Ternary Operator in C Explained
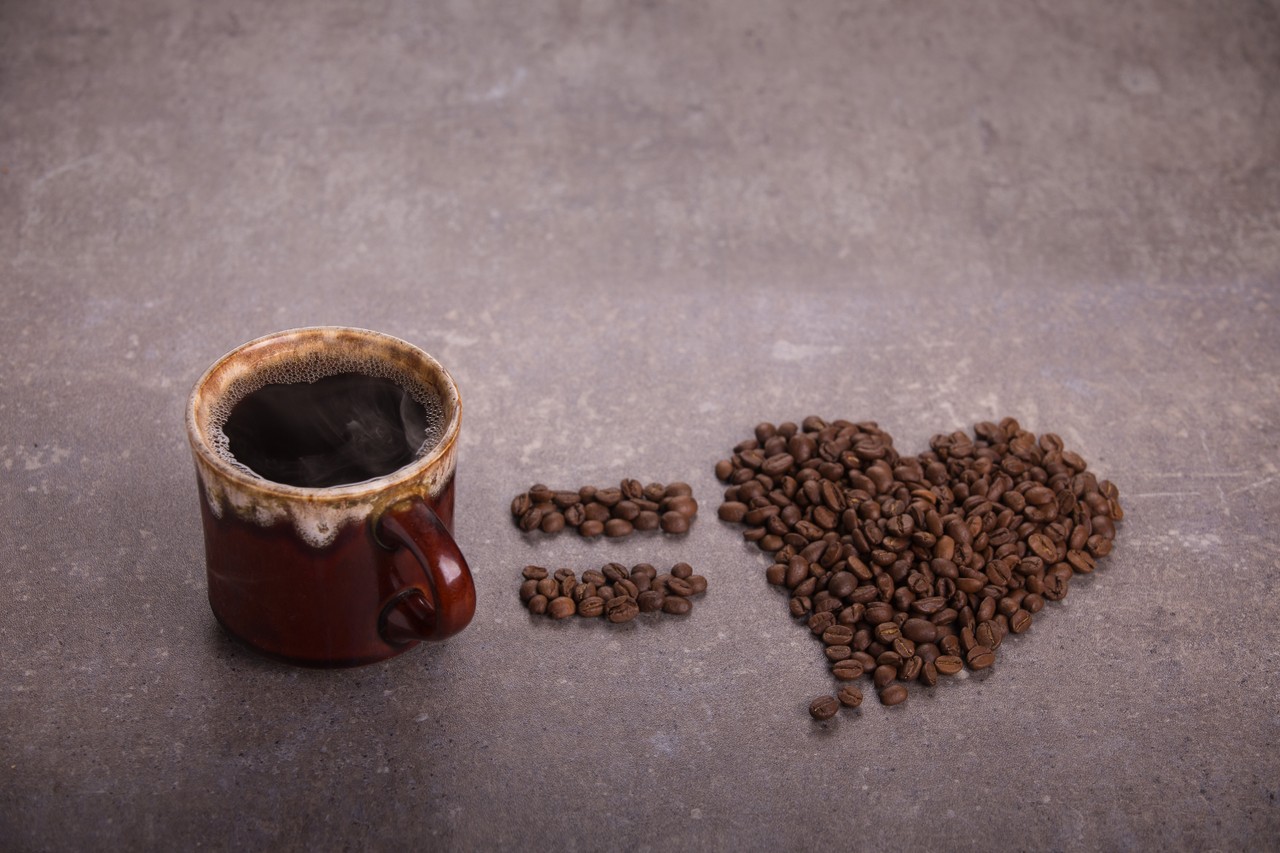
Programmers use the ternary operator for decision making in place of longer if and else conditional statements.
The ternary operator take three arguments:
- The first is a comparison argument
- The second is the result upon a true comparison
- The third is the result upon a false comparison
It helps to think of the ternary operator as a shorthand way or writing an if-else statement. Here’s a simple decision-making example using if and else :
This example takes more than 10 lines, but that isn’t necessary. You can write the above program in just 3 lines of code using a ternary operator.
condition ? value_if_true : value_if_false
The statement evaluates to value_if_true if condition is met, and value_if_false otherwise.
Here’s the above example rewritten to use the ternary operator:
Output of the example above should be:
c is set equal to a , because the condition a < b was true.
Remember that the arguments value_if_true and value_if_false must be of the same type, and they must be simple expressions rather than full statements.
Ternary operators can be nested just like if-else statements. Consider the following code:
Here's the code above rewritten using a nested ternary operator:
The output of both sets of code above should be:
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
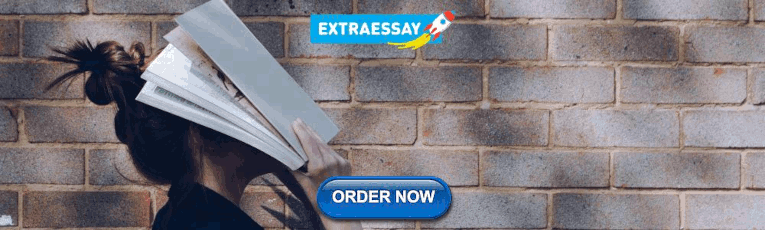
C Ternary Operator (With Examples)
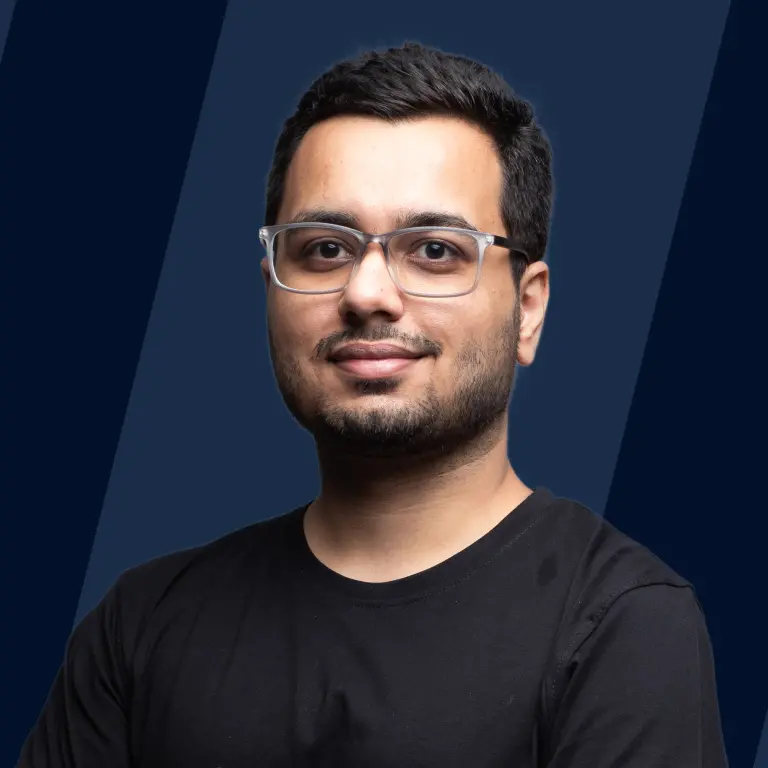
The C ternary operator, often represented as exp1 ? exp2 : exp3, is a valuable tool for making conditional decisions in C programming. This compact operator evaluates a condition and performs one of two expressions based on whether the condition is true or false. With its concise syntax and flexibility, the ternary operator is especially useful for streamlining conditional assignments.
The syntax of the Ternary Operator in C is:
Working of Syntax :
- If the condition in the ternary operator is met (true), then the exp2 executes.
- If the condition is false, then the exp3 executes.
Example of C Ternary Operator
The following example explains the working of Ternary Operator in C.
so, if the condition 10 > 15 is true (which is false in this case) mxNumber is initialized with the value 10 otherwise with 15 . As the condition is false so mxNumber will contain 15 . This is how Ternary Operator in C works.
NOTE: Ternary operator in C, like if-else statements, can be nested.
Assign the Ternary Operator to a Variable:
You can assign the result of the ternary operator to a variable, as shown in the previous example with the variable max. This is a common use case for the ternary operator, where the result of the condition is stored in a variable for later use.
Ternary Operator Vs. if...else Statement in C:
The ternary operator and the if...else statement serve similar purposes but differ in syntax and use cases.
- The C Ternary Operator is represented as exp1 ? exp2 : exp3 , providing a concise way to make conditional decisions.
- If the condition exp1 is true, exp2 executes; otherwise, exp3 executes.
- It's useful for simple conditional assignments and can improve code readability in such cases.
- For more complex conditions, the if...else statement may be a better choice.
- Understanding the ternary operator enhances your ability to write concise and expressive C code for conditional operations.
Content Fusion: Uniting Bloggers for Shared Success on Buzziova
Mastering the Ternary Operator in C: A Comprehensive Guide
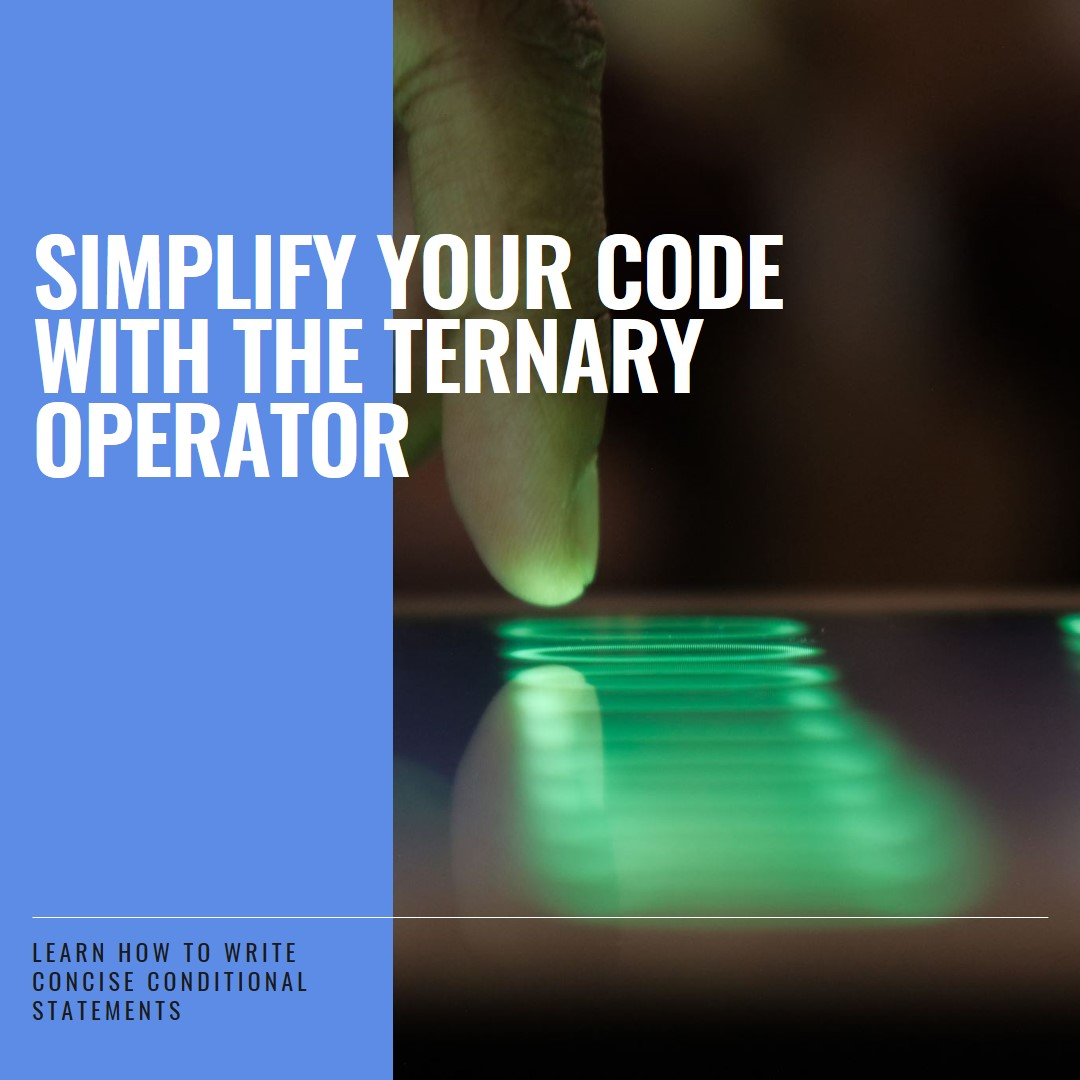
The ternary operator in C is a powerful and concise tool that allows conditional expressions to be written in a compact form. It provides a means to streamline decision-making within code, enhancing readability and efficiency. In this comprehensive guide, we’ll explore the nuances, applications, and best practices associated with the ternary operator in C , empowering you to leverage its full potential in your programming endeavors, courtesy of Scholarhat.
Understanding the Ternary Operator
The ternary operator, denoted by the ‘?’ symbol in C programming, is a conditional operator that evaluates an expression and returns a value based on whether the expression is true or false. Its syntax follows the format: condition ? expression1 : expression2 . Here, if the condition is true, expression1 is executed; otherwise, expression2 is executed. This operator acts as a shorthand form of an if-else statement, enabling more concise code representation in certain scenarios.
Mechanics of the Ternary Operator
The ternary operator operates by evaluating the condition provided. If the condition resolves to true, it executes the expression before the ‘:’ symbol; if false, it executes the expression after the ‘:’ symbol. For instance, consider an example: int result = (a > b) ? a : b; . In this case, if ‘a’ is greater than ‘b’, the value of ‘result’ becomes ‘a’; otherwise, it becomes ‘b’.
Advantages of Using the Ternary Operator
The use of the ternary operator in C offers several advantages. Firstly, it aids in writing more concise and readable code. It condenses the if-else statement into a single line, making the code easier to understand, especially for simpler conditional expressions. Secondly, it can enhance code efficiency by reducing the number of lines and avoiding the need for repetitive if-else structures.
Applying the Ternary Operator in C
The ternary operator finds application in various scenarios within C programming. It is particularly useful when assigning values to variables based on conditions. For example, it can be utilized in functions to return different values depending on certain conditions without the need for an extensive if-else block. Additionally, it is commonly employed in conditional assignments, where the value of a variable depends on a condition.
Best Practices and Considerations
While the ternary operator offers succinctness, it’s essential to use it judiciously. Overusing it in complex expressions can lead to reduced code readability and maintainability. It’s crucial to prioritize code clarity over brevity in situations where complex conditions might compromise readability. Additionally, the ternary operator doesn’t support multiple statements within its branches, unlike the if-else statement, which allows multiple lines of code in each branch.
Nested Ternary Operators
One of the advanced aspects of the ternary operator is its capability to be nested within itself. This allows for multiple conditions to be evaluated in a compact manner. For example, result = (a > b) ? ((a > c) ? a : c) : ((b > c) ? b : c); This nested structure enables handling more complex scenarios where multiple conditions need to be checked and values assigned based on these conditions.
Usage in Conditional Statements
The ternary operator is frequently employed within conditional statements. For instance, it can be used to control the flow of execution within loops or functions. By incorporating the ternary operator, developers can succinctly handle conditional checks within these structures, enhancing code readability and reducing the complexity of the logic.
Handling Null Checks and Assignments
In C programming, the ternary operator can also be used for null checks and assignments. It’s often utilized to assign default values to variables when dealing with potential null pointers or uninitialized variables. For instance, int value = (ptr != NULL) ? *ptr : defaultValue; This concise syntax enables efficient handling of potential null situations, preventing unexpected behavior within the code.
Ternary Operator vs. If-Else Statements
While the ternary operator offers brevity and compactness, it’s important to understand its limitations compared to traditional if-else statements. Complex conditions or scenarios that involve multiple lines of code are generally better suited for if-else constructs due to their ability to accommodate larger code blocks within each branch. Additionally, if-else statements provide better readability for intricate logic structures compared to nested ternary operators.
Performance Considerations
In terms of performance, the ternary operator generally doesn’t offer significant advantages over if-else statements. Compilers often optimize both constructs similarly, and the choice between them usually revolves around code readability and maintainability rather than performance gains.
Ternary Operator in Function Arguments
The ternary operator can also be used effectively within function arguments. It allows for conditional evaluation of expressions passed as arguments to functions. This application ensures that the correct value or operation is passed to the function based on the condition, enhancing flexibility and conciseness in function calls.
Ternary Operator and Bitwise Operations
In C, the ternary operator can be combined with bitwise operations to perform conditional bitwise manipulations. For instance, result = (condition) ? (a | b) : (a & b); Here, depending on the condition, either a bitwise OR or a bitwise AND operation between ‘a’ and ‘b’ will be performed, and the result will be assigned to ‘result’. This integration allows for efficient handling of bitwise operations based on specific conditions.
Ternary Operator for String Concatenation
Although C doesn’t have native string concatenation using the ‘+’ operator as in some other languages, the ternary operator can be employed creatively to concatenate strings conditionally. By using the ternary operator with the strcat() function, developers can concatenate strings based on specific conditions. For example, strcat(result, (condition) ? "String1" : "String2"); This succinct approach facilitates string concatenation without needing complex if-else blocks.
Using Ternary Operator in Macros
Macros in C are preprocessor directives that allow for the definition of reusable code snippets. The ternary operator can be effectively utilized within macros to create conditional code blocks. This can help streamline and make macro definitions more versatile and adaptable to various conditions, improving code maintainability and reducing redundancy.
Ternary Operator in Array Indexing
The ternary operator can also be used for conditional array indexing. It allows for the selection of array elements based on specific conditions. For instance, int result = (condition) ? arr[index1] : arr[index2]; This operation selects either ‘arr[index1]’ or ‘arr[index2]’ based on the condition and assigns it to the ‘result’ variable. This usage simplifies conditional array access within the code.
Using Ternary Operator for Assignment in Loops
In loops, particularly when dealing with ranges or iterations, the ternary operator can aid in concise assignment statements. For instance, array[i] = (i % 2 == 0) ? evenValue : oddValue; This line of code assigns different values to array elements based on the index being even or odd, providing a compact and readable representation of conditional assignments within loops.
Conclusion: Mastering the Ternary Operator
The ternary operator in C serves as a valuable tool for enhancing code clarity and efficiency. Understanding its mechanics and best practices empowers programmers to write concise yet readable code. Leveraging the ternary operator, developers can streamline conditional expressions and make code more expressive, contributing to efficient and maintainable programming practices.
Also know about
Understanding Recursion in C Programming for Enhanced Problem Solving
charlottelee
Related posts.
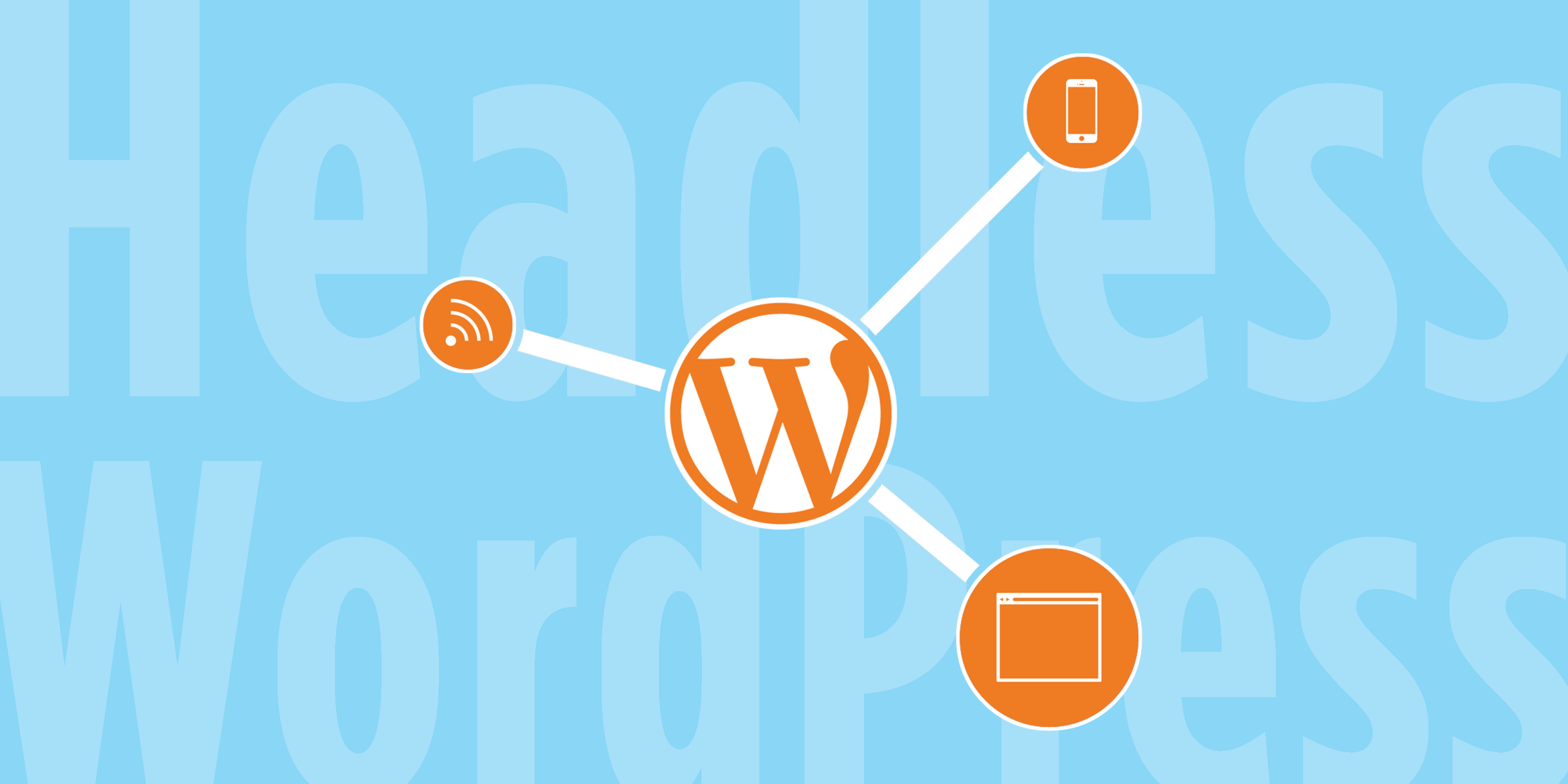
Unleashing the Power: WordPress as a Headless CMS
Do you realize what your website could achieve? Have you any idea of its true potential? Have you ever heard…
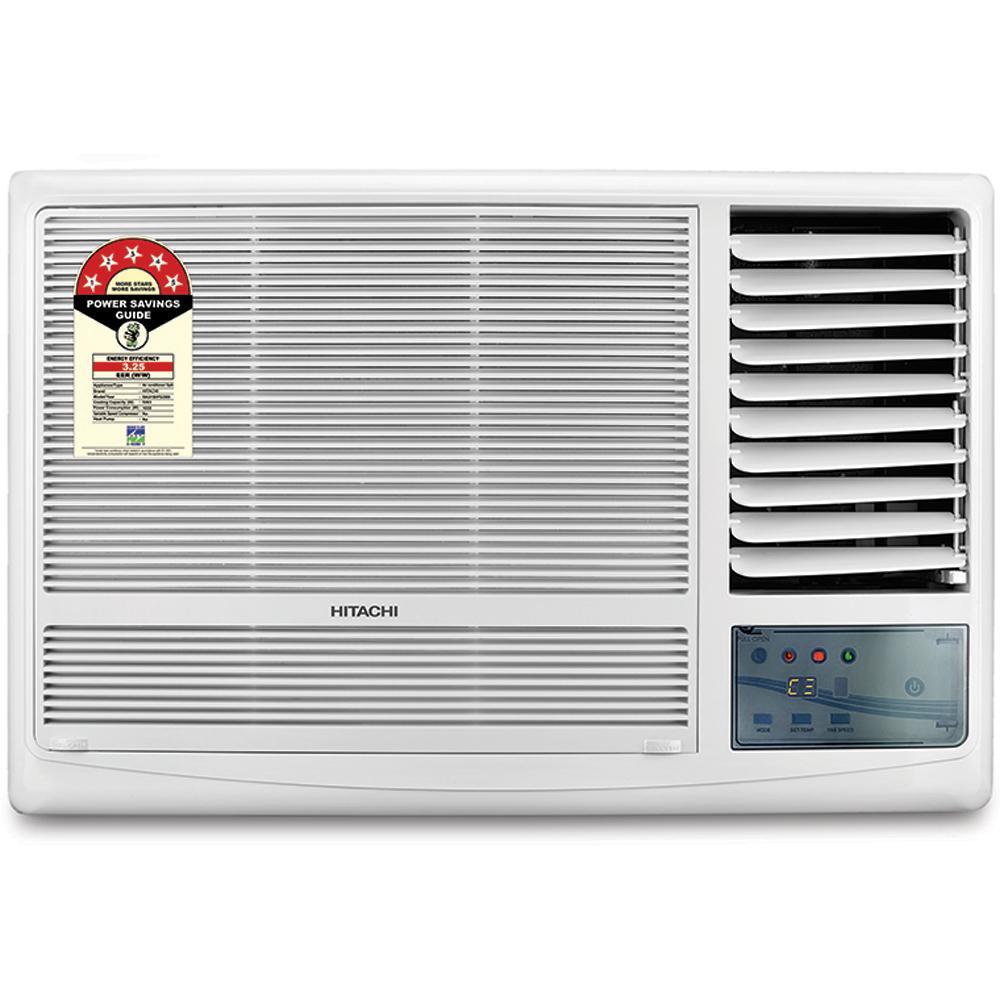
Hitachi Window AC Price 1.5 Ton 3 Star: Your Ultimate Buying Guide
In the realm of home cooling solutions, Hitachi window ACs stand out for their superior performance, energy efficiency, and durability.…
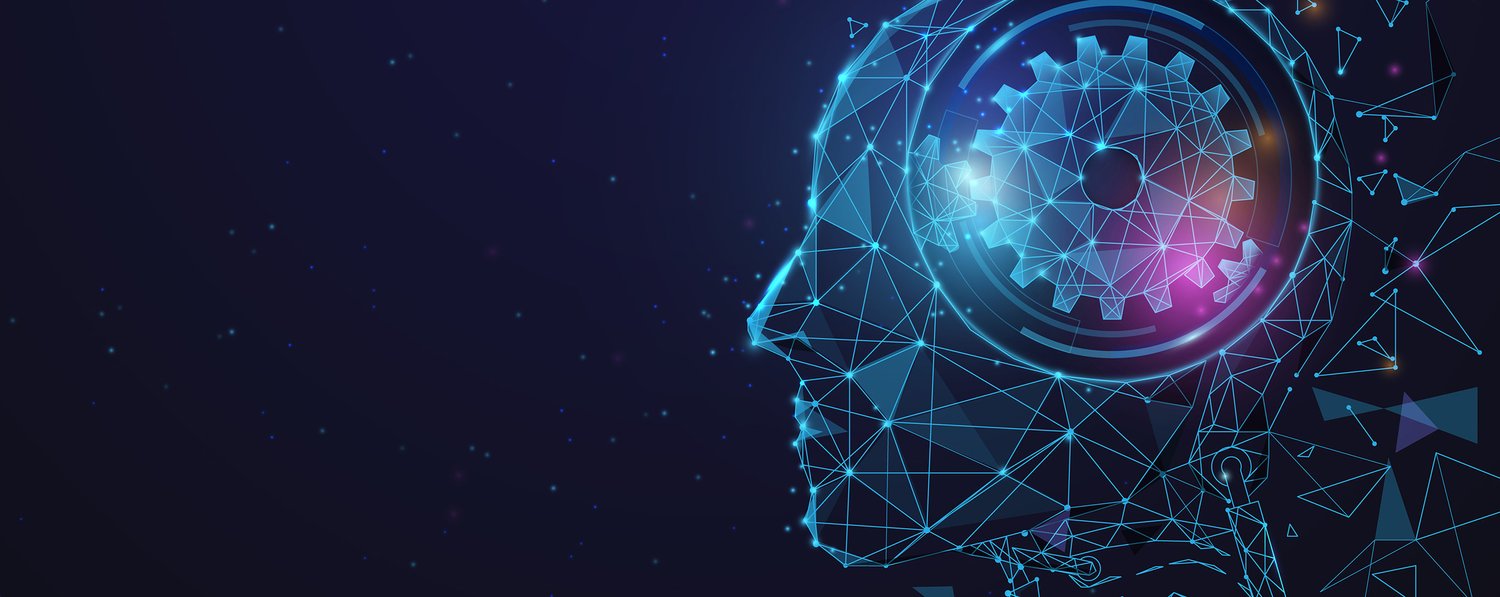
The Essential Role of Upskilling in the Age of Automation and AI
The current workforce is at a crossroads, brought about by unparalleled disruptions caused by rapid technological changes, especially in automation…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Ternary Operators in C self.__wrap_b=(t,n,e)=>{e=e||document.querySelector(`[data-br="${t}"]`);let a=e.parentElement,r=R=>e.style.maxWidth=R+"px";e.style.maxWidth="";let o=a.clientWidth,c=a.clientHeight,i=o/2-.25,l=o+.5,u;if(o){for(;i+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(a):process.env.NODE_ENV==="development"&&console.warn("The browser you are using does not support the ResizeObserver API. Please consider add polyfill for this API to avoid potential layout shifts or upgrade your browser. Read more: https://github.com/shuding/react-wrap-balancer#browser-support-information"))};self.__wrap_b(":R4mr36:",1)
Introduction to Ternary Operator
Syntax and usage, practical examples, comparison with if-else statements, common pitfalls and best practices.

In the realm of C programming, the ternary operator emerges as a powerful yet often underutilized tool. Unlike its more verbose counterparts, the ternary operator offers a succinct and elegant way to express conditional logic. This compact form of writing conditional statements not only enhances readability but also streamlines the code-writing process, making it a valuable asset for any C programmer.
The ternary operator is a conditional operator that serves as a concise alternative to the traditional if-else statements. It is called “ternary” because it involves three operands: a condition, a result upon the condition being true, and a result upon the condition being false. The syntax of the ternary operator in C is as follows:
If the condition evaluates to true, expression1 is executed, otherwise, expression2 is executed.
Definition and Syntax
At its core, the ternary operator is defined by its simple yet expressive syntax. This syntax enables developers to perform conditional operations in a single line of code, which can significantly reduce the complexity and improve the readability of the code.
While if-else statements are straightforward and familiar to most programmers, they often lead to more verbose code, especially for simple conditions. The ternary operator, with its compact syntax, offers a neater alternative. For example, assigning a value based on a simple condition requires at least four lines of code with if-else but just one with the ternary operator.
Advantages of Using Ternary Operators
The primary advantages of using ternary operators include code compactness and improved readability, especially for simple conditional assignments and decisions. They are particularly useful for inline operations and can make code easier to understand at a glance, which is a boon for both the writer and subsequent reviewers.
Understanding the syntax and operational logic of the ternary operator is crucial for its effective use. The operator takes three operands: a condition to evaluate, an expression to execute if the condition is true, and an expression to execute if the condition is false.
Basic Usage
A common use case for the ternary operator is in simple variable assignments based on a condition. For example:
This code snippet succinctly assigns the greater of two values to the max variable.
Condition Evaluation
The ternary operator evaluates the condition first. Based on this evaluation, it decides which of the two expressions ( expression1 or expression2 ) to execute. The decision is binary—only one of the two expressions will be executed, never both.
The real-world applications of ternary operators are vast and varied, often involving scenarios where simple decisions need to be made quickly and efficiently.
Simple Variable Assignment
Consider the task of assigning a status message based on the value of a numeric variable:
Nesting ternary operators can significantly compact the code but at the cost of readability. Consider this example where we want to categorize the age of a person:
While concise, nested ternary operators can lead to confusion, especially if not formatted properly. It’s crucial to balance the use of such constructs to maintain code clarity.
Use in Function Calls
Ternary operators shine in making function calls more concise. For instance, deciding between two functions based on a condition:
This approach minimizes the need for additional if-else blocks, leading to cleaner and more straightforward code.
Side-by-Side Comparison
Let’s compare ternary operators with if-else statements. For determining if a number is positive or negative:
// Using if-else char * result ; if ( num > 0 ) { result = "Positive" ; } else { result = "Negative" ; }
The ternary operator variant is more succinct, ideal for simple conditions.
When to Use Ternary Over If-Else
Ternary operators are preferable for simple, concise conditions where the operation or assignment is straightforward. They are particularly useful in assignments and function arguments, where using if-else would introduce unnecessary complexity.
Impact on Readability and Maintainability
While ternary operators can make code more concise, overusing them or applying them in complex conditions can harm readability and maintainability. It’s vital to use them judiciously, ensuring that the code remains clear and understandable.
Common Mistakes
Common mistakes include nesting ternary operators excessively and using them in places where an if-else statement would be more readable and maintainable. Misusing ternary operators can make code difficult to understand and debug.
Best Practices
To use ternary operators without sacrificing clarity:
- Avoid deep nesting.
- Use them in simple conditions or assignments.
- Ensure the code remains readable and understandable.
Sharing is caring
Did you like what Rishabh Rao wrote? Thank them for their work by sharing it on social media.
No comment s so far
Curious about this topic? Continue your journey with these coding courses:
Surendra varma Pericherla
C Programming Fundamentals
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?: operator - the ternary conditional operator
- 11 contributors
The conditional operator ?: , also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false , as the following example shows:
As the preceding example shows, the syntax for the conditional operator is as follows:
The condition expression must evaluate to true or false . If condition evaluates to true , the consequent expression is evaluated, and its result becomes the result of the operation. If condition evaluates to false , the alternative expression is evaluated, and its result becomes the result of the operation. Only consequent or alternative is evaluated. Conditional expressions are target-typed. That is, if a target type of a conditional expression is known, the types of consequent and alternative must be implicitly convertible to the target type, as the following example shows:
If a target type of a conditional expression is unknown (for example, when you use the var keyword) or the type of consequent and alternative must be the same or there must be an implicit conversion from one type to the other:
The conditional operator is right-associative, that is, an expression of the form
is evaluated as
You can use the following mnemonic device to remember how the conditional operator is evaluated:
Conditional ref expression
A conditional ref expression conditionally returns a variable reference, as the following example shows:
You can ref assign the result of a conditional ref expression, use it as a reference return or pass it as a ref , out , in , or ref readonly method parameter . You can also assign to the result of a conditional ref expression, as the preceding example shows.
The syntax for a conditional ref expression is as follows:
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative .
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed.
Conditional operator and an if statement
Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following example demonstrates two ways to classify an integer as negative or nonnegative:
Operator overloadability
A user-defined type can't overload the conditional operator.
C# language specification
For more information, see the Conditional operator section of the C# language specification .
Specifications for newer features are:
- Target-typed conditional expression
- Simplify conditional expression (style rule IDE0075)
- C# reference
- C# operators and expressions
- if statement
- ?. and ?[] operators
- ?? and ??= operators
- ref keyword
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Software Engineering
- Ankit Mittal Created by
- 4 (445 ratings)
- 28 Comments
- 01/03/2024 Last Updated
1. Introduction
2. History of C Language
3. Features of C Language
4. Use of C Language
5. C Language Download
6. Coding Vs. Programming
7. Structures in C
8. Difference Between Compiler and Interpreter
9. Difference Between Arguments And Parameters
10. C Program to Find ASCII Value of a Character
11. Define And include in C
12. What is Variables in C
13. Boolean in C
14. Conditional Statements in C
15. Constants in C
16. Data Types in C
17. Switch Case in C
18. Data Structures in C
19. C Compiler for Windows
20. C Compiler for Mac
21. Compilation process in C
22. Storage Classes in C
23. Array in C
24. One Dimensional Array in C
25. Two Dimensional Array in C
26. Dynamic Array in C
27. Array of Structure in C
28. Length of an Array in C
29. Array of Pointers in C
30. If Else Statement in C
31. Nested if else statement in C
32. Do While Loop In C
33. Nested Loop in C
34. For Loop in C
35. Difference Between If Else and Switch
36. If Statement in C
37. Operators in C
38. Bitwise Operators in C
39. C Ternary Operator
40. Logical Operators in C
41. Increment and decrement operators in c
42. Conditional operator in the C
43. Relational Operators in C
44. Assignment Operator in C
45. Unary Operator in C
46. Operator Precedence and Associativity in C
47. String Functions in C
48. String Input Output Functions in C
49. Function Pointer in C
50. Functions in C
51. Input and Output Functions in C
52. User Defined Functions in C
53. C Function Call Stack
54. Static function in C
55. Library Function in C
56. Toupper Function in C
57. Ceil Function in C
58. C string declaration
59. String Length in C
60. String Comparison in C
61. Pointers in C
62. Dangling Pointer in C
63. Pointer to Pointer in C
64. Constant Pointer in C
65. String Pointer in C
66. File Handling in C
67. Header Files in C
68. Stack in C
69. Stack Using Linked List in C
70. Linked list in C
71. Implementation of Queue Using Linked List
72. Heap Sort in C Program
73. Tokens in C
74. Enumeration (or enum) in C
75. Format Specifiers in C
76. Strcpy in C
77. Type Casting in C
78. Stdio.h in C
79. Transpose of a Matrix in C
80. Jump Statements in C
81. goto statement in C
82. Double In C
83. Comments in C
84. Types of Error in C
85. strcat() in C
86. Binary to Decimal in C
87. Pre-increment And Post-increment
88. C/C++ Preprocessors
89. How To Install C Language In Mac
90. Evaluation of Arithmetic Expression
91. Random Number Generator in C
92. Random Access Files in C
93. Pattern Programs in C
94. Palindrome Program in C
95. Prime Number Program in C
96. Hello World Program in C
97. Simple interest program in C
98. Anagram Program in C
99. Calculator Program in C
100. C Hello World Program
101. Structure of C Program
102. Program for Linear Search in C
103. C Program for Bubble Sort
104. C Program for Factorial
105. C Program for Prime Numbers
106. Reverse a String in C
107. C Program to Reverse a Number
108. C Program for String Palindrome
109. Debugging C Program
110. How to compile a C program in Linux
111. How to Find a Leap Year Using C Programming
112. Lcm of Two Numbers in C
113. Addition of Two Numbers in C
114. Armstrong Number in C
115. Recursion in C
116. Binary Search in C
117. Matrix multiplication in C
118. Overflow And Underflow in C
119. Dynamic Memory Allocation in C
120. Pseudo-Code In C
121. Fibonacci Series Program in C Using Recursion
122. Macros in C
123. Call by Value and Call by Reference in C
124. Identifiers in C
125. Factorial of A Number in C
126. strlen() in C
127. Convert Decimal to Binary in C
128. Command Line Arguments in C/C++
129. Strcmp in C
130. Square Root in C
C Ternary Operator
In the world of programming, operators are essential tools that perform specific operations on one or more operands. Among various operators in C, the Ternary operator is an intriguing and efficient one. This article aims to provide a detailed understanding of the Ternary Operator in C, its working examples, comparison with if...else statements, and a few practice problems.
What is a Ternary Operator in C?
A Ternary Operator in C is a conditional operator that takes three arguments, hence the term 'ternary.' It provides a shorter way to code if-else statements and is extensively used to assign conditional values to variables. It is unique as it's the only operator in C that takes three operands.
The general syntax of the Ternary Operator in C is as follows:
Here, the operator first evaluates the 'condition.' If the 'condition' is true (non-zero), it returns 'value_if_true.' If the 'condition' is false (zero), it returns 'value_if_false.'
Working of Conditional/Ternary Operator in C
To understand the working of the ternary operator, let's use an example.
In this example, we use the ternary operator to find the maximum between two numbers, 'a' and 'b'. The output is "Maximum value is: 20" as 'b' is greater than 'a'.
Flow Chart for Ternary Operator in C
Here is a simple flow diagram illustrating the ternary operator's working:
This flowchart visualises the process the Ternary Operator in C undergoes while evaluating the condition and deciding the outcome based on the condition's truth value.
The Ternary Operator in C can be applied in a multitude of ways. Let's delve into some examples with various twists to highlight its versatility.
Example 1: Basic Example
This Ternary Operator in C example showcases the simplicity and straightforwardness of the ternary operator in a basic comparison operation:
In this code, 'max' will have the value of 'b' because 'a' is not greater than 'b'. Therefore, the condition is false.
Example 2: Nested Ternary Operator
The ternary operator can be nested. However, this can lead to code that is complicated to read:
This example finds the maximum of three numbers using nested ternary operators.
Example 3: Using Ternary Operator in Function Arguments
The ternary operator can be used directly in function arguments to make your code more compact:
In this example, the ternary operator is used to select the message to be printed based on the 'isEven' parameter.
Example 4: Using Ternary Operator for Variable Assignment
The ternary operator can be used for efficient variable assignment:
This code assigns a string pointer variable based on the condition. If the grade is greater than or equal to 50, it assigns "Pass"; otherwise, it assigns "Fail".
Example 5: Using Ternary Operator with Arrays
The ternary operator can also be used in conjunction with arrays:
This code uses the ternary operator to perform a safe array lookup. If 'index' is within the array bounds, it returns the value at that index; otherwise, it returns -1.
Ternary Operator Vs. if...else Statement in C
The ternary operator and if-else statements in C are used for conditional operations. They evaluate certain conditions and execute different codes based on whether they are true or false.
While both have specific use cases, clear distinctions can influence which method to use for conditional logic. Let's first discuss these differences in detail and then follow up with a comparison table for a snapshot understanding.
Ternary Operator
A ternary operator is essentially a shorthand version of an if-else statement. It provides a way to make your code more compact, condensing the if-else statement into a single line of code. This operator can be especially useful when assigning values to variables based on a condition.
Here's a sample usage of the ternary operator:
In this example, the ternary operator checks whether 'a' is greater than 'b'. If the condition is true, 'a' is assigned to 'max'. If the condition is false, 'b' is assigned to 'max'.
if...else Statement
On the other hand, if-else statements are more flexible and can handle complex logic and multiple conditions better than the ternary operator. They're easier to read, especially when the code block within the if-else construct is large.
Here's how the same problem would be solved using an if-else statement:
Comparison Table
Cons of using the ternary operator in c.
While the Ternary Operator in C provides a concise alternative to the if-else statement, overuse or improper use of this operator can lead to some issues. Here are some of the main cons of using the Ternary Operator in C:
1. Readability Issues : The primary disadvantage of the ternary operator is that it can make the code difficult to read, especially for those unfamiliar with the operator. This is especially true when it comes to complex or nested ternary expressions. Overusing ternary operators can make your code seem cluttered and cryptic, making it harder for others (and sometimes even yourself) to understand your logic.
2. Debugging Difficulties : The ternary operator's conciseness can lead to debugging challenges. Identifying and resolving errors in a line of code with a ternary operator may be more difficult compared to a more explicit if-else statement.
3. Misuse of Ternary Operators : Ternary operators are not meant to replace every if-else statement in your code. They're best suited for simple, straightforward conditional logic. Using ternary operators for complex conditions or procedures can lead to complicated, hard-to-read code.
4. Limited Use Cases : Unlike if-else statements, the ternary operator is not suitable for conditions that need to execute blocks of code or multiple statements. It's designed for simple, single-statement conditions.
5. Negatively Affects Maintainability : Code maintainability is crucial to software development. A code that is easy to read and understand can be updated and fixed more easily. Overuse of ternary operators can reduce the maintainability of your code, making it harder for you or other developers to update or expand on your code in future.
Practice Problems On Arithmetic Operator In C
Here are some of the problems that you can look at to get a better grasp of how C ternary operators work:
Problem 1 : Write a C program that uses the ternary operator to determine if a year is a leap year or not.
Problem 2 : Write a C program that uses the ternary operator to determine the quadrant in which a given coordinate point lies.
Problem 3 : Write a C program that uses the ternary operator to determine the type of a triangle given the lengths of its sides (equilateral, isosceles, or scalene).
Problem 4 : Write a C program that uses the ternary operator to check if a person is eligible to vote or not.
Problem 5 : Write a C program that uses the ternary operator to calculate the grade of a student based on their average score. The grade should be calculated as follows:
Grade A if the score is 90 or above
Grade B if the score is between 70 and 89
Grade C if the score is between 50 and 69
Grade F if the score is less than 50
The Ternary Operator in C is a powerful tool that simplifies code and improves efficiency by making it more compact. To effectively utilise the ternary operator, it is crucial to understand its syntax and working. While its conciseness can be advantageous, it's important to avoid overuse, as it can result in code that is challenging to understand and debug.
If you've found the Ternary Operator in C intriguing and wish to explore the vast world of programming further, consider upGrad's comprehensive DevOps Engineer Bootcamp to dive deep into programming, hone your skills, and emerge a coding wizard!
1. What is the ternary Operator in C symbol?
The Ternary Operator in C uses the '?' and ':' symbols. The general syntax is condition ? value_if_true : value_if_false.
2. How many ternary operator in C?
In C, there is only one ternary operator, which is the conditional operator (? :).
3. Can you provide an example of the ternary operator in C?
Yes, here is a simple example:
In this code, max will have the value of b because a is not greater than b. Therefore, the condition is false.
4. Is it good practice to always use the ternary operator in C for conditional operations?
While the ternary operator can make your code more concise, using it excessively or for complex conditions can lead to code that's hard to read and debug. For more complex conditions, traditional if-else statements may be more suitable.
5. Can the ternary operator in C be nested?
Yes, the Ternary Operator in C can be nested. However, this can make the code complicated and difficult to read, and it's generally advisable to avoid it when possible.
Leave a Reply
Your email address will not be published. Required fields are marked *
Suggested Tutorials
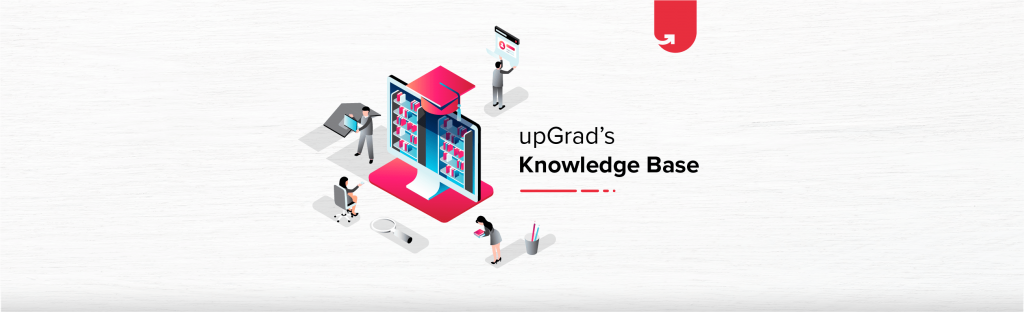
- by Mukesh kumar
- 08 Dec 2023
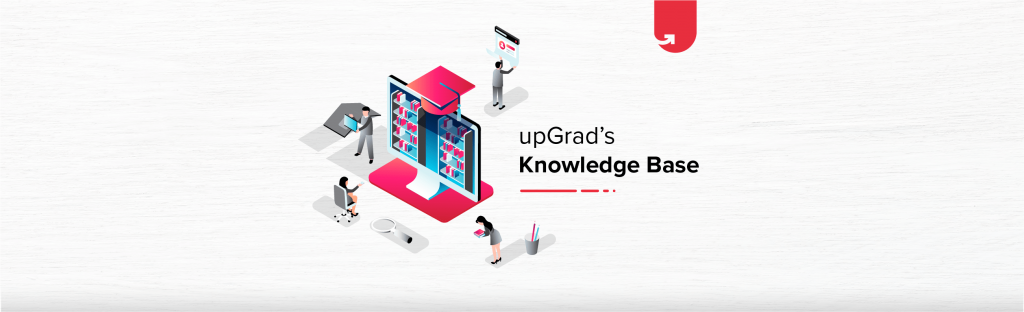
- 17 Nov 2023
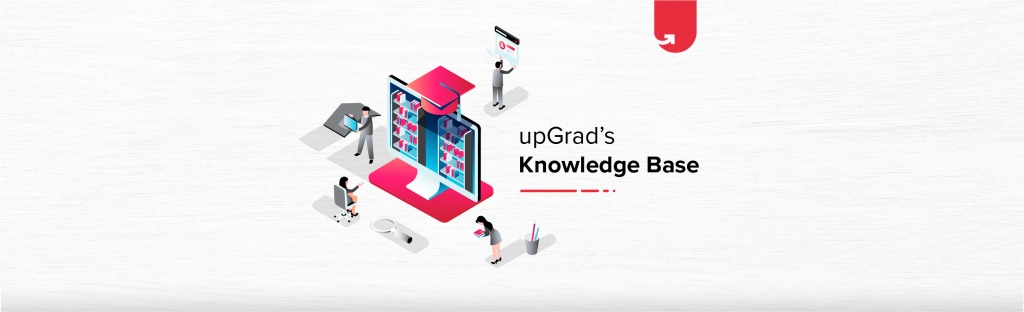
- 26 Sep 2023
Subscribe to our newsletter.
- Login Forgot Password

C Language Ternary Operator
Switch to English
Introduction
- Understanding the C Language Ternary Operator
Table of Contents
Comparing the Ternary Operator with If-Else Statement
Chaining the ternary operator, tips and common mistakes.
- Anatomy of the Ternary Operator
- Using If-Else Statement
- Using Ternary Operator
- Understand Operator Precedence
- Avoid Overcomplicating
- Remember the Return Type
Assignment and ternary(unary) operator in C
In C programming, Assignment operators are one of the most useful operators. C has different types of assignment operators between any integral or floating values.
The below table contains all assignment operators which are actively used in C programming.
Above six assignment operators has their own unique behaviours.
Assignment (equal to)(=) operator
An assignment operator is useful to assign the value of one object into another object of similar type.
Addition and assignment (plus equals to) (+=) operator
Addition and assignment operator adds the right-side value with left side value and assigns back to left value.
Subtraction and assignment (minus equals to)(-=) operator
Subtraction and assignment operator subtracts the right value with left value and assigns it back to its left values (lvalue).
Multiplication and assignment (star equals to) (*=) operator
Multiplication and assignment operator multiples the right value with its left value and assigns the multiplied value back to its left value.
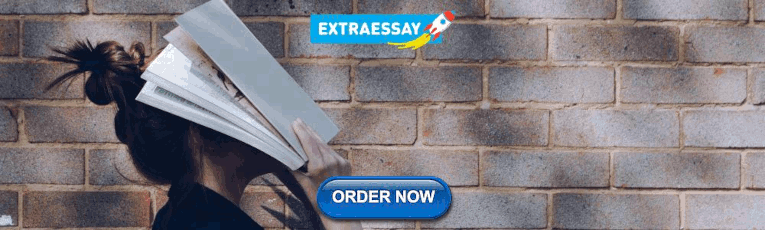
Division and assignment (divide equals to) (/=) operator
The division and assignment operator divides the left value with its right side value and assigns back to its left value (lvalue).
Modulus and assignment (mod equals to) (%=) operator
Finally, modulus and assignment operator calculates mod value between left and right values, and the remainder assigns back to its left value. This should be done between two integer values.
Above are all the assignment operators using in C programming. In addition to this there is a special type of assignment called the ternary operator.
Ternary operator (Question mark-colon) Operator
Ternary operator is a kind of assignment operator, here it assigns value based on a condition.
These are all different assignment operators and their uses in C programming. If you have any better examples please let our readers know by commenting here below.
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Telegram (Opens in new window)
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, c++ introduction.
- C++ Variables and Literals
- C++ Data Types
- C++ Basic I/O
- C++ Type Conversion
C++ Operators
- C++ Comments
C++ Flow Control
- C++ if...else
- C++ for Loop
- C++ do...while Loop
- C++ continue
- C++ switch Statement
- C++ goto Statement
- C++ Functions
- C++ Function Types
- C++ Function Overloading
- C++ Default Argument
- C++ Storage Class
- C++ Recursion
- C++ Return Reference
C++ Arrays & String
- Multidimensional Arrays
- C++ Function and Array
- C++ Structures
- Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
C++ Object & Class
- C++ Objects and Class
- C++ Constructors
- C++ Objects & Function
C++ Operator Overloading
- C++ Pointers
- C++ Pointers and Arrays
- C++ Pointers and Functions
- C++ Memory Management
- C++ Inheritance
- Inheritance Access Control
- C++ Function Overriding
- Inheritance Types
- C++ Friend Function
- C++ Virtual Function
- C++ Templates
C++ Tutorials
C++ if, if...else and Nested if...else
- Check Whether Number is Even or Odd
- Add Complex Numbers by Passing Structure to a Function
- Subtract Complex Number Using Operator Overloading
C++ Operator Precedence and Associativity
- C++ Ternary Operator
In C++, the ternary operator (also known as the conditional operator ) can be used to replace if...else in certain scenarios.
Ternary Operator in C++
A ternary operator evaluates the test condition and executes a block of code based on the result of the condition.
Its syntax is
Here, condition is evaluated and
- if condition is true , expression1 is executed.
- And, if condition is false , expression2 is executed.
The ternary operator takes 3 operands ( condition , expression1 and expression2 ). Hence, the name ternary operator .
Example : C++ Ternary Operator
Suppose the user enters 80 . Then, the condition marks >= 40 evaluates to true . Hence, the first expression "passed" is assigned to result .
Now, suppose the user enters 39.5 . Then, the condition marks >= 40 evaluates to false . Hence, the second expression "failed" is assigned to result .
- When to use a Ternary Operator?
In C++, the ternary operator can be used to replace certain types of if...else statements.
For example, we can replace this code
Here, both programs give the same output. However, the use of the ternary operator makes our code more readable and clean.
Note: We should only use the ternary operator if the resulting statement is short.
- Nested Ternary Operators
It is also possible to use one ternary operator inside another ternary operator. It is called the nested ternary operator in C++.
Here's a program to find whether a number is positive, negative, or zero using the nested ternary operator.
In the above example, notice the use of ternary operators,
- (number == 0) is the first test condition that checks if number is 0 or not. If it is, then it assigns the string value "Zero" to result .
- Else, the second test condition (number > 0) is evaluated if the first condition is false .
Note : It is not recommended to use nested ternary operators. This is because it makes our code more complex.
Table of Contents
- Example :Ternary Operator
Sorry about that.
Related Tutorials
C++ Tutorial
cppreference.com
C operator precedence.
The following table lists the precedence and associativity of C operators. Operators are listed top to bottom, in descending precedence.
- ↑ The operand of prefix ++ and -- can't be a type cast. This rule grammatically forbids some expressions that would be semantically invalid anyway. Some compilers ignore this rule and detect the invalidity semantically.
- ↑ The operand of sizeof can't be a type cast: the expression sizeof ( int ) * p is unambiguously interpreted as ( sizeof ( int ) ) * p , but not sizeof ( ( int ) * p ) .
- ↑ The expression in the middle of the conditional operator (between ? and : ) is parsed as if parenthesized: its precedence relative to ?: is ignored.
- ↑ Assignment operators' left operands must be unary (level-2 non-cast) expressions. This rule grammatically forbids some expressions that would be semantically invalid anyway. Many compilers ignore this rule and detect the invalidity semantically. For example, e = a < d ? a ++ : a = d is an expression that cannot be parsed because of this rule. However, many compilers ignore this rule and parse it as e = ( ( ( a < d ) ? ( a ++ ) : a ) = d ) , and then give an error because it is semantically invalid.
When parsing an expression, an operator which is listed on some row will be bound tighter (as if by parentheses) to its arguments than any operator that is listed on a row further below it. For example, the expression * p ++ is parsed as * ( p ++ ) , and not as ( * p ) ++ .
Operators that are in the same cell (there may be several rows of operators listed in a cell) are evaluated with the same precedence, in the given direction. For example, the expression a = b = c is parsed as a = ( b = c ) , and not as ( a = b ) = c because of right-to-left associativity.
[ edit ] Notes
Precedence and associativity are independent from order of evaluation .
The standard itself doesn't specify precedence levels. They are derived from the grammar.
In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and -- and assignment operators don't have the restrictions about their operands.
Associativity specification is redundant for unary operators and is only shown for completeness: unary prefix operators always associate right-to-left ( sizeof ++* p is sizeof ( ++ ( * p ) ) ) and unary postfix operators always associate left-to-right ( a [ 1 ] [ 2 ] ++ is ( ( a [ 1 ] ) [ 2 ] ) ++ ). Note that the associativity is meaningful for member access operators, even though they are grouped with unary postfix operators: a. b ++ is parsed ( a. b ) ++ and not a. ( b ++ ) .
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- A.2.1 Expressions
- C11 standard (ISO/IEC 9899:2011):
- C99 standard (ISO/IEC 9899:1999):
- C89/C90 standard (ISO/IEC 9899:1990):
- A.1.2.1 Expressions
[ edit ] See also
Order of evaluation of operator arguments at run time.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 31 July 2023, at 09:28.
- This page has been accessed 2,665,989 times.
- Privacy policy
- About cppreference.com
- Disclaimers

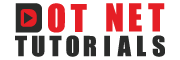
Unary vs Binary vs Ternary Operators in C
Back to: C Tutorials For Beginners and Professionals
Unary vs. Binary vs. Ternary Operators in C
In this article, I will discuss Unary vs. Binary vs. Ternary Operators in C with Examples. Please read our previous article discussing Unary Operators in C Language. In C programming, operators are symbols that tell the compiler to perform specific mathematical or logical manipulations. Operators are used in programs to manipulate data and variables. They can be categorized into unary, binary, and ternary operators based on the number of operands they take.
Unary Operators in C
Unary operators in C are operators that operate on a single operand. They are used to perform various operations, such as incrementing/decrementing a value, negating an expression, or dereferencing a pointer. Here are the most commonly used unary operators in C:
Increment (++): Increases the value of its operand by 1. It can be used in two forms:
- Prefix (++x): Increments the value of x and then returns the new value.
- Postfix (x++): Returns the value of x and then increments it.
Decrement (–): Decreases the value of its operand by 1. Similar to increment, it also has two forms:
- Prefix (–x): Decrements the value of x and then returns the new value.
- Postfix (x–): Returns the value of x and then decrements it.
Unary Minus (-): Negates the value of its operand.
Logical NOT (!): Inverts the boolean value of its operand. If the operand is non-zero, it returns 0 (false), and if the operand is zero, it returns 1 (true).
Bitwise NOT (~): Performs a bitwise negation on its operand, inverting each bit.
Address-of (&): Returns the memory address of its operand.
Dereference (*): Accesses the value at the address pointed to by its operand (the opposite of the address-of operator).
Sizeof: Returns the size of a data type or an object in bytes.
These unary operators are widely used in C programming for various purposes, including memory management, arithmetic operations, and logical operations.
Binary Operators in C
In the C programming language, binary operators are used to perform operations on two operands. Here are the most commonly used binary operators in C:
Arithmetic Operators:
- Addition (+): Adds two operands. E.g., a + b.
- Subtraction (-): Subtracts the second operand from the first. E.g., a – b.
- Multiplication (*): Multiplies two operands. E.g., a * b.
- Division (/): Divides the numerator by the denominator. E.g., a / b.
- Modulus (%): Returns the remainder of a division. E.g., a % b.
Relational Operators:
- Equal to (==): Checks if two operands are equal. Returns true if they are. E.g., a == b.
- Not equal to (!=): Checks if two operands are not equal. E.g., a != b.
- Greater than (>): Checks if the left operand is greater than the right operand. E.g., a > b.
- Less than (<): Checks if the left operand is less than the right operand. E.g., a < b.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right operand. E.g., a >= b.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right operand. E.g., a <= b.
Logical Operators:
- Logical AND (&&): Returns true if both operands are true. E.g., a && b.
- Logical OR (||): Returns true if either of the operands is true. E.g., a || b.
- Logical NOT (!): Used to reverse the logical state of its operand. E.g., !a.
Bitwise Operators:
- Bitwise AND (&): Performs a bitwise AND on two integers. E.g., a & b.
- Bitwise OR (|): Performs a bitwise OR on two integers. E.g., a | b.
- Bitwise XOR (^): Performs a bitwise exclusive OR on two integers. E.g., a ^ b.
- Bitwise left shift (<<): Shifts the bits of the first operand to the left by the number of positions specified by the second operand. E.g., a << 2.
- Bitwise right shift (>>): Shifts the bits of the first operand to the right by the number of positions specified by the second operand. E.g., a >> 2.
Assignment Operators:
- Simple assignment (=): Assigns the value of the right operand to the left operand. E.g., a = b.
- Add and assign (+=): Adds the right operand to the left operand and assigns the result to the left operand. E.g., a += b.
- Subtract and assign (-=): Subtracts the right operand from the left operand and assigns the result to the left operand. E.g., a -= b.
- Multiply and assign (*=): Multiply the right operand with the left operand and assign the result to the left operand. E.g., a *= b.
- Divide and assign (/=): Divides the left operand by the right operand and assigns the result to the left operand. E.g., a /= b.
- Modulus and assign (%=): Applies modulus operation and assigns the result to the left operand. E.g., a %= b.
These binary operators are fundamental to performing calculations, making comparisons, and manipulating data at the bit level in C programming.
Ternary Operators in C
Ternary operators in C are a shorthand way of writing conditional statements. They are a compact form of the if-else statement and are often used for simple conditional assignments. The syntax of the ternary operator in C is:
condition ? expression1 : expression2;
Here’s how it works:
- condition is an expression that evaluates to true or false.
- expression1 is the value or statement that is executed if the condition is true.
- expression2 is the value or statement that is executed if the condition is false.
For example:
In this example, the ternary operator checks if a is greater than b. If true, max is assigned the value of a; otherwise, it is assigned the value of b.
In the next article, I am going to discuss Variables in C Programming Language with Examples. Here, in this article, I explain Unary vs Binary vs Ternary Operators in C Language with examples. I hope you enjoy this Unary vs Binary vs Ternary Operators in C Language article. Please give your feedback and suggestions about this article.
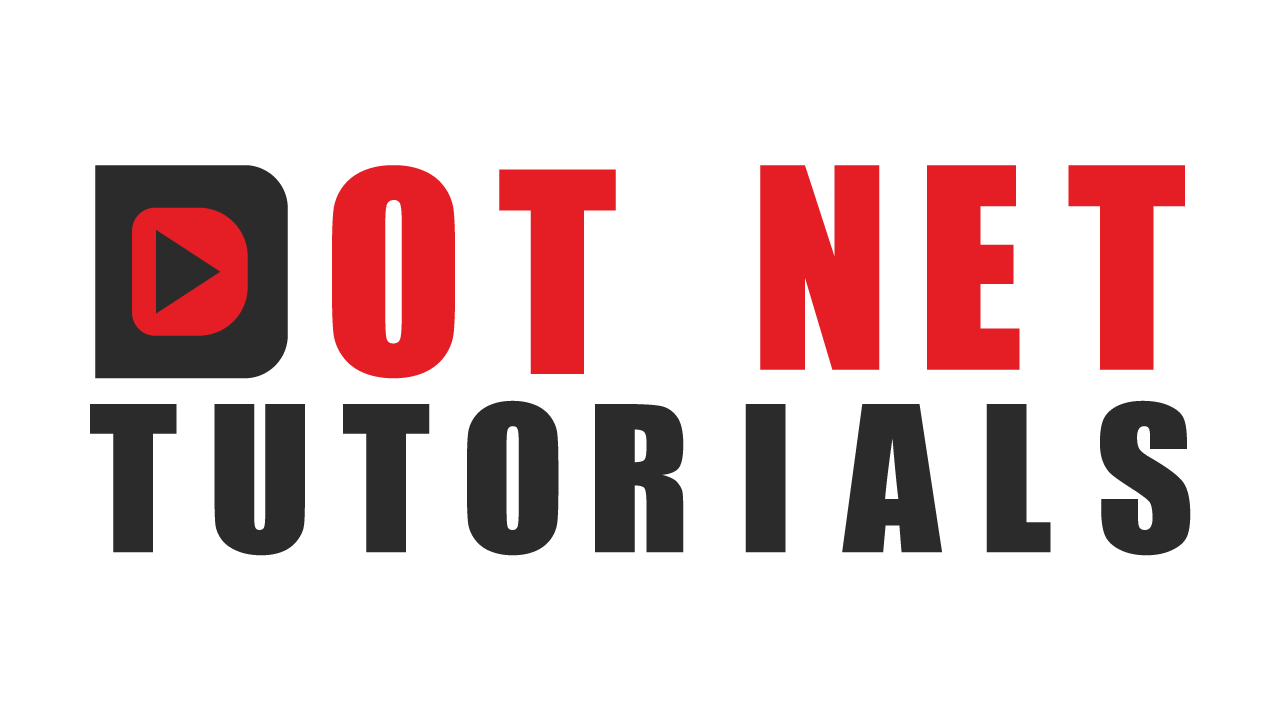
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- Solve Coding Problems
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
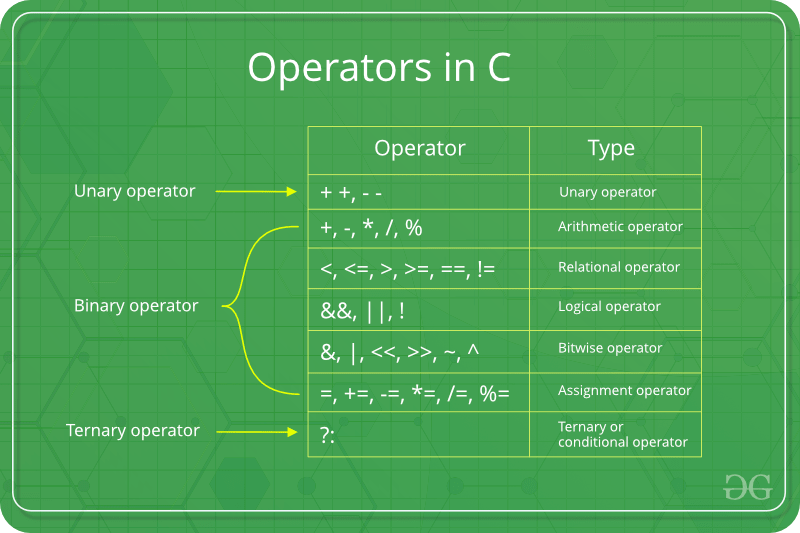
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
- C-Operators
- cpp-operator
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Online Degree Explore Bachelor’s & Master’s degrees
- MasterTrack™ Earn credit towards a Master’s degree
- University Certificates Advance your career with graduate-level learning
- Top Courses
- Join for Free
Operators: What Role Do They Play in Programming?
Learn about different types of operators and why they’re essential in computer programming, along with the different operator types in three popular languages.
![assignment in ternary operator c [Featured Image] A programmer wearing headphones is working on her laptop with types of operators.](https://d3njjcbhbojbot.cloudfront.net/api/utilities/v1/imageproxy/https://images.ctfassets.net/wp1lcwdav1p1/3Jjm45VPEENtqBBVTGNiRU/3f911f8e86c5e616cd0cada3e91b41bb/GettyImages-1032708132.jpg?w=1500&h=680&q=60&fit=fill&f=faces&fm=jpg&fl=progressive&auto=format%2Ccompress&dpr=1&w=1000)
Operators are symbols with defined functions. Programmers use these symbols to tell the interpreter or compiler in high-level computer languages, such as C++, Java, and Python, to perform a particular action. These symbols form the program's foundation, allowing you to perform various actions ranging from simple maths to complex encryption.
Operators are essential for performing calculations, assigning specific values to variables, and making condition-based decisions. You can learn more about operator types and how they work within three popular computer languages.
Types of operators
Different types perform different tasks within the programme. You can choose from three main types of operators, each with unique functions and capabilities.
Arithmetic operator
Arithmetic operators allow computers to perform mathematical calculations on specific values. For example, you might write ‘A+B = 40’ or ‘A*B = 100’. Common arithmetic operators include:
Addition: +
Subtraction: -
Multiplication: *
Division: /
Integer division: DIV
Remainder: MOD
Relational operator
Relational operators facilitate condition testing, allowing you to create variables and assign them values. For example, if A equals 45 and B equals 50, you might write A < B or A is less than B. That < symbol is a relational operator that produces true or false results. Common relational operators include:
Assignment: =
Equivalence: ==
Less than: <
Greater than: >
Less than or equal to: <=
Greater than or equal to: >=
Does not equal: !=
Logical operator
Logical operators allow programmers to gain increasingly complex computer-based decisions by combining relational operators. For example, with the logical AND operator, if A equals 10 and B equals zero, and you write ‘A AND B is false,’ the condition is true if both variables are the same. If you use the logical OR operator and just one of the variables is something other than zero, ‘A OR B is true’ would be true.
You can reverse the variable’s logical state with the local NOT operator. So, what would otherwise be true becomes false and vice versa.
Common operators include:
AND (&&)
Types of operators in C++
C++ uses more than five types of operators to carry out different functions. You may also use multiple operators in one expression. In these cases, C++ has established operator precedence to determine how things get evaluated. For example, postfix operators work left to right. So do additive, multiplicative, and shift operators. Unary, conditional, and assignment operators have precedence from right to left. Types of C++ operators include:
Assignment: These operators assign the left-hand side operand the right-hand side operand's value and are variations on = , including += (addition assignment), -= (subtraction assignment), *= (multiplication assignment), /= (division assignment), and %= (modulus assignment)
Arithmetic: These operators allow you to perform calculations between two values using symbols such as + (addition), - (subtraction), * (multiplication), / (division), % (modulus), ++ (increment), and — (decrement)
Relational: These operators perform comparisons between operands and include symbols like == equal to), > (greater than), < (less than), != (not equal to), >= (greater than or equal), and <= (less than or equal to)
Logical: These C++ operators check conditions and expressions to determine whether they are true or false to aid decision-making. They include symbols like && (logical AND operator if all operands are true), || (logical OR operator if at least one operand is true), and ! (logical NOT operator that reverses the logical state from true to false or false to true)
Bitwise: These C++ operators allow you to perform operations by treating operands like a string of bits for output in decimals. Symbols include & (logical AND operator performs AND on the bits of two operands), | (logical OR operator performs OR on the bits of two operands), ^ (bitwise XOR operator performs XOR on all bits of two numbers), ~ (bitwise one complement inverts all an operand's bits), << (binary left shift shifts the bits in one operand using the second operand as a determinant), >> (binary right shift shifts the bits in one operand using the second operand as a determinant)
Other: C++ also has other operators, including:
Size of , which determines a variable's size
Conditional , a ternary operator that evaluates test conditions and puts a code block into place based on test results
Comma, which triggers the start of an operational sequence
Types of Operators in Java
In Java, you use operators to perform local operations and mathematical tasks. You can break them into three categories based on the number of operands or the entities they operate upon and use. Unary operators work on one operand, binary operators work on two operands, and ternary operators utilise three operands to carry out the task. Java’s operators include:
Assignment: This binary operator uses the symbol = to assign the value of the operand on the right of an expression to the one on the left.
Arithmetic: These operators may be unary and binary and get placed in three categories, including primary, increment and decrement, and shorthand. Symbols include + (addition), - (subtraction), * (multiplication), / (division), % (modulus), + (unary plus to indicate a positive number), or - (unary minus to indicate a negative number)
Increment and decrement include ++ (increment) and — (decrement) to increment or decrement operands' values
Shorthand operators pair with assignment operators, making it possible to write less code by including symbols such as += (addition assignment), -= (subtraction assignment), and *= (multiplication assignment)
Relational: These binary operators work as comparison or equality operators and include symbols such as > (greater than), < (less than), >= (greater than equal to), <= (less than equal to), == (equal equal to), and != (not equal to)
Logical: Java operators in this category work on boolean operands to perform functions using symbols like & (logical AND), | (logical OR), ! (logical NOT), ^ (logical XOR), == (equal equal to), != (not equal to), && (conditional AND), and || (conditional OR)
Bitwise: To manipulate an operand's bits, you can use these operators, which include symbols like & (bitwise AND), | (bitwise OR), ~ (bitwise NOT), and ^ (bitwise XOR)
Types of Operators in Python
In Python, operators are symbols that perform specific tasks. The operator you choose for any operation changes the results, allowing you to manipulate operands' values. Python’s operators include:
Arithmetic: These operators include symbols that perform mathematical functions, including addition ( + ), subtraction ( - ), multiplication ( * ), division ( / ), and modulus ( % )
Assignment: These operators allow you to assign values and include various symbols, including = (X=2), -= (X-=2), += (X+=2), and *= (X*=2)
Comparison: When you want to compare two values, you will use symbols such as = (equal), != (not equal), > (greater than), and < (less than)
Logical: When you want to compare conditional statements, you can use operators such as x>2 and x>3 , x>2 or x>3 , or not (x>2 and x>3)
Membership: These are operators that allow you to calculate and figure out if an object contains a sequence, including in , which comes back true in the presence of the sequence, or not in , which comes back as true if the sequence doesn't exist
Bitwise: These operators compare binary values using symbols such as & (sets all bits to 1 if all bits are 1), | (sets all bits to 1 if one of the bits is 1), or ^ (sets all bits for 1 if one of the bits is 1). To invert all bits, you could use ~ (Bitwise NOT), or you can use operators to shift left ( << ) or shift right ( >> )
Identity: The two identity operators include is (comes back true if both variables are the same objects) and is not (comes back true if the variables are different objects)
What careers use operators
Operators are commonly used in computer programming, making programmers the primary career that uses them. You may use operators in other professions that require coding, including roles such as data analysts or data scientists.
Software engineers, data scientists, and data analysts are among India's most in-demand jobs [ 1 ]. Many applicants find positions in cities like Bangalore, sometimes called India’s Silicon Valley. Mumbai, Kolkata, and Delhi are common locations for these types of jobs. According to Glassdoor India, as of March 2024, the average base salaries for these careers are as follows:
Programmers: ₹4,00,000
Data scientists: ₹12,59,927
Data analysts: ₹8,47,000
Now that you’re more familiar with the types of operators and how they work in different languages, continue learning to increase your skills and achieve your goals. For example, if you’re new to programming, consider earning a Professional Certificate, such as Google IT with Python , or completing a Specialisation like Learn SQL Basics for Data Science offered by the University of California Davis on Coursera.
If you have programming experience and want to brush up on operators, complete a Guided Project like JavaScript Variables and Assignment Operators on Coursera Project Network or Looker Functions and Operators , a Project that's part of Google Cloud Training—both on Coursera.
Article sources
The Hindu. “ With a Placement Rate of 93.5% Software Engineers have Witnessed a 120% Increase in Salary in 2021-2022, https://www.thehindu.com/brandhub/with-a-placement-rate-of-935-software-engineers-have-witnessed-120-increase-in-salary-in-2021-2022/article65370576.ece.” Accessed March 22, 2024.
Keep reading
Coursera staff.
Editorial Team
Coursera’s editorial team is comprised of highly experienced professional editors, writers, and fact...
This content has been made available for informational purposes only. Learners are advised to conduct additional research to ensure that courses and other credentials pursued meet their personal, professional, and financial goals.
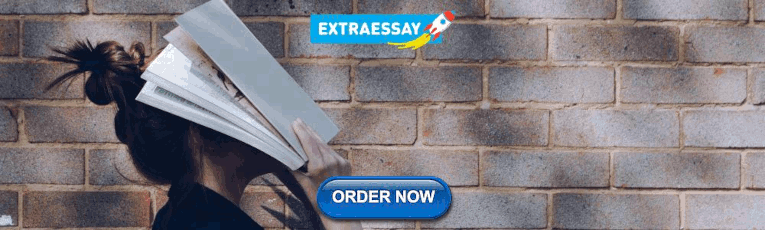
IMAGES
VIDEO
COMMENTS
8. The fact that the ternary operator is an expression, not a statement, allows it to be used in macro expansions for function-like macros that are used as part of an expression. Const may not have been part of original C, but the macro pre-processor goes way back. One place where I've seen it used is in an array package that used macros for ...
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.. Syntax of Conditional/Ternary Operator in C
Assign the ternary operator to a variable. In C programming, we can also assign the expression of the ternary operator to a variable. For example, Here, if the test condition is true, expression1 will be assigned to the variable. Otherwise, expression2 will be assigned. // create variables char operator = '+';
Ternary Operator in C Explained. Programmers use the ternary operator for decision making in place of longer if and else conditional statements. It helps to think of the ternary operator as a shorthand way or writing an if-else statement. Here's a simple decision-making example using if and else: c = a; } else {.
NOTE: Ternary operator in C, like if-else statements, can be nested. Assign the Ternary Operator to a Variable: You can assign the result of the ternary operator to a variable, as shown in the previous example with the variable max. This is a common use case for the ternary operator, where the result of the condition is stored in a variable for ...
The ternary operator in C is a powerful and concise tool that allows conditional expressions to be written in a compact form. It provides a means to streamline decision-making within code, enhancing readability and efficiency. ... Additionally, it is commonly employed in conditional assignments, where the value of a variable depends on a ...
In computer programming, the ternary conditional operator is a ternary operator that is part of the syntax for basic conditional expressions in several programming languages. ... Bear in mind also that some types allow initialization, but do not allow assignment, or even that the assignment operator and the constructor do totally different ...
In the realm of C programming, the ternary operator emerges as a powerful yet often underutilized tool. Unlike its more verbose counterparts, the ternary operator offers a succinct and elegant way to express conditional logic. This compact form of writing conditional statements not only enhances readability but also streamlines the code-writing process, making it a
The conditional operator ?:, also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false, as the following example shows: C#. string GetWeatherDisplay(double tempInCelsius) => tempInCelsius < 20.0 ?
A Ternary Operator in C is a conditional operator that takes three arguments, hence the term 'ternary.'. It provides a shorter way to code if-else statements and is extensively used to assign conditional values to variables. It is unique as it's the only operator in C that takes three operands.
It's most commonly used in assignment operations, although it has other uses as well. The ternary operator ? is a way of shortening an if-else clause, and is also called an immediate-if statement in other languages ( IIf (condition,true-clause,false-clause) in VB, for example). For example: bool Three = SOME_VALUE;
In the realm of programming, operators play a critical role in manipulating data. One such operator in the C language is the ternary operator. It's a unique operator that takes three operands, hence the name 'ternary', and it's often used as a shortcut for the if-else statement. Anatomy of the Ternary Operator
Above are all the assignment operators using in C programming. In addition to this there is a special type of assignment called the ternary operator. Ternary operator (Question mark-colon) Operator. Ternary operator is a kind of assignment operator, here it assigns value based on a condition.
The ternary operator evaluates the condition (7 % 2 == 0). Since this is false (7 is not divisible by 2 without remainder), the expression evaluates to "Odd". The output statement prints "The number is Odd." Conditional Operator in C++: The syntax for the ternary operator in C++ is the same as in C. It follows the pattern:
Ternary Operator in C++. A ternary operator evaluates the test condition and executes a block of code based on the result of the condition. Its syntax is. condition ? expression1 : expression2; Here, condition is evaluated and. if condition is true, expression1 is executed. And, if condition is false, expression2 is executed.
Note: The ternary operator have third most lowest precedence, so we need to use the expressions such that we can avoid errors due to improper operator precedence management. C++ Nested Ternary Operator. A nested ternary operator is defined as using a ternary operator inside another ternary operator. Like if-else statements, the ternary operator can also be nested inside one another.
The C++ syntax is defined as a syntactic grammar, but that grammar is designed to be consistent with a simple precedence table. You say the table is "incorrect when used on the left side of the colon", but by that reasoning the precedence is also incorrect when used on the left side of ] in an expression such as a[b+c].The claim of a precedence table is that a sub-expression seen by two ...
The following table lists the precedence and associativity of C operators. Operators are listed top to bottom, in descending precedence. Precedence Operator ... Ternary conditional: Right-to-left 14 = Simple assignment ... ↑ Assignment operators' left operands must be unary (level-2 non-cast) expressions. This rule grammatically forbids some ...
These binary operators are fundamental to performing calculations, making comparisons, and manipulating data at the bit level in C programming. Ternary Operators in C. Ternary operators in C are a shorthand way of writing conditional statements. They are a compact form of the if-else statement and are often used for simple conditional assignments.
so assignment inside ternary operator should also have been allowed but it does not work. Share. Improve this answer. Follow answered Apr 21, 2021 at 16:31. novice novice. 179 1 1 gold badge 5 5 silver badges 15 15 bronze badges. Add a comment | Your Answer
This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example: (a += b) can be written as (a = a + b) If initially value stored in a is 5. Then (a += 6) = 11. 3. "-=" This operator is combination of '-' and '=' operators.
Unary operators work on one operand, binary operators work on two operands, and ternary operators utilise three operands to carry out the task. Java's operators include: Assignment: This binary operator uses the symbol = to assign the value of the operand on the right of an expression to the one on the left.
3. Try using it like this : x = isCompleted ? intValue(if true) : intValue(if false); Since you have declared x as int, you will have to provide the values in int irrespective of the condition evaluated. Any other type value you use wont work here. Hence the false that you wrote is wrong.