If else statement
Welcome to tutorial number 8 of our Golang tutorial series .
if is a statement that has a boolean condition and it executes a block of code if that condition evaluates to true . It executes an alternate else block if the condition evaluates to false . In this tutorial, we will look at the various syntaxes and ways of using if statement.
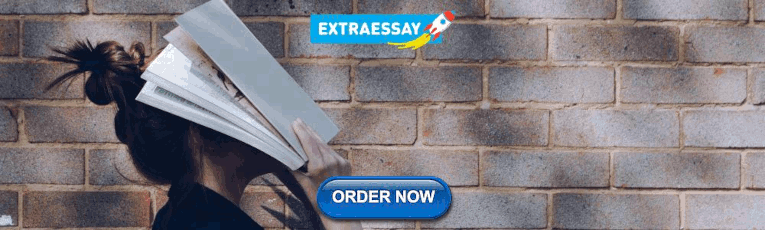
If statement syntax
The syntax of the if statement is provided below
If the condition is true, the lines of code between the braces { and } is executed.
Unlike in other languages like C, the braces { } are mandatory even if there is only one line of code between the braces { } .
Let’s write a simple program to find out whether a number is even or odd.
Run in Playground
In the above program, the condition num%2 in line no. 9 finds whether the remainder of dividing num by 2 is zero or not. Since it is 0 in this case, the text The number 10 is even is printed and the program returns.
The if statement has an optional else construct which will be executed if the condition in the if statement evaluates to false .
In the above snippet, if condition evaluates to false , then the lines of code between else { and } will be executed.
Let’s rewrite the program to find whether the number is odd or even using if else statement.
Run in playground
In the above code, instead of returning if the condition is true as we did in the previous section , we create an else statement that will be executed if the condition is false . In this case, since 11 is odd, the if condition is false and the lines of code within the else statement is executed. The above program will print.
If … else if … else statement
The if statement also has optional else if and else components. The syntax for the same is provided below
The condition is evaluated for the truth from the top to bottom.
In the above statement if condition1 is true, then the lines of code within if condition1 { and the closing brace } are executed.
If condition1 is false and condition2 is true, then the lines of code within else if condition2 { and the next closing brace } is executed.
If both condition1 and condition2 are false, then the lines of code in the else statement between else { and } are executed.
There can be any number of else if statements.
In general, whichever if or else if ’s condition evaluates to true , it’s corresponding code block is executed. If none of the conditions are true then else block is executed.
Let’s write a program that uses else if .
In the above program, the condition else if num >= 51 && num <= 100 in line no. 11 is true and hence the program will print
If with assignment
There is one more variant of if which includes an optional shorthand assignment statement that is executed before the condition is evaluated. Its syntax is
In the above snippet, assignment-statement is first executed before the condition is evaluated.
Let’s rewrite the program which finds whether the number is even or odd using the above syntax.
In the above program num is initialized in the if statement in line no. 8. One thing to be noted is that num is available only for access from inside the if and else . i.e. the scope of num is limited to the if else blocks. If we try to access num from outside the if or else , the compiler will complain. This syntax often comes in handy when we declare a variable just for the purpose of if else construct. Using this syntax in such cases ensures that the scope of the variable is only within the if else statement.
The else statement should start in the same line after the closing curly brace } of the if statement. If not the compiler will complain.
Let’s understand this by means of a program.
In the program above, the else statement does not start in the same line after the closing } of the if statement in line no. 11. Instead, it starts in the next line. This is not allowed in Go. If you run this program, the compiler will output the error,
The reason is because of the way Go inserts semicolons automatically. You can read about the semicolon insertion rule here https://golang.org/ref/spec#Semicolons .
In the rules, it’s specified that a semicolon will be inserted after closing brace } , if that is the final token of the line. So a semicolon is automatically inserted after the if statement’s closing braces } in line no. 11 by the Go compiler.
So our program actually becomes
after semicolon insertion. The compiler would have inserted a semicolon in line no. 4 of the above snippet.
Since if{...} else {...} is one single statement, a semicolon should not be present in the middle of it. Hence this program fails to compile. Therefore it is a syntactical requirement to place the else in the same line after the if statement’s closing brace } .
I have rewritten the program by moving the else after the closing } of the if statement to prevent the automatic semicolon insertion.
Now the compiler will be happy and so are we đ.
Idiomatic Go
We have seen various if-else constructs and we have in fact seen multiple ways to write the same program. For example, we have seen multiple ways to write a program that checks whether the number is even or odd using different if else constructs. Which one is the idiomatic way of coding in Go? In Go’s philosophy, it is better to avoid unnecessary branches and indentation of code. It is also considered better to return as early as possible. I have provided the program from the previous section below,
The idiomatic way of writing the above program in Go’s philosophy is to avoid the else and return from the if if the condition is true.
In the above program, as soon as we find out the number is even, we return immediately. This avoids the unnecessary else code branch. This is the way things are done in Go đ. Please keep this in mind whenever writing Go programs.
I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter or LinkedIn . Have a good day.
If you would like to advertise on this website, hire me, or if you have any other software development needs please email me at naveen[at]golangbot[dot]com .
Next tutorial - Loops
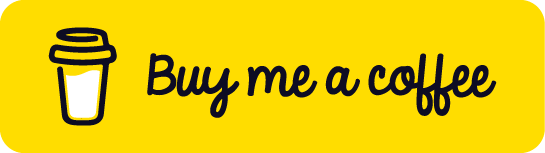
Go if..else, if..else..if, nested if with Best Practices
Introduction.
In computer programming, conditional statements help you make decisions based on a given condition. The conditional statement evaluates if a condition is true or false, therefore it is worth noting that if statements work with boolean values. Just like other programming languages, Go has its own construct for conditional statements. In this tutorial , we will learn about different types of conditional statements in Go.
Below are the topics that we will cover
- If statement
- If - Else statement
- If -else if else statement
- Nested If statement
- Logical operators AND , OR and NOT
- Using multiple conditions if if else
Supported Comparison Operators in GO
Before we go ahead learning about golang if else statement, let us be familiar with supported comparison operators as you may need to use them in the if else conditions for decision making:
if statement
If a statement is used to specify a block of code that should be executed if a certain condition is true .
Explanation
In the above syntax, the keyword if is used to declare the beginning of an if statement, followed by a condition that is being tested. The condition should be a value of boolean type , the the block of code between opening and closing curly brackets will be executed only if the condition is true . In the next example, we define code that will only run if age is above 18 years.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to true because 19 is greater than 18. The code block will be executed because the condition is true .
One liner if statement
We can also convert our if condition to run in one line, for example here I have written the same if condition in 2 different ways, one is multi line while the other is using single line:
if else Statement
If Else statement is used to evaluate both conditions that might be true or false. In the previous example, we did not really care if a condition evaluates to false. We use If Else conditional statements to handle both true and false outcomes.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to false because 12 is less than 18. The second code block will be executed because the condition is false .
if else if statement
If Else If statement is used to specify a new condition if the first condition is false. When using If Else If statement, you can nest as many If Else conditions as you desire.
In the above example, the first if statement evaluates to false because age (17) is not greater than ageLimit(18) . We then check again if age is equal to 17, which evaluates to true . The block of code inside this section will be executed.
Nested if Statement
Nested if Statement, is a If conditional statement that has an If statement inside another If statement. The nested If statement will always be executed only if the outer If statement evaluates to true.
In the above syntax, the second if statement with condition labeled conditionY is housed inside the outer If statement with condition labeled conditionX . As long as condition labeled conditionX is true , if conditionY will be executed.
Supported Logical Operators in GO
We also have some logical operators on golang which help us when we have to check for multiple conditions:
if else statements using logical operator
In Go we use logical operators together with If conditional statements to achieve certain goals in our code. Logical operators return/execute to boolean values, hence making more sense using them conditional statements. Below are definitions of the three logical operators in Go.
Using logical AND operator
Checks of both operands are none zero. In case both operands are none-zero, a true value will be returned, else a false value will be returned. The operator representing logical AND is && .
Using logical OR operator
Check if one operand is true. In case one operand is true code will be executed. The operator representing logical OR is || .
Using logical NOT operator
Reverses the logical state of its operands. If a condition is true, the logical operator NOT will change it to false. The operator representing logical Not is ! .
Using multiple condition in golang if else statements
We can also add multiple conditional blocks inside the if else statements to further enhance the decision making. Let us check some more examples covering if else multiple condition:
if statement with 2 logical OR Operator
Here we are checking if our num is more than 100 or less than 900:
if statement with 3 logical OR Operator
In this example we add one more OR condition to the if condition:
Using both logical AND and OR operator in single if statement
We can also use both AND and OR operator inside a single if statement, here is an example:
Using multiple conditions with if..else..if..else statement
Now we have used multiple conditions in our if condition but you can use the same inside else..if condition or combine it with if..else..if statements.
In Go, we use conditional statements to handle decisions precisely in code. They enable our code to perform different computations or actions depending on whether a condition is true or false . In this article we have learned about different if conditional statements like If, If Else, If Else If Else, Nested If statements
https://go.dev/tour/flowcontrol https://www.golangprograms.com/golang-if-else-statements.html
Related Keywords: golang if else shorthand, golang if else one line, golang if else multiple conditions, golang if else string, golang if true, golang if else best practices, golang if statement with assignment, golang if not
Antony Shikubu
He is highly skilled software developer with expertise in Python, Golang, and AWS cloud services. Skilled in building scalable solutions, he specializes in Django, Flask, Pandas, and NumPy for web apps and data processing, ensuring robust and maintainable code for diverse projects. You can connect with him on Linkedin .
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.

For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
Golang If Statement
Switch to English
Introduction As an online educator for GoLang, a statically typed, compiled language that was created at Google, I'm here to simplify the concept of the If statement. The if statement is a fundamental part of GoLang that allows your programs to make decisions based on certain conditions. It's a powerful feature that can greatly increase the versatility of your programs and scripts.
- Understanding the GoLang If Statement
Table of Contents
Using the If Statement Correctly
Golang if-else statement, golang if-elseif-else statement, common errors and how to avoid them.
- The if statement in GoLang is used for decision making. It allows your program to execute a particular section of code if a specific condition is true. If the condition is false, the program will skip that section of code and move on to the next part.
- When using the if statement, you should always ensure that your condition is valid and that it will return either true or false. If your condition does not return a boolean value, you will get a compilation error.
- In addition to the if statement, GoLang also has an if-else statement. This allows you to specify an alternate section of code to be executed if the condition in the if statement is false.
- For more complex decision making, GoLang provides the if-elseif-else statement. This allows you to specify multiple conditions and execute different sections of code based on which condition is true.
- One common error is forgetting to use braces {} to enclose the code block that should be executed if the condition is true. Unlike some other programming languages, GoLang requires these braces even if there's only one line of code in the block.
- Another common error is incorrect use of the equality operator (==). Remember that in GoLang, as in most programming languages, a single equals sign (=) is used for assignment, while a double equals sign (==) is used for comparison.
- Also, keep in mind that GoLang is case sensitive. This means that the variables 'a' and 'A' are considered to be different. So, always ensure that you're using the correct case when referencing variables in your conditions.
- .NET Framework
- C# Data Types
- C# Keywords
- C# Decision Making
- C# Delegates
- C# Constructors
- C# ArrayList
- C# Indexers
- C# Interface
- C# Multithreading
- C# Exception
- Solve Coding Problems
- Go Programming Language (Introduction)
- How to Install Go on Windows?
- How to Install Golang on MacOS?
- Hello World in Golang
Fundamentals
- Identifiers in Go Language
- Go Keywords
- Data Types in Go
- Go Variables
- Constants- Go Language
- Go Operators
Control Statements
Go decision making (if, if-else, nested-if, if-else-if).
- Loops in Go Language
- Switch Statement in Go
Functions & Methods
- Functions in Go Language
- Variadic Functions in Go
- Anonymous function in Go Language
- main and init function in Golang
- What is Blank Identifier(underscore) in Golang?
- Defer Keyword in Golang
- Methods in Golang
- Structures in Golang
- Nested Structure in Golang
- Anonymous Structure and Field in Golang
- Arrays in Go
- How to Copy an Array into Another Array in Golang?
- How to pass an Array to a Function in Golang?
- Slices in Golang
- Slice Composite Literal in Go
- How to sort a slice of ints in Golang?
- How to trim a slice of bytes in Golang?
- How to split a slice of bytes in Golang?
- Strings in Golang
- How to Trim a String in Golang?
- How to Split a String in Golang?
- Different ways to compare Strings in Golang
- Pointers in Golang
- Passing Pointers to a Function in Go
- Pointer to a Struct in Golang
- Go Pointer to Pointer (Double Pointer)
- Comparing Pointers in Golang
Concurrency
- Goroutines - Concurrency in Golang
- Select Statement in Go Language
- Multiple Goroutines
- Channel in Golang
- Unidirectional Channel in Golang
Decision making in programming is similar to decision making in real life. In decision making, a piece of code is executed when the given condition is fulfilled. Sometimes these are also termed as the Control flow statements. Golang uses control statements to control the flow of execution of the program based on certain conditions. These are used to cause the flow of execution to advance and branch based on changes to the state of a program. The Decision making statements of Go programming are:
if statement
This is the most simple decision-making statement. It is used to decide whether a certain statement or block of statements will be executed or not i.e if a certain condition is true then a block of statement is executed otherwise not. Syntax:
Flow Chart:
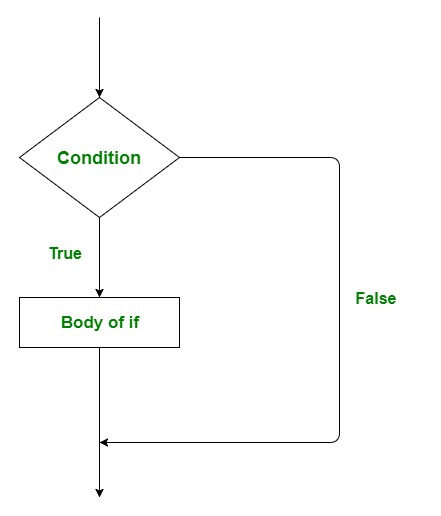
Example:
Output:
Time Complexity: O(1) Auxiliary Space: O(1)
if…else Statement
The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it wonât. But what if we want to do something else if the condition is false. Here comes the else statement. We can use the else statement with if statement to execute a block of code when the condition is false. Syntax:
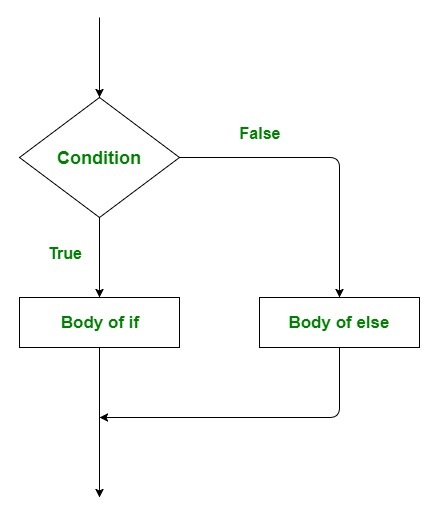
Output:
Time Complexity: O(1) Auxiliary Space: O(1)
Nested if Statement
In Go Language, a nested if is an if statement that is the target of another if or else. Nested if statements mean an if statement inside an if statement. Yes, Golang allows us to nest if statements within if statements. i.e, we can place an if statement inside another if statement. Syntax:
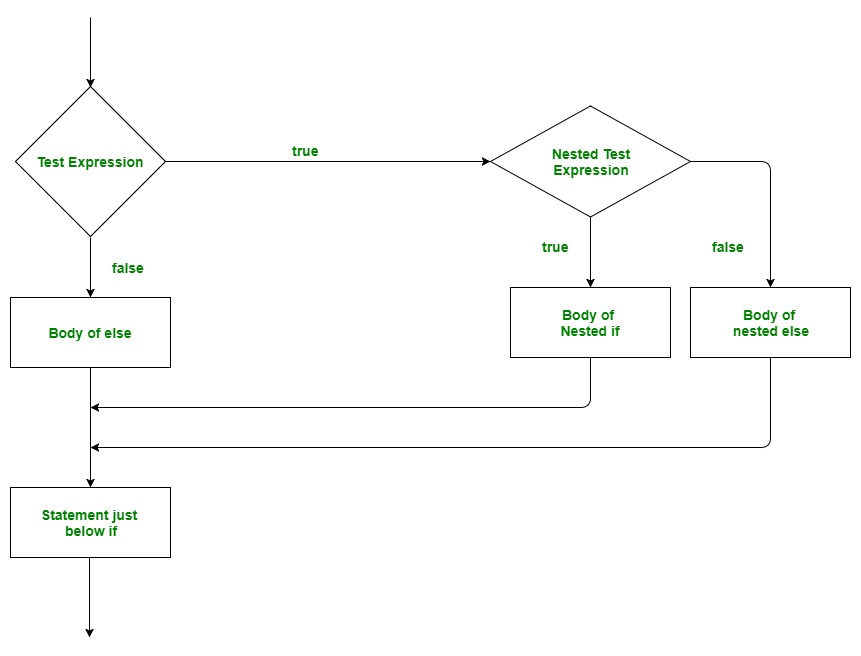
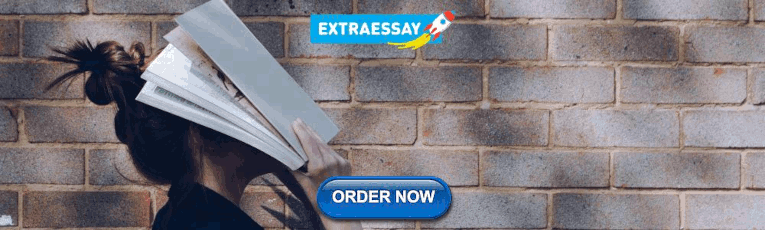
if..else..if ladder
Here, a user can decide among multiple options. The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed. Important Points:
- if statement can have zero or one else’s and it must come after any else if’s.
- if statement can have zero to many else if’s and it must come before the else.
- None of the remaining else if’s or else’s will be tested if an else if succeeds,
Syntax:
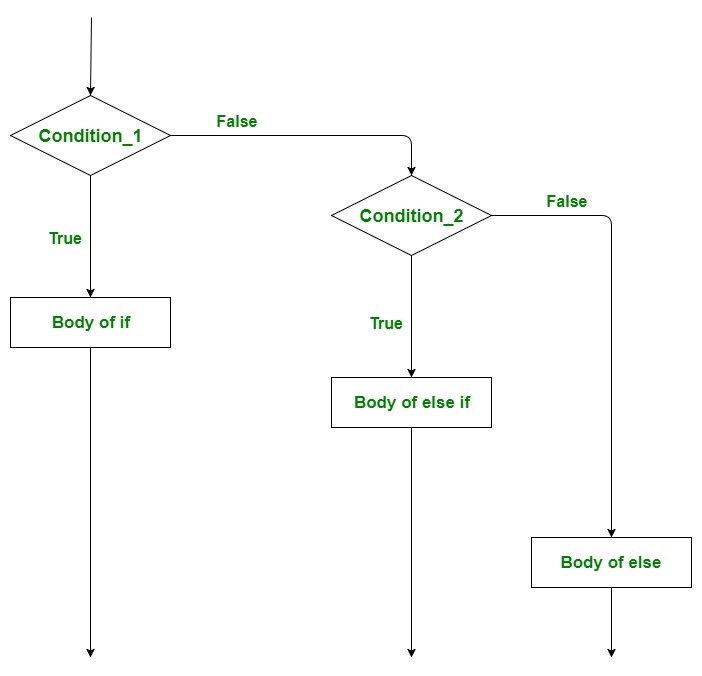
Time Complexity: O(1) Auxiliary Space: O(1)
Please Login to comment...
- Node.js 21 is here: Whatâs new
- Zoom: Worldâs Most Innovative Companies of 2024
- 10 Best Skillshare Alternatives in 2024
- 10 Best Task Management Apps for Android in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Go If-else Statements
The if statement in Go lang is a decision-making statement that controls the execution flow of the program based on the boolean condition. Here, you will learn about if, elseif, else, and nested if-else statements in Go lang.
if Statement
The if statement contains a boolean condition followed by a single or multi-line code block to be executed. At runtime, if a boolean condition evaluates to true, then the code block will be executed, otherwise not.
The following example demonstrates the if statements.
Above, the first if statement will be executed because the condition x < y evalutes to true. The code block of the second if statement will not be executed because the condition x > y evalutes to false.
Note that the condition expression must return a boolean value, true or false; otherwise compiler will give an error.
The starting code block brace { must be specified after the if clause. Go compiler will throw an error if specified in the new line after the if clause.
The boolean condition can be specified without the parentheses ( ) but the braces { } are required, as shown below.
Like for loop, the if statement can start with a statement to execute before the condition where you can declare a variable whose scope would be limited to the code block until the end of the if.
else Clause
Use the else clause after the if statement to execute the code block when the if condition evaluates to false. The else clause must come after the if block. Only one else block is allowed after the if statement.
The following demonstrates the if else statement.
The else keyword must come after the closing brace } ; otherwise the compiler will give an error.
Although, the starting brace of else clause can be specified in the new line.
else if statement
Use if clause after the else clause to execute a code block based on a condition when the first if condition evalutes to false. You can use multiple else-if statements between the if clause and else clause.
In the above example, temp < 15 is false, so the compiler will check next else if condition temp < 30 , which is true. So, it will execute the else if code block only.
You can have multiple else-if blocks where only one else-if block will be executed whose condition evalutes to true.
Nested if Statements
Go supports if else statements inside another if , else , or else if code blocks. The nested if statements make the code more readable.
The following example demonstrates the nested if condition.
.NET Tutorials
Database tutorials, javascript tutorials, programming tutorials.
Golang Control Flow Statements: If, Switch and For
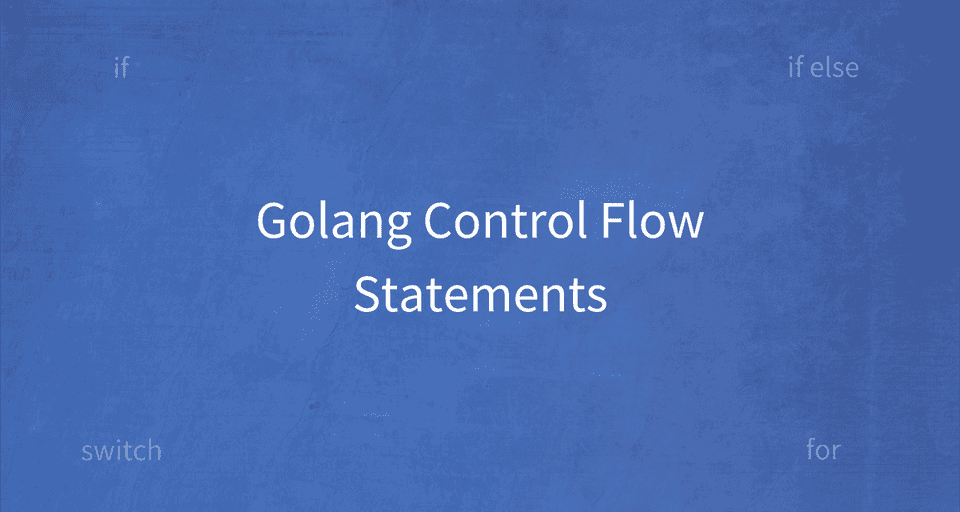
If Statement
If statements are used to specify whether a block of code should be executed or not depending on a given condition.
Following is the syntax of if statements in Golang -
Here is a simple example -
Note that , You can omit the parentheses () from an if statement in Golang, but the curly braces {} are mandatory -
You can combine multiple conditions using short circuit operators && and || like so -
If-Else Statement
An if statement can be combined with an else block. The else block is executed if the condition specified in the if statement is false -
If-Else-If Chain
if statements can also have multiple else if parts making a chain of conditions like this -
If with a short statement
An if statement in Golang can also contain a short declaration statement preceding the conditional expression -
The variable declared in the short statement is only available inside the if block and itâs else or else-if branches -
Note that, If youâre using a short statement, then you canât use parentheses. So the following code will generate a syntax error -
Switch Statement
A Switch statement takes an expression and matches it against a list of possible cases. Once a match is found, it executes the block of code specified in the matched case.
Here is a simple example of switch statement -
Go evaluates all the switch cases one by one from top to bottom until a case succeeds. Once a case succeeds, it runs the block of code specified in that case and then stops (it doesnât evaluate any further cases).
This is contrary to other languages like C, C++, and Java, where you explicitly need to insert a break statement after the body of every case to stop the evaluation of cases that follow.
If none of the cases succeed, then the default case is executed.
Switch with a short statement
Just like if , switch can also contain a short declaration statement preceding the conditional expression. So you could also write the previous switch example like this -
The only difference is that the variable declared by the short statement ( dayOfWeek ) is only available inside the switch block.
Combining multiple Switch cases
You can combine multiple switch cases into one like so -
This comes handy when you need to run a common logic for multiple cases.
Switch with no expression
In Golang, the expression that we specify in the switch statement is optional. A switch statement without an expression is same as switch true . It evaluates all the cases one by one, and runs the first case that evaluates to true -
Switch without an expression is simply a concise way of writing if-else-if chains.
A loop is used to run a block of code repeatedly. Golang has only one looping statement - the for loop.
Following is the generic syntax of for loop in Go -
The initialization statement is executed exactly once before the first iteration of the loop. In each iteration, the condition is checked. If the condition evaluates to true then the body of the loop is executed, otherwise, the loop terminates. The increment statement is executed at the end of every iteration.
Here is a simple example of a for loop -
Unlike other languages like C, C++, and Java, Goâs for loop doesnât contain parentheses, and the curly braces are mandatory.
Note that, both initialization and increment statements in the for loop are optional and can be omitted
Omitting the initialization statement
Omitting the increment statement
Note that, you can also omit the semicolons from the for loop in the above example and write it like this -
The above for loop is similar to a while loop in other languages. Go doesnât have a while loop because we can easily represent a while loop using for .
Finally, You can also omit the condition from the for loop in Golang. This will give you an infinite loop -
break statement
You can use break statement to break out of a loop before its normal termination. Here is an example -
continue statement
The continue statement is used to stop running the loop body midway and continue to the next iteration of the loop.
Thatâs all folks. In this article, you learned how to work with control flow statements like if , switch and for in Golang.
In the next article, Youâll learn how to define functions in Go. See you in the next post!
Next Article: Introduction to Functions in Go
Code Samples: github.com/callicoder/golang-tutorials
Share on social media
- Getting started with Go
- Awesome Book
- Awesome Community
- Awesome Course
- Awesome Tutorial
- Awesome YouTube
- Base64 Encoding
- Best practices on project structure
- Build Constraints
- Concurrency
- Console I/O
- Cross Compilation
- Cryptography
- Developing for Multiple Platforms with Conditional Compiling
- Error Handling
- Executing Commands
- Getting Started With Go Using Atom
- HTTP Client
- HTTP Server
- Inline Expansion
- Installation
- JWT Authorization in Go
- Memory pooling
- Object Oriented Programming
- Panic and Recover
- Parsing Command Line Arguments And Flags
- Parsing CSV files
- Profiling using go tool pprof
- Protobuf in Go
- Select and Channels
- Send/receive emails
- Text + HTML Templating
- The Go Command
- Type conversions
- Basic Variable Declaration
- Blank Identifier
- Checking a variable's type
- Multiple Variable Assignment
- Worker Pools
- Zero values
Go Variables Multiple Variable Assignment
In Go, you can declare multiple variables at the same time.
If a function returns multiple values, you can also assign values to variables based on the function's return values.
Got any Go Question?

- Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks.
Conditional if else statement in Go (Examples)
- Jan 28, 2024

This tutorials shows examples and syntaxes for if and else statements in Golang.
# Golang Conditional if statements
Like many programming languages, Go Language provides control structures for decision-making. If and else statements are one of type. If statements use to execute a piece of code based on conditional expressions. If the condition is satisfied, the code block is executed.
if and else in golang are reserved words.
This blog post covers examples and syntaxes for the following things.
- Simple if statements
- If shorthand statements
- if-else statements
- if-else nested statements
Here is the Syntax for if statements in Golang
Conditional Expression is evaluated and always results in in true or false .
The expression can be made of simple boolean values or complex expressions created using comparison operators == , = , or != .
The expression can be single or multiple .
Multiple expressions are joined with logical operators like &&,||,! operators. Conditional Expressions are not required to be enclosed with parentheses,
But the code block must be enclosed with curly braces. In golang, curly braces are required for code block which contains sing statements.
# Simple if statement in Golang
This example evaluates the expression if the expression is true, the code block is executed and returns âEvent Number test passedâ to the console. Below is an example of a simple if-conditional expression.
The output of the above code:
Letâs see an error case in the if-else block example.
Below the example, the code gives a compilation error.
The reason is if the statements code block has no curly braces, It throws syntax error: unexpected newline, expecting { after if clause
Multiple expressions combine with logical operators.
# If with shorthand statementsâ syntax in Golang
If statement always contains the shorthand statements , before going control to the conditional expression.
shorthand statements can contain variable declaration and the scope of the variable is limited to the scope of this block only.
If you include shorthand syntax in if statements, the parenthesis is not required.
It gives compilation error - syntax error: unexpected:=, expecting ) if the parenthesis is in shorthand syntax.
# If-else statement in Golang
This syntax contains an else block for the if statement. If the conditional expression is true if the block is executed, otherwise else block is executed.
Example for the if-else statement
the output of the above program is
# multiple if-else - nested if statements in Golang
if the statement contains if-else statements that contain chains of if-else statements
The output of the above program is
Compilation error if any line breaks before else statement as like below example the below program gives syntax error: unexpected else, expecting }
# Conclusion
In this tutorial, You learned conditional flow if-else blocks in Golang for example.
Go by Example : Multiple Return Values
Next example: Variadic Functions .
by Mark McGranaghan and Eli Bendersky | source | license
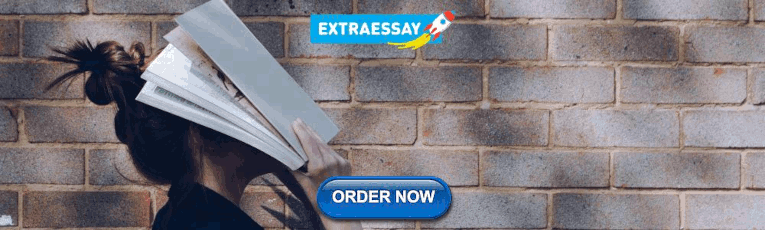
IMAGES
VIDEO
COMMENTS
No. Only one 'simple statement' is permitted at the beginning of an if-statement, per the spec. The recommended approach is multiple tests which might return an error, so I think you want something like: genUri := buildUri() if err := setRedisIdentity(genUri, email); err != nil {. return "", err.
Welcome to tutorial number 8 of our Golang tutorial series. if is a statement that has a boolean condition and it executes a block of code if that condition evaluates to true. It executes an alternate else block if the condition evaluates to false. In this tutorial, we will look at the various syntaxes and ways of using if statement. If ...
Using multiple condition in golang if else statements. We can also add multiple conditional blocks inside the if else statements to further enhance the decision making. Let us check some more examples covering if else multiple condition: if statement with 2 logical OR Operator. Here we are checking if our num is more than 100 or less than 900:
Often refereed to as Golang because of the domain name, this language also has the If/else conditions. Usually the If/else/else if condition when written with one condition makes the program lengthy and increases the complexity thus we can combine two conditions.
If Statements. We will start with the if statement, which will evaluate whether a statement is true or false, and run code only in the case that the statement is true. In a plain text editor, open a file and write the following code: import "fmt" func main() {. grade := 70 if grade >= 65 {.
The article provides a detailed explanation on using if, if-else, and if-elseif-else statements in Golang, along with common errors and how to avoid them. ... This allows you to specify multiple conditions and execute different sections of code based on which condition is true. ... equality operator (==). Remember that in GoLang, as in most ...
Nested if Statement. In Go Language, a nested if is an if statement that is the target of another if or else. Nested if statements mean an if statement inside an if statement. Yes, Golang allows us to nest if statements within if statements. i.e, we can place an if statement inside another if statement. Syntax:
The if statement in Go lang is a decision-making statement that controls the execution flow of the program based on the boolean condition. Here, you will learn about if, elseif, else, and nested if-else statements in Go lang. if Statement. The if statement contains a boolean condition followed by a single or multi-line code block to be executed.
Sometimes they are required, either because only a single statement is available (e.g. in an if statement) or because the values will change after the statement (e.g. in the case of swap). All values on the right-hand side of the assignment operator are evaluated before the assignment is performed.
This comes handy when you need to run a common logic for multiple cases. Switch with no expression. In Golang, the expression that we specify in the switch statement is optional. A switch statement without an expression is same as switch true. It evaluates all the cases one by one, and runs the first case that evaluates to true -
Println (num, "has multiple digits")}} Note that you don't need parentheses around conditions in Go, but that the braces are required. $ go run if-else.go 7 is odd 8 is divisible by 4 either 8 or 7 are even 9 has 1 digit: There is no ternary if in Go, so you'll need to use a full if statement even for basic conditions. Next example: Switch.
After the execution of post statement, condition statement will be evaluated again. If condition returns true, code inside for loop will be executed again else for loop terminates. Let's get ...
Multiple Variable Assignment. Help us to keep this website almost Ad Free! It takes only 10 seconds of your time: > Step 1: Go view our video on YouTube: EF Core Bulk Extensions > Step 2: And Like the video. BONUS: You can also share it! Example. In Go, you can declare multiple variables at the same time.
Like many programming languages, Go Language provides control structures for decision-making. If and else statements are one of type. If statements use to execute a piece of code based on conditional expressions. If the condition is satisfied, the code block is executed. if and else in golang are reserved words. This blog post covers examples ...
If with a short statement. Like for, the if statement can start with a short statement to execute before the condition.. Variables declared by the statement are only in scope until the end of the if. (Try using v in the last return statement.) < 6/14 >
Here we use the 2 different return values from the call with multiple assignment. a, b:= vals fmt. Println (a) fmt. Println (b) ... _, c:= vals fmt. Println (c)} $ go run multiple-return-values.go 3 7 7: Accepting a variable number of arguments is another nice feature of Go functions; we'll look at this next. Next example: Variadic Functions.
Actually you may implement this with a single switch statement:. a, b, c, d, e, f := 1, 2, 3, 4, 5, 6 switch a { case b, c, d, e, f: fmt.Println("'a' matches another ...
Why can't I use := as part of the if statement? You can, but then the variables are defined within the scope of the if block. So, file is not defined outside of your if block. The same rule applies to definitions in for, switch, and similar blocks.
Short variable declarations. Inside a function, the := short assignment statement can be used in place of a var declaration with implicit type. Outside a function, every statement begins with a keyword ( var, func, and so on) and so the := construct is not available. c, python, java := true, false, "no!"