
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
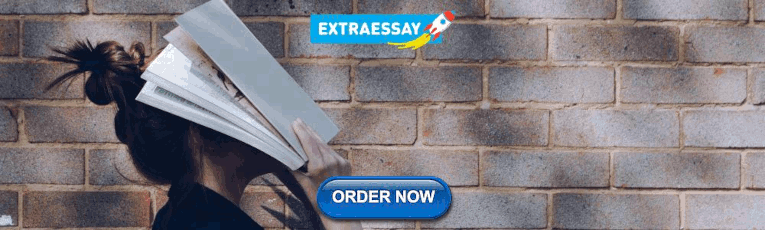
Assignment Operators in C
In C, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable or an expression. The value to be assigned forms the right hand operand, whereas the variable to be assigned should be the operand to the left of = symbol, which is defined as a simple assignment operator in C. In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple assignment operator (=)
The = operator is the most frequently used operator in C. As per ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed. You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented assignment operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression a+=b has the same effect of performing a+b first and then assigning the result back to the variable a.
Similarly, the expression a<<=b has the same effect of performing a<<b first and then assigning the result back to the variable a.
Here is a C program that demonstrates the use of assignment operators in C:
When you compile and execute the above program, it produces the following result −
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
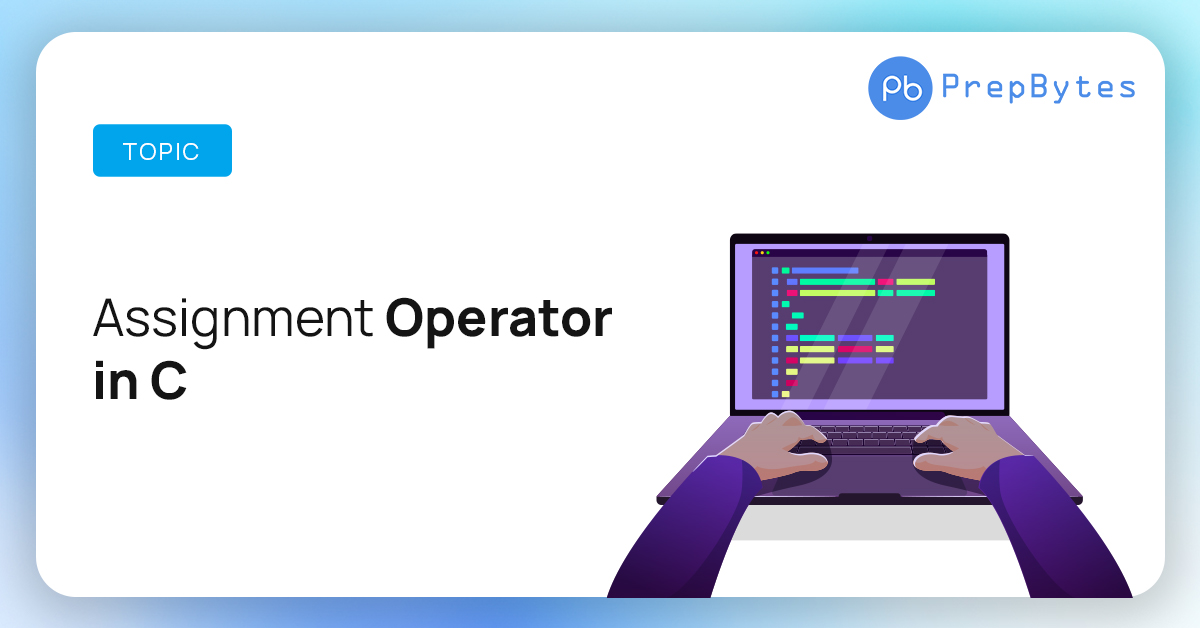
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
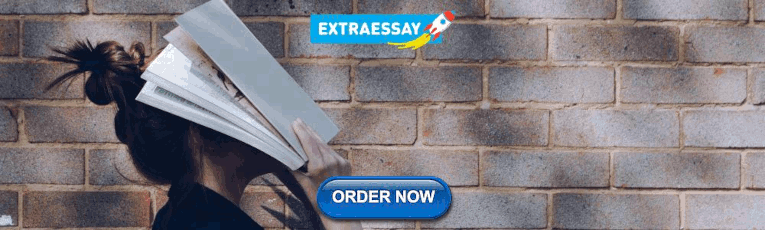
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
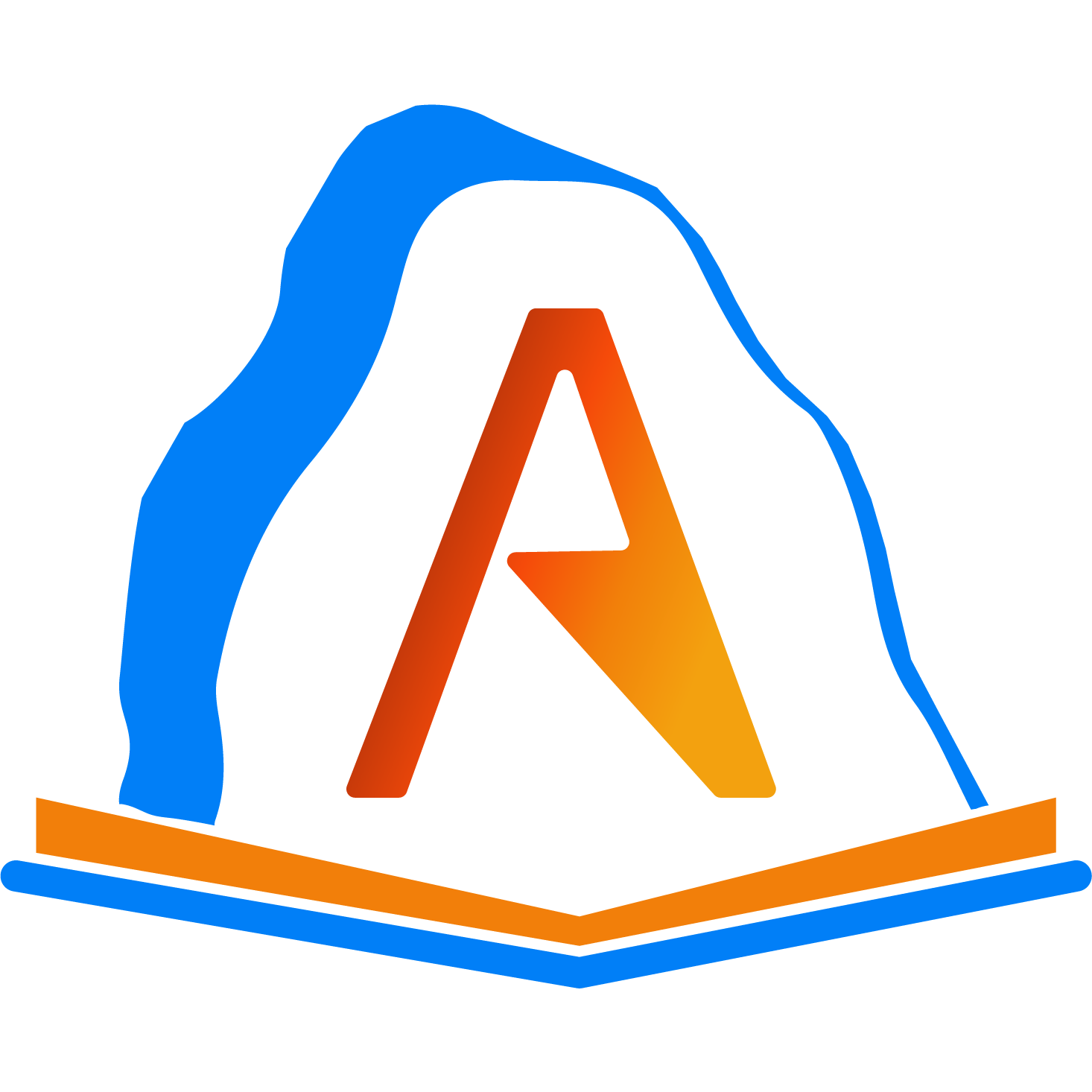
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- Coding Practice
- Activecode Exercises
- Mixed Up Code Practice
- 6.1 Multiple assignment
- 6.2 Iteration
- 6.3 The while statement
- 6.5 Two-dimensional tables
- 6.6 Encapsulation and generalization
- 6.7 Functions
- 6.8 More encapsulation
- 6.9 Local variables
- 6.10 More generalization
- 6.11 Glossary
- 6.12 Multiple Choice Exercises
- 6.13 Mixed-Up Code Exercises
- 6.14 Coding Practice
- 6. Iteration" data-toggle="tooltip">
- 6.2. Iteration' data-toggle="tooltip" >
Before you keep reading...
Runestone Academy can only continue if we get support from individuals like you. As a student you are well aware of the high cost of textbooks. Our mission is to provide great books to you for free, but we ask that you consider a $10 donation, more if you can or less if $10 is a burden.
Making great stuff takes time and $$. If you appreciate the book you are reading now and want to keep quality materials free for other students please consider a donation to Runestone Academy. We ask that you consider a $10 donation, but if you can give more thats great, if $10 is too much for your budget we would be happy with whatever you can afford as a show of support.
6.1. Multiple assignment ¶
I haven’t said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value.
The active code below reassigns fred from 5 to 7 and prints both values out.
The output of this program is 57 , because the first time we print fred his value is 5, and the second time his value is 7.
The active code below reassigns fred from 5 to 7 without printing out the initial value.
However, if we do not print fred the first time, the output is only 7 because the value of fred is just 7 when it is printed.
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure:
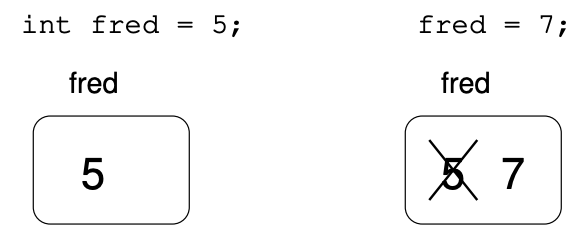
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because C++ uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
An assignment statement uses a single = symbol. For example, x = 3 assigns the value of 3 to the variable x . On the other hand, an equality statement uses two = symbols. For example, x == 3 is a boolean that evaluates to true if x is equal to 3 and evaluates to false otherwise.
First of all, equality is commutative, and assignment is not. For example, in mathematics if \(a = 7\) then \(7 = a\) . But in C++ the statement a = 7; is legal, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If \(a = b\) now, then \(a\) will always equal \(b\) . In C++, an assignment statement can make two variables equal, but they don’t have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.
- Checking if a is equal to b
- Assigning a to the value of b
- Setting the value of a to 4
Q-4: What will print?
- There are no spaces between the numbers.
- Remember, in C++ spaces must be printed.
- Carefully look at the values being assigned.
Q-5: What is the correct output?
- Remember that printing a boolean results in either 0 or 1.
- Is x equal to y?
- x is equal to y, so the output is 1.
- C++ Language
- Ascii Codes
- Boolean Operations
- Numerical Bases
Introduction
Basics of c++.
- Structure of a program
- Variables and types
- Basic Input/Output
Program structure
- Statements and flow control
- Overloads and templates
- Name visibility
Compound data types
- Character sequences
- Dynamic memory
- Data structures
- Other data types
- Classes (I)
- Classes (II)
- Special members
- Friendship and inheritance
- Polymorphism
Other language features
- Type conversions
- Preprocessor directives
Standard library
- Input/output with files
Assignment operator (=)
Arithmetic operators ( +, -, *, /, % ), compound assignment (+=, -=, *=, /=, %=, >>=, <<=, &=, ^=, |=), increment and decrement (++, --), relational and comparison operators ( ==, =, >, <, >=, <= ), logical operators ( , &&, || ), conditional ternary operator ( ), comma operator ( , ), bitwise operators ( &, |, ^, ~, <<, >> ), explicit type casting operator, other operators, precedence of operators.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Compound Statements in C++
- Rethrowing an Exception in C++
- Variable Shadowing in C++
- Condition Variables in C++ Multithreading
- C++23 <print> Header
- Literals In C++
- How to Define Constants in C++?
- C++ Variable Templates
- Introduction to GUI Programming in C++
- Concurrency in C++
- Hybrid Inheritance In C++
- Dereference Pointer in C
- shared_ptr in C++
- Nested Inline Namespaces In C++20
- C++20 std::basic_syncbuf
- std::shared_mutex in C++
- Partial Template Specialization in C++
- Pass By Reference In C
- Address Operator & in C
Assignment Operators In C++
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Geeks Premier League
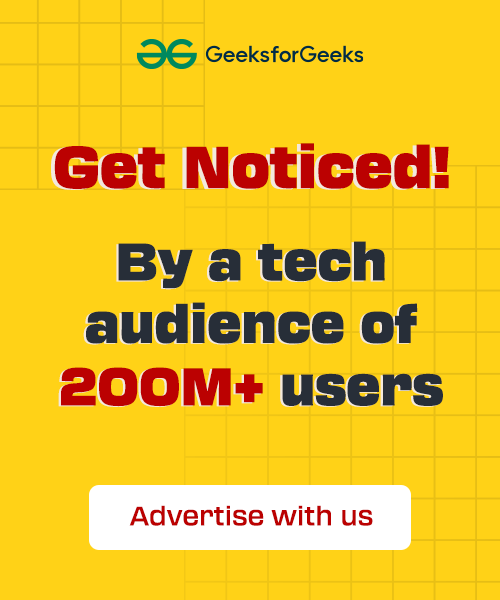
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Multiple assignment operators
Description.
Assign values to name(s).
%<-% and %->% invisibly return value .
These operators are used primarily for their assignment side-effect. %<-% and %->% assign into the environment in which they are evaluated.
Name Structure
At its simplest, the name structure may be a single variable name, in which case %<-% and %->% perform regular assignment, x %<-% list(1, 2, 3) or list(1, 2, 3) %->% x .
To specify multiple variable names use a call to c() , for example c(x, y, z) %<-% c(1, 2, 3) .
When value is neither an atomic vector nor a list, %<-% and %->% will try to destructure value into a list before assigning variables, see destructure() .
object parts
Like assigning a variable, one may also assign part of an object, c(x, x[[1]]) %<-% list(list(), 1) .
nested names
One can also nest calls to c() when needed, c(x, c(y, z)) . This nested structure is used to unpack nested values, c(x, c(y, z)) %<-% list(1, list(2, 3)) .
collector variables
To gather extra values from the beginning, middle, or end of value use a collector variable. Collector variables are indicated with a ... prefix, c(...start, z) %<-% list(1, 2, 3, 4) .
skipping values
Use . in place of a variable name to skip a value without raising an error or assigning the value, c(x, ., z) %<-% list(1, 2, 3) .
Use ... to skip multiple values without raising an error or assigning the values, c(w, ..., z) %<-% list(1, NA, NA, 4) .
default values
Use = to specify a default value for a variable, c(x, y = NULL) %<-% tail(1, 2) .
When assigning part of an object a default value may not be specified because of the syntax enforced by R . The following would raise an "unexpected '=' ..." error, c(x, x[[1]] = 1) %<-% list(list()) .
For more on unpacking custom objects please refer to destructure() .
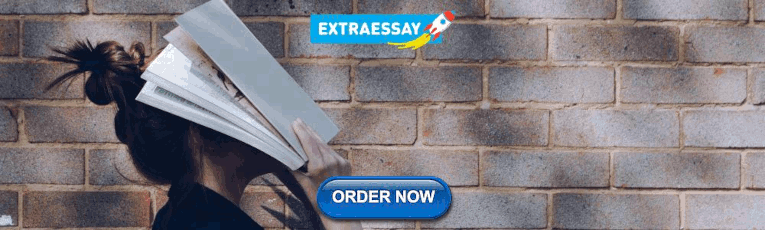
IMAGES
VIDEO
COMMENTS
As others have said, the order in which this gets executed is deterministic. The operator precedence of the = operator guarantees that this is executed right-to-left. In other words, it guarantees that sample2 is given a value before sample1. However, multiple assignments on one row is bad practice and banned by many coding standards (*). First ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=. Subtract AND assignment operator.
The documentation you cite derives its statement from the C++ standard's [class.copy] (C++14) / [class.copy.assign] (C++17) section:. 15.8.2 Copy/move assignment operator. A user-declared copy assignment operator X::operator= is a non-static non-template member function of class X with exactly one parameter of type X,X&,const X&,volatile X& or const volatile X&. 121 [Note: An overloaded ...
Here is a list of the assignment operators that you can find in the C language: Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side. Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
The reason for this is the operator precedence of the assignment operator over comma as explained below. Property of Precedence in Comma as an Operator. ... A comma as a separator is used to separate multiple variables in a variable declaration, and multiple arguments in a function call. It is the most common use of comma operator in C.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
The expressions are evaluated from right to left, and the final result of the expression is the value of the last assignment. Each variable is assigned a value of 0. Another way to put multiple assignments in a single statement is to use the ,sequence operator. We could write a simple swap statement in one line with three variables, as follows:
No, that assigns a, b, and c to c+5. try parenthesizing the "c+=5". frice2014. No, that assigns a, b, and c to c+5. I did it that way because you were adding the same values to them (5) and they all started with the same values (10) That is starting to look more like a recursive operation than variable assignment.
In this C++ tutorial video, you will learn about combined assignment operators and multiple assignment techniques. Discover how to efficiently perform operat...
6.1. Multiple assignment. I haven't said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value. The active code below reassigns fred from 5 to 7 and prints both values out. The output of this program is 57 ...
Assignment operator (=) The assignment operator assigns a value to a variable. 1: ... A single expression may have multiple operators. For example: 1: x = 5 + 7 % 2; In C++, the above expression always assigns 6 to variable x, because the % operator has a higher precedence than the + operator, and is always evaluated before. Parts of the ...
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
At its simplest, the name structure may be a single variable name, in which case %<-% and %->% perform regular assignment, x. %<-% list(1, 2, 3) or list(1, 2, 3) %->% x . To specify multiple variable names use a call to c(), for example c(x, y, z) %<-% c(1, 2, 3) . When value is neither an atomic vector nor a list, %<-% and %->% will try to ...
I am self-learning C++. Following the example of operator + at this link.Suppose I want to modify the operator + a little: instead of adding box1 and box2, I want to input a scaling factor z to the overloaded operator +, so that the intended operator is box1 + z*box2, where z is multiplied by box2.length, box2.height and box2.breadth.How should I modify the code to achieve that?