Python Assignment Operators: Explained With Examples
Table of Contents
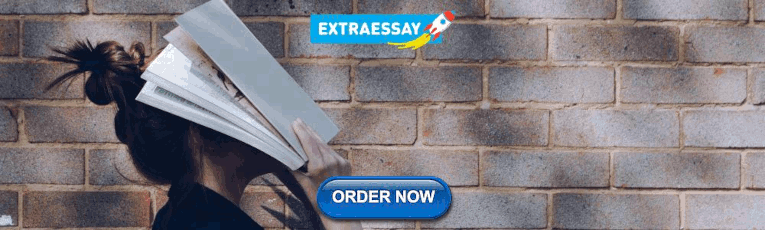
Assignment Operators in Python
1. basic assignment (=), 2. addition assignment (+=), 3. subtraction assignment (-=), 4. multiplication assignment (*=), 5. division assignment (/=), 6. modulus assignment (%=), 7. floor division assignment (//=), 8. exponent assignment (**=), 9. bitwise and assignment (&=), 10. bitwise or assignment (|=), 11. bitwise xor assignment (^=), 12. bitwise right shift assignment (>>=), 13. bitwise left shift assignment (<<=), simple savings calculator.
Python assignment operators play a crucial role by allocating a specific value to a variable. This operator is symbolized by the equals sign (=), marking its significance as one of the most frequently used operators in Python programming.
Python assignment operators facilitate the storage of a value in a variable by assigning the value on the right side of the operator to the variable on the left. This process is vital in programming because it enables developers to save data in variables for later use within their programs.
Examples of Python Assignment Operators
The basic assignment operator assigns the value on the right to the variable on the left. It’s a fundamental operation in Python.
Run the code using our Python Online Compiler .
This operator adds the right operand to the left operand and assigns the result back to the left operand.
Subtracts the right operand from the left and assigns the result to the left operand.
Multiplies the left operand with the right and assigns the result back to the left operand.
Divides the left operand by the right and assigns the quotient to the left operand.
0.5714285714285714
Applies modulus operation and assigns the remainder to the left operand.
Performs floor division and assigns the result to the left operand.
Raises the left operand to the power of the right operand and assigns the result back to the left.
Performs a bitwise AND operation and assigns the result to the left operand.
Applies a bitwise OR operation and assigns the result to the left operand.
Performs a bitwise XOR operation and updates the left operand with the result.
Shifts the left operand right by the number of bits specified by the right operand and assigns the result to the left.
Shifts the left operand left by the number of bits specified by the right operand and assigns the result back to the left.
Real-World Application Example of Python Assignment Operators
Imagine you want to calculate how much money you will have in your savings account after adding monthly deposits and applying annual interest. This simple calculator will:
- Start with an initial savings balance.
- Add a fixed monthly deposit amount.
- Apply annual interest at the end of the year.
Implementation:
Explanation:
- Initial and Monthly Savings: The program starts with an initial savings balance and simulates adding a fixed monthly deposit using the += operator, which adds the right operand to the left operand and assigns the result to the left operand.
- Applying Interest: At the end of each year, the program applies annual interest to the savings balance. This is done using the *= operator, which multiplies the left operand by the right operand and assigns the result to the left operand.
- Looping Through Years and Months: The program uses nested loops to iterate through each year and each month, updating the savings balance accordingly.
Related Posts:
- Python Variables: Explained with Examples
- Python Functions: Explained With Examples
- Python String format() Method: Explained With Examples
- Python Syntax: Explained with Examples
- Python Literals: Explained With Examples
- Python Regular Expression: Explained With Examples
- Python Numbers: Explained with Examples
- Python Operators: Explained With Examples
- Python Collections Module: Explained With Examples
- Python Built-in Modules: Explained With Examples
Gaurav Karwayun is the founder and executive editor of FullStackDive . He has done Computer Science Engineering and has over 12+ Years of Experience in the software industry. He has experience working in both service and product-based companies. He has vast experience in all popular programming languages, Web technologies, DevOps, etc. Follow him on LinkedIn and Twitter .
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
- Python Data Types
- Python Type Conversion
- Python I/O and Import
Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
- Python List
- Python Tuple
- Python String
- Python Dictionary
Python Files
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Precedence and Associativity of Operators in Python
Python Operator Overloading
Python if...else Statement
Python 3 Tutorial
- Python Strings
- Python any()
Operators are special symbols that perform operations on variables and values. For example,
Here, + is an operator that adds two numbers: 5 and 6 .
- Types of Python Operators
Here's a list of different types of Python operators that we will learn in this tutorial.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Special Operators
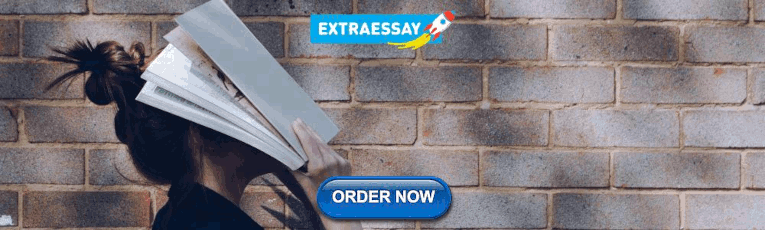
1. Python Arithmetic Operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc. For example,
Here, - is an arithmetic operator that subtracts two values or variables.
Example 1: Arithmetic Operators in Python
In the above example, we have used multiple arithmetic operators,
- + to add a and b
- - to subtract b from a
- * to multiply a and b
- / to divide a by b
- // to floor divide a by b
- % to get the remainder
- ** to get a to the power b
2. Python Assignment Operators
Assignment operators are used to assign values to variables. For example,
Here, = is an assignment operator that assigns 5 to x .
Here's a list of different assignment operators available in Python.
Example 2: Assignment Operators
Here, we have used the += operator to assign the sum of a and b to a .
Similarly, we can use any other assignment operators as per our needs.
3. Python Comparison Operators
Comparison operators compare two values/variables and return a boolean result: True or False . For example,
Here, the > comparison operator is used to compare whether a is greater than b or not.
Example 3: Comparison Operators
Note: Comparison operators are used in decision-making and loops . We'll discuss more of the comparison operator and decision-making in later tutorials.
4. Python Logical Operators
Logical operators are used to check whether an expression is True or False . They are used in decision-making. For example,
Here, and is the logical operator AND . Since both a > 2 and b >= 6 are True , the result is True .
Example 4: Logical Operators
Note : Here is the truth table for these logical operators.
5. Python Bitwise operators
Bitwise operators act on operands as if they were strings of binary digits. They operate bit by bit, hence the name.
For example, 2 is 10 in binary, and 7 is 111 .
In the table below: Let x = 10 ( 0000 1010 in binary) and y = 4 ( 0000 0100 in binary)
6. Python Special operators
Python language offers some special types of operators like the identity operator and the membership operator. They are described below with examples.
- Identity operators
In Python, is and is not are used to check if two values are located at the same memory location.
It's important to note that having two variables with equal values doesn't necessarily mean they are identical.
Example 4: Identity operators in Python
Here, we see that x1 and y1 are integers of the same values, so they are equal as well as identical. The same is the case with x2 and y2 (strings).
But x3 and y3 are lists. They are equal but not identical. It is because the interpreter locates them separately in memory, although they are equal.
- Membership operators
In Python, in and not in are the membership operators. They are used to test whether a value or variable is found in a sequence ( string , list , tuple , set and dictionary ).
In a dictionary, we can only test for the presence of a key, not the value.
Example 5: Membership operators in Python
Here, 'H' is in message , but 'hello' is not present in message (remember, Python is case-sensitive).
Similarly, 1 is key, and 'a' is the value in dictionary dict1 . Hence, 'a' in y returns False .
- Precedence and Associativity of operators in Python
Table of Contents
- Introduction
- Python Arithmetic Operators
- Python Assignment Operators
- Python Comparison Operators
- Python Logical Operators
- Python Bitwise operators
- Python Special operators
Video: Operators in Python
Sorry about that.
Related Tutorials
Python Tutorial
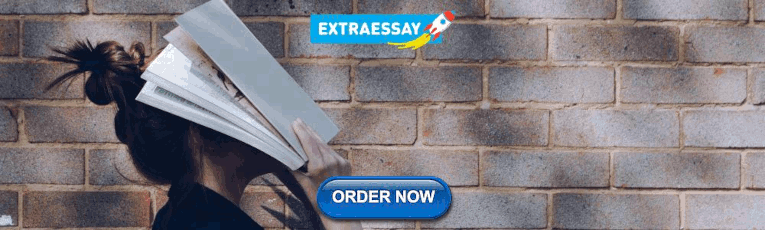
VIDEO
COMMENTS
The problem is that you’re using the assignment operator (=) instead of the equality comparison operator (==). In C, x = y is an expression that evaluates to the value of y. In this example, x = y is evaluated as 8, which is considered truthy in the context of the if statement. Take a look at a corresponding example in Python.
Assignment Operators in Python. Python assignment operators play a crucial role by allocating a specific value to a variable. This operator is symbolized by the equals sign (=), marking its significance as one of the most frequently used operators in Python programming.
Assignment operators are used to assign values to variables. For example, # assign 5 to x var x = 5. Here, = is an assignment operator that assigns 5 to x. Here's a list of different assignment operators available in Python.