – elsif – else ladder Statement
Here, a user can decide among multiple options. ‘if’ statements are executed from the top down. As soon as one of the conditions controlling the ‘if’ is true, the statement associated with that ‘if’ is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed.
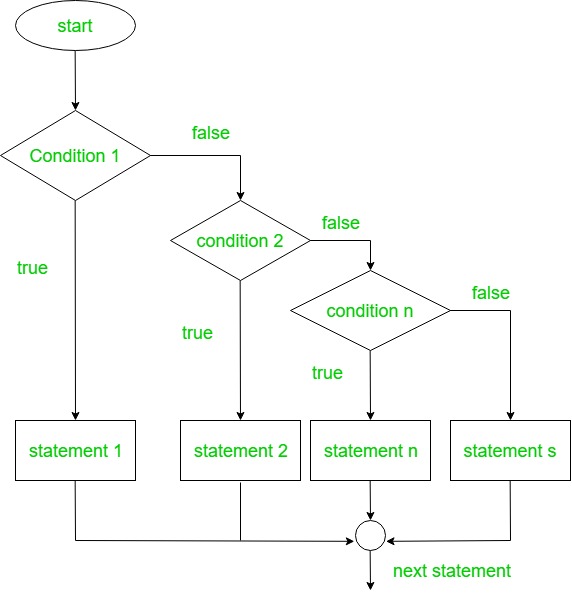
Ternary Statement
In Ruby ternary statement is also termed as the shortened if statement . It will first evaluate the expression for true or false value and then execute one of the statements. If the expression is true, then the true statement is executed else false statement will get executed.
Syntax:
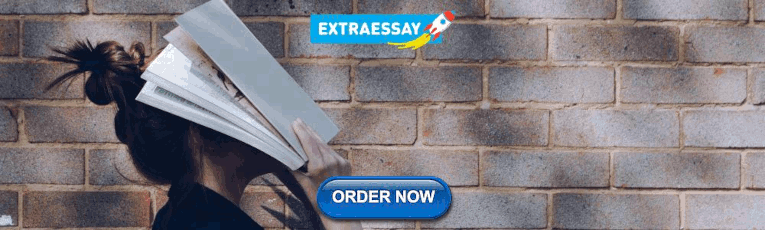
Please Login to comment...
Similar reads.
- Ruby-Basics
- Ruby-Decision-Making
- 10 Best Todoist Alternatives in 2024 (Free)
- How to Get Spotify Premium Free Forever on iOS/Android
- Yahoo Acquires Instagram Co-Founders' AI News Platform Artifact
- OpenAI Introduces DALL-E Editor Interface
- Top 10 R Project Ideas for Beginners in 2024
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How-To Geek
Ruby if, else if command syntax.
The Ruby language has a very simple control structure that is easy to read and follow.
if var == 10 print "Variable is 10" end
If Else Syntax
if var == 10 print "Variable is 10" else print "Variable is something else" end
If Else If Syntax
Here's the key difference between Ruby and most other languages. Note that "else if" is actually spelled "elsif" without the e.
if var == 10 print "Variable is 10" elsif var == "20" print "Variable is 20" else print "Variable is something else" end
Ternary (shortened if statement) Syntax
Ternary syntax is the same in Ruby as most languages. The following sample will print "The variable is 10" if var is equal to 10. Otherwise it will print "The variable is Not 10".
print "The variable is " + (var == 10 ? "10" : "Not 10")
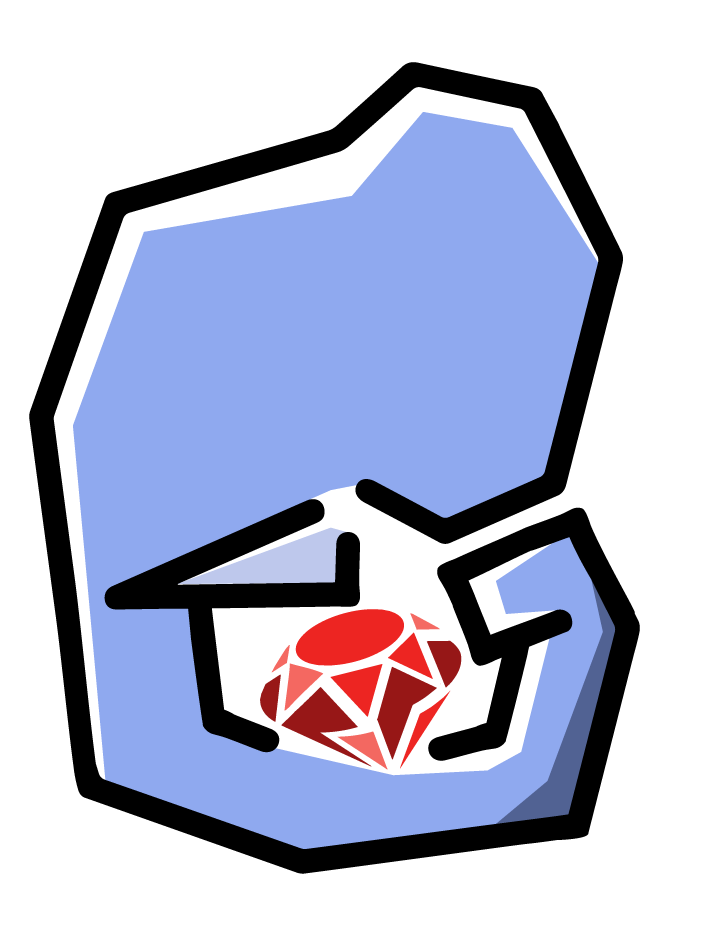
PHP to Ruby
If statements in ruby.
May 12, 2018
Prehaps the most central concept to all of program. The classic if statement:
The basic if statement is nearly identical in Ruby:
It really only becomes slighty different when you get to the if…else if…else statements:
Now I apologize ahead of time, but it’s really not that different in Ruby. The only real difference is the keyword elsif instead of the fully spelled out else if like we’re used to:
Variable Assignments in If Statements
Here’s where PHP and Ruby start to diverge in the simple if statement. In PHP if we want to assign a variable while using a conditional, we cannot do something like this:
PHP will complain loudly. Instead we do thing like this:
We can assign variables inside of the condition. However, we cannot assign the result of a function to a variable.
Ruby says, why not?
Guard Statements
Guard statements are a unique concept to Ruby - at least coming from a PHP background.
They’re actually used quite a bit as a way of controlling program flow in PHP:
In my PHP pseudo code example, we check to see if the current user is not authenticated - a.k.a. is a guest. If they’re not logged in, then we do not allow them to order delicious pancakes.
Instead, we throw them an unauthenciated page of some sort. Once they login, then they can think about ordering breakfast.
This is an example of a guard statement. The only difference that Ruby tacks on to this concept is that you can accomplish the same idea in one line :
This way the user.create_order isn’t called if the request.authenticated? returns false.
Unless vs. !
In PHP we’re used to ! mean NOT or the opposite.
Here we only update the user->name if the $name variable is not null. It’s only one small character, but it essentially reverses the logic of the conditional. It’s such a small detail that it’s easily overlooked, and an overlooked ! in a conditional can be a huge pain in the rear.
Using ! in a conditional is very un-Ruby like. Instead of that tiny little character reversing the flow of the logic, Ruby has a reserved keyword for that. Let’s introduce our friend unless :
Ruby tries it’s darnest to read like human language. After years of ! it may take some time for this keyword to be intuitive but it will click. It literally reads unless the name is nil then update the user’s name.
Let’s combine Ruby Guard statements with unless to refactor this code into a one liner:
Common operations like checking for presence of variables is a breeze thanks to clever conditional placement.
What the heck is ? doing in method names?
You may have raised an eyebrow to a question mark character in my method example name.nil? . This is another weird concept that’s in Ruby but not in PHP. You are allowed to place certain punction marks in method names.
It’s very Ruby-list to end a method with a ? if that method returns a boolean value.
For example .nil? is a built in method in every Ruby Object. It returns true or false if the current Object is actually Nil .
In PHP, we have a pseudo standard of naming methods that return a boolean by prefixing them with is . You’ll come across some methods in PHP classes like isAdmin() or isFulfilled , etc.
Extra Ruby fun, yes you can use !’s in method names!
As you venture out into real Ruby code, you’ll occasionally see methods that end with a ! . This simply means that the operatio will affect the original variable .
For example .map is a common function that maps a functio over an array. Each item in the array is transformed by the function passed to .map . When the array is fully iterated over, the result is stored in a result variable:
The result is the result of the mapping. However, the original numbers array emerges unscathed.
Now let’s add a .map! to see the difference:
.map! totally applied the mapping to the original variable a.k.a. numbers . Now your original list is gone and replaced by the mapping. You can see how this is useful, but also potentially dangerous. I’ll let you use your best judgement on how to wield this power.

- Shop to Support Independent Journalism
- We Have Issues
- Investigations
- Ethics Policy
- Ad-Free Login
Trump's statement on murder victim Ruby Garcia cribbed words from N.Y. Post obit: report

David Edwards
Senior editor, david edwards has spent over a decade reporting on social justice, human rights and politics for raw story. he also writes crooks and liars. he has a background in enterprise resource planning and previously managed the network infrastructure for the north carolina department of correction..

Former President Donald Trump reused words offered in a murder victim's obituary while claiming to have spoken to her family while paying tribute to her.
Fox 17 reported that Trump used Ruby Garcia's murder as the backdrop for his Tuesday attack on President Joe Biden, accusing him of a "bloodbath" on the U.S. border.
Garcia's sister said Trump had not spoken with the family before the event. Trump, however, claimed to know the victim's family in his Michigan speech.
ALSO READ: No, Donald Trump, fraud is not protected by the First Amendment
"Ruby's loved ones and community are left grieving for this incredible young woman," Trump said Tuesday. "They said she had just this most contagious laughter and when she walked into a room, she lit up that room — and I've heard that from so many people . I spoke to some of her family."
Trump's words echoed a statement made in Garcia's obituary, Media Matters' Matthew Gertz observed.
"Ruby's love for plants and traveling came nothing close to her affectionate smile that illuminated the room or contagious laughter that recreated the atmosphere," the obituary said .
Garcia was allegedly killed by her boyfriend Brandon Ortiz-Vite, a Mexico native who was in the U.S. illegally, the Michigan State Police said.
Trump got Garcia's age wrong in his speech, and was then criticized by the victim's sister who said it was "shocking" that he'd claimed to have been in touch with the family.
“He did not speak with any of us, so it was kind of shocking seeing that he had said that he had spoke with us," said Mavi Garcia.
Stories Chosen For You
Should trump be allowed to run for office, expert says letitia james has two ways to get 'not yet public' trump bond information.
New York Attorney General Letitia James wants answers on how former President Donald Trump's bond in the civil fraud case is structured — and she is strategizing multiple avenues to get the information she needs, wrote legal expert Lisa Rubin on X.
"Earlier today, the New York Attorney General filed a motion seeking more information about Trump's $175 million bond in their case," wrote Rubin. "But they're also apparently going at the issue sideways as well through a letter sent to Judge Arthur Engoron tonight."
"Specifically, that letter asks Engoron to modify his post-trial order concerning the powers and duties of the independent monitor in two ways. One of the AG's requests is that Engoron clarify that the monitor can communicate with any of the parties ex parte -- meaning, without all sides present," wrote Rubin, posting a copy of James' proposed order.
ALSO READ: 'Outlooks mediocre or worse': Trump Media investors warned off in alarming Forbes analysis
What makes this so significant, Rubin noted, is that the monitor, retired federal judge Barbara Jones, has information about the bond "that is not yet public."
"Given that provision, the AG's team could be hedging its bets. Either they'll get information on how the bond is collateralized through their motion for justification of the surety - -or, if Engoron expands the monitor's ability to speak to either party ex parte , they can just ask her for it," Rubin concluded.
Trump is on the hook for over $450 million after Judge Engoron found he and his adult sons systematically falsified property valuations in order to manipulate tax liabilities and the terms of loans — but he is appealing it.
A state appellate court lowered the bond he had to post to $175 million, after which he managed to get the bond secured by Don Hankey, a billionaire who made his fortune in subprime car loans and was previously investigated by Trump's own administration .
Colorado Dem lawmaker announces alcoholism treatment after slurring during hearing
The No. 3 Democrat in the Colorado Senate is reportedly seeking treatment for substance abuse related to alcoholism.
Assistant Senate Majority Leader Faith Winter made the announcement after she appeared intoxicated this Wednesday during a community hearing, slurring her speech and seeming confused, according to the Colorado Sun .
“I deeply regret my behavior last night,” Winter said in a written statement. “I made a mistake and I’m truly sorry for any inconvenience or discomfort I caused. I take full responsibility for my actions and I am committed to making things right. I especially apologize to the city of Northglenn and the citizens that came out — I deeply care about your thoughts and community. I am now under the care of medical professionals and receiving treatment for my substance abuse disorder.”
According to reports, concerned colleagues have tried to confront Winter about her drinking and have encouraged her to seek treatment.
Winter said she's taking a break from her role as chair of the Senate Transportation and Energy Committee “in order to focus on my health.”
“I apologize to anyone who was affected by my actions and I appreciate your understanding,” she said. “Thank you for respecting me and my family’s privacy at this time.”
Winter was recently diagnosed with an autoimmune disease which she said “led to the development of pulmonary hypertension and put incredible stress on my heart.”
In September, she suffered a serious head injury and had to undergo surgery after an accident while riding her bike. In a post to her X account, she said she crashed into a curb while trying to “avoid being hit by a large truck.”
With about a month left to go in Colorado’s 2024 legislative session, it's not clear how her treatment will conflict with her duties as a lawmaker.
'Democrats are coming after me': Ted Cruz reportedly 'getting nervous' about Senate seat
Sen. Ted Cruz (R-TX) is reportedly scrambling to hold off a formidable challenge for his seat from the left.
The politician appeared on Fox News "Hannity" on Wednesday, pleading for financial reinforcements after claiming that he's being outpaced in fundraising to notch a third term.
"The Democrats are coming after me, they are gonna spend more than $100 million this year, George Soros is already spending millions of dollars in the state of Texas,” he told the host Sean Hannity, and first reported by Rolling Stone . “My opponent, a liberal Democrat named Colin Allred, is out raising Beto O’Rourke, my last opponent, 3 to 1. They are flooding millions of dollars into Texas — and the reason is simple. You remember my last reelection, it was a 3-point race. I won by 2.6 percent.”
READ ALSO: No, Donald Trump, fraud is not protected by the First Amendment
Texas has notoriously remained red ever since 1976, when Jimmy Carter won a single term .
Cruz's bid for staying in D.C. is going to have to best former NFL player and current U.S. Rep. Colin Allred (D-Texas), who breezed to victory last month in Texas’ Democratic Senate primary.
After claiming victory, Allred took a shot at his Republican rival.
"The fundamental reason that democracy works is that people elect leaders to represent them and their interests, try to fix things for them, not look out for themselves," he said . "We've had enough of that with Ted Cruz."
The senator was already trying to tamp down controversy involving iHeartMedia doling a $630,850 payment to the Cruz-affiliated super PAC, Truth and Courage .
The substantial offering raised ethics questions as to whether it was an "unlawful contribution" since it's possible that Cruz solicited donations of over $5,000 to his PAC, which would be illegal.
A spokesperson for Cruz responded to the accusations, telling The Houston Chronicle that Cruz appears on his podcast multiple "times a week for free."
Watch the video below or click the link.

How Donald Trump is spreading a dangerous mental illness to his supporters
A criminologist explains why keeping trump from the white house is all that matters, maga congressional candidate: michelle obama might be a man, bring back aunt jemima.
Copyright © 2024 Raw Story Media, Inc. PO Box 21050, Washington, D.C. 20009 | Masthead | Privacy Policy | Manage Preferences | Debug Logs For corrections contact [email protected] , for support contact [email protected] .
- Entertainment
- internet culture
What the Evidence Released From Ruby Franke’s Child Abuse Case Reveals
I n February, the former YouTuber Ruby Franke and her business partner Jodi Hildebrandt were sentenced to up to 30 years in prison for four counts of aggravated child abuse. Last week, the Washington County Attorney’s Office released evidence from the case, including body cam footage of the two women’s arrests, Franke’s handwritten journal entries in which she justifies abuse, over 200 photos, witness statements, and more.
Investigators say that both Franke and Hildebrandt were motivated by “religious extremism” to commit the abuse against Franke’s children in an attempt to “teach the children how to properly repent for imagined ‘sins’ and to cast the evil spirits out of their bodies,” according to a statement from the Washington County Attorney’s Office.
Before her arrest, Franke ran the “8 Passengers” vlog channel where she shared snippets of her life raising eight children with her now estranged husband. Over time, viewers found that her content grew increasingly disturbing as she detailed the ways in which her children were disciplined to an audience of over two million people. Hildebrandt, who was a Mormon life-coach, began working with Franke in 2022, and the two managed a Mormon life-coaching service together called “ConneXions.” Former patients of Hildebrandt’s told NBC News that she often did more harm than good. The two also shared parenting advice on their YouTube channel , much of which was noted by viewers to be homophobic, transphobic, racist, and ableist.
The two were arrested in August after Franke’s son escaped Hildebrandt’s house and ran to a neighbor’s to ask for help. Both were charged with six counts of child abuse and both pleaded guilty to four of the counts.
Ruby Franke’s diary detailed her justification the abuse
The Washington County Attorney’s Office shared multiple pages of Franke’s diary in the evidence release, in which she wrote about trying to get rid of “the devil” from her children. The earliest entry that was shared is dated July 9, and in the entries written after that point, she describes the abuse in detail. Franke writes about making the kids sleep on the floor, shaving their heads, forcing her son’s head underwater and plugging his nose, and starving them.
The kid’s names are redacted in the entries and listed as “E” and “R.” In one journal entry, Franke writes that she will not “feed the demon.” She also goes on to call her kids “weak-minded” and “manipulative.” Franke describes torturing them, making them do physically demanding labor in the sun, and keeping them isolated.
What happened after Franke’s son escaped Hildebrandt’s house?
Footage released by police from the home of the two people who alerted the authorities to Franke’s son escaping the house shows the encounter with the young boy in a witness statement. He says the boy appeared to be emaciated, and was walking on the hot asphalt with socks and duct tape around his ankles. When they asked him about the tape, he said that it was “personal business but that it was his fault.”
In the video footage provided by the Attorney’s Office, the young boy can be seen wearing an oversized, long-sleeved button-down shirt as he approaches the house. The witnesses say they pulled his sleeves up to find more duct tape around his wrists. The young boy told the witnesses, “I got these wounds because of me.” The witnesses called the police, and the ambulance came to their house and treated the boy’s injuries. Local authorities later found his sister, Ruby’s daughter, in a closet.
What the arrest footage shows
Footage released of both of the women’s arrests and interrogations shows that Franke did not speak until she had an attorney present. When Hildebrandt was arrested, she was already on the phone with an attorney.
In a recorded call between Franke and her now estranged husband a day after her arrest, she calls the situation a “witch hunt.” She says that “the devil has been after [her] for years” and that all of the abuse has been “exaggerated.”
“Adults have a really hard time understanding that children can be full of evil and what that takes to fight it,” Franke said on the phone. “And so, I don’t know any other adults who are going to see the truth.” She goes on to say that “satan” has taken away everything that she loves and that she’s a “good woman” who “doesn’t do naughty things.”
More Must-Reads From TIME
- Jane Fonda Champions Climate Action for Every Generation
- Passengers Are Flying up to 30 Hours to See Four Minutes of the Eclipse
- Biden’s Campaign Is In Trouble. Will the Turnaround Plan Work?
- Essay: The Complicated Dread of Early Spring
- Why Walking Isn’t Enough When It Comes to Exercise
- The Financial Influencers Women Actually Want to Listen To
- The Best TV Shows to Watch on Peacock
- Want Weekly Recs on What to Watch, Read, and More? Sign Up for Worth Your Time
Write to Moises Mendez II at [email protected]
You May Also Like
Trump made Ruby Garcia's murder part of the border debate. Her family says he's lying about it.
L ast month it was Laken Riley in Georgia . Now it's Ruby Garcia , a Michigan woman authorities say was killed by her undocumented partner, who is being thrust into the political spotlight by former President Donald Trump.
Garcia's case received little national publicity before Trump latched onto it this week. Yet it fits into a pattern of the former president seizing on a sensational story involving an undocumented individual to juice his campaign, which has focused heavily on the immigration issue.
Trump highlighted Garcia’s death during a speech in Michigan Tuesday, prompting critics to say he is politicizing her murder. Garcia’s sister also rebuked Trump for claiming he spoke with the slain woman’s family, which she said is not true.
Start the day smarter. Get all the news you need in your inbox each morning.
Hardline immigration rhetoric always has been central to Trump's political brand, and he has taken a special interest in crimes committed by undocumented immigrants.
In kicking off his first campaign for president in 2016 at Trump Tower in New York City, Trump declared that "When Mexico sends its people, they're not sending their best... they’re bringing drugs, they’re bringing crime, they’re rapists. And some, I assume, are good people.”
The former president often focuses on the most lurid criminal cases. Garcia was shot multiple times and left on the side of the road.
Yet evidence suggests undocumented immigrants don’t commit crimes at higher rates than the general population, and some studies show they may commit crime at lower rates, although the data is limited said Graham Ousey, a professor at William & Mary who co-wrote the book "Immigration and Crime: Taking Stock," which summarizes research on the issue. The trends are the same for violent crime, he said.
“This is a big political issue, maybe a wedge issue that I think is being adopted for a specific purpose," Ousey said. "But I think the evidence that we have doesn’t really back up the idea that we’re facing a crime wave as a result of immigration generally, or even undocumented immigration more specifically.”
Data on homicides: Laken Riley's death made the news, but undocumented migrants commit fewer homicides
Yet polls show voters are concerned about immigration and crime, and Trump is leaning into the issues hard as he works to meld them together.
GOP strategist John Yob said the issue could help Trump win over soft Republican voters in the suburbs, particularly "soccer moms" concerned about public safety. Some of these voters have been turned off by Trump and issues such as Jan. 6, 2021, when a mob of Trump supporters stormed the U.S. Capitol, and GOP efforts to restrict abortion.
"The swing voting soccer moms... have morphed into security moms who are concerned about the safety of their families as illegal immigrants move north into their communities and commit violent crimes," said Yob, a GOP consultant who has worked extensively in Michigan GOP politics and on multiple presidential campaigns.
Trump echoed those comments Tuesday during the Grand Rapids event, saying "the suburban housewives actually like Donald Trump. You know why? Because I'm the one who's gonna keep them safe."
Trump's Michigan speech was billed as "remarks on Biden's border bloodbath" and the Republican National Committee rolled out a website Tuesday with the same theme.
An RNC press release said the website is "dedicated to highlighting the horrors of Biden Migrant Crime." Trump also sent out a fundraising email Tuesday with the subject line "Build the wall - Deport them all!"
Flanked by uniformed law enforcement officers Tuesday at the Grand Rapids Convention Center, Trump repeated comments he has made previously that crimes committed by undocumented immigrants constitutes "a new form of crime, it's called migrant crime."
"It should be called Biden migrant crime, but that's too long," Trump said.
The former president devoted a significant portion of his speech to Garcia, saying she was "savagely murdered by an illegal alien criminal."
Trump rallied his supporters around Riley's death earlier this year. A Georgia nursing student, Riley was murdered in February while jogging. Police charged Jose Antonio Ibarra, who is in the country illegally, with Riley's murder.
President Joe Biden also mentioned Riley during his State of the Union address. Biden called Ibarra "an illegal" and later said he regretted using the term, prompting Trump to criticize him.
Trump has accused Biden of not doing enough to stop illegal immigration, prompting Biden to point to bipartisan border legislation he supported and Trump opposed.
U.S. Rep. Hillary Scholten, a Democrat who represents the Grand Rapids area, also mentioned the border bill Tuesday in arguing that Trump is more interested in "political theatre" than solving the immigration problem.
“He’s come to our community to try to sow fear and hatred and division," Scholten said. "And when we are facing such a monumental crisis at our southern border what we need now more than ever is unity, bipartisanship and people who are willing to put the political theatre aside so we can get things done."
Garcia's case garnered attention in local media in west Michigan but received little national exposure until Tuesday.
Garcia's body was found along a road near downtown Grand Rapids late in the evening on March 22. Brandon Ortiz-Vite, 25, was charged with Garcia's murder and confessed to the crime. The two were in a romantic relationship, officials said.
Ortiz-Vite came to the country "unlawfully" as a child and was given legal status through the Deferred Action for Childhood Arrivals program, but that expired in 2019. He was deported in 2020, according to U.S. Immigration and Customs Enforcement, but returned illegally to the U.S. at "an unknown date."
"We threw him out of the country and crooked Joe Biden took him back," Trump said Tuesday.
Scholten called it "deeply unsettling" and "horrifying" that Trump is trying to "politicize" Garcia's death, and said he is ignoring the fact that Garcia was a victim of domestic violence and that her alleged killer obtained the weapon illegally.
"Yes, absolutely we should be talking about the fact that the individual who killed her was in this country without proper authorization," she said, adding: "But make no mistake, Ruby Garcia also died because of domestic violence and because someone had a gun who shouldn’t have had one."
Scholten noted that many women are victims of domestic violence but "you don’t see the same kind of outrage."
"I think it’s very telling that they want to politicize the immigration aspect of this because that’s the only aspect that they’re talking about,” she added.
A woman who identified herself as Garcia's sister expressed frustration on Facebook that her death had become "political," saying "I hope reporters stop suing my sisters story to turn it into some political (expletive)." Riley's father voiced similar concerns in an interview that "she's being used somewhat politically ," although other members of her family appeared with Trump at a rally last month in Georgia.
Trump said Tuesday he spoke with members of Garcia's family, but her sister told the FOX17 and Target 8 television stations that was false.
“He did not speak with any of us, so it was kind of shocking seeing that he had said that he had spoke with us, and misinforming people on live TV,” Mavi Garcia told Target 8.
Mavi Garcia also appeared to question Trump's focus on crimes committed by undocumented immigrants.
“It’s always been about illegal immigrants,” she said. “Nobody really speaks about when Americans do heinous crimes, and it’s kind of shocking why he would just bring up illegals. What about Americans who do heinous crimes like that?"
After highlighting Garcia's death Tuesday, Trump also briefly mentioned another murder by an undocumented immigrant last year in western Michigan. Luis Bernal-Sosa was convicted in February of killing Leah Gomez. A statement from ICE says Bernal-Sosa was in the country illegally.
While Garcia's death is "tragic," Ousey said that focusing on Ortiz-Vite's immigration status "stereotypes and demonizes a group that doesn’t deserve it."
“It’s wrong and it’s unfortunate to suggest a person’s immigration status is somehow a causal factor in their behavior," Ousey said.
Yob said the murders deserve attention.
“Two murders in West Michigan is two too many and illegal immigrants do not have a right to be in our community and our voters have a right to feel safe in their own backyards," he said.
Pollster Doug Kaplan says immigration is a more potent issue than many Democrats realize, pointing to broad support for building the wall.
“People laughed at Donald Trump for it and now over 50% of the population says build the wall,” Kaplan said.
Yet Kaplan isn’t sure the issue will move college-educated women voters, as Republicans hope.
“Suburban women, will they care about that or Biden’s issues - Democracy, women’s issues?” Kaplan said. “It can go either way.”
This article originally appeared on USA TODAY: Trump made Ruby Garcia's murder part of the border debate. Her family says he's lying about it.
Ruby Franke's husband claims Jodi Hildebrandt was possessed
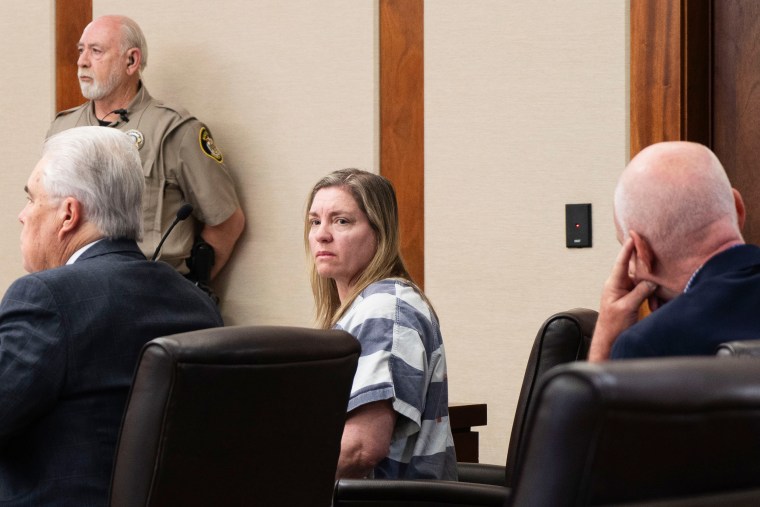
The estranged husband of Ruby Franke, the Utah family vlogger convicted of child abuse, alleged that her business partner, Jodi Hildebrandt, was possessed, according to an interview released last week by prosecutors in Washington County, Utah.
Kevin Franke, who filed for divorce from Ruby Franke in November, said he became Hildebrandt's “resident exorcist” during the time they allowed the former life coach to stay in their house.
The two were arrested in August after police found one of Franke’s sons emaciated with open wounds and bound with duct tape. He had escaped Hildebrandt’s home to a neighbor’s house. One of Franke’s daughters was found in a similar malnourished condition in Hildebrandt’s home.
Both women were sentenced in February to four one- to 15-year terms in prison, served consecutively. Under Utah law, the maximum aggregate sentence for consecutive terms is 30 years.
In the interview, Kevin Franke — who has not been charged with any crimes — said he witnessed Hildebrandt at times “go into possession mode” and “talk in different voices.”
“It was really creepy,” he said, “but the voices would say: ‘She’s ours. We’re not letting go. She is Satan’s bride.’”
Attorneys for Kevin Franke and Hildebrandt did not immediately respond to requests for comment.
Winward Law, which represents Ruby Franke, said it was “unaware of this interview with Kevin Franke.”
“At this time, Ruby Franke does not want to make public comments. She is committed to seeing her family heal and using this time to focus on her own health and healing,” a spokesperson for the law firm said in an email statement to NBC News. “It is our hope that the all involved will find peace and justice.”
The interview is the latest window into the monthslong child abuse case that became a public spectacle . Last week, the Washington County Attorney’s Office also released Ruby Franke’s heavily redacted handwritten journal entries, which detailed months of abuse.
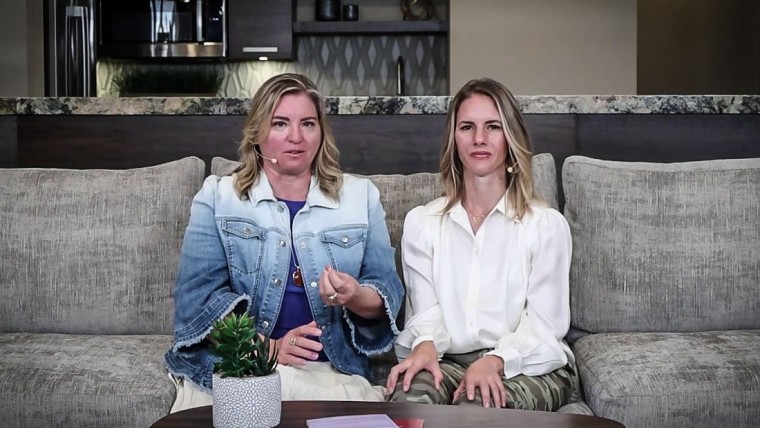
The Franke family rose to prominence on YouTube, where they amassed 2.3 million subscribers to their now-defunct channel, “8 Passengers.” Ruby Franke’s strict parenting style on the channel had drawn concern from viewers and neighbors in the years before her arrest.
Hildebrandt also faced scrutiny over her life-coaching service, ConneXions, which some former clients have described to NBC News as a program that isolated them from loved ones and destroyed marriages.
Kevin Franke told authorities why he and Ruby Franke decided to take Hildebrandt in, sharing his timeline of events in the years the two women became close.
He said he got involved with ConneXions in 2020 after his wife and one of her close friends persuaded him to join a men’s group that met with Hildebrandt every week. For a while, he said, the meetings seemed to help strengthen their marriage.
But things began to change in March 2021, he said, when Hildebrandt allegedly told her inner circle that she “believed she was being tormented and haunted by shadow figures every night.” By mid-April that year, he said, Hildebrandt had reached out to Ruby Franke for help.
“Ruby was convinced that we could intervene and help Jodi,” he told investigators. “I didn’t want anything to do with it.”
Kevin Franke said he visited Hildebrandt’s home for the first time in May 2021, when she opened up to the couple about her struggles. That was when he noticed “crashes in the basement while we were talking upstairs and plates in the kitchen just flying off by themselves, like full speed smashing on the wall and falling to the floor by themselves.”
Eventually, he said, he reluctantly agreed to take Hildebrandt into his and Ruby Franke’s home. He said he saw Hildebrandt go into trances.
“The moment she showed up at my house, just the weirdest crap started happening: lights turning on and off, sounds of people walking in walls — like footprints going up walls and across the ceiling — and stuff floating around,” he said. “It was weird and I hated it. And I became the resident exorcist.”
He said Ruby Franke had begun going into her own trances by September 2021, during which she allegedly believed she was in heaven speaking with God and Jesus. He claimed she and Hildebrandt would lock themselves in a room for hours, after which, he said, Ruby Franke would tell him about her visions and the work God had called upon them to do.
Read NBC News' coverage of Ruby Franke:
- Ruby Franke sentenced in child abuse case
- ‘Big day for evil’: Former YouTuber Ruby Franke detailed how she abused her children in handwritten journal entries
- Ruby Franke’s business partner gave life coaching that ruined lives, some former clients say
Kevin Franke described growing more suspicious of Hildebrandt and her intentions as Ruby Franke appeared to grow closer to her. He claimed that prompted Ruby Franke to ask for a separation in July 2022. After he moved out, he told investigators, every week turned into “psychological hell.”
“The only way I would ever get back into my house was I had to get Jodi’s approval, because if I didn’t get Jodi’s approval. I would never get Ruby’s approval,” he said.
“As I’m looking back, I’m realizing there wasn’t a solution, and it was, you either had Jodi’s approval or you didn’t,” he said, adding, “She became like the arbiter of truth, the arbiter of forgiveness, God’s own mouthpiece.”
In October last year, just before Kevin Franke filed for divorce, his attorney Randy Kester told TODAY.com that the Frankes had been separated “at Ruby’s directive.”
“Kevin did not want to be separated,” Kester said. “He wanted to work through concerns as a family. There was never any formal, written decree of separate maintenance or separation agreement. The separation was under terms prescribed by Ruby and Jodi Hildebrandt.”
Lawyers for Ruby Franke said at the time of the divorce filing that she was “devastated” by the news but that she understood Kevin Franke’s reasoning and respected his decision.
During the trial, lawyers for Ruby Franke said Hildebrandt “systematically isolated” her from her family over a long period, which caused her to adopt a “distorted sense of morality” under Hildebrandt’s influence.
“For the past four years, I’ve chosen to follow counsel and guidance that has led me into a dark delusion,” Ruby Franke said at her sentencing hearing. “My distorted version of reality went largely unchecked as I would isolate from anyone who challenged me.”
Hildebrandt also gave a brief statement to the court during the sentencing.
“I desire for [the children] to heal physically and emotionally,” she said. “One of the reasons I did not go to trial is because I did not want them to emotionally relive the experience, which would have been detrimental to them. My hope and prayer is that they will heal and move forward to have beautiful lives.”

Angela Yang is a culture and trends reporter for NBC News.
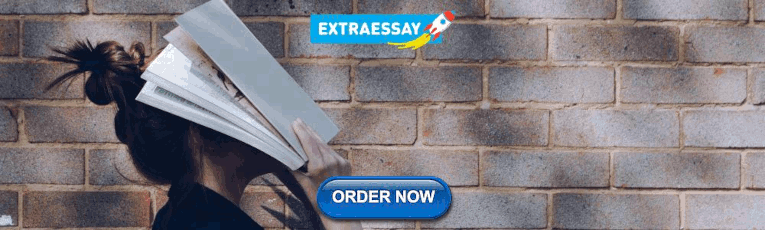
IMAGES
COMMENTS
Yes, it will. It's an instance variable. In ruby, if you prefix your variable with @, it makes the variable an instance variable that will be available outside the block. In addition to that, Ruby does not have block scope (block in the traditional sense, if/then/else in this case).
Since Ruby parses the bare a left of the if first and has not yet seen an assignment to a it assumes you wish to call a method. Ruby then sees the assignment to a and will assume you are referencing a local method. The confusion comes from the out-of-order execution of the expression. First the local variable is assigned-to then you attempt to ...
If something is true (the condition) then you can do something. In Ruby, you do this using if statements: stock = 10. if stock < 1. puts "Sorry we are out of stock!" end. Notice the syntax. It's important to get it right. The stock < 1 part is what we call a "condition".
The if. The if statement is how we create a branch in our program flow. The if statement includes a true-or-false expression: if 4 == 2 + 2. puts "The laws of arithmetic work today" end. If that expression evaluates to true, then the Ruby interpreter will execute the puts statement that follows the if statement.
Ruby has a notion of true and false. This is best illustrated through some example. Start irb and type the following: The if statements evaluates whether the expression (e.g. ' city == "Toronto") is true or false and acts accordingly. Warning: Notice the difference between ' = ' and ' == '. ' = ' is an assignment operator.
Conditional assignment. Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read. There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
SyntaxError: invalid syntax. Most likely, the assignment in a conditional expression is a typo where the programmer meant to do a comparison == instead. This kind of typo is a big enough issue that there is a programming style called Yoda Conditionals , where the constant portion of a comparison is put on the left side of the operator.
In Ruby, we use the && ( AND) operator to separate the conditions we want to check are true. Here's an example of an if statement with multiple conditions: if user_discount = = true && age < 5 // Run code. End. We can use the && operator as many times as we want to check if a certain statement evaluates to be true.
Ruby has a variety of ways to control execution. All the expressions described here return a value. For the tests in these control expressions, nil and false are false-values and true and any other object are true-values. In this document "true" will mean "true-value" and "false" will mean "false-value".
Assignment. In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. An assignment expression result is always the assigned value, including assignment methods.
If - Elseif - Else Statements. If the if statement is not true, the block of code in the elseif statement will be executed if the condition is true. There may me multiple elseif statements. Finally, if none of the conditions are true, the block of code in the else statement will be executed.
Ruby supports one-line if-statements. The "if" is used to modify the preceding statement. These statements are only run if the if-expression evaluates to true. ... Ruby will report a warning if you use just one equals sign. An assignment uses one equals. A conditional, two. But: The program still compiles. In some languages, this syntax results ...
If Statements. The most basic form of a conditional statement is the if statement. It allows us to execute a block of code only if a certain condition is met. Here is a simple example: age = 25. if age > 18. puts "You are an adult!" end. In this example, we first declare a variable age with a value of 25.
Ruby: If Statements. One of the most powerful features present in most programming and scripting languages is the ability to change the flow of the program as certain conditions change. The simplest form of flow control and logic in Ruby is called an "if statement" (or technically speaking in Ruby, since everything is an expression, an "if ...
Ternary Statement. In Ruby ternary statement is also termed as the shortened if statement. It will first evaluate the expression for true or false value and then execute one of the statements. If the expression is true, then the true statement is executed else false statement will get executed. Syntax:
Ruby Case & Ranges. The case statement is more flexible than it might appear at first sight. Let's see an example where we want to print some message depending on what range a value falls in. case capacity. when 0. "You ran out of gas." when 1..20. "The tank is almost empty. Quickly, find a gas station!"
The Ruby language has a very simple control structure that is easy to read and follow. If syntax. if var == 10 print "Variable is 10" end If Else Syntax. if var == 10 print "Variable is 10" ... Ternary (shortened if statement) Syntax. Ternary syntax is the same in Ruby as most languages. The following sample will print "The variable is 10" if ...
Variable Assignments in If Statements. Here's where PHP and Ruby start to diverge in the simple if statement. In PHP if we want to assign a variable while using a conditional, we cannot do something like this: ... This is an example of a guard statement. The only difference that Ruby tacks on to this concept is that you can accomplish the ...
The fact that if and case return values makes for some very tight, tidy, and yet still understandable code. It's a common pattern in Ruby when you're dealing with assignment through branching. The way I tend to approach formatting these is to apply a level of indentation to make the assignment clear, but not overly "push" the code in too far:
A statement obtained from a spokesperson at U.S. Immigration and Customs Enforcement noted that it is accurate that Ortiz-Vite was removed to Mexico on Sept. 29, 2020, when Mr. Trump was still ...
Fox 17 reported that Trump used Ruby Garcia's murder as the backdrop for his Tuesday attack on President Joe Biden, accusing him of a "bloodbath" on the U.S. border. Garcia's sister said Trump had ...
March 26, 2024 2:55 PM EDT. I n February, the former YouTuber Ruby Franke and her business partner Jodi Hildebrandt were sentenced to up to 30 years in prison for four counts of aggravated child ...
A statement from ICE says Bernal-Sosa was in the country illegally. While Garcia's death is "tragic," Ousey said that focusing on Ortiz-Vite's immigration status "stereotypes and demonizes a group ...
I am wondering if there is a way in Ruby to write a one-line if-then-else statement using words as operators, in a manner similar to python's a if b else c I am aware of the classic ternary conditional, but I have the feeling Ruby is more of a "wordsey" language than a "symboley" language, so I am trying to better encapsulate the spirit of the ...
The estranged husband of Ruby Franke, the Utah family vlogger convicted of child abuse, alleged that her business partner, Jodi Hildebrandt, was possessed, according to an interview released last ...
This is equivalent to: value = key rescue nil if value .. end or. value = begin key rescue nil end if value .. end Remember nil and false are the only two objects that are falsey in ruby and since value here could be nil, that if statement could return false.