- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.
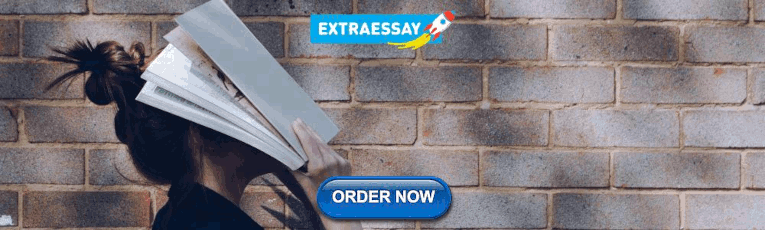
Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

Assignment Statement
The assignment statement is an instruction that stores a value in a variable . You use this instruction any time you want to update the value of a variable.
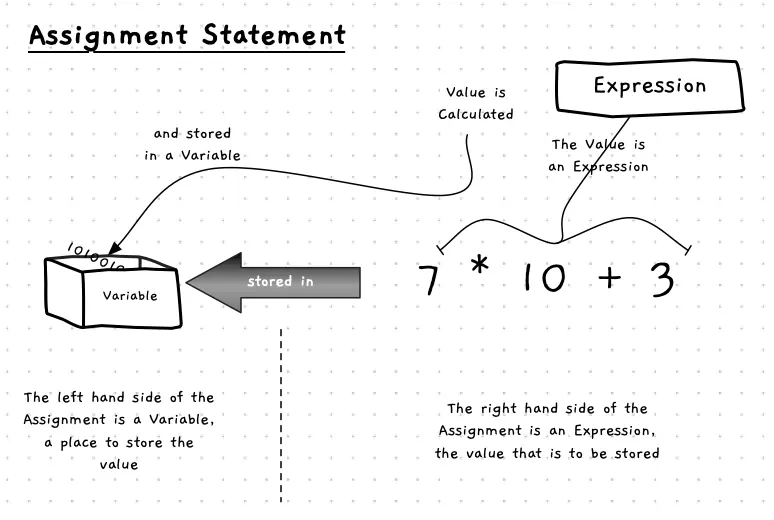
The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ). Once it has the value, it stores the value (assigns it) to the variable on the left-hand side of the assignment operator.
Assignment Statement — when, why, and how
When you create a variable, you have identified a piece of information that you want to be able to change as your program runs. Whenever you need to give a variable an initial or new value, you use an assignment statement .
The assignment statement uses the assignment operator = . Whatever is on the right-hand side of = represents the value to be assigned. This could be a literal , a method call , or any other expression . On the left-hand side you write the identifier of the variable you want to store this value in.
For example, you might decide to ask the user for their name. First, you need a variable to store the value. You might decide to call this variable name . Then, the assignment statement lets you read a response from the user and store it in that variable. In this case, the right-hand side of the assignment would be a call to ReadLine , which reads input from standard in and returns it to you. The left-hand side would be the identifier of our variable, name .
It is important to remember that every assignment statement has 2 actions :
- Calculate the value on the right-hand side
- Store it in the variable on the left-hand side.
The ordering of these actions allow you to update the value of a variable using an expression involving the variable being updated. This can be very useful. For example, you might want to update the value of a variable storing the number of steps you have taken today.
In C# the assignment operator is = . Most assignment statements are written using = , with an identifier on the left-hand side and an expression on the right-hand side. The assignment operator can optionally be modified with + , - , * , or / , which are shorthands for adding to, subtracting from, multiplying, and dividing the variable identified on the left-hand side of the statement.
Some assignment statements are written without = . These are assignment statements using increment ( ++ ) or decrement ( -- ), which allow you to add or remove one from a variable’s current value.
For example, x = x - 1 , x -= 1 , and x-- are all assignment statements which do the same thing — assign the variable x a new value that is one lower than its current value.
Basic assignment statement
In this example we use ReadLine to get input from the user and store it in a name variable.
Shorthand assignment statements
The following code shows an example of how to use some of the shorthand assignment statements.
If you ran the above code and entered 17 as the start count you should get this output:
You do not always need to store values in variables. Sometimes you can just use the value and then forget it. For example, in the above code, we read the initial count from the user. This requires us to read it as text, and then convert that text to a number. Given that we do not ever use the details in line again, we do not need to create this variable in the first place. Instead, we could pass the value to the convert function directly as shown below.
Assignment statement up close
The following sliders show how the assignment statement works in detail. These are both relatively simple programs, but notice how much is going on behind the scenes!
Assigning an int division result to an int variable
Assigning an int division result to a double variable.
Assignment Statements
Assignment statements in a program come in two forms – with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. Assignments with mutation are variable assignments that use the old value of a variable to calculate a value for the variable.
For example, an increment statement like x = x + 1 MUTATES the value of x by updating its value to be one bigger than it was before. In order to make sense of such a statement, we need to know the previous value of x .
In contrast, a statement like y = x + 1 assigns to y one more than the value in x . We do not need to know the previous value of y , as we are not using it in the assignment statement. (We do need to know the value of x ).
Assignments without mutation
We have already seen the steps necessary to process assignment statements that do not involve variable mutation. Recall that we can declare as a premise any assignment statement or claim from a previous logic block involving variables that have not since changed.
For example, suppose we want to verify the following program so the assert statement at the end will hold:
Since none of the statements involve variable mutation, we can do the verification in a single logic block:
Note that we did need to do ∧i so that the last claim was y == z ∧ y == 6 , even though we had previously established the claims y == z and y == 6 . In order for an assert to hold (at least until we switch Logika modes in chapter 10), we need to have established EXACTLY the claim in the assert in a previous logic block.
Assignments with mutation
Assignments with mutation are trickier – we need to know the old value of a variable in order to reason about its new value. For example, if we have the following program:
Then we might try to add the following logic blocks:
…but then we get stuck in the second logic block. There, x is supposed to refer to the CURRENT value of x (after being incremented), but both our attempted claims are untrue. The current value of x is not one more than itself (this makes no sense!), and we can tell from reading the code that x is now 5, not 4.
To help reason about changing variables, Logika has a special name_old value that refers to the OLD value of a variable called name , just before the latest update. In the example above, we can use x_old in the second logic block to refer to x ’s value just before it was incremented. We can now change our premises and finish the verification as follows:
By the end of the logic block following a variable mutation, we need to express everything we know about the variable’s current value WITHOUT using the _old terminology, as its scope will end when the logic block ends. Moreover, we only ever have one _old value available in a logic block – the variable that was most recently changed. This means we will need logic blocks after each variable mutation to process the changes to any related facts.
Variable swap example
Suppose we have the following Logika program:
We can see that this program gets two user input values, x and y , and then swaps their values. So if x was originally 4 and y was originally 6, then at the end of the program x would be 6 and y would be 4.
We would like to be able to assert what we did – that x now has the original value from y , and that y now has the original value from x . To do this, we might invent dummy constants called xOrig and yOrig that represent the original values of those variables. Then we can add our assert:
We can complete the verification by adding logic blocks after assignment statements, being careful to update all we know (without using the _old value) by the end of each block:
Notice that in each logic block, we express as much as we can about all variables/values in the program. In the first logic block, even though xOrig and yOrig were not used in the previous assignment statement, we still expressed how the current values our other variables compared to xOrig and yOrig . It helps to think about what you are trying to claim in the final assert – since our assert involved xOrig and yOrig , we needed to relate the current values of our variables to those values as we progressed through the program.
Last modified by: Julie Thornton Nov 15, 2023
CS101: Introduction to Computer Science I
Variables and Assignment Statements
Read this chapter, which covers variables and arithmetic operations and order precedence in Java.
9. Assignment Statements
No. The incorrect splittings are highlighted in red:
Assignment Statement
So far, the example programs have been using the value initially put into a variable. Programs can change the value in a variable. An assignment statement changes the value that is held in a variable. The program uses an assignment statement.
The assignment statement puts the value 123 into the variable. In other words, while the program is executing there will be a 64 bit section of memory that holds the value 123.
Remember that the word "execute" is often used to mean "run". You speak of "executing a program" or "executing" a line of the program.
Question 10:
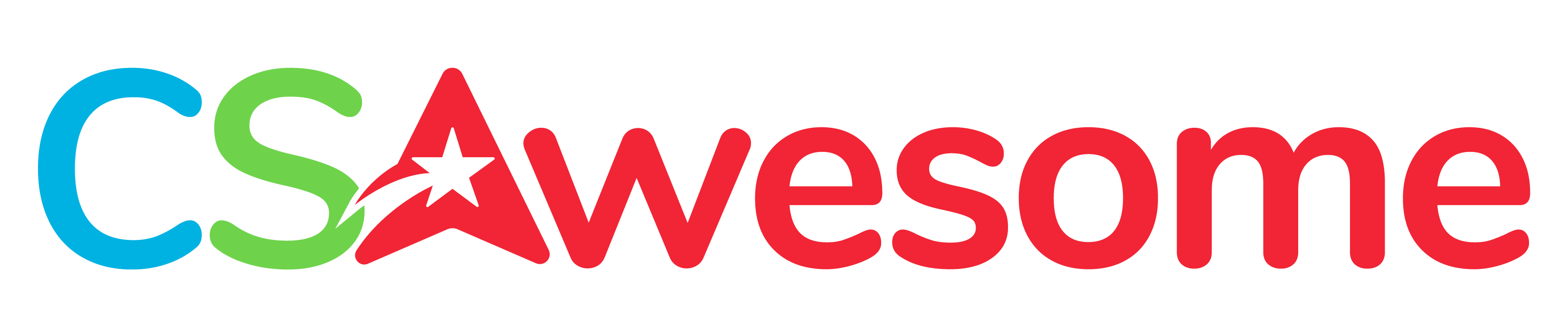
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Getting Started
- 1.1.1 Preface
- 1.1.2 About the AP CSA Exam
- 1.1.3 Transitioning from AP CSP to AP CSA
- 1.1.4 Java Development Environments
- 1.1.5 Growth Mindset and Pair Programming
- 1.1.6 Pretest for the AP CSA Exam
- 1.1.7 Survey
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Values
- 1.7 Unit 1 Summary
- 1.8 Mixed Up Code Practice
- 1.9 Toggle Mixed Up or Write Code Practice
- 1.10 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
Time estimate: 90 min.
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side. The value of the expression (which can contain math operators and other variables) on the right of the = sign is stored in the variable on the left.
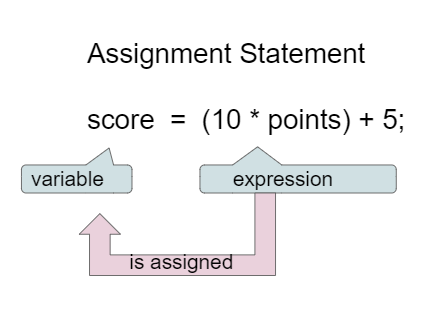
Figure 1: Assignment Statement (variable = expression;) ¶
Instead of saying equals for the = in an assignment statement, say “gets” or “is assigned” to remember that the variable gets or is assigned the value on the right. In the figure above score is assigned the value of the expression 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable’s value to a copy of the value of another variable like y = x; . This won’t change the value of the variable that you are copying from.
Let’s step through the following code in the Java visualizer to see the values in memory. Click on the Next button at the bottom of the code to see how the values of the variables change. You can run the visualizer on any Active Code in this e-book by just clicking on the Code Lens button at the top of each Active Code.
Activity: CodeLens 1.4.1.2 (asgn_viz1)

1-4-3: What are the values of x, y, and z after the following code executes? You can step through this code by clicking on this Java visualizer link.
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 0, y = 0, z = 3
The following has the correct code to ‘swap’ the values in x and y (so that x ends up with y’s initial value and y ends up with x’s initial value), but the code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right. Check your solution by clicking on the Check button. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks. After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
1.4.2. Adding 1 to a Variable ¶
If you use a variable to keep score, you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one ( score = score + 1 ) as shown below. The formula would look strange in math class, but it makes sense in coding because it is assigning a new value to the variable on the left that comes from evaluating the arithmetic expression on the right. So, the score variable is set to the previous value of score plus 1.
Try the code below to see how score is incremented by 1. Try substituting 2 instead of 1 to see what happens.
1.4.3. Input with Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables. The code below ( Java Scanner Input Repl using the Scanner class or Java Console Input Repl using the Console class) will say hello to anyone who types in their name for different name values. Click on run and then type in your name. Then, try run again and type in a friend’s name. The code works for any name: behold, the power of variables!
Although you will not be tested in the AP CSA exam on using the Java input or the Scanner or Console classes, learning how to do input in Java is very useful and fun. For more information on using the Scanner class, go to https://www.w3schools.com/java/java_user_input.asp , and for the newer Console class, https://howtodoinjava.com/java-examples/console-input-output/ .
1.4.4. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), and division ( / ). The multiplication operator is written as * , as it is in most programming languages, since the character sets used until relatively recently didn’t have a character for a real multiplication sign, × , and keyboards still don’t have a key for it. Likewise no ÷ .
You may be used to using ^ for exponentiation, either from a graphing calculator or tools like Desmos. Confusingly ^ is an operator in Java, but it has a completely different meaning than exponentiation and isn’t even exactly an arithmetic operator. You will learn how to use the Math.pow method to do exponents in Unit 2.
Arithmetic expressions can be of type int or double . An arithmetic expression consisting only of int values will evaluate to an int value. An arithmetic expression that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to combine String and other values into new String s. More on this when we talk about String s more fully in Unit 2.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == . They mean very different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Also note that using == and != with double values can produce surprising results. Because double values are only an approximation of the real numbers even things that should be mathematically equivalent might not be represented by the exactly same double value and thus will not be == . To see this for yourself, write a line of code below to print the value of the expression 0.3 == 0.1 + 0.2 ; it will be false !

Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3? Isn’t it surprising that it prints 0? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer. This is called truncating division . If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, just like when we do math (remember PEMDAS?), so that * , / , and % are done before + and - . However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first or just to make it more clear.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence . How do the parentheses change the precedence?
1.4.5. The Remainder Operator ¶
The operator % in Java is the remainder operator. Like the other arithmetic operators is takes two operands. Mathematically it returns the remainder after dividing the first number by the second, using truncating integer division. For instance, 5 % 2 evaluates to 1 since 2 goes into 5 two times with a remainder of 1.
While you may not have heard of remainder as an operator, think back to elementary school math. Remember when you first learned long division, before they taught you about decimals, how when you did a long division that didn’t divide evenly, you gave the answer as the number of even divisions and the remainder. That remainder is what is returned by this operator. In the figures below, the remainders are the same values that would be returned by 2 % 3 and 5 % 2 .
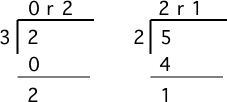
Figure 1: Long division showing the integer result and the remainder ¶
Sometimes people—including Professor Lewis in the next video—will call % the modulo , or mod , operator. That is not actually correct though the difference between remainder and modulo, which uses Euclidean division instead of truncating integer division, only matters when negative operands are involved and the signs of the operands differ. With positive operands, remainder and mod give the same results. Java does have a method Math.floorMod in the Math class if you need to use modulo instead of remainder, but % is all you need in the AP exam.
Here’s the video .
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x. The value y can’t go into x at all (goes in 0 times), since x is smaller than y, so the result is just x. So if you see 2 % 3 the result is 2.
1-4-10: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. % gives you the remainder.
- % gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-11: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.6. Programming Challenge : Dog Years ¶
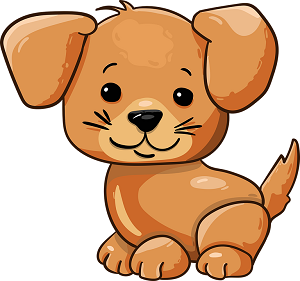
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out. If you are pair programming, switch drivers (who has control of the keyboard in pair programming) after every line of code.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years.
Your teacher may suggest that you use a Java IDE like repl.it for this challenge so that you can use input to get these values using the Scanner class . Here is a repl template that you can use to get started if you want to try the challenge with input.
1.4.7. Summary ¶
Arithmetic expressions include expressions of type int and double .
The arithmetic operators consist of + , - , * , / , and % also known as addition, subtraction, multiplication, division, and remainder.
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. ( * , / , % have precedence over + and - , unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException .
The assignment operator ( = ) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the types of the values and operators used in the expression.
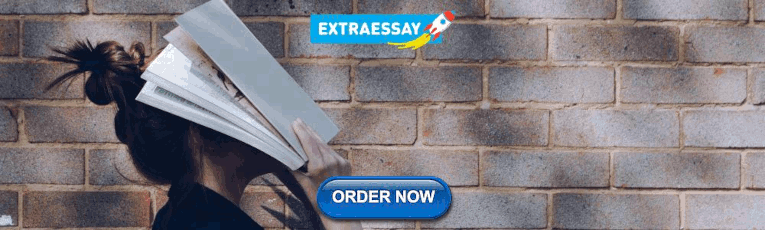
1.4.8. AP Practice ¶
The following is a 2019 AP CSA sample question.
1-4-13: Consider the following code segment.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int truncated result where everything to the right of the decimal point is dropped.
2. Assignment Statements
One of the most common statements (instructions) in C++ is the assignment statement , which has the form:
= is the assignment operator . This statement means that the expression on the right hand side should be evaluated, and the resulting value stored at the desitnation named on the left. Most often this destination is a variable name, although in come cases the destination is itself arrived at by evaluating an expression to compute where we want to save the value.
Some examples of assignment statements would be
Now, a few things worth noting:
These statements manipulate 4 different variables: pi , r , areaOfCircle and circumferenceOfCircle .
We have to assume that r already contains a sensible value if we are to believe that these assignments will do anythign useful.
The last two only make sense if the first assignment has been performed already. Luckily, when we arrange statements into a straightline arrangement like this, they are performed in that same order.
Note that we have reused pi in two different statements. We didn't need to do this. I could instead have written
but I think the original version is easier to read.
When using variables on either side of an assignment, we need to declare the variables first:
Actually, we can combine the operations of declaring a varable and of assigning its first, or initial value:
Technically these are no longer assignments. Instead they are called initialization statements. But the effect is much the same.
I actually prefer this second, combined version, by the way. One of the more common programming errors is declarign a variable, forgetting to assign it a value, but later trying to use it in a computation anyway. If the variable isn't initialized, you basically get whatever bits happened to be left in memory by the last program that used that address. So you wind up taking an essentially random group of bits, feeding them as input to a calculation, feeding that result into another calculation, and so on, until eventually some poor schmuck gets a telephone bill for $1,245,834 or some piece of expensive computer-controlled machinery tears itself to pieces.
By getting into a habit of always initializing variables while declaring them, I avoid most of the opportunities for ever making this particular mistake.
In the Forum:
no comments available
Variable Assignment
To "assign" a variable means to symbolically associate a specific piece of information with a name. Any operations that are applied to this "name" (or variable) must hold true for any possible values. The assignment operator is the equals sign which SHOULD NEVER be used for equality, which is the double equals sign.
The '=' symbol is the assignment operator. Warning, while the assignment operator looks like the traditional mathematical equals sign, this is NOT the case. The equals operator is '=='
Design Pattern
To evaluate an assignment statement:
- Evaluate the "right side" of the expression (to the right of the equal sign).
- Once everything is figured out, place the computed value into the variables bucket.
We've already seen many examples of assignment. Assignment means: "storing a value (of a particular type) under a variable name" . Think of each assignment as copying the value of the righthand side of the expression into a "bucket" associated with the left hand side name!
Read this as, the variable called "name" is "assigned" the value computed by the expression to the right of the assignment operator ('=');
Now that you have seen some variables being assigned, tell me what the following code means?
The answer to above questions: the assignment means that lkjasdlfjlskdfjlksjdflkj is a variable (a really badly named one), but a variable none-the-less. jlkajdsf and lkjsdflkjsdf must also be variables. The sum of the two numbers held in jlkajdsf and lkjsdflkjsdf is stored in the variable lkjasdlfjlskdfjlksjdflkj.
Examples of builtin Data and Variables (and Constants)
For more info, use the "help" command: (e.g., help realmin);
Examples of using Data and Variable
Pattern to memorize, assignment pattern.
The assignment pattern creates a new variable, if this is the first time we have seen the "name", or, updates the variable to a new value!
Read the following code in English as: First, compute the value of the thing to the right of the assignment operator (the =). then store the computed value under the given name, destroying anything that was there before.
Or more concisely: assign the variable "name" the value computed by "right_hand_expression"
Assignment Statement in C
How to assign values to the variables? C provides an assignment operator for this purpose, assigning the value to a variable using assignment operator is known as an assignment statement in C.
The function of this operator is to assign the values or values in variables on right hand side of an expression to variables on the left hand side.
The syntax of the assignment expression
Variable = constant / variable/ expression;
The data type of the variable on left hand side should match the data type of constant/variable/expression on right hand side with a few exceptions where automatic type conversions are possible.
Examples of assignment statements,
b = c ; /* b is assigned the value of c */ a = 9 ; /* a is assigned the value 9*/ b = c+5; /* b is assigned the value of expr c+5 */
The expression on the right hand side of the assignment statement can be:
An arithmetic expression; A relational expression; A logical expression; A mixed expression.
The above mentioned expressions are different in terms of the type of operators connecting the variables and constants on the right hand side of the variable. Arithmetic operators, relational
Arithmetic operators, relational operators and logical operators are discussed in the following sections.
For example, int a; float b,c ,avg, t; avg = (b+c) / 2; /*arithmetic expression */ a = b && c; /*logical expression*/ a = (b+c) && (b<c); /* mixed expression*/
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
Related Posts
- #define to implement constants
- Preprocessor in C Language
- Pointers and Strings
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Notify me of follow-up comments by email.
Notify me of new posts by email.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
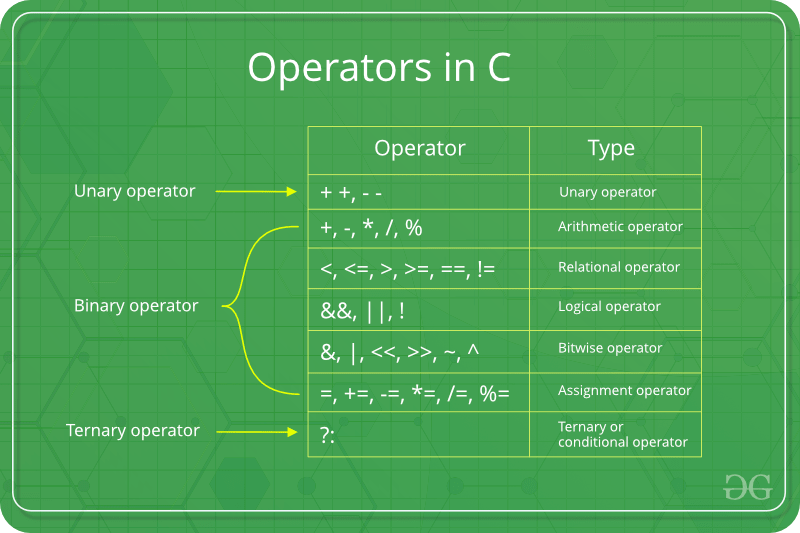
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
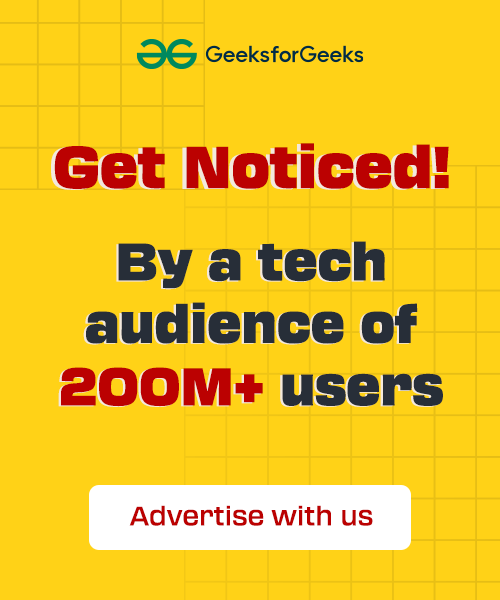
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Statement vs Expression – What's the Difference in Programming?

Learning the syntax of a programming language is key if you want to use that language effectively. This is true for both new and experienced developers.
And one of the most important things to pay attention to while learning a programming language is whether the code you're dealing with is a statement or an expression.
It can sometimes be confusing to differentiate between statements and expressions in programming. So this article is meant to simplify the differences so that you can improve your programming skills and become a better developer.
What is an Expression in Programming?
An expression is any word or group of words or symbols that is a value. In programming, an expression is a value, or anything that executes and ends up being a value.
It is necessary to understand that a value is unique. For example, const , let , 2 , 4 , s , a , true , false , and world are values because each of them is unique in meaning or character.
Let's look at some code as an example:
Judging from the code above, const , price , = , and 500 are expressions because each of them has a definite and unique meaning or value. But if we take all of them together const price = 500 - then we have a statement.
Let's look at another example:
Looking at the code above, you can see an anonymous function is assigned to a variable. Oh, wait! You might know that any function is a statement. Can it also be an expression?
Yes! A "function" and a "class" are both statements and expressions because they can perform actions (do or not do tasks) and still execute to a value.
This brings us to statements – so what are they?
What is a Statement in Programming?
A statement is a group of expressions and/or statements that you design to carry out a task or an action.
Statements are two-sided – that is, they either do tasks or don't do them. Any statement that can return a value is automatically qualified to be used as an expression. That is why a function or class is a statement and also an expression in JavaScript.
If you look at the example of the function under the section on expressions, you can see it is assigned and execute to a value passed to a variable. That is why it is an expression in that case.
Examples of Statements in Programming
Inline statements.
The whole of the code above is a statement because it carries out the task of assigning $2000 to amount . It is safe to say a line of code is a statement because most compilers or interpreters don't execute any standalone expression.
Block statements
Look at the below if statement:
The if statement is a statement because it helps us check whether I love you or not. As I have said before, it is two-sided: this code finds out whether "I love you" or not, and that is why it is a statement. Also, it doesn't return any value but it can create side effects.
Here's a loop statement:
In short, any loop is a statement because if it can only do the tasks it is meant to do or not – does loop and doesn't loop. But a loop can't execute to a value in the end. They can only have side effects in JavaScript. Once they can execute to a value in a programming language, then they can also be used as an expression.
For example, you can use forloop and if statement as expressions in Python.
There is also an "IF" expression in Python. That means that something that is a statement in one language can be an expression (or both statement and expression) in another.
Look at the below function statement:
We declare the function add(firstNumber, secondNumber) and it returns a value. The function is called with two arguments as in add(2, 3) by declaration and so it is a statement. If you pay close attention, you will realize that calling the function as a statement is useless since it has no side effect.
Hey, stop! How can we turn it into an expression? Oh yeah, we can do it like this:
Though the function is now an expression the way it is called above, the whole of the code is still a statement.
Check out this class statement:
You can see that we declare the class "Person" and instantiate and assign it to "User" immediately. So, it is used as an expression.
Now, let's use it as a statement:
A class is similar to a function in the sense that it can be declared, assigned, or used as an operand just like a class. So, a class is a statement and/or an expression.
The Main Differences Between an Expression and a Statement in Programming
Expressions can be assigned or used as operands, while statements can only be declared.
Statements create side effects to be useful, while expressions are values or execute to values.
Expressions are unique in meaning, while statements are two-sided in execution. For example, 1 has a certain value while go( ) may be executed or not.
Statements are the whole structure, while expressions are the building blocks. For example, a line or a block of code is a statement.
Why You Should Know the Difference
First of all, understanding the difference between statements and expressions should make learning new programming languages less surprising. If you're used to JavaScript, you may be surprised by Python's ability to assign an if statement as a variable which is not possible in JavaScript.
Second, it makes it easy to use programming paradigms across different programming languages.
For example, a JavaScript "if statement" cannot be used as an expression because it can't execute to a value – it can only create side effects. Yet, you can use the ternary operator if you want to avoid the side effects of using an if statement in JavaScript.
For this reason, you can understand why some programmers avoid if statements by using the ternary operator in JavaScript. It is because they want to avoid side effects .
It also makes your realize why you have to be always careful about the scope of your variables whenever you use a statement. This is true because statements mostly have side effects to be useful, and it is reasonable to understand the scope of your variables and operations. For example,
Hey wait! What would be logged in the console if you ran the code above?
Tell yourself the answer first and then paste the code in the console to confirm. If you you're wrong, you need to learn more about scope and side effects. But if you're right, try to make those functions a bit better to avoid the confusion they may generate.
Knowing the difference also helps you to easily identify non-composable and composable syntaxes (functions, classes, modules, and so on) of a programming language. This makes porting your experience from one programming language to another more interesting and direct.
Wrapping Up
Now that you understand the difference between expressions and statements in programming, and you know why understanding the differences is important, you can identify pieces of code as expressions or statements while coding.
Next time, we'll go even further and help make learning a second programming language easier.
Go and get things done now! See you soon.
I am planning to share a lot about programming tips and tutorials in 2023. If you're struggling to build projects or you want to stay connected with my write-ups and videos, please join my list at YouTooCanCode or subscribe to my YouTube channel at You Too Can Code on YouTube .
Ayobami loves writing history with JavaScript(React) and PHP(Laravel). He has been making programming fun to learn for learners. Check him out on YouTube: https://bit.ly/3usOu3s
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
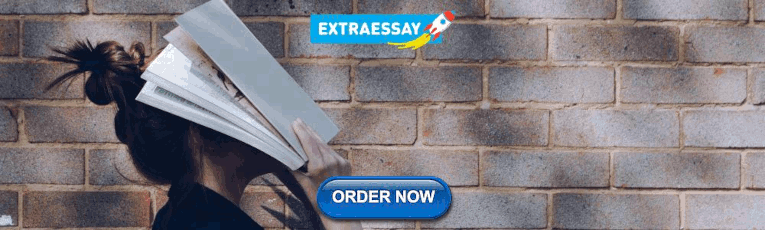
IMAGES
VIDEO
COMMENTS
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
An Assignment statement is a statement that is used to set a value to the variable name in a program. Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
The assignment statement is used to store a value in a variable. As in most programming languages these days, the assignment statement has the form: <variable>= <expression>; For example, once we have an int variable j, we can assign it the value of expression 4 + 6: int j; j= 4+6; As a convention, we always place a blank after the = sign but ...
The assignment operator = is used to associate a variable name with a given value. For example, type the command: a=3.45. in the command line window. This command assigns the value 3.45 to the variable named a. Next, type the command: a. in the command window and hit the enter key. You should see the value contained in the variable a echoed to ...
Assignment: An assignment is a statement in computer programming that is used to set a value to a variable name. The operator used to do assignment is denoted with an equal sign (=). This operand works by assigning the value on the right-hand side of the operand to the operand on the left-hand side. It is possible for the same variable to hold ...
The program segment below declares three INTEGER variables. The first assignment statement saves an integer value to variable Unit. The second saves a real number 100.99 into variable Amount. However, since Amount is an INTEGER variable, the real value 100.99 is converted to ...
The assignment statement is an instruction that stores a value in a variable. You use this instruction any time you want to update the value of a variable. The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ).
Assignment Statements. Assignment statements in a program come in two forms - with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. ... For example, suppose we want to verify the following program so the assert statement at the end will hold: import org ...
The program uses an assignment statement. The assignment statement puts the value 123 into the variable. In other words, while the program is executing there will be a 64 bit section of memory that holds the value 123. Remember that the word "execute" is often used to mean "run". You speak of "executing a program" or "executing" a line of the ...
Three interrelated programming concepts are variables, declarations and assignment statements. Variables The concept of a variable is a powerful programming idea. It's called a variable because - now pay attention - it varies. When you see it used in a program, the variable is often written like this r = 255; (r is the variable and the ...
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator =.
Rule 1. Name must be comprised of digits, upper case letters, lower case letters, and the underscore character "_". Rule 2. Must begin with a letter or underscore. A good name for a variable is short but suggestive of its role: Circle_Area.
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Assignment statements initialize or change the value stored in a variable using the assignment operator =. An assignment statement always has a single variable on the left hand side.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
2. Assignment Statements. One of the most common statements (instructions) in C++ is the assignment statement, which has the form: destination = expression ; = is the assignment operator. This statement means that the expression on the right hand side should be evaluated, and the resulting value stored at the desitnation named on the left.
Assignment means: "storing a value (of a particular type) under a variable name". Think of each assignment as copying the value of the righthand side of the expression into a "bucket" associated with the left hand side name! Read this as, the variable called "name" is "assigned" the value computed by the expression to the right of the ...
Learn the basics of assignment statements in Python in this tutorial. We'll cover the syntax and usage of the assignment operator, including multiple assignm...
C provides an assignment operator for this purpose, assigning the value to a variable using assignment operator is known as an assignment statement in C. The function of this operator is to assign the values or values in variables on right hand side of an expression to variables on the left hand side. The syntax of the assignment expression
Assignment. x >>= y. x = x >> y. Table 2. When writing any kind of program, there will be many instances where you will need to use a variable as part of an expression and you will want to assign the result of that expression into the same variable. An example of this kind of operation is when you want to increment a variable by some value ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
Examples of Statements in Programming Inline statements let amount = $2000; The whole of the code above is a statement because it carries out the task of assigning $2000 to amount. It is safe to say a line of code is a statement because most compilers or interpreters don't execute any standalone expression. Photo by Nimi Diffa / Unsplash Block ...