TypeError: Assignment to Constant Variable in JavaScript
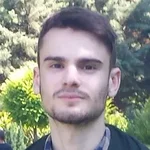
Last updated: Mar 2, 2024 Reading time · 3 min
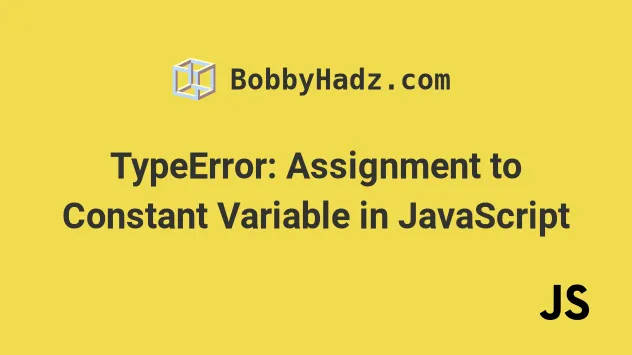
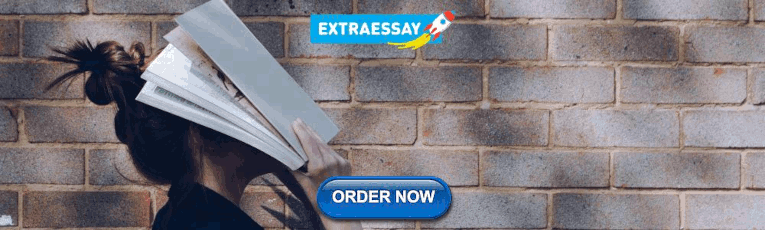
# TypeError: Assignment to Constant Variable in JavaScript
The "Assignment to constant variable" error occurs when trying to reassign or redeclare a variable declared using the const keyword.
When a variable is declared using const , it cannot be reassigned or redeclared.

Here is an example of how the error occurs.
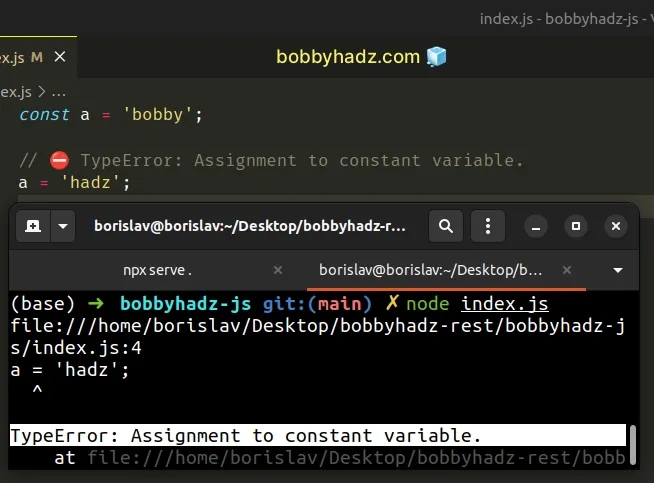
# Declare the variable using let instead of const
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const .
Variables declared using the let keyword can be reassigned.
We used the let keyword to declare the variable in the example.
Variables declared using let can be reassigned, as opposed to variables declared using const .
You can also use the var keyword in a similar way. However, using var in newer projects is discouraged.
# Pick a different name for the variable
Alternatively, you can declare a new variable using the const keyword and use a different name.
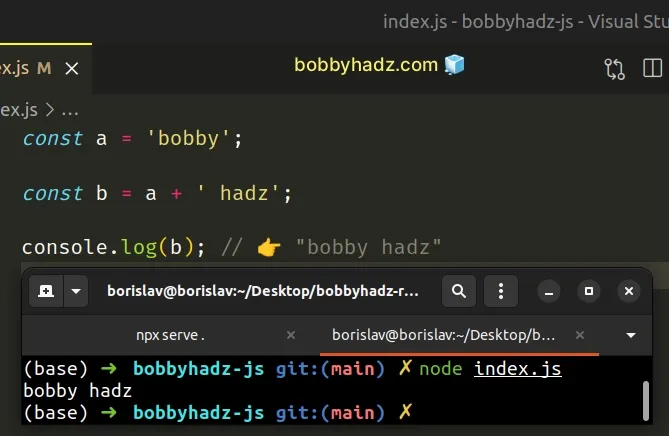
We declared a variable with a different name to resolve the issue.
The two variables no longer clash, so the "assignment to constant" variable error is no longer raised.
# Declaring a const variable with the same name in a different scope
You can also declare a const variable with the same name in a different scope, e.g. in a function or an if block.
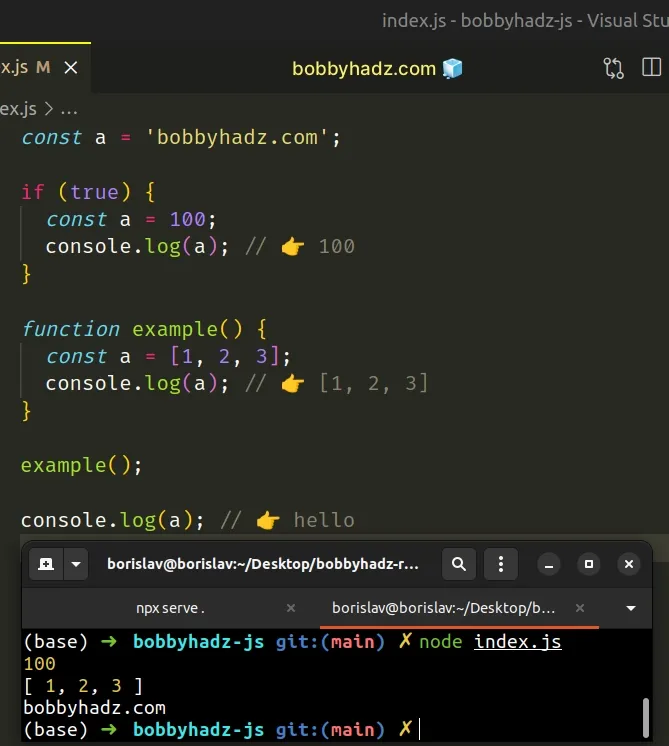
The if statement and the function have different scopes, so we can declare a variable with the same name in all 3 scopes.
However, this prevents us from accessing the variable from the outer scope.
# The const keyword doesn't make objects immutable
Note that the const keyword prevents us from reassigning or redeclaring a variable, but it doesn't make objects or arrays immutable.
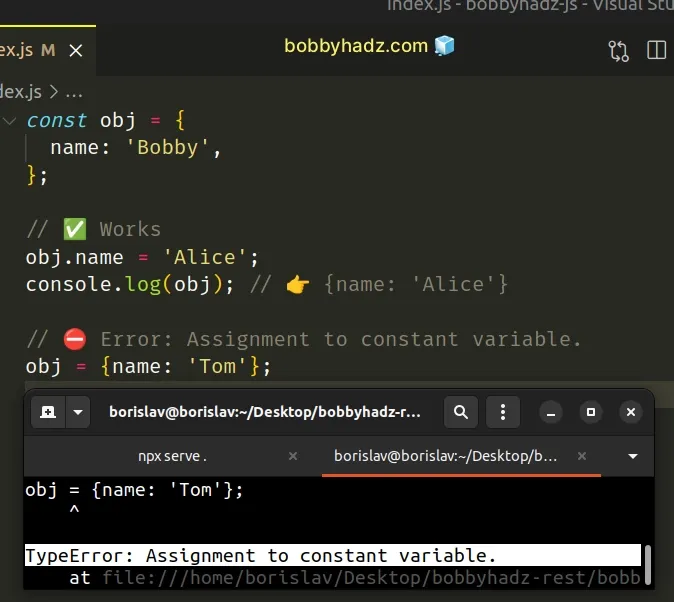
We declared an obj variable using the const keyword. The variable stores an object.
Notice that we are able to directly change the value of the name property even though the variable was declared using const .
The behavior is the same when working with arrays.
Even though we declared the arr variable using the const keyword, we are able to directly change the values of the array elements.
The const keyword prevents us from reassigning the variable, but it doesn't make objects and arrays immutable.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: Unterminated string constant in JavaScript
- TypeError (intermediate value)(...) is not a function in JS
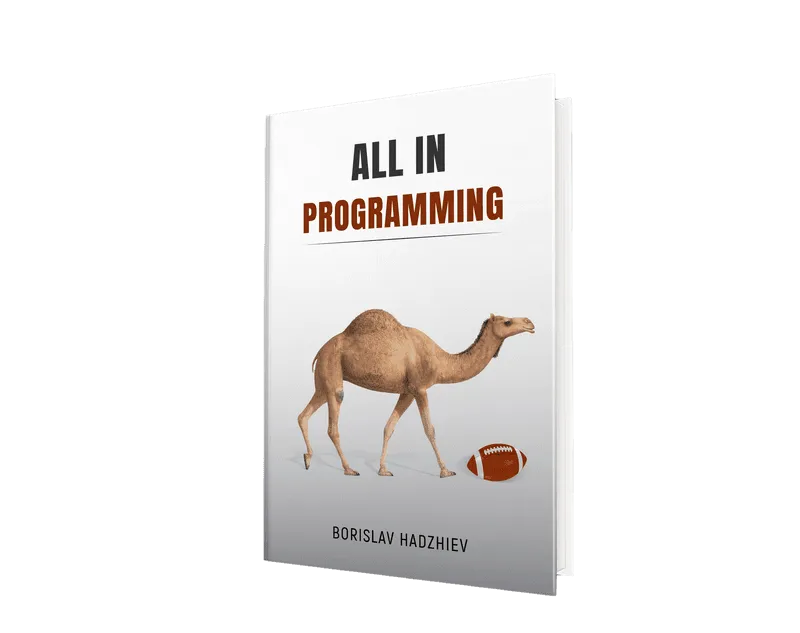
Borislav Hadzhiev
Web Developer
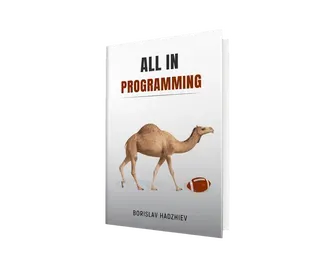
Copyright © 2024 Borislav Hadzhiev
- Skip to main content
- Select language
- Skip to search
TypeError: invalid assignment to const "x"
Const and immutability, what went wrong.
A constant is a value that cannot be altered by the program during normal execution. It cannot change through re-assignment, and it can't be redeclared. In JavaScript, constants are declared using the const keyword.
Invalid redeclaration
Assigning a value to the same constant name in the same block-scope will throw.
Fixing the error
There are multiple options to fix this error. Check what was intended to be achieved with the constant in question.
If you meant to declare another constant, pick another name and re-name. This constant name is already taken in this scope.
const , let or var ?
Do not use const if you weren't meaning to declare a constant. Maybe you meant to declare a block-scoped variable with let or global variable with var .
Check if you are in the correct scope. Should this constant appear in this scope or was is meant to appear in a function, for example?
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable:
But you can mutate the properties in a variable:
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy." href="Property_access_denied.html">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing variable name
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: cyclic object value
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting a property that has only a getter
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- [email protected]
- 🇮🇳 +91 (630)-411-6234
- 🇺🇸 +1 (334) 517-0924
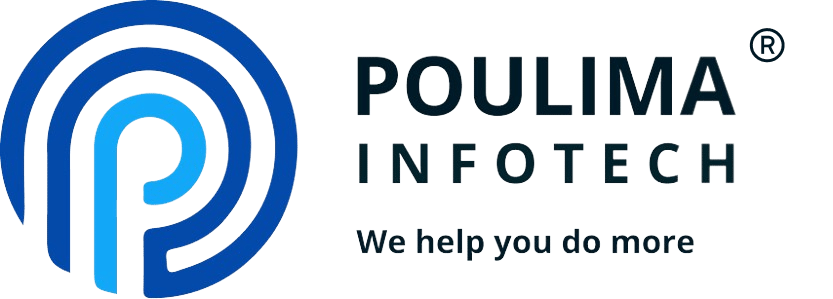
Web Development
Mobile app development, nodejs typeerror: assignment to constant variable.
Published By: Divya Mahi
Published On: November 17, 2023
Published In: Development
Grasping and Fixing the 'NodeJS TypeError: Assignment to Constant Variable' Issue
Introduction.
Node.js, a powerful platform for building server-side applications, is not immune to errors and exceptions. Among the common issues developers encounter is the “NodeJS TypeError: Assignment to Constant Variable.” This error can be a source of frustration, especially for those new to JavaScript’s nuances in Node.js. In this comprehensive guide, we’ll explore what this error means, its typical causes, and how to effectively resolve it.
Understanding the Error
In Node.js, the “TypeError: Assignment to Constant Variable” occurs when there’s an attempt to reassign a value to a variable declared with the const keyword. In JavaScript, const is used to declare a variable that cannot be reassigned after its initial assignment. This error is a safeguard in the language to ensure the immutability of variables declared as constants.
Diving Deeper
This TypeError is part of JavaScript’s efforts to help developers write more predictable code. Immutable variables can prevent bugs that are hard to trace, as they ensure that once a value is set, it cannot be inadvertently changed. However, it’s important to distinguish between reassigning a variable and modifying an object’s properties. The latter is allowed even with variables declared with const.
Common Scenarios and Fixes
Example 1: reassigning a constant variable.
Javascript:
Fix: Use let if you need to reassign the variable.
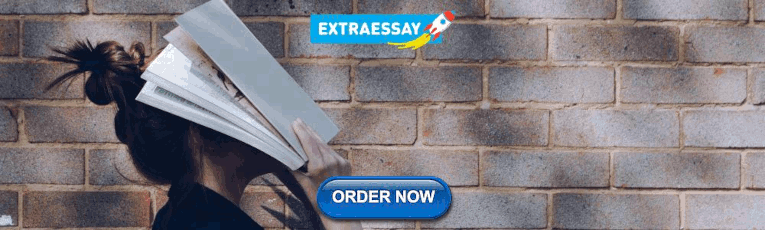
Example 2: Modifying an Object's Properties
Fix: Modify the property instead of reassigning the object.
Example 3: Array Reassignment
Fix: Modify the array’s contents without reassigning it.
Example 4: Within a Function Scope
Fix: Declare a new variable or use let if reassignment is needed.
Example 5: In Loops
Fix: Use let for variables that change within loops.
Example 6: Constant Function Parameters
Fix: Avoid reassigning function parameters directly; use another variable.
Example 7: Constants in Conditional Blocks
Fix: Use let if the variable needs to change.
Example 8: Reassigning Properties of a Constant Object
Fix: Modify only the properties of the object.
Strategies to Prevent Errors
Understand const vs let: Familiarize yourself with the differences between const and let. Use const for variables that should not be reassigned and let for those that might change.
Code Reviews: Regular code reviews can catch these issues before they make it into production. Peer reviews encourage adherence to best practices.
Linter Usage: Tools like ESLint can automatically detect attempts to reassign constants. Incorporating a linter into your development process can prevent such errors.
Best Practices
Immutability where Possible: Favor immutability in your code to reduce side effects and bugs. Normally use const to declare variables, and use let only if you need to change their values later .
Descriptive Variable Names: Use clear and descriptive names for your variables. This practice makes it easier to understand when a variable should be immutable.
Keep Functions Pure: Avoid reassigning or modifying function arguments. Keeping functions pure (not causing side effects) leads to more predictable and testable code.
The “NodeJS TypeError: Assignment to Constant Variable” error, while common, is easily avoidable. By understanding JavaScript’s variable declaration nuances and adopting coding practices that embrace immutability, developers can write more robust and maintainable Node.js applications. Remember, consistent coding standards and thorough code reviews are your best defense against common errors like these.
Related Articles
March 13, 2024
Expressjs Error: 405 Method Not Allowed
March 11, 2024
Expressjs Error: 502 Bad Gateway
I’m here to assist you.
Something isn’t Clear? Feel free to contact Us, and we will be more than happy to answer all of your questions.
JavaScript const Keyword Explained with Examples
by Nathan Sebhastian
Posted on Oct 27, 2023
Reading time: 4 minutes
Hi friends! Today, I will teach you how the const keyword works in JavaScript works and when you should use it.
The const keyword is used to declare a variable that can’t be changed after its declaration. This keyword is short for constant (which means “doesn’t change”), and it’s used in a similar manner to the let and var keywords.
You write the keyword, followed it up with a variable name, the assignment = operator, and the variable’s value:
We’ll see how the const keyword differs from the let keyword next. Let’s write a variable called name that has a string value, and we change the value after the declaration:
The code runs without any problem, and the console.log displays the new value ‘Good morning!’ instead of the first value ‘Hello!’.
Now let’s replace let with const and run the code again:
This time, we get an error as follows:
Since we declared the message variable as a constant, JavaScript responded with an error when we tried to assign a new value to the variable.
A constant variable is usually written in an all-uppercase format ( message becomes MESSAGE )
If the variable name is more than one word, separate the words using underscores. For example: TOTAL_MESSAGE_COUNT .
The const Keyword Only Prevents Reassignment
The const keyword doesn’t allow you to change the object the variable points to, but you can still change the data in the object.
For example, suppose you have a person object that has a name property. You can change the name property value even when you declare the object using a const as follows:
But if you try to change the object the variable points to, you’ll get the same error:
Note carefully the differences between the two examples above. In the first example, the name property is modified, but the variable person still points to the same object. This is allowed.
In the second example, we tried to change the object the person variable points to, and this is not allowed.
The same goes when you have a variable pointing to an array. You can change the elements stored in the array, but not the array itself.
The const keyword prevents reassignment (making the variable point to a different value) but not mutation (editing the value itself)
When to use the const keyword
Now that you know how the const keyword works, when do you need to use it in your code?
The answer is when you need to declare a variable that will never change during the lifecycle of your program.
For example, suppose you’re writing a program that has a lot of time calculations. You can declare a few constants that store the relation between hours, minutes, and seconds as follows:
There will always be 60 seconds in a minute, 3600 seconds in a minute, and 24 hours in a day, no matter where you live, as long as it’s on Earth 😄
You also always have 7 days in a week, 4 weeks in a month, and 12 months in a year. A month can have different days (27, 28, 30, 31) so don’t make it a constant.
Another example is when you need to work with geometry. To calculate the circumference of a circle, you need the PI value, which is a constant:
I hope these examples help you get the point. The key to knowing when to use const is to ask yourself this: would you have to assign a new value to the variable later, after its declaration? If not, use const . Otherwise, use let .
This article has shown you how the const keyword works, how it doesn’t prevent mutation, and when to use it in your project.
The const keyword is kind of bewildering in how it doesn’t prevent mutation. Indeed, JavaScript is a tricky language with some weird behaviors that can lead to errors.
I have some more articles related to JavaScript that you can read here:
Bubble Sort in JavaScript Understanding Encapsulation in JavaScript
I also have a JavaScript course that’s currently in progress. I want to make this course the best and complete JavaScript course for beginners. If you enjoy this article, you might want to check it out.
Thanks for reading. See you next time! 👋
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:
- SYEDHUSSIM.COM
SAP Business One
Difference between const, let and var.
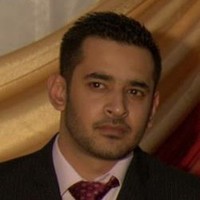
- Author Syed Hussim
- Published 12 July 2019
- Viewed 1730
Part 2 of A Javascript Implementation Of The Logo Programming Language. In the previous article I explained how to develop a simple lexer. In this part we develop a TokenCollection class to help traverse the array of tokens returned from the lexer.
In this four part article, I explain how to develop the iconic Logo programming language in JavaScript. In this part, we discuss how to take a source input and convert it into a series of tokens.
If you're building a REST API, chances are you're going to need to generate secure random API keys. In this article, I explain how to use the Node.js crypto module to generate random secure API keys.
A simple port scanner to scan a range of ports.
An introduction to sorting algorithms.
This article explains how to create a simple HTTP server using Node.js without using any dependencies.
Node.Js provides several ways to read user input from the terminal. This article explains how to use the process object to read user input.
SAP HANA has several functions to help query JSON data and in this article, we'll take a look at how to use the JSON_TABLE function to query JSON data stored in a regular column.
The hdbconnect package makes it easy to connect to a HANA database using Rust and in this article, we'll take a look at how to establish a connection and perform some queries.
In this last article on developing a command line program, we'll develop a program that counts the occurrence of different file types in a directory and its sub-directories. The program will accept a directory as an argument and recursively scan all sub-directories. We'll use a HashMap to keep track of the count for each file type.
In the previous article, we developed a simple command line program that counted how many times a word occurred in a text file. The article gave us a brief introduction to the env, collections, and fs modules. In this article, we'll take a look at the TcpStream struct from the net module to develop a simple port scanner.
The best way to learn any programming language is to develop small meaningful programs that are easy to write and serve a purpose. In this three part article, we'll develop several small command line programs. Each article will focus on a different part of the standard library.
In part 1, we developed a simple single-threaded HTTP server that processed requests synchronously. In part 2, we modified the server so that each request was handled in a separate thread, which improved concurrency but increased the load on the CPU and memory. In this article, we'll modify the server to use a ThreadPool which will help to improve concurrency while reducing the CPU and memory usage.
In part 1, we developed a simple single-threaded HTTP server that returned a static string. While single-threaded servers have their use cases, they lack scalability. The nature of a single-threaded server means it can only process one request at a time. If there's a request that's taking a long time to process, all other requests will be blocked until the slow request has been completed. If we want to handle multiple requests at the same time, we need to use threads.
NodeJs has built-in http modules that allow developers to build web applications without the need to configure and run a proprietary HTTP server such as Apache or Nginx. Other programming languages provide similar libraries that allow developers to bundle a server with an application. So in this article, we'll take a look at how easy it is to write a simple single-threaded HTTP server in Java. Once we're done, we can run a simple test using Apaches ab tool to determine how many requests per second the server can handle.
Batch managed items can be transferred between warehouses and bins by including the BatchNumbers property in the StockTransfers API post request. The BatchNumbers property is an array of batch numbers. Listing 1 below shows an example JSON object that contains an item with a single batch. Notice that the quantity specified for the batch number object is equal to the quantity at the item level.
Developing inventory management tools requires a good understanding of SAP Business One API's. Many API's in the Service Layer documentation lack payload samples leaving developers to do their own research. This article aims to help developers understand the payload data that is required to create a stock transfer.
In this article we continue developing the Logo Programming language further by writing a simple TokenCollection class to help traverse the array of tokens returned from the lexer we created in part 1. This class will be used in Part 3, when we develop the parser.
The Logo programming language was designed by Wally Feurzeig, Seymour Papert, and Cynthia Solomon in 1967 as an educational tool. The language was used to program a robotic turtle that would draw graphics given a series of instructions. In later years the physical robot was replaced by a virtual on-screen turtle. Over the years, various institutions and organizations have implemented the language with additional features. Notable major implementations are UCBLogo and MSWLogo.
If you're building a REST API, chances are you're going to need to generate secure random API keys. You may be tempted to use a third-party library but before you do, consider using the crypto package from Node.js. The crypto module can generate random bytes, which can then be used to create your API keys.
NodeJs has become one of my go to tools for developing small utilities and services extremely quickly. One such tool that I've developed, is a simple port scanner to help determine which ports are being used.
I don't often start an article asking a question, but I'll make an exception today. Can you take the following array [8,3,1,9,6,5,7,2,4] and sort it in ascending order? Fairly easy right? Many programming languages provide sorting functions that make tasks such as this a one liner.
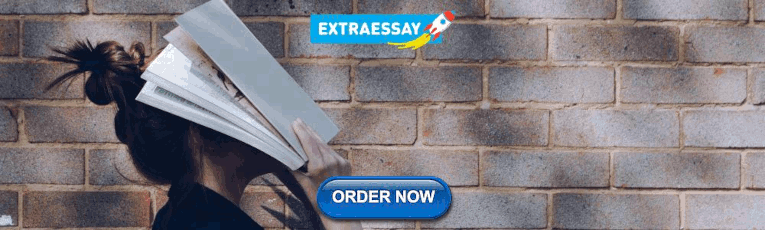
COMMENTS
To fix this error, consider using the let keyword instead of const if you need to update the variable's value. Alternatively, ensure that you are not attempting to reassign the constant variable inadvertently. Remember, const is appropriate for values that remain constant during execution, while let is more suitable for mutable variables.
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const. Variables declared using the let keyword can be reassigned. The code for this article is available on GitHub. We used the let keyword to declare the variable in the example. Variables declared using let can be ...
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable: js.
i try to read the user input and sent it as a email. but when i run this code it gives me this error: Assignment to constant variable. Any help will be appreciate var mail= require('./email.js') ...
In JavaScript, const is used to declare variables that are meant to remain constant and cannot be reassigned. Therefore, if you try to assign a new value to a constant variable, such as: 1 const myConstant = 10; 2 myConstant = 20; // Error: Assignment to constant variable 3. The above code will throw a "TypeError: Assignment to constant ...
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable:
The “NodeJS TypeError: Assignment to Constant Variable” error, while common, is easily avoidable. By understanding JavaScript’s variable declaration nuances and adopting coding practices that embrace immutability, developers can write more robust and maintainable Node.js applications.
The const keyword is used to declare a variable that can’t be changed after its declaration. This keyword is short for constant (which means “doesn’t change”), and it’s used in a similar manner to the let and var keywords. You write the keyword, followed it up with a variable name, the assignment = operator, and the variable’s value ...
Like the let keyword, var variables can be re-declared and re-assigned a value. The difference between var and let is that var variables are executed within the context they were defined. Either a function scope or a global scope. To understand this better, let's take a look at an example.
Solution 2: Choose a New Variable Name. Another solution is to select a different variable name and declare it as a constant. This is useful when you need to update the value of a variable but want to adhere to the principle of immutability.