Multiple assignment in Python: Assign multiple values or the same value to multiple variables
In Python, the = operator is used to assign values to variables.
You can assign values to multiple variables in one line.
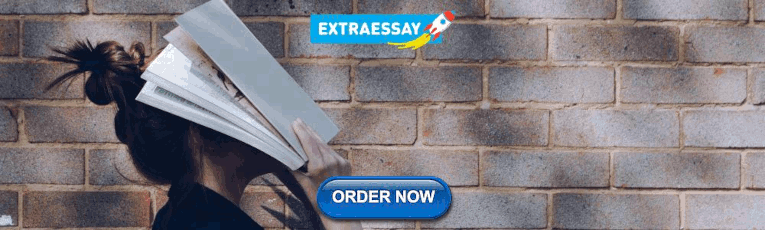
Assign multiple values to multiple variables
Assign the same value to multiple variables.
You can assign multiple values to multiple variables by separating them with commas , .
You can assign values to more than three variables, and it is also possible to assign values of different data types to those variables.
When only one variable is on the left side, values on the right side are assigned as a tuple to that variable.
If the number of variables on the left does not match the number of values on the right, a ValueError occurs. You can assign the remaining values as a list by prefixing the variable name with * .
For more information on using * and assigning elements of a tuple and list to multiple variables, see the following article.
- Unpack a tuple and list in Python
You can also swap the values of multiple variables in the same way. See the following article for details:
- Swap values in a list or values of variables in Python
You can assign the same value to multiple variables by using = consecutively.
For example, this is useful when initializing multiple variables with the same value.
After assigning the same value, you can assign a different value to one of these variables. As described later, be cautious when assigning mutable objects such as list and dict .
You can apply the same method when assigning the same value to three or more variables.
Be careful when assigning mutable objects such as list and dict .
If you use = consecutively, the same object is assigned to all variables. Therefore, if you change the value of an element or add a new element in one variable, the changes will be reflected in the others as well.
If you want to handle mutable objects separately, you need to assign them individually.
after c = []; d = [] , c and d are guaranteed to refer to two different, unique, newly created empty lists. (Note that c = d = [] assigns the same object to both c and d .) 3. Data model — Python 3.11.3 documentation
You can also use copy() or deepcopy() from the copy module to make shallow and deep copies. See the following article.
- Shallow and deep copy in Python: copy(), deepcopy()
Related Categories
Related articles.
- NumPy: arange() and linspace() to generate evenly spaced values
- Chained comparison (a < x < b) in Python
- pandas: Get first/last n rows of DataFrame with head() and tail()
- pandas: Filter rows/columns by labels with filter()
- Get the filename, directory, extension from a path string in Python
- Sign function in Python (sign/signum/sgn, copysign)
- How to flatten a list of lists in Python
- None in Python
- Create calendar as text, HTML, list in Python
- NumPy: Insert elements, rows, and columns into an array with np.insert()
- Shuffle a list, string, tuple in Python (random.shuffle, sample)
- Add and update an item in a dictionary in Python
- Cartesian product of lists in Python (itertools.product)
- Remove a substring from a string in Python
- pandas: Extract rows that contain specific strings from a DataFrame

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assign multiple variables with a Python list values
Depending on the need of the program we may an requirement of assigning the values in a list to many variables at once. So that they can be further used for calculations in the rest of the part of the program. In this article we will explore various approaches to achieve this.
Using for in
The for loop can help us iterate through the elements of the given list while assigning them to the variables declared in a given sequence.We have to mention the index position of values which will get assigned to the variables.
Live Demo
Running the above code gives us the following result −
With itemgetter
The itergetter function from the operator module will fetch the item for specified indexes. We directly assign them to the variables.
With itertools.compress
The compress function from itertools module will catch the elements by using the Boolean values for index positions. So for index position 0,2 and 3 we mention the value 1 in the compress function and then assign the fetched value to the variables.

Related Articles
- How do we assign values to variables in a list using a loop in Python?
- How to assign values to variables in Python
- How to assign multiple values to a same variable in Python?
- Assign multiple variables to the same value in JavaScript?
- How to assign values to variables in C#?
- New ways to Assign values to Variables in C++ 17 ?
- How to assign same value to multiple variables in single statement in C#?
- How do we assign a value to several variables simultaneously in Python?
- How to assign multiple values to same variable in C#?\n
- Assign ids to each unique value in a Python list
- Assign range of elements to List in Python
- Python - Create a dictionary using list with none values
- How to check multiple variables against a value in Python?
- Returning Multiple Values in Python?
- Python Scatter Plot with Multiple Y values for each X
Kickstart Your Career
Get certified by completing the course
To Continue Learning Please Login
Multiple Assignment Syntax in Python
- python-tricks
The multiple assignment syntax, often referred to as tuple unpacking or extended unpacking, is a powerful feature in Python. There are several ways to assign multiple values to variables at once.
Let's start with a first example that uses extended unpacking . This syntax is used to assign values from an iterable (in this case, a string) to multiple variables:
a : This variable will be assigned the first element of the iterable, which is 'D' in the case of the string 'Devlabs'.
*b : The asterisk (*) before b is used to collect the remaining elements of the iterable (the middle characters in the string 'Devlabs') into a list: ['e', 'v', 'l', 'a', 'b']
c : This variable will be assigned the last element of the iterable: 's'.
The multiple assignment syntax can also be used for numerous other tasks:
Swapping Values
This swaps the values of variables a and b without needing a temporary variable.
Splitting a List
first will be 1, and rest will be a list containing [2, 3, 4, 5] .
Assigning Multiple Values from a Function
This assigns the values returned by get_values() to x, y, and z.
Ignoring Values
Here, you're ignoring the first value with an underscore _ and assigning "Hello" to the important_value . In Python, the underscore is commonly used as a convention to indicate that a variable is being intentionally ignored or is a placeholder for a value that you don't intend to use.
Unpacking Nested Structures
This unpacks a nested structure (Tuple in this example) into separate variables. We can use similar syntax also for Dictionaries:
In this case, we first extract the 'person' dictionary from data, and then we use multiple assignment to further extract values from the nested dictionaries, making the code more concise.
Extended Unpacking with Slicing
first will be 1, middle will be a list containing [2, 3, 4], and last will be 5.
Split a String into a List
*split, is used for iterable unpacking. The asterisk (*) collects the remaining elements into a list variable named split . In this case, it collects all the characters from the string.
The comma , after *split is used to indicate that it's a single-element tuple assignment. It's a syntax requirement to ensure that split becomes a list containing the characters.
Cookie Policy
We use cookies to operate this website, improve usability, personalize your experience, and improve our marketing. Privacy Policy .
By clicking "Accept" or further use of this website, you agree to allow cookies.
- Data Science
- Data Analytics
- Machine Learning
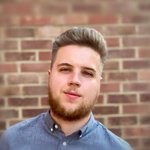
Python List Comprehension: single, multiple, nested, & more
The general syntax for list comprehension in Python is:
Quick Example
We've got a list of numbers called num_list , as follows:
Using list comprehension , we'd like to append any values greater than ten to a new list. We can do this as follows:
This solution essentially follows the same process as using a for loop to do the job, but using list comprehension can often be a neater and more efficient technique. The example below shows how we could create our new list using a for loop.
Using list comprehension instead of a for loop, we've managed to pack four lines of code into one clean statement.
In this article, we'll first look at the different ways to use list comprehensions to generate new lists. Then we'll see what the benefits of using list comprehensions are. Finally, we'll see how we can tackle multiple list comprehensions .
How list comprehension works
A list comprehension works by translating values from one list into another by placing a for statement inside a pair of brackets, formally called a generator expression .
A generator is an iterable object, which yields a range of values. Let's consider the following example, where for num in num_list is our generator and num is the yield.
In this case, Python has iterated through each item in num_list , temporarily storing the values inside of the num variable. We haven't added any conditions to the list comprehension, so all values are stored in the new list.
Conditional statements in list comprehensions
Let's try adding in an if statement so that the comprehension only adds numbers greater than four:
The image below represents the process followed in the above list comprehension:
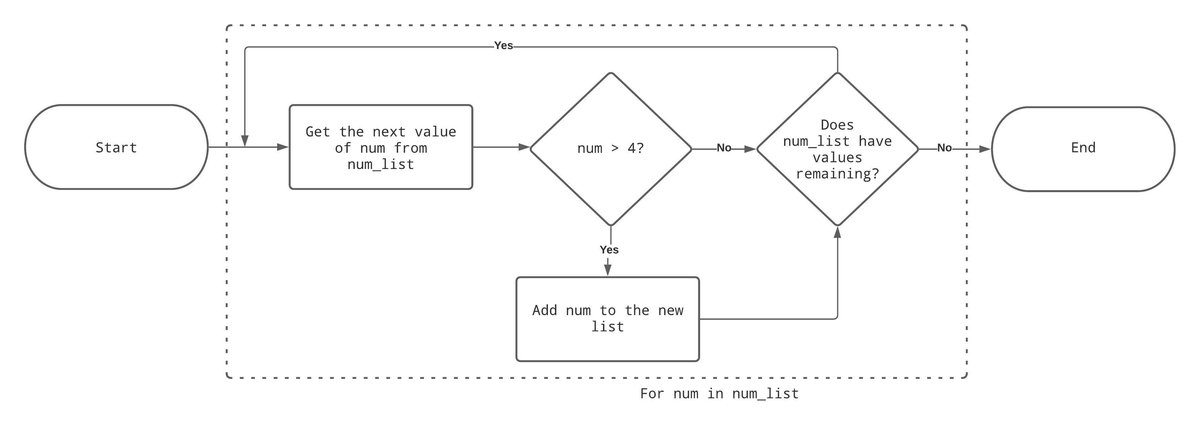
We could even add in another condition to omit numbers smaller than eight. Here, we can use and inside of a list comprehension:
But we could also write this without and as:
When using conditionals, Python checks whether our if statement returns True or False for each yield. When the if statement returns True , the yield is appended to the new list.
Adding functionality to list comprehensions
List comprehensions aren't just limited to filtering out unwanted list values, but we can also use them to apply functionality to the appended values. For example, let's say we'd like to create a list that contains squared values from the original list:
We can also combine any added functionality with comparison operators. We've got a lot of use out of num_list , so let's switch it up and start using a different list for our examples:
In the above example, our list comprehension has squared any values in alternative_list that fall between thirty and fifty. To help demonstrate what's happening above, see the diagram below:
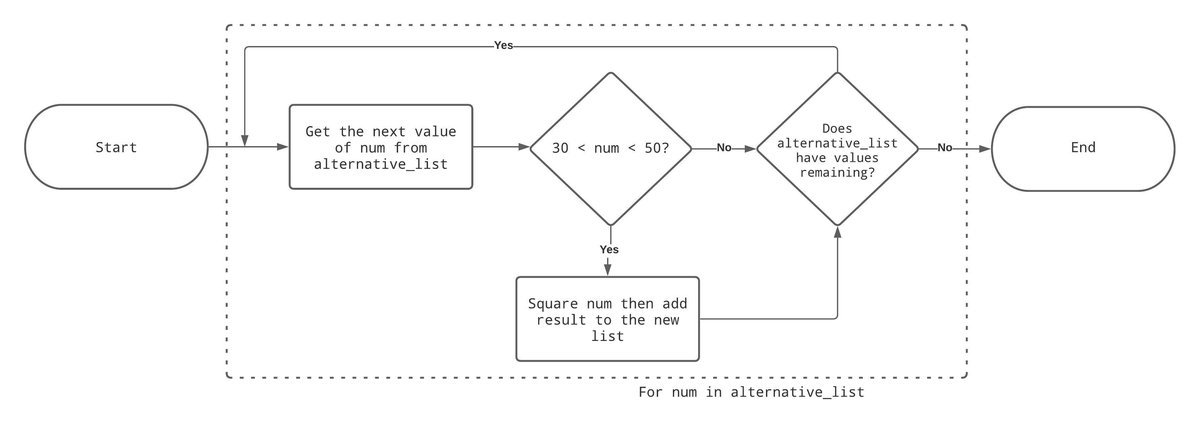
Using comparison operators
List comprehension also works with or , in and not .
Like in the example above using and , we can also use or :
Using in , we can check other lists as well:
Likewise, not in is also possible:
Lastly, we can use if statements before generator expressions within a list comprehension. By doing this, we can tell Python how to treat different values:
The example above stores values in our new list if they are greater than forty; this is covered by num if num > 40 . Python stores zero in their place for values that aren't greater than forty, as instructed by else 0 . See the image below for a visual representation of what's happening:
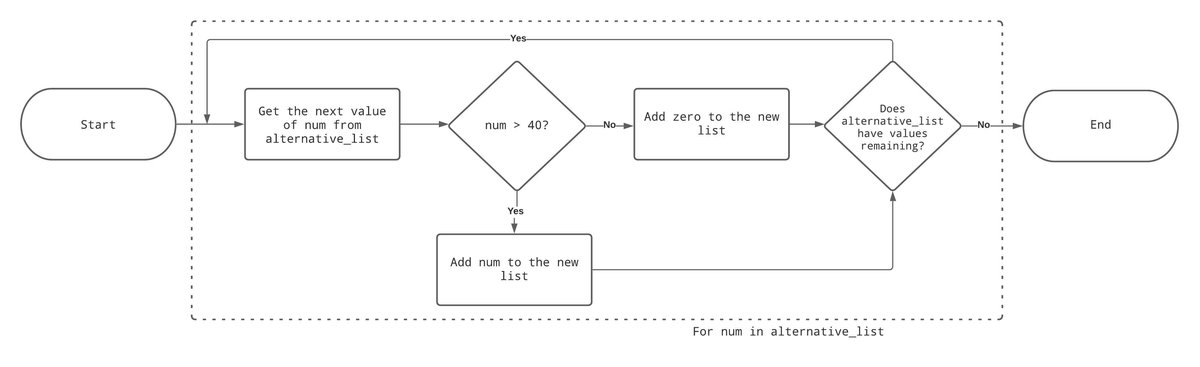
Multiple List Comprehension
Naturally, you may want to use a list comprehension with two lists at the same time. The following examples demonstrate different use cases for multiple list comprehension.
Flattening lists
The following synax is the most common version of multiple list comprehension, which we'll use to flatten a list of lists:
The order of the loops in this style of list comprehension is somewhat counter-intuitive and difficult to remember, so be prepared to look it up again in the future! Regardless, the syntax for flattening lists is helpful for other problems that would require checking two lists for values.
Nested lists
We can use multiple list comprehension when nested lists are involved. Let's say we've got a list of lists populated with string-type values. If we'd like to convert these values from string-type to integer-type, we could do this using multiple list comprehensions as follows:
Readability
The problem with using multiple list comprehensions is that they can be hard to read, making life more difficult for other developers and yourself in the future. To demonstrate this, here's how the first solution looks when combining a list comprehension with a for loop:
Our hybrid solution isn't as sleek to look at, but it's also easier to pick apart and figure out what's happening behind the scenes. There's no limit on how deep multiple list comprehensions can go. If list_of_lists had more lists nested within its nested lists, we could do our integer conversion as follows:
As the example shows, our multiple list comprehensions have now become very difficult to read. It's generally agreed that multiple list comprehensions shouldn't go any deeper than two levels ; otherwise, it could heavily sacrifice readability. To prove the point, here's how we could use for loops instead to solve the problem above:
Speed Test: List Comprehension vs. for loop
When working with lists in Python, you'll likely often find yourself in situations where you'll need to translate values from one list to another based on specific criteria.
Generally, if you're working with small datasets, then using for loops instead of list comprehensions isn't the end of the world. However, as the sizes of your datasets start to increase, you'll notice that working through lists one item at a time can take a long time.
Let's generate a list of ten thousand random numbers, ranging in value from one to a million, and store this as num_list . We can then use a for loop and a list comprehension to generate a new list containing the num_list values greater than half a million. Finally, using %timeit , we can compare the speed of the two approaches:
The list comprehension solution runs twice as fast, so not only does it use less code, but it's also much quicker. With that in mind, it's also worth noting that for loops can be much more readable in certain situations, such as when using multiple list comprehensions.
Ultimately, if you're in a position where multiple list comprehensions are required, it's up to you if you'd prefer to prioritize performance over readability.
List comprehensions are an excellent tool for generating new lists based on your requirements. They're much faster than using a for loop and have the added benefit of making your code look neat and professional.
For situations where you're working with nested lists, multiple list comprehensions are also available to you. The concept of using comprehensions may seem a little complex at first, but once you've wrapped your head around them, you'll never look back!
Get updates in your inbox
Join over 7,500 data science learners.
Recent articles:
The 6 best python courses for 2024 – ranked by software engineer, best course deals for black friday and cyber monday 2024, sigmoid function, dot product, meet the authors.
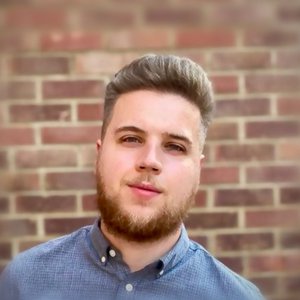
Alfie graduated with a Master's degree in Mechanical Engineering from University College London. He's currently working as Data Scientist at Square Enix. Find him on LinkedIn .

Back to blog index
Unpacking And Multiple Assignment in
About unpacking and multiple assignment.
Unpacking refers to the act of extracting the elements of a collection, such as a list , tuple , or dict , using iteration. Unpacked values can then be assigned to variables within the same statement. A very common example of this behavior is for item in list , where item takes on the value of each list element in turn throughout the iteration.
Multiple assignment is the ability to assign multiple variables to unpacked values within one statement. This allows for code to be more concise and readable, and is done by separating the variables to be assigned with a comma such as first, second, third = (1,2,3) or for index, item in enumerate(iterable) .
The special operators * and ** are often used in unpacking contexts. * can be used to combine multiple lists / tuples into one list / tuple by unpacking each into a new common list / tuple . ** can be used to combine multiple dictionaries into one dictionary by unpacking each into a new common dict .
When the * operator is used without a collection, it packs a number of values into a list . This is often used in multiple assignment to group all "leftover" elements that do not have individual assignments into a single variable.
It is common in Python to also exploit this unpacking/packing behavior when using or defining functions that take an arbitrary number of positional or keyword arguments. You will often see these "special" parameters defined as def some_function(*args, **kwargs) and the "special" arguments used as some_function(*some_tuple, **some_dict) .
*<variable_name> and **<variable_name> should not be confused with * and ** . While * and ** are used for multiplication and exponentiation respectively, *<variable_name> and **<variable_name> are used as packing and unpacking operators.
Multiple assignment
In multiple assignment, the number of variables on the left side of the assignment operator ( = ) must match the number of values on the right side. To separate the values, use a comma , :
If the multiple assignment gets an incorrect number of variables for the values given, a ValueError will be thrown:
Multiple assignment is not limited to one data type:
Multiple assignment can be used to swap elements in lists . This practice is pretty common in sorting algorithms . For example:
Since tuples are immutable, you can't swap elements in a tuple .
The examples below use lists but the same concepts apply to tuples .
In Python, it is possible to unpack the elements of list / tuple / dictionary into distinct variables. Since values appear within lists / tuples in a specific order, they are unpacked into variables in the same order:
If there are values that are not needed then you can use _ to flag them:
Deep unpacking
Unpacking and assigning values from a list / tuple inside of a list or tuple ( also known as nested lists/tuples ), works in the same way a shallow unpacking does, but often needs qualifiers to clarify the values context or position:
You can also deeply unpack just a portion of a nested list / tuple :
If the unpacking has variables with incorrect placement and/or an incorrect number of values, you will get a ValueError :
Unpacking a list/tuple with *
When unpacking a list / tuple you can use the * operator to capture the "leftover" values. This is clearer than slicing the list / tuple ( which in some situations is less readable ). For example, we can extract the first element and then assign the remaining values into a new list without the first element:
We can also extract the values at the beginning and end of the list while grouping all the values in the middle:
We can also use * in deep unpacking:
Unpacking a dictionary
Unpacking a dictionary is a bit different than unpacking a list / tuple . Iteration over dictionaries defaults to the keys . So when unpacking a dict , you can only unpack the keys and not the values :
If you want to unpack the values then you can use the values() method:
If both keys and values are needed, use the items() method. Using items() will generate tuples with key-value pairs. This is because of dict.items() generates an iterable with key-value tuples .
Packing is the ability to group multiple values into one list that is assigned to a variable. This is useful when you want to unpack values, make changes, and then pack the results back into a variable. It also makes it possible to perform merges on 2 or more lists / tuples / dicts .
Packing a list/tuple with *
Packing a list / tuple can be done using the * operator. This will pack all the values into a list / tuple .
Packing a dictionary with **
Packing a dictionary is done by using the ** operator. This will pack all key - value pairs from one dictionary into another dictionary, or combine two dictionaries together.
Usage of * and ** with functions
Packing with function parameters.
When you create a function that accepts an arbitrary number of arguments, you can use *args or **kwargs in the function definition. *args is used to pack an arbitrary number of positional (non-keyworded) arguments and **kwargs is used to pack an arbitrary number of keyword arguments.
Usage of *args :
Usage of **kwargs :
*args and **kwargs can also be used in combination with one another:
You can also write parameters before *args to allow for specific positional arguments. Individual keyword arguments then have to appear before **kwargs .
Arguments have to be structured like this:
def my_function(<positional_args>, *args, <key-word_args>, **kwargs)
If you don't follow this order then you will get an error.
Writing arguments in an incorrect order will result in an error:
Unpacking into function calls
You can use * to unpack a list / tuple of arguments into a function call. This is very useful for functions that don't accept an iterable :
Using * unpacking with the zip() function is another common use case. Since zip() takes multiple iterables and returns a list of tuples with the values from each iterable grouped:
Learn Unpacking And Multiple Assignment
Unlock 3 more exercises to practice unpacking and multiple assignment.
- Python »
- 3.12.3 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |
7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
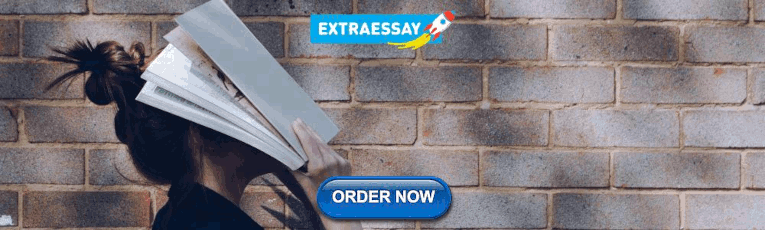
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment expression like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
For expressions as assignment targets, the annotations are evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
Added in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
Adventures in Machine Learning
Efficient python list manipulation: append & insert multiple values.
Python is one of the most popular programming languages today because of its simplicity, versatility, and ease of use. One of Python’s incredible features is its ability to work with lists seamlessly.
Lists are an essential data structure used in Python to store a collection of similar or dissimilar items. They are versatile and can be modified, added to, or deleted from, making them an excellent tool for building Python programs.
This article will explore two critical Python list manipulation techniques: appending multiple values to a list and inserting multiple elements into a list at an index.
1) Append multiple values to a List in Python
Appending several values to a list is a common programming task that Python developers face every day. There are several ways to add multiple values to a python list, depending on the use case.
Using list.extend() method
The list.extend() method in Python is used to append several items to an existing list. This method is highly efficient when working with larger lists containing a vast amount of data.
The syntax of list.extend() is:
list.extend(iterable)
Where iterable is an iterable object to be added to the end of the list. Using for loop and list.append() method
Another way is to iterate through several values and add them one by one using a for loop.
To do this, we use the list.append() method to add each item to the list. Here’s an example:
my_list = []
for i in range(10):
my_list.append(i)
This will add all the values of the range from 0 to 9 to the list.
Using addition (+) operator
We can also use the addition operator to combine two or more lists. To add multiple values, we first create a list using a square bracket, and finally, we use the plus (+) operator to join two or more lists.
Here’s an example:
my_list = [1, 2, 3]
my_list += [4, 5, 6]
This code joins my_list with the list containing the numbers 4, 5, and 6. Using itertools.chain() method
The itertools.chain() method in Python is used to combine several iterables into a single iterable object.
This method can be used to combine multiple lists, tuples, or sets into a single list. Here’s an example:
import itertools
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
combined_list = list(itertools.chain(list1, list2, list3))
This will output [1, 2, 3, 4, 5, 6, 7, 8, 9] as the combined_list.
2) Insert multiple elements into a List at index in Python
Inserting items at a specific index in a Python list can be achieved using several methods.
Using list slicing assignment
The simplest way to insert elements at a specific index is to use list slicing. We slice the original list into two halves, then add the new elements in-between.
The syntax of list slicing is:
my_list[index:index] = [element1, element2, …, elementN]
For example:
my_list = [1, 2, 3, 4, 5]
my_list[2:2] = [11, 12, 13]
This code will add the numbers 11, 12, and 13 to my_list at index 2. Using list.extend() method
The list.extend() method that we introduced earlier can also be used to insert multiple items at an index.
We create a list using square brackets containing the new items to be added, then use the list.extend() method to add them to the desired index. Here’s an example:
my_list[index:index] = [11, 12, 13]
This code successfully inserts the numbers 11, 12, and 13 to my_list at index 1.
The addition operator can also be used to insert multiple elements into a Python list. We use slicing to split the list into two halves and add the new elements in-between.
my_list = my_list[:index] + [11, 12, 13] + my_list[index:]
This line of code will add numbers 11, 12, and 13 into my_list at index 2.
Python is an excellent programming language, mainly because of its ability to manipulate lists and collections of data efficiently. This article highlights four crucial methods for appending and inserting items to lists in Python.
By using these techniques, developers can save time, manipulate data with ease and become more productive. With the plethora of Python libraries available today, there is no limit to what developers can do with Python.
Would you like to start writing Python? Start by learning how to manipulate Python lists using these methods.
In summary, this article explores the techniques used to append and insert multiple values into Python lists. The four methods discussed include using list.extend() method, for loop and list.append() method, addition (+) operator, and itertools.chain() method to append.
For insertion, list slicing assignment, list.extend() method, and addition operator are used. Understanding how to manipulate lists in Python is crucial for developers, providing easier handling of data and boosting productivity.
Whether a novice or a seasoned developer, familiarizing oneself with these techniques is critical for effective Python programming.
Popular Posts
Unlocking the power of primary and foreign keys in relational databases, enhance readability: adding commas to integer strings in python, mastering sql databases and data engineering: essential resources and career path.
- Terms & Conditions
- Privacy Policy
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python lists.
Lists are used to store multiple items in a single variable.
Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple , Set , and Dictionary , all with different qualities and usage.
Lists are created using square brackets:
Create a List:
List items are ordered, changeable, and allow duplicate values.
List items are indexed, the first item has index [0] , the second item has index [1] etc.
When we say that lists are ordered, it means that the items have a defined order, and that order will not change.
If you add new items to a list, the new items will be placed at the end of the list.
Note: There are some list methods that will change the order, but in general: the order of the items will not change.
The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
Allow Duplicates
Since lists are indexed, lists can have items with the same value:
Lists allow duplicate values:
Advertisement
List Length
To determine how many items a list has, use the len() function:
Print the number of items in the list:
List Items - Data Types
List items can be of any data type:
String, int and boolean data types:
A list can contain different data types:
A list with strings, integers and boolean values:
From Python's perspective, lists are defined as objects with the data type 'list':
What is the data type of a list?
The list() Constructor
It is also possible to use the list() constructor when creating a new list.
Using the list() constructor to make a List:
Python Collections (Arrays)
There are four collection data types in the Python programming language:
- List is a collection which is ordered and changeable. Allows duplicate members.
- Tuple is a collection which is ordered and unchangeable. Allows duplicate members.
- Set is a collection which is unordered, unchangeable*, and unindexed. No duplicate members.
- Dictionary is a collection which is ordered** and changeable. No duplicate members.
*Set items are unchangeable, but you can remove and/or add items whenever you like.
**As of Python version 3.7, dictionaries are ordered . In Python 3.6 and earlier, dictionaries are unordered .
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python | Initializing multiple lists
- Python Initialize List of Lists
- Python | Interleave multiple lists of same length
- Python - K Matrix Initialization
- Python | Append multiple lists at once
- Are Python Lists Mutable
- Python | Boolean list initialization
- Python - Constant Multiplication over List
- Merge Multiple Lists into one List
- Python | Interval Initialization in list
- How To Combine Multiple Lists Into One List Python
- Python | Initialize dictionary with multiple keys
- Indexing Lists Of Lists In Python
- Get Index of Multiple List Elements in Python
- Convert List of Tuples To Multiple Lists in Python
- Python - All possible pairs in List
- Python | Iterating two lists at once
- Iterate Over a List of Lists in Python
- Python | Which is faster to initialize lists?
- Print a List in Python Horizontally
In real applications, we often have to work with multiple lists, and initialize them with empty lists hampers the readability of code. Hence a one-liner is required to perform this task in short so as to give a clear idea of the type and number of lists declared to be used.
Method #1: Using loops
We can enlist all the required list comma separated and then initialize them with a loop of empty lists.
Time complexity: O(n), where n is the number of lists to be initialized.
Auxiliary space: O(n), where n is the number of lists to be initialized.
Method #2: Using defaultdict() Method
This is a method different and also performs a slightly different utility than the above two methods discussed. This creates a dictionary with a specific name and we have the option to make any number of keys and perform the append operations straight away as they get initialized by the list.
Time Complexity: O(n), where n is the length of the input list. This is because we’re using defaultdict() which has a time complexity of O(n) in the worst case. Auxiliary Space: O(1), as we’re using constant additional space.
Method #3: Using * operator:
It does not create independent lists, but variables referring to the same (empty) list!
Method #4: Using repeat:
To initialize multiple lists using the repeat method, you can do the following:
The time complexity of this method is O(n), where n is the number of lists you want to create.
Method 5: use a dictionary with list values to store the lists.
This method allows for easy access to the lists using their keys and avoids the need to create four separate variables. It can be particularly useful when dealing with a large number of lists or when the number of lists is not known beforehand.
Time complexity: O(1), so the total time complexity of the four operations is also O(1). Auxiliary space: O(1) because the size of the dictionary does not depend on the size of the input data.
Please Login to comment...
Similar reads.
- Python list-programs
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
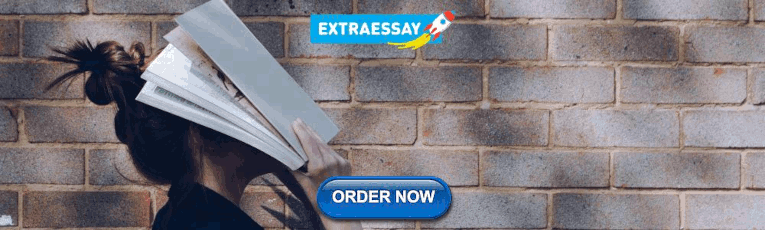
IMAGES
VIDEO
COMMENTS
Unpack a tuple and list in Python; You can also swap the values of multiple variables in the same way. See the following article for details: Swap values in a list or values of variables in Python; Assign the same value to multiple variables. You can assign the same value to multiple variables by using = consecutively.
4. Python employs assignment unpacking when you have an iterable being assigned to multiple variables like above. In Python3.x this has been extended, as you can also unpack to a number of variables that is less than the length of the iterable using the star operator: >>> a,b,*c = [1,2,3,4] >>> a. 1. >>> b. 2.
Method #4: Using dictionary unpacking Approach. using dictionary unpacking. We can create a dictionary with keys corresponding to the variables and values corresponding to the indices we want, and then unpack the dictionary using dictionary unpacking.
And you can assign the same value to multiple variables in one line: Example. x = y = z = "Orange" ... print(y) print(z) Try it Yourself » Unpack a Collection. If you have a collection of values in a list, tuple etc. Python allows you to extract the values into variables. This is called unpacking. Example. Unpack a list: fruits = ["apple ...
Python's list is a flexible, versatile, powerful, and popular built-in data type. It allows you to create variable-length and mutable sequences of objects. In a list, you can store objects of any type. You can also mix objects of different types within the same list, although list elements often share the same type.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Assign multiple variables with a Python list values - Depending on the need of the program we may an requirement of assigning the values in a list to many variables at once. So that they can be further used for calculations in the rest of the part of the program. In this article we will explore various approaches to achieve this.Using for inThe for loo
The multiple assignment syntax, often referred to as tuple unpacking or extended unpacking, is a powerful feature in Python. There are several ways to assign multiple values to variables at once. Let's start with a first example that uses extended unpacking. This syntax is used to assign values from an iterable (in this case, a string) to ...
We've got a list of numbers called num_list, as follows: num_list = [4, 11, 2, 19, 7, 6, 25, 12] Learn Data Science with. Using list comprehension, we'd like to append any values greater than ten to a new list. We can do this as follows: new_list = [num for num in num_list if num > 10] new_list. Learn Data Science with.
About Unpacking And Multiple Assignment. Unpacking refers to the act of extracting the elements of a collection, such as a list, tuple, or dict, using iteration. Unpacked values can then be assigned to variables within the same statement. A very common example of this behavior is for item in list, where item takes on the value of each list ...
Python assigns values from right to left. When assigning multiple variables in a single line, different variable names are provided to the left of the assignment operator separated by a comma. The same goes for their respective values except they should be to the right of the assignment operator. While declaring variables in this fashion one ...
The difference is not in the multiple assignment, but in what you subsequently do with the objects. With the int, you do +=, and with the list you do .append. However, even if you do += for both, you won't necessarily see the same result, because what += does depends on what type you use it on. So that is the basic answer: operations like ...
Lists and tuples are arguably Python's most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here's what you'll learn in this tutorial: You'll cover the important characteristics of lists and tuples. You'll learn how to define them and how to manipulate them.
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right. Assignment is defined recursively depending on the form of the target (list).
my_list.append (i) This will add all the values of the range from 0 to 9 to the list. Using addition (+) operator. We can also use the addition operator to combine two or more lists. To add multiple values, we first create a list using a square bracket, and finally, we use the plus (+) operator to join two or more lists.
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
Python multiple assignment and references. 29. Assign multiple values of a list. 1. Python list assign by value. 1. Python list assignation. 0. Assigning lists to eachother in Python. 2. List assignment in python. 1. Python assignment in just one line. 1. Python List Comprehension: assign to multiple variables.
Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets:
Oct 6, 2011 at 16:20. 2. list[:] specifies a range within the list, in this case it defines the complete range of the list, i.e. the whole list and changes them. list=range(100), on the other hand, kind of wipes out the original contents of list and sets the new contents. But try the following:
Method #1: Using loops. We can enlist all the required list comma separated and then initialize them with a loop of empty lists. Time complexity: O (n), where n is the number of lists to be initialized. Auxiliary space: O (n), where n is the number of lists to be initialized. Method #2: Using defaultdict () Method.
One case when you need to include more structure on the left hand side of the assignment is when you're asking Python unpack a slightly more complicated sequence. E.g.: File "<stdin>", line 1, in <module>. This has proved useful for me in the past, for example, when using enumerate to iterate over a sequence of 2-tuples.
return trade_result_list + blotter_result_list returns one list, created by concatenating those two lists together. If you want to return multiple values, you separate them with ,, not +. - Barmar. 2 days ago. But the type annotation says it only returns one list: -> List[Union[TradeResult, BlotterResults]] - Barmar.