
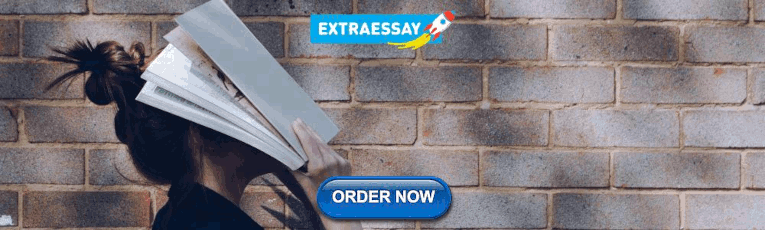
Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! 😊
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python | Decimal scaleb() method
- SymPy | Subset.ksubsets() in Python
- Python vs Ruby
- How to make Choropleth Maps with Labels using Mapbox API
- SymPy | Prufer.to_tree() in Python
- Graph Plotting in Python | Set 2
- SymPy | Subset.superset_size() in Python
- SymPy | Prufer.next() in Python
- SymPy | Prufer.edges() in Python
- SymPy | Subset.unrank_binary() in Python
- Python | sympy.combinatorics.Subset().cardinality method
- SymPy | Subset.unrank_gray() in Python
- Python | sep parameter in print()
- SymPy | Subset.subset_from_bitlist() in Python
- SymPy | Subset.subset_indices() in Python
- Working with csv files in Python
- Using Else Conditional Statement With For loop in Python
- Single Page Portfolio Using Flask
- Python | Assertion Error
Conditional Statements in Python
Understanding and mastering Python’s conditional statements is fundamental for any programmer aspiring to write efficient and robust code. In this guide, we’ll delve into the intricacies of conditional statements in Python, covering the basics, advanced techniques, and best practices.
- What are Conditional Statements?
Conditional Statements are statements in Python that provide a choice for the control flow based on a condition. It means that the control flow of the Python program will be decided based on the outcome of the condition.
Now let us see how Conditional Statements are implemented in Python.
Types of Conditional Statements in Python
Table of Content
- Types of Conditional Statement in Python
1. If Conditional Statement in Python
- 2. If else Conditional Statement in Python
- 3. Nested if..else Conditional Statement in Python
- 4. If-elif-else Conditional Statement in Python
- 5. Ternary Expression Conditional Statement in Python
Best Practices for Using Conditional Statements
If the simple code of block is to be performed if the condition holds then the if statement is used. Here the condition mentioned holds then the code of the block runs otherwise not.
Syntax of If Statement :
2. If else Conditional Statements in Python
In a conditional if Statement the additional block of code is merged as an else statement which is performed when if condition is false.
Syntax of Python If-Else :
3. Nested if..else Conditional Statements in Python
Nested if..else means an if-else statement inside another if statement. Or in simple words first, there is an outer if statement, and inside it another if – else statement is present and such type of statement is known as nested if statement. We can use one if or else if statement inside another if or else if statements.
4. If-elif-else Conditional Statements in Python
The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final “else” statement will be executed.
5. Ternary Expression Conditional Statements in Python
The Python ternary Expression determines if a condition is true or false and then returns the appropriate value in accordance with the result. The ternary Expression is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more concise — all in just one line of code.
Syntax of Ternary Expression
- Keep conditions simple and expressive for better readability.
- Avoid deeply nested conditional blocks; refactor complex logic into smaller, more manageable functions.
- Comment on complex conditions to clarify their purpose.
- Prefer the ternary operator for simple conditional assignments.
- Advanced Techniques:
- Using short-circuit evaluation for efficiency in complex conditions.
- Leveraging the any() and all() functions with conditions applied to iterables.
- Employing conditional expressions within list comprehensions and generator expressions.
Please Login to comment...
Similar reads.
- 10 Ways to Use Slack for Effective Communication
- 10 Ways to Use Google Docs for Collaborative Writing
- NEET MDS 2024 Result: Toppers List, Category-wise Cutoff, and Important Dates
- NDA Admit Card 2024 Live Updates: Download Your Hall Ticket Soon on upsc.gov.in!
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Conditional expression (ternary operator) in Python
Python has a conditional expression (sometimes called a "ternary operator"). You can write operations like if statements in one line with conditional expressions.
- 6. Expressions - Conditional expressions — Python 3.11.3 documentation
Basics of the conditional expression (ternary operator)
If ... elif ... else ... by conditional expressions, list comprehensions and conditional expressions, lambda expressions and conditional expressions.
See the following article for if statements in Python.
- Python if statements (if, elif, else)
In Python, the conditional expression is written as follows.
The condition is evaluated first. If condition is True , X is evaluated and its value is returned, and if condition is False , Y is evaluated and its value is returned.
If you want to switch the value based on a condition, simply use the desired values in the conditional expression.
If you want to switch between operations based on a condition, simply describe each corresponding expression in the conditional expression.
An expression that does not return a value (i.e., an expression that returns None ) is also acceptable in a conditional expression. Depending on the condition, either expression will be evaluated and executed.
The above example is equivalent to the following code written with an if statement.
You can also combine multiple conditions using logical operators such as and or or .
- Boolean operators in Python (and, or, not)
By combining conditional expressions, you can write an operation like if ... elif ... else ... in one line.
However, it is difficult to understand, so it may be better not to use it often.
The following two interpretations are possible, but the expression is processed as the first one.
In the sample code below, which includes three expressions, the first expression is interpreted like the second, rather than the third:
By using conditional expressions in list comprehensions, you can apply operations to the elements of the list based on the condition.
See the following article for details on list comprehensions.
- List comprehensions in Python
Conditional expressions are also useful when you want to apply an operation similar to an if statement within lambda expressions.
In the example above, the lambda expression is assigned to a variable for convenience, but this is not recommended by PEP8.
Refer to the following article for more details on lambda expressions.
- Lambda expressions in Python
Related Categories
Related articles.
- Shallow and deep copy in Python: copy(), deepcopy()
- Composite two images according to a mask image with Python, Pillow
- OpenCV, NumPy: Rotate and flip image
- pandas: Check if DataFrame/Series is empty
- Check pandas version: pd.show_versions
- Python if statement (if, elif, else)
- pandas: Find the quantile with quantile()
- Handle date and time with the datetime module in Python
- Get image size (width, height) with Python, OpenCV, Pillow (PIL)
- Convert between Unix time (Epoch time) and datetime in Python
- Convert BGR and RGB with Python, OpenCV (cvtColor)
- Matrix operations with NumPy in Python
- pandas: Replace values in DataFrame and Series with replace()
- Uppercase and lowercase strings in Python (conversion and checking)
- Calculate mean, median, mode, variance, standard deviation in Python
How to Use Conditional Statements in Python – Examples of if, else, and elif

Conditional statements are an essential part of programming in Python. They allow you to make decisions based on the values of variables or the result of comparisons.
In this article, we'll explore how to use if, else, and elif statements in Python, along with some examples of how to use them in practice.
How to Use the if Statement in Python
The if statement allows you to execute a block of code if a certain condition is true. Here's the basic syntax:
The condition can be any expression that evaluates to a Boolean value (True or False). If the condition is True, the code block indented below the if statement will be executed. If the condition is False, the code block will be skipped.
Here's an example of how to use an if statement to check if a number is positive:
In this example, we use the > operator to compare the value of num to 0. If num is greater than 0, the code block indented below the if statement will be executed, and the message "The number is positive." will be printed.
How to Use the else Statement in Python
The else statement allows you to execute a different block of code if the if condition is False. Here's the basic syntax:
If the condition is True, the code block indented below the if statement will be executed, and the code block indented below the else statement will be skipped.
If the condition is False, the code block indented below the else statement will be executed, and the code block indented below the if statement will be skipped.
Here's an example of how to use an if-else statement to check if a number is positive or negative:
In this example, we use an if-else statement to check if num is greater than 0. If it is, the message "The number is positive." is printed. If it is not (that is, num is negative or zero), the message "The number is negative." is printed.
How to Use the elif Statement in Python
The elif statement allows you to check multiple conditions in sequence, and execute different code blocks depending on which condition is true. Here's the basic syntax:
The elif statement is short for "else if", and can be used multiple times to check additional conditions.
Here's an example of how to use an if-elif-else statement to check if a number is positive, negative, or zero:
Use Cases For Conditional Statements
Example 1: checking if a number is even or odd..
In this example, we use the modulus operator (%) to check if num is evenly divisible by 2.
If the remainder of num divided by 2 is 0, the condition num % 2 == 0 is True, and the code block indented below the if statement will be executed. It will print the message "The number is even."
If the remainder is not 0, the condition is False, and the code block indented below the else statement will be executed, printing the message "The number is odd."
Example 2: Assigning a letter grade based on a numerical score
In this example, we use an if-elif-else statement to assign a letter grade based on a numerical score.
The if statement checks if the score is greater than or equal to 90. If it is, the grade is set to "A". If not, the first elif statement checks if the score is greater than or equal to 80. If it is, the grade is set to "B". If not, the second elif statement checks if the score is greater than or equal to 70, and so on. If none of the conditions are met, the else statement assigns the grade "F".
Example 3: Checking if a year is a leap year
In this example, we use nested if statements to check if a year is a leap year. A year is a leap year if it is divisible by 4, except for years that are divisible by 100 but not divisible by 400.
The outer if statement checks if year is divisible by 4. If it is, the inner if statement checks if it is also divisible by 100. If it is, the innermost if statement checks if it is divisible by 400. If it is, the code block indented below that statement will be executed, printing the message "is a leap year."
If it is not, the code block indented below the else statement inside the inner if statement will be executed, printing the message "is not a leap year.".
If the year is not divisible by 4, the code block indented below the else statement of the outer if statement will be executed, printing the message "is not a leap year."
Example 4: Checking if a string contains a certain character
In this example, we use the in operator to check if the character char is present in the string string. If it is, the condition char in string is True, and the code block indented below the if statement will be executed, printing the message "The string contains the character" followed by the character itself.
If char is not present in string, the condition is False, and the code block indented below the else statement will be executed, printing the message "The string does not contain the character" followed by the character itself.
Conditional statements (if, else, and elif) are fundamental programming constructs that allow you to control the flow of your program based on conditions that you specify. They provide a way to make decisions in your program and execute different code based on those decisions.
In this article, we have seen several examples of how to use these statements in Python, including checking if a number is even or odd, assigning a letter grade based on a numerical score, checking if a year is a leap year, and checking if a string contains a certain character.
By mastering these statements, you can create more powerful and versatile programs that can handle a wider range of tasks and scenarios.
It is important to keep in mind that proper indentation is crucial when using conditional statements in Python, as it determines which code block is executed based on the condition.
With practice, you will become proficient in using these statements to create more complex and effective Python programs.
Let’s connect on Twitter and Linkedin .
Data Scientist||Machine Learning Engineer|| Data Analyst|| Microsoft Student Learn Ambassador
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
A Comprehensive Guide to Using Conditionals in Python with Real-World Examples
Conditionals are a fundamental concept in programming that allow code to execute differently based on certain conditions. In Python, conditionals take the form of if , elif , and else statements. Mastering conditionals is key to writing dynamic, flexible programs that can handle different scenarios and make decisions.
This comprehensive guide will provide a deep dive into using conditionals in Python for real-world applications. We will cover the following topics:
Table of Contents
Basic syntax and structure of conditionals, comparison operators, logic operators, if statements, if-else statements, if-elif-else statements, nested conditionals, ternary operator, common errors and mistakes, user input validation, handling different user types, recommendation systems, data analysis and visualization, game design and gameplay logic.
The basic syntax for an if statement in Python is:
The condition can be any expression that evaluates to True or False. The code block indented under the if statement runs only when the condition is True.
Some key points:
- The condition follows the if keyword and ends with a colon (:)
- The code block after the condition is indented (usually 4 spaces)
- if , elif , and else are lowercase
- Code blocks end when the indentation returns to the left margin
Let’s look at a simple example:
Here we check if the value of age is greater than or equal to 18. If so, we print a message saying the person can vote. The print statement is indented under the if to indicate it runs conditionally.
Comparison operators allow us to compare two values and evaluate to True or False. They are essential for writing conditional expressions.
Here are some examples of using comparison operators in conditional statements:
We can also chain multiple comparisons using logic operators like and and or .
Logic operators allow us to combine multiple conditional expressions and evaluate the overall logic.
The two main logic operators are:
- and - Both conditions must be True for overall expression to be True
- or - Either one condition must be True for overall expression to be True
Both age >= 18 and citizen must be True for the print statement to execute.
Other logical operators include:
- not - Negates or flips the Boolean value
- in - Checks if a value is present in a sequence
- not in - Checks if a value is not present
Logic operators allow us to handle complex conditional logic in a concise way.
The if statement is used when we want to execute code only when some condition is fulfilled. For example:
Here we only want to print “Great job!” when the score is 80 or higher. The if statement allows us to specify this condition.
Some things to note about if statements:
- They execute the code block only when condition evaluates to True
- The condition can use any comparison or logical operators
- We can use complex logic by chaining multiple conditions with and , or , not
- The code block must be indented under the if statement
Let’s look at some more examples:
The if statement allows us to execute code conditioned on any criteria we specify in the conditional expression.
The if-else statement extends the simple if by allowing us to specify code that executes when the condition evaluates to False.
The syntax is:
Let’s look at an example:
Here if age is less than 18, we print a different message using the else block.
Key points on if-else :
- The else can only be used after an if statement
- The else block runs when the if condition is False
- We can chain multiple elif blocks for more conditions (see next section)
- Only one code block will execute - either if or else
More examples:
The if-else statement allows us to conditionally run different code blocks based on the evaluation of the condition expression.
The elif statement is used to chain multiple conditional checks. Using elif we can have multiple conditions evaluated in order.
This allows us to check many conditions and selectively run code for each case. For example:
Here we check the score against multiple grade thresholds. First if to check for A, then elif to check for B, etc. The final else acts as a default case if none match.
Some key points on if-elif-else :
- Only one block will execute
- Each condition is checked in order
- elif lets us chain multiple conditions
- The else block is optional
The elif conditionals allow us to concisely handle multiple scenarios without writing nested if statements.
Nested conditionals refer to if statements within if statements. We can nest conditionals indefinitely to handle complex logic.
For example:
The outer if checks age, and the inner if-else selectively prints messages for students vs non-students.
Nested conditionals are useful when:
- We want to check secondary conditions after initial condition passes
- Breaking down complex conditional logic into simple steps
- Handling specific cases before handling general cases
However, deeply nested conditionals can make code hard to read. In those cases, functions may be better for readability.
The ternary operator provides a compact syntax for basic conditional logic:
This condenses a basic if-else check into one line.
Some points on ternary operator usage:
- Best for simple one line conditionals
- Hard to read for complex logic
- Can be nested but not recommended
- Has form value_if_true if condition else value_if_false
The ternary operator is ideal for quick conditional assignments or returning values conditionally from functions.
Some common errors when using conditionals include:
- Forgetting colons : after conditionals
- Indentation errors with code blocks
- Using assignment = instead of comparisons ==
- Misspellings in conditionals like adn , ro , etc.
- Missing parentheses around conditions
- Checking equality on two different types
These often cause syntax errors or unexpected logic errors. Always double check the condition expressions and indentations when debugging conditional issues.
Proper code commenting and leaving notes during coding can help identify issues with complex conditional statements. Start small and test conditionals thoroughly when chaining many elif clauses.
Real-World Examples and Exercises
Next we’ll explore some real-world examples to illustrate how conditionals are used in Python programming for tasks like user input validation, handling different user types, recommendation engines, data analysis, game design, and more.
Validating user input is crucial for many programs. For example:
We first check if the input is a digit, then convert to an integer. Next we check if age meets the 18+ requirement for access. The else handles any non-digit input.
Here are some other user input validation examples:
Careful input validation prevents bugs and errors down the line.
We can use conditionals to handle different features or pricing for various user types:
Different access levels can also be handled:
Conditionals allow flexible user handling in large applications.
Many recommendation systems use conditional logic to provide personalized suggestions based on certain factors. For example:
Products can be intelligently recommended using if-elif conditional chains.
When analyzing and visualizing data in Python, we can use conditionals to handle missing data or special cases:
Conditionals help account for incomplete data and customize data visualization.
Games make heavy use of conditionals to implement gameplay mechanics, physics, ballistics, animations, etc.
This implements a basic combat loop with damage dealt conditionally based on hit chance rolls. The end condition checks remaining health to determine winner.
Many other gameplay elements can be implemented using conditionals - physics, animations, resource management, abilities, etc.
Conditionals allow us to execute code selectively based on Boolean logic and are a core programming concept in any language. Python provides an intuitive syntax using if , else , elif for implementing conditional code execution.
In this guide, we covered the basics of conditionals in Python including operators, complex conditional chains, nesting conditionals, ternary expressions, and common errors. We examined real-world examples of using conditional logic for input validation, handling user types, recommendation systems, data analysis, and game mechanics.
Conditionals enable you to write dynamic, flexible programs that can make intelligent decisions and handle varying scenarios. Mastering their usage takes practice, but being comfortable with conditional logic will enable you to take on more advanced programming tasks.
- python
- control-flow
- Module 2: The Essentials of Python »
- Conditional Statements
- View page source
Conditional Statements
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
In this section, we will be introduced to the if , else , and elif statements. These allow you to specify that blocks of code are to be executed only if specified conditions are found to be true, or perhaps alternative code if the condition is found to be false. For example, the following code will square x if it is a negative number, and will cube x if it is a positive number:
Please refer to the “Basic Python Object Types” subsection to recall the basics of the “boolean” type, which represents True and False values. We will extend that discussion by introducing comparison operations and membership-checking, and then expanding on the utility of the built-in bool type.
Comparison Operations
Comparison statements will evaluate explicitly to either of the boolean-objects: True or False . There are eight comparison operations in Python:
The first six of these operators are familiar from mathematics:
Note that = and == have very different meanings. The former is the assignment operator, and the latter is the equality operator:
Python allows you to chain comparison operators to create “compound” comparisons:
Whereas == checks to see if two objects have the same value, the is operator checks to see if two objects are actually the same object. For example, creating two lists with the same contents produces two distinct lists, that have the same “value”:
Thus the is operator is most commonly used to check if a variable references the None object, or either of the boolean objects:
Use is not to check if two objects are distinct:
bool and Truth Values of Non-Boolean Objects
Recall that the two boolean objects True and False formally belong to the int type in addition to bool , and are associated with the values 1 and 0 , respectively:
Likewise Python ascribes boolean values to non-boolean objects. For example,the number 0 is associated with False and non-zero numbers are associated with True . The boolean values of built-in objects can be evaluated with the built-in Python command bool :
and non-zero Python integers are associated with True :
The following built-in Python objects evaluate to False via bool :
Zero of any numeric type: 0 , 0.0 , 0j
Any empty sequence, such as an empty string or list: '' , tuple() , [] , numpy.array([])
Empty dictionaries and sets
Thus non-zero numbers and non-empty sequences/collections evaluate to True via bool .
The bool function allows you to evaluate the boolean values ascribed to various non-boolean objects. For instance, bool([]) returns False wherease bool([1, 2]) returns True .
if , else , and elif
We now introduce the simple, but powerful if , else , and elif conditional statements. This will allow us to create simple branches in our code. For instance, suppose you are writing code for a video game, and you want to update a character’s status based on her/his number of health-points (an integer). The following code is representative of this:
Each if , elif , and else statement must end in a colon character, and the body of each of these statements is delimited by whitespace .
The following pseudo-code demonstrates the general template for conditional statements:
In practice this can look like:
In its simplest form, a conditional statement requires only an if clause. else and elif clauses can only follow an if clause.
Similarly, conditional statements can have an if and an else without an elif :
Conditional statements can also have an if and an elif without an else :
Note that only one code block within a single if-elif-else statement can be executed: either the “if-block” is executed, or an “elif-block” is executed, or the “else-block” is executed. Consecutive if-statements, however, are completely independent of one another, and thus their code blocks can be executed in sequence, if their respective conditional statements resolve to True .
Reading Comprehension: Conditional statements
Assume my_list is a list. Given the following code:
What will happen if my_list is [] ? Will IndexError be raised? What will first_item be?
Assume variable my_file is a string storing a filename, where a period denotes the end of the filename and the beginning of the file-type. Write code that extracts only the filename.
my_file will have at most one period in it. Accommodate cases where my_file does not include a file-type.
"code.py" \(\rightarrow\) "code"
"doc2.pdf" \(\rightarrow\) "doc2"
"hello_world" \(\rightarrow\) "hello_world"
Inline if-else statements
Python supports a syntax for writing a restricted version of if-else statements in a single line. The following code:
can be written in a single line as:
This is suggestive of the general underlying syntax for inline if-else statements:
The inline if-else statement :
The expression A if <condition> else B returns A if bool(<condition>) evaluates to True , otherwise this expression will return B .
This syntax is highly restricted compared to the full “if-elif-else” expressions - no “elif” statement is permitted by this inline syntax, nor are multi-line code blocks within the if/else clauses.
Inline if-else statements can be used anywhere, not just on the right side of an assignment statement, and can be quite convenient:
We will see this syntax shine when we learn about comprehension statements. That being said, this syntax should be used judiciously. For example, inline if-else statements ought not be used in arithmetic expressions, for therein lies madness:
Short-Circuiting Logical Expressions
Armed with our newfound understanding of conditional statements, we briefly return to our discussion of Python’s logic expressions to discuss “short-circuiting”. In Python, a logical expression is evaluated from left to right and will return its boolean value as soon as it is unambiguously determined, leaving any remaining portions of the expression unevaluated . That is, the expression may be short-circuited .
For example, consider the fact that an and operation will only return True if both of its arguments evaluate to True . Thus the expression False and <anything> is guaranteed to return False ; furthermore, when executed, this expression will return False without having evaluated bool(<anything>) .
To demonstrate this behavior, consider the following example:
According to our discussion, the pattern False and short-circuits this expression without it ever evaluating bool(1/0) . Reversing the ordering of the arguments makes this clear.
In practice, short-circuiting can be leveraged in order to condense one’s code. Suppose a section of our code is processing a variable x , which may be either a number or a string . Suppose further that we want to process x in a special way if it is an all-uppercased string. The code
is problematic because isupper can only be called once we are sure that x is a string; this code will raise an error if x is a number. We could instead write
but the more elegant and concise way of handling the nestled checking is to leverage our ability to short-circuit logic expressions.
See, that if x is not a string, that isinstance(x, str) will return False ; thus isinstance(x, str) and x.isupper() will short-circuit and return False without ever evaluating bool(x.isupper()) . This is the preferable way to handle this sort of checking. This code is more concise and readable than the equivalent nested if-statements.
Reading Comprehension: short-circuited expressions
Consider the preceding example of short-circuiting, where we want to catch the case where x is an uppercased string. What is the “bug” in the following code? Why does this fail to utilize short-circuiting correctly?
Links to Official Documentation
Truth testing
Boolean operations
Comparisons
‘if’ statements
Reading Comprehension Exercise Solutions:
Conditional statements
If my_list is [] , then bool(my_list) will return False , and the code block will be skipped. Thus first_item will be None .
First, check to see if . is even contained in my_file . If it is, find its index-position, and slice the string up to that index. Otherwise, my_file is already the file name.
Short-circuited expressions
fails to account for the fact that expressions are always evaluated from left to right. That is, bool(x.isupper()) will always be evaluated first in this instance and will raise an error if x is not a string. Thus the following isinstance(x, str) statement is useless.
- Python »
- 3.12.3 Documentation »
- The Python Language Reference »
- 6. Expressions
- Theme Auto Light Dark |
6. Expressions ¶
This chapter explains the meaning of the elements of expressions in Python.
Syntax Notes: In this and the following chapters, extended BNF notation will be used to describe syntax, not lexical analysis. When (one alternative of) a syntax rule has the form
and no semantics are given, the semantics of this form of name are the same as for othername .
6.1. Arithmetic conversions ¶
When a description of an arithmetic operator below uses the phrase “the numeric arguments are converted to a common type”, this means that the operator implementation for built-in types works as follows:
If either argument is a complex number, the other is converted to complex;
otherwise, if either argument is a floating point number, the other is converted to floating point;
otherwise, both must be integers and no conversion is necessary.
Some additional rules apply for certain operators (e.g., a string as a left argument to the ‘%’ operator). Extensions must define their own conversion behavior.
6.2. Atoms ¶
Atoms are the most basic elements of expressions. The simplest atoms are identifiers or literals. Forms enclosed in parentheses, brackets or braces are also categorized syntactically as atoms. The syntax for atoms is:
6.2.1. Identifiers (Names) ¶
An identifier occurring as an atom is a name. See section Identifiers and keywords for lexical definition and section Naming and binding for documentation of naming and binding.
When the name is bound to an object, evaluation of the atom yields that object. When a name is not bound, an attempt to evaluate it raises a NameError exception.
Private name mangling: When an identifier that textually occurs in a class definition begins with two or more underscore characters and does not end in two or more underscores, it is considered a private name of that class. Private names are transformed to a longer form before code is generated for them. The transformation inserts the class name, with leading underscores removed and a single underscore inserted, in front of the name. For example, the identifier __spam occurring in a class named Ham will be transformed to _Ham__spam . This transformation is independent of the syntactical context in which the identifier is used. If the transformed name is extremely long (longer than 255 characters), implementation defined truncation may happen. If the class name consists only of underscores, no transformation is done.
6.2.2. Literals ¶
Python supports string and bytes literals and various numeric literals:
Evaluation of a literal yields an object of the given type (string, bytes, integer, floating point number, complex number) with the given value. The value may be approximated in the case of floating point and imaginary (complex) literals. See section Literals for details.
All literals correspond to immutable data types, and hence the object’s identity is less important than its value. Multiple evaluations of literals with the same value (either the same occurrence in the program text or a different occurrence) may obtain the same object or a different object with the same value.
6.2.3. Parenthesized forms ¶
A parenthesized form is an optional expression list enclosed in parentheses:
A parenthesized expression list yields whatever that expression list yields: if the list contains at least one comma, it yields a tuple; otherwise, it yields the single expression that makes up the expression list.
An empty pair of parentheses yields an empty tuple object. Since tuples are immutable, the same rules as for literals apply (i.e., two occurrences of the empty tuple may or may not yield the same object).
Note that tuples are not formed by the parentheses, but rather by use of the comma. The exception is the empty tuple, for which parentheses are required — allowing unparenthesized “nothing” in expressions would cause ambiguities and allow common typos to pass uncaught.
6.2.4. Displays for lists, sets and dictionaries ¶
For constructing a list, a set or a dictionary Python provides special syntax called “displays”, each of them in two flavors:
either the container contents are listed explicitly, or
they are computed via a set of looping and filtering instructions, called a comprehension .
Common syntax elements for comprehensions are:
The comprehension consists of a single expression followed by at least one for clause and zero or more for or if clauses. In this case, the elements of the new container are those that would be produced by considering each of the for or if clauses a block, nesting from left to right, and evaluating the expression to produce an element each time the innermost block is reached.
However, aside from the iterable expression in the leftmost for clause, the comprehension is executed in a separate implicitly nested scope. This ensures that names assigned to in the target list don’t “leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated directly in the enclosing scope and then passed as an argument to the implicitly nested scope. Subsequent for clauses and any filter condition in the leftmost for clause cannot be evaluated in the enclosing scope as they may depend on the values obtained from the leftmost iterable. For example: [x*y for x in range(10) for y in range(x, x+10)] .
To ensure the comprehension always results in a container of the appropriate type, yield and yield from expressions are prohibited in the implicitly nested scope.
Since Python 3.6, in an async def function, an async for clause may be used to iterate over a asynchronous iterator . A comprehension in an async def function may consist of either a for or async for clause following the leading expression, may contain additional for or async for clauses, and may also use await expressions. If a comprehension contains either async for clauses or await expressions or other asynchronous comprehensions it is called an asynchronous comprehension . An asynchronous comprehension may suspend the execution of the coroutine function in which it appears. See also PEP 530 .
New in version 3.6: Asynchronous comprehensions were introduced.
Changed in version 3.8: yield and yield from prohibited in the implicitly nested scope.
Changed in version 3.11: Asynchronous comprehensions are now allowed inside comprehensions in asynchronous functions. Outer comprehensions implicitly become asynchronous.
6.2.5. List displays ¶
A list display is a possibly empty series of expressions enclosed in square brackets:
A list display yields a new list object, the contents being specified by either a list of expressions or a comprehension. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and placed into the list object in that order. When a comprehension is supplied, the list is constructed from the elements resulting from the comprehension.
6.2.6. Set displays ¶
A set display is denoted by curly braces and distinguishable from dictionary displays by the lack of colons separating keys and values:
A set display yields a new mutable set object, the contents being specified by either a sequence of expressions or a comprehension. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and added to the set object. When a comprehension is supplied, the set is constructed from the elements resulting from the comprehension.
An empty set cannot be constructed with {} ; this literal constructs an empty dictionary.
6.2.7. Dictionary displays ¶
A dictionary display is a possibly empty series of dict items (key/value pairs) enclosed in curly braces:
A dictionary display yields a new dictionary object.
If a comma-separated sequence of dict items is given, they are evaluated from left to right to define the entries of the dictionary: each key object is used as a key into the dictionary to store the corresponding value. This means that you can specify the same key multiple times in the dict item list, and the final dictionary’s value for that key will be the last one given.
A double asterisk ** denotes dictionary unpacking . Its operand must be a mapping . Each mapping item is added to the new dictionary. Later values replace values already set by earlier dict items and earlier dictionary unpackings.
New in version 3.5: Unpacking into dictionary displays, originally proposed by PEP 448 .
A dict comprehension, in contrast to list and set comprehensions, needs two expressions separated with a colon followed by the usual “for” and “if” clauses. When the comprehension is run, the resulting key and value elements are inserted in the new dictionary in the order they are produced.
Restrictions on the types of the key values are listed earlier in section The standard type hierarchy . (To summarize, the key type should be hashable , which excludes all mutable objects.) Clashes between duplicate keys are not detected; the last value (textually rightmost in the display) stored for a given key value prevails.
Changed in version 3.8: Prior to Python 3.8, in dict comprehensions, the evaluation order of key and value was not well-defined. In CPython, the value was evaluated before the key. Starting with 3.8, the key is evaluated before the value, as proposed by PEP 572 .
6.2.8. Generator expressions ¶
A generator expression is a compact generator notation in parentheses:
A generator expression yields a new generator object. Its syntax is the same as for comprehensions, except that it is enclosed in parentheses instead of brackets or curly braces.
Variables used in the generator expression are evaluated lazily when the __next__() method is called for the generator object (in the same fashion as normal generators). However, the iterable expression in the leftmost for clause is immediately evaluated, so that an error produced by it will be emitted at the point where the generator expression is defined, rather than at the point where the first value is retrieved. Subsequent for clauses and any filter condition in the leftmost for clause cannot be evaluated in the enclosing scope as they may depend on the values obtained from the leftmost iterable. For example: (x*y for x in range(10) for y in range(x, x+10)) .
The parentheses can be omitted on calls with only one argument. See section Calls for details.
To avoid interfering with the expected operation of the generator expression itself, yield and yield from expressions are prohibited in the implicitly defined generator.
If a generator expression contains either async for clauses or await expressions it is called an asynchronous generator expression . An asynchronous generator expression returns a new asynchronous generator object, which is an asynchronous iterator (see Asynchronous Iterators ).
New in version 3.6: Asynchronous generator expressions were introduced.
Changed in version 3.7: Prior to Python 3.7, asynchronous generator expressions could only appear in async def coroutines. Starting with 3.7, any function can use asynchronous generator expressions.
6.2.9. Yield expressions ¶
The yield expression is used when defining a generator function or an asynchronous generator function and thus can only be used in the body of a function definition. Using a yield expression in a function’s body causes that function to be a generator function, and using it in an async def function’s body causes that coroutine function to be an asynchronous generator function. For example:
Due to their side effects on the containing scope, yield expressions are not permitted as part of the implicitly defined scopes used to implement comprehensions and generator expressions.
Changed in version 3.8: Yield expressions prohibited in the implicitly nested scopes used to implement comprehensions and generator expressions.
Generator functions are described below, while asynchronous generator functions are described separately in section Asynchronous generator functions .
When a generator function is called, it returns an iterator known as a generator. That generator then controls the execution of the generator function. The execution starts when one of the generator’s methods is called. At that time, the execution proceeds to the first yield expression, where it is suspended again, returning the value of expression_list to the generator’s caller, or None if expression_list is omitted. By suspended, we mean that all local state is retained, including the current bindings of local variables, the instruction pointer, the internal evaluation stack, and the state of any exception handling. When the execution is resumed by calling one of the generator’s methods, the function can proceed exactly as if the yield expression were just another external call. The value of the yield expression after resuming depends on the method which resumed the execution. If __next__() is used (typically via either a for or the next() builtin) then the result is None . Otherwise, if send() is used, then the result will be the value passed in to that method.
All of this makes generator functions quite similar to coroutines; they yield multiple times, they have more than one entry point and their execution can be suspended. The only difference is that a generator function cannot control where the execution should continue after it yields; the control is always transferred to the generator’s caller.
Yield expressions are allowed anywhere in a try construct. If the generator is not resumed before it is finalized (by reaching a zero reference count or by being garbage collected), the generator-iterator’s close() method will be called, allowing any pending finally clauses to execute.
When yield from <expr> is used, the supplied expression must be an iterable. The values produced by iterating that iterable are passed directly to the caller of the current generator’s methods. Any values passed in with send() and any exceptions passed in with throw() are passed to the underlying iterator if it has the appropriate methods. If this is not the case, then send() will raise AttributeError or TypeError , while throw() will just raise the passed in exception immediately.
When the underlying iterator is complete, the value attribute of the raised StopIteration instance becomes the value of the yield expression. It can be either set explicitly when raising StopIteration , or automatically when the subiterator is a generator (by returning a value from the subgenerator).
Changed in version 3.3: Added yield from <expr> to delegate control flow to a subiterator.
The parentheses may be omitted when the yield expression is the sole expression on the right hand side of an assignment statement.
The proposal for adding generators and the yield statement to Python.
The proposal to enhance the API and syntax of generators, making them usable as simple coroutines.
The proposal to introduce the yield_from syntax, making delegation to subgenerators easy.
The proposal that expanded on PEP 492 by adding generator capabilities to coroutine functions.
6.2.9.1. Generator-iterator methods ¶
This subsection describes the methods of a generator iterator. They can be used to control the execution of a generator function.
Note that calling any of the generator methods below when the generator is already executing raises a ValueError exception.
Starts the execution of a generator function or resumes it at the last executed yield expression. When a generator function is resumed with a __next__() method, the current yield expression always evaluates to None . The execution then continues to the next yield expression, where the generator is suspended again, and the value of the expression_list is returned to __next__() ’s caller. If the generator exits without yielding another value, a StopIteration exception is raised.
This method is normally called implicitly, e.g. by a for loop, or by the built-in next() function.
Resumes the execution and “sends” a value into the generator function. The value argument becomes the result of the current yield expression. The send() method returns the next value yielded by the generator, or raises StopIteration if the generator exits without yielding another value. When send() is called to start the generator, it must be called with None as the argument, because there is no yield expression that could receive the value.
Raises an exception at the point where the generator was paused, and returns the next value yielded by the generator function. If the generator exits without yielding another value, a StopIteration exception is raised. If the generator function does not catch the passed-in exception, or raises a different exception, then that exception propagates to the caller.
In typical use, this is called with a single exception instance similar to the way the raise keyword is used.
For backwards compatibility, however, the second signature is supported, following a convention from older versions of Python. The type argument should be an exception class, and value should be an exception instance. If the value is not provided, the type constructor is called to get an instance. If traceback is provided, it is set on the exception, otherwise any existing __traceback__ attribute stored in value may be cleared.
Changed in version 3.12: The second signature (type[, value[, traceback]]) is deprecated and may be removed in a future version of Python.
Raises a GeneratorExit at the point where the generator function was paused. If the generator function then exits gracefully, is already closed, or raises GeneratorExit (by not catching the exception), close returns to its caller. If the generator yields a value, a RuntimeError is raised. If the generator raises any other exception, it is propagated to the caller. close() does nothing if the generator has already exited due to an exception or normal exit.
6.2.9.2. Examples ¶
Here is a simple example that demonstrates the behavior of generators and generator functions:
For examples using yield from , see PEP 380: Syntax for Delegating to a Subgenerator in “What’s New in Python.”
6.2.9.3. Asynchronous generator functions ¶
The presence of a yield expression in a function or method defined using async def further defines the function as an asynchronous generator function.
When an asynchronous generator function is called, it returns an asynchronous iterator known as an asynchronous generator object. That object then controls the execution of the generator function. An asynchronous generator object is typically used in an async for statement in a coroutine function analogously to how a generator object would be used in a for statement.
Calling one of the asynchronous generator’s methods returns an awaitable object, and the execution starts when this object is awaited on. At that time, the execution proceeds to the first yield expression, where it is suspended again, returning the value of expression_list to the awaiting coroutine. As with a generator, suspension means that all local state is retained, including the current bindings of local variables, the instruction pointer, the internal evaluation stack, and the state of any exception handling. When the execution is resumed by awaiting on the next object returned by the asynchronous generator’s methods, the function can proceed exactly as if the yield expression were just another external call. The value of the yield expression after resuming depends on the method which resumed the execution. If __anext__() is used then the result is None . Otherwise, if asend() is used, then the result will be the value passed in to that method.
If an asynchronous generator happens to exit early by break , the caller task being cancelled, or other exceptions, the generator’s async cleanup code will run and possibly raise exceptions or access context variables in an unexpected context–perhaps after the lifetime of tasks it depends, or during the event loop shutdown when the async-generator garbage collection hook is called. To prevent this, the caller must explicitly close the async generator by calling aclose() method to finalize the generator and ultimately detach it from the event loop.
In an asynchronous generator function, yield expressions are allowed anywhere in a try construct. However, if an asynchronous generator is not resumed before it is finalized (by reaching a zero reference count or by being garbage collected), then a yield expression within a try construct could result in a failure to execute pending finally clauses. In this case, it is the responsibility of the event loop or scheduler running the asynchronous generator to call the asynchronous generator-iterator’s aclose() method and run the resulting coroutine object, thus allowing any pending finally clauses to execute.
To take care of finalization upon event loop termination, an event loop should define a finalizer function which takes an asynchronous generator-iterator and presumably calls aclose() and executes the coroutine. This finalizer may be registered by calling sys.set_asyncgen_hooks() . When first iterated over, an asynchronous generator-iterator will store the registered finalizer to be called upon finalization. For a reference example of a finalizer method see the implementation of asyncio.Loop.shutdown_asyncgens in Lib/asyncio/base_events.py .
The expression yield from <expr> is a syntax error when used in an asynchronous generator function.
6.2.9.4. Asynchronous generator-iterator methods ¶
This subsection describes the methods of an asynchronous generator iterator, which are used to control the execution of a generator function.
Returns an awaitable which when run starts to execute the asynchronous generator or resumes it at the last executed yield expression. When an asynchronous generator function is resumed with an __anext__() method, the current yield expression always evaluates to None in the returned awaitable, which when run will continue to the next yield expression. The value of the expression_list of the yield expression is the value of the StopIteration exception raised by the completing coroutine. If the asynchronous generator exits without yielding another value, the awaitable instead raises a StopAsyncIteration exception, signalling that the asynchronous iteration has completed.
This method is normally called implicitly by a async for loop.
Returns an awaitable which when run resumes the execution of the asynchronous generator. As with the send() method for a generator, this “sends” a value into the asynchronous generator function, and the value argument becomes the result of the current yield expression. The awaitable returned by the asend() method will return the next value yielded by the generator as the value of the raised StopIteration , or raises StopAsyncIteration if the asynchronous generator exits without yielding another value. When asend() is called to start the asynchronous generator, it must be called with None as the argument, because there is no yield expression that could receive the value.
Returns an awaitable that raises an exception of type type at the point where the asynchronous generator was paused, and returns the next value yielded by the generator function as the value of the raised StopIteration exception. If the asynchronous generator exits without yielding another value, a StopAsyncIteration exception is raised by the awaitable. If the generator function does not catch the passed-in exception, or raises a different exception, then when the awaitable is run that exception propagates to the caller of the awaitable.
Returns an awaitable that when run will throw a GeneratorExit into the asynchronous generator function at the point where it was paused. If the asynchronous generator function then exits gracefully, is already closed, or raises GeneratorExit (by not catching the exception), then the returned awaitable will raise a StopIteration exception. Any further awaitables returned by subsequent calls to the asynchronous generator will raise a StopAsyncIteration exception. If the asynchronous generator yields a value, a RuntimeError is raised by the awaitable. If the asynchronous generator raises any other exception, it is propagated to the caller of the awaitable. If the asynchronous generator has already exited due to an exception or normal exit, then further calls to aclose() will return an awaitable that does nothing.
6.3. Primaries ¶
Primaries represent the most tightly bound operations of the language. Their syntax is:
6.3.1. Attribute references ¶
An attribute reference is a primary followed by a period and a name:
The primary must evaluate to an object of a type that supports attribute references, which most objects do. This object is then asked to produce the attribute whose name is the identifier. The type and value produced is determined by the object. Multiple evaluations of the same attribute reference may yield different objects.
This production can be customized by overriding the __getattribute__() method or the __getattr__() method. The __getattribute__() method is called first and either returns a value or raises AttributeError if the attribute is not available.
If an AttributeError is raised and the object has a __getattr__() method, that method is called as a fallback.
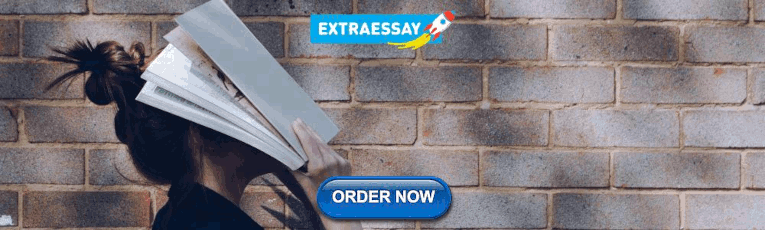
6.3.2. Subscriptions ¶
The subscription of an instance of a container class will generally select an element from the container. The subscription of a generic class will generally return a GenericAlias object.
When an object is subscripted, the interpreter will evaluate the primary and the expression list.
The primary must evaluate to an object that supports subscription. An object may support subscription through defining one or both of __getitem__() and __class_getitem__() . When the primary is subscripted, the evaluated result of the expression list will be passed to one of these methods. For more details on when __class_getitem__ is called instead of __getitem__ , see __class_getitem__ versus __getitem__ .
If the expression list contains at least one comma, it will evaluate to a tuple containing the items of the expression list. Otherwise, the expression list will evaluate to the value of the list’s sole member.
For built-in objects, there are two types of objects that support subscription via __getitem__() :
Mappings. If the primary is a mapping , the expression list must evaluate to an object whose value is one of the keys of the mapping, and the subscription selects the value in the mapping that corresponds to that key. An example of a builtin mapping class is the dict class.
Sequences. If the primary is a sequence , the expression list must evaluate to an int or a slice (as discussed in the following section). Examples of builtin sequence classes include the str , list and tuple classes.
The formal syntax makes no special provision for negative indices in sequences . However, built-in sequences all provide a __getitem__() method that interprets negative indices by adding the length of the sequence to the index so that, for example, x[-1] selects the last item of x . The resulting value must be a nonnegative integer less than the number of items in the sequence, and the subscription selects the item whose index is that value (counting from zero). Since the support for negative indices and slicing occurs in the object’s __getitem__() method, subclasses overriding this method will need to explicitly add that support.
A string is a special kind of sequence whose items are characters . A character is not a separate data type but a string of exactly one character.
6.3.3. Slicings ¶
A slicing selects a range of items in a sequence object (e.g., a string, tuple or list). Slicings may be used as expressions or as targets in assignment or del statements. The syntax for a slicing:
There is ambiguity in the formal syntax here: anything that looks like an expression list also looks like a slice list, so any subscription can be interpreted as a slicing. Rather than further complicating the syntax, this is disambiguated by defining that in this case the interpretation as a subscription takes priority over the interpretation as a slicing (this is the case if the slice list contains no proper slice).
The semantics for a slicing are as follows. The primary is indexed (using the same __getitem__() method as normal subscription) with a key that is constructed from the slice list, as follows. If the slice list contains at least one comma, the key is a tuple containing the conversion of the slice items; otherwise, the conversion of the lone slice item is the key. The conversion of a slice item that is an expression is that expression. The conversion of a proper slice is a slice object (see section The standard type hierarchy ) whose start , stop and step attributes are the values of the expressions given as lower bound, upper bound and stride, respectively, substituting None for missing expressions.
6.3.4. Calls ¶
A call calls a callable object (e.g., a function ) with a possibly empty series of arguments :
An optional trailing comma may be present after the positional and keyword arguments but does not affect the semantics.
The primary must evaluate to a callable object (user-defined functions, built-in functions, methods of built-in objects, class objects, methods of class instances, and all objects having a __call__() method are callable). All argument expressions are evaluated before the call is attempted. Please refer to section Function definitions for the syntax of formal parameter lists.
If keyword arguments are present, they are first converted to positional arguments, as follows. First, a list of unfilled slots is created for the formal parameters. If there are N positional arguments, they are placed in the first N slots. Next, for each keyword argument, the identifier is used to determine the corresponding slot (if the identifier is the same as the first formal parameter name, the first slot is used, and so on). If the slot is already filled, a TypeError exception is raised. Otherwise, the argument is placed in the slot, filling it (even if the expression is None , it fills the slot). When all arguments have been processed, the slots that are still unfilled are filled with the corresponding default value from the function definition. (Default values are calculated, once, when the function is defined; thus, a mutable object such as a list or dictionary used as default value will be shared by all calls that don’t specify an argument value for the corresponding slot; this should usually be avoided.) If there are any unfilled slots for which no default value is specified, a TypeError exception is raised. Otherwise, the list of filled slots is used as the argument list for the call.
CPython implementation detail: An implementation may provide built-in functions whose positional parameters do not have names, even if they are ‘named’ for the purpose of documentation, and which therefore cannot be supplied by keyword. In CPython, this is the case for functions implemented in C that use PyArg_ParseTuple() to parse their arguments.
If there are more positional arguments than there are formal parameter slots, a TypeError exception is raised, unless a formal parameter using the syntax *identifier is present; in this case, that formal parameter receives a tuple containing the excess positional arguments (or an empty tuple if there were no excess positional arguments).
If any keyword argument does not correspond to a formal parameter name, a TypeError exception is raised, unless a formal parameter using the syntax **identifier is present; in this case, that formal parameter receives a dictionary containing the excess keyword arguments (using the keywords as keys and the argument values as corresponding values), or a (new) empty dictionary if there were no excess keyword arguments.
If the syntax *expression appears in the function call, expression must evaluate to an iterable . Elements from these iterables are treated as if they were additional positional arguments. For the call f(x1, x2, *y, x3, x4) , if y evaluates to a sequence y1 , …, yM , this is equivalent to a call with M+4 positional arguments x1 , x2 , y1 , …, yM , x3 , x4 .
A consequence of this is that although the *expression syntax may appear after explicit keyword arguments, it is processed before the keyword arguments (and any **expression arguments – see below). So:
It is unusual for both keyword arguments and the *expression syntax to be used in the same call, so in practice this confusion does not often arise.
If the syntax **expression appears in the function call, expression must evaluate to a mapping , the contents of which are treated as additional keyword arguments. If a parameter matching a key has already been given a value (by an explicit keyword argument, or from another unpacking), a TypeError exception is raised.
When **expression is used, each key in this mapping must be a string. Each value from the mapping is assigned to the first formal parameter eligible for keyword assignment whose name is equal to the key. A key need not be a Python identifier (e.g. "max-temp °F" is acceptable, although it will not match any formal parameter that could be declared). If there is no match to a formal parameter the key-value pair is collected by the ** parameter, if there is one, or if there is not, a TypeError exception is raised.
Formal parameters using the syntax *identifier or **identifier cannot be used as positional argument slots or as keyword argument names.
Changed in version 3.5: Function calls accept any number of * and ** unpackings, positional arguments may follow iterable unpackings ( * ), and keyword arguments may follow dictionary unpackings ( ** ). Originally proposed by PEP 448 .
A call always returns some value, possibly None , unless it raises an exception. How this value is computed depends on the type of the callable object.
The code block for the function is executed, passing it the argument list. The first thing the code block will do is bind the formal parameters to the arguments; this is described in section Function definitions . When the code block executes a return statement, this specifies the return value of the function call.
The result is up to the interpreter; see Built-in Functions for the descriptions of built-in functions and methods.
A new instance of that class is returned.
The corresponding user-defined function is called, with an argument list that is one longer than the argument list of the call: the instance becomes the first argument.
The class must define a __call__() method; the effect is then the same as if that method was called.
6.4. Await expression ¶
Suspend the execution of coroutine on an awaitable object. Can only be used inside a coroutine function .
New in version 3.5.
6.5. The power operator ¶
The power operator binds more tightly than unary operators on its left; it binds less tightly than unary operators on its right. The syntax is:
Thus, in an unparenthesized sequence of power and unary operators, the operators are evaluated from right to left (this does not constrain the evaluation order for the operands): -1**2 results in -1 .
The power operator has the same semantics as the built-in pow() function, when called with two arguments: it yields its left argument raised to the power of its right argument. The numeric arguments are first converted to a common type, and the result is of that type.
For int operands, the result has the same type as the operands unless the second argument is negative; in that case, all arguments are converted to float and a float result is delivered. For example, 10**2 returns 100 , but 10**-2 returns 0.01 .
Raising 0.0 to a negative power results in a ZeroDivisionError . Raising a negative number to a fractional power results in a complex number. (In earlier versions it raised a ValueError .)
This operation can be customized using the special __pow__() method.
6.6. Unary arithmetic and bitwise operations ¶
All unary arithmetic and bitwise operations have the same priority:
The unary - (minus) operator yields the negation of its numeric argument; the operation can be overridden with the __neg__() special method.
The unary + (plus) operator yields its numeric argument unchanged; the operation can be overridden with the __pos__() special method.
The unary ~ (invert) operator yields the bitwise inversion of its integer argument. The bitwise inversion of x is defined as -(x+1) . It only applies to integral numbers or to custom objects that override the __invert__() special method.
In all three cases, if the argument does not have the proper type, a TypeError exception is raised.
6.7. Binary arithmetic operations ¶
The binary arithmetic operations have the conventional priority levels. Note that some of these operations also apply to certain non-numeric types. Apart from the power operator, there are only two levels, one for multiplicative operators and one for additive operators:
The * (multiplication) operator yields the product of its arguments. The arguments must either both be numbers, or one argument must be an integer and the other must be a sequence. In the former case, the numbers are converted to a common type and then multiplied together. In the latter case, sequence repetition is performed; a negative repetition factor yields an empty sequence.
This operation can be customized using the special __mul__() and __rmul__() methods.
The @ (at) operator is intended to be used for matrix multiplication. No builtin Python types implement this operator.
The / (division) and // (floor division) operators yield the quotient of their arguments. The numeric arguments are first converted to a common type. Division of integers yields a float, while floor division of integers results in an integer; the result is that of mathematical division with the ‘floor’ function applied to the result. Division by zero raises the ZeroDivisionError exception.
This operation can be customized using the special __truediv__() and __floordiv__() methods.
The % (modulo) operator yields the remainder from the division of the first argument by the second. The numeric arguments are first converted to a common type. A zero right argument raises the ZeroDivisionError exception. The arguments may be floating point numbers, e.g., 3.14%0.7 equals 0.34 (since 3.14 equals 4*0.7 + 0.34 .) The modulo operator always yields a result with the same sign as its second operand (or zero); the absolute value of the result is strictly smaller than the absolute value of the second operand [ 1 ] .
The floor division and modulo operators are connected by the following identity: x == (x//y)*y + (x%y) . Floor division and modulo are also connected with the built-in function divmod() : divmod(x, y) == (x//y, x%y) . [ 2 ] .
In addition to performing the modulo operation on numbers, the % operator is also overloaded by string objects to perform old-style string formatting (also known as interpolation). The syntax for string formatting is described in the Python Library Reference, section printf-style String Formatting .
The modulo operation can be customized using the special __mod__() method.
The floor division operator, the modulo operator, and the divmod() function are not defined for complex numbers. Instead, convert to a floating point number using the abs() function if appropriate.
The + (addition) operator yields the sum of its arguments. The arguments must either both be numbers or both be sequences of the same type. In the former case, the numbers are converted to a common type and then added together. In the latter case, the sequences are concatenated.
This operation can be customized using the special __add__() and __radd__() methods.
The - (subtraction) operator yields the difference of its arguments. The numeric arguments are first converted to a common type.
This operation can be customized using the special __sub__() method.
6.8. Shifting operations ¶
The shifting operations have lower priority than the arithmetic operations:
These operators accept integers as arguments. They shift the first argument to the left or right by the number of bits given by the second argument.
This operation can be customized using the special __lshift__() and __rshift__() methods.
A right shift by n bits is defined as floor division by pow(2,n) . A left shift by n bits is defined as multiplication with pow(2,n) .
6.9. Binary bitwise operations ¶
Each of the three bitwise operations has a different priority level:
The & operator yields the bitwise AND of its arguments, which must be integers or one of them must be a custom object overriding __and__() or __rand__() special methods.
The ^ operator yields the bitwise XOR (exclusive OR) of its arguments, which must be integers or one of them must be a custom object overriding __xor__() or __rxor__() special methods.
The | operator yields the bitwise (inclusive) OR of its arguments, which must be integers or one of them must be a custom object overriding __or__() or __ror__() special methods.
6.10. Comparisons ¶
Unlike C, all comparison operations in Python have the same priority, which is lower than that of any arithmetic, shifting or bitwise operation. Also unlike C, expressions like a < b < c have the interpretation that is conventional in mathematics:
Comparisons yield boolean values: True or False . Custom rich comparison methods may return non-boolean values. In this case Python will call bool() on such value in boolean contexts.
Comparisons can be chained arbitrarily, e.g., x < y <= z is equivalent to x < y and y <= z , except that y is evaluated only once (but in both cases z is not evaluated at all when x < y is found to be false).
Formally, if a , b , c , …, y , z are expressions and op1 , op2 , …, opN are comparison operators, then a op1 b op2 c ... y opN z is equivalent to a op1 b and b op2 c and ... y opN z , except that each expression is evaluated at most once.
Note that a op1 b op2 c doesn’t imply any kind of comparison between a and c , so that, e.g., x < y > z is perfectly legal (though perhaps not pretty).
6.10.1. Value comparisons ¶
The operators < , > , == , >= , <= , and != compare the values of two objects. The objects do not need to have the same type.
Chapter Objects, values and types states that objects have a value (in addition to type and identity). The value of an object is a rather abstract notion in Python: For example, there is no canonical access method for an object’s value. Also, there is no requirement that the value of an object should be constructed in a particular way, e.g. comprised of all its data attributes. Comparison operators implement a particular notion of what the value of an object is. One can think of them as defining the value of an object indirectly, by means of their comparison implementation.
Because all types are (direct or indirect) subtypes of object , they inherit the default comparison behavior from object . Types can customize their comparison behavior by implementing rich comparison methods like __lt__() , described in Basic customization .
The default behavior for equality comparison ( == and != ) is based on the identity of the objects. Hence, equality comparison of instances with the same identity results in equality, and equality comparison of instances with different identities results in inequality. A motivation for this default behavior is the desire that all objects should be reflexive (i.e. x is y implies x == y ).
A default order comparison ( < , > , <= , and >= ) is not provided; an attempt raises TypeError . A motivation for this default behavior is the lack of a similar invariant as for equality.
The behavior of the default equality comparison, that instances with different identities are always unequal, may be in contrast to what types will need that have a sensible definition of object value and value-based equality. Such types will need to customize their comparison behavior, and in fact, a number of built-in types have done that.
The following list describes the comparison behavior of the most important built-in types.
Numbers of built-in numeric types ( Numeric Types — int, float, complex ) and of the standard library types fractions.Fraction and decimal.Decimal can be compared within and across their types, with the restriction that complex numbers do not support order comparison. Within the limits of the types involved, they compare mathematically (algorithmically) correct without loss of precision.
The not-a-number values float('NaN') and decimal.Decimal('NaN') are special. Any ordered comparison of a number to a not-a-number value is false. A counter-intuitive implication is that not-a-number values are not equal to themselves. For example, if x = float('NaN') , 3 < x , x < 3 and x == x are all false, while x != x is true. This behavior is compliant with IEEE 754.
None and NotImplemented are singletons. PEP 8 advises that comparisons for singletons should always be done with is or is not , never the equality operators.
Binary sequences (instances of bytes or bytearray ) can be compared within and across their types. They compare lexicographically using the numeric values of their elements.
Strings (instances of str ) compare lexicographically using the numerical Unicode code points (the result of the built-in function ord() ) of their characters. [ 3 ]
Strings and binary sequences cannot be directly compared.
Sequences (instances of tuple , list , or range ) can be compared only within each of their types, with the restriction that ranges do not support order comparison. Equality comparison across these types results in inequality, and ordering comparison across these types raises TypeError .
Sequences compare lexicographically using comparison of corresponding elements. The built-in containers typically assume identical objects are equal to themselves. That lets them bypass equality tests for identical objects to improve performance and to maintain their internal invariants.
Lexicographical comparison between built-in collections works as follows:
For two collections to compare equal, they must be of the same type, have the same length, and each pair of corresponding elements must compare equal (for example, [1,2] == (1,2) is false because the type is not the same).
Collections that support order comparison are ordered the same as their first unequal elements (for example, [1,2,x] <= [1,2,y] has the same value as x <= y ). If a corresponding element does not exist, the shorter collection is ordered first (for example, [1,2] < [1,2,3] is true).
Mappings (instances of dict ) compare equal if and only if they have equal (key, value) pairs. Equality comparison of the keys and values enforces reflexivity.
Order comparisons ( < , > , <= , and >= ) raise TypeError .
Sets (instances of set or frozenset ) can be compared within and across their types.
They define order comparison operators to mean subset and superset tests. Those relations do not define total orderings (for example, the two sets {1,2} and {2,3} are not equal, nor subsets of one another, nor supersets of one another). Accordingly, sets are not appropriate arguments for functions which depend on total ordering (for example, min() , max() , and sorted() produce undefined results given a list of sets as inputs).
Comparison of sets enforces reflexivity of its elements.
Most other built-in types have no comparison methods implemented, so they inherit the default comparison behavior.
User-defined classes that customize their comparison behavior should follow some consistency rules, if possible:
Equality comparison should be reflexive. In other words, identical objects should compare equal:
x is y implies x == y
Comparison should be symmetric. In other words, the following expressions should have the same result:
x == y and y == x x != y and y != x x < y and y > x x <= y and y >= x
Comparison should be transitive. The following (non-exhaustive) examples illustrate that:
x > y and y > z implies x > z x < y and y <= z implies x < z
Inverse comparison should result in the boolean negation. In other words, the following expressions should have the same result:
x == y and not x != y x < y and not x >= y (for total ordering) x > y and not x <= y (for total ordering)
The last two expressions apply to totally ordered collections (e.g. to sequences, but not to sets or mappings). See also the total_ordering() decorator.
The hash() result should be consistent with equality. Objects that are equal should either have the same hash value, or be marked as unhashable.
Python does not enforce these consistency rules. In fact, the not-a-number values are an example for not following these rules.
6.10.2. Membership test operations ¶
The operators in and not in test for membership. x in s evaluates to True if x is a member of s , and False otherwise. x not in s returns the negation of x in s . All built-in sequences and set types support this as well as dictionary, for which in tests whether the dictionary has a given key. For container types such as list, tuple, set, frozenset, dict, or collections.deque, the expression x in y is equivalent to any(x is e or x == e for e in y) .
For the string and bytes types, x in y is True if and only if x is a substring of y . An equivalent test is y.find(x) != -1 . Empty strings are always considered to be a substring of any other string, so "" in "abc" will return True .
For user-defined classes which define the __contains__() method, x in y returns True if y.__contains__(x) returns a true value, and False otherwise.
For user-defined classes which do not define __contains__() but do define __iter__() , x in y is True if some value z , for which the expression x is z or x == z is true, is produced while iterating over y . If an exception is raised during the iteration, it is as if in raised that exception.
Lastly, the old-style iteration protocol is tried: if a class defines __getitem__() , x in y is True if and only if there is a non-negative integer index i such that x is y[i] or x == y[i] , and no lower integer index raises the IndexError exception. (If any other exception is raised, it is as if in raised that exception).
The operator not in is defined to have the inverse truth value of in .
6.10.3. Identity comparisons ¶
The operators is and is not test for an object’s identity: x is y is true if and only if x and y are the same object. An Object’s identity is determined using the id() function. x is not y yields the inverse truth value. [ 4 ]
6.11. Boolean operations ¶
In the context of Boolean operations, and also when expressions are used by control flow statements, the following values are interpreted as false: False , None , numeric zero of all types, and empty strings and containers (including strings, tuples, lists, dictionaries, sets and frozensets). All other values are interpreted as true. User-defined objects can customize their truth value by providing a __bool__() method.
The operator not yields True if its argument is false, False otherwise.
The expression x and y first evaluates x ; if x is false, its value is returned; otherwise, y is evaluated and the resulting value is returned.
The expression x or y first evaluates x ; if x is true, its value is returned; otherwise, y is evaluated and the resulting value is returned.
Note that neither and nor or restrict the value and type they return to False and True , but rather return the last evaluated argument. This is sometimes useful, e.g., if s is a string that should be replaced by a default value if it is empty, the expression s or 'foo' yields the desired value. Because not has to create a new value, it returns a boolean value regardless of the type of its argument (for example, not 'foo' produces False rather than '' .)
6.12. Assignment expressions ¶
An assignment expression (sometimes also called a “named expression” or “walrus”) assigns an expression to an identifier , while also returning the value of the expression .
One common use case is when handling matched regular expressions:
Or, when processing a file stream in chunks:
Assignment expressions must be surrounded by parentheses when used as expression statements and when used as sub-expressions in slicing, conditional, lambda, keyword-argument, and comprehension-if expressions and in assert , with , and assignment statements. In all other places where they can be used, parentheses are not required, including in if and while statements.
New in version 3.8: See PEP 572 for more details about assignment expressions.
6.13. Conditional expressions ¶
Conditional expressions (sometimes called a “ternary operator”) have the lowest priority of all Python operations.
The expression x if C else y first evaluates the condition, C rather than x . If C is true, x is evaluated and its value is returned; otherwise, y is evaluated and its value is returned.
See PEP 308 for more details about conditional expressions.
6.14. Lambdas ¶
Lambda expressions (sometimes called lambda forms) are used to create anonymous functions. The expression lambda parameters: expression yields a function object. The unnamed object behaves like a function object defined with:
See section Function definitions for the syntax of parameter lists. Note that functions created with lambda expressions cannot contain statements or annotations.
6.15. Expression lists ¶
Except when part of a list or set display, an expression list containing at least one comma yields a tuple. The length of the tuple is the number of expressions in the list. The expressions are evaluated from left to right.
An asterisk * denotes iterable unpacking . Its operand must be an iterable . The iterable is expanded into a sequence of items, which are included in the new tuple, list, or set, at the site of the unpacking.
New in version 3.5: Iterable unpacking in expression lists, originally proposed by PEP 448 .
A trailing comma is required only to create a one-item tuple, such as 1, ; it is optional in all other cases. A single expression without a trailing comma doesn’t create a tuple, but rather yields the value of that expression. (To create an empty tuple, use an empty pair of parentheses: () .)
6.16. Evaluation order ¶
Python evaluates expressions from left to right. Notice that while evaluating an assignment, the right-hand side is evaluated before the left-hand side.
In the following lines, expressions will be evaluated in the arithmetic order of their suffixes:
6.17. Operator precedence ¶
The following table summarizes the operator precedence in Python, from highest precedence (most binding) to lowest precedence (least binding). Operators in the same box have the same precedence. Unless the syntax is explicitly given, operators are binary. Operators in the same box group left to right (except for exponentiation and conditional expressions, which group from right to left).
Note that comparisons, membership tests, and identity tests, all have the same precedence and have a left-to-right chaining feature as described in the Comparisons section.
Table of Contents
- 6.1. Arithmetic conversions
- 6.2.1. Identifiers (Names)
- 6.2.2. Literals
- 6.2.3. Parenthesized forms
- 6.2.4. Displays for lists, sets and dictionaries
- 6.2.5. List displays
- 6.2.6. Set displays
- 6.2.7. Dictionary displays
- 6.2.8. Generator expressions
- 6.2.9.1. Generator-iterator methods
- 6.2.9.2. Examples
- 6.2.9.3. Asynchronous generator functions
- 6.2.9.4. Asynchronous generator-iterator methods
- 6.3.1. Attribute references
- 6.3.2. Subscriptions
- 6.3.3. Slicings
- 6.3.4. Calls
- 6.4. Await expression
- 6.5. The power operator
- 6.6. Unary arithmetic and bitwise operations
- 6.7. Binary arithmetic operations
- 6.8. Shifting operations
- 6.9. Binary bitwise operations
- 6.10.1. Value comparisons
- 6.10.2. Membership test operations
- 6.10.3. Identity comparisons
- 6.11. Boolean operations
- 6.12. Assignment expressions
- 6.13. Conditional expressions
- 6.14. Lambdas
- 6.15. Expression lists
- 6.16. Evaluation order
- 6.17. Operator precedence
Previous topic
5. The import system
7. Simple statements
- Report a Bug
- Show Source
Conditional Assignment Operator in Python
- Python How-To's
- Conditional Assignment Operator in …
Meaning of ||= Operator in Ruby
Implement ruby’s ||= conditional assignment operator in python using the try...except statement, implement ruby’s ||= conditional assignment operator in python using local and global variables.
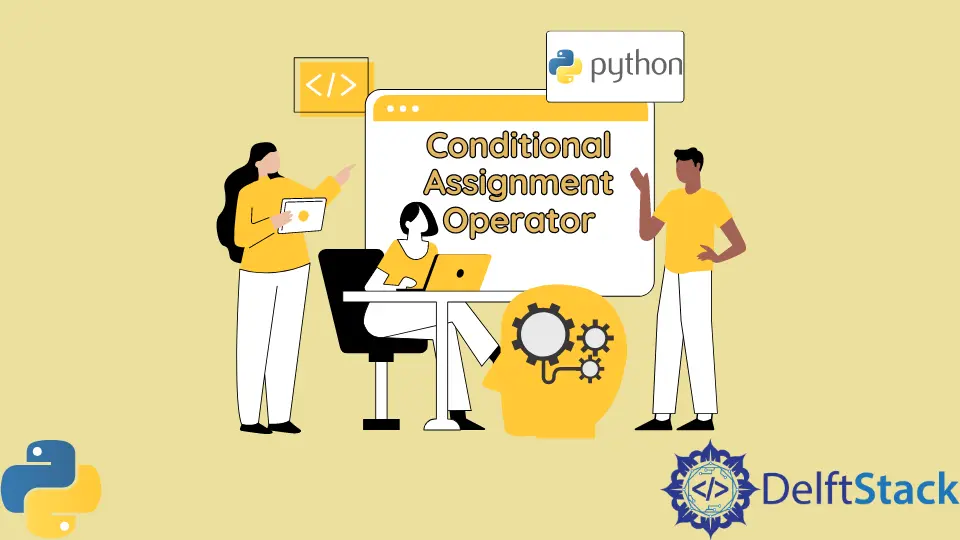
There isn’t any exact equivalent of Ruby’s ||= operator in Python. However, we can use the try...except method and concepts of local and global variables to emulate Ruby’s conditional assignment operator ||= in Python.
The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed.
But this operator is much more complex and confusing than other simpler conditional operators like += , -= because whenever any variable is encountered as undefined, the console throws out NameError .
a+=b evaluates to a=a+b .
a||=b looks as a=a||b but actually behaves as a||a=b .
We use try...except to catch and handle errors. Whenever the try except block runs, at first, the code lying within the try block executes. If the block of code within the try block successfully executes, then the except block is ignored; otherwise, the except block code will be executed, and the error is handled. Ruby’s ||= operator can roughly be translated in Python’s try-catch method as :
Here, if the variable x is defined, the try block will execute smoothly with no NameError exception. Hence, no assignment operation is performed. If x is not defined, the try block will generate NameError , then the except block gets executed, and variable x is assigned to 10 .
The scope of local variables is confined within a specific code scope, whereas global variables have their scope defined in the entire code space.
All the local variables in a particular scope are available as keys of the locals dictionary in that particular scope. All the global variables are stored as keys of the globals dictionary. We can access those variables whenever necessary using the locals and the globals dictionary.
We can check if a variable exists in any of the dictionaries and set its value only if it does not exist to translate Ruby’s ||= conditional assignment operator in Python.
Here, if the variable x is present in either global or local scope, we don’t perform any assignment operation; otherwise, we assign the value of x to 10 . It is similar to x||=10 in Ruby.
Related Article - Python Operator
- Python Bitwise NOT
- How to Unpack Operator ** in Python
- How to Overload Operator in Python
- Python Annotation ->
- The Walrus Operator := in Python
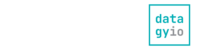
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Inline If in Python: The Ternary Operator in Python
- September 16, 2021 December 20, 2022
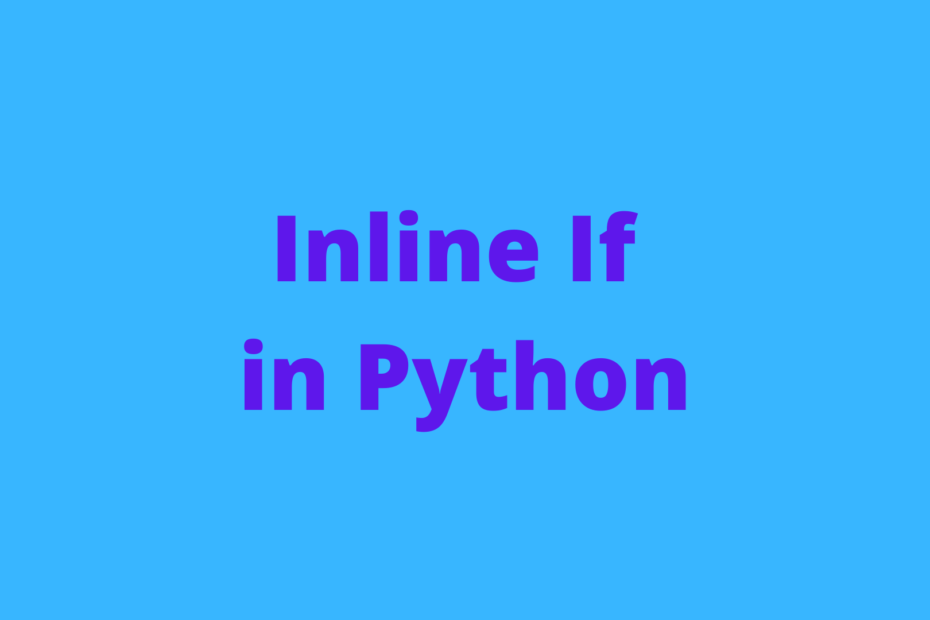
In this tutorial, you’ll learn how to create inline if statements in Python. This is often known as the Python ternary operator, which allows you to execute conditional if statements in a single line, allowing statements to take up less space and often be written in my easy-to-understand syntax! Let’s take a look at what you’ll learn.
The Quick Answer: Use the Python Ternary Operator
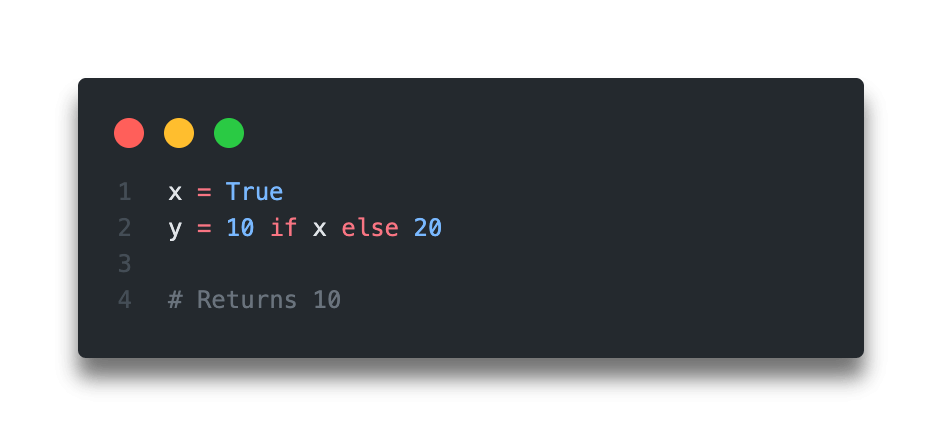
Table of Contents
What is the Python Ternary Operator?
A ternary operator is an inline statement that evaluates a condition and returns one of two outputs. It’s an operator that’s often used in many programming languages, including Python, as well as math. The Python ternary operator has been around since Python 2.5, despite being delayed multiple times.
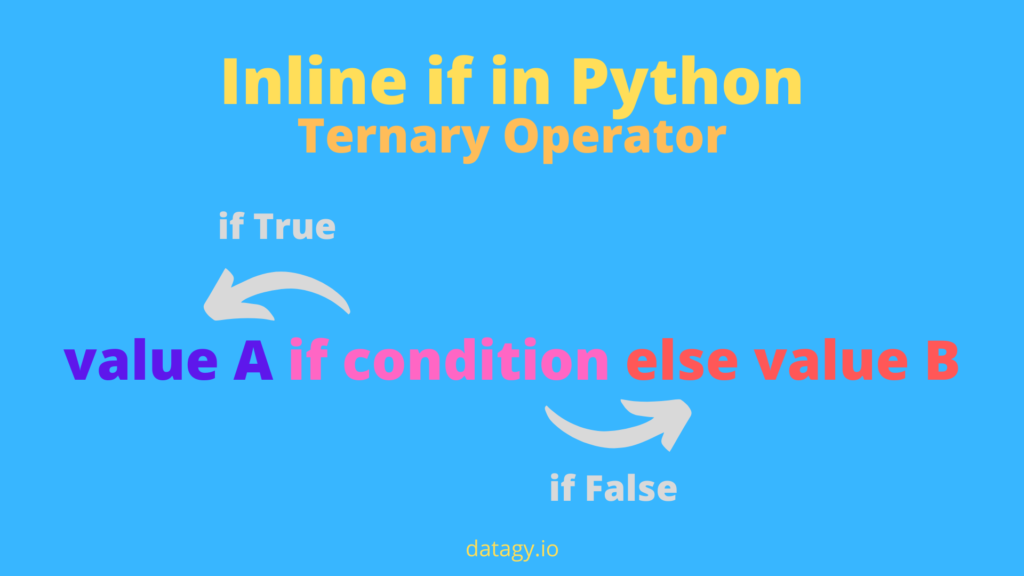
The syntax of the Python ternary operator is a little different than that of other languages. Let’s take a look at what it looks like:
Now let’s take a look at how you can actually write an inline if statement in Python.
How Do you Write an Inline If Statement in Python?
Before we dive into writing an inline if statement in Python, let’s take a look at how if statements actually work in Python. With an if statement you must include an if , but you can also choose to include an else statement, as well as one more of else-ifs, which in Python are written as elif .
The traditional Python if statement looks like this:
This can be a little cumbersome to write, especially if you conditions are very simple. Because of this, inline if statements in Python can be really helpful to help you write your code faster.
Let’s take a look at how we can accomplish this in Python:
This is significantly easier to write. Let’s break this down a little bit:
- We assign a value to x , which will be evaluated
- We declare a variable, y , which we assign to the value of 10, if x is True. Otherwise, we assign it a value of 20.
We can see how this is written out in a much more plain language than a for-loop that may require multiple lines, thereby wasting space.
Tip! This is quite similar to how you’d written a list comprehension. If you want to learn more about Python List Comprehensions, check out my in-depth tutorial here . If you want to learn more about Python for-loops, check out my in-depth guide here .
Now that you know how to write a basic inline if statement in Python, let’s see how you can simplify it even further by omitting the else statement.
How To Write an Inline If Statement Without an Else Statement
Now that you know how to write an inline if statement in Python with an else clause, let’s take a look at how we can do this in Python.
Before we do this, let’s see how we can do this with a traditional if statement in Python
You can see that this still requires you to write two lines. But we know better – we can easily cut this down to a single line. Let’s get started!
We can see here that really what this accomplishes is remove the line break between the if line and the code it executes.
Now let’s take a look at how we can even include an elif clause in our inline if statements in Python!
Check out some other Python tutorials on datagy.io, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas !
How to Write an Inline If Statement With an Elif Statement
Including an else-if, or elif , in your Python inline if statement is a little less intuitive. But it’s definitely doable! So let’s get started. Let’s imagine we want to write this if-statement:
Let’s see how we can easily turn this into an inline if statement in Python:
This is a bit different than what we’ve seen so far, so let’s break it down a bit:
- First, we evaluate is x == 1. If that’s true, the conditions end and y = 10.
- Otherwise, we create another condition in brackets
- First we check if x == 20, and if that’s true, then y = 20. Note that we did not repeated y= here.
- Finally, if neither of the other decisions are true, we assign 30 to y
This is definitely a bit more complex to read, so you may be better off creating a traditional if statement.
In this post, you learned how to create inline if statement in Python! You learned about the Python ternary operator and how it works. You also learned how to create inline if statements with else statements, without else statements, as well as with else if statements.
To learn more about Python ternary operators, check out the official documentation here .
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Python Ternary For Loop: Simplifying Conditional Expressions in Iterations
Python’s ternary operator , also known as the conditional operator , evaluates a condition and returns one of two values, depending on whether the condition is true or false. This useful feature enables you to write clean and concise Python code, using one line to achieve the functionality of an if-else statement.
💡 Tip : Like a nested if ... else statement, you can use a nested ternary to express multiple conditions in a single line.
Python’s ternary operator is often used in conjunction with for loops to create more compact and readable code.
When working with for loops in Python, you might find yourself needing to apply different actions to elements in your loop based on specific conditions.
⭐ Instead of writing lengthy if-else statements within the loop, the ternary operator can streamline the process and make your code easier to understand.
Understanding Python Ternary For Loop

Basics of Ternary Operator
The ternary operator is a concise way to express a conditional expression in Python. It is essentially a one-line alternative to the traditional if-else statement. It evaluates a condition and returns one of the two possible values based on whether the condition evaluates to true or false.
Here’s a basic example:
In this case, the result variable will be assigned the value "Even" if x is divisible by 2 and "Odd" otherwise.
Syntax and Expressions of Ternary For Loop
A ternary for loop in Python combines the concepts of the ternary operator and for loop to create a compact looping structure. It is not a built-in Python feature but can be achieved using a combination of assignment statements and conditional expressions.
The general syntax for a ternary for loop is:
Here’s an example that squares each element in a list if it’s even and adds 1 to each odd element:
In this case, the result variable will be a new list with the transformed values [2, 4, 4, 16, 6] .
Function of Lambda with Ternary Operator
The power of the ternary operator can be further enhanced by using it in conjunction with lambda functions.
💡 Info : Lambda functions are small, anonymous functions that can accept multiple inputs and return a single expression. They are useful for quick in-line operations where a full function definition might be undesired or unnecessary.
The ternary operator can be incorporated into a lambda function like this:
You can use lambda functions with ternary operators inside a for loop or with functions like map() and filter() to create more complex and efficient looping structures.
Here’s an example that squares each even element and increments each odd element in a list:
The result, just like before, would be the transformed list [2, 4, 4, 16, 6] .
🛑 Keep in mind that using ternary operators and lambda functions can reduce readability, so use them judiciously.
Practical Examples with Limitations
In Python, you can use ternary for loops with list comprehensions, tuples, dictionary, and lambda functions. However, there are some limitations to consider when using ternary operators in these scenarios. Let’s explore a few examples and discuss their readability,
Example 1: List Comprehension
Utilizing a ternary operator within list comprehension can be a concise way to handle conditional statements.
Here’s a basic implementation:
In this example, the result is [-1, 2, -3, 4, -5] and each even number will remain the same, while the odd numbers are negated.
Even though this example is pretty simple, combining multiple expressions or nested ternary operators may reduce the readability.
Example 2: Tuple Unpacking
You can use tuples to incorporate ternary operators by using index-based access. However, make sure you understand that this method is not exactly the same as using a regular ternary operator.
Nevertheless, it provides a similar functionality:
In this case, greet_person will equal 'Hi' .
While this may look like a ternary operator, it is actually using tuple indexing. The greet boolean variable is implicitly converted to an integer (False -> 0, True -> 1) and thus indexes the tuple accordingly.
Example 3: Dictionary and Lambda Function
When handling multiple conditions, a dictionary with lambda functions may offer a cleaner solution compared to a nested ternary operator.
For instance, consider the following age-based scenario:
This will assign 'Adult' to age_group . Although it seems complex, once you are familiar with lambda functions, it can be more readable compared to a deeply nested ternary operator.
😂 Disclaimer : While ternary operators provide a concise way of expressing conditional statements in Python, you should be aware of the potential limitations, especially when it comes to readability. Additionally, remember that ternary operators are unique to Python and may not be directly translatable to languages like Java. Ensure that your usage of ternary operators is successful by maintaining simplicity and prioritizing clarity over brevity.
Frequently Asked Questions
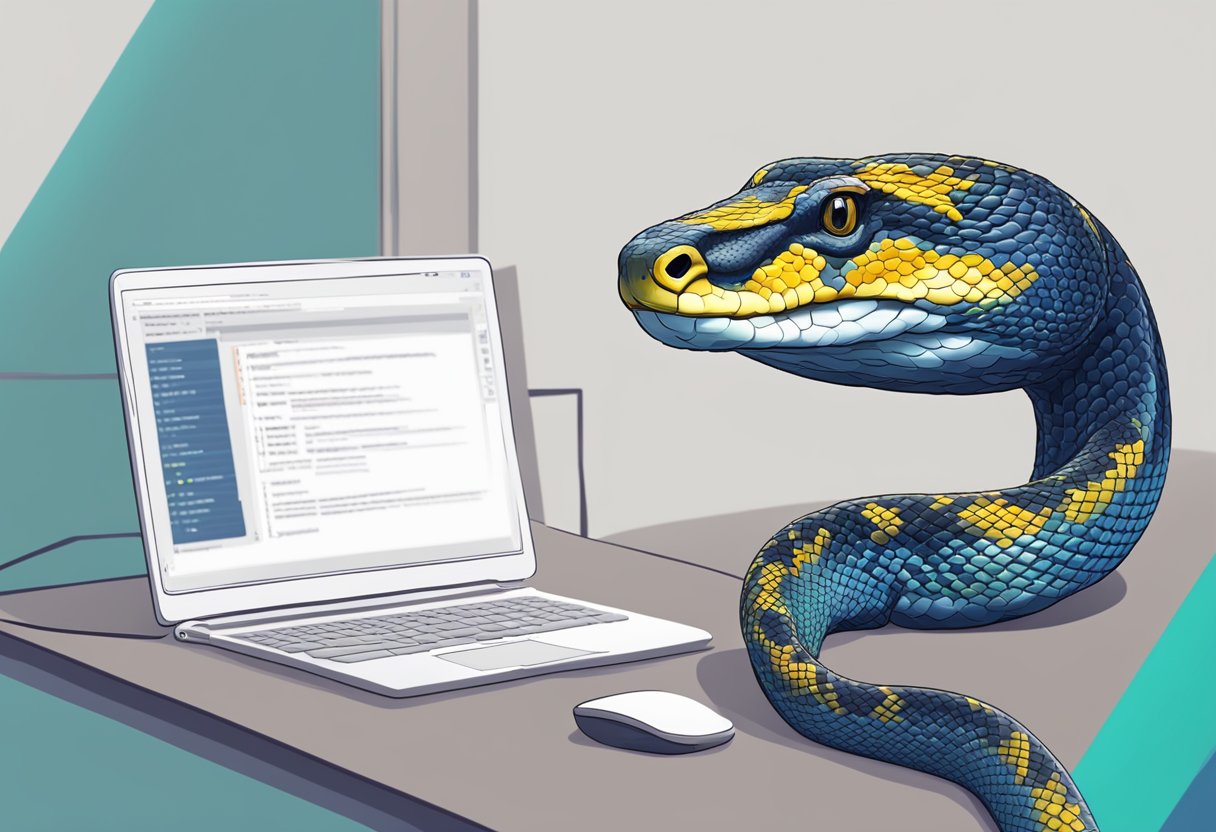
How do you use a ternary operator in Python list comprehension?
You can use a ternary operator in Python list comprehension to create a new list with elements based on a condition. Here’s the syntax:
new_list = [value_if_true if condition else value_if_false for item in old_list]
An example of using a ternary operator in list comprehension:
What is the syntax for Python ternary conditional operator?
The syntax for the ternary conditional operator in Python follows this pattern:
value_if_true if condition else value_if_false
For example:
Can you apply ternary operators in function arguments in Python?
Yes, you can apply ternary operators in function arguments in Python. It allows you to conditionally pass different arguments depending on a condition. For instance:
Are ternary operators faster than if-else in Python?
In most cases, ternary operators and if-else statements have similar performance. Ternary operators can be faster and more concise in some cases, such as when used in list comprehensions. However, the difference in performance is usually negligible and should not be the primary reason for using one over the other.
How to use ternary operator for conditional assignment in Python?
To use a ternary operator for conditional assignment in Python, follow this syntax:
variable = value_if_true if condition else value_if_false
For example, assigning the larger value to a variable:
What is the equivalent of the null-conditional operator in Python?
In Python, you can use the ternary operator to achieve similar functionality to a null-conditional operator by checking for None :
variable = value_if_not_none if variable is not None else default_value
For example, getting the length of a string or returning 0 if the string is None :
To learn more about the ternary operator, check out our full tutorial on the Finxter blog. 👇
💡 Recommended : Python One Line Ternary
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
How to Write the Python if Statement in one Line

- online practice
Have you ever heard of writing a Python if statement in a single line? Here, we explore multiple ways to do exactly that, including using conditional expressions in Python.
The if statement is one of the most fundamental statements in Python. In this article, we learn how to write the Python if in one line.
The if is a key piece in writing Python code. It allows developers to control the flow and logic of their code based on information received at runtime. However, many Python developers do not know they may reduce the length and complexity of their if statements by writing them in a single line.
For this article, we assume you’re somewhat familiar with Python conditions and comparisons. If not, don’t worry! Our Python Basics Course will get you up to speed in no time. This course is included in the Python Basics Track , a full-fledged Python learning track designed for complete beginners.
We start with a recap on how Python if statements work. Then, we explore some examples of how to write if statements in a single line. Let’s get started!
How the if Statement Works in Python
Let’s start with the basics. An if statement in Python is used to determine whether a condition is True or False . This information can then be used to perform specific actions in the code, essentially controlling its logic during execution.
The structure of the basic if statement is as follows:
The <expression> is the code that evaluates to either True or False . If this code evaluates to True, then the code below (represented by <perform_action> ) executes.
Python uses whitespaces to indicate which lines are controlled by the if statement. The if statement controls all indented lines below it. Typically, the indentation is set to four spaces (read this post if you’re having trouble with the indentation ).
As a simple example, the code below prints a message if and only if the current weather is sunny:
The if statement in Python has two optional components: the elif statement, which executes only if the preceding if/elif statements are False ; and the else statement, which executes only if all of the preceding if/elif statements are False. While we may have as many elif statements as we want, we may only have a single else statement at the very end of the code block.
Here’s the basic structure:
Here’s how our previous example looks after adding elif and else statements. Change the value of the weather variable to see a different message printed:
How to Write a Python if in one Line
Writing an if statement in Python (along with the optional elif and else statements) uses a lot of whitespaces. Some people may find it confusing or tiresome to follow each statement and its corresponding indented lines.
To overcome this, there is a trick many Python developers often overlook: write an if statement in a single line !
Though not the standard, Python does allow us to write an if statement and its associated action in the same line. Here’s the basic structure:
As you can see, not much has changed. We simply need to “pull” the indented line <perform_action> up to the right of the colon character ( : ). It’s that simple!
Let’s check it with a real example. The code below works as it did previously despite the if statement being in a single line. Test it out and see for yourself:
Writing a Python if Statement With Multiple Actions in one Line
That’s all well and good, but what if my if statement has multiple actions under its control? When using the standard indentation, we separate different actions in multiple indented lines as the structure below shows:
Can we do this in a single line? The surprising answer is yes! We use semicolons to separate each action in the same line as if placed in different lines.
Here’s how the structure looks:
And an example of this functionality:
Have you noticed how each call to the print() function appears in its own line? This indicates we have successfully executed multiple actions from a single line. Nice!
By the way, interested in learning more about the print() function? We have an article on the ins and outs of the print() function .
Writing a Full Python if/elif/else Block Using Single Lines
You may have seen this coming, but we can even write elif and else statements each in a single line. To do so, we use the same syntax as writing an if statement in a single line.
Here’s the general structure:
Looks simple, right? Depending on the content of your expressions and actions, you may find this structure easier to read and understand compared to the indented blocks.
Here’s our previous example of a full if/elif/else block, rewritten as single lines:
Using Python Conditional Expressions to Write an if/else Block in one Line
There’s still a final trick to writing a Python if in one line. Conditional expressions in Python (also known as Python ternary operators) can run an if/else block in a single line.
A conditional expression is even more compact! Remember it took at least two lines to write a block containing both if and else statements in our last example.
In contrast, here’s how a conditional expression is structured:
The syntax is somewhat harder to follow at first, but the basic idea is that <expression> is a test. If the test evaluates to True , then <value_if_true> is the result. Otherwise, the expression results in <value_if_false> .
As you can see, conditional expressions always evaluate to a single value in the end. They are not complete replacements for an if/elif/else block. In fact, we cannot have elif statements in them at all. However, they’re most helpful when determining a single value depending on a single condition.
Take a look at the code below, which determines the value of is_baby depending on whether or not the age is below five:
This is the exact use case for a conditional expression! Here’s how we rewrite this if/else block in a single line:
Much simpler!
Go Even Further With Python!
We hope you now know many ways to write a Python if in one line. We’ve reached the end of the article, but don’t stop practicing now!
If you do not know where to go next, read this post on how to get beyond the basics in Python . If you’d rather get technical, we have a post on the best code editors and IDEs for Python . Remember to keep improving!
You may also like
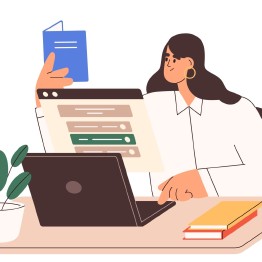
How Do You Write a SELECT Statement in SQL?
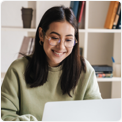
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
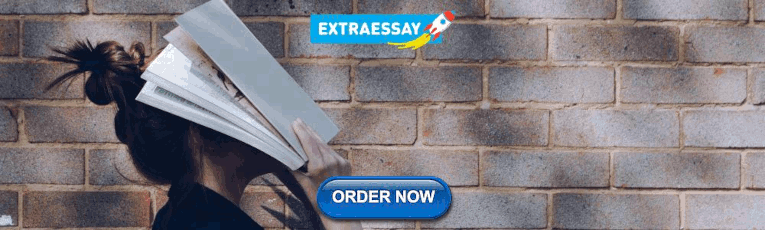
IMAGES
VIDEO
COMMENTS
Learn how to use the if statement and other control structures to perform conditional execution of statements or groups of statements in Python. The tutorial covers the basics of the if statement, grouping statements, the else and elif clauses, the one-line if statement, the ternary operator, the pass statement and more.
Learn how to assign a value to a variable based on some condition using ternary operator, if-else statement, or logical short circuit evaluation. See examples of using conditional assignment with lists, numbers, and strings.
For more conditional code: a = b if b else val For your code: a = get_something() if get_something() else val With that you can do complex conditions like this: ... Python conditional assignment operator. Related. 5. Python: avoiding if condition? 5. pythonic way to rewrite an assignment in an if statement. 3.
Ternary Expression Conditional Statements in Python The Python ternary Expression determines if a condition is true or false and then returns the appropriate value in accordance with the result. The ternary Expression is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more ...
Learn how to use the conditional expression (ternary operator) in Python to write one-line if statements. See examples of basic, multiple, and nested conditional expressions, and how to apply them to list comprehensions and lambda expressions.
Learn how to use the Python ternary operator (or conditional operator) to test a condition and return a value in one line of code. See when it's useful, how it differs from the if-else statement, and what are its limitations.
Learn how to use if, else, and elif statements in Python to make decisions based on the values of variables or the result of comparisons. See examples of how to use them in practice, such as checking if a number is positive, negative, or zero, or assigning a letter grade based on a score.
In this guide, we covered the basics of conditionals in Python including operators, complex conditional chains, nesting conditionals, ternary expressions, and common errors. We examined real-world examples of using conditional logic for input validation, handling user types, recommendation systems, data analysis, and game mechanics.
In its simplest form, a conditional statement requires only an if clause. else and elif clauses can only follow an if clause. # A conditional statement consisting of # an "if"-clause, only. x = -1 if x < 0: x = x ** 2 # x is now 1. Similarly, conditional statements can have an if and an else without an elif:
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Learn how to write expressions in Python, including arithmetic conversions, atoms, parenthesized forms, and displays for lists, sets and dictionaries. See the syntax rules, examples, and notes for each element of expressions.
Meaning of ||= Operator in Ruby. x ||= y. The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed. But this operator is much more complex and confusing than other simpler conditional operators like +=, -= because ...
Method 1: Ternary Operator. The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True. Otherwise, if the expression c evaluates to False, the ternary operator returns the alternative expression y. <OnTrue> if <Condition> else <OnFalse>. Operand.
A ternary operator is an inline statement that evaluates a condition and returns one of two outputs. It's an operator that's often used in many programming languages, including Python, as well as math. The Python ternary operator has been around since Python 2.5, despite being delayed multiple times.
The syntax for a Python ternary conditional operator is: value_if_true if condition else value_if_false. This code evaluates the condition. If it's true, the code returns the value of value_if_true; if it's false, it returns the value of value_if_false.It's important to note that the if and else are mandatory.. Let's look at an example to see how the Python conditional expression works:
A ternary for loop in Python combines the concepts of the ternary operator and for loop to create a compact looping structure. It is not a built-in Python feature but can be achieved using a combination of assignment statements and conditional expressions. The general syntax for a ternary for loop is:
To overcome this, there is a trick many Python developers often overlook: write an if statement in a single line! Though not the standard, Python does allow us to write an if statement and its associated action in the same line. Here's the basic structure: if <expression>: <perform_action></perform_action></expression>.
PEP 572 seeks to add assignment expressions (or "inline assignments") to the language, but it has seen a prolonged discussion over multiple huge threads on the python-dev mailing list—even after multiple rounds on python-ideas. Those threads were often contentious and were clearly voluminous to the point where many probably just tuned them out.
(Type import this at the Python prompt to read the whole thing). You can use a ternary expression in Python, but only for expressions, not for statements: >>> a = "Hello" if foo() else "Goodbye" ... Sometimes with a simple conditional assignment as your example, it is possible to use a mathematical expression to perform the conditional ...