- C - Introduction
- C - Comments
- C - Data Types
- C - Type Casting
- C - Operators
- C - Strings
- C - Booleans
- C - If Else
- C - While Loop
- C - For Loop
- C - goto Statement
- C - Continue Statement
- C - Break Statement
- C - Functions
- C - Scope of Variables
- C - Pointers
- C - Typedef
- C - Format Specifiers
- C Standard Library
- C - Data Structures
- C - Examples
- C - Interview Questions
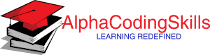
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
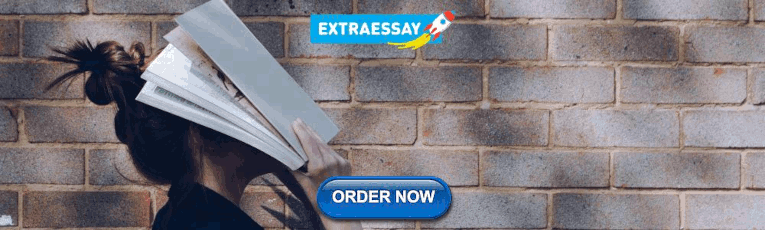
C - Bitwise OR and assignment operator
The Bitwise OR and assignment operator (|=) assigns the first operand a value equal to the result of Bitwise OR operation of two operands.
(x |= y) is equivalent to (x = x | y)
The Bitwise OR operator (|) is a binary operator which takes two bit patterns of equal length and performs the logical OR operation on each pair of corresponding bits. It returns 1 if either or both bits at the same position are 1, else returns 0.
The example below describes how bitwise OR operator works:
The code of using Bitwise OR operator (|) is given below:
The output of the above code will be:
Example: Find largest power of 2 less than or equal to given number
Consider an integer 1000. In the bit-wise format, it can be written as 1111101000. However, all bits are not written here. A complete representation will be 32 bit representation as given below:
Performing N |= (N>>i) operation, where i = 1, 2, 4, 8, 16 will change all right side bit to 1. When applied on 1000, the result in 32 bit representation is given below:
Adding one to this result and then right shifting the result by one place will give largest power of 2 less than or equal to 1000.
The below code will calculate the largest power of 2 less than or equal to given number.
The above code will give the following output:
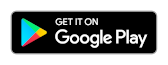
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - While loop
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Recursion
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Bitwise Operators in C
The bitwise operators in C allow a low-level manipulation of data stored in computer’s memory. The bitwise operators contrast with logical operators in C, which perform variable level operations. For example, the logical AND operator (represented by && symbol) performs AND operation on two Boolean expressions, the bitwise AND operator (represented by & symbol) performs the AND operation on each corresponding bit of the two operands.
For the three logical operators &&, || and !, the corresponding bitwise operators in C are &, | and ~. Additionally, the symbols ^ (XOR), << (left shift) and >> (right shift) are the other bitwise operators.
Even though these operators work on individual bits, they need the operands in the form C data types or variables only, as a variable occupies a specific number of bytes in the memory.
& operator
The bitwise AND (&) operator performs as per the following truth table −
Bitwise binary AND performs logical operation on the bits in each position of a number in its binary form.
Assuming that the two int variables a and b have the values 60 (equivalent to 0011 1100 in binary) and 13 (equivalent to 0000 1101 in binary), the a&b operation results in 13, as per the bitwise ANDing of their corresponding bits illustrated below −
The binary number 00001100 corresponds to 12 in decimal.
The bitwise OR (|) operator performs as per the following truth table −
Bitwise binary OR performs logical operation on the bits in each position of a number in its binary form.
Assuming that the two int variables a and b have the values 60 (equivalent to 0011 1100 in binary) and 13 (equivalent to 0000 1101 in binary), the a|b operation results in 61, as per the bitwise OR of their corresponding bits illustrated below −
The binary number 00111101 corresponds to 61 in decimal.
The bitwise XOR (^) operator performs as per the following truth table −
Bitwise binary XOR performs logical operation on the bits in each position of a number in its binary form. The XOR operation is called exclusive OR. The result of XOR is 1 if and only if one of the operands is one. Unlike OR, if both bits are 1, XOR results in 0.
Assuming that the two int variables a and b have the values 60 (equivalent to 0011 1100 in binary) and 13 (equivalent to 0000 1101 in binary), the a^b operation results in 49, as per the bitwise XOR of their corresponding bits illustrated below −
The binary number 00110001 corresponds to 49 in decimal.
<< operator
The left shift operator is represented by the << symbol and shifts each bit in its left-hand operand to the left by the number of positions indicated by the right-hand operand. Any blank spaces generated while shifting are filled up by zeroes.
Assuming that the int variable a has the value 60 (equivalent to 0011 1100 in binary), the a<<2 operation results in 240, as per the bitwise left-shift of its corresponding bits illustrated below −
The binary number 00111100 corresponds to 240 in decimal.
>> operator
The right shift operator is represented by the >> symbol and shifts each bit in its left-hand operand to the right by the number of positions indicated by the right-hand operand. Any blank spaces generated while shifting are filled up by zeroes.
Assuming that the int variable a has the value 60 (equivalent to 0011 1100 in binary), the a>>2 operation results in 15, as per the bitwise right-shift of its corresponding bits illustrated below −
The binary number 00001111 corresponds to 15 in decimal.
The ~ symbol is defined as One’s compliment operator in C. It is a unary operator, needing just one operand. It has the effect of 'flipping' bits, which means that 1 is replaced by 0, and 0 by 1 in the binary representation of any number.
Assuming that the int variable a has the value 60 (equivalent to 0011 1100 in binary), the ~a operation results in -61 in 2’s complement form, as per the bitwise right-shift of its corresponding bits illustrated below −
The binary number 1100 0011 corresponds to -61 in decimal.
- Bitwise Operations in C
Bitwise & and &= Operators
Bitwise | and |= operators, bitwise ^ and ^= operators, bitwise shift ( >> ) and ( << ) operators, arithmetic shorthands (compound assignment statements), lexical analysis and what you can’t do.
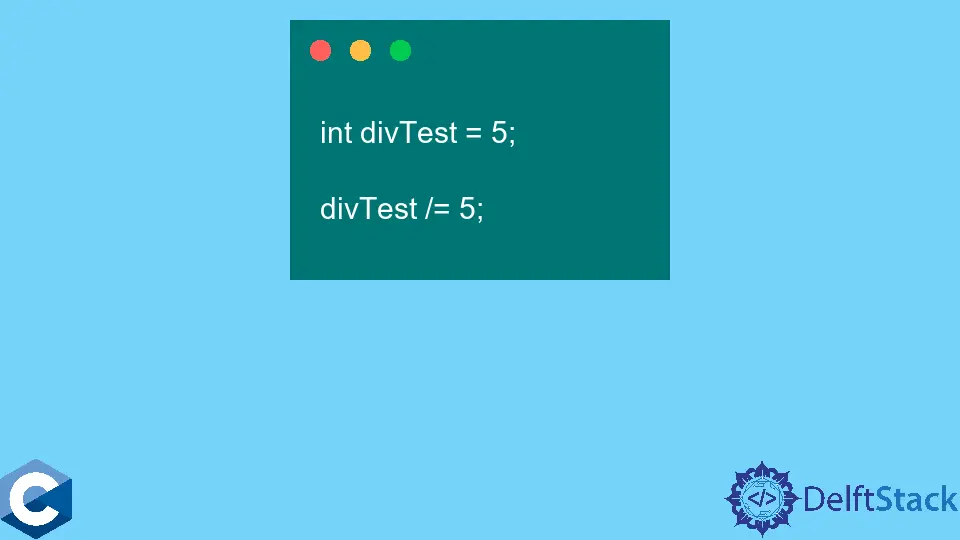
This article discusses bitwise operations (and operators) in C. These concepts extend to C++ as well.
In addition to these operators, the article discusses a commonly misunderstood operator assignment shorthand notation (such as the += operator assignment). We will discuss this in the context of bitwise operators.
The article will have an example of each of these operators showing both notations and their respective outputs. We will also discuss shorthand notations that are not supported by C.
Lastly, we will briefly discuss the lexical analysis, which is a stage in the compilation of the program where the compiler generates tokens. These are the smallest parts of the code, such as keywords, identifiers, constants, string literals, etc.
& is the bitwise and operator. It is used like this:
The above code runs the bitwise and comparison between 0x6A and 0x50 and saves the result to the test variable. The bitwise and operation compares each bit of both arguments.
The result is 1 for each bit if both operands’ bits are 1 , and the result is 0 otherwise.
The binary for 0x6A is 1101010 , and the binary for 0x50 is 1010000 . Therefore, the and operation result is 1000000 .
Now we can discuss the shorthand notation. The above piece of code can conveniently be written as the following:
In general, x &= 5 is equivalent to x = x & 5 . However, this can be extended to other operations too; the most common would be += , -= , *= , /= .
More uncommon examples of this shorthand notation are with bitwise operations such as >>= , <<= , |= , &= , ^= .
| is the bitwise inclusive or operator.
The shorthand notation for this will be:
The resulting value of test is 1111010 .
The bitwise inclusive or operation compares each bit of both arguments. The result is 1 for each bit if either of the operands’ bits is 1 , and the result is 0 only when both operands are 0 .
^ is the bitwise exclusive or operator.
The resulting value of test is: 0111010
The bitwise exclusive or operation compares each bit of both arguments. The resulting bit value is 1 for each bit of the result if the two bits have different values; it is 0 if both bits have the same value.
The shift operators have two operands.
The shift is applied on the operand to the left. And the number of bits that the shift is applied is determined by the right operand.
There are two kinds of shifts: the left shift << , and the right shift >> . It is generally used as follows:
Please see this linked document for more details about the result of the shift operator if you are unsure about its details.
In this example, the bits stored in test5 will be left-shifted (as indicated by the operator’s direction) by 2 bits.
Similarly, for the right shift:
In this example, the bits stored in test5 will be right-shifted by 2 bits.
The shorthand notation for the right and left shifts is similar to what we have above.
Shorthand right shift notation:
Shorthand left shift notation:
This section will briefly discuss shorthands for arithmetic operations. The following two statements after the variable declaration are equivalent:
For addition:
For multiplication:
For division:
For subtraction:
For modulo/remainder ( % ):
Lexical analysis is a stage in which each operand and the operator are separated into tokens. For example, in a statement x + 2 , where x is a variable, the analyzer will separate x and 2 as operands and + as the operator.
For shorthand notation, operators such as |= are stored with a token code, which is enough to identify them. If any shorthands do not work, they (simply) have not been defined for the C lexical analyzer; even if they pass the lexical analyzer, the syntax analyzer will reject them.
This is why shorthands such as >>>> or ||= or &&= do not work. Perhaps there would be a programming language where they are accepted as valid operators.
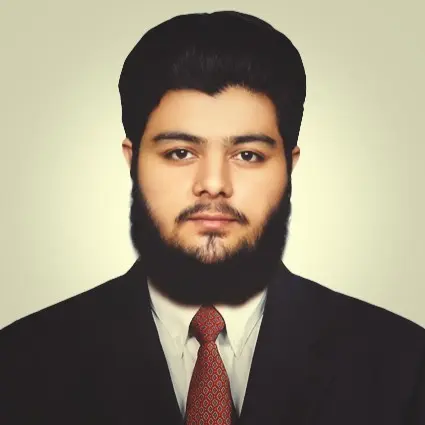
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
Related Article - C Bit
- Sign Extend a 9-Bit Integer in C
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Web Browser
- Single Program Multiple Data (SPMD) Model
- Hybrid Blockchain
- SciPy - Cluster
- How to Reduce Code Duplication in Scala?
- Stacked Bar Chart With Selection Using Altair in Python
- Git - Move Files
- Git - LFS (Large File Storage)
- Git - Hooks
- 5 Best Email Marketing Software
- Principal Components Analysis in Data Mining
- How to Use FlycoSystemBar Library in Android App?
- How to Handle Big Repositories with Git?
- What are Bitcoin Mitigating Attacks?
- How to Create a Waffle Chart in Excel
- How to Add Trendline in Excel Chart
- How To Create Dot Plots In Excel?
- How To Create a Tornado Chart In Excel?
- How to Fix “Failed to install the following Android SDK packages as some licenses have not been accepted” Error in Android Studio?
Bitwise AND operator in Programming
In programming, Bitwise Operators play a crucial role in manipulating individual bits of data. One of the fundamental bitwise operators is the Bitwise AND operator (&). In this article, we’ll dive deep into what is Bitwise AND operator, its syntax, properties, applications, and optimization techniques, and conclude with its significance in programming.
Table of Content
What is Bitwise AND?
- Bitwise AND Operator
- Bitwise AND Operator Syntax
- Bitwise AND in C/C++
- Bitwise AND in Java
- Bitwise AND in Python
- Bitwise AND in JavaScript
- Bitwise AND in C#
- Properties of Bitwise AND Operator
- Applications of Bitwise AND Operator
The Bitwise AND operation ( & ) is a binary operation that operates on each bit of its operands independently. It returns a new value where each bit of the result is determined by applying the AND operation to the corresponding bits of the operands.
The truth table for the Bitwise AND operation is as follows:
In this table, A and B are the variables, and A AND B is the expression representing the logical AND operation. The table shows all possible combinations of truth values for A and B, and the resulting truth value of A AND B for each combination.
The AND operation returns 1 (true) if the both the inputs are 1, and 0 (false) otherwise. This is why the output is 1 for the fourth row, where A and B have values = 1, and 0 for the first, second and third rows, where at least one of A or B has value = 0.
Bitwise AND Operator:
The Bitwise AND operator, represented by the symbol & , is used to perform a logical AND operation on corresponding bits of two integers. It compares each bit of the first operand with the corresponding bit of the second operand and yields a result where a bit is set only if both corresponding bits are set in the operands. Both the operands should be of integral types.
Bitwise AND Operator Syntax:
The Bitwise AND operator (&) is a fundamental component of bitwise operations in programming languages. It operates on individual bits of two integers, comparing each corresponding bit and producing a result based on whether both bits are set or not.
Below, we’ll explore the syntax of the Bitwise AND operator in various programming languages:
Bitwise AND in C/C++:
In C and C++, the syntax of the Bitwise AND operator is straightforward:
Here, operand1 and operand2 are the two integers on which the bitwise AND operation is performed. The result is stored in the variable result.
Bitwise AND in Java:
In Java, the Bitwise AND operator is represented by the symbol &:
Similar to C and C++, operand1 and operand2 are the two operands, and the result is stored in the variable result.
Bitwise AND in Python:
Python also supports bitwise operations, including the Bitwise AND operator, represented by the symbol &:
Similarly, operand1 and operand2 are the two operands, and the result is assigned to the variable result.
Bitwise AND in JavaScript:
In JavaScript, the Bitwise AND operator is also represented by the symbol &:
Again, operand1 and operand2 are the operands, and the result is stored in the variable result.
Bitwise AND in C#:
In C#, the Bitwise AND operator is similar to other languages, represented by the symbol &:
Once again, operand1 and operand2 are the operands, and the result is assigned to the variable result.
Properties of Bitwise AND Operator:
- Commutativity: The order of operands does not affect the result of the bitwise AND operation. In other words, a & b yields the same result as b & a.
- Identity : Performing a bitwise AND operation with zero (0) always results in zero. That is, a & 0 = 0 for any value of a.
- Idempotence : Performing a bitwise AND operation with itself results in the same value. That is, a & a = a.
Applications of Bitwise AND Operator:
- Masking : Bitwise AND is commonly used in masking operations to extract specific bits or flags from a bit pattern.
- Checking Parity of a number : By performing a bitwise AND operation with 1, you can determine whether a number is even or odd. An even number & 1 results in 0, while an odd number & 1 results in 1.
- Clearing Bits : To clear specific bits in an integer, you can use the bitwise AND operator with a mask where the bits you want to clear are set to 0.
Conclusion:
The Bitwise AND operator plays a crucial role in low-level programming, enabling efficient manipulation of individual bits within integers. Understanding its properties, syntax, and applications can significantly enhance your ability to write optimized and efficient code, especially in scenarios where performance is critical. By leveraging the bitwise AND operator effectively, programmers can unlock powerful capabilities for bitwise manipulation and optimization in their programs.
Please Login to comment...
- Programming
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- TutorialKart
- SAP Tutorials
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Bash Script
- Julia Tutorial
- CouchDB Tutorial
- MongoDB Tutorial
- PostgreSQL Tutorial
- Android Compose
- Flutter Tutorial
- Kotlin Android
Web & Server
- Selenium Java
- C++ Tutorials
- C++ Tutorial
- C++ Hello World Program
- C++ Comments
- C++ Variables
- Print datatype of variable
- C++ If Else
- Addition Operator
- Subtraction Operator
- Multiplication Operator
- Division Operator
- Modulus Operator
- Increment Operator
- Decrement Operator
- Simple Assignment
- Addition Assignment
- Subtraction Assignment
- Multiplication Assignment
- Division Assignment
- Remainder Assignment
- Bitwise AND Assignment
- Bitwise OR Assignment
- Bitwise XOR Assignment
- Left Shift Assignment
- Right Shift Assignment
- ADVERTISEMENT
- Bitwise AND
- Bitwise XOR
- Bitwise Complement
- Bitwise Left shift
- Bitwise Right shift
- Logical AND
- Logical NOT
- Not Equal to
- Greater than
- Greater than or Equal to
- Less than or Equal to
- Ternary Operator
- Do-while loop
- Infinite While loop
- Infinite For loop
- C++ Recursion
- Create empty string
- String length
- String comparison
- Get character at specific index
- Get first character
- Get last character
- Find index of substring
- Iterate over characters
- Print unique characters
- Check if strings are equal
- Append string
- Concatenate strings
- String reverse
- String replace
- Swap strings
- Convert string to uppercase
- Convert string to lowercase
- Append character at end of string
- Append character to beginning of string
- Insert character at specific index
- Remove last character
- Replace specific character
- Convert string to integer
- Convert integer to string
- Convert string to char array
- Convert char array to string
- Initialize array
- Array length
- Print array
- Loop through array
- Basic array operations
- Array of objects
- Array of arrays
- Convert array to vector
- Sum of elements in array
- Average of elements in array
- Find largest number in array
- Find smallest number in array
- Sort integer array
- Find string with least length in array
- Create an empty vector
- Create vector of specific size
- Create vector with initial values
- Copy a vector to another
- Vector length or size
- Vector of vectors
- Vector print elements
- Vector iterate using For loop
- Vector iterate using While loop
- Vector Foreach
- Get reference to element at specific index
- Check if vector is empty
- Check if vectors are equal
- Check if vector contains element
- Update/Transform
- Add element(s) to vector
- Append element to vector
- Append vector
- Insert element at the beginning of vector
- Remove first element from vector
- Remove last element from vector
- Remove element at specific index from vector
- Remove duplicates from vector
- Remove elements from vector based on condition
- Resize vector
- Swap elements of two vectors
- Remove all elements from vector
- Reverse a vector
- Sort a vector
- Conversions
- Convert vector to map
- Join vector elements to a string
- Filter even numbers in vector
- Filter odd numbers in vector
- Get unique elements of a vector
- Remove empty strings from vector
- Sort integer vector in ascending order
- Sort integer vector in descending order
- Sort string vector based on length
- Sort string vector lexicographically
- Split vector into two equal halves
- Declare tuple
- Initialise tuple
- Swap tuples
- Unpack tuple elements into variables
- Concatenate tuples
- Constructor
- Copy constructor
- Virtual destructor
- Friend class
- Friend function
- Inheritance
- Multiple inheritance
- Virtual inheritance
- Function overloading
- Operator overloading
- Function overriding
- C++ Try Catch
- Math acos()
- Math acosh()
- Math asin()
- Math asinh()
- Math atan()
- Math atan2()
- Math atanh()
- Math cbrt()
- Math ceil()
- Math copysign()
- Math cosh()
- Math exp2()
- Math expm1()
- Math fabs()
- Math fdim()
- Math floor()
- Math fmax()
- Math fmin()
- Math fmod()
- Math frexp()
- Math hypot()
- Math ilogb()
- Math ldexp()
- Math llrint()
- Math llround()
- Math log10()
- Math log1p()
- Math log2()
- Math logb()
- Math lrint()
- Math lround()
- Math modf()
- Math nearbyint()
- Math nextafter()
- Math nexttoward()
- Math remainder()
- Math remquo()
- Math rint()
- Math round()
- Math scalbln()
- Math scalbn()
- Math sinh()
- Math sqrt()
- Math tanh()
- Math trunc()
- Armstrong number
- Average of three numbers
- Convert decimal to binary
- Factorial of a number
- Factors of a number
- Fibonacci series
- Find largest of three numbers
- Find quotient and remainder
- HCF/GCD of two numbers
- Hello World
- LCM of two numbers
- Maximum of three numbers
- Multiply two numbers
- Sum of two numbers
- Sum of three numbers
- Sum of natural numbers
- Sum of digits in a number
- Swap two numbers
- Palindrome number
- Palindrome string
- Pattern printing
- Power of a number
- Prime number
- Prime Numbers between two numbers
- Print number entered by User
- Reverse a Number
- Read input from user
- ❯ C++ Tutorial
- ❯ C++ Operators
- ❯ Assignment Operators
- ❯ Bitwise OR Assignment
C++ Bitwise OR Assignment (|=) Operator
In this C++ tutorial, you shall learn about Bitwise OR Assignment operator, its syntax, and how to use this operator, with examples.
C++ Bitwise OR Assignment
In C++, Bitwise OR Assignment Operator is used to compute the Bitwise OR operation of left and right operands, and assign the result back to left operand.
The syntax to compute bitwise OR a value of 2 and value in variable x , and assign the result back to x using Bitwise OR Assignment Operator is
In the following example, we take a variable x with an initial value of 9 , add bitwise OR it with value of 2 , and assign the result to x , using Bitwise OR Assignment Operator.
In this C++ Tutorial , we learned about Bitwise OR Assignment Operator in C++, with examples.
Popular Courses by TutorialKart
App developement, web development, online tools.
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
Bitwise Operators in C Programming
C Precedence And Associativity Of Operators
- Compute Quotient and Remainder
- Find the Size of int, float, double and char
C if...else Statement
- Demonstrate the Working of Keyword long
C Programming Operators
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition.
C has a wide range of operators to perform various operations.
C Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
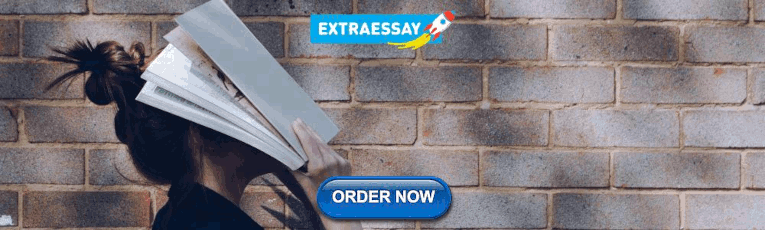
Example 1: Arithmetic Operators
The operators + , - and * computes addition, subtraction, and multiplication respectively as you might have expected.
In normal calculation, 9/4 = 2.25 . However, the output is 2 in the program.
It is because both the variables a and b are integers. Hence, the output is also an integer. The compiler neglects the term after the decimal point and shows answer 2 instead of 2.25 .
The modulo operator % computes the remainder. When a=9 is divided by b=4 , the remainder is 1 . The % operator can only be used with integers.
Suppose a = 5.0 , b = 2.0 , c = 5 and d = 2 . Then in C programming,
C Increment and Decrement Operators
C programming has two operators increment ++ and decrement -- to change the value of an operand (constant or variable) by 1.
Increment ++ increases the value by 1 whereas decrement -- decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.
Example 2: Increment and Decrement Operators
Here, the operators ++ and -- are used as prefixes. These two operators can also be used as postfixes like a++ and a-- . Visit this page to learn more about how increment and decrement operators work when used as postfix .
C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is =
Example 3: Assignment Operators
C relational operators.
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Relational operators are used in decision making and loops .
Example 4: Relational Operators
C logical operators.
An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming .
Example 5: Logical Operators
Explanation of logical operator program
- (a == b) && (c > 5) evaluates to 1 because both operands (a == b) and (c > b) is 1 (true).
- (a == b) && (c < b) evaluates to 0 because operand (c < b) is 0 (false).
- (a == b) || (c < b) evaluates to 1 because (a = b) is 1 (true).
- (a != b) || (c < b) evaluates to 0 because both operand (a != b) and (c < b) are 0 (false).
- !(a != b) evaluates to 1 because operand (a != b) is 0 (false). Hence, !(a != b) is 1 (true).
- !(a == b) evaluates to 0 because (a == b) is 1 (true). Hence, !(a == b) is 0 (false).
C Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Visit bitwise operator in C to learn more.
Other Operators
Comma operator.
Comma operators are used to link related expressions together. For example:
The sizeof operator
The sizeof is a unary operator that returns the size of data (constants, variables, array, structure, etc).
Example 6: sizeof Operator
Other operators such as ternary operator ?: , reference operator & , dereference operator * and member selection operator -> will be discussed in later tutorials.
Table of Contents
- Arithmetic Operators
- Increment and Decrement Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- sizeof Operator
Video: Arithmetic Operators in C
Sorry about that.
Related Tutorials
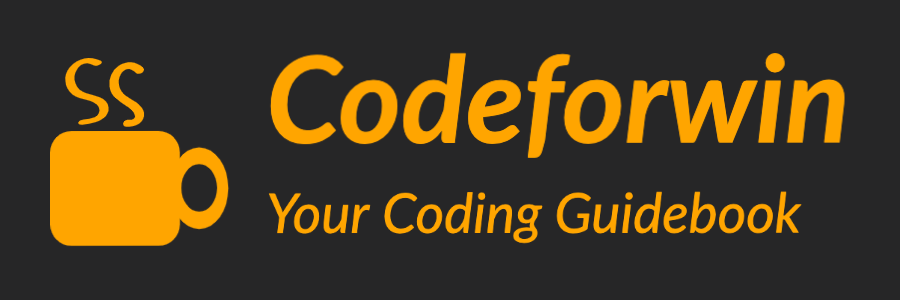
Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
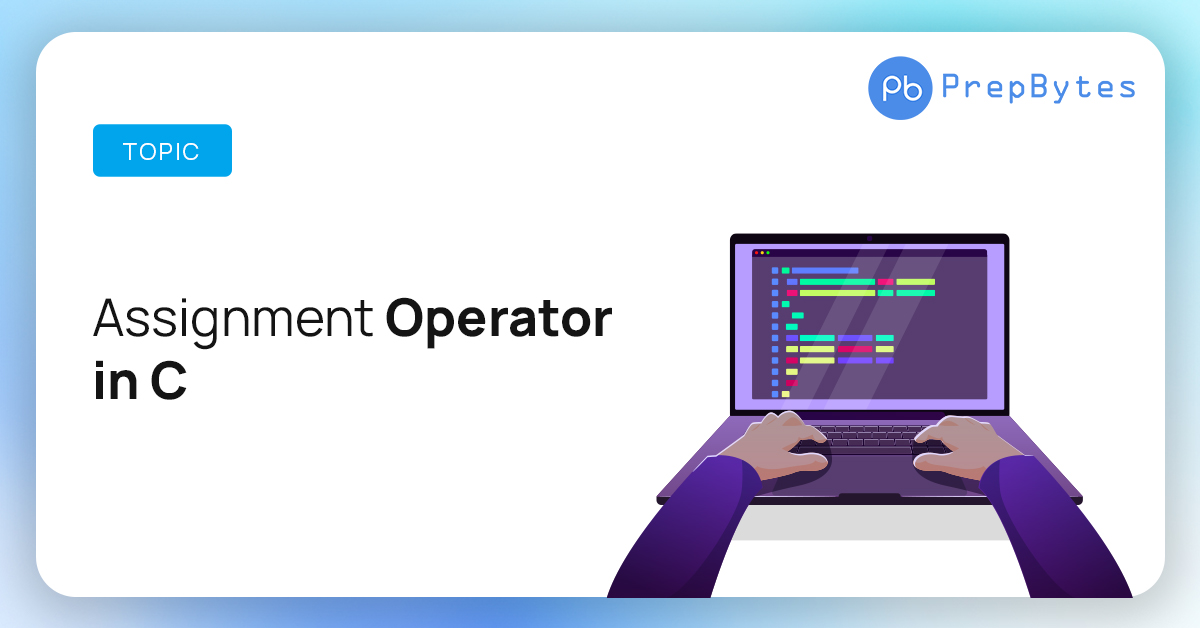
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Bitwise OR assignment (|=)
The bitwise OR assignment ( |= ) operator performs bitwise OR on the two operands and assigns the result to the left operand.
Description
x |= y is equivalent to x = x | y , except that the expression x is only evaluated once.
Using bitwise OR assignment
Specifications, browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Bitwise OR ( | )
- Logical OR assignment ( ||= )
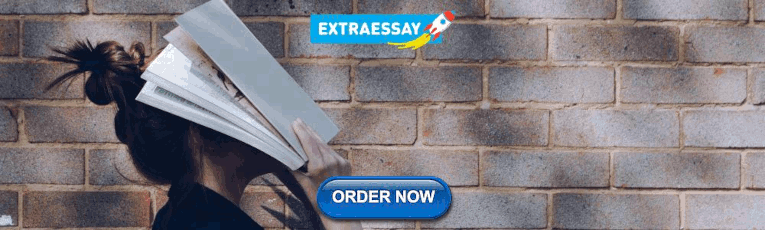
IMAGES
VIDEO
COMMENTS
The Bitwise OR and assignment operator (|=) assigns the first operand a value equal to the result of Bitwise OR operation of two operands. The Bitwise OR operator (|) is a binary operator which takes two bit patterns of equal length and performs the logical OR operation on each pair of corresponding bits. It returns 1 if either or both bits at ...
The output of bitwise AND is 1 if the corresponding bits of two operands is 1. If either bit of an operand is 0, the result of corresponding bit is evaluated to 0. In C Programming, the bitwise AND operator is denoted by &. Let us suppose the bitwise AND operation of two integers 12 and 25. 12 = 00001100 (In Binary) 25 = 00011001 (In Binary)
Bitwise assignment operators. C provides a compound assignment operator for each binary arithmetic and bitwise operation. Each operator accepts a left operand and a right operand, performs the appropriate binary operation on both and stores the result in the left operand. The bitwise assignment operators are as follows. ...
Bitwise Operators in C. In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C. The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
First change type of vals to long int, so it will be able to store at least 30 bits and type of curVal to int to match the scanf call.. Then make sure you only assing 5 bits to vals:. vals = (vals << SIZE) | ( curVal | 0x1F ); And when you print the last value, use the and bitwise operator, since you only want to keep the least significant 5 bits.. printf("%ld", vals & 0x1F );
The bitwise operators in C allow a low-level manipulation of data stored in computer's memory. The bitwise operators contrast with logical operators in C, which perform variable level operations. For example, the logical AND operator (represented by && symbol) performs AND operation on two Boolean expressions, the bitwise AND operator ...
Bitwise right shift is binary operator used to shift bits to right. Consider the below example: int a=15; Which in 8-bit binary will be represented as: a = 0000 1111. c = a >> 3. The above expression a >> 3 shifts bits of variable a three times right and will evaluate to 0000 0001 which is 1 in decimal.
This article discusses bitwise operations (and operators) in C. These concepts extend to C++ as well. In addition to these operators, the article discusses a commonly misunderstood operator assignment shorthand notation (such as the += operator assignment). We will discuss this in the context of bitwise operators. The article will have an ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Bitwise operators are fundamental operators used in computer programming for manipulating individual data bits. They operate at the binary level, performing operations on binary data representations. These operators are commonly used in low-level programming, such as device drivers, embedded systems, and cryptography, where manipulation at the bit level is required.
In C language, the bitwise operators (work at bit-level) are:-. The & ( bitwise AND) in C takes two numbers as operands and performs logical AND on every bit of two numbers. The result of logical AND is 1 only if both bits are 1. The | ( bitwise OR) in C takes two numbers as operands and performs logical OR on every bit of two numbers.
Bitwise AND Operator Syntax: The Bitwise AND operator (&) is a fundamental component of bitwise operations in programming languages. It operates on individual bits of two integers, comparing each corresponding bit and producing a result based on whether both bits are set or not. result = operand1 & operand2;
In C++, Bitwise OR Assignment Operator is used to compute the Bitwise OR operation of left and right operands, and assign the result back to left operand. The syntax to compute bitwise OR a value of 2 and value in variable x, and assign the result back to x using Bitwise OR Assignment Operator is. x |= 2.
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition. In this tutorial, you will learn about different C operators such as arithmetic, increment, assignment, relational, logical, etc. with the help of examples.
Shorthand assignment operator. C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. The above expression a = a + 2 is equivalent to a += 2.
C++20 bitwise compound assignment operators for volatile types were deprecated while being useful for some platforms they are not deprecated See also. Operator precedence. Operator overloading. Common operators assignment: increment decrement: arithmetic: logical: comparison: member access: other: a = b a + = b a -= b a * = b a / = b a % = b a ...
The Bitwise XOR operator (^) is a binary operator which takes two bit patterns of equal length and performs the logical exclusive OR operation on each pair of corresponding bits. It returns 1 if only one of the bits is 1, else returns 0. The example below describes how bitwise XOR operator works: 50 -> 110010 (In Binary)
Here is a list of the assignment operators that you can find in the C language: Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side. Example: int x = 10; // Assigns the value 10 to the variable "x". Addition assignment operator (+=): This ...
Unary, conditional, and assignment operators have precedence from right to left. Types of C++ operators include: Assignment: These operators assign the left-hand side operand the right-hand side operand's value and are ... Bitwise: These C++ operators allow you to perform operations by treating operands like a string of bits for output in ...
2. Using bitwise operations for bool helps save unnecessary branch prediction logic by the processor, resulting from a 'cmp' instruction brought in by logical operations. Replacing the logical with bitwise operations (where all operands are bool) generates more efficient code offering the same result.
The OR bitwise operator is often used in order to create create a bitfield using already existing bitfield and a new flag. It could also be used in order to combine two flags together into a new bitfield. Here is an example with explanation: // Enums are usually used in order to represent. // the bitfields flags, but you can just use the.
The bitwise OR assignment (|=) operator performs bitwise OR on the two operands and assigns the result to the left operand. Try it. Syntax. js. x |= y Description. x |= y is equivalent to x = x | y, except that the expression x is only evaluated once. Examples. Using bitwise OR assignment. js.
Sorted by: 17. The &= and the ++ together are the same as X = (X + 1) % 1024; but can be computed faster by the CPU. 1024-1 is 11 1111 1111 in binary, so for numbers where X < 1024, a bitwise AND will have no effect (since any bit & 1 = the same bit). Interesting things only start to happen where X ≥ 1024, so let's consider the iteration of ...