If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
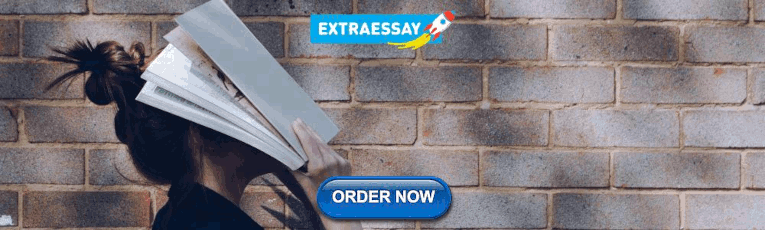
Computer science theory

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
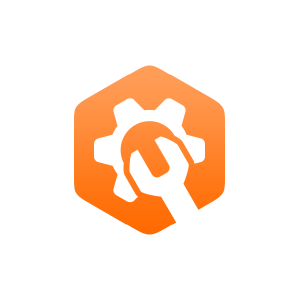
8.4: Graph Representations
- Last updated
- Save as PDF
- Page ID 49318
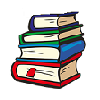
- Wikibooks - Data Structures
Adjacency Matrix Representation
An adjacency matrix is one of the two common ways to represent a graph. The adjacency matrix shows which nodes are adjacent to one another. Two nodes are adjacent if there is an edge connecting them. In the case of a directed graph, if node \(j\) is adjacent to node \(i\), there is an edge from \(i\) to \(j\). In other words, if \(j\) is adjacent to \(i\), you can get from \(i\) to \(j\) by traversing one edge. For a given graph with \(n\) nodes, the adjacency matrix will have dimensions of \(n\times n\). For an unweighted graph, the adjacency matrix will be populated with boolean values.
For any given node \(i\), you can determine its adjacent nodes by looking at row \(\left(i,\left[1..n\right]\right)\) of the adjacency matrix. A value of true at \(\left(i,j\right)\) indicates that there is an edge from node \(i\) to node \(j\), and false indicating no edge. In an undirected graph, the values of \(\left(i,j\right)\) and \(\left(j,i\right)\) will be equal. In a weighted graph, the boolean values will be replaced by the weight of the edge connecting the two nodes, with a special value that indicates the absence of an edge.
The memory use of an adjacency matrix is \(O(n^{2})\).
Adjacency List Representation
The adjacency list is another common representation of a graph. There are many ways to implement this adjacency representation. One way is to have the graph maintain a list of lists, in which the first list is a list of indices corresponding to each node in the graph. Each of these refer to another list that stores the index of each adjacent node to this one. It might also be useful to associate the weight of each link with the adjacent node in this list.
Example: An undirected graph contains four nodes 1, 2, 3 and 4. 1 is linked to 2 and 3. 2 is linked to 3. 3 is linked to 4.
3 - [1, 2, 4]
It might be useful to store the list of all the nodes in the graph in a hash table. The keys then would correspond to the indices of each node and the value would be a reference to the list of adjacent node indices.
Another implementation might require that each node keep a list of its adjacent nodes.
- Dynamic Arrays
- Linked Lists
- Singly Linked Lists
- Doubly Linked Lists
- Queues and Circular Queues
- Tree Data Structure
- Tree Representation
- Binary Trees
- Binary Heaps
- Priority Queues
- Binomial Heaps
- Binary Search Trees
- Self-balancing Binary Search Trees
- 2-3-4 Trees
- Red Black Trees
- Splay Trees
- Randomly Built Binary Search Trees
- Graphs and Graph Terminologies
Graph Representation: Adjacency List and Matrix
Introduction.
In the previous post , we introduced the concept of graphs. In this post, we discuss how to store them inside the computer. There are two popular data structures we use to represent graph: (i) Adjacency List and (ii) Adjacency Matrix. Depending upon the application, we use either adjacency list or adjacency matrix but most of the time people prefer using adjacency list over adjacency matrix.
Adjacency Lists
Adjacency lists are the right data structure for most applications of graphs.
Adjacency lists, in simple words, are the array of linked lists. We create an array of vertices and each entry in the array has a corresponding linked list containing the neighbors. In other words, if a vertex 1 has neighbors 2, 3, 4, the array position corresponding the vertex 1 has a linked list of 2, 3, and 4. We can use other data structures besides a linked list to store neighbors. I personally prefer to use a hash table and I am using the hash table in my implementation. You can also use balanced binary search trees as well. To store the adjacency list, we need $O(V + E)$ space as we need to store every vertex and their neighbors (edges).
To find if a vertex has a neighbor, we need to go through the linked list of the vertex. This requires $O(1 + deg(V))$ time. If we use balanced binary search trees, it becomes $O(1 + \log(deg(V))$ and using appropriately constructed hash tables, the running time lowers to $O(1)$.
Figure 1 shows the linked list representation of a directed graph.
In an undirected graph, to store an edge between vertices $A$ and $B$, we need to store $B$ in $A$’s linked list and vice versa. Figure 2 depicts this.
Adjacency Matrices
An adjacency matrix is a $V \times V$ array. It is obvious that it requires $O(V^2)$ space regardless of a number of edges. The entry in the matrix will be either 0 or 1. If there is an edge between vertices $A$ and $B$, we set the value of the corresponding cell to 1 otherwise we simply put 0. Adjacency matrices are a good choice when the graph is dense since we need $O(V^2)$ space anyway. We can easily find whether two vertices are neighbors by simply looking at the matrix. This can be done in $O(1)$ time. Figure 1 and 2 show the adjacency matrix representation of a directed and undirected graph.
Representing Weighted Graphs
We can modify the previous adjacency lists and adjacency matrices to store the weights. In the adjacency list, instead of storing the only vertex, we can store a pair of numbers one vertex and other the weight. Similarly, in the adjacency matrix, instead of just storing 1 we can store the actual weight. Figure 3 illustrates this.
The table below summarizes the operations and their running time in adjacency list and adjacency matrix.
Implementation
Since I will be doing all the graph related problem using adjacency list, I present here the implementation of adjacency list only. You can find the codes in C++, Java, and Python below.
- Cormen, T. H., Leiserson, C. E., Rivest, R. L., & Stein, C. (n.d.). Introduction to algorithms (3rd ed.). The MIT Press.
- Jeff Erickson. Algorithms (Prepublication draft). http://algorithms.wtf
- Steven S. Skiena. 2008. The Algorithm Design Manual (2nd ed.). Springer Publishing Company, Incorporated.

Adjacency List
Explore with wolfram|alpha.

More things to try:
- Apollonian network
- graph properties
Referenced on Wolfram|Alpha
Cite this as:.
Weisstein, Eric W. "Adjacency List." From MathWorld --A Wolfram Web Resource. https://mathworld.wolfram.com/AdjacencyList.html
Subject classifications
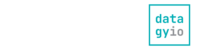
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Representing Graphs in Python (Adjacency List and Matrix)
- January 15, 2024 December 31, 2023
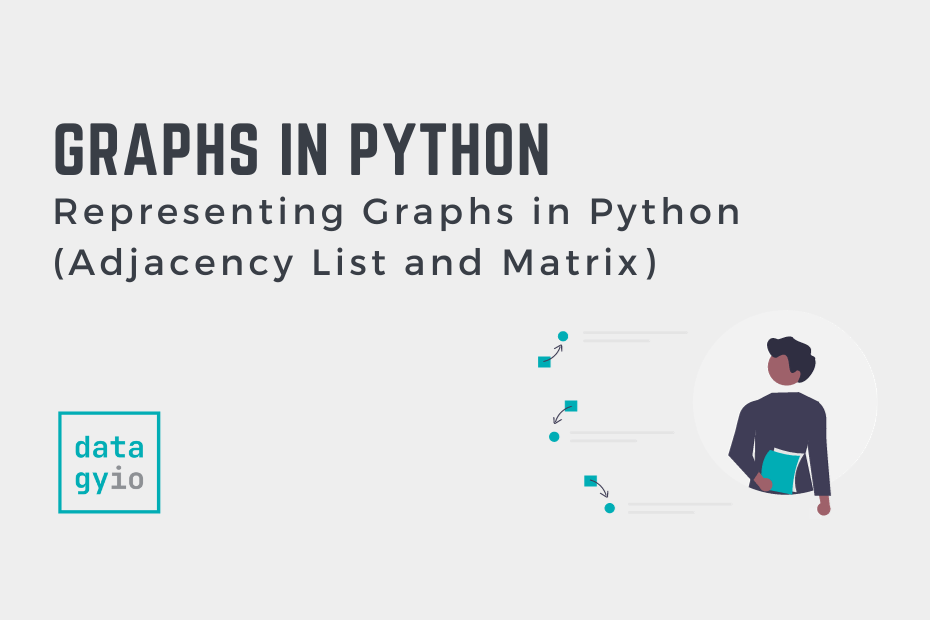
In this tutorial, you’ll learn how to represent graphs in Python using edge lists, an adjacency matrix, and adjacency lists . While graphs can often be an intimidating data structure to learn about, they are crucial for modeling information. Graphs allow you to understand and model complex relationships, such as those in LinkedIn and Twitter (X) social networks.
By the end of this tutorial, you’ll have learned the following:
- What graphs are and what their components, nodes, and edges, are
- What undirected, directed, and weighted graphs are
- How to represent graphs in Python using edge lists, adjacency lists, and adjacency matrices
- How to convert between graph representations in Python
Table of Contents
What are Graphs, Nodes, and Edges?
In its simplest explanation, a graph is a group of dots connected by lines. Each of these dots represents things, such as people or places. The lines are used to represent the connections between things. From a technical perspective, these circles are referred to as nodes . Similarly, the lines connecting them are called edges .
For example, we could create a graph of some Facebook relationships. Let’s imagine that we have three friends: Evan, Nik, and Katie. Each of them are friends with one another. We can represent this as a graph as shown below:
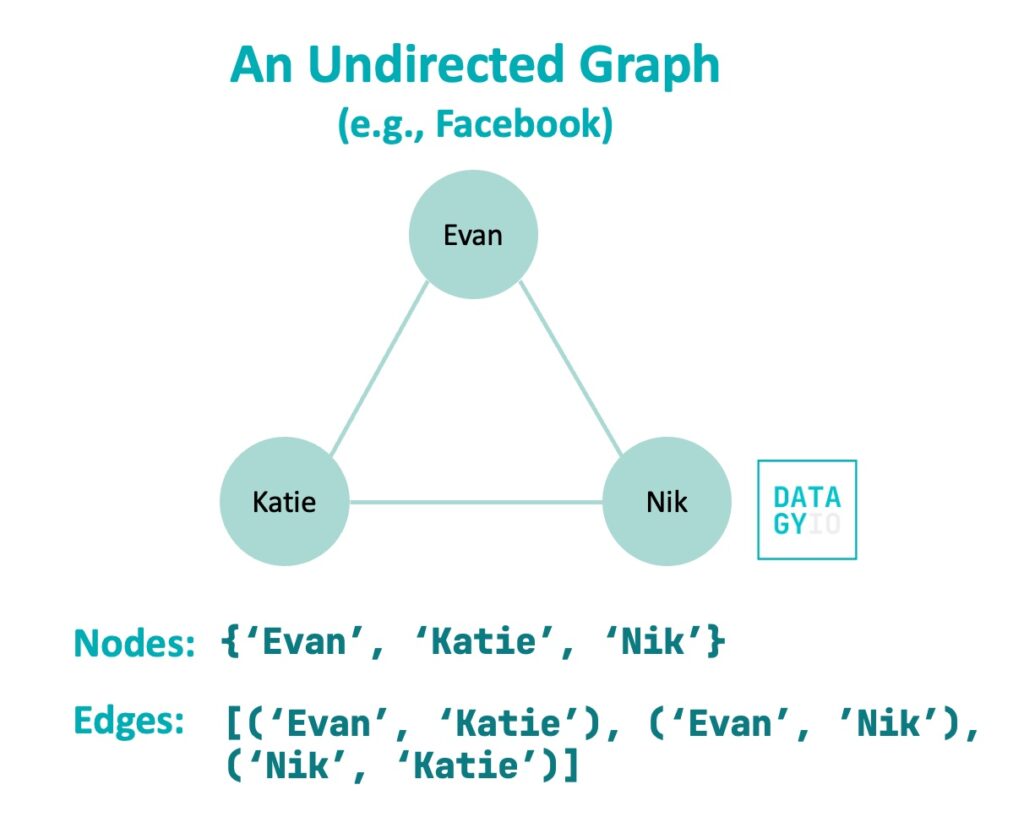
This is an example of an undirected graph . This means that the relationship between two nodes occurs in both directions. Because of this, a sample edge may connect Evan and Katie. This edge would be represented by {'Evan', 'Katie'} . Note that we used a set to represent this. In this case, the relationship goes both ways.
Now let’s take a look at another example. Imagine that we’re modeling relationships in X (formerly Twitter). Because someone can follow someone without the other person following them back, we can end up with a relationship like the one shown below.
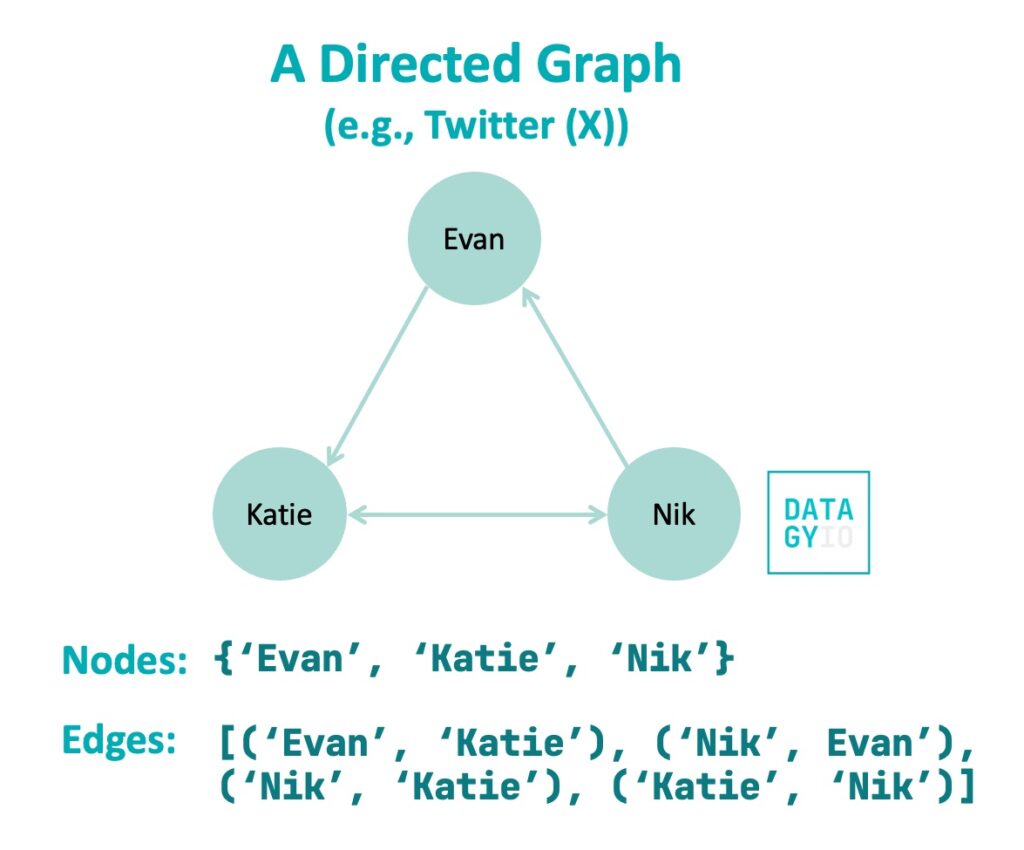
This is an example of a directed graph . Notice that the edges here are represented as arrows. In this case, Nik and Katie follow one another, but the same isn’t true for the others. Evan follows Katie (but not the other way around). Similarly, Nik follows Evan, but not the other way around.
In this case, while our nodes remain the same as {'Nik', 'Evan', 'Katie'} , our edge list now has a different meaning. In fact, it’s easier to use a list of tuples to represent this now, where the first value is the starting node and the second is the end node. This would look like this: [('Nik', 'Katie'), ('Katie', 'Nik'), ('Evan', 'Katie'), ('Nik', 'Evan')] .
Let’s now dive a little further into the different types of graphs and how they can be represented in Python.
Want to learn how to traverse these graphs? Check out my in-depth guides on breadth-first search in Python and depth-first search in Python .
Understanding Graph Data Structure Representations
In this tutorial, we’ll explore three of the most fundamental ways in which to represent graphs in Python. We’ll also explore the benefits and drawbacks of each of these representations. Later, we’ll dive into how to implement these for different types of graphs and how to create these in Python.
One of the ways you have already seen is called an edge list . The edge list lists our each of the node-pair relationships in a graph. The list can include additional information, such as weights, as you’ll see later on in this tutorial.
In Python, edge lists are often represented as lists of tuples . Let’s take a look at an example:
In the code block above, we can see a sample edge list. In this case, each pair represents an unweighted relationship (since no weight is given) between two nodes. In many cases, edge lists for undirected graphs omit the reverse pair (so ('A', 'B') represents both sides of the relationship, for example).
Another common graph representation is the adjacency matrix . Adjacency matrices are often represented as lists of lists, where each list represents a node. The list’s items represent whether an edge exists between the node and another node.
Let’s take a look at what this may look like:
In the example above, we can see all the node’s connections. For example, node 'A' comes first. We can see that it has an edge only with node 'B' . This is represented by 1, where all other nodes are 0.
One of the key benefits of an adjacency matrix over an edge list is that we can immediately see any node’s neighbors, without needing to iterate over the list.
However, adjacency matrices are often very sparse, especially for graphs with few edges. Because of this, the preferred method is the adjacency list.
An adjacency list is a hash map that maps each node to a list of neighbors. This combines the benefits of both the edge list and the adjacency matrix, by providing a contained structure that allows you to easily see a node’s neighbors.
In Python, adjacency lists are often represented as dictionaries . Each key is the node label and the value is either a set or a list of neighbors. Let’s take a look at an example:
Let’s quickly summarize the three main structures for different graph types:
Now that you have a good understanding of how graphs can be represented, let’s dive into some more practical examples by exploring different graph types.
Understanding Undirected Graph Data Structures
As we saw earlier, an undirected graph has edges that connect nodes without specifying direction, because the edges are bidirectional. In the edge list representation an edge connecting ('A', 'B') is the same as that connecting ('B', 'A') .
Let’s take a look at a more complete example of what this can look like. We’ll use nodes labeled A through F for this and look at different ways in which this graph can be represented.
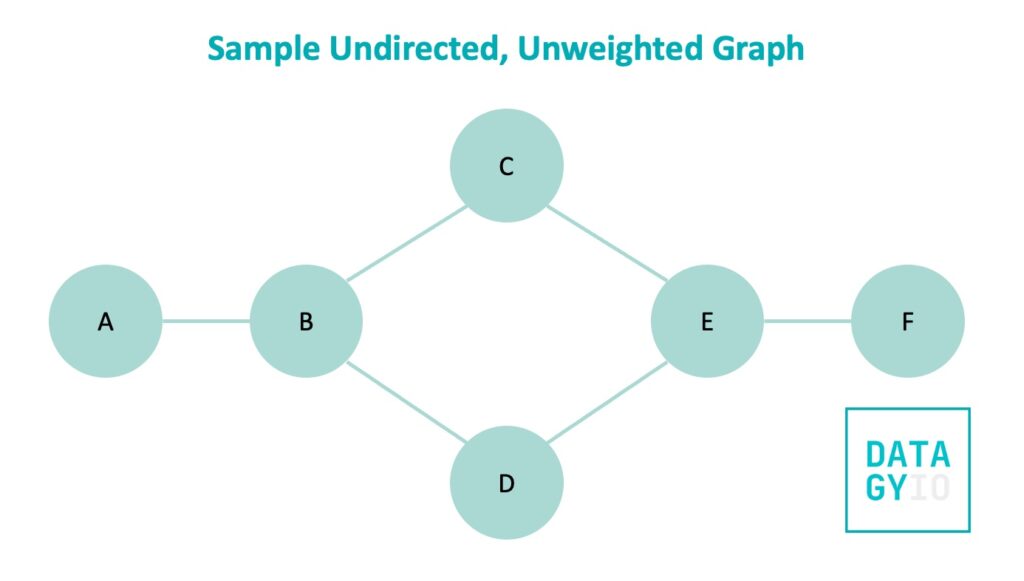
We can see that in the image above all nodes are connected by lines without heads. This indicates that the graph is undirected and that the relationship is bidirectional. As an edge list, this graph would be represented as shown below:
In this case, it’s important to note that the graph is undirected. Without this knowledge, half the relationships would be lost. Because of this, it can sometimes be helpful to be more explicit and list out all possible relationships, as shown below:
In the code block above, we’re repeating each relationship for each node-pair relationship. While this is more explicit, it’s also redundant.
One problem with edge lists is that if we want to see any node’s neighbors, we have to iterate over the entire list. Because of this, there are different representations that we can use.
Adjacency Matrix for Undirected, Unweighted Graphs
Another common way to represent graphs is to use an adjacency matrix . In Python, these are lists of lists that store the corresponding relationship for each node. For example, our graph has seven nodes. Because of this, the adjacency matrix will have seven lists, each with seven values.
Take a look at the image below to see what our adjacency matrix for our undirected, unweighted graph looks like:
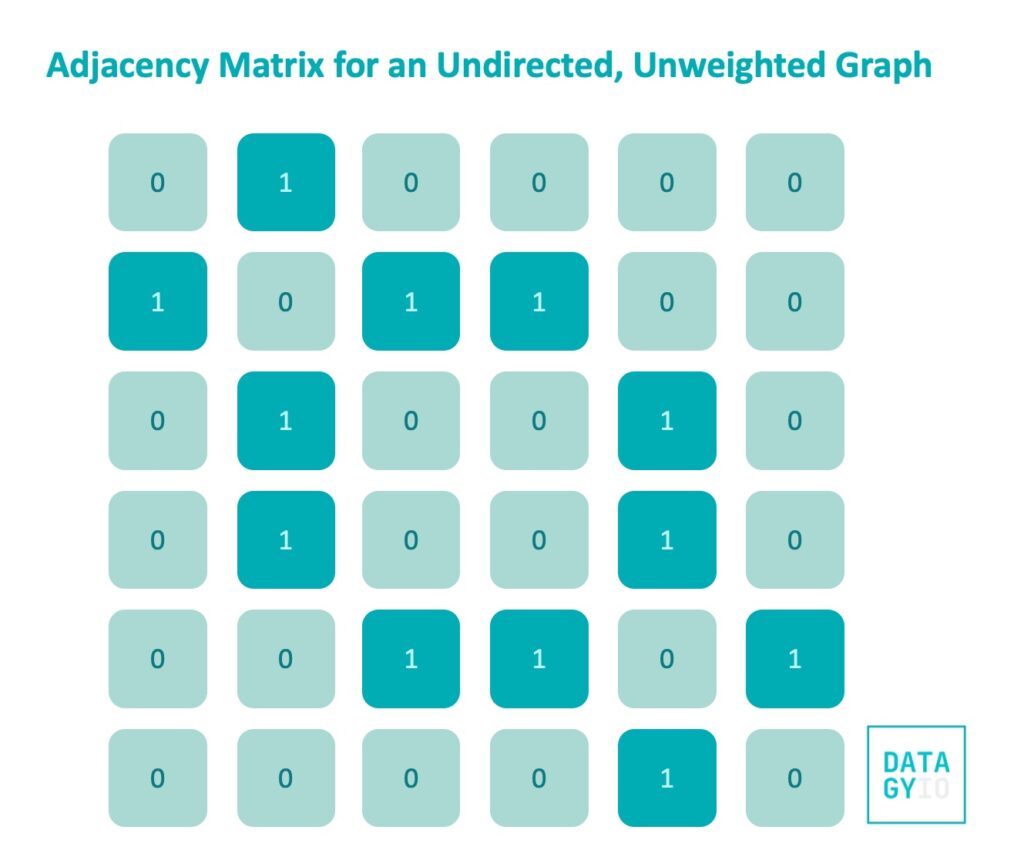
In the image above, we can see our matrix of seven rows and seven values each. The first row shows all the relationships that node 'A' has. We can see that it only connects to the second node, 'B' . In this case, edges are labelled at 1 and non-existent edges are labelled as 0.
One unique property of an adjacency matrix for undirected graphs is that they are symmetrical! If we look along the diagonal, we can see that the values are mirrored.
Let’s take a look at how we can write a function to accept an edge list and return an adjacency matrix in Python:
In the code block above, we created a function that creates an adjacency matrix out of an edge list. The function creates a set out of our nodes and then sorts that set. We then create a matrix that creates a list of lists of 0s for each node in the graph.
The function then iterates over the edges and finds the indices for each node. It adds 1 for each edge it finds. By default, the function assumes an undirected graph; if this is the case, it also adds a 1 for each reverse pair.
When we run the function on a sample edge list, we get the following code back:
Now let’s take a look at adjacency lists for undirected, unweighted graphs.
Adjacency List for Undirected, Unweighted Graphs
For graphs that don’t have many edges, adjacency matrices can become very sparse. Because of this, we can use one of the most common graph representations, the adjacency list . The adjacency list combines the benefits of both the edge list and the adjacency matrix by creating a hash map of nodes and their neighbors.
Let’s take a look at what an adjacency list would look like for an undirected, unweighted graph:
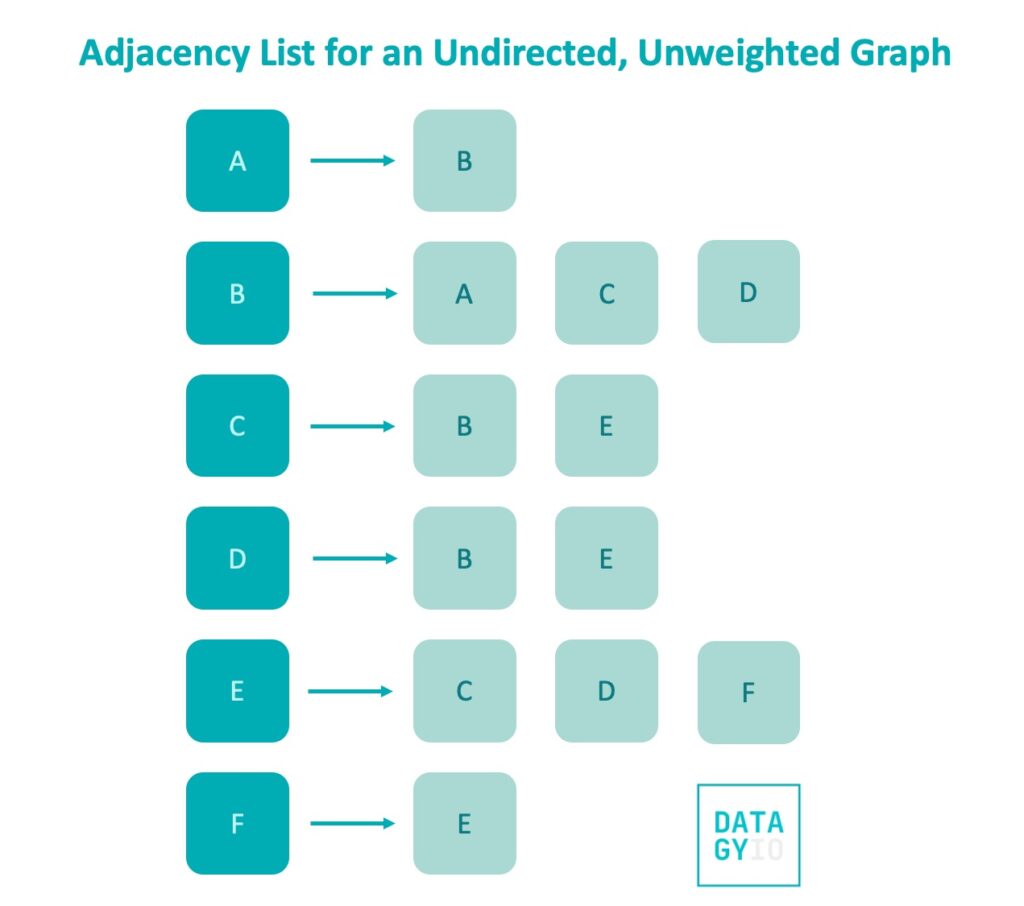
In Python, we would represent this using a dictionary. Let’s take a look at how we can create a function that accepts an edge list and returns an adjacency list:
This function is a bit simpler than our previous function. We accept both an edge list and whether our graph is directed or not. We then create a dictionary to hold our adjacency list. The iterate over each edge in our list. We check if the starting node exists in our dictionary – if it doesn’t we create it with an empty list. We then append the second node to that list.
Similarly, if the graph is not directed, we do the same thing for the inverse relationship.
Let’s take a look at what this looks like for a sample edge list:
Now that you have a strong understanding of the three representations of unweighted, undirected graphs, let’s take a look at directed graphs in Python.
Understanding Directed Graph Data Structures
Directed graphs, also known as digraphs, are networks where edges between nodes have a defined direction. Unlike undirected graphs, edges in directed graphs are unidirectional, representing a directed relationship from one node (the source) to another node (the target or destination).
Directed graphs can be helpful when modeling one-way relationships, such as one-way streets, task dependencies in project management, or social media followings.
Let’s take a look at what one of these graphs may look like:
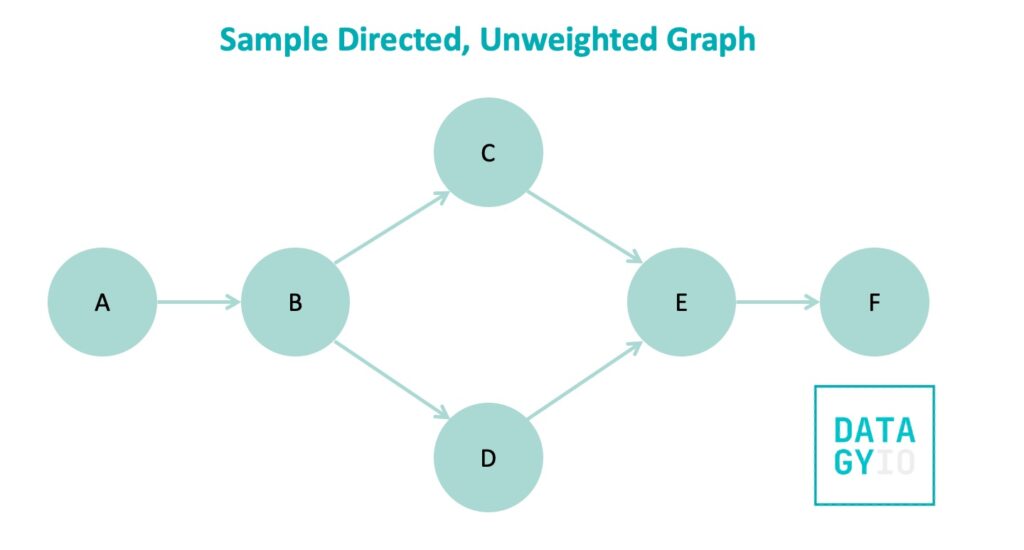
We can see that this graph looks very similar to the one that we saw before. However, each edge has a direction on it. In this example, each edge is one-directional. However, directed graphs can also have bi-directional edges.
In this case, we can use an adjacency matrix to represent this graph as well. This is shown in the image below:
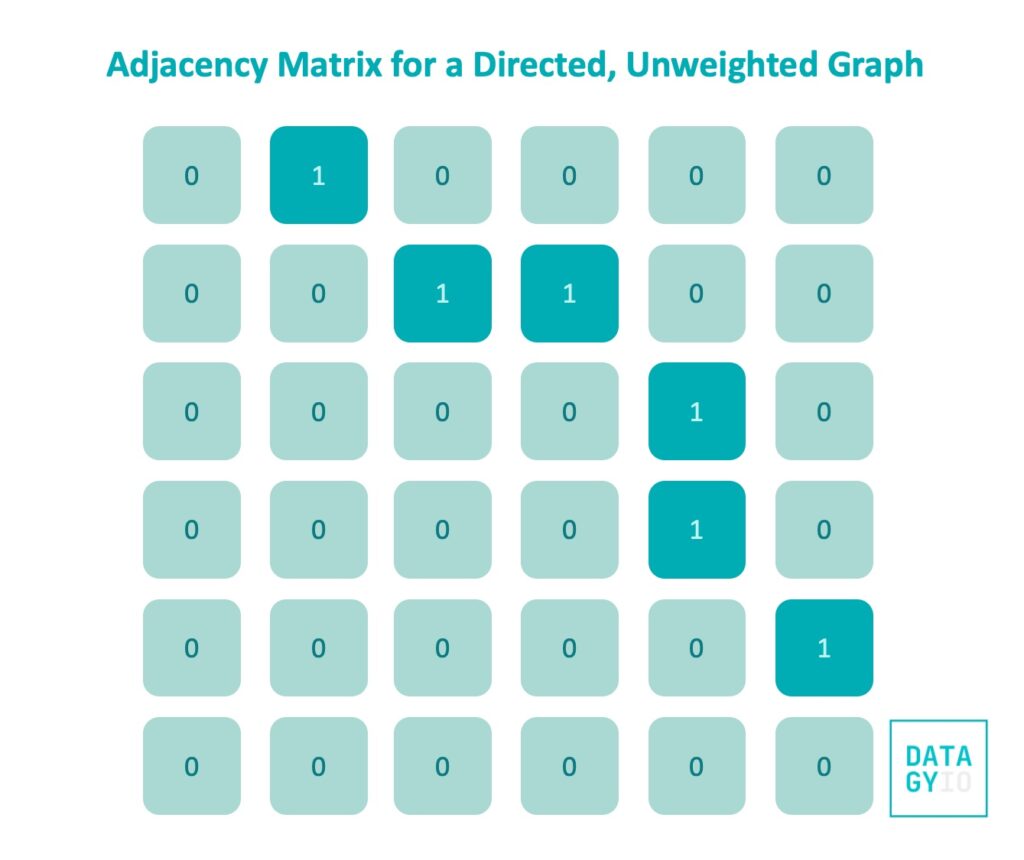
The function that we developed earlier is already built around the concept of directed graphs. In order to use it, we simply need to indicate that the graph is directed. Let’s take a look at what this looks like:
The only change that we made was in calling the function: we modified the default argument of directed=False to True . This meant that the bi-directional pair was not added to our matrix.
Similarly, we can use adjacency lists to represent directed graphs. Let’s take a look at what this would look like:
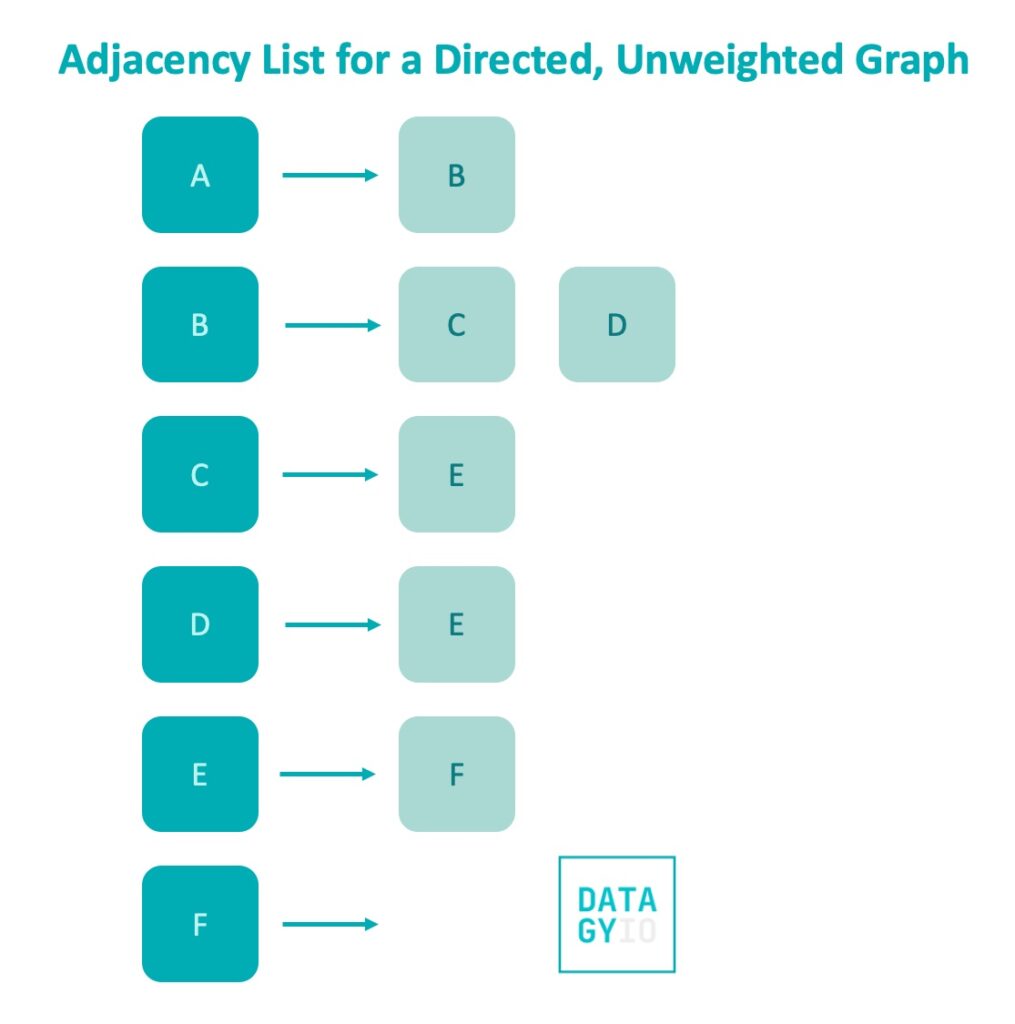
In the image above, each dictionary key is still represented by the starting node and the list of values is represented by its neighbors.
Similarly, the function we previously created allows us to pass in that we’re working with a directed graph. Let’s see what this looks like:
Similar to our previous example, we only modified the default argument of directed= to True . This allowed us to create an adjacency list for a directed graph.
Now that we’ve covered unweighted graphs, let’s dive into weighted graphs, which add additional information to our graph.
Understanding Weighted Graph Data Structures
Weighted directed graphs expand upon directed graphs by incorporating edge weights, assigning a value or cost to each directed edge between nodes. In this graph type, edges not only depict directional relationships but also carry additional information in the form of weights, indicating the strength, distance, cost, or any other relevant metric associated with the connection from one node to another.
These weights add an extra layer of complexity and significance, allowing the representation of various scenarios where the intensity or cost of relationships matters. Weighted directed graphs find applications in diverse fields such as logistics, transportation networks, telecommunications, and biology, where the weights might represent distances between locations, communication costs, strengths of interactions, or genetic similarities.
Let’s use our previous example graph and add some weights to it:
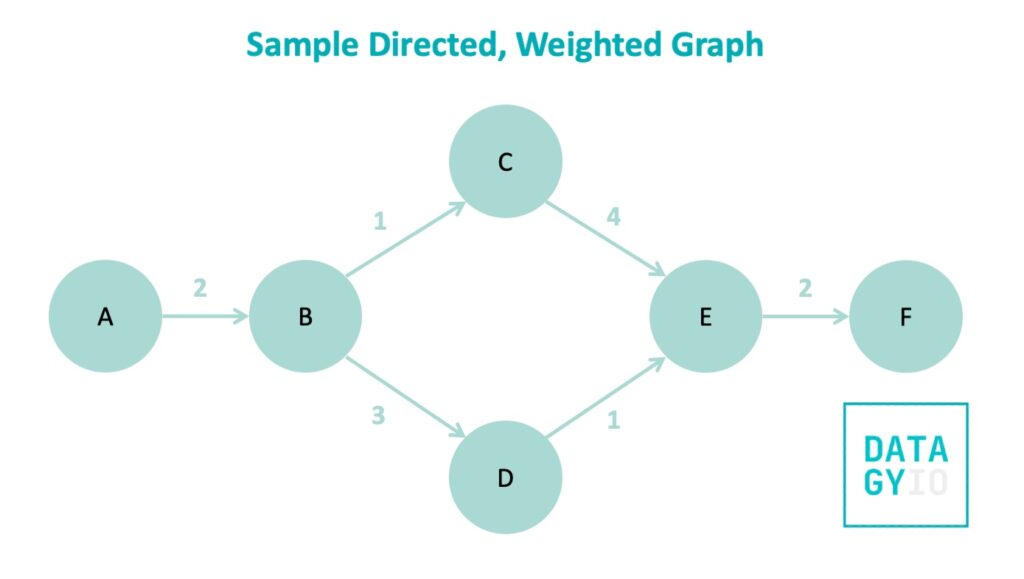
We can represent these graphs as adjacency matrices as well. In this case, rather than using the default value of 1, we represent each node by the weight that it has. Take a look at the image below for how this representation works:

To convert an edge list to an adjacency matrix using Python, we only have to make a small adjustment to our function. Let’s take a look at what this looks like:
In this case, we added a second optional parameter that indicates whether a graph is weighted or not. We use the ternary operator to assign the weight if the graph is weighted, otherwise use the default value of 1.
In an adjacency list, the keys continue to represent the nodes of the graph. The values are now lists of tuples that contain the end node and the weight for that relationship. Take a look at the image below to see what this looks like:

We can make small modifications to our earlier function to allow for weighted graphs. Let’s see what this looks like:
In the code block above, we modified our function to accept a third argument, indicating whether the graph is weighted or not. We then added a number of if-else statements to get the weight from our edge list if the edge list has weights in it.
We can see that the function returns a dictionary that contains lists of tuples, which represent the end node and the weight of the relationship.
Understanding how to represent graphs in Python is essential for anyone working with complex relationships and networks. In this tutorial, we explored three fundamental ways to represent graphs: edge lists, adjacency matrices, and adjacency lists. Each method has its strengths and trade-offs, catering to different scenarios and preferences.
We began by dissecting what graphs, nodes, and edges are, showcasing examples of undirected and directed graphs. From there, we delved into the details of each representation method:
- Edge Lists: Simple and intuitive, representing relationships between nodes as tuples.
- Adjacency Matrices: Efficient for determining connections between nodes but can become sparse in large, sparse graphs.
- Adjacency Lists: Efficient for sparse graphs, offering quick access to a node’s neighbors.
We explored these representations for different graph types, including undirected, directed, and weighted graphs. For each type, we demonstrated how to convert between representations using Python functions.
Understanding these representations equips you to handle diverse scenarios, from modeling social networks to logistical networks, project management, and more. Graphs are a powerful tool for modeling relationships, and grasping their representations in Python empowers you to analyze and work with complex interconnected data effectively.
The official Python documentation also has useful information on building graphs in Python .
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Introduction to Adjacency List for Graph self.__wrap_b=(t,n,e)=>{e=e||document.querySelector(`[data-br="${t}"]`);let a=e.parentElement,r=R=>e.style.maxWidth=R+"px";e.style.maxWidth="";let o=a.clientWidth,c=a.clientHeight,i=o/2-.25,l=o+.5,u;if(o){for(;i+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(a):process.env.NODE_ENV==="development"&&console.warn("The browser you are using does not support the ResizeObserver API. Please consider add polyfill for this API to avoid potential layout shifts or upgrade your browser. Read more: https://github.com/shuding/react-wrap-balancer#browser-support-information"))};self.__wrap_b(":R4mr36:",1)
Introduction
What is an adjacency list.
- Features of an Adjacency List
Advantages of an Adjacency List
Drawbacks of an adjacency lists, graph representing adjacency list, example of an adjacency list, implementation of an adjacency list.

Graphs are an important data structure in computer science and are widely used to represent real-world relationships between objects. In this blog, we will be introducing a common way of representing graphs, known as an adjacency list.
An Adjacency list is a collection of nodes that have an edge over each other. The advantage of this list over a regular graph is that you can edit or remove nodes from it easily. You can also add additional edges between two nodes if needed by using the add_edge method on your list object or calling another method (such as add_node() ).
It is a list that can be used to represent a graph. It is implemented as an array, with each element of the array representing a vertex in the graph. The elements of the array store the vertices that are adjacent to the vertex represented by the element. Each item contains two pieces of information:
- The first element of the array represents the number of neighbours (if any) surrounding it. While the second element holds its location in space, i.e., left/right/top/bottom etc.
- You may have noticed that there are some similarities between an adjacency list and a directed graph, where nodes connect only if they share an edge or have some kind of relationship with each other. However, unlike directed graphs where edges are always identified by numbers instead of text labels like A-B or 1->2->3->4->5. In contrast, undirected graphs don’t require such identification labels. Because all paths between two points are available simultaneously, without having any restrictions on how many paths could exist between them. Therefore, no restriction on how many paths exist.
Features of an Adjacency List
Adjacency lists are a data structure that stores the relationship between vertices in a graph. The nodes in an adjacency list are referred to as vertices, and their neighbours are stored at the same level of abstraction (e.g., two adjacent vertices). They also allow you to add new edges or remove existing ones easily by simply adding or removing items from your list respectively.
Adjacent pairs are stored in sorted order so that you can easily find them when needed. This makes it easy for us to perform operations such as finding all paths between two vertices using their indices within these structures.
- An adjacency list only stores the edges of a graph, not the vertices, making it a space-efficient representation of a graph.
- It is also simple to implement and easy to modify.
- An adjacency list can be easily modified to store additional information about the edges in the graph, such as weights or labels.
It is a data structure that stores the set of all vertices that are adjacent in a graph. It has several advantages over other graph structures such as adjacency lists being more efficient in terms of storage and access.
Adjacency lists can also be used to store and retrieve data. In addition, they can be used to implement algorithms on graphs. Like the shortest path or topological ordering which requires numeric values (the distance between two vertices).
- Adjacency lists are one of the most common graphs. They have an O(n) time complexity, and they use memory to store their nodes.
- The size of an adjacency list is also very high. This means that if you want to store large amounts of data on your adjacency list. It will be slow because of the amount of memory used by each node in the graph.
- It is not efficient for finding the edges connecting a particular vertex. As we have to traverse the entire linked list to find the desired edge.
- Another disadvantage is that it is not easy to find the degree of a vertex in an adjacency list. As we have to count the number of edges in the corresponding linked list.
A graph is a collection of nodes and edges. Nodes are connected by edges, which can be directed or undirected. An edge is a connection between two nodes, where one node is called the source and the other one is called the target. Edges are often represented as lines on paper or screens, but they can also be visualized as arrows in space (e.g., in Google Earth).
An important concept to remember about graphs is that we can add more than one edge between two given vertices. Like this:
This means we have multiple paths connecting two vertices together. Also note that there may be multiple paths connecting two vertices if they share some common property (e.g., location).
Below is an example of a graph represented using an adjacency list:

Vertex 0: [1, 2]
Vertex 1: [0, 2]
The Vertex 2: [0, 1, 3]
And Vertex 3: [2]
In this example, the adjacency list for vertex 0 is [1, 2], which means that vertex 0 is connected to vertices 1 and 2. Similarly, the adjacency list for vertex 1 is [0, 2], which means that vertex 1 is connected to vertices 0 and 2.
Consider the following graph:

To represent this graph as list:
and Vertex 2: [1]
This can be implemented in several ways, such as using an array of linked lists or an array of sets. Below is an example of its implementation using an array of linked lists in Python:
The above code creates a graph with 4 vertices and 4 edges and adds the edges to the adjacency list using the add_edge function. The print_graph function is then used to print the adjacency list. The output of this code will be:
In summary, These lists are a simple and space-efficient way of representing a graph and are easy to modify and implement. However, it has some drawbacks, such as inefficiency in finding the edges of a particular vertex and difficulty in finding the degree of a vertex. It is important to choose the appropriate representation of a graph depending on the specific needs of the application.
What is an adjacency list example?
It is a data structure that is used to represent a finite graph. It is a list of lists, where each list represents a vertex in the graph and contains the vertices that are adjacent to it.
Such as, consider the following graph:

How do you represent an adjacency list?
Representation of the adjacency list shown in the above graph would be:
What is an adjacency list in C++?
To represent this in C++ , you can use an array of linked lists.
Is an adjacency list an array?
No, It is a list of lists, not an array. It is an efficient representation of a graph when the graph is sparse. That is when it has a small number of edges compared to the number of vertices.
What are the advantages and disadvantages of an adjacency list?
There are several advantages of using it, to represent a graph. First, it is easy to implement and requires only a small amount of memory. Second, it allows for efficient insertion and deletion of edges. Finally, it can be used to represent both directed and undirected graphs.
There are also some disadvantages to using it. First, it can be slower than other representations. Such as an adjacency matrix, when it comes to querying the graph. Second, it is not well-suited for dense graphs, that is, graphs with a large number of edges. Finally, it can be more difficult to implement some graph algorithms using an adjacency list compared to other representations.
Sharing is caring
Did you like what Srishti Kumari wrote? Thank them for their work by sharing it on social media.
No comment s so far
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, dsa introduction.
- What is an algorithm?
- Data Structure and Types
- Why learn DSA?
- Asymptotic Notations
- Master Theorem
- Divide and Conquer Algorithm
Data Structures (I)
- Types of Queue
- Circular Queue
- Priority Queue
Data Structures (II)
- Linked List
- Linked List Operations
- Types of Linked List
- Heap Data Structure
- Fibonacci Heap
- Decrease Key and Delete Node Operations on a Fibonacci Heap
Tree based DSA (I)
- Tree Data Structure
- Tree Traversal
- Binary Tree
- Full Binary Tree
- Perfect Binary Tree
- Complete Binary Tree
- Balanced Binary Tree
- Binary Search Tree
Tree based DSA (II)
- Insertion in a B-tree
- Deletion from a B-tree
- Insertion on a B+ Tree
- Deletion from a B+ Tree
- Red-Black Tree
- Red-Black Tree Insertion
- Red-Black Tree Deletion
Graph based DSA
- Graph Data Structure
- Spanning Tree
- Strongly Connected Components
- Adjacency Matrix
- Adjacency List
- DFS Algorithm
- Breadth-first Search
- Bellman Ford's Algorithm
Sorting and Searching Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Counting Sort
- Bucket Sort
- Linear Search
- Binary Search
Greedy Algorithms
- Greedy Algorithm
- Ford-Fulkerson Algorithm
- Dijkstra's Algorithm
- Kruskal's Algorithm
- Prim's Algorithm
- Huffman Coding
- Dynamic Programming
- Floyd-Warshall Algorithm
- Longest Common Sequence
Other Algorithms
- Backtracking Algorithm
- Rabin-Karp Algorithm
DSA Tutorials
Types of Linked List - Singly linked, doubly linked and circular
Linked List Operations: Traverse, Insert and Delete
Circular Linked List
Doubly Linked List
- Tree Traversal - inorder, preorder and postorder
Linked list Data Structure
A linked list is a linear data structure that includes a series of connected nodes. Here, each node stores the data and the address of the next node. For example,

You have to start somewhere, so we give the address of the first node a special name called HEAD . Also, the last node in the linked list can be identified because its next portion points to NULL .
Linked lists can be of multiple types: singly , doubly , and circular linked list . In this article, we will focus on the singly linked list . To learn about other types, visit Types of Linked List .
Note: You might have played the game Treasure Hunt, where each clue includes the information about the next clue. That is how the linked list operates.
Representation of Linked List
Let's see how each node of the linked list is represented. Each node consists:
- A data item
- An address of another node
We wrap both the data item and the next node reference in a struct as:
Understanding the structure of a linked list node is the key to having a grasp on it.
Each struct node has a data item and a pointer to another struct node. Let us create a simple Linked List with three items to understand how this works.
If you didn't understand any of the lines above, all you need is a refresher on pointers and structs .
In just a few steps, we have created a simple linked list with three nodes.

The power of a linked list comes from the ability to break the chain and rejoin it. E.g. if you wanted to put an element 4 between 1 and 2, the steps would be:
- Create a new struct node and allocate memory to it.
- Add its data value as 4
- Point its next pointer to the struct node containing 2 as the data value
- Change the next pointer of "1" to the node we just created.
Doing something similar in an array would have required shifting the positions of all the subsequent elements.
In python and Java, the linked list can be implemented using classes as shown in the codes below .
- Linked List Utility
Lists are one of the most popular and efficient data structures, with implementation in every programming language like C, C++, Python, Java, and C#.
Apart from that, linked lists are a great way to learn how pointers work. By practicing how to manipulate linked lists, you can prepare yourself to learn more advanced data structures like graphs and trees.
Linked List Implementations in Python, Java, C, and C++ Examples
- Linked List Complexity
Time Complexity
Space Complexity: O(n)
Linked List Applications
- Dynamic memory allocation
- Implemented in stack and queue
- In undo functionality of softwares
- Hash tables, Graphs
Recommended Readings
1. tutorials.
- Linked List Operations (Traverse, Insert, Delete)
- Java LinkedList
2. Examples
- Get the middle element of Linked List in a single iteration
- Convert the Linked List into an Array and vice versa
- Detect loop in a Linked List
Table of Contents
- Introduction
- Linked List Representation
- How Next Node is Referenced?
- Python, Java and C/C++ Examples
- Linked List Application
Sorry about that.
Related Tutorials
DS & Algorithms
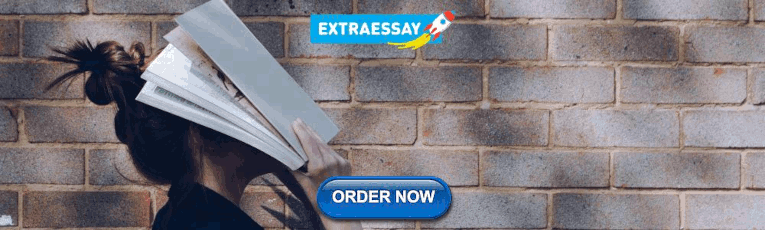
What’s the difference between open and closed list proportional representation?
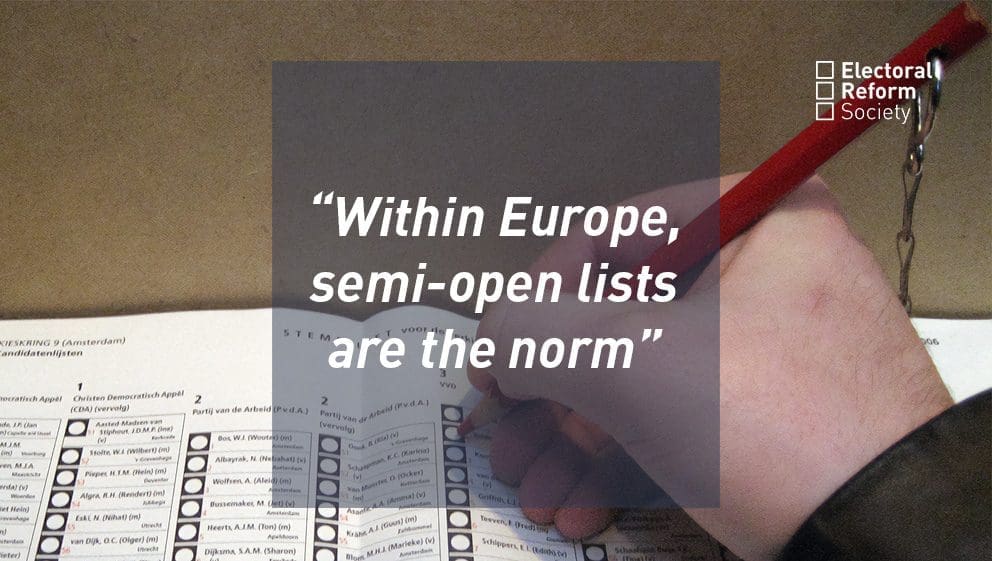
Posted on the 15th November 2021
When discussing proportional voting systems, or voting systems in general, we usually talk about how they allocate seats to political parties. But parliaments are more than parties and it also matters how they decide which individuals are elected and get to become MPs. This is a particularly crucial question with Party List PR systems where there is a clear divide between open and closed systems.
In a List PR election, voters vote for a particular party and its attached list of candidates – usually ordered according to the party’s preferred order of election.
Closed list proportional representation
Under closed list systems, candidates are elected according to their pre-stated position on this list – if a party wins six seats, the first six candidates on that list take the seats. A vote for a particular party is read as an endorsement of their list.
Open list proportional representation
Open list systems, on the other hand, allow voters to cast votes for individual candidates on one party’s list. The exact rules of open lists vary from system to system, but they can usually be classified as either fully- or semi-open. Under fully-open list systems, control over who is elected is entirely in the hands of the voters – the candidates with the most individual votes are elected. But under semi-open systems, candidates are only elected if they cross a set threshold to overrule the party’s ordering. Any remaining seats are awarded as per a closed list election.
Panachage systems
‘Panachage’ systems are also sometimes included alongside open list systems but are really sort of distinct from other Party List PR systems in that voters are given multiple votes for candidates which they can use across party lines.
Party list systems in Europe
Closed lists can be found in a few southern European countries, such as Spain and Portugal, and were previously used to elect British MEPs between 1999 and 2019. But closed lists are more normally found in Party List systems outside of Europe and as part of mixed-member systems, such as those used in Germany , Scotland and Wales . And, despite closed lists being a regular source of criticism of First Past the Post supporters, FPTP itself is effectively a closed list of one – with voters having to accept the candidate the party they support puts forward.
Noted psephologist Professor John Curtice joined us at Conservative Party Conference in 2017 to answer questions about Proportional Representation.
Professor Curtice gets to the bottom of the idea that proportional representation means parties get to pick who gets elected.
Proportional systems like the Single Transferable Vote don't have lists of candidates picked by parties and, conceptually, First Past the Post is a party list of one.
Within Europe, however, semi-open lists are the norm – though the exact thresholds vary from the fairly open systems of Sweden and the Netherlands to the practically closed system of Norway . Fully-open systems are relatively rare – Finland and Latvia being two of the few using it to elect their national parliaments – while panachage is largely the preserve of Luxembourg and Switzerland.
What’s better? Open vs closed list proportional representation
In terms of democratic legitimacy, open lists clearly trump closed lists. A system where voters have a choice over which individuals get to become MPs is undeniably more democratic than a system in which who is elected is determined by their placement on a list created by party leaderships or self-selected party memberships. There will always be a concern that closed lists can be used vindictively to make it harder for internal opponents to get elected, regardless of any personal popularity.
But defenders of closed lists argue that they are simply more practical – claiming that many voters only really care about parties and it is unreasonable to expect them to choose between often fairly long lists of largely unknown candidates from the same party. They point to the fact that in many semi-open systems it is a rarity for any candidate to be elected in spite of their position on a party list, with most voters choosing candidates already at the top of the list. Despite a relatively open system, the Netherlands has never had more than four MPs elected purely because of their individual votes.
However, even if most voters usually choose candidates that would have been elected anyway, does that really invalidate semi-open systems? Supporters would argue that of course list leaders are nearly always the most popular candidates, that is why they have been placed at the top of the list! Surely it is preferable for voters to have a choice to overrule their party, even if they use such an ability sparingly, they would argue.
But while open list and panachage systems can almost reach the level of voter power of the Single Transferable Vote (STV) – the ERS’ preferred electoral system – they still fall a little short. While they allow MPs to win seats on their own merits, the preferential nature of STV combines the voter control of a totally open list with the ability to still be represented if their first choice isn’t able to win a seat.
Read more posts...
Electoral commission chair shares perspective with mps and peers at ers backed event.
Last month we hosted an APPG for Electoral Reform event at Portcullis House, The future of the electoral system – in conversation with John Pullinger CB, Chair of the Electoral Commission. We were delighted to...
Posted 11 Apr 2024
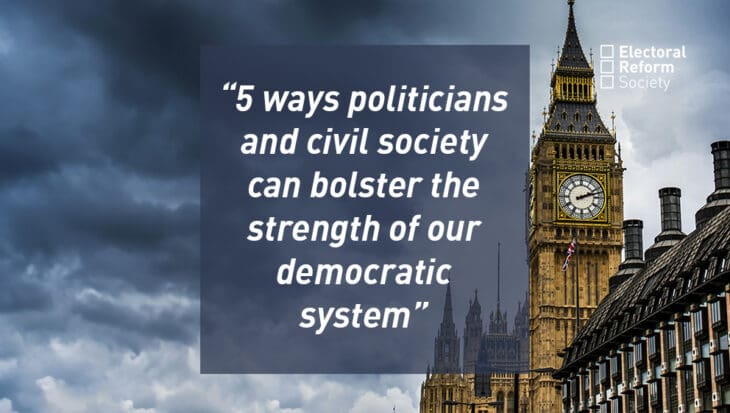
Where is Single Transferable Vote used in the UK?
Whilst First Past the Post is used for Westminster elections in the UK, it’s not the only way we elect people to office in the UK. Other voting systems have a long history and are...
Posted 29 Mar 2024
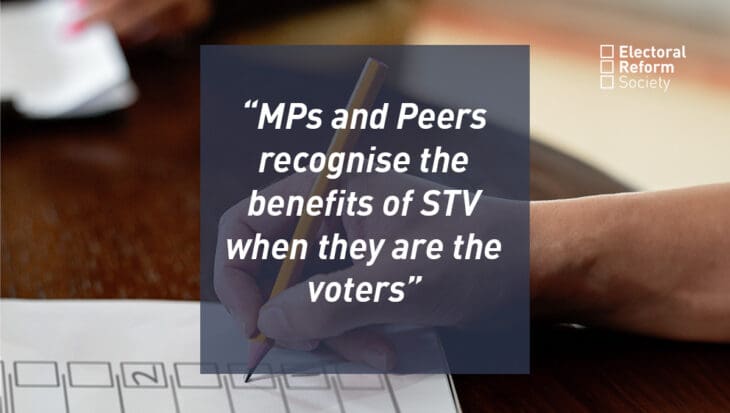

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
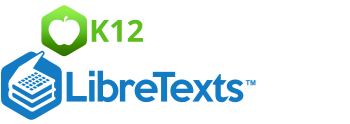
2.1: Types of Data Representation
- Last updated
- Save as PDF
- Page ID 5696
Two common types of graphic displays are bar charts and histograms. Both bar charts and histograms use vertical or horizontal bars to represent the number of data points in each category or interval. The main difference graphically is that in a bar chart there are spaces between the bars and in a histogram there are not spaces between the bars. Why does this subtle difference exist and what does it imply about graphic displays in general?
Displaying Data
It is often easier for people to interpret relative sizes of data when that data is displayed graphically. Note that a categorical variable is a variable that can take on one of a limited number of values and a quantitative variable is a variable that takes on numerical values that represent a measurable quantity. Examples of categorical variables are tv stations, the state someone lives in, and eye color while examples of quantitative variables are the height of students or the population of a city. There are a few common ways of displaying data graphically that you should be familiar with.
A pie chart shows the relative proportions of data in different categories. Pie charts are excellent ways of displaying categorical data with easily separable groups. The following pie chart shows six categories labeled A−F. The size of each pie slice is determined by the central angle. Since there are 360 o in a circle, the size of the central angle θ A of category A can be found by:

CK-12 Foundation - https://www.flickr.com/photos/slgc/16173880801 - CCSA
A bar chart displays frequencies of categories of data. The bar chart below has 5 categories, and shows the TV channel preferences for 53 adults. The horizontal axis could have also been labeled News, Sports, Local News, Comedy, Action Movies. The reason why the bars are separated by spaces is to emphasize the fact that they are categories and not continuous numbers. For example, just because you split your time between channel 8 and channel 44 does not mean on average you watch channel 26. Categories can be numbers so you need to be very careful.
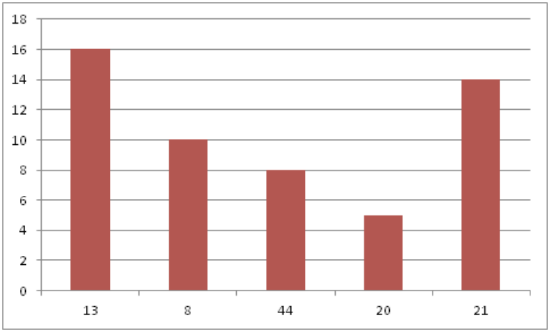
CK-12 Foundation - https://www.flickr.com/photos/slgc/16173880801 - CCSA
A histogram displays frequencies of quantitative data that has been sorted into intervals. The following is a histogram that shows the heights of a class of 53 students. Notice the largest category is 56-60 inches with 18 people.
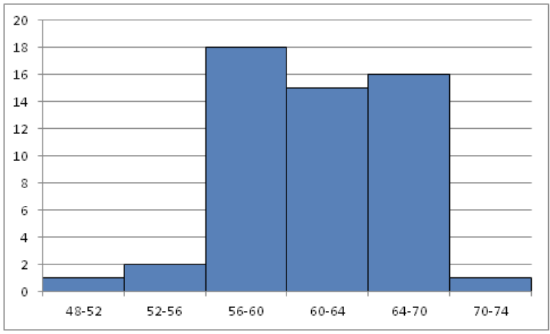
A boxplot (also known as a box and whiskers plot ) is another way to display quantitative data. It displays the five 5 number summary (minimum, Q1, median , Q3, maximum). The box can either be vertically or horizontally displayed depending on the labeling of the axis. The box does not need to be perfectly symmetrical because it represents data that might not be perfectly symmetrical.

Earlier, you were asked about the difference between histograms and bar charts. The reason for the space in bar charts but no space in histograms is bar charts graph categorical variables while histograms graph quantitative variables. It would be extremely improper to forget the space with bar charts because you would run the risk of implying a spectrum from one side of the chart to the other. Note that in the bar chart where TV stations where shown, the station numbers were not listed horizontally in order by size. This was to emphasize the fact that the stations were categories.
Create a boxplot of the following numbers in your calculator.
8.5, 10.9, 9.1, 7.5, 7.2, 6, 2.3, 5.5
Enter the data into L1 by going into the Stat menu.
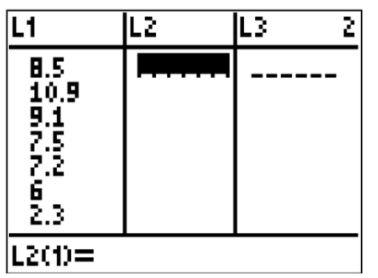
CK-12 Foundation - CCSA
Then turn the statplot on and choose boxplot.
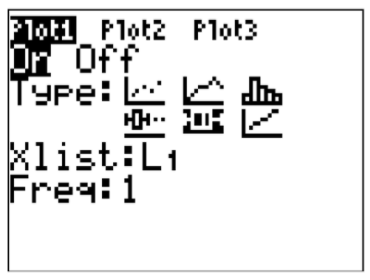
Use Zoomstat to automatically center the window on the boxplot.
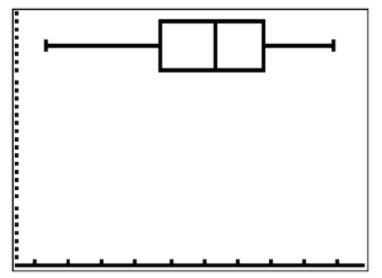
Create a pie chart to represent the preferences of 43 hungry students.
- Other – 5
- Burritos – 7
- Burgers – 9
- Pizza – 22
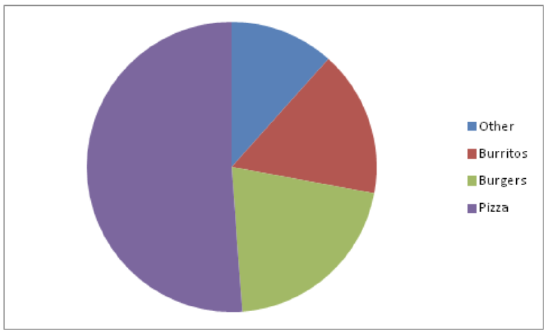
Create a bar chart representing the preference for sports of a group of 23 people.
- Football – 12
- Baseball – 10
- Basketball – 8
- Hockey – 3
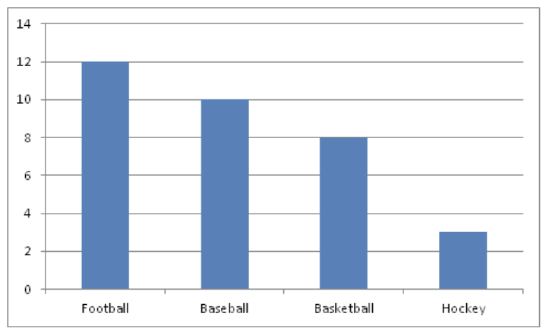
Create a histogram for the income distribution of 200 million people.
- Below $50,000 is 100 million people
- Between $50,000 and $100,000 is 50 million people
- Between $100,000 and $150,000 is 40 million people
- Above $150,000 is 10 million people
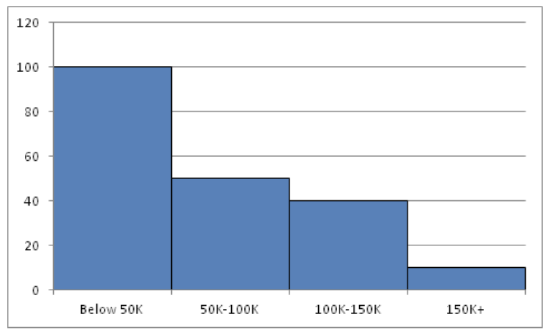
1. What types of graphs show categorical data?
2. What types of graphs show quantitative data?
A math class of 30 students had the following grades:
3. Create a bar chart for this data.
4. Create a pie chart for this data.
5. Which graph do you think makes a better visual representation of the data?
A set of 20 exam scores is 67, 94, 88, 76, 85, 93, 55, 87, 80, 81, 80, 61, 90, 84, 75, 93, 75, 68, 100, 98
6. Create a histogram for this data. Use your best judgment to decide what the intervals should be.
7. Find the five number summary for this data.
8. Use the five number summary to create a boxplot for this data.
9. Describe the data shown in the boxplot below.
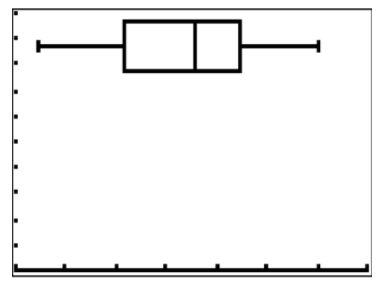
10. Describe the data shown in the histogram below.
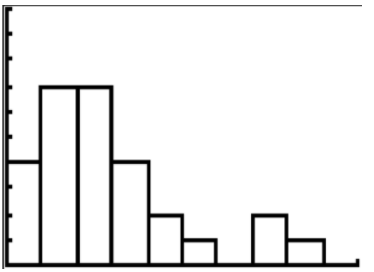
A math class of 30 students has the following eye colors:
11. Create a bar chart for this data.
12. Create a pie chart for this data.
13. Which graph do you think makes a better visual representation of the data?
14. Suppose you have data that shows the breakdown of registered republicans by state. What types of graphs could you use to display this data?
15. From which types of graphs could you obtain information about the spread of the data? Note that spread is a measure of how spread out all of the data is.
Review (Answers)
To see the Review answers, open this PDF file and look for section 15.4.
Additional Resources
PLIX: Play, Learn, Interact, eXplore - Baby Due Date Histogram
Practice: Types of Data Representation
Real World: Prepare for Impact

- Cambridge Dictionary +Plus
Meaning of representation in English
Your browser doesn't support HTML5 audio
representation noun ( ACTING FOR )
- Defendants have a right to legal representation and must be informed of that right when they are arrested .
- The farmers demanded greater representation in parliament .
- The main opposing parties have nearly equal representation in the legislature .
- The scheme is intended to increase representation of minority groups .
- The members are chosen by a system of proportional representation.
- admissibility
- extinguishment
- extrajudicial
- extrajudicially
- fatal accident inquiry
- federal case
- pay damages
- plea bargain
- plea bargaining
- the Webster ruling
- walk free idiom
- witness to something
representation noun ( DESCRIPTION )
- anti-realism
- anti-realist
- complementary
- confederate
- naturalistically
- non-figurative
- non-representational
- poetic licence
- symbolization
representation noun ( INCLUDING ALL )
- all manner of something idiom
- alphabet soup
- it takes all sorts (to make a world) idiom
- non-segregated
- odds and ends
- of every stripe/of all stripes idiom
- this and that idiom
- variety is the spice of life idiom
- wide choice
representation | Business English
Examples of representation, collocations with representation.
- representation
These are words often used in combination with representation .
Click on a collocation to see more examples of it.
Translations of representation
Get a quick, free translation!
Word of the Day
balancing act
a difficult situation in which someone has to try to give equal amounts of importance, time, attention, etc. to two or more different things at the same time

Alike and analogous (Talking about similarities, Part 1)

Learn more with +Plus
- Recent and Recommended {{#preferredDictionaries}} {{name}} {{/preferredDictionaries}}
- Definitions Clear explanations of natural written and spoken English English Learner’s Dictionary Essential British English Essential American English
- Grammar and thesaurus Usage explanations of natural written and spoken English Grammar Thesaurus
- Pronunciation British and American pronunciations with audio English Pronunciation
- English–Chinese (Simplified) Chinese (Simplified)–English
- English–Chinese (Traditional) Chinese (Traditional)–English
- English–Dutch Dutch–English
- English–French French–English
- English–German German–English
- English–Indonesian Indonesian–English
- English–Italian Italian–English
- English–Japanese Japanese–English
- English–Norwegian Norwegian–English
- English–Polish Polish–English
- English–Portuguese Portuguese–English
- English–Spanish Spanish–English
- English–Swedish Swedish–English
- Dictionary +Plus Word Lists
- representation (ACTING FOR)
- representation (DESCRIPTION)
- representation (INCLUDING ALL)
- make representations to sb
- Collocations
- Translations
- All translations
Add representation to one of your lists below, or create a new one.
{{message}}
Something went wrong.
There was a problem sending your report.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Linked List Data Structure
- Understanding the basics of Linked List
- Introduction to Linked List - Data Structure and Algorithm Tutorials
- Applications, Advantages and Disadvantages of Linked List
- Linked List vs Array
Types of Linked List
- Singly Linked List Tutorial
- Doubly Linked List Tutorial
- Introduction to Circular Linked List
Basic Operations on Linked List
- Insertion in Linked List
- Search an element in a Linked List (Iterative and Recursive)
- Find Length of a Linked List (Iterative and Recursive)
- Reverse a Linked List
- Deletion in Linked List
- Delete a Linked List node at a given position
- Write a function to delete a Linked List
- Write a function to get Nth node in a Linked List
- Program for Nth node from the end of a Linked List
- Top 50 Problems on Linked List Data Structure asked in SDE Interviews
A linked list is a fundamental data structure in computer science. It consists of nodes where each node contains data and a reference (link) to the next node in the sequence. This allows for dynamic memory allocation and efficient insertion and deletion operations compared to arrays.
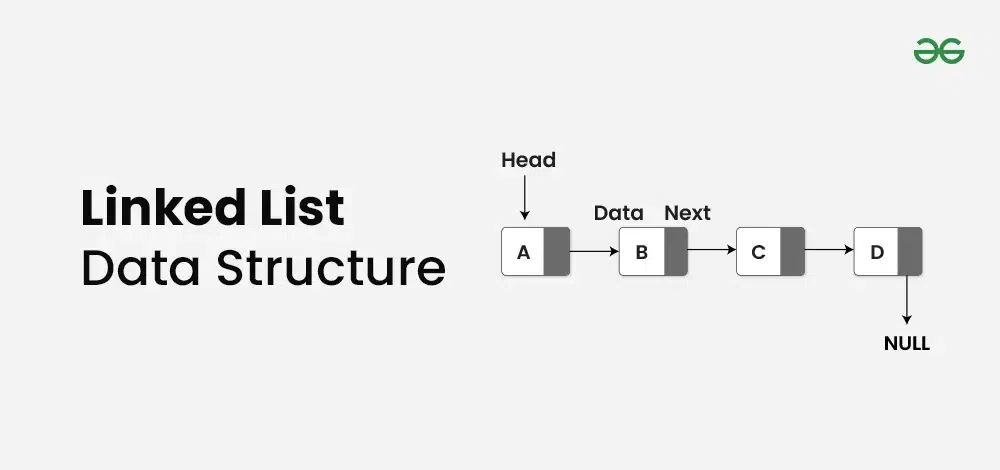
Table of Content
What is a Linked List?
Linked lists vs arrays.
- Operations of Linked Lists
Linked List Applications
- Basics of Linked List
- Easy Problem on Linked List
- Medium Problem on Linked List
- Hard Problem on Linked List
A linked list is a linear data structure that consists of a series of nodes connected by pointers. Each node contains data and a reference to the next node in the list. Unlike arrays, linked lists allow for efficient insertion or removal of elements from any position in the list, as the nodes are not stored contiguously in memory.
Here’s the comparison of Linked List vs Arrays
Linked List:
- Data Structure: Non-contiguous
- Memory Allocation: Dynamic
- Insertion/Deletion: Efficient
- Access: Sequential
- Data Structure: Contiguous
- Memory Allocation: Static
- Insertion/Deletion: Inefficient
- Access: Random
- Singly Linked List
- Doubly Linked List
- Circular Linked List
- Circular Doubly Linked List
- Header Linked List
Operations of Linked Lists:
- Linked List Insertion
- Reverse a linked list
- Linked List Deletion (Deleting a given key)
- Linked List Deletion (Deleting a key at given position)
- Nth node from the end of a Linked List
- Implementing stacks and queues using linked lists.
- Using linked lists to handle collisions in hash tables.
- Representing graphs using linked lists.
- Allocating and deallocating memory dynamically.
Basics of Linked List:
- What is Linked List
- Introduction to Linked List – Data Structure and Algorithm Tutorials
Easy Problem on Linked List:
- Print the middle of a given linked list
- Write a function that counts the number of times a given int occurs in a Linked List
- Check if a linked list is Circular Linked List
- Count nodes in Circular linked list
- Convert singly linked list into circular linked list
- Exchange first and last nodes in Circular Linked List
- Reverse a Doubly Linked List
- Program to find size of Doubly Linked List
- An interesting method to print reverse of a linked list
- Can we reverse a linked list in less than O(n)?
- Circular Linked List Traversal
- Delete a node in a Doubly Linked List
Medium Problem on Linked List:
- Detect loop in a linked list
- Find length of loop in linked list
- Remove duplicates from a sorted linked list
- Intersection of two Sorted Linked Lists
- QuickSort on Singly Linked List
- Split a Circular Linked List into two halves
- Deletion from a Circular Linked List
- Merge Sort for Doubly Linked List
- Find pairs with given sum in doubly linked list
- Insert value in sorted way in a sorted doubly linked list
- Remove duplicates from an unsorted doubly linked list
- Rotate Doubly linked list by N nodes
- Given only a pointer to a node to be deleted in a singly linked list, how do you delete it?
- Modify contents of Linked List
Hard Problem on Linked List:
- Intersection point of two Linked Lists.
- Circular Queue | Set 2 (Circular Linked List Implementation)
- Josephus Circle using circular linked list
- The Great Tree-List Recursion Problem.
- Copy a linked list with next and arbit pointer
- Convert a given Binary Tree to Doubly Linked List | Set
- Priority Queue using doubly linked list
- Reverse a doubly linked list in groups of given size
- Reverse a stack without using extra space in O(n)
- Linked List representation of Disjoint Set Data Structures
- Sublist Search (Search a linked list in another list)
- Construct a linked list from 2D matrix
Quick Links :
- ‘Practice Problems’ on Linked List
- ‘Videos’ on Linked List
- ‘Quizzes’ on Linked List
Recomended:
- Learn Data Structure and Algorithms | DSA Tutorial
Please Login to comment...
Related articles.
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- More from M-W
- To save this word, you'll need to log in. Log In
representation
Definition of representation
Examples of representation in a sentence.
These examples are programmatically compiled from various online sources to illustrate current usage of the word 'representation.' Any opinions expressed in the examples do not represent those of Merriam-Webster or its editors. Send us feedback about these examples.
Word History
15th century, in the meaning defined at sense 1
Phrases Containing representation
- proportional representation
- self - representation
Dictionary Entries Near representation
representant
representationalism
Cite this Entry
“Representation.” Merriam-Webster.com Dictionary , Merriam-Webster, https://www.merriam-webster.com/dictionary/representation. Accessed 17 Apr. 2024.
Kids Definition
Kids definition of representation, legal definition, legal definition of representation, more from merriam-webster on representation.
Thesaurus: All synonyms and antonyms for representation
Nglish: Translation of representation for Spanish Speakers
Britannica English: Translation of representation for Arabic Speakers
Britannica.com: Encyclopedia article about representation
Subscribe to America's largest dictionary and get thousands more definitions and advanced search—ad free!
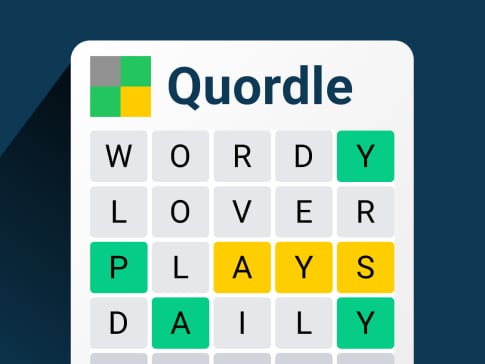
Can you solve 4 words at once?
Word of the day, circumlocution.
See Definitions and Examples »
Get Word of the Day daily email!
Popular in Grammar & Usage
Your vs. you're: how to use them correctly, every letter is silent, sometimes: a-z list of examples, more commonly mispronounced words, how to use em dashes (—), en dashes (–) , and hyphens (-), absent letters that are heard anyway, popular in wordplay, a great big list of bread words, the words of the week - apr. 12, 10 scrabble words without any vowels, 12 more bird names that sound like insults (and sometimes are), 9 superb owl words, games & quizzes.
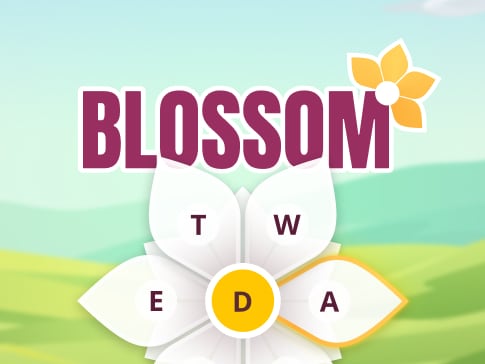
Words and phrases
Personal account.
- Access or purchase personal subscriptions
- Get our newsletter
- Save searches
- Set display preferences
Institutional access
Sign in with library card
Sign in with username / password
Recommend to your librarian
Institutional account management
Sign in as administrator on Oxford Academic
representation noun 1
- Hide all quotations
What does the noun representation mean?
There are 19 meanings listed in OED's entry for the noun representation , three of which are labelled obsolete. See ‘Meaning & use’ for definitions, usage, and quotation evidence.
representation has developed meanings and uses in subjects including
How common is the noun representation ?
How is the noun representation pronounced, british english, u.s. english, where does the noun representation come from.
Earliest known use
Middle English
The earliest known use of the noun representation is in the Middle English period (1150—1500).
OED's earliest evidence for representation is from around 1450, in St. Elizabeth of Spalbeck .
representation is of multiple origins. Either (i) a borrowing from French. Or (ii) a borrowing from Latin.
Etymons: French representation ; Latin repraesentātiōn- , repraesentātiō .
Nearby entries
- reprehensory, adj. 1576–1825
- repremiation, n. 1611
- represent, n. a1500–1635
- represent, v.¹ c1390–
- re-present, v.² 1564–
- representable, adj. & n. 1630–
- representamen, n. 1677–
- representance, n. 1565–
- representant, n. 1622–
- representant, adj. 1851–82
- representation, n.¹ c1450–
- re-presentation, n.² 1805–
- representational, adj. 1850–
- representationalism, n. 1846–
- representationalist, adj. & n. 1846–
- representationary, adj. 1856–
- representationism, n. 1842–
- representationist, n. & adj. 1842–
- representation theory, n. 1928–
- representative, adj. & n. a1475–
- representative fraction, n. 1860–
Thank you for visiting Oxford English Dictionary
To continue reading, please sign in below or purchase a subscription. After purchasing, please sign in below to access the content.
Meaning & use
Pronunciation, compounds & derived words, entry history for representation, n.¹.
representation, n.¹ was revised in December 2009.
representation, n.¹ was last modified in March 2024.
oed.com is a living text, updated every three months. Modifications may include:
- further revisions to definitions, pronunciation, etymology, headwords, variant spellings, quotations, and dates;
- new senses, phrases, and quotations.
Revisions and additions of this kind were last incorporated into representation, n.¹ in March 2024.
Earlier versions of this entry were published in:
OED First Edition (1906)
- Find out more
OED Second Edition (1989)
- View representation in OED Second Edition
Please submit your feedback for representation, n.¹
Please include your email address if you are happy to be contacted about your feedback. OUP will not use this email address for any other purpose.
Citation details
Factsheet for representation, n.¹, browse entry.
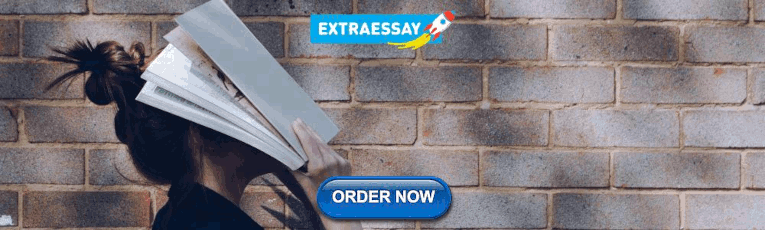
IMAGES
VIDEO
COMMENTS
Adjacency list. This undirected cyclic graph can be described by the three unordered lists {b, c }, {a, c }, {a, b }. In graph theory and computer science, an adjacency list is a collection of unordered lists used to represent a finite graph. Each unordered list within an adjacency list describes the set of neighbors of a particular vertex in ...
An adjacency list represents a graph as an array of linked lists. The index of the array represents a vertex and each element in its linked list represents the other vertices that form an edge with the vertex. For example, we have a graph below. We can represent this graph in the form of a linked list on a computer as shown below. Here, 0, 1, 2 ...
The adjacency list representation for an undirected graph is just an adjacency list for a directed graph, where every undirected edge connecting A to B is represented as two directed edges: -one from A->B -one from B->A e.g. if you have a graph with undirected edges connecting 0 to 1 and 1 to 2 your adjacency list would be: [ [1] //edge 0->1
Adjacency list representation. Since sparse graphs are common, the adjacency list representation is often preferred. This representation keeps track of the outgoing edges from each vertex, typically as a linked list. For example, the graph above might be represented with the following data structure:
The keys then would correspond to the indices of each node and the value would be a reference to the list of adjacent node indices. Another implementation might require that each node keep a list of its adjacent nodes. This page titled 8.4: Graph Representations is shared under a CC BY-SA license and was authored, remixed, and/or curated by ...
An adjacency list is a data structure used to represent a graph where each node in the graph stores a list of its neighboring vertices. Characteristics of the Adjacency List: The size of the matrix is determined by the number of nodes in the network. The number of graph edges is easily computed. The adjacency list is a jagged array.
A Graph is a non-linear data structure consisting of vertices and edges. The vertices are sometimes also referred to as nodes and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph is composed of a set of vertices ( V ) and a set of edges ( E ). The graph is denoted by G (V, E).
To store the adjacency list, we need O(V + E) O ( V + E) space as we need to store every vertex and their neighbors (edges). To find if a vertex has a neighbor, we need to go through the linked list of the vertex. This requires O(1 + deg(V)) O ( 1 + d e g ( V)) time. If we use balanced binary search trees, it becomes O(1 + log(deg(V)) O ( 1 ...
The adjacency list representation of a graph consists of n lists one for each vertex v_i, 1<=i<=n, which gives the vertices to which v_i is adjacent. The adjacency lists of a graph g may be computed in the Wolfram Language using AdjacencyList[g, #]& /@ VertexList[g]and a graph may be constructed from adjacency lists l using Graph[UndirectedEdge @@@ Union[ Sort /@ Flatten[ MapIndexed[{#, #2[[1 ...
Adjacency "list" conceptual representation •Vertices: •Set of vertex labels •Set<Integer> for example graph •Adjacencies: •Dictionary mapping from vertex labels to sets of vertex labels •Map<Integer, Set<Integer>> for example graph •But the reason for the name "list" is you can do it all with just linked lists… 1 2 3 4
An adjacency list is a hash map that maps each node to a list of neighbors. This combines the benefits of both the edge list and the adjacency matrix, by providing a contained structure that allows you to easily see a node's neighbors. In Python, adjacency lists are often represented as dictionaries.
An adjacency list only stores the edges of a graph, not the vertices, making it a space-efficient representation of a graph. It is also simple to implement and easy to modify. An adjacency list can be easily modified to store additional information about the edges in the graph, such as weights or labels. Advantages of an Adjacency List
Python's list is a flexible, versatile, powerful, and popular built-in data type. It allows you to create variable-length and mutable sequences of objects. In a list, you can store objects of any type. You can also mix objects of different types within the same list, although list elements often share the same type.
A linked list is a linear data structure that includes a series of connected nodes. Here, each node stores the data and the address of the next node. For example, Linked list Data Structure. You have to start somewhere, so we give the address of the first node a special name called HEAD. Also, the last node in the linked list can be identified ...
A vote for a particular party is read as an endorsement of their list. Open list proportional representation. Open list systems, on the other hand, allow voters to cast votes for individual candidates on one party's list. The exact rules of open lists vary from system to system, but they can usually be classified as either fully- or semi-open.
2.1: Types of Data Representation. Page ID. Two common types of graphic displays are bar charts and histograms. Both bar charts and histograms use vertical or horizontal bars to represent the number of data points in each category or interval. The main difference graphically is that in a bar chart there are spaces between the bars and in a ...
Party list systems are the most common methods of proportional representation used around the world. The three major variants of party list proportional representation systems vary to the degree that they allow voters to cast votes for candidates and for political parties. Closed List. The simplest, most common party list system is the closed list.
REPRESENTATION definition: 1. a person or organization that speaks, acts, or is present officially for someone else: 2. the…. Learn more.
A linked list is a linear data structure that consists of a series of nodes connected by pointers. Each node contains data and a reference to the next node in the list. Unlike arrays, linked lists allow for efficient insertion or removal of elements from any position in the list, as the nodes are not stored contiguously in memory.
In logic, a set of symbols is commonly used to express logical representation. The following table lists many common symbols, together with their name, how they should be read out loud, and the related field of mathematics.Additionally, the subsequent columns contains an informal explanation, a short example, the Unicode location, the name for use in HTML documents, and the LaTeX symbol.
representation: [noun] one that represents: such as. an artistic likeness or image. a statement or account made to influence opinion or action. an incidental or collateral statement of fact on the faith of which a contract is entered into. a dramatic production or performance. a usually formal statement made against something or to effect a ...
e. Party-list representation in the House of Representatives of the Philippines refers to a system in which 20% of the House of Representatives is elected. While the House is predominantly elected by a plurality voting system, known as a first-past-the-post system, party-list representatives are elected by a type of party-list proportional ...
There are 19 meanings listed in OED's entry for the noun representation, three of which are labelled obsolete. See 'Meaning & use' for definitions, usage, and quotation evidence. representation has developed meanings and uses in subjects including. visual arts (Middle English) theatre (late 1500s) philosophy (early 1600s) law (early 1600s ...