How to solve “Error: Assignment to expression with array type” in C?

In C programming, you might have encountered the “ Error: Assignment to expression with array type “. This error occurs when trying to assign a value to an already initialized array , which can lead to unexpected behavior and program crashes. In this article, we will explore the root causes of this error and provide examples of how to avoid it in the future. We will also learn the basic concepts involving the array to have a deeper understanding of it in C. Let’s dive in!
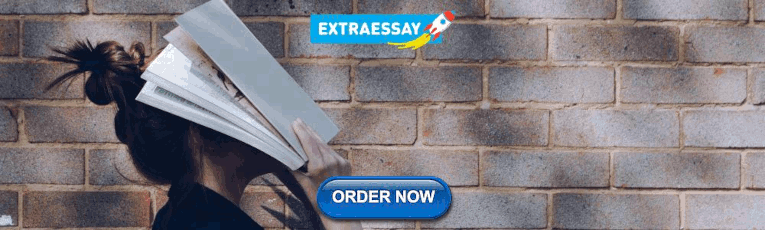
What is “Error: Assignment to expression with array type”? What is the cause of it?
An array is a collection of elements of the same data type that are stored in contiguous memory locations. Each element in an array is accessed using an index number. However, when trying to assign a value to an entire array or attempting to assign an array to another array, you may encounter the “Error: Assignment to expression with array type”. This error occurs because arrays in C are not assignable types, meaning you cannot assign a value to an entire array using the assignment operator.
First example: String
Here is an example that may trigger the error:
In this example, we have declared a char array “name” of size 10 and initialized it with the string “John”. Then, we are trying to assign a new string “Mary” to the entire array. However, this is not allowed in C because arrays are not assignable types. As a result, the compiler will throw the “Error: Assignment to expression with array type”.
Initialization
When you declare a char array in C, you can initialize it with a string literal or by specifying each character separately. In our example, we initialized the char array “name” with the string literal “John” as follows:
This creates a char array of size 10 and initializes the first four elements with the characters ‘J’, ‘o’, ‘h’, and ‘n’, followed by a null terminator ‘\0’. It’s important to note that initializing the array in this way does not cause the “Error: Assignment to expression with array type”.
On the other hand, if you declare a char array without initializing it, you will need to assign values to each element of the array separately before you can use it. Failure to do so may lead to undefined behavior. Considering the following code snippet:
We declared a char array “name” of size 10 without initializing it. Then, we attempted to assign a new string “Mary” to the entire array, which will result in the error we are currently facing.
When you declare a char array in C, you need to specify its size. The size determines the maximum number of characters the array can hold. In our example, we declared the char array “name” with a fixed size of 10, which can hold up to 9 characters plus a null terminator ‘\0’.
If you declare a char array without specifying its size, the compiler will automatically determine the size based on the number of characters in the string literal you use to initialize it. For instance:
This code creates a char array “name” with a size of 5, which is the number of characters in the string literal “John” plus a null terminator. It’s important to note that if you assign a string that is longer than the size of the array, you may encounter a buffer overflow.
Second example: Struct
We have known, from the first example, what is the cause of the error with string, after that, we dived into the definition of string, its properties, and the method on how to initialize it properly. Now, we can look at a more complex structure:
This struct “struct_type” contains an integer variable “id” and a character array variable “string” with a fixed size of 10. Now let’s create an instance of this struct and try to assign a value to the “string” variable as follows:
As expected, this will result in the same “Error: Assignment to expression with array type” that we encountered in the previous example. If we compare them together:
- The similarity between the two examples is that both involve assigning a value to an initialized array, which is not allowed in C.
- The main difference between the two examples is the scope and type of the variable being assigned. In the first example, we were dealing with a standalone char array, while in the second example, we are dealing with a char array that is a member of a struct. This means that we need to access the “string” variable through the struct “s1”.
So basically, different context, but the same problem. But before dealing with the big problem, we should learn, for this context, how to initialize a struct first. About methods, we can either declare and initialize a new struct variable in one line or initialize the members of an existing struct variable separately.
Take the example from before, to declare and initialize a new struct variable in one line, use the following syntax:
To initialize the members of an existing struct variable separately, you can do like this:
Both of these methods will initialize the “id” member to 1 and the “struct_name” member to “structname”. The first one is using a brace-enclosed initializer list to provide the initial values of the object, following the law of initialization. The second one is specifically using strcpy() function, which will be mentioned in the next section.
How to solve “Error: Assignment to expression with array type”?
Initialize the array type member during the declaration.
As we saw in the first examples, one way to avoid this error is to initialize the array type member during declaration. For example:
This approach works well if we know the value of the array type member at the time of declaration. This is also the basic method.
Use the strcpy() function
We have seen the use of this in the second example. Another way is to use the strcpy() function to copy a string to the array type member. For example:
Remember to add the string.h library to use the strcpy() function. I recommend going for this approach if we don’t know the value of the array type member at the time of declaration or if we need to copy a string to the member dynamically during runtime. Consider using strncpy() instead if you are not sure whether the destination string is large enough to hold the entire source string plus the null character.
Use pointers
We can also use pointers to avoid this error. Instead of assigning a value to the array type member, we can assign a pointer to the member and use malloc() to dynamically allocate memory for the member. Like the example below:
Before using malloc(), the stdlib.h library needs to be added. This approach is also working well for the struct type. In the next approach, we will talk about an ‘upgrade-version’ of this solution.
Use structures with flexible array members (FAMs)
If we are working with variable-length arrays, we can use structures with FAMs to avoid this error. FAMs allow us to declare an array type member without specifying its size, and then allocate memory for the member dynamically during runtime. For example:
The code is really easy to follow. It is a combination of the struct defined in the second example, and the use of pointers as the third solution. The only thing you need to pay attention to is the size of memory allocation to “s”. Because we didn’t specify the size of the “string” array, so at the allocating memory process, the specific size of the array(10) will need to be added.
This a small insight for anyone interested in this example. As many people know, the “sizeof” operator in C returns the size of the operand in bytes. So when calculating the size of the structure that it points to, we usually use sizeof(*), or in this case: sizeof(*s).
But what happens when the structure contains a flexible array member like in our example? Assume that sizeof(int) is 4 and sizeof(char) is 1, the output will be 4. You might think that sizeof(*s) would give the total size of the structure, including the flexible array member, but not exactly. While sizeof is expected to compute the size of its operand at runtime, it is actually a compile-time operator. So, when the compiler sees sizeof(*s), it only considers the fixed-size members of the structure and ignores the flexible array member. That’s why in our example, sizeof(*s) returns 4 and not 14.
How to avoid “Error: Assignment to expression with array type”?
Summarizing all the things we have discussed before, there are a few things you can do to avoid this error:
- Make sure you understand the basics of arrays, strings, and structures in C.
- Always initialize arrays and structures properly.
- Be careful when assigning values to arrays and structures.
- Consider using pointers instead of arrays in certain cases.
The “ Error: Assignment to expression with array type ” in C occurs when trying to assign a value to an already initialized array . This error can also occur when attempting to assign a value to an array within a struct . To avoid this error, we need to initialize arrays with a specific size, use the strcpy() function when copying strings, and properly initialize arrays within structures. Make sure to review the article many times to guarantee that you can understand the underlying concepts. That way you will be able to write more efficient and effective code in C. Have fun coding!
“Expected unqualified-id” error in C++ [Solved]
How to Solve does not name a type error in C++
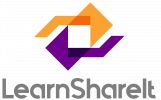
How To Solve Error “Assignment To Expression With Array Type” In C

“ Error: assignment to expression with array type ” is a common error related to the unsafe assignment to an array in C. The below explanations can help you know more about the cause of error and solutions.
Table of Contents
How does the “error: assignment to expression with array type” happen?
This error happens due to the following reasons: First, the error happens when attempting to assign an array variable. Technically, the expression (called left-hand expression) before the assignment token (‘=’) has the type of array, and C language does not allow any assignment whose left-hand expression is of array type like that. For example:
Or another example:
Output:
The example above shows that the left hand of the assignment is the arr variable, which is of the type array declared above. However, the left type does not have to be an array variable. Instead, it can be a call expression that returns an array, such as a string :
In C, the text that is put between two double quotes will be compiled as an array of characters, not a string type, just like other programming languages.
How to solve the error?
Solution 1: using array initializer.
To solve “error: assignment to expression with array type” (which is because of the array assignment in C) you will have to use the following command to create an array:
type arrayname[N] = {arrayelement1, arrayelement2,..arrayelementN};
For example:
Another example:
The first example guides you to create an array with 4 given elements with the value 1,2,3,4 respectively. The second example helps you create an array of char type, each char is a letter which is same as in the string in the double quotes. As we have explained, a string in double quotes is actually of array type. Hence, using it has the same effects as using the brackets.
Solution 2: Using a for loop
Sometimes you cannot just initialize an array because it has been already initialized before, with different elements. In this case, you might want to change the elements value in the array, so you should consider using a for loop to change it.
Or, if this is an integer array:
When you want to change the array elements, you have to declare a new array representing exactly the result you expect to change. You can create that new array using the array initialiser we guided above. After that, you loop through the elements in that new array to assign them to the elements in the original array you want to change.
We have learned how to deal with the error “ error: assignment to expression with array type ” in C. By avoiding array assignment, as long as finding out the reasons causing this problem in our tutorial , you can quickly solve it.
Maybe you are interested :
- Undefined reference to ‘pthread_create’ in Linux
- Print Char Array in C

I’m Edward Anderson. My current job is as a programmer. I’m majoring in information technology and 5 years of programming expertise. Python, C, C++, Javascript, Java, HTML, CSS, and R are my strong suits. Let me know if you have any questions about these programming languages.
Name of the university: HCMUT Major : CS Programming Languages : Python, C, C++, Javascript, Java, HTML, CSS, R
Related Posts
C tutorials.
- November 8, 2022
Learning C programming is the most basic step for you to approach embedded programming, or […]

How To Print Char Array In C
- October 5, 2022
We will learn how to print char array in C using for loops and built-in […]
- Edward Anderson
- October 4, 2022
“Error: assignment to expression with array type” is a common error related to the unsafe […]
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
cppreference.com
Array declaration.
Array is a type consisting of a contiguously allocated nonempty sequence of objects with a particular element type . The number of those objects (the array size) never changes during the array lifetime.
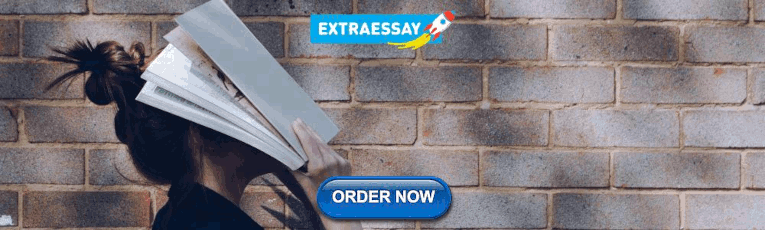
[ edit ] Syntax
In the declaration grammar of an array declaration, the type-specifier sequence designates the element type (which must be a complete object type), and the declarator has the form:
[ edit ] Explanation
There are several variations of array types: arrays of known constant size, variable-length arrays, and arrays of unknown size.
[ edit ] Arrays of constant known size
If expression in an array declarator is an integer constant expression with a value greater than zero and the element type is a type with a known constant size (that is, elements are not VLA) (since C99) , then the declarator declares an array of constant known size:
Arrays of constant known size can use array initializers to provide their initial values:
[ edit ] Arrays of unknown size
If expression in an array declarator is omitted, it declares an array of unknown size. Except in function parameter lists (where such arrays are transformed to pointers) and when an initializer is available, such type is an incomplete type (note that VLA of unspecified size, declared with * as the size, is a complete type) (since C99) :
[ edit ] Qualifiers
[ edit ] assignment.
Objects of array type are not modifiable lvalues , and although their address may be taken, they cannot appear on the left hand side of an assignment operator. However, structs with array members are modifiable lvalues and can be assigned:
[ edit ] Array to pointer conversion
Any lvalue expression of array type, when used in any context other than
- as the operand of the address-of operator
- as the operand of sizeof
- as the operand of typeof and typeof_unqual (since C23)
- as the string literal used for array initialization
undergoes an implicit conversion to the pointer to its first element. The result is not an lvalue.
If the array was declared register , the behavior of the program that attempts such conversion is undefined.
When an array type is used in a function parameter list, it is transformed to the corresponding pointer type: int f ( int a [ 2 ] ) and int f ( int * a ) declare the same function. Since the function's actual parameter type is pointer type, a function call with an array argument performs array-to-pointer conversion; the size of the argument array is not available to the called function and must be passed explicitly:
[ edit ] Multidimensional arrays
When the element type of an array is another array, it is said that the array is multidimensional:
Note that when array-to-pointer conversion is applied, a multidimensional array is converted to a pointer to its first element, e.g., pointer to the first row:
[ edit ] Notes
Zero-length array declarations are not allowed, even though some compilers offer them as extensions (typically as a pre-C99 implementation of flexible array members ).
If the size expression of a VLA has side effects, they are guaranteed to be produced except when it is a part of a sizeof expression whose result doesn't depend on it:
[ edit ] References
- C23 standard (ISO/IEC 9899:2023):
- 6.7.6.2 Array declarators (p: TBD)
- C17 standard (ISO/IEC 9899:2018):
- 6.7.6.2 Array declarators (p: 94-96)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.6.2 Array declarators (p: 130-132)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.5.2 Array declarators (p: 116-118)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.5.4.2 Array declarators
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 16 January 2024, at 16:08.
- This page has been accessed 250,901 times.
- Privacy policy
- About cppreference.com
- Disclaimers

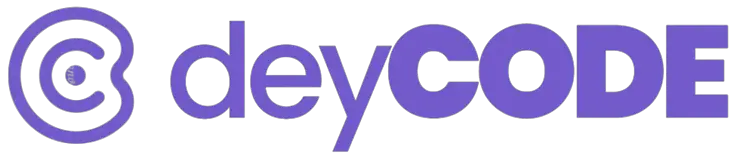
[Fixed] "error: assignment to expression with array type error" when I assign a struct field (C) – C
Quick Fix: In the provided code, the issue lies when assigning a value to a struct field, resulting in an "error: assignment to expression with array type error". To resolve this, use strcpy() to copy the value into the array instead of direct assignment. Additionally, you can initialize the struct using a brace-enclosed initializer list, where each member is assigned a value in order.
The Problem:
While using C structs, assigning values to struct fields directly using the dot operator (.) generates an error, such as assignment to expression with array type error , specifically when trying to assign string literals. This error occurs when attempting to assign a string literal, like "Paolo" , to a character array within a struct field, like s1.name . The compiler doesn’t allow such direct assignment because character arrays are treated as pointers to their first element, making the assignment invalid.
The Solutions:
Solution 1: modifying lvalue and brace-enclosed initializer list.
In C, an array is considered an lvalue, but unlike other data types, arrays are not directly modifiable. Therefore, you cannot directly assign a value to an array element using the assignment operator (=). Instead, you must use a function like strcpy() to copy the value into the array.
In your code, you attempted to directly assign the string "Paolo" to s1.name , which resulted in the compilation error. The correct way to assign a string to s1.name is to use strcpy() , like this:
You can also use a brace-enclosed initializer list to set the values of a struct when it is declared. This is a more concise way to initialize a struct, and it avoids the need to use strcpy() . For example, you could rewrite your main function as follows:
Using a brace-enclosed initializer list is generally the preferred way to initialize a struct, as it is more concise and easier to read. However, there are times when you may need to use strcpy() to assign a value to an array element, such as when you are dynamically allocating memory for the array.
Solution 2: Further Interpretation of Data Types and Assignments in C Structs
C structs are user-defined types that allow you to group related data together. When you declare a struct variable, a block of memory is allocated on the stack, and the compiler knows the size of this memory block based on the type of data you’re storing.
In your specific case, the issue was with this line of code:
You can’t assign a string directly to a char array inside a struct because it tries to assign a pointer to a string to a string. The char array s1.name is a fixed-size array with 30 characters, while " Paolo " is a pointer to a string literal.
To copy the string "Paolo" into the name field of the data struct, you need to use strcpy or memcpy to manually copy the characters into the array. Here’s an example using strcpy :
Alternatively, you can define your struct to have pointers to character arrays, like this:
In this case, you would assign the strings directly to the pointers:
This works because you’re now assigning pointers to strings, which is valid in C.
In summary, when working with structs in C, it’s important to understand the data types of the struct members and how assignments work. Always ensure you’re assigning the correct type of data to the struct members to avoid compilation errors.
Solution 3: Initialize the struct fields using strcpy
In C, strings are arrays of characters, and arrays are not assignable. This means that you can’t assign a string literal directly to a struct field that is an array of characters. Instead, you need to use the strcpy() function to copy the string literal into the struct field.
The corrected code below uses the strcpy() function to initialize the name and surname fields of the s1 struct:
Now, the code will compile and run without errors, and it will print the following output:
Solution 4: Using strcpy() function
The assignment error occurs because you cannot directly assign a string literal to a character array in C. Instead, you need to use the strcpy() function to copy the string literal into the character array. The strcpy() function takes two arguments: the destination character array and the source string literal. It copies the characters from the source string literal into the destination character array, overwriting any existing characters in the destination array.
Here is the modified code using the strcpy() function:
In this code, we first include the string.h header file, which contains the declaration of the strcpy() function. Then, we use the strcpy() function to copy the string literals “Paolo” and “Rossi” into the name and surname fields of the s1 structure, respectively.
With this modification, the code should compile and run without errors.
Is there any way to disable a service in docker-compose.yml – Docker
@Autowired – No qualifying bean of type found for dependency – Autowired
© 2024 deycode.com
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
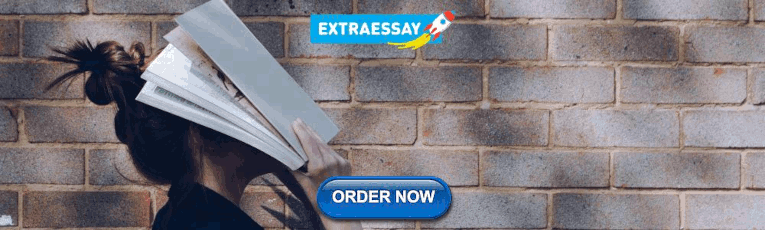
IMAGES
VIDEO
COMMENTS
First part, you try to copy two array of character (string is not a pointer, it is array of character that is terminated by null character \0 ). If you want to copy value of an array to another, you can use memcpy, but for string, you can also use strcpy. E[0].nom = "reda"; change to: strcpy(E[0].nom,"reda"); Second part, you make the pointer ...
Array is not a pointer. Arrays only decay to pointers causing a lots of confusion when people learn C language. You cant assign arrays in C. You only assign scalar types (integers, pointers, structs and unions) but not the arrays. Structs can be used as a workaround if you want to copy the array
Now let's create an instance of this struct and try to assign a value to the "string" variable as follows: struct struct_type s1; s1.struct_name = "structname"; // Error: Assignment to expression with array type. As expected, this will result in the same "Error: Assignment to expression with array type" that we encountered in the ...
The "Assignment to Expression with Array Type" occurs when we try to assign a value to an entire array or use an array as an operand in an expression where it is not allowed. In C and C++, arrays cannot be assigned directly. Instead, we need to assign individual elements or use functions to manipulate the array.
Using the unused variable br to store that return value, and the counter variable zbroj1 as an index into the array you can build up rec using the following code: rec[zbroj1]=br; zbroj1++; Strings in C need to have a \0 character at the end to terminate them so you'll also need to have the line to finish it.
Output: LearnShareIT. The first example guides you to create an array with 4 given elements with the value 1,2,3,4 respectively. The second example helps you create an array of char type, each char is a letter which is same as in the string in the double quotes.
Variable-length arrays. If expression is not an integer constant expression, the declarator is for an array of variable size.. Each time the flow of control passes over the declaration, expression is evaluated (and it must always evaluate to a value greater than zero), and the array is allocated (correspondingly, lifetime of a VLA ends when the declaration goes out of scope).
Solution 1: Assignment to Array Types. The issue occurs in the following statement: s1.name =" ;Paolo"; Copy. This is because you are trying to assign a string literal directly to an array ( s1.name) which is not permitted. According to the C11 standard, a modifiable lvalue (left-hand value) in an assignment statement must be of a type ...
Always ensure you're assigning the correct type of data to the struct members to avoid compilation errors. Solution 3: Initialize the struct fields using strcpy. In C, strings are arrays of characters, and arrays are not assignable. This means that you can't assign a string literal directly to a struct field that is an array of characters.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
How to fix the error:assignment to expression with array type#syntax #c #howto #clanguage #error #codeblocks
In your code, brand and model are already of array type, they have memory allocated to them based on their size (char [40]) on declaration. You need not allocate any memory using the allocator function (unlike pointers, on other hand). You cannot assign to an array type.
You cannot add 1 to an array of 5 ints, nor can you assign an array of 5 ints. A pointer to an array is not a widely-used type in C. To refer to the first element through a pointer to an array, you must use (*ptr)[0] = (*ptr)[0] + 1. This syntax is a bit cumbersome and gives you no real advantages over a using a simple int *ptr = data, which is ...
Cuando usas NuevoPtr->ID (sin subíndices) en una expresión, lo que realmente obtienes es la dirección de memoria del inicio del array. Como la memoria del array está en una posición fija, simplemente no puedes asignarle un nuevo valor. Si quieres cambiar valores, tendrás que hacerlo especificando los índices. NuevoPtr->ID[0] = id;
I'm assigning an array value to an array. The problem I believe is in the strtok function because I believe it serves as a char value. What I am thinking of doing is splitting the input and putting it into different var and then putting it into an array, but that isn't efficient.
You don't need arrays for the different types of days, just counter variables, which you increment based on the temperature range. total should not be sizeof(num) for two reasons: sizeof counts bytes, not array elements. You only want the count of inputs, not the total size of the array. You can get the total by adding the counters of each type ...
1. Arrays do not have the assignment operator. Arrays are non-modifiable lvalues. To change the content of an array use for example the standard string function strcpy. #include <string.h >. //... strcpy( month, "March" ); An alternative approach is to declare the variable month as a pointer. For example.
That's the way the language goes: you cannot assign to an array, full stop. You can assign to pointers, or copy arrays with memcopy, but never try to assign to an array. BTW, char a={"a"}; is a horror: a is a single character while "a" is a string literal that is the const array {'a', '\0'}. Please use char a = 'a'; for a single char or char a ...
1. You can use typedefs to simplify your task. A single array-based tree that stores pointers would look like this: typedef car** car_bst; It's just an array of pointers. An array of three such things would look like this: car_bst cars[3]; Now if you think about it a bit, this is equivalent to. car **cars[3];