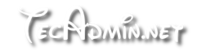
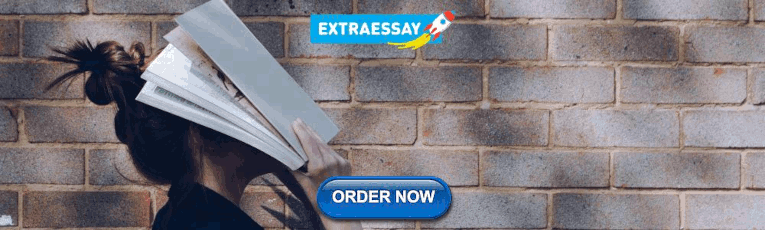
Assignment Operators in Bash
In the Bash shell, assignment operators are used to assign values to variables. They are essential tools in scripting and programming, providing a method to store and manipulate data. This article will take you through the fundamental assignment operators in Bash, along with examples of their usage.
Standard Assignment Operator
In Bash, the standard assignment operator is the `=` symbol. It is used to assign the value on the right-hand side to the variable on the left-hand side. There should not be any spaces around the `=` operator. Here is an example:
In this example, the variable `NAME` is assigned the value “John Doe” . If you use `echo $NAME` , the output will be “John Doe” .
Compound Assignment Operators
Compound assignment operators combine an operation and an assignment into a single operation.
Please note that Bash only supports integer arithmetic natively. If you need to perform operations with floating-point numbers, you will need to use external tools like bc.
Read-only Assignment Operator
The readonly operator is used to make a variable’s value constant, which means the value assigned to the variable cannot be changed later. If you try to change the value of a readonly variable, Bash will give an error.
In the above example, PI is declared as a readonly variable and assigned a value of 3.14 . When we try to reassign the value 3.1415 to PI , Bash will give an error message: bash: PI: readonly variable .
Local Assignment Operator
The local operator is used within functions to create a local variable – a variable that can only be accessed within the function where it was declared.
In the above example, MY_VAR is declared as a local variable in the my_func function. When we call the function, it prints “I am local” . However, when we try to echo MY_VAR outside of the function, it prints nothing because MY_VAR is not accessible outside my_func .
Bash assignment operators are a crucial part of shell scripting, enabling the storage and manipulation of data. By understanding and using these operators effectively, you can enhance the functionality and efficiency of your scripts. This article covered the basic assignment operator, compound assignment operators, and special assignment operators like readonly and local. Understanding how and when to use each operator is a key aspect of mastering Bash scripting.
Related Posts
What is the “/dev/null 2>&1” in bash: a comprehensive guide, using the ‘-ge’ operator in bash: a comprehensive guide.
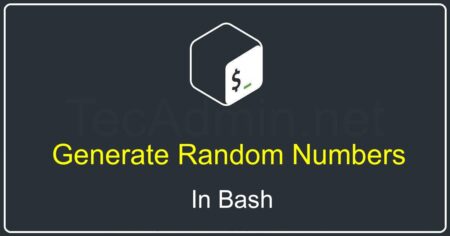
Generate Random Numbers in Bash
Save my name, email, and website in this browser for the next time I comment.
Type above and press Enter to search. Press Esc to cancel.
Learn Scripting
Coding Knowledge Unveiled: Empower Yourself
Mastering Operators in Shell Scripting: A Comprehensive Guide
Shell scripting is a powerful tool for automating tasks and performing various operations on Unix-like operating systems. Operators play a fundamental role in shell scripts, allowing you to manipulate data, perform calculations, and make decisions. In this blog, we’ll delve into the world of operators in shell scripting, exploring their types, usage, and real-world applications.
Operators in Shell Scripting
Operators in shell scripting are symbols or special keywords that perform operations on variables, constants, and values. They allow you to work with data, make comparisons, and control the flow of your scripts. Shell scripting supports several types of operators:
1. Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations in shell scripts. They include:
- Addition (+): Adds two values.
- Subtraction (-): Subtracts the second value from the first.
- Multiplication (*): Multiplies two values.
- Division (/): Divides the first value by the second.
- Modulus (%): Computes the remainder of the division.
- Exponentiation ( or ^):** Raises the first value to the power of the second (not available in all shells).
Here’s an example of using arithmetic operators in a shell script:
2. Comparison Operators
Comparison operators are used to compare values or expressions in shell scripts. They return a Boolean result (either true or false) based on the comparison. Common comparison operators include:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if the first value is greater than the second.
- Less than (<): Checks if the first value is less than the second.
- Greater than or equal to (>=): Checks if the first value is greater than or equal to the second.
- Less than or equal to (<=): Checks if the first value is less than or equal to the second.
Here’s an example of using comparison operators in a shell script:
3. Logical Operators
Logical operators are used to perform logical operations in shell scripts, especially within conditional statements. They include:
- AND (&&): Returns true if both operands are true.
- OR (||): Returns true if at least one operand is true.
- NOT (!): Inverts the Boolean value of the operand.
Here’s an example of using logical operators in a shell script:
4. Assignment Operators
Assignment operators are used to assign values to variables in shell scripts. The basic assignment operator is = . However, there are also compound assignment operators that combine an operation with assignment, such as += , -= , *= , and /= .
Here’s an example of using assignment operators in a shell script:
5. String Operators
String operators are used for string manipulation in shell scripts. They include:
- Concatenation (+=): Combines two strings.
- Equality (==): Checks if two strings are equal.
- Inequality (!=): Checks if two strings are not equal.
- Length (strlen): Returns the length of a string.
Here’s an example of using string operators in a shell script:
Real-World Applications
Operators are essential in shell scripting and have numerous real-world applications:
- Data Processing: Operators are used to manipulate data, perform calculations, and format output.
- Conditionals: Comparison and logical operators enable you to create conditional statements for decision-making in scripts.
- Looping: Operators play a crucial role in controlling loops, allowing you to set conditions for loop termination.
- String Manipulation: String operators help in tasks like concatenating, splitting, and checking strings.
- File Operations: Operators are used in shell scripts for file operations, such as checking file existence, permissions, and modification dates.
- Script Automation: Operators facilitate automation by enabling scripts to make decisions and perform actions based on conditions.
Operators are the building blocks of shell scripting, enabling you to perform a wide range of tasks efficiently and effectively. By understanding and mastering the various types of operators, you can write more powerful and flexible shell scripts to automate tasks, manipulate data, and make intelligent decisions. Whether you’re a system administrator, developer, or data analyst, a solid grasp of shell scripting operators is a valuable skill in the world of Unix-like operating systems.
Share this:
Related posts.
- Shell Scripting
Advanced Linux Commands to Make You Expert User
- April 1, 2019
Linux commands and their optional parameter are very useful for the user who wants to take advance of the full feature of Linux CLI. In […]
Elevating Your Skills: Advanced Shell Scripting Techniques
- October 1, 2023
Introduction Shell scripting is a versatile and powerful tool for automating tasks, managing system resources, and processing data in Unix-like environments. As you become more […]
Streamlining System Startup and Shutdown with Shell Scripts
Title: Streamlining System Startup and Shutdown with Shell Scripts Introduction The startup and shutdown processes are critical components of system administration. They define how a […]
Leave a Reply Cancel reply
You must be logged in to post a comment.
8.1. Operators
Initializing or changing the value of a variable
All-purpose assignment operator, which works for both arithmetic and string assignments.
arithmetic operators
multiplication
modulo, or mod (returns the remainder of an integer division operation)
This operator finds use in, among other things, generating numbers within a specific range (see Example 9-24 and Example 9-27 ) and formatting program output (see Example 26-15 and Example A-6 ). It can even be used to generate prime numbers, (see Example A-16 ). Modulo turns up surprisingly often in various numerical recipes.
Example 8-1. Greatest common divisor
"plus-equal" (increment variable by a constant)
let "var += 5" results in var being incremented by 5 .
"minus-equal" (decrement variable by a constant)
"times-equal" (multiply variable by a constant)
let "var *= 4" results in var being multiplied by 4 .
"slash-equal" (divide variable by a constant)
"mod-equal" (remainder of dividing variable by a constant)
Arithmetic operators often occur in an expr or let expression.
Example 8-2. Using Arithmetic Operations
As of version 2.05b, Bash supports 64-bit integers.
bitwise operators. The bitwise operators seldom make an appearance in shell scripts. Their chief use seems to be manipulating and testing values read from ports or sockets . "Bit flipping" is more relevant to compiled languages, such as C and C++, which run fast enough to permit its use on the fly.
bitwise operators
bitwise left shift (multiplies by 2 for each shift position)
"left-shift-equal"
let "var <<= 2" results in var left-shifted 2 bits (multiplied by 4 )
bitwise right shift (divides by 2 for each shift position)
"right-shift-equal" (inverse of <<= )
bitwise and
"bitwise and-equal"
"bitwise OR-equal"
bitwise negate
bitwise NOT
bitwise XOR
"bitwise XOR-equal"
logical operators
and (logical)
or (logical)
Example 8-3. Compound Condition Tests Using && and ||
The && and || operators also find use in an arithmetic context.
miscellaneous operators
comma operator
The comma operator chains together two or more arithmetic operations. All the operations are evaluated (with possible side effects ), but only the last operation is returned.
The comma operator finds use mainly in for loops . See Example 10-12 .

Next: Aliases , Previous: Bash Conditional Expressions , Up: Bash Features [ Contents ][ Index ]
6.5 Shell Arithmetic
The shell allows arithmetic expressions to be evaluated, as one of the shell expansions or by using the (( compound command, the let builtin, or the -i option to the declare builtin.
Evaluation is done in fixed-width integers with no check for overflow, though division by 0 is trapped and flagged as an error. The operators and their precedence, associativity, and values are the same as in the C language. The following list of operators is grouped into levels of equal-precedence operators. The levels are listed in order of decreasing precedence.
variable post-increment and post-decrement
variable pre-increment and pre-decrement
unary minus and plus
logical and bitwise negation
exponentiation
multiplication, division, remainder
addition, subtraction
left and right bitwise shifts
equality and inequality
bitwise AND
bitwise exclusive OR
logical AND
conditional operator
Shell variables are allowed as operands; parameter expansion is performed before the expression is evaluated. Within an expression, shell variables may also be referenced by name without using the parameter expansion syntax. A shell variable that is null or unset evaluates to 0 when referenced by name without using the parameter expansion syntax. The value of a variable is evaluated as an arithmetic expression when it is referenced, or when a variable which has been given the integer attribute using ‘ declare -i ’ is assigned a value. A null value evaluates to 0. A shell variable need not have its integer attribute turned on to be used in an expression.
Integer constants follow the C language definition, without suffixes or character constants. Constants with a leading 0 are interpreted as octal numbers. A leading ‘ 0x ’ or ‘ 0X ’ denotes hexadecimal. Otherwise, numbers take the form [ base # ] n , where the optional base is a decimal number between 2 and 64 representing the arithmetic base, and n is a number in that base. If base # is omitted, then base 10 is used. When specifying n , if a non-digit is required, the digits greater than 9 are represented by the lowercase letters, the uppercase letters, ‘ @ ’, and ‘ _ ’, in that order. If base is less than or equal to 36, lowercase and uppercase letters may be used interchangeably to represent numbers between 10 and 35.
Operators are evaluated in order of precedence. Sub-expressions in parentheses are evaluated first and may override the precedence rules above.
Bash Shell Scripting/Shell Arithmetic
Arithmetic expressions in Bash are closely modeled on those in C, so they are very similar to those in other C-derived languages, such as C++, Java, Perl, JavaScript, C#, and PHP. One major difference is that Bash only supports integer arithmetic (whole numbers), not floating-point arithmetic (decimals and fractions); something like 3 + 4 means what you'd expect (7), but something like 3.4 + 4.5 is a syntax error. Something like 13 / 5 is fine, but performs integer division, so evaluates to 2 rather than to 2.6.
- 1 Arithmetic expansion
- 2 expr (deprecated)
- 3 Numeric operators
- 4 Referring to variables
- 5 Assigning to variables
- 6 Arithmetic expressions as their own commands
- 7 The comma operator
- 8 Comparison, Boolean, and conditional operators
- 9 Arithmetic for-loops
- 10 Bitwise operators
- 11 Integer literals
- 12 Integer variables
- 13 Non-integer arithmetic
Arithmetic expansion [ edit | edit source ]
Perhaps the most common way to use arithmetic expressions is in arithmetic expansion , where the result of an arithmetic expression is used as an argument to a command. Arithmetic expansion is denoted $(( … )) . For example, this command:
prints 19 .
expr (deprecated) [ edit | edit source ]
Another way to use arithmetic expressions is using the Unix program "expr", which was popular before Bash supported math. [1] Similar to Arithmetic expansion, this command:
prints 19 . Note that using "expr" requires an escape character "\" before the multiplication operator "*" and parentheses. Further note the spaces between each operator symbol, including the parentheses.
Numeric operators [ edit | edit source ]
In addition to the familiar notations + (addition) and - (subtraction), arithmetic expressions also support * (multiplication), / (integer division, described above), % (modulo division, the "remainder" operation; for example, 11 divided by 5 is 2 remainder 1, so 11 % 5 is 1 ), and ** ("exponentiation", i.e. involution; for example, 2 4 = 16, so 2 ** 4 is 16 ).
The operators + and - , in addition to their "binary" (two-operand) senses of "addition" and "subtraction", have "unary" (one-operand) senses of "positive" and "negative". Unary + has basically no effect; unary - inverts the sign of its operand. For example, -(3*4) evaluates to -12 , and -(-(3*4)) evaluates to 12 .
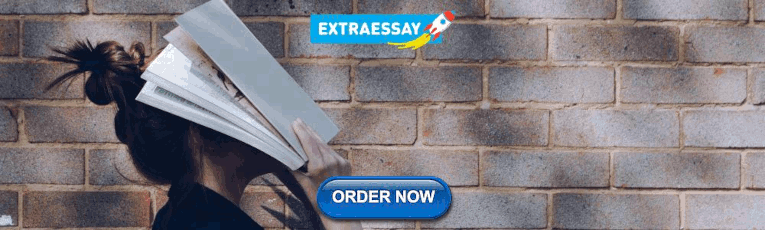
Referring to variables [ edit | edit source ]
Inside an arithmetic expression, shell variables can be referred to directly, without using variable expansion (that is, without the dollar sign $ ). For example, this:
prints 35 . (Note that i is evaluated first, producing 5, and then it's multiplied by 7. If we had written $i rather than i , mere string substitution would have been performed; 7 * 2+3 equals 14 + 3, that is, 17 — probably not what we want.)
The previous example shown using "expr":
prints 35 .
Assigning to variables [ edit | edit source ]
Shell variables can also be assigned to within an arithmetic expression. The notation for this is similar to that of regular variable assignment, but is much more flexible. For example, the previous example could be rewritten like this:
except that this sets $i to 5 rather than to 2+3 . Note that, although arithmetic expansion looks a bit like command substitution, it is not executed in a subshell; this command actually sets $i to 5 , and later commands can use the new value. (The parentheses inside the arithmetic expression are just the normal mathematical use of parentheses to control the order of operations.)
In addition to the simple assignment operator = , Bash also supports compound operators such as += , -= , *= , /= , and %= , which perform an operation followed by an assignment. For example, (( i *= 2 + 3 )) is equivalent to (( i = i * (2 + 3) )) . In each case, the expression as a whole evaluates to the new value of the variable; for example, if $i is 4 , then (( j = i *= 3 )) sets both $i and $j to 12 .
Lastly, Bash supports increment and decrement operators. The increment operator ++ increases a variable's value by 1; if it precedes the variable-name (as the "pre-increment" operator), then the expression evaluates to the variable's new value, and if it follows the variable-name (as the "post-increment" operator), then the expression evaluates to the variable's old value. For example, if $i is 4 , then (( j = ++i )) sets both $i and $j to 5 , while (( j = i++ )) sets $i to 5 and $j to 4 . The decrement operator -- is exactly the same, except that it decreases the variable's value by 1. Pre-decrement and post-decrement are completely analogous to pre-increment and post-increment.
Arithmetic expressions as their own commands [ edit | edit source ]
A command can consist entirely of an arithmetic expression, using either of the following syntaxes:
Either of these commands will set $i to 5 . Both styles of command return an exit status of zero ("successful" or "true") if the expression evaluates to a non-zero value, and an exit status of one ("failure" or "false") if the expression evaluates to zero. For example, this:
will print this:
The reason for this counterintuitive behavior is that in C, zero means "false" and non-zero values (especially one) mean "true". Bash maintains that legacy inside arithmetic expressions, then translates it into the usual Bash convention at the end.
The comma operator [ edit | edit source ]
Arithmetic expressions can contain multiple sub-expressions separated by commas , . The result of the last sub-expression becomes the overall value of the full expression. For example, this:
sets $i to 2 , sets $j to 4 , and prints 8 .
The let built-in actually supports multiple expressions directly without needing a comma; therefore, the following three commands are equivalent:
Comparison, Boolean, and conditional operators [ edit | edit source ]
Arithmetic expressions support the integer comparison operators < , > , <= (meaning ≤), >= (meaning ≥), == (meaning =), and != (meaning ≠). Each evaluates to 1 for "true" or 0 for "false".
They also support the Boolean operators && ("and"), which evaluates to 0 if either of its operands is zero, and to 1 otherwise; || ("or"), which evaluates to 1 if either of its operands is nonzero, and to 0 otherwise; and ! ("not"), which evaluates to 1 if its operand is zero, and to 0 otherwise. Aside from their use of zero to mean "false" and nonzero values to mean "true", these are just like the operators && , || , and ! that we've seen outside arithmetic expressions. Like those operators, these are "short-cutting" operators that do not evaluate their second argument if their first argument is enough to determine a result. For example, (( ( i = 0 ) && ( j = 2 ) )) will not evaluate the ( j = 2 ) part, and therefore will not set $j to 2 , because the left operand of && is zero ("false").
And they support the conditional operator b ? e1 : e2 . This operator evaluates e1 , and returns its result, if b is nonzero; otherwise, it evaluates e2 and returns its result.
These operators can be combined in complex ways:
Arithmetic for-loops [ edit | edit source ]
Above, we saw one style of for-loop, that looked like this:
Bash also supports another style, modeled on the for-loops of C and related languages, using shell arithmetic:
This for-loop uses three separate arithmetic expressions, separated by semicolons ; (and not commas , — these are completely separate expressions, not just sub-expressions). The first is an initialization expression, run before the loop begins. The second is a test expression; it is evaluated before every potential loop iteration (including the first), and if it evaluates to zero ("false"), then the loop exits. The third is a counting expression; it is evaluated at the end of each loop iteration. In other words, this for-loop is exactly equivalent to this while-loop:
but, once you get used to the syntax, it makes it more clear what is going on.
Bitwise operators [ edit | edit source ]
In addition to regular arithmetic and Boolean operators, Bash also offers "bitwise" operators, meaning operators that operate on integers qua bit-strings rather than qua integers. If you are not already familiar with this concept, you can safely ignore these.
Just as in C, the bitwise operators are & (bitwise "and"), | (bitwise "or"), ^ (bitwise "exclusive or"), ~ (bitwise "not"), << (bitwise left-shift), and >> (bitwise right-shift), as well as &= and |= and ^= (which include assignment, just like += ).
Integer literals [ edit | edit source ]
An integer constant is expressed as an integer literal . We have already seen many of these; 34 , for example, is an integer literal denoting the number 34. All of our examples have been decimal (base ten) integer literals, which is the default; but in fact, literals may be expressed in any base in the range 2–64, using the notation base # value (with the base itself being expressed in base-ten). For example, this:
will print 12 six times. (Note that this notation only affects how an integer literal is interpreted. The result of the arithmetic expansion is still expressed in base ten, regardless.)
For bases 11 through 36, the English letters A through Z are used for digit-values 10 through 35. This is not case-sensitive. For bases 37 through 64, however, it is specifically the lowercase English letters that are used for digit-values 10 through 35, with the uppercase letters being used for digit-values 36 through 61, the at-sign @ being used for digit-value 62, and the underscore _ being used for digit-value 63. For example, 64#@A3 denotes 256259 ( 62 × 64 2 + 36 × 64 + 3 ).
There are also two special notations: prefixing a literal with 0 indicates base-eight (octal), and prefixing it with 0x or 0X indicates base-sixteen (hexadecimal). For example, 030 is equivalent to 8#30 , and 0x6F is equivalent to 16#6F .
Integer variables [ edit | edit source ]
A variable may be declared as an integer variable — that is, its "integer attribute" may be "set" — by using this syntax:
After running the above command, any subsequent assignments to n will automatically cause the right-hand side to be interpreted as an arithmetic expression. For example, this:
is more or less equivalent to this:
except that the first version's declare -i n will continue to affect later assignments as well.
In the first version, note the use of quotes around the right-hand side of the assignment. Had we written n=2 + 3 > 4 , it would have meant "run the command + with the argument 3 , passing in the environment variable n set to 2 , and redirecting standard output into the file 4 "; which is to say, setting a variable's integer attribute doesn't affect the overall parsing of assignment statements, but merely controls the interpretation of the value that is finally assigned to the variable.
We can "unset" a variable's integer attribute, turning off this behavior, by using the opposite command:
The declare built-in command has a number of other uses as well: there are a few other attributes a variable can have, and declare has a few other features besides turning attributes on and off. In addition, a few of its properties bear note:
- As with local and export , the argument can be a variable assignment; for example, declare -i n = 2 +3 sets $n 's integer attribute and sets it to 5 .
- As with local and export , multiple variables (and/or assignments) can be specified at once; for example, declare -i m n sets both $m 's integer attribute and $n 's.
- When used inside a function, declare implicitly localizes the variable (unless the variable is already local), which also has the effect of locally unsetting it (unless the assignment syntax is used).
Non-integer arithmetic [ edit | edit source ]
As mentioned above, Bash shell arithmetic only supports integer arithmetic. However, external programs can often be used to obtain similar functionality for non-integer values. In particular, the common Unix utility bc is often used for this. The following command:
prints 5.6 . Needless to say, since bc is not so tightly integrated with Bash as shell arithmetic is, it is not as convenient; for example, something like this:
would, to support non-integers, become something like this:
Part of this is because we can no longer use an arithmetic for-loop; part of it is because referring to variables and assigning to variables is trickier now (since bc is not aware of the shell's variables, only its own, unrelated ones); and part of it is because bc communicates with the shell only via input and output.
- ↑ http://www.sal.ksu.edu/faculty/tim/unix_sg/bash/math.html
- Book:Bash Shell Scripting
- Pages using deprecated enclose attributes
Navigation menu
- Shell Scripting
- Docker in Linux
- Kubernetes in Linux
- Linux interview question
- String Operators | Shell Script
- Shell Script to Export ML Model to Stakeholders
- Average of given numbers in Bash
- Shell Script Examples
- Shell script to print table of a given number
- How to find and replace the content from the file or string using PowerShell
- Shell script to convert binary to decimal
- Bash program to find A to the power B
- Keyboard Shortcuts for Ubuntu | Set - 1
- Wikit in Linux
- Simple Calculator in Bash
- Array Basics in Shell Scripting | Set 1
- How to find the file properties using powershell
- Few Tips for Fast & Productive Work on a Linux Terminal
- Bash Script to Check whether character entered is Upper, Lower, Digit, Consonant, or Vowel
- Shell Script to print odd and even numbers
- Disk Cleanup Using Powershell Scripts
- Shell script to display Good Morning, Good Afternoon Good Evening according to system time
- Shell Script To Show All the Internal and External Links From a URL
Basic Operators in Shell Scripting
There are 5 basic operators in bash/shell scripting:
- Arithmetic Operators
- Relational Operators
- Boolean Operators
- Bitwise Operators
- File Test Operators
1. Arithmetic Operators : These operators are used to perform normal arithmetics/mathematical operations. There are 7 arithmetic operators:
- Addition (+) : Binary operation used to add two operands.
- Subtraction (-) : Binary operation used to subtract two operands.
- Multiplication (*) : Binary operation used to multiply two operands.
- Division (/) : Binary operation used to divide two operands.
- Modulus (%) : Binary operation used to find remainder of two operands.
- Increment Operator (++) : Unary operator used to increase the value of operand by one.
- Decrement Operator (- -) : Unary operator used to decrease the value of a operand by one
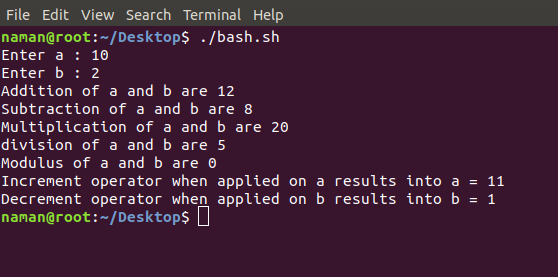
2. Relational Operators : Relational operators are those operators which define the relation between two operands. They give either true or false depending upon the relation. They are of 6 types:
- ‘==’ Operator : Double equal to operator compares the two operands. Its returns true is they are equal otherwise returns false.
- ‘!=’ Operator : Not Equal to operator return true if the two operands are not equal otherwise it returns false.
- ‘<‘ Operator : Less than operator returns true if first operand is less than second operand otherwise returns false.
- ‘<=’ Operator : Less than or equal to operator returns true if first operand is less than or equal to second operand otherwise returns false
- ‘>’ Operator : Greater than operator return true if the first operand is greater than the second operand otherwise return false.
- ‘>=’ Operator : Greater than or equal to operator returns true if first operand is greater than or equal to second operand otherwise returns false
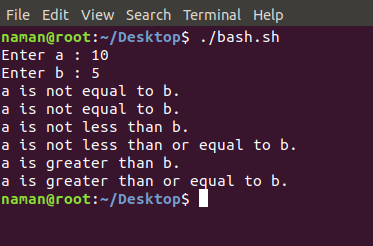
3. Logical Operators : They are also known as boolean operators. These are used to perform logical operations. They are of 3 types:
- Logical AND (&&) : This is a binary operator, which returns true if both the operands are true otherwise returns false.
- Logical OR (||) : This is a binary operator, which returns true is either of the operand is true or both the operands are true and return false if none of then is false.
- Not Equal to (!) : This is a unary operator which returns true if the operand is false and returns false if the operand is true.
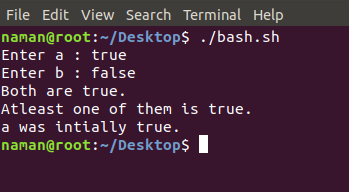
4. Bitwise Operators : A bitwise operator is an operator used to perform bitwise operations on bit patterns. They are of 6 types:
- Bitwise And (&) : Bitwise & operator performs binary AND operation bit by bit on the operands.
- Bitwise OR (|) : Bitwise | operator performs binary OR operation bit by bit on the operands.
- Bitwise XOR (^) : Bitwise ^ operator performs binary XOR operation bit by bit on the operands.
- Bitwise complement (~) : Bitwise ~ operator performs binary NOT operation bit by bit on the operand.
- Left Shift (<<) : This operator shifts the bits of the left operand to left by number of times specified by right operand.
- Right Shift (>>) : This operator shifts the bits of the left operand to right by number of times specified by right operand.
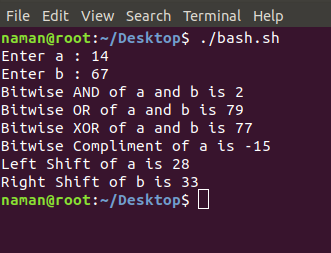
5. File Test Operator : These operators are used to test a particular property of a file.
- -b operator : This operator check whether a file is a block special file or not. It returns true if the file is a block special file otherwise false.
- -c operator : This operator checks whether a file is a character special file or not. It returns true if it is a character special file otherwise false.
- -d operator : This operator checks if the given directory exists or not. If it exists then operators returns true otherwise false.
- -e operator : This operator checks whether the given file exists or not. If it exits this operator returns true otherwise false.
- -r operator : This operator checks whether the given file has read access or not. If it has read access then it returns true otherwise false.
- -w operator : This operator check whether the given file has write access or not. If it has write then it returns true otherwise false.
- -x operator : This operator check whether the given file has execute access or not. If it has execute access then it returns true otherwise false.
- -s operator : This operator checks the size of the given file. If the size of given file is greater than 0 then it returns true otherwise it is false.
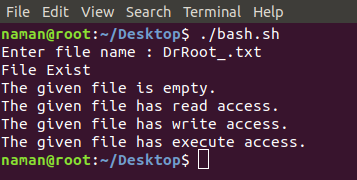
Please Login to comment...
Similar reads.
- Shell Script
- Technical Scripter
- 10 Best Todoist Alternatives in 2024 (Free)
- How to Get Spotify Premium Free Forever on iOS/Android
- Yahoo Acquires Instagram Co-Founders' AI News Platform Artifact
- OpenAI Introduces DALL-E Editor Interface
- Top 10 R Project Ideas for Beginners in 2024
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Home > Bash Scripting Tutorial > Bash Operator > Arithmetic Operators in Bash
Arithmetic Operators in Bash

Arithmetic operators are operators that facilitate the evaluation of a desired mathematical expression. Bash shell supports a lot of arithmetic operators. One can perform simple addition, and subtraction and evaluate complex arithmetic expressions using these operators. In this article, I will talk about different arithmetic operators with their execution in Bash.
Practice Files for Arithmetic Operators in Bash
Different Arithmetic Operators in Bash
Bash offers various operators to perform arithmetic operations. These operators have different levels of precedence.
For example, the expression $((3*5+6)/7-1)) is evaluated in accordance with the rules of precedence. (3*5+6) is computed first as it is enclosed by parenthesis. Within the parentheses, the multiplication operation 3*5 is computed first, resulting in 15. Then, the addition operation 15 + 6 is performed, yielding 21. Subsequently, division 21 / 7 is evaluated before subtraction, resulting in 3. Finally, the subtraction 3 - 1 is computed, yielding the final result of 2. Below is a list of operators sorted by increasing order of precedence:
Now, I am going to discuss each of the arithmetic operators in detail:
The plus sign ( + ) is used for addition operation in Bash. For example,

The minus sign ( – ) is used to perform subtraction. Such as,

The asterisk sign ( * ) is used to multiply two numbers in Bash. For instance,

Forward slash ( / ) is used to divide one number by another:

One can calculate the modulus or remainder using the percentage sign ( % ). For example,

Exponential
To calculate the exponential of a number raised to another number, use the double asterisk sign ( ** ):

Assignment Operators for Arithmetic Calculation in Bash
Apart from arithmetic operators, there are a few assignment operators in Bash. These operators are used to assign values to variables. They take a value on the right side of the operator and change the variable on the left side based on the given value and the operator. These are also useful to perform arithmetic operations in some cases.
All the assignment operators have equal levels of precedence. The following list contains assignment operators and their functions on numerical values:
Let’s discuss each of these assignment operators in short:
Increment by Constant (+=)
To increase a variable by a constant use the increment by constant += operator. For instance,
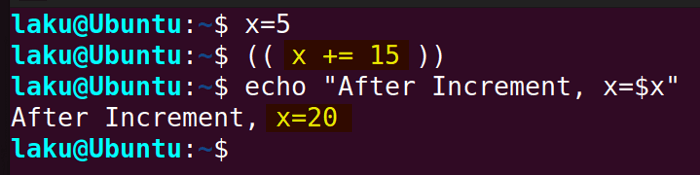
Decrement by Constant (-=)
To decrease a variable by a constant use the -= operator:
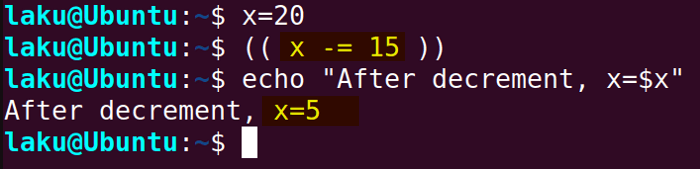
Multiply by Constant (*=)
To multiply a variable with a constant use the *= operator:
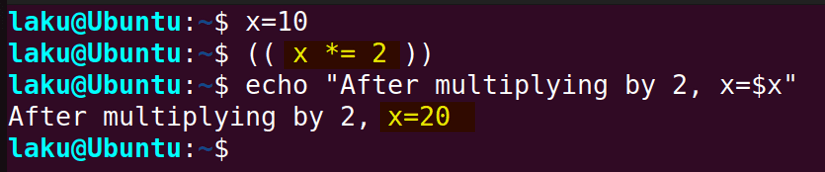
Divide by Constant (/=)
/= replaces the variable with the result of division after dividing it by a constant:
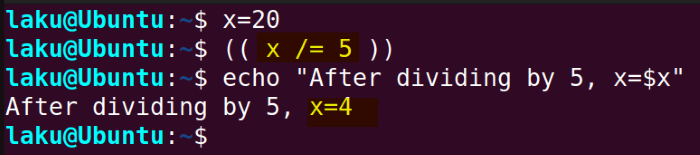
Remainder After Dividing by Constant (%=)
%= operator replaces the variable with the remainder after dividing it by a constant. For example,
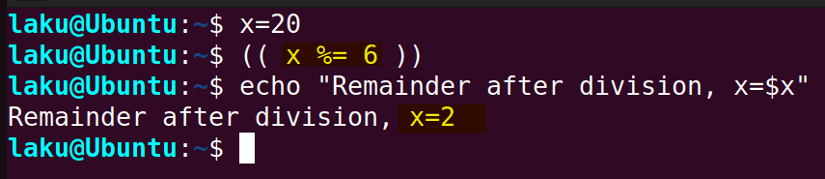
Practical Application of Arithmetic Operators in Bash
Bash arithmetic operators have various real-life usages. In this tutorial, I will show two basic examples involving the calculation with multiple operators. These will help you to understand how arithmetic operators can be used in practical cases:
Calculate the Perimeter of a Rectangular
To calculate the perimeter of a rectangle, one can use the addition and multiplication operators simultaneously.
The program adds the length and width using the plus + arithmetic operator. Then the summation is multiplied by 2 using the multiplication operator * to get the perimeter of the rectangle.
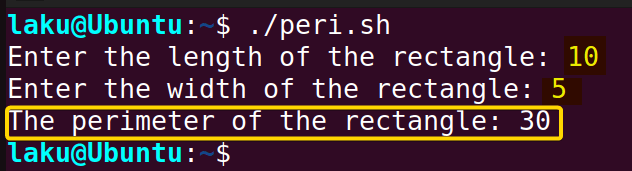
Here the length and width of the rectangle are 10 and 5 respectively. The program calculates the perimeter of the rectangle 30 using the addition and multiplication operator.
Check Odd or Even Number
Divide a number by 2 and compare the remainder with zero to determine whether a number is odd or even. To check it in Bash, the modulo ( % ) operator is quite useful.
The % operator is used to find the remainder when the variable number is divided by 2 .
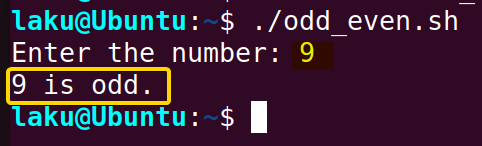
The number provided by the user is 9 . The program determines it as an odd number by successfully using the modulo ( % ) operator in Bash.
In conclusion, arithmetic operators are for performing mathematical calculations. Bash users should familiarize themselves with arithmetic operators to become more efficient scriptwriters.
People Also Ask
What are the ways to perform arithmetic calculations in bash.
There are multiple ways to perform arithmetic calculations in Bash.
- Arithmetic expansion: $(( )) allows users to evaluate arithmetic expressions like the
- expr command: The expr command evaluates expressions and performs arithmetic
operations.
- let command: The let command is also useful for evaluating a mathematical
expression in Bash. To be specific let '5 + 3' perform the addition of 5 and 3 and result in 8 .
How to evaluate arithmetic expressions in the if statement?
To evaluate arithmetic expression within an if statement one can use double parenthesis . For instance, ((13 % 2 == 0)) calculates the remainder using the modulo ( % ) operator when 13 is divided by 2 and compares it with zero.
How to perform floating point calculations in Bash?
To perform floating point calculations pass the expression to the bc command . For instance, echo 2.2*3.5 | bc performs the multiplication of two floating point numbers 2.2 and 3.5 .
How to fix “invalid arithmetic operator” in bash?
In Bash,‘invalid arithmetic operator’ error occurs due to many reasons. To fix the error first identify the reason behind it. In most cases, it occurs due to the intention of calculating floating point using arithmetic expansion. Arithmetic expansion can’t handle floating point arithmetics. For instance, echo $((2.5+5)) will show ‘invalid arithmetic operator’ because of the floating point number 2.5 . Use the bc command to perform this type of calculation.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Sum Up Numbers in Bash [Explained With Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
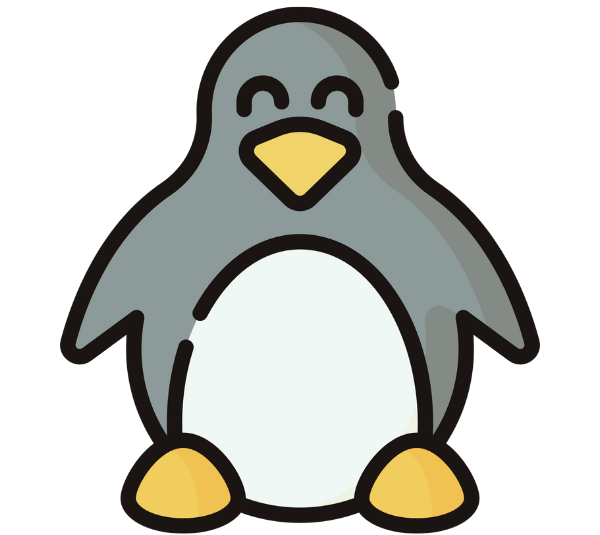
Md Zahidul Islam Laku
Hey, I'm Zahidul Islam Laku currently working as a Linux Content Developer Executive at SOFTEKO. I completed my graduation from Bangladesh University of Engineering and Technology (BUET). I write articles on a variety of tech topics including Linux. Learning and writing on Linux is nothing but fun as it gives me more power on my machine. What can be more efficient than interacting with the Operating System without Graphical User Interface! Read Full Bio
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
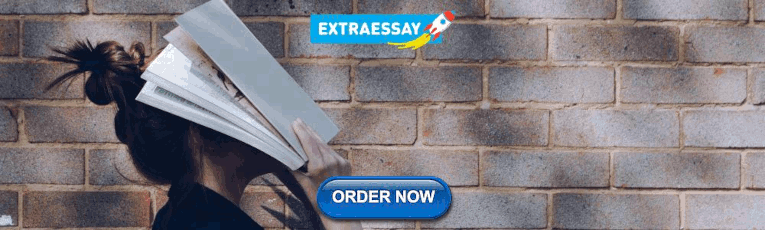
IMAGES
VIDEO
COMMENTS
If b is a variable and you want to expand it with a default value to be used when the variable is unset, then use ${b-c}. If you also want the default value to be used when the variable is set but empty, then use ${b:-c}. The c in these examples is a string, and like all other strings in the shell it can be built with expansions: i=3.
In the Bash shell, assignment operators are used to assign values to variables. They are essential tools in scripting and programming, providing a method to store and manipulate data. This article will take you through the fundamental assignment operators in Bash, along with examples of their usage. Standard Assignment Operator In Bash, the standard assignment
Using operators in a Bash script is how you determine whether a condition is true or not. And testing for conditions is how we program a Bash script to be dynamic by giving it the ability to respond differently depending on a scenario. Creating conditionals is why it is essential to know the various Bash operators. Operators let us test things like arithmetic functions, compare strings, check ...
4. Assignment Operators. Assignment operators are used to assign values to variables in shell scripts. The basic assignment operator is =. However, there are also compound assignment operators that combine an operation with assignment, such as +=, -=, *=, and /=. Here's an example of using assignment operators in a shell script:
3.1 Shell Syntax. When the shell reads input, it proceeds through a sequence of operations. If the input indicates the beginning of a comment, the shell ignores the comment symbol ('#'), and the rest of that line. Otherwise, roughly speaking, the shell reads its input and divides the input into words and operators, employing the quoting rules to select which meanings to assign various ...
bitwise operators. The bitwise operators seldom make an appearance in shell scripts. Their chief use seems to be manipulating and testing values read from ports or sockets. "Bit flipping" is more relevant to compiled languages, such as C and C++, which run fast enough to permit its use on the fly.
This technique allows for a variable to be assigned a value if another variable is either empty or is undefined. NOTE: This "other variable" can be the same or another variable. excerpt. ${parameter:-word} If parameter is unset or null, the expansion of word is substituted. Otherwise, the value of parameter is substituted.
4.2. Variable Assignment. the assignment operator ( no space before and after) Do not confuse this with = and -eq, which test , rather than assign! Note that = can be either an assignment or a test operator, depending on context. Example 4-2. Plain Variable Assignment. #!/bin/bash. # Naked variables.
The importance of this type-checking lies in the operator's most common use—in conditional assignment statements. In this usage it appears as an expression on the right side of an assignment statement, as follows:
Bash: let Statement vs. Assignment. 1. Overview. The built-in let command is part of the Bash and Korn shell fundamental software. Its main usage focuses on building variable assignments with basic arithmetic operations. In this article, we describe the typical usage syntax of the command. Thereupon, we show all meaningful properties that ...
conditional operator = *= /= %= += -= <<= >>= &= ^= |= assignment expr1 , expr2. comma Shell variables are allowed as operands; parameter expansion is performed before the expression is evaluated. Within an expression, shell variables may also be referenced by name without using the parameter expansion syntax.
In addition to the simple assignment operator =, Bash also supports compound operators such as +=, -=, *=, /=, and %=, which perform an operation followed by an assignment. ... As mentioned above, Bash shell arithmetic only supports integer arithmetic. However, external programs can often be used to obtain similar functionality for non-integer ...
This Bash script reads two user inputs, a and b.It then performs various arithmetic operations on these numbers: addition, subtraction, multiplication, division, and modulus.Then the echo command displays the results of these operations. Additionally, the script demonstrates the use of the increment operator (++a), which increases the value of a by 1, and the decrement operator (-b), which ...
Output: 3. Logical Operators: They are also known as boolean operators.These are used to perform logical operations. They are of 3 types: Logical AND (&&): This is a binary operator, which returns true if both the operands are true otherwise returns false. Logical OR (||): This is a binary operator, which returns true is either of the operand is true or both the operands are true and return ...
bash integer variables. In addition to, dutCh, Vladimir and ghostdog74's corrects answers and because this question is regarding integer and tagged bash: Is there a way to do something like this. int a = (b == 5) ? c : d; There is a nice and proper way to work with integers under bash:
var=23 assigns 23 to the variable var.. var =23 tries to run command (or alias, or function) var with argument =23. var = 23 ditto, but arguments = and 23. var= 23 sets var environment variable to blank string, then runs command 23. Yes, shell is weird as a programming language. But it makes perfect sense as a shell for interactive use, where spaces separate commands and arguments.
Apart from arithmetic operators, there are a few assignment operators in Bash. These operators are used to assign values to variables. They take a value on the right side of the operator and change the variable on the left side based on the given value and the operator. These are also useful to perform arithmetic operations in some cases. All ...
3. There are two types of vars: Environment (exported) vars, and shell vars. VAR=val sets the env var named VAR if it already exists. VAR=val sets the shell var named VAR if env var VAR doesn't exist. VAR=val cmd sets the env var named VAR for cmd only. The env vars are provided to child processes as their environment.
$ a=1; b=2; c=3 $ echo $(( max = a > b ? ( a > c ? a : c) : (b > c ? b : c) )) 3. In this case, the second and third operands or the main ternary expression are themselves ternary expressions.If a is greater than b, then a is compared to c and the larger of the two is returned. Otherwise, b is compared to c and the larger is returned. Numerically, since the first operand evaluates to zero ...
Ternary operator (?:) in Bash. If this were AS3 or Java, I would do the following: fileName = dirName + "/" + (useDefault ? defaultName : customName) + ".txt"; But in shell, that seems needlessly complicated, requiring several lines of code, as well as quite a bit of repeated code.
3. In Bash (and many other programming languages developed for or on the UNIX environment), the != operator means 'is not equal to'. In mathematical notation you would write ≠, but that character did not exist in ASCII, so most programming languages adopted an alternative. (APL just adopted its own character set instead, so it could go right ...