Verilog Blocking & Non-Blocking
Blocking assignment statements are assigned using = and are executed one after the other in a procedural block. However, this will not prevent execution of statments that run in a parallel block.
Note that there are two initial blocks which are executed in parallel when simulation starts. Statements are executed sequentially in each block and both blocks finish at time 0ns. To be more specific, variable a gets assigned first, followed by the display statement which is then followed by all other statements. This is visible in the output where variable b and c are 8'hxx in the first display statement. This is because variable b and c assignments have not been executed yet when the first $display is called.
In the next example, we'll add a few delays into the same set of statements to see how it behaves.
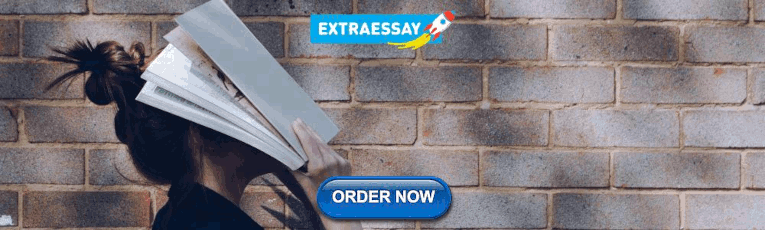
Non-blocking
Non-blocking assignment allows assignments to be scheduled without blocking the execution of following statements and is specified by a symbol. It's interesting to note that the same symbol is used as a relational operator in expressions, and as an assignment operator in the context of a non-blocking assignment. If we take the first example from above, replace all = symobls with a non-blocking assignment operator , we'll see some difference in the output.
See that all the $display statements printed 'h'x . The reason for this behavior lies in the way non-blocking assignments are executed. The RHS of every non-blocking statement of a particular time-step is captured, and moves onto the next statement. The captured RHS value is assigned to the LHS variable only at the end of the time-step.
So, if we break down the execution flow of the above example we'll get something like what's shown below.
Next, let's use the second example and replace all blocking statements into non-blocking.
Once again we can see that the output is different than what we got before.
If we break down the execution flow we'll get something like what's shown below.
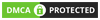
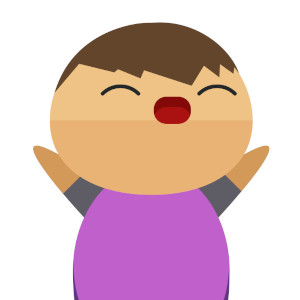
Blocking and Non-blocking Assignment in Verilog
- Assignment is only done in procedural block(always ot initial block)
- Both combintational and sequential circuit can be described.
- Assignment can only possible to reg type irrespect of circuit type
Let's say we want to describe a 4-bit shift register in Verilog. For this, we are required to declare a 3-bit reg type variable.
The output of shift[0] is the input of shift[1], output of shift[1] is input of shift[2], and all have the same clock. Let's complete the description using both assignment operator.
Non-Blocking Assignment
When we do synthesis, it consider non-blocking assignment separately for generating a netlist. If we see register assignment in below Verilog code, all register are different if we consider non-blocking assignment separately. If you do the synthesis, it will generate 3 registers with three input/output interconnects with a positive edge clock interconnect for all register. Based on the Verilog description, all are connected sequentially because shift[0] is assigned d, shift[1] is assigned shift[0], and shift[2] is assigned shift[1].
Blocking Assignment
If we use blocking assignment and do the syhtheis, the synthesis tool first generate netlist for first blocking assignment and then go for the next blocking assignment. If in next blocking assignment, if previous output of the register is assigned to next, it will generate only a wire of previously assigned register.
In below Verilog code, even though all looks three different assignment but synthesis tool generate netlist for first blocking assigment which is one register, working on positive edge of clock, input d and output shift[0]. Since blocking assignment is used, for next blocking assignment, only wire is generated which is connected to shift[0]. Same is for next statement a wire is generated which is connected to shift[0].
Click like if you found this useful
Add Comment

This policy contains information about your privacy. By posting, you are declaring that you understand this policy:
- Your name, rating, website address, town, country, state and comment will be publicly displayed if entered.
- Your IP address (not displayed)
- The time/date of your submission (displayed)
- Administrative purposes, should a need to contact you arise.
- To inform you of new comments, should you subscribe to receive notifications.
- A cookie may be set on your computer. This is used to remember your inputs. It will expire by itself.
This policy is subject to change at any time and without notice.
These terms and conditions contain rules about posting comments. By submitting a comment, you are declaring that you agree with these rules:
- Although the administrator will attempt to moderate comments, it is impossible for every comment to have been moderated at any given time.
- You acknowledge that all comments express the views and opinions of the original author and not those of the administrator.
- You agree not to post any material which is knowingly false, obscene, hateful, threatening, harassing or invasive of a person's privacy.
- The administrator has the right to edit, move or remove any comment for any reason and without notice.
Failure to comply with these rules may result in being banned from submitting further comments.
These terms and conditions are subject to change at any time and without notice.
Comments (1)

hey in blocking assignment do we get shift in data i dont think so . we get all values same and equal to d.
Please do not focus on the module name; focus on how the netlist is generated after the synthesis.
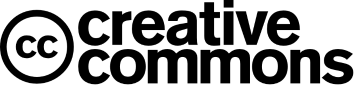

Blocking vs. Nonblocking in Verilog
The concept of Blocking vs. Nonblocking signal assignments is a unique one to hardware description languages. The main reason to use either Blocking or Nonblocking assignments is to generate either combinational or sequential logic. In software, all assignments work one at a time. So for example in the C code below:
The second line is only allowed to be executed once the first line is complete. Although you probably didn’t know it, this is an example of a blocking assignment. One assignment blocks the next from executing until it is done. In a hardware description language such as Verilog there is logic that can execute concurrently or at the same time as opposed to one-line-at-a-time and there needs to be a way to tell which logic is which.
<= Nonblocking Assignment
= Blocking Assignment
The always block in the Verilog code above uses the Nonblocking Assignment, which means that it will take 3 clock cycles for the value 1 to propagate from r_Test_1 to r_Test_3. Now consider this code:
See the difference? In the always block above, the Blocking Assignment is used. In this example, the value 1 will immediately propagate to r_Test_3 . The Blocking assignment immediately takes the value in the right-hand-side and assigns it to the left hand side. Here’s a good rule of thumb for Verilog:
In Verilog, if you want to create sequential logic use a clocked always block with Nonblocking assignments. If you want to create combinational logic use an always block with Blocking assignments. Try not to mix the two in the same always block.
Nonblocking and Blocking Assignments can be mixed in the same always block. However you must be careful when doing this! It’s actually up to the synthesis tools to determine whether a blocking assignment within a clocked always block will infer a Flip-Flop or not. If it is possible that the signal will be read before being assigned, the tools will infer sequential logic. If not, then the tools will generate combinational logic. For this reason it’s best just to separate your combinational and sequential code as much as possible.
One last point: you should also understand the semantics of Verilog. When talking about Blocking and Nonblocking Assignments we are referring to Assignments that are exclusively used in Procedures (always, initial, task, function). You are only allowed to assign the reg data type in procedures. This is different from a Continuous Assignment . Continuous Assignments are everything that’s not a Procedure, and only allow for updating the wire data type.
Leave A Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Blocking (immediate) and Non-Blocking (deferred) Assignments in Verilog
There are Two types of Procedural Assignments in Verilog.
- Blocking Assignments
- Nonblocking Assignments
To learn more about Delay: Read Delay in Assignment (#) in Verilog
Blocking assignments
- Blocking assignments (=) are done sequentially in the order the statements are written.
- A second assignment is not started until the preceding one is complete. i.e, it blocks all the further execution before it itself gets executed.
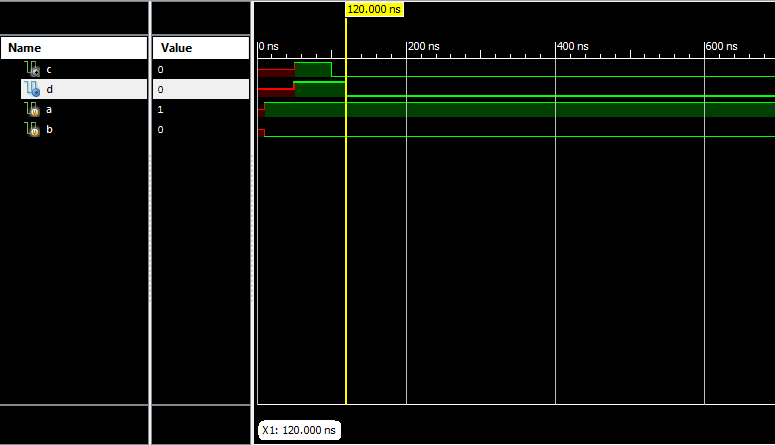
Non-Blocking assignments
- Nonblocking assignments (<=), which follow each other in the code, are started in parallel.
- The right hand side of nonblocking assignments is evaluated starting from the completion of the last blocking assignment or if none, the start of the procedure.
- The transfer to the left hand side is made according to the delays. An intra- assignment delay in a non-blocking statement will not delay the start of any subsequent statement blocking or non-blocking. However normal delays are cumulative and will delay the output.
- Non-blocking schedules the value to be assigned to the variables but the assignment does not take place immediately. First the rest of the block is executed and the assignment is last operation that happens for that instant of time.

To learn more about Blocking and Non_Blocking Assignments: Read Synthesis and Functioning of Blocking and Non-Blocking Assignments
The following example shows interactions between blocking and non-blocking for simulation only (not for synthesis).
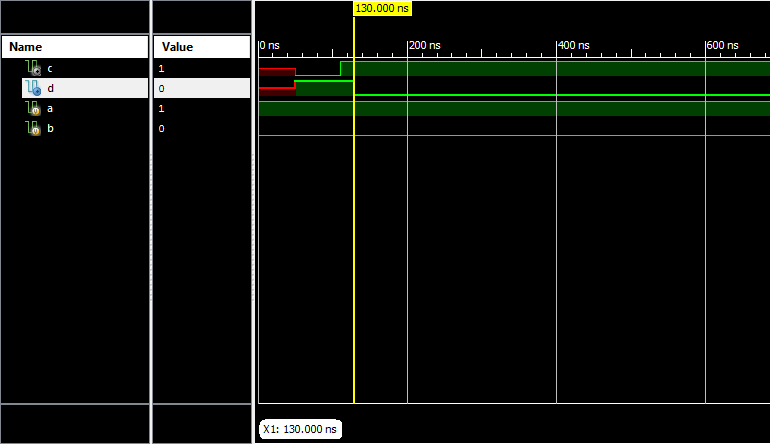
For Synthesis (Points to Remember):
- One must not mix “<=” or “=” in the same procedure.
- “<=” best mimics what physical flip-flops do; use it for “always @ (posedge clk..) type procedures.
- “=” best corresponds to what c/c++ code would do; use it for combinational procedures.
Spread the Word
- Click to share on Facebook (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Pocket (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to print (Opens in new window)
Related posts:
- Synthesis and Functioning of Blocking and Non-Blocking Assignments.
- Delay in Assignment (#) in Verilog
- Ports in Verilog Module
- Module Instantiation in Verilog
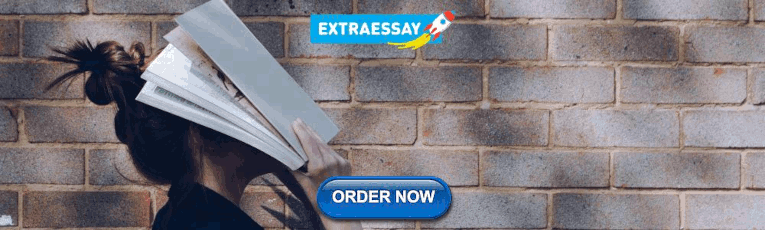
Post navigation
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Notify me of follow-up comments by email.
Notify me of new posts by email.

News the global electronics community can trust

The trusted news source for power-conscious design engineers

Supply chain news for the electronics industry

The can't-miss forum engineers and hobbyists

The electronic components resource for engineers and purchasers

Design engineer' search engine for electronic components

Product news that empowers design decisions

The educational resource for the global engineering community

The learning center for future and novice engineers

The design site for electronics engineers and engineering managers

Where makers and hobbyists share projects

The design site for hardware software, and firmware engineers

Where electronics engineers discover the latest tools

Hardware design made easy

Brings you all the tools to tackle projects big and small - combining real-world components with online collaboration

Circuit simulation made easy

A free online environment where users can create, edit, and share electrical schematics, or convert between popular file formats like Eagle, Altium, and OrCAD.

Transform your product pages with embeddable schematic, simulation, and 3D content modules while providing interactive user experiences for your customers.

Find the IoT board you’ve been searching for using this interactive solution space to help you visualize the product selection process and showcase important trade-off decisions.

A worldwide innovation hub servicing component manufacturers and distributors with unique marketing solutions

SiliconExpert provides engineers with the data and insight they need to remove risk from the supply chain.

Transim powers many of the tools engineers use every day on manufacturers' websites and can develop solutions for any company.

Search EEWeb
- Newsletters
- Internal/External
- Embedded Microstrip
- Symmetric Stripline
- Asymmetric Stripline
- Wire Microstrip
- Wire Stripline
- Edge-Coupled Microstrip
- Edge-Coupled Stripline
- Broadside Coupled Stripline
- Twisted Pair
- RF Unit Converter
- Online SPICE Simulator
- Schematic Converter/Viewer
- Schematic Capture Tool
- Standard Values
- Magnetic Field Calculator
- Wire Self Inductance
- Parallel Wires
- Wire over Plane
- Rectangle Loop
- Broadside-Coupled Traces
- Edge-Coupled Traces
- Engineering Paper (Printable PDF)
- Log-Log Graph Paper (PDF Download)
- Semi-Log Graph Paper (PDF Download)
- Smith Chart Graph Paper (PDF Download)
- Math Reference Sheets Overview
- Algebra Reference Sheet
- Geometry Reference Sheet
- Trigonometry Definitions and Functions
- Trigonometry Rules, Laws, and Identities
- Calculus Derivatives, Rules, and Limits
- Calculus Integrals Reference Sheet
- Online Basic Calculator
- Scientific Calculator
- Tech Communities
- Analog Design
- Power Design
- Digital Design
- Embedded Systems
- Test & Measurement
- Electromechanical
- Passive Components
- Design Library
- Extreme Circuits
- Magazines Archive
- Design Contest
Verilog Blocking vs Non-Blocking Assignments

In writing verilog one of the common misunderstandings is the difference between a blocking and a non-blocking assignment. Explain the difference and the syntax used with these assignments?
There are synthesis differences between a blocking statement and a non-blocking statement.
Blocking Assignment
The syntax for a blocking assignment is:
always @ (pos edge clk) begin x=y; y=z; end
In this blocking assignment immediately after rising transition of the clk signal, x=y=z.
Non-Blocking Assignment
The syntax for a non-blocking assignment is:
always @ (pos edge clk) begin x<=y; y<=z; end
In this non-blocking assignment immediately after rising transition of the clk signal, x=y and y=z, but x!=z. Synthesis for this code would typically create a register for x and a register for y. Where blocking assignments often are synthesized as logic.
Other Related Topics
- Photonic Device as Miniature Toolkit for Measurements
- 3D Print Prototype for ‘Bionic Eye’
- Glass Data Storage
- Biodegradable, Paper-based Biobatteries
Join the Conversation!
Add comment cancel reply.
You must be logged in to post a comment.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
- FarhnSh1d 2024-04-03 02:40:00 1. Energy Source Availability: Identify available energy sources such as solar, wind, vibration, thermal gradients, or ambient RF signals. Choose a technology capable of effectively capturing and converting this energy into usable electrical power. 2. Energy Density and Power Output: Assess the energy density and power output of each potential source to determine if it can meet the application's power requirements, considering factors like sensor power consumption and duty cycle. 3. Environmental Conditions: Evaluate the environmental conditions of the remote location, including temperature extremes, humidity, and exposure to elements. Choose a technology that can withstand these conditions and operate reliably...
- FarhnSh1d 2024-04-03 02:39:02 Reducing the output power of an ultrasonic Piezo Ceramic disc from 35 watts to 10 watts by adjusting the control board is possible but entails several considerations: Effectiveness and Performance Lowering power may compromise the effectiveness of the ultrasonic device, potentially reducing its ability to achieve desired results like cleaning or atomization. Reduced power might lead to weaker vibrations, impacting performance, especially if the device operates away from its optimal resonance frequency. Durability and Lifespan Lowering power could potentially increase the device's lifespan by reducing stress on the piezo ceramic disc. However, extreme power reductions may lead to inadequate vibrations...
- forlinxembedded 2024-03-21 04:05:55 Hello Erdem, It's interesting to learn about the differences in electric pole usage between countries. In many countries, wooden poles are commonly used due to their availability, cost-effectiveness, and ease of installation. However, in regions with higher load requirements like Turkey, stronger poles are necessary to support the infrastructure. In the USA and some European countries, wooden poles are reinforced and treated to withstand the required loads. Additionally, alternative materials like steel and concrete are also used for electric poles in various applications. If you're interested in learning more about electric pole types and standards in different countries, I recommend...
- Lumispot 2024-03-04 11:00:10 Microcontroller For a project like yours, the choice of a microcontroller (MCU) largely depends on the complexity of the device, the number of input/output peripherals you need (like GPIOs for the sensor, speaker, LED lights, and the green light indicator), and any additional features you might require (such as PWM outputs for LED dimming or speaker volume control). Arduino Uno is a robust choice for beginners and prototypes. It's based on the ATmega328P and offers a good balance of GPIO pins, is easy to program, and has extensive community support. Arduino Nano or Arduino Micro could be more suitable for...
- richard-gabric 2024-03-04 11:00:01 You are setting yourself up to kill yourself, or at least to take an eye out. You don't say what DC voltage you need, the voltage you would get from directly applying the mains to a bridge rectifier were typically used, for example, to supply the input to a flyback switch mode power supply, where the switching transformer provides mains isolation. The voltage would typically be around 300V DC, and the rectifier and capacitors have to be rated for that voltage plus a safety factor. I assume that you are needing a low voltage DC, since you have 25Vdc capacitors...
- suvsystemltd 2024-03-01 09:15:42 one suitable device is the LTC4371 from Analog Devices. The LTC4371 is an automotive-grade ideal diode controller designed to operate in harsh environments, making it compliant with the AEC-Q100 standard. Key features of the LTC4371 include: Input Voltage Range: The LTC4371 typically operates with an input voltage range that covers your specified 2.5V requirement. AEC-Q100 Qualification: The LTC4371 is qualified for automotive applications, meeting the AEC-Q100 standard. This ensures reliability and performance under automotive operating conditions. Ideal Diode Functionality: The LTC4371 is specifically designed as an ideal diode/OR-ing controller. It allows for seamless power source transition and provides a low-loss...
- AdeZep 2024-02-23 07:14:54 74AC14 willl do the job ? Thanks!...
- ViasionTech 2024-02-13 09:30:44 In the USA and parts of the EU, wooden electric transmission poles are commonly used due to their cost-effectiveness, widespread availability, and environmental friendliness. The decision is influenced by regional engineering standards, emphasizing factors like renewable resources and biodegradability. While wooden poles may have lower load-bearing capacity than those used in Turkey, they meet safety requirements for specific voltage and environmental conditions. To obtain detailed information about electric pole types and standards in a specific country, it is advisable to contact local electric utilities, government agencies, or industry associations for relevant documentation or standards....
Browse by Categories
- Amplifiers (52)
- Analog Design (139)
- Digital Design (63)
- Diodes, Transistors and Thyristors (44)
- General (82)
- Passive Components (45)
- Power Design (90)
- Power Management (44)
- RF Design (102)
- RF and Microwave (50)
- Test & Measurement (37)
- Uncategorized (125)
Browse by Topics
- Amplifiers (58)
- Circuits (32)
- Current (41)
- Electronics (44)
- Transmission Line (25)
- Voltage (39)
- List of Topics
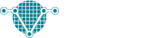
Non Blocking Proceduaral assignments
The non-blocking assignment statement starts its execution by evaluating the RHS operand at the beginning of a time slot and schedules its update to the LHS operand at the end of a time slot. Other Verilog statements can be executed between the evaluation of the RHS operand and the update of the LHS operand. As it does not block other Verilog statement assignments, it is called a non-blocking assignment.
A less than or equal to ‘<=’ is used as a symbol for the non-blocking assignment operator.
- If <= symbol is used in an expression then it is interpreted as a relational operator.
- If <= symbol is used in an assignment then it is interpreted as a non blocking operator.
How race around condition is resolved in a nonblocking assignment?
If a variable is used in LHS of blocking assignment in one procedural block and the same variable is used in RHS of another blocking assignment in another procedural block.
In this example,
Since procedural blocks (both initial and always) can be executed in any order.
In a non-blocking assignment statement no matter what is the order of execution, both RHS of the assignments (y <= data and data <= y) are evaluated at the beginning of the timeslot and LHS operands are updated at the end of a time slot. Thus, race around condition is avoided as there is no dependency on execution order and the order of execution of these two statements can be said to happen parallelly.
Verilog procedural assignment guidelines
For a beginner in Verilog, blocking and non-blocking assignments may create confusion. If are used blindly, it may create race conditions or incorrect synthesizable design. Hence, it is important to understand how to use them. To achieve synthesized RTL correctly, Verilog coding guidelines for blocking and non-blocking assignments are mentioned below
- Use non-blocking assignments for modeling flip flops, latches, and sequential logic.
- Use blocking assignment to implement combinational logic in always block.
- Use non-blocking assignment to implement sequential logic in always block.
- Do not mix blocking and non-blocking assignments in single always block i.e. For the implementation of sequential and combination logic in a single ‘always’ block, use non-blocking assignments.
- Do not assign value to the same variable in the different procedural blocks.
- Use non-blocking assignments while modeling both combination and sequential logic within the same always block.
- Avoid using #0 delay in the assignments.
Verilog Tutorials
[Verilog] 理解 Blocking non-Blocking assignments(一)
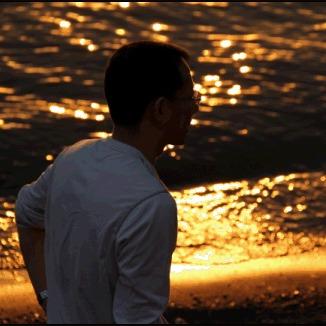
推荐Sutherland的PPT:
Verilog里有连续赋值(Continuous assignment) ,过程赋值(Procedural assignment),还有过程连续赋值(Procedural Continuous assignment)。
过程赋值又有阻塞赋值和非阻塞赋值。
"=" 表示阻塞过程赋值(Blocking Procedural assignment), "<="表示非阻塞过程赋值(Non-blocking Procedural assignment)。
过程赋值的过程可以理解为两个步骤:右式计算和左式赋值。
例如 a = a + 1,先计算"="右边的 a+1,然后将这个值赋给"="左边的a。
=,阻塞赋值,可以认为:
- 过程中的执行流被阻塞,直到赋值完成
- 在同一时间步上的并发语句的计算被阻塞,直到赋值完成
<=,非阻塞赋值,可以理解为:
- 左式赋值延迟到当前同一时间步的其它右式计算都完成以后进行
- 过程中的执行流继续进行,不被阻塞
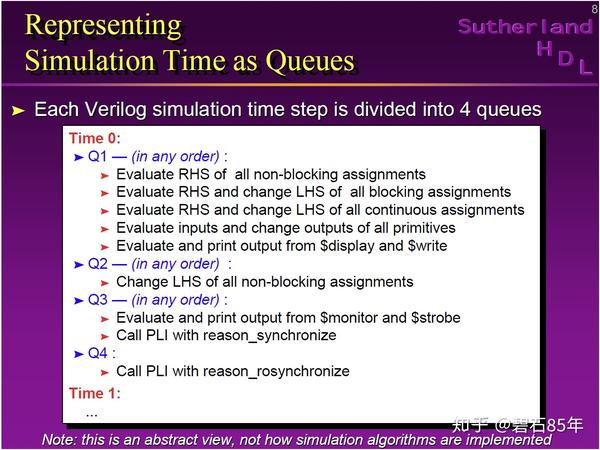
- non-blocking assignment,RHS(right-hand side)计算在Q1,LHS(left-hand side)赋值在Q2
- blocking assignment,计算和赋值在Q1一步完成
3. 连续赋值也是在Q1计算与赋值一步完成
4. $display, $write 计算和打印输出在Q1完成
5. $strobe, $monitor计算和打印输出在Q3完成
这对上面的描述,看下面的例子:
在clk的上升沿,有4个语句:
- 阻塞赋值, blocking = a+1
- 非阻塞赋值, non_blocking <= a+1
这四个语句同时发生。在同一个时间步,发生的过程分析如下:
Q1(按语句先后顺序。见特别申明的变化。)
阻塞赋值一步完成。即:blocking=a+1,计算和赋值一步完成,blocking=2
非阻塞赋值,即:non_blocking<=a+1 的右式计算。a+1计算结果为2(但还未赋给左式!)
$display计算及打印输出,此时结果: blocking为2,non_blocking还保留初始值0
非阻塞赋值语句,给左式赋值,即:non_blocking=2
$strobe计算及打印输出,此时:blocking=2,non_blocking=2
所以,最终看到的运行输出是:
特别申明一下。在Q1里,存在一个竞争:变量blocking的阻塞赋值和$display。由于仿真软件的限制,仿真的结果是按照语句的先后顺序。 但理论上它们同时发生,不保证先后顺序。存在竞争的风险,意味着交换两条语句的前后顺序,会导致不同的结果。
$display在前,阻塞赋值在后,结果如下
但是,变量non_blocking的赋值和$display(或$strobe)不存在这样的竞争,因为$display在Q1,赋值在Q2,$strobe在Q3。交换非阻塞赋值和$display的顺序不影响结果。
404 Not found

Snapsolve any problem by taking a picture. Try it in the Numerade app?
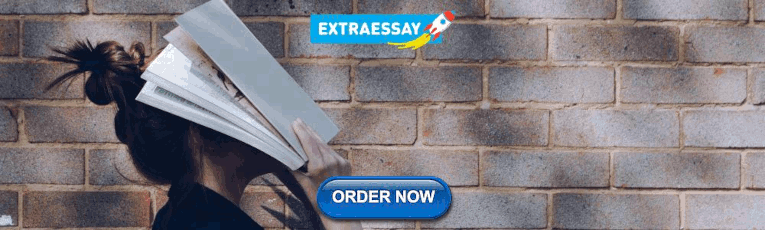
IMAGES
VIDEO
COMMENTS
Non-blocking. Non-blocking assignment allows assignments to be scheduled without blocking the execution of following statements and is specified by a = symbol. It's interesting to note that the same symbol is used as a relational operator in expressions, and as an assignment operator in the context of a non-blocking assignment.
Evaluate b&(~c) but defer assignment of z 1. Evaluate a | b, assign result tox x 2. Evaluate a^b^c, assign result to y 3. Evaluate b&(~c), assign result to zz I. Blocking vs. Nonblocking Assignments • Verilog supports two types of assignments within always blocks, with subtly different behaviors. • Blocking assignment: evaluation and ...
was fairly sure that nonblocking assignments were sequential while blocking assignments were parallel. Blocking assignment executes "in series" because a blocking assignment blocks execution of the next statement until it completes. Therefore the results of the next statement may depend on the first one being completed. Non-blocking assignment ...
Blocking and Non-blocking Assignment in Verilog. When working with behavioural modeling in Verilog, there are two types of assigment which is known as blocking and non blocking assigment and both of them there is a operator, '=' operator for blocking assignment and '=' operator for non blocking assigment.At short, blocking assignment executes one by one sequentially and non-blocking assignemnt ...
The Blocking assignment immediately takes the value in the right-hand-side and assigns it to the left hand side. Here's a good rule of thumb for Verilog: In Verilog, if you want to create sequential logic use a clocked always block with Nonblocking assignments. If you want to create combinational logic use an always block with Blocking ...
Blocking assignments. Blocking assignments (=) are done sequentially in the order the statements are written. A second assignment is not started until the preceding one is complete. i.e, it blocks all the further execution before it itself gets executed. Example: Non-Blocking assignments.
An edge-sensitive intra-assignment timing control permits a special use of the repeat loop. The edge sensitive time control may be repeated several times before the delay is completed. Either the blocking or the non-blocking assignment may be used. always always @(IN) @(IN) OUT OUT <= <= repeat.
Blocking And Nonblocking In Verilog. Blocking Statements: A blocking statement must be executed before the execution of the statements that follow it in a sequential block. In the example below the first time statement to get executed is a = b followed by. Nonblocking Statements: Nonblocking statements allow you to schedule assignments without ...
end. There are now two extra states and an else. The else is needed because two dependent blocking assign-ments happen in the first clock cycle, except when the input is 2. In that case, there is only one assignment (of the input to the output). As discussed earlier, equiva-lent non-blocking code requires an if else.
There are synthesis differences between a blocking statement and a non-blocking statement. Blocking Assignment. The syntax for a blocking assignment is: always @ (pos edge clk) begin. x=y; y=z; end. In this blocking assignment immediately after rising transition of the clk signal, x=y=z.
The verilog simulator treats = and <= quite differently. Blocking assignments mean 'assign the value to the variable right away this instant'. Nonblocking assignments mean 'figure out what to assign to this variable, and store it away to assign at some future time'.
This episode provides a comprehensive analysis of the key differences between blocking and non-blocking assignments in Verilog. The host begins by introducin...
In this Verilog tutorial, we demonstrate the usage of Verilog blocking and nonblocking assignments inside sequential blocks.Complete example from the Verilog...
Blocking vs Non-Blocking Cont • Non-blocking assignments literally do not blockthe execution of the next statements. The right side of all statements are determined first, then the left sides are assigned together. - Consequently, non-blocking assignments result in simultaneous or parallel statement execution. For example: assume a = b = 0 ...
The significance of blocking and non-blocking assignments in Verilog coding cannot be overstated. These elements serve as the foundation for precise and effective digital circuit design, offering ...
Non Blocking Proceduaral assignments. The non-blocking assignment statement starts its execution by evaluating the RHS operand at the beginning of a time slot and schedules its update to the LHS operand at the end of a time slot. Other Verilog statements can be executed between the evaluation of the RHS operand and the update of the LHS operand ...
这一页信息量很多! non-blocking assignment,RHS(right-hand side)计算在Q1,LHS(left-hand side)赋值在Q2; blocking assignment,计算和赋值在Q1一步完成
The Blocking assignment immediately takes this value in the right-hand-side and assignment computer to the left reach side. Here's a good rule of thumb for Verilog: In Verilog, if you want to create sequential logic use a clocked always block with Nonblocking assignments. If to want to build combinational logic use an always block for ...
#verilog #blocking #nonblocking #assignments #verilogDifference between Blocking and Nonblocking assignment statement with example and simulation outputs.0:4...
As far as correctness is concerned, there is no problem with mixing blocking and non-blocking assignments, but you need to have a clear understanding of which signal is sequential and which signal is combinational block (note that the inputs of this combinational block come either from other sequential blocks or primary inputs).
Verilog is an event-driven simulator, which means that it runs simulation till there is any activity in the code (signal values change). There are several sequential event-driven buckets. For this explanation two of them are important: the bucket when blocking assignments are evaluated and a subsequent bucket where non-blocking assignments are ...
Clock I0 Figure Q4(b) (5 marks) (c) Determine the Verilog code for Figure Q4(c) using non-blocking assignment and positive edge-triggered D flip-flop. Q x2 Clock Figure Q4(c) (5 marks) (d) Determine the Verilog code that represents a T (Toggle) flip-flop with an asynchronous reset input.
I have following codes with blocking (code 1) and nonblocking (code 2) assignment in always block. But output is different in both cases. Why? Event queue I know, but probably I am not able to understand where "always @ (clk)" statement will be placed in event queue. // Code 1. module osc2 (clk, d); output clk;