- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
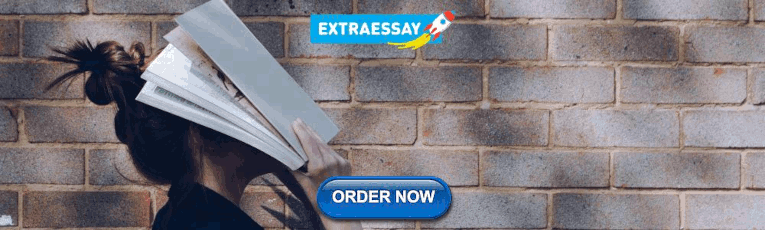
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
- Check Whether a Number is Even or Odd
- Make a Simple Calculator Using switch...case
C Programming Operators
C switch Statement
C if...else Statement
- Find LCM of two Numbers
C Ternary Operator
We use the ternary operator in C to run one code when the condition is true and another code when the condition is false. For example,
Here, when the age is greater than or equal to 18 , Can Vote is printed. Otherwise, Cannot Vote is printed.
Syntax of Ternary Operator
The syntax of ternary operator is :
The testCondition is a boolean expression that results in either true or false . If the condition is
- true - expression1 (before the colon) is executed
- false - expression2 (after the colon) is executed
The ternary operator takes 3 operands (condition, expression1 and expression2) . Hence, the name ternary operator .
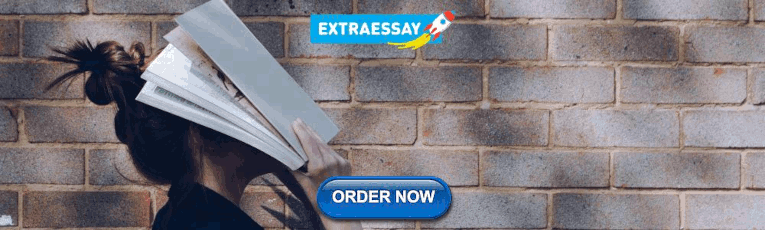
Example: C Ternary Operator
In the above example, we have used a ternary operator that checks whether a user can vote or not based on the input value. Here,
- age >= 18 - test condition that checks if input value is greater or equal to 18
- printf("You can vote") - expression1 that is executed if condition is true
- printf("You cannot vote") - expression2 that is executed if condition is false
Here, the user inputs 12 , so the condition becomes false . Hence, we get You cannot vote as output.
This time the input value is 24 which is greater than 18 . Hence, we get You can vote as output.
Assign the ternary operator to a variable
In C programming, we can also assign the expression of the ternary operator to a variable. For example,
Here, if the test condition is true , expression1 will be assigned to the variable. Otherwise, expression2 will be assigned.
Let's see an example
In the above example, the test condition (operator == '+') will always be true . So, the first expression before the colon i.e the summation of two integers num1 and num2 is assigned to the result variable.
And, finally the result variable is printed as an output giving out the summation of 8 and 7 . i.e 15 .
Ternary Operator Vs. if...else Statement in C
In some of the cases, we can replace the if...else statement with a ternary operator. This will make our code cleaner and shorter.
Let's see an example:
We can replace this code with the following code using the ternary operator.
Here, both the programs are doing the same task, checking even/odd numbers. However, the code using the ternary operator looks clean and concise.
In such cases, where there is only one statement inside the if...else block, we can replace it with a ternary operator .
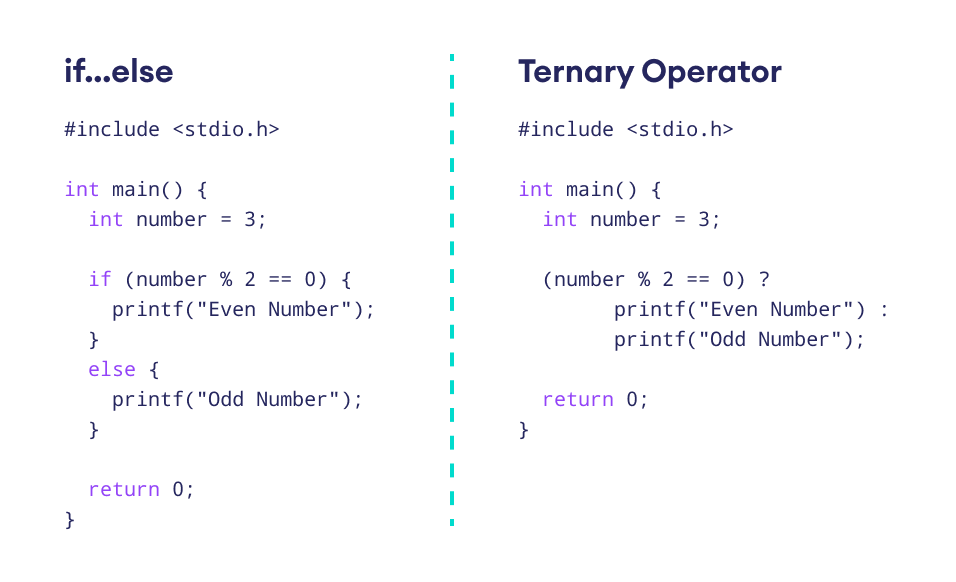
Table of Contents
- Introduction
- Ternary Operator
- Example: Ternary Operator
- Ternary Operator with Variable
- Ternary Operator Vs. if...else
Sorry about that.
Related Tutorials
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?: operator - the ternary conditional operator
- 11 contributors
The conditional operator ?: , also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false , as the following example shows:
As the preceding example shows, the syntax for the conditional operator is as follows:
The condition expression must evaluate to true or false . If condition evaluates to true , the consequent expression is evaluated, and its result becomes the result of the operation. If condition evaluates to false , the alternative expression is evaluated, and its result becomes the result of the operation. Only consequent or alternative is evaluated. Conditional expressions are target-typed. That is, if a target type of a conditional expression is known, the types of consequent and alternative must be implicitly convertible to the target type, as the following example shows:
If a target type of a conditional expression is unknown (for example, when you use the var keyword) or the type of consequent and alternative must be the same or there must be an implicit conversion from one type to the other:
The conditional operator is right-associative, that is, an expression of the form
is evaluated as
You can use the following mnemonic device to remember how the conditional operator is evaluated:
Conditional ref expression
A conditional ref expression conditionally returns a variable reference, as the following example shows:
You can ref assign the result of a conditional ref expression, use it as a reference return or pass it as a ref , out , in , or ref readonly method parameter . You can also assign to the result of a conditional ref expression, as the preceding example shows.
The syntax for a conditional ref expression is as follows:
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative .
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed.
Conditional operator and an if statement
Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following example demonstrates two ways to classify an integer as negative or nonnegative:
Operator overloadability
A user-defined type can't overload the conditional operator.
C# language specification
For more information, see the Conditional operator section of the C# language specification .
Specifications for newer features are:
- Target-typed conditional expression
- Simplify conditional expression (style rule IDE0075)
- C# reference
- C# operators and expressions
- if statement
- ?. and ?[] operators
- ?? and ??= operators
- ref keyword
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Unary, Binary, and Ternary Operators in JavaScript – Explained with Examples
There are many operators in JavaScript that let you carry out different operations.
These operators can be categorized based on the number of operands they require, and I'll be using examples to explain these categories in this tutorial.
The three categories of operators based on the number of operands they require are:
- Unary operators: which require one operand (Un)
- Binary operators: which require two operands (Bi)
- Ternary operators: which require three operands (Ter)
Note that these categories do not only apply to JavaScript. They apply to programming in general.
In the rest of this article, I will share some examples of operators that fall under each category.
I have a video version of this topic you can watch if you're interested.
What is an Operand?
First, let's understand what an operand is. In an operation, an operand is the data that is being operated on . The operand combined with the operator makes an operation.
Look at this example:
Here we have a sum operation (which we will learn more about later). This operation involves the plus operator + , and there are two operands here: 20 and 30 .
Now that we understand operands, let's see examples of operators and the categories they fall under.
What is a Unary Operator?
These operators require one operand for operation. Providing two or more can result in a syntax error. Here are some examples of operators that fall under this category.
the typeof operator
The typeof operator returns the data type of a value. It requires only one operand. Here's an example:
If you pass two operands to it, you'd get an error:
The delete operator
You can use the delete operator to delete an item in an array or delete a property in an object. It's a unary operator that requires only one operand. Here's an example with an array:
Note that deleting items from an array with the delete operator is not the right way to do this. I explained why in this article here
And here's an example with an object:
The Unary plus + operator
This operator is not to be confused with the arithmetic plus operator which I will explain later in this article. The unary plus operator attempts to convert a non-number value to a number. It returns NaN where impossible. Here's an example:
As you can see here again, only one operand is required, which comes after the operator.
I'll stop with these three examples. But know that there are more unary operators such as the increment ++ , decrement ++ , and Logical NOT ! operators, to name a few.
What is a Binary Operator?
These operators require two operands for operation. If one or more than two operands are provided, such operators result in a syntax error.
Let's look at some operators that fall under this category
Arithmetic Operators
All arithmetic operators are binary operators. You have the first operand on the left of the operator, and the second operand on the right of the operator. Here are some examples:
If you don't provide two operands, you will get a syntax error. For example:
Comparison Operators
All comparison operators also require two operands. Here are some examples:
Assignment Operator =
The assignment operator is also a binary operator as it requires two operands. For example:
On the left, you have the first operand, the variable ( const number ), and on the right, you have the second operand, the value ( 20 ).
You're probably asking: "isn't const number two operands?". Well, const and number makes up one operand. The reason for this is const defines the behavior of number . The assignment operator = does not need const . So you can actually use the operator like this:
But it's good practice to always use a variable keyword.
So like I said, think of const number as one operand, and the value on the right as the second operand.
What is a Ternary Operator?
These operators require three operands. In JavaScript, there is one operator that falls under this category – the conditional operator. In other languages, perhaps, there could be more examples.
The Conditional Operator ? ... :
The conditional operator requires three operands:
- the conditional expression
- the truthy expression which gets evaluated if the condition is true
- the falsy expression which gets evaluated if the condition is false .
You can learn more about the Conditional Operator here
Here's an example of how it works:
The first operand – the conditional expression – is score > 50 .
The second operand – the truthy expression – is "Good", which will be returned to the variable scoreRating if the condition is true .
The third operand – the falsy expression – is "Poor", which will be returned to the variable scoreRating if the condition is false .
I've written an article related to this operator that you can check out here . It's about why a ternary operator is not a conditional operator in JavaScript.
Operations in JavaScript involve one or more operands and an operator. And operators can be categorized based on the number of operands they require.
In this article, we've looked at the three categories of operators: unary , binary , and ternary . We also looked at the examples of operators in JavaScript that fall under each category.
Please share this article if you find it helpful.
Developer Advocate and Content Creator passionate about sharing my knowledge on Tech. I simplify JavaScript / ReactJS / NodeJS / Frameworks / TypeScript / et al My YT channel: youtube.com/c/deeecode
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Solve Coding Problems
- Download and Install Python 3 Latest Version
- Statement, Indentation and Comment in Python
- Python | Set 2 (Variables, Expressions, Conditions and Functions)
- Global and Local Variables in Python
- Type Conversion in Python
- Private Variables in Python
- __name__ (A Special variable) in Python
- Taking input in Python
- Taking multiple inputs from user in Python
- Python | Output using print() function
- Python end parameter in print()
- Python | Output Formatting
- Python Operators
Ternary Operator in Python
- Operator Overloading in Python
- Python | a += b is not always a = a + b
- Difference between == and is operator in Python
- Python | Set 3 (Strings, Lists, Tuples, Iterations)
- Python String
- Python Lists
- Python Tuples
- Python Sets
- Dictionaries in Python
- Python Arrays
- Python If Else Statements - Conditional Statements
In this article, you’ll learn how to use the ternary operator in Python. The ternary operator in Python is simply a shorter way of writing an if and if…else statement. We’ll see its syntax along with some practical examples.
Python Ternary Operator and its Benefits
The Python ternary operator determines if a condition is true or false and then returns the appropriate value in accordance with the result. The ternary operator is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more concise — all in just one line of code. It’s particularly handy when you want to avoid writing multiple lines for a simple if-else situation.
Syntax of Ternary Operator in Python
Syntax : [on_true] if [expression] else [on_false] expression : conditional_expression | lambda_expr
Simple Method to Use Ternary Operator
In this example, we are comparing and finding the minimum number by using the ternary operator. The expression min is used to print a or b based on the given condition. For example, if a is less than b then the output is a, if a is not less than b then the output is b.
Ternary Operator Examples
Here we will see the different example to use Python Ternary Operator:
Ternary Operator in Python If-Else
Python ternary operator using tuples, python ternary operator using dictionary, python ternary operator using lambda, print in if ternary operator, limitations of python ternary operator.
Example: Using Native way
Python program to demonstrate nested ternary operator. In this example, we have used simple if-else without using ternary operator.
Example: Using Ternary Operator
In this example, we are using a nested if-else to demonstrate ternary operator. If a and b are equal then we will print a and b are equal and else if a>b then we will print a is greater than b otherwise b is greater than a.
In this example, we are using tuple s to demonstrate ternary operator. We are using tuple for selecting an item and if [a<b] is true it return 1, so element with 1 index will print else if [a<b] is false it return 0, so element with 0 index will print.
In this example, we are using Dictionary to demonstrate ternary operator. We are using tuple for selecting an item and if [a<b] is true it return 1, so element with 1 index will print else if [a<b] is false it return 0, so element with 0 index will print.
In this example, we are using Lambda to demonstrate ternary operator. We are using tuple for selecting an item and if [a<b] is true it return 1, so element with 1 index will print else if [a<b] is false it return 0, so element with 0 index will print.
In this example, we are finding the larger number among two numbers using ternary operator in python3.
Python ternary is used to write concise conditional statements but it too have some limitations.
- Readability: T ernary operator can make simple conditional expressions more concise, it can also reduce the readability of your code, especially if the condition and the expressions are complex.
- Potential for Error : Incorrect placement of parentheses, missing colons, or incorrect order of expressions can lead to syntax errors that might be harder to spot.
- Debugging : When debugging, it might be harder to inspect the values of variables involved in a complex ternary expression.
- Maintenance and Extensibility : Complex ternary expressions might become harder to maintain and extend especially when the codebase grows.
- Can’t use assignment statements: Each operand of the Python ternary operator is an expression , not a statement, that means we can’t use assignment statements inside any of them. Otherwise, the program will throw an error.
Please Login to comment...
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
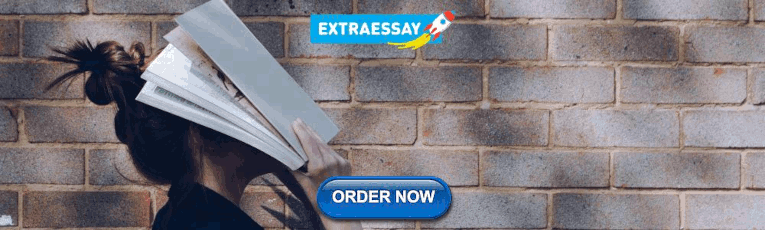
IMAGES
VIDEO
COMMENTS
Your linked question's (Does the C/C++ ternary operator actually have the same precedence as assignment operators?) answer by @hvd shows the answer. The C++ and C grammars for ?: are different.. In C++, the rightmost operand is allowed to be an assignment expression (so the compiler [greedily] treats the = are part of the ?:) while in C the rightmost operand is a conditional-expression instead.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
In computer programming, the ternary conditional operator is a ternary operator that is part of the syntax for basic conditional expressions in several programming languages. ... Bear in mind also that some types allow initialization, but do not allow assignment, or even that the assignment operator and the constructor do totally different ...
Assignment Operator; Relational Operators; Logical Operators; Ternary Operator; Bitwise Operators; Shift Operators; This article explains all that one needs to know regarding Arithmetic Operators. Ternary Operator in Java. Java ternary operator is the only conditional operator that takes three operands. It's a one-liner replacement for the if ...
The shorthand assignment operator += can also be used to concatenate strings. For example, var mystring = 'alpha'; mystring += 'bet'; // evaluates to "alphabet" and assigns this value to mystring. Conditional (ternary) operator. The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.. Syntax of Conditional/Ternary Operator in C
However, the use of the ternary operator makes our code more readable and clean. Note: We should only use the ternary operator if the resulting statement is short. Nested Ternary Operators. It is also possible to use one ternary operator inside another ternary operator. It is called the nested ternary operator in C++.
While the ternary operator is a way of re-writing a classic if-else block, in a certain sense, it behaves like a function since it returns a value. Indeed, we can assign the result of this operation to a variable: my_var = a if condition else b. For example: x = "Is true" if True else "Is false" print(x) Is true.
Assign the ternary operator to a variable. In C programming, we can also assign the expression of the ternary operator to a variable. For example, Here, if the test condition is true, expression1 will be assigned to the variable. Otherwise, expression2 will be assigned. // create variables char operator = '+';
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
The second group of operators we're going to explore is the assignment operators. Assignment operators are used to assign a specific value to a variable. The basic assignment operator is marked by the equal = symbol, and you've already seen this operator in action before: let x = 5; After the basic assignment operator, there are 5 more ...
The conditional operator ?:, also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false, as the following example shows: C#. string GetWeatherDisplay(double tempInCelsius) => tempInCelsius < 20.0 ?
This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example: (a += b) can be written as (a = a + b) If initially value stored in a is 5. Then (a += 6) = 11. 3. "-=" This operator is combination of '-' and '=' operators.
Here's an example: +"200" // 20 - number +false // 0 - number representation +"hello" // NaN. As you can see here again, only one operand is required, which comes after the operator. I'll stop with these three examples. But know that there are more unary operators such as the increment ++, decrement ++, and Logical NOT ! operators, to name a few.
JavaScript Ternary Operator. JavaScript Ternary Operator (Conditional Operator) is a concise way to write a conditional (if-else) statement. Ternary Operator takes three operands i.e. condition, true value and false value. In this article, we are going to learn about Ternary Operator.
The ternary operator is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more concise — all in just one line of code. ... Can't use assignment statements: Each operand of the Python ternary operator is an expression, not a statement, that means we can't use assignment ...
Unary operators work on one operand, binary operators work on two operands, and ternary operators utilise three operands to carry out the task. Java's operators include: Assignment: This binary operator uses the symbol = to assign the value of the operand on the right of an expression to the one on the left.
1. For those interested in the ternary operator (also called a conditional expression ), here is a way to use it to accomplish half of the original goal: q = d [x] if x in d else {} The conditional expression, of the form x if C else y, will evaluate and return the value of either x or y depending on the condition C.