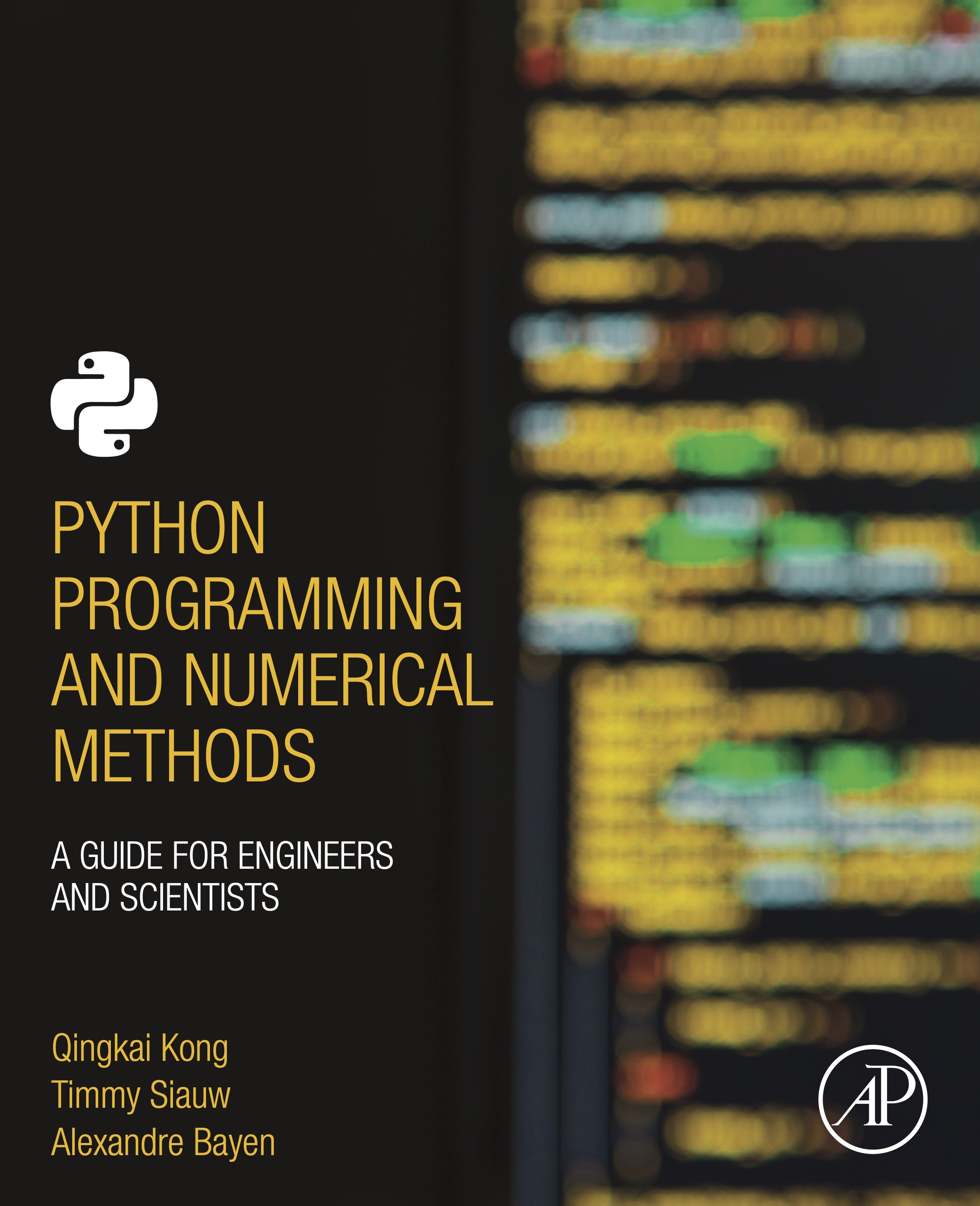
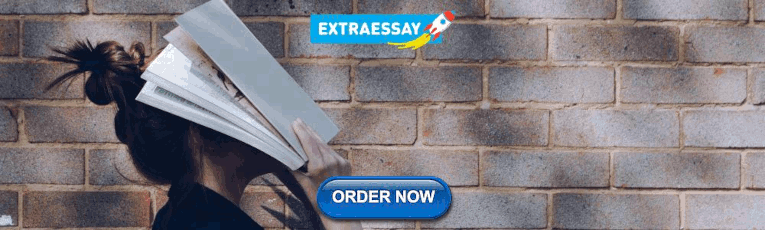
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
Python Variables
In Python, a variable is a container that stores a value. In other words, variable is the name given to a value, so that it becomes easy to refer a value later on.
Unlike C# or Java, it's not necessary to explicitly define a variable in Python before using it. Just assign a value to a variable using the = operator e.g. variable_name = value . That's it.
The following creates a variable with the integer value.
In the above example, we declared a variable named num and assigned an integer value 10 to it. Use the built-in print() function to display the value of a variable on the console or IDLE or REPL .
In the same way, the following declares variables with different types of values.
Multiple Variables Assignment
You can declare multiple variables and assign values to each variable in a single statement, as shown below.
In the above example, the first int value 10 will be assigned to the first variable x, the second value to the second variable y, and the third value to the third variable z. Assignment of values to variables must be in the same order in they declared.
You can also declare different types of values to variables in a single statement separated by a comma, as shown below.
Above, the variable x stores 10 , y stores a string 'Hello' , and z stores a boolean value True . The type of variables are based on the types of assigned value.
Assign a value to each individual variable separated by a comma will throw a syntax error, as shown below.
Variables in Python are objects. A variable is an object of a class based on the value it stores. Use the type() function to get the class name (type) of a variable.
In the above example, num is an object of the int class that contains integre value 10 . In the same way, amount is an object of the float class, greet is an object of the str class, isActive is an object of the bool class.
Unlike other programming languages like C# or Java, Python is a dynamically-typed language, which means you don't need to declare a type of a variable. The type will be assigned dynamically based on the assigned value.
The + operator sums up two int variables, whereas it concatenates two string type variables.
Object's Identity
Each object in Python has an id. It is the object's address in memory represented by an integer value. The id() function returns the id of the specified object where it is stored, as shown below.
Variables with the same value will have the same id.
Thus, Python optimize memory usage by not creating separate objects if they point to same value.
Naming Conventions
Any suitable identifier can be used as a name of a variable, based on the following rules:
- The name of the variable should start with either an alphabet letter (lower or upper case) or an underscore (_), but it cannot start with a digit.
- More than one alpha-numeric characters or underscores may follow.
- The variable name can consist of alphabet letter(s), number(s) and underscore(s) only. For example, myVar , MyVar , _myVar , MyVar123 are valid variable names, but m*var , my-var , 1myVar are invalid variable names.
- Variable names in Python are case sensitive. So, NAME , name , nAME , and nAmE are treated as different variable names.
- Variable names cannot be a reserved keywords in Python.
- Compare strings in Python
- Convert file data to list
- Convert User Input to a Number
- Convert String to Datetime in Python
- How to call external commands in Python?
- How to count the occurrences of a list item?
- How to flatten list in Python?
- How to merge dictionaries in Python?
- How to pass value by reference in Python?
- Remove duplicate items from list in Python
- More Python articles
- Python Questions & Answers
- Python Skill Test
- Python Latest Articles
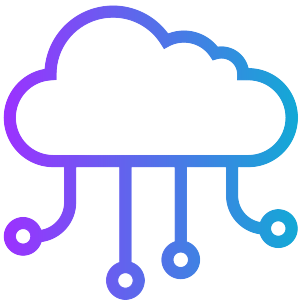
Leon Lovett
Python Variables – A Guide to Variable Assignment and Naming
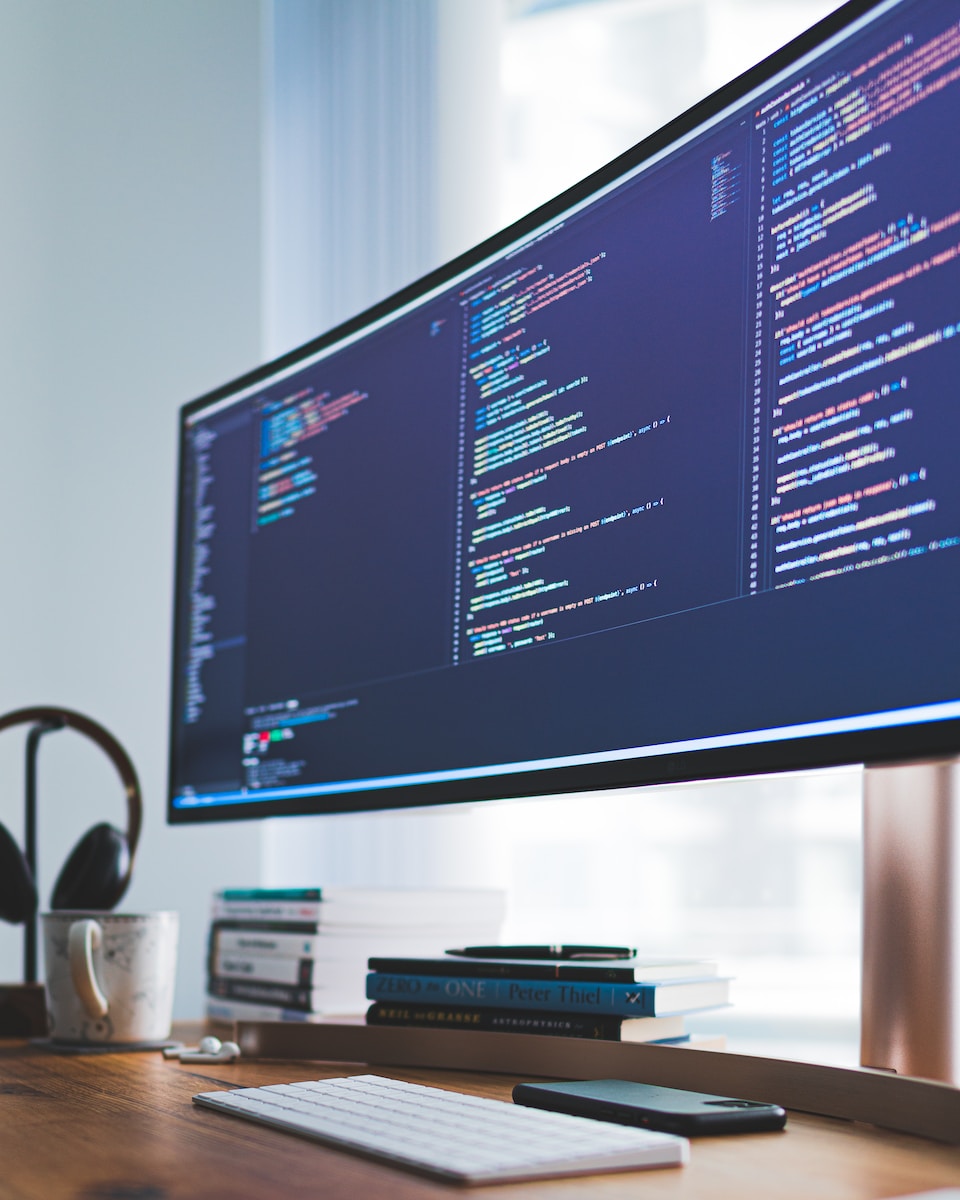
In Python, variables are essential elements that allow developers to store and manipulate data. When writing Python code, understanding variable assignment and naming conventions is crucial for effective programming.
Python variables provide a way to assign a name to a value and use that name to reference the value later in the code. Variables can be used to store various types of data, including numbers, strings, and lists.
In this article, we will explore the basics of Python variables, including variable assignment and naming conventions. We will also dive into the different variable types available in Python and how to use them effectively.
Key Takeaways
- Python variables are used to store and manipulate data in code.
- Variable assignment allows developers to assign a name to a value and reference it later.
- Proper variable naming conventions are essential for effective programming.
- Python supports different variable types, including integers, floats, strings, and lists.
Variable Assignment in Python
Python variables are created when a value is assigned to them using the equals sign (=) operator. For example, the following code snippet assigns the integer value 5 to the variable x :
From this point forward, whenever x is referenced in the code, it will have the value 5.
Variables can also be assigned using other variables or expressions. For example, the following code snippet assigns the value of x plus 2 to the variable y :
It is important to note that variables in Python are dynamically typed, meaning that their type can change as the program runs. For example, the following code snippet assigns a string value to the variable x , then later reassigns it to an integer value:
x = "hello" x = 7
Common Mistakes in Variable Assignment
One common mistake is trying to reference a variable before it has been assigned a value. This will result in a NameError being raised. For example:
print(variable_name) NameError: name ‘variable_name’ is not defined
Another common mistake is assigning a value to the wrong variable name. For example, the following code snippet assigns the value 5 to the variable y instead of x :
y = 5 print(x) NameError: name ‘x’ is not defined
To avoid these mistakes, it is important to carefully review code and double-check variable names and values.
Using Variables in Python
Variables are used extensively in Python code for a variety of purposes, from storing user input to performing complex calculations. The following code snippet demonstrates the basic usage of variables in a simple addition program:
number1 = input("Enter the first number: ") number2 = input("Enter the second number: ") sum = float(number1) + float(number2) print("The sum of", number1, "and", number2, "is", sum)
This program prompts the user to enter two numbers, converts them to floats using the float() function, adds them together, and prints the result using the print() function.
Variables can also be used in more complex operations, such as string concatenation and list manipulation. The following code snippet demonstrates how variables can be used to combine two strings:
greeting = "Hello" name = "Alice" message = greeting + ", " + name + "!" print(message)
This program defines two variables containing a greeting and a name, concatenates them using the plus (+) operator, and prints the result.
Variable Naming Conventions in Python
In Python, proper variable naming conventions are crucial for writing clear and maintainable code. Consistently following naming conventions makes code more readable and easier to understand, especially when working on large projects with many collaborators. Here are some commonly accepted conventions:
It’s recommended to use lowercase or snake case for variable names as they are easier to read and more commonly used in Python. Camel case is common in other programming languages, but can make Python code harder to read.
Variable names should be descriptive and meaningful. Avoid using abbreviations or single letters, unless they are commonly understood, like “i” for an iterative variable in a loop. Using descriptive names will make your code easier to understand and maintain by you and others.
Lastly, it’s a good practice to avoid naming variables with reserved words in Python such as “and”, “or”, and “not”. Using reserved words can cause errors in your code, making it hard to debug.
Scope of Variables in Python
Variables in Python have a scope, which dictates where they can be accessed and used within a code block. Understanding variable scope is important for writing efficient and effective code.
Local Variables in Python
A local variable is created within a particular code block, such as a function. It can only be accessed within that block and is destroyed when the block is exited. Local variables can be defined using the same Python variable assignment syntax as any other variable.
Example: def my_function(): x = 10 print(“Value inside function:”, x) my_function() print(“Value outside function:”, x) Output: Value inside function: 10 NameError: name ‘x’ is not defined
In the above example, the variable ‘x’ is a local variable that is defined within the function ‘my_function()’. It cannot be accessed outside of that function, which is why the second print statement results in an error.
Global Variables in Python
A global variable is a variable that can be accessed from anywhere within a program. These variables are typically defined outside of any code block, at the top level of the program. They can be accessed and modified from any code block within the program.
Example: x = 10 def my_function(): print(“Value inside function:”, x) my_function() print(“Value outside function:”, x) Output: Value inside function: 10 Value outside function: 10
In the above example, the variable ‘x’ is a global variable that is defined outside of any function. It can be accessed from within the ‘my_function()’ as well as from outside it.
When defining a function, it is possible to access and modify a global variable from within the function using the ‘global’ keyword.
Example: x = 10 def my_function(): global x x = 20 my_function() print(x) Output: 20
In the above example, the ‘global’ keyword is used to indicate that the variable ‘x’ inside the function is the same as the global variable ‘x’. The function modifies the global variable, causing the final print statement to output ’20’ instead of ’10’.
One of the most fundamental concepts in programming is the use of variables. In Python, variables allow us to store and manipulate data efficiently. Here are some practical examples of how to use variables in Python:
Mathematical Calculations
Variables are often used to perform mathematical calculations in Python. For instance, we can assign numbers to variables and then perform operations on those variables. Here’s an example:
x = 5 y = 10 z = x + y print(z) # Output: 15
In this code, we have assigned the value 5 to the variable x and the value 10 to the variable y. We then create a new variable z by adding x and y together. Finally, we print the value of z, which is 15.
String Manipulation
Variables can also be used to manipulate strings in Python. Here is an example:
first_name = “John” last_name = “Doe” full_name = first_name + ” ” + last_name print(full_name) # Output: John Doe
In this code, we have assigned the strings “John” and “Doe” to the variables first_name and last_name respectively. We then create a new variable full_name by combining the values of first_name and last_name with a space in between. Finally, we print the value of full_name, which is “John Doe”.
Working with Data Structures
Variables are also essential when working with data structures such as lists and dictionaries in Python. Here’s an example:
numbers = [1, 2, 3, 4, 5] sum = 0 for num in numbers: sum += num print(sum) # Output: 15
In this code, we have assigned a list of numbers to the variable numbers. We then create a new variable sum and initialize it to 0. We use a for loop to iterate over each number in the list, adding it to the sum variable. Finally, we print the value of sum, which is 15.
As you can see, variables are an essential tool in Python programming. By using them effectively, you can manipulate data and perform complex operations with ease.
Variable Types in Python
Python is a dynamically typed language, which means that variables can be assigned values of different types without explicit type declaration. Python supports a wide range of variable types, each with its own unique characteristics and uses.
Numeric Types:
Python supports several numeric types, including integers, floats, and complex numbers. Integers are whole numbers without decimal points, while floats are numbers with decimal points. Complex numbers consist of a real and imaginary part, expressed as a+bi.
Sequence Types:
Python supports several sequence types, including strings, lists, tuples, and range objects. Strings are sequences of characters, while lists and tuples are sequences of values of any type. Range objects are used to represent sequences of numbers.
Mapping Types:
Python supports mapping types, which are used to store key-value pairs. The most commonly used mapping type is the dictionary, which supports efficient lookup of values based on their associated keys.
Boolean Type:
Python supports a Boolean type, which is used to represent truth values. The Boolean type has two possible values: True and False.
Python has a special value called None, which represents the absence of a value. This type is often used to indicate the result of functions that do not return a value.
Understanding the different variable types available in Python is essential for effective coding. Each type has its own unique properties and uses, and choosing the right type for a given task can help improve code clarity, efficiency, and maintainability.
Python variables are a fundamental concept that every aspiring Python programmer must understand. In this article, we have covered the basics of variable assignment and naming conventions in Python. We have also explored the scope of variables and their different types.
It is important to remember that variables play a crucial role in programming, and their effective use can make your code more efficient and easier to read. Proper naming conventions and good coding practices can also help prevent errors and improve maintainability.
As you continue to explore the vast possibilities of Python programming, we encourage you to practice using variables in your code. With a solid understanding of Python variables, you will be well on your way to becoming a proficient Python programmer.
Similar Posts
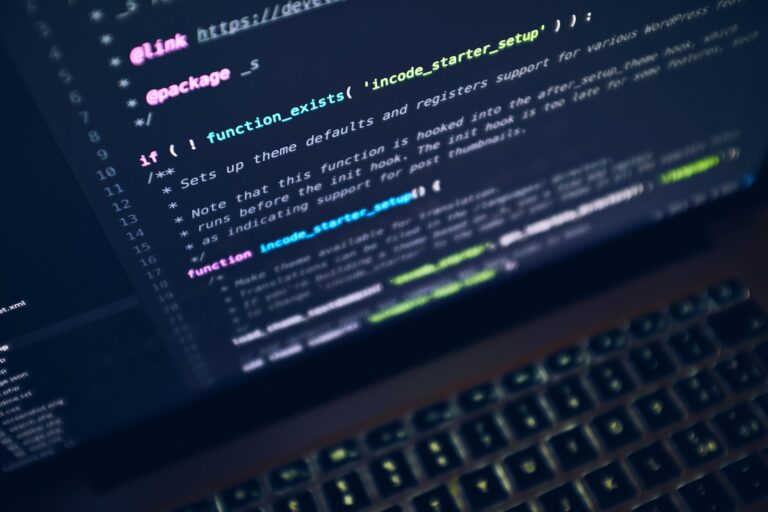
Exception Handling in Python – A Tutorial
Python is a powerful and versatile programming language used in a wide range of applications. However, even the most skilled Python developers encounter errors and bugs in their code. That’s where exception handling comes in – it is a crucial aspect of Python programming that helps prevent errors and ensure smooth code execution. Exception handling…
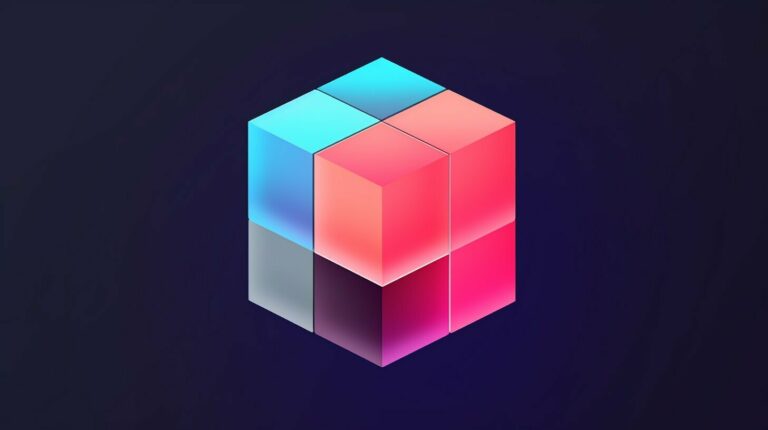
Object Oriented Programming in Python – A Beginner’s Guide
Python is a versatile programming language that offers support for object-oriented programming (OOP). OOP is a programming paradigm that provides a modular and reusable way to design software. In this article, we will provide a beginner’s guide to object-oriented programming in Python with a focus on Python classes. Python classes are the building blocks of…
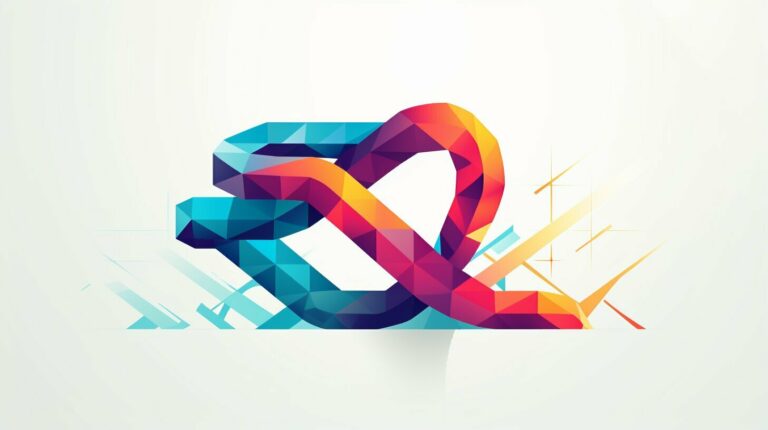
Introduction to Anonymous Functions in Python
Python is a popular language used widely for coding because of its simplicity and versatility. One of the most powerful aspects of Python is its ability to use lambda functions, also known as anonymous functions. In this article, we will explore the concept of anonymous functions in Python, and how they can help simplify your…
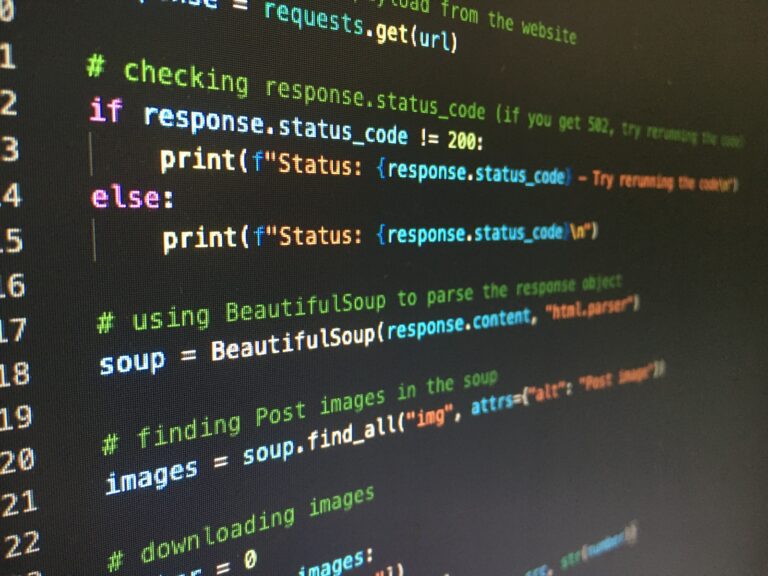
Yield and Generators in Python
Python generators are an essential part of modern Python programming. They allow for efficient memory usage and enable developers to iterate over large data sets in a streamlined manner. Python generator functions use the “yield” keyword to generate a sequence of values that can be iterated over. In this article, we will explore the benefits…
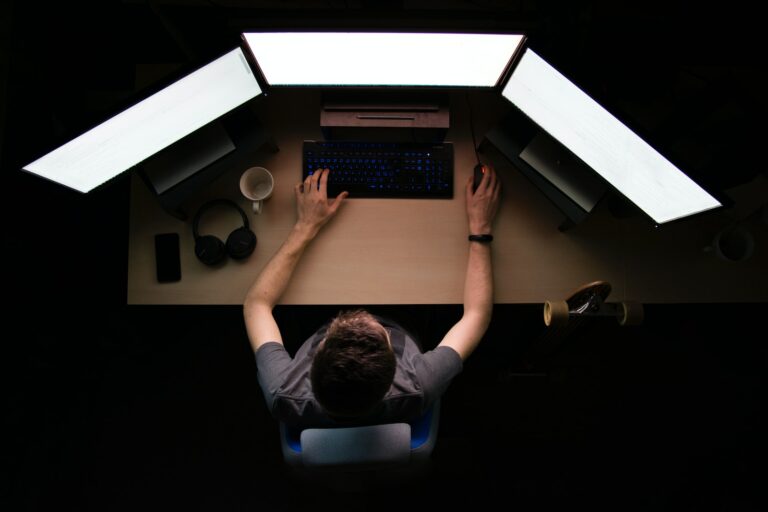
Understanding Scope and Namespace in Python
Python is a high-level programming language that provides powerful tools for developers to create efficient and effective code. Understanding the scope and namespace in Python is crucial for writing programs that work as intended. Python scope refers to the area of a program where a variable or function is accessible. This scope can be local…
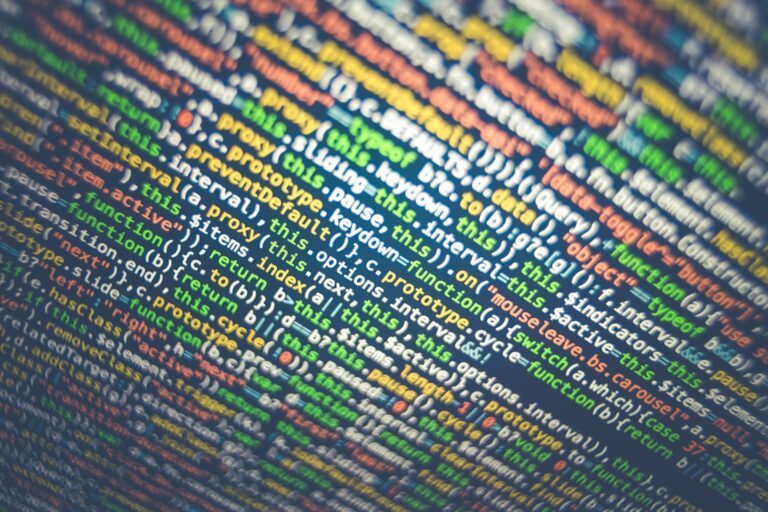
An Introduction to Python’s Core Data Types
Python is a widely used programming language that is renowned for its simplicity and accessibility. At the core of Python lies its data types, which are fundamental units that enable programmers to store and manipulate information. Understanding Python data types is essential for effective programming in the language. This article provides an introduction to Python’s…
- Hands-on Python Tutorial »
- 1. Beginning With Python »
1.6. Variables and Assignment ¶
Each set-off line in this section should be tried in the Shell.
Nothing is displayed by the interpreter after this entry, so it is not clear anything happened. Something has happened. This is an assignment statement , with a variable , width , on the left. A variable is a name for a value. An assignment statement associates a variable name on the left of the equal sign with the value of an expression calculated from the right of the equal sign. Enter
Once a variable is assigned a value, the variable can be used in place of that value. The response to the expression width is the same as if its value had been entered.
The interpreter does not print a value after an assignment statement because the value of the expression on the right is not lost. It can be recovered if you like, by entering the variable name and we did above.
Try each of the following lines:
The equal sign is an unfortunate choice of symbol for assignment, since Python’s usage is not the mathematical usage of the equal sign. If the symbol ↤ had appeared on keyboards in the early 1990’s, it would probably have been used for assignment instead of =, emphasizing the asymmetry of assignment. In mathematics an equation is an assertion that both sides of the equal sign are already, in fact, equal . A Python assignment statement forces the variable on the left hand side to become associated with the value of the expression on the right side. The difference from the mathematical usage can be illustrated. Try:
so this is not equivalent in Python to width = 10 . The left hand side must be a variable, to which the assignment is made. Reversed, we get a syntax error . Try
This is, of course, nonsensical as mathematics, but it makes perfectly good sense as an assignment, with the right-hand side calculated first. Can you figure out the value that is now associated with width? Check by entering
In the assignment statement, the expression on the right is evaluated first . At that point width was associated with its original value 10, so width + 5 had the value of 10 + 5 which is 15. That value was then assigned to the variable on the left ( width again) to give it a new value. We will modify the value of variables in a similar way routinely.
Assignment and variables work equally well with strings. Try:
Try entering:
Note the different form of the error message. The earlier errors in these tutorials were syntax errors: errors in translation of the instruction. In this last case the syntax was legal, so the interpreter went on to execute the instruction. Only then did it find the error described. There are no quotes around fred , so the interpreter assumed fred was an identifier, but the name fred was not defined at the time the line was executed.
It is both easy to forget quotes where you need them for a literal string and to mistakenly put them around a variable name that should not have them!
Try in the Shell :
There fred , without the quotes, makes sense.
There are more subtleties to assignment and the idea of a variable being a “name for” a value, but we will worry about them later, in Issues with Mutable Objects . They do not come up if our variables are just numbers and strings.
Autocompletion: A handy short cut. Idle remembers all the variables you have defined at any moment. This is handy when editing. Without pressing Enter, type into the Shell just
Assuming you are following on the earlier variable entries to the Shell, you should see f autocompleted to be
This is particularly useful if you have long identifiers! You can press Alt-/ several times if more than one identifier starts with the initial sequence of characters you typed. If you press Alt-/ again you should see fred . Backspace and edit so you have fi , and then and press Alt-/ again. You should not see fred this time, since it does not start with fi .
1.6.1. Literals and Identifiers ¶
Expressions like 27 or 'hello' are called literals , coming from the fact that they literally mean exactly what they say. They are distinguished from variables, whose value is not directly determined by their name.
The sequence of characters used to form a variable name (and names for other Python entities later) is called an identifier . It identifies a Python variable or other entity.
There are some restrictions on the character sequence that make up an identifier:
The characters must all be letters, digits, or underscores _ , and must start with a letter. In particular, punctuation and blanks are not allowed.
There are some words that are reserved for special use in Python. You may not use these words as your own identifiers. They are easy to recognize in Idle, because they are automatically colored orange. For the curious, you may read the full list:
There are also identifiers that are automatically defined in Python, and that you could redefine, but you probably should not unless you really know what you are doing! When you start the editor, we will see how Idle uses color to help you know what identifies are predefined.
Python is case sensitive: The identifiers last , LAST , and LaSt are all different. Be sure to be consistent. Using the Alt-/ auto-completion shortcut in Idle helps ensure you are consistent.
What is legal is distinct from what is conventional or good practice or recommended. Meaningful names for variables are important for the humans who are looking at programs, understanding them, and revising them. That sometimes means you would like to use a name that is more than one word long, like price at opening , but blanks are illegal! One poor option is just leaving out the blanks, like priceatopening . Then it may be hard to figure out where words split. Two practical options are
- underscore separated: putting underscores (which are legal) in place of the blanks, like price_at_opening .
- using camel-case : omitting spaces and using all lowercase, except capitalizing all words after the first, like priceAtOpening
Use the choice that fits your taste (or the taste or convention of the people you are working with).
Table Of Contents
- 1.6.1. Literals and Identifiers
Previous topic
1.5. Strings, Part I
1.7. Print Function, Part I
- Show Source
Quick search
Enter search terms or a module, class or function name.
- Module 2: The Essentials of Python »
- Variables & Assignment
- View page source
Variables & Assignment
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
Variables permit us to write code that is flexible and amendable to repurpose. Suppose we want to write code that logs a student’s grade on an exam. The logic behind this process should not depend on whether we are logging Brian’s score of 92% versus Ashley’s score of 94%. As such, we can utilize variables, say name and grade , to serve as placeholders for this information. In this subsection, we will demonstrate how to define variables in Python.
In Python, the = symbol represents the “assignment” operator. The variable goes to the left of = , and the object that is being assigned to the variable goes to the right:
Attempting to reverse the assignment order (e.g. 92 = name ) will result in a syntax error. When a variable is assigned an object (like a number or a string), it is common to say that the variable is a reference to that object. For example, the variable name references the string "Brian" . This means that, once a variable is assigned an object, it can be used elsewhere in your code as a reference to (or placeholder for) that object:
Valid Names for Variables
A variable name may consist of alphanumeric characters ( a-z , A-Z , 0-9 ) and the underscore symbol ( _ ); a valid name cannot begin with a numerical value.
var : valid
_var2 : valid
ApplePie_Yum_Yum : valid
2cool : invalid (begins with a numerical character)
I.am.the.best : invalid (contains . )
They also cannot conflict with character sequences that are reserved by the Python language. As such, the following cannot be used as variable names:
for , while , break , pass , continue
in , is , not
if , else , elif
def , class , return , yield , raises
import , from , as , with
try , except , finally
There are other unicode characters that are permitted as valid characters in a Python variable name, but it is not worthwhile to delve into those details here.
Mutable and Immutable Objects
The mutability of an object refers to its ability to have its state changed. A mutable object can have its state changed, whereas an immutable object cannot. For instance, a list is an example of a mutable object. Once formed, we are able to update the contents of a list - replacing, adding to, and removing its elements.
To spell out what is transpiring here, we:
Create (initialize) a list with the state [1, 2, 3] .
Assign this list to the variable x ; x is now a reference to that list.
Using our referencing variable, x , update element-0 of the list to store the integer -4 .
This does not create a new list object, rather it mutates our original list. This is why printing x in the console displays [-4, 2, 3] and not [1, 2, 3] .
A tuple is an example of an immutable object. Once formed, there is no mechanism by which one can change of the state of a tuple; and any code that appears to be updating a tuple is in fact creating an entirely new tuple.
Mutable & Immutable Types of Objects
The following are some common immutable and mutable objects in Python. These will be important to have in mind as we start to work with dictionaries and sets.
Some immutable objects
numbers (integers, floating-point numbers, complex numbers)
“frozen”-sets
Some mutable objects
dictionaries
NumPy arrays
Referencing a Mutable Object with Multiple Variables
It is possible to assign variables to other, existing variables. Doing so will cause the variables to reference the same object:
What this entails is that these common variables will reference the same instance of the list. Meaning that if the list changes, all of the variables referencing that list will reflect this change:
We can see that list2 is still assigned to reference the same, updated list as list1 :
In general, assigning a variable b to a variable a will cause the variables to reference the same object in the system’s memory, and assigning c to a or b will simply have a third variable reference this same object. Then any change (a.k.a mutation ) of the object will be reflected in all of the variables that reference it ( a , b , and c ).
Of course, assigning two variables to identical but distinct lists means that a change to one list will not affect the other:
Reading Comprehension: Does slicing a list produce a reference to that list?
Suppose x is assigned a list, and that y is assigned a “slice” of x . Do x and y reference the same list? That is, if you update part of the subsequence common to x and y , does that change show up in both of them? Write some simple code to investigate this.
Reading Comprehension: Understanding References
Based on our discussion of mutable and immutable objects, predict what the value of y will be in the following circumstance:
Reading Comprehension Exercise Solutions:
Does slicing a list produce a reference to that list?: Solution
Based on the following behavior, we can conclude that slicing a list does not produce a reference to the original list. Rather, slicing a list produces a copy of the appropriate subsequence of the list:
Understanding References: Solutions
Integers are immutable, thus x must reference an entirely new object ( 9 ), and y still references 3 .
Python Variables and Assignment
Python variables, variable assignment rules, every value has a type, memory and the garbage collector, variable swap, variable names are superficial labels, assignment = is shallow, decomp by var.
Python Variables – The Complete Beginner's Guide

Variables are an essential part of Python. They allow us to easily store, manipulate, and reference data throughout our projects.
This article will give you all the understanding of Python variables you need to use them effectively in your projects.
If you want the most convenient way to review all the topics covered here, I've put together a helpful cheatsheet for you right here:
Download the Python variables cheatsheet (it takes 5 seconds).
What is a Variable in Python?
So what are variables and why do we need them?
Variables are essential for holding onto and referencing values throughout our application. By storing a value into a variable, you can reuse it as many times and in whatever way you like throughout your project.
You can think of variables as boxes with labels, where the label represents the variable name and the content of the box is the value that the variable holds.
In Python, variables are created the moment you give or assign a value to them.
How Do I Assign a Value to a Variable?
Assigning a value to a variable in Python is an easy process.
You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example:
In this example, we've created two variables: country and year_founded. We've assigned the string value "United States" to the country variable and integer value 1776 to the year_founded variable.
There are two things to note in this example:
- Variables in Python are case-sensitive . In other words, watch your casing when creating variables, because Year_Founded will be a different variable than year_founded even though they include the same letters
- Variable names that use multiple words in Python should be separated with an underscore _ . For example, a variable named "site name" should be written as "site_name" . This convention is called snake case (very fitting for the "Python" language).
How Should I Name My Python Variables?
There are some rules to follow when naming Python variables.
Some of these are hard rules that must be followed, otherwise your program will not work, while others are known as conventions . This means, they are more like suggestions.
Variable naming rules
- Variable names must start with a letter or an underscore _ character.
- Variable names can only contain letters, numbers, and underscores.
- Variable names cannot contain spaces or special characters.
Variable naming conventions
- Variable names should be descriptive and not too short or too long.
- Use lowercase letters and underscores to separate words in variable names (known as "snake_case").
What Data Types Can Python Variables Hold?
One of the best features of Python is its flexibility when it comes to handling various data types.
Python variables can hold various data types, including integers, floats, strings, booleans, tuples and lists:
Integers are whole numbers, both positive and negative.
Floats are real numbers or numbers with a decimal point.
Strings are sequences of characters, namely words or sentences.
Booleans are True or False values.
Lists are ordered, mutable collections of values.
Tuples are ordered, immutable collections of values.
There are more data types in Python, but these are the most common ones you will encounter while working with Python variables.
Python is Dynamically Typed
Python is what is known as a dynamically-typed language. This means that the type of a variable can change during the execution of a program.
Another feature of dynamic typing is that it is not necessary to manually declare the type of each variable, unlike other programming languages such as Java.
You can use the type() function to determine the type of a variable. For instance:
What Operations Can Be Performed?
Variables can be used in various operations, which allows us to transform them mathematically (if they are numbers), change their string values through operations like concatenation, and compare values using equality operators.
Mathematic Operations
It's possible to perform basic mathematic operations with variables, such as addition, subtraction, multiplication, and division:
It's also possible to find the remainder of a division operation by using the modulus % operator as well as create exponents using the ** syntax:
String operators
Strings can be added to one another or concatenated using the + operator.
Equality comparisons
Values can also be compared in Python using the < , > , == , and != operators.
These operators, respectively, compare whether values are less than, greater than, equal to, or not equal to each other.
Finally, note that when performing operations with variables, you need to ensure that the types of the variables are compatible with each other.
For example, you cannot directly add a string and an integer. You would need to convert one of the variables to a compatible type using a function like str() or int() .
Variable Scope
The scope of a variable refers to the parts of a program where the variable can be accessed and modified. In Python, there are two main types of variable scope:
Global scope : Variables defined outside of any function or class have a global scope. They can be accessed and modified throughout the program, including within functions and classes.
Local scope : Variables defined within a function or class have a local scope. They can only be accessed and modified within that function or class.
In this example, attempting to access local_var outside of the function_with_local_var function results in a NameError , as the variable is not defined in the global scope.
Don't be afraid to experiment with different types of variables, operations, and scopes to truly grasp their importance and functionality. The more you work with Python variables, the more confident you'll become in applying these concepts.
Finally, if you want to fully learn all of these concepts, I've put together for you a super helpful cheatsheet that summarizes everything we've covered here.
Just click the link below to grab it for free. Enjoy!
Download the Python variables cheatsheet
Become a Professional React Developer
React is hard. You shouldn't have to figure it out yourself.
I've put everything I know about React into a single course, to help you reach your goals in record time:
Introducing: The React Bootcamp
It’s the one course I wish I had when I started learning React.
Click below to try the React Bootcamp for yourself:

Full stack developer sharing everything I know.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
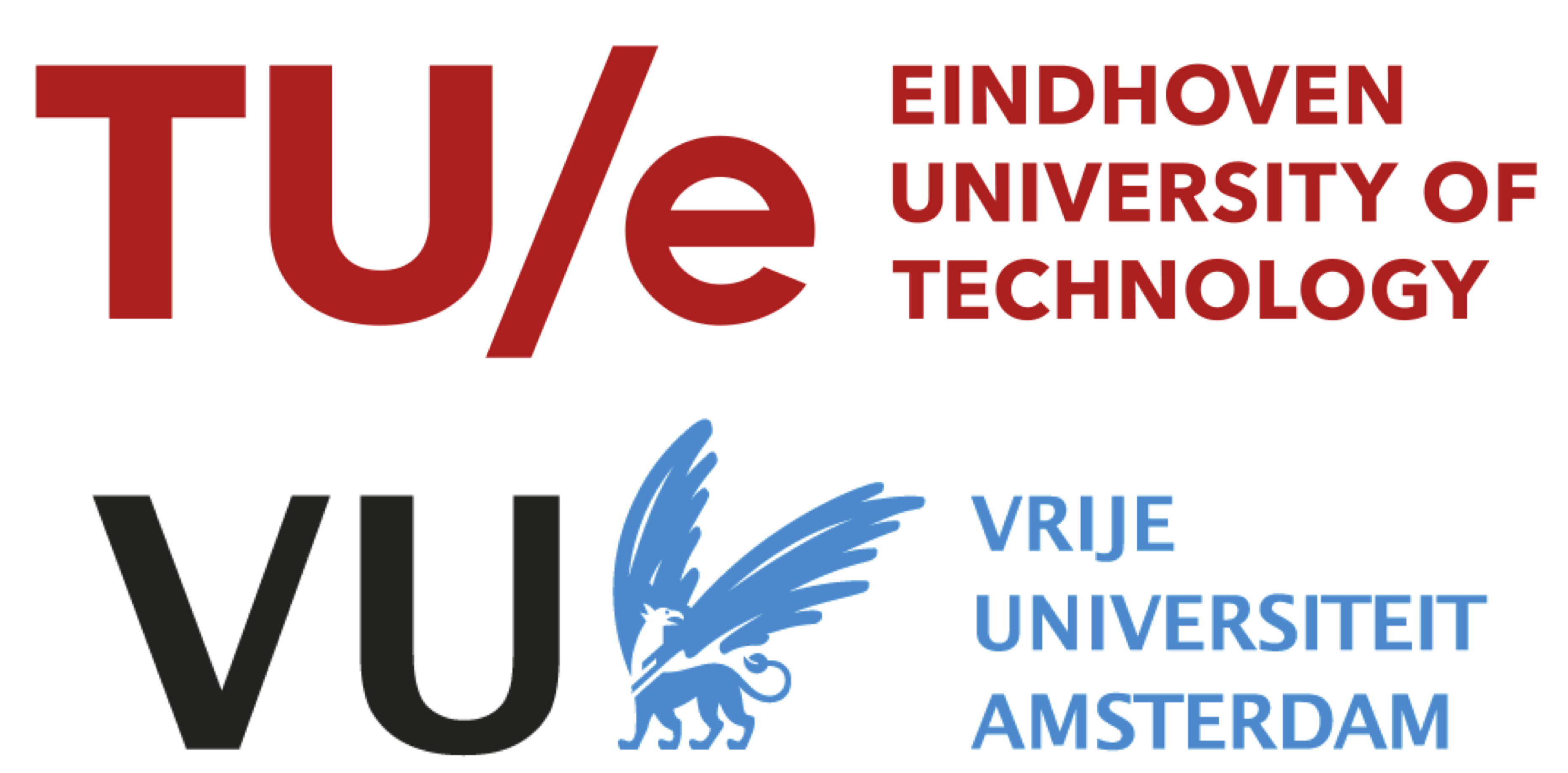
Learning Python by doing
- suggest edit
Variables, Expressions, and Assignments
Variables, expressions, and assignments 1 #, introduction #.
In this chapter, we introduce some of the main building blocks needed to create programs–that is, variables, expressions, and assignments. Programming related variables can be intepret in the same way that we interpret mathematical variables, as elements that store values that can later be changed. Usually, variables and values are used within the so-called expressions. Once again, just as in mathematics, an expression is a construct of values and variables connected with operators that result in a new value. Lastly, an assignment is a language construct know as an statement that assign a value (either as a constant or expression) to a variable. The rest of this notebook will dive into the main concepts that we need to fully understand these three language constructs.
Values and Types #
A value is the basic unit used in a program. It may be, for instance, a number respresenting temperature. It may be a string representing a word. Some values are 42, 42.0, and ‘Hello, Data Scientists!’.
Each value has its own type : 42 is an integer ( int in Python), 42.0 is a floating-point number ( float in Python), and ‘Hello, Data Scientists!’ is a string ( str in Python).
The Python interpreter can tell you the type of a value: the function type takes a value as argument and returns its corresponding type.
Observe the difference between type(42) and type('42') !
Expressions and Statements #
On the one hand, an expression is a combination of values, variables, and operators.
A value all by itself is considered an expression, and so is a variable.
When you type an expression at the prompt, the interpreter evaluates it, which means that it calculates the value of the expression and displays it.
In boxes above, m has the value 27 and m + 25 has the value 52 . m + 25 is said to be an expression.
On the other hand, a statement is an instruction that has an effect, like creating a variable or displaying a value.
The first statement initializes the variable n with the value 17 , this is a so-called assignment statement .
The second statement is a print statement that prints the value of the variable n .
The effect is not always visible. Assigning a value to a variable is not visible, but printing the value of a variable is.
Assignment Statements #
We have already seen that Python allows you to evaluate expressions, for instance 40 + 2 . It is very convenient if we are able to store the calculated value in some variable for future use. The latter can be done via an assignment statement. An assignment statement creates a new variable with a given name and assigns it a value.
The example in the previous code contains three assignments. The first one assigns the value of the expression 40 + 2 to a new variable called magicnumber ; the second one assigns the value of π to the variable pi , and; the last assignment assigns the string value 'Data is eatig the world' to the variable message .
Programmers generally choose names for their variables that are meaningful. In this way, they document what the variable is used for.
Do It Yourself!
Let’s compute the volume of a cube with side \(s = 5\) . Remember that the volume of a cube is defined as \(v = s^3\) . Assign the value to a variable called volume .
Well done! Now, why don’t you print the result in a message? It can say something like “The volume of the cube with side 5 is \(volume\) ”.
Beware that there is no checking of types ( type checking ) in Python, so a variable to which you have assigned an integer may be re-used as a float, even if we provide type-hints .
Names and Keywords #
Names of variable and other language constructs such as functions (we will cover this topic later), should be meaningful and reflect the purpose of the construct.
In general, Python names should adhere to the following rules:
It should start with a letter or underscore.
It cannot start with a number.
It must only contain alpha-numeric (i.e., letters a-z A-Z and digits 0-9) characters and underscores.
They cannot share the name of a Python keyword.
If you use illegal variable names you will get a syntax error.
By choosing the right variables names you make the code self-documenting, what is better the variable v or velocity ?
The following are examples of invalid variable names.
These basic development principles are sometimes called architectural rules . By defining and agreeing upon architectural rules you make it easier for you and your fellow developers to understand and modify your code.
If you want to read more on this, please have a look at Code complete a book by Steven McConnell [ McC04 ] .
Every programming language has a collection of reserved keywords . They are used in predefined language constructs, such as loops and conditionals . These language concepts and their usage will be explained later.
The interpreter uses keywords to recognize these language constructs in a program. Python 3 has the following keywords:
False class finally is return
None continue for lambda try
True def from nonlocal while
and del global not with
as elif if or yield
assert else import pass break
except in raise
Reassignments #
It is allowed to assign a new value to an existing variable. This process is called reassignment . As soon as you assign a value to a variable, the old value is lost.
The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well.
You have a variable salary that shows the weekly salary of an employee. However, you want to compute the monthly salary. Can you reassign the value to the salary variable according to the instruction?
Updating Variables #
A frequently used reassignment is for updating puposes: the value of a variable depends on the previous value of the variable.
This statement expresses “get the current value of x , add one, and then update x with the new value.”
Beware, that the variable should be initialized first, usually with a simple assignment.
Do you remember the salary excercise of the previous section (cf. 13. Reassignments)? Well, if you have not done it yet, update the salary variable by using its previous value.
Updating a variable by adding 1 is called an increment ; subtracting 1 is called a decrement . A shorthand way of doing is using += and -= , which stands for x = x + ... and x = x - ... respectively.
Order of Operations #
Expressions may contain multiple operators. The order of evaluation depends on the priorities of the operators also known as rules of precedence .
For mathematical operators, Python follows mathematical convention. The acronym PEMDAS is a useful way to remember the rules:
Parentheses have the highest precedence and can be used to force an expression to evaluate in the order you want. Since expressions in parentheses are evaluated first, 2 * (3 - 1) is 4 , and (1 + 1)**(5 - 2) is 8 . You can also use parentheses to make an expression easier to read, even if it does not change the result.
Exponentiation has the next highest precedence, so 1 + 2**3 is 9 , not 27 , and 2 * 3**2 is 18 , not 36 .
Multiplication and division have higher precedence than addition and subtraction . So 2 * 3 - 1 is 5 , not 4 , and 6 + 4 / 2 is 8 , not 5 .
Operators with the same precedence are evaluated from left to right (except exponentiation). So in the expression degrees / 2 * pi , the division happens first and the result is multiplied by pi . To divide by 2π, you can use parentheses or write: degrees / 2 / pi .
In case of doubt, use parentheses!
Let’s see what happens when we evaluate the following expressions. Just run the cell to check the resulting value.
Floor Division and Modulus Operators #
The floor division operator // divides two numbers and rounds down to an integer.
For example, suppose that driving to the south of France takes 555 minutes. You might want to know how long that is in hours.
Conventional division returns a floating-point number.
Hours are normally not represented with decimal points. Floor division returns the integer number of hours, dropping the fraction part.
You spend around 225 minutes every week on programming activities. You want to know around how many hours you invest to this activity during a month. Use the \(//\) operator to give the answer.
The modulus operator % works on integer values. It computes the remainder when dividing the first integer by the second one.
The modulus operator is more useful than it seems.
For example, you can check whether one number is divisible by another—if x % y is zero, then x is divisible by y .
String Operations #
In general, you cannot perform mathematical operations on strings, even if the strings look like numbers, so the following operations are illegal: '2'-'1' 'eggs'/'easy' 'third'*'a charm'
But there are two exceptions, + and * .
The + operator performs string concatenation, which means it joins the strings by linking them end-to-end.
The * operator also works on strings; it performs repetition.
Speedy Gonzales is a cartoon known to be the fastest mouse in all Mexico . He is also famous for saying “Arriba Arriba Andale Arriba Arriba Yepa”. Can you use the following variables, namely arriba , andale and yepa to print the mentioned expression? Don’t forget to use the string operators.
Asking the User for Input #
The programs we have written so far accept no input from the user.
To get data from the user through the Python prompt, we can use the built-in function input .
When input is called your whole program stops and waits for the user to enter the required data. Once the user types the value and presses Return or Enter , the function returns the input value as a string and the program continues with its execution.
Try it out!
You can also print a message to clarify the purpose of the required input as follows.
The resulting string can later be translated to a different type, like an integer or a float. To do so, you use the functions int and float , respectively. But be careful, the user might introduce a value that cannot be converted to the type you required.
We want to know the name of a user so we can display a welcome message in our program. The message should say something like “Hello \(name\) , welcome to our hello world program!”.
Script Mode #
So far we have run Python in interactive mode in these Jupyter notebooks, which means that you interact directly with the interpreter in the code cells . The interactive mode is a good way to get started, but if you are working with more than a few lines of code, it can be clumsy. The alternative is to save code in a file called a script and then run the interpreter in script mode to execute the script. By convention, Python scripts have names that end with .py .
Use the PyCharm icon in Anaconda Navigator to create and execute stand-alone Python scripts. Later in the course, you will have to work with Python projects for the assignments, in order to get acquainted with another way of interacing with Python code.
This Jupyter Notebook is based on Chapter 2 of the books Python for Everybody [ Sev16 ] and Think Python (Sections 5.1, 7.1, 7.2, and 5.12) [ Dow15 ] .
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
Python Data Types
- Python Type Conversion
- Python I/O and Import
- Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
- Python List
- Python Tuple
- Python String
- Python Dictionary
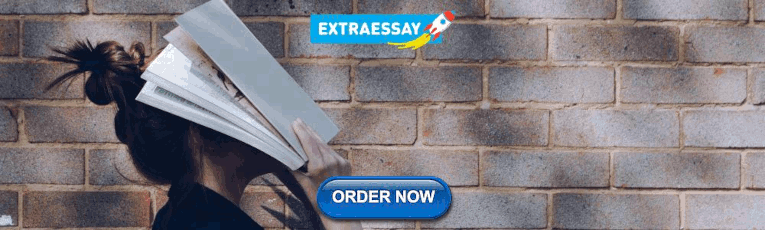
Python Files
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
- Python Examples
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Python Keywords and Identifiers
Python Numbers, Type Conversion and Mathematics
Python Variable Scope
- Python Basic Input and Output
- Python String Interpolation
Python Variables, Constants and Literals
In programming, a variable is a container (storage area) to hold data. For example,
Here, number is the variable storing the value 10 .
- Assigning values to Variables in Python
As we can see from the above example, we use the assignment operator = to assign a value to a variable.
In the above example, we assigned the value 'programiz.pro' to the site_name variable. Then, we printed out the value assigned to site_name .
Note : Python is a type-inferred language, so you don't have to explicitly define the variable type. It automatically knows that programiz.pro is a string and declares the site_name variable as a string.
- Changing the Value of a Variable in Python
Here, the value of site_name is changed from 'programiz.pro' to 'apple.com' .
Example: Assigning multiple values to multiple variables
If we want to assign the same value to multiple variables at once, we can do this as:
Here, we have assigned the same string value 'programiz.com' to both the variables site1 and site2 .
- Rules for Naming Python Variables
- Constant and variable names should have a combination of letters in lowercase (a to z) or uppercase ( A to Z ) or digits ( 0 to 9 ) or an underscore ( _ ) . For example:
- Create a name that makes sense. For example, vowel makes more sense than v .
- If you want to create a variable name having two words, use underscore to separate them. For example:
- Python is case-sensitive. So num and Num are different variables. For example,
- Avoid using keywords like if , True , class , etc. as variable names.
- Python Constants
A constant is a special type of variable whose value cannot be changed.
In Python, constants are usually declared and assigned in a module (a new file containing variables, functions , etc which is imported to the main file).
Let's see how we declare constants in separate file and use it in the main file,
Create a constant.py :
Create a main.py :
In the above example, we created the constant.py module file. Then, we assigned the constant value to PI and GRAVITY .
After that, we create the main.py file and import the constant module. Finally, we printed the constant value.
Note : In reality, we don't use constants in Python. Naming them in all capital letters is a convention to separate them from variables, however, it does not actually prevent reassignment.
- Python Literals
Literals are representations of fixed values in a program. They can be numbers, characters, or strings, etc. For example, 'Hello, World!' , 12 , 23.0 , 'C' , etc.
Literals are often used to assign values to variables or constants. For example,
In the above expression, site_name is a variable, and 'programiz.com' is a literal.
- Python Numeric Literals
Numeric Literals are immutable (unchangeable). Numeric literals can belong to 3 different numerical types: Integer , Float , and Complex .
- Python Boolean Literals
There are two boolean literals: True and False .
For example,
Here, True is a boolean literal assigned to result1 .
- String and Character Literals in Python
Character literals are unicode characters enclosed in a quote. For example,
Here, S is a character literal assigned to some_character .
Similarly, String literals are sequences of Characters enclosed in quotation marks.
Here, 'Python is fun' is a string literal assigned to some_string .
- Special Literal in Python
Python contains one special literal None . We use it to specify a null variable. For example,
Here, we get None as an output as the value variable has no value assigned to it.
- Literal Collections
There are four different literal collections List literals, Tuple literals, Dict literals, and Set literals.
In the above example, we created a:
- list of fruits
- tuple of numbers
- dictionary of alphabets having values with keys designated to each value
- set of vowels
To learn more about literal collections, refer to Python Data Types .
Table of Contents
Video: python variables and print().
Sorry about that.
Related Tutorials
Python Tutorial
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python variables, varia b l e s.
Variables are containers for storing data values.
Creating Variables
Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.
Variables do not need to be declared with any particular type , and can even change type after they have been set.
If you want to specify the data type of a variable, this can be done with casting.
Advertisement
Get the Type
You can get the data type of a variable with the type() function.
Single or Double Quotes?
String variables can be declared either by using single or double quotes:
Case-Sensitive
Variable names are case-sensitive.
This will create two variables:

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.

Hiring? Flexiple helps you build your dream team of developers and designers .
How To Assign Values To Variables In Python?

Harsh Pandey
Last updated on 19 Mar 2024
Assigning values in Python to variables is a fundamental and straightforward process. This action forms the basis for storing and manipulating Python data. The various methods for assigning values to variables in Python are given below.
Assign Values To Variables Direct Initialisation Method
Assigning values to variables in Python using the Direct Initialization Method involves a simple, single-line statement. This method is essential for efficiently setting up variables with initial values.
To use this method, you directly assign the desired value to a variable. The format follows variable_name = value . For instance, age = 21 assigns the integer 21 to the variable age.
This method is not just limited to integers. For example, assigning a string to a variable would be name = "Alice" . An example of this implementation in Python is.
Python Variables – Assign Multiple Values
In Python, assigning multiple values to variables can be done in a single, efficient line of code. This feature streamlines the initializing of several variables at once, making the code more concise and readable.
Python allows you to assign values to multiple variables simultaneously by separating each variable and value with commas. For example, x, y, z = 1, 2, 3 simultaneously assigns 1 to x, 2 to y, and 3 to z.
Additionally, Python supports unpacking a collection of values into variables. For example, if you have a list values = [1, 2, 3] , you can assign these values to a, b, c by writing a, b, c = values .
Python’s capability to assign multiple values to multiple variables in a single line enhances code efficiency and clarity. This feature is applicable across various data types and includes unpacking collections into multiple variables, as shown in the examples.
Assign Values To Variables Using Conditional Operator
Assigning values to variables in Python using a conditional operator allows for more dynamic and flexible value assignments based on certain conditions. This method employs the ternary operator, a concise way to assign values based on a condition's truth value.
The syntax for using the conditional operator in Python follows the pattern: variable = value_if_true if condition else value_if_false . For example, status = 'Adult' if age >= 18 else 'Minor' assigns 'Adult' to status if age is 18 or more, and 'Minor' otherwise.
This method can also be used with more complex conditions and various data types. For example, you can assign different strings to a variable based on a numerical comparison
Using the conditional operator for variable assignment in Python enables more nuanced and condition-dependent variable initialization. It is particularly useful for creating readable one-liners that eliminate the need for longer if-else statements, as illustrated in the examples.
Python One Liner Conditional Statement Assigning
Python allows for one-liner conditional statements to assign values to variables, providing a compact and efficient way of handling conditional assignments. This approach utilizes the ternary operator for conditional expressions in a single line of code.
The ternary operator syntax in Python is variable = value_if_true if condition else value_if_false . For instance, message = 'High' if temperature > 20 else 'Low' assigns 'High' to message if temperature is greater than 20, and 'Low' otherwise.
This method can also be applied to more complex conditions.
Using one-liner conditional statements in Python for variable assignment streamlines the process, especially when dealing with simple conditions. It replaces the need for multi-line if-else statements, making the code more concise and readable.
Work with top startups & companies. Get paid on time.
Try a top quality developer for 7 days. pay only if satisfied..
// Find jobs by category
You've got the vision, we help you create the best squad. Pick from our highly skilled lineup of the best independent engineers in the world.
- Ruby on Rails
- Elasticsearch
- Google Cloud
- React Native
- Contact Details
- 2093, Philadelphia Pike, DE 19703, Claymont
- [email protected]
- Explore jobs
- We are Hiring!
- Write for us
- 2093, Philadelphia Pike DE 19703, Claymont
Copyright @ 2024 Flexiple Inc
Exploring the Central Park Squirrel Scurry
Pre-class assignment: thinking more about functions, pre-class assignment: thinking more about functions #, ✅ put your name here #, goals for today’s pre-class assignment #.
By the end of this assignment, you should be able to:
More easily define and use functions in Python.
Assignment instructions #
Watch any videos below, read all included and linked to content, and complete any assigned programming problems.
1. Basic function structure #
The following short video serves as a reminder of the basic structure of a function and how you call functions that you write. (If the YouTube video doesn’t work, you can access a back-up version on MediaSpace )
2. Defining function input parameters #
Here’s another short video that reminds you how to define input parameters in Python. The video also points out how you can be specific about defining your input variable to be exactly what you want them to be, without worrying about the specific order of the variables. (If the YouTube video doesn’t work, you can access a back-up version on MediaSpace )
3. Setting default function values #
Now that we’re comfortable with writing functions and defining our input variables, this next video will show us how to define defaults for our input variables so that we don’t always have to define every single input value. This can be useful if we want a function to have a default mode of operation that works most of the time, but we still want to be able to change the values of some of the variables when necessary. (If the YouTube video doesn’t work, you can access a back-up version on MediaSpace )
Now let’s try to incorporate the things we learned in the last three videos into one small function.
✅ Question: Create a function that takes a person’s name as a string input and prints
Happy Birthday to < name >
where “< name >” is the person’s name that is given to the function. Set up the function so that if no name is given, it just says “Happy Birthday to you!” . You can give the function any name you want, but you should try to pick a function name that makes sense to someone else who might be using the function! Also, make sure to comment the function to explain what it does!
4. Writing functions that Return Values #
The previous three videos focused on defining the input variables for function, giving them defaults, and walking you through different ways to feed your input values into your functions, but they didn’t talk about returning variables from functions. In Python, we commonly want to write functions that not only take in a variety of input values, but we also want the function to return some new values when the function exits. For example, I might have a bit of code that looks like this:
This function takes in three values, s1 , s2 , and s3 , concatenates (adds together) the three strings into a sentence, and then returns that sentence. That means when I call the function, I can assign a new variable to be the value that is returned by the function. Specifically, I might do something that looks like this:
With that first line of code, I’ve created a new variable called my_sentence that stores the result of my concatenate_strings function. It is important to note that if I don’t intentionally “catch” the returned value from my function, I won’t have access to that value later on because the combined variable in my function is local to the function . When we say that a variable is local to the function, it means that it can only be accessed in the place that it was created, inside the function. This means that if we simply do:
We aren’t actually storing the value we want. Python will print the value to the screen because we didn’t catch the value with a variable. Again, if I wanted to access that value later, I don’t have any way of doing that. The function concatenated the strings, but we never stored the result anywhere, so calling the function was only useful if we wanted to see the sentence but didn’t care about using the sentence. Remembering to store the output from your functions is very important when writing code!
✅ Task : Trying it yourself
In the cell below try writing a function called check_for_strings that takes a value as an input argument and returns the Boolean value “ True ” if the value is a string and the Boolean value “ False ” if the value is not. While we have been making use of Boolean True / False values in a variety of ways in class up to this point, we may not have discussed them explicitly previously. You can learn a bit about the Boolean data type here . Basically, when we evaluate a conditional statement, we either get a True Boolean value or a False Boolean value – it really is that simple.
Useful tip: You can use the built-in type() function to determine the type of a variable. You may wanted to experiment with using the type() function to convince yourself how it works.
Once you’ve completed your function, make sure you also test your function to ensure that it works as you intend!
Important reminder : The moment a function comes across a return statement, it will exit the function and ignore any code that comes later in the function. This can actually be kind of useful if you want a function to return a value based on set of conditional statements.
5. Putting it All Together #
In this section, we are going to level up what we can do with functions!
So far, you have practiced writing functions with different kinds of inputs, outputs, and default values, but now we will to explore ways of building more complex (but still compartmentalized and transferable) code by combining functions.
One particularly useful way to build more complex code is to have functions that call other functions inside them.
When we call a function inside another function, remember to keep in mind variable scope . Any variables the function you are calling inside another function needs will need to be provided as input arguments in your outer function!
In the cell below, write a function that combines your concatenate_strings and check_for_strings functions to:
Take in 3 values
Check if those 3 values are strings
If all three are strings, concatenate those strings into one new string
Return the new concatenated string
Something to think about : What will your code do if not all three of the values are strings? Implement a way of handling this in your code.
✅ Questions : Now that your function runs, try to add some default values to your input arguments.
What variables would be most useful to have default arguments for?
Give an example of a built-in Python function that uses default arguments. Describe what the function does and what purpose the default arguments serve.
✎ Put your answers here
Follow-up Questions #
Copy and paste the following questions into the appropriate box in the assignment survey include below and answer them there. (Note: You’ll have to fill out the section number and the assignment number and go to the “NEXT” section of the survey to paste in these questions.)
What happens if I don’t include a “return” statement when writing a python function?
Why might it be useful to define a Python function that has default values for your input arguments?
How are you feeling about writing functons in Python, are there any aspects you’re still not feeling confident about?
Assignment wrap-up #
For convenience, we’re going to include a couple of Python function references again so that you can check them out if you’re still looking for more information about functions :
Tutorial on functions in python
More function basics with executable examples
Now , please fill out the form that appears when you run the code below. You must completely fill this out in order to receive credit for the assignment!
Every dunder method in Python
You've just made a class. You made a __init__ method. Now what?
Python includes tons of dunder methods ("double underscore" methods) which allow us to deeply customize how our custom classes interact with Python's many features. What dunder methods could you add to your class to make it friendly for other Python programmers who use it?
Let's take a look at every dunder method in Python , with a focus on when each method is useful.
Note that the Python documentation refers to these as special methods and notes the synonym "magic method" but very rarely uses the term "dunder method". However, "dunder method" is a fairly common Python colloquialism, as noted in my unofficial Python glossary .
You can use the links scattered throughout this page for more details on any particular dunder method. For a list of all of them, see the cheat sheet in the final section.
The 3 essential dunder methods 🔑
There are 3 dunder methods that most classes should have: __init__ , __repr__ , and __eq__ .
The __init__ method is the initializer (not to be confused with the constructor ), the __repr__ method customizes an object's string representation, and the __eq__ method customizes what it means for objects to be equal to one another.
The __repr__ method is particularly helpful at the the Python REPL and when debugging.
Equality and hashability 🟰
In addition to the __eq__ method, Python has 2 other dunder methods for determining the "value" of an object in relation to other objects.
Python's __eq__ method typically returns True , False , or NotImplemented (if objects can't be compared). The default __eq__ implementation relies on the is operator, which checks for identity .
The default implementation of __ne__ calls __eq__ and negates any boolean return value given (or returns NotImplemented if __eq__ did). This default behavior is usually "good enough", so you'll almost never see __ne__ implemented .
Hashable objects can be used as keys in dictionaries or values in sets. All objects in Python are hashable by default, but if you've written a custom __eq__ method then your objects won't be hashable without a custom __hash__ method. But the hash value of an object must never change or bad things will happen so typically only immutable objects implement __hash__ .
For implementing equality checks, see __eq__ in Python . For implementing hashability, see making hashable objects in Python .
Orderability ⚖️
Python's comparison operators ( < , > , <= , >= ) can all be overloaded with dunder methods as well. The comparison operators also power functions that rely on the relative ordering of objects, like sorted , min , and max .
If you plan to implement all of these operators in the typical way (where x < y would be the same as asking y > x ) then the total_ordering decorator from Python's functools module will come in handy.
Type conversions and string formatting ⚗️
Python has a number of dunder methods for converting objects to a different type.
The __bool__ function is used for truthiness checks, though __len__ is used as a fallback.
If you needed to make an object that acts like a number (like decimal.Decimal or fractions.Fraction ), you'll want to implement __int__ , __float__ , and __complex__ so your objects can be converted to other numbers. If you wanted to make an object that could be used in a memoryview or could otherwise be converted to bytes , you'll want a __bytes__ method.
The __format__ and __repr__ methods are different string conversion flavors. Most string conversions rely the __str__ method, but the default __str__ implementation simply calls __repr__ .
The __format__ method is used by all f-string conversions , by the str class's format method, and by the (rarely used) built-in format function. This method allows datetime objects to support custom format specifiers .
Context managers 🚪
A context manager is an object that can be used in a with block.
For more on context managers see, what is a context manager and creating a context manager .
Containers and collections 🗃️
Collections (a.k.a. containers) are essentially data structures or objects that act like data stuctures. Lists, dictionaries, sets, strings, and tuples are all examples of collections.
The __iter__ method is used by the iter function and for all forms of iteration: for loops , comprehensions , tuple unpacking , and using * for iterable unpacking .
While the __iter__ method is necessary for creating a custom iterable, the __next__ method is necessary for creating a custom iterator (which is much less common). The __missing__ method is only ever called by the dict class on itself, unless another class decides to implement __missing__ . The __length_hint__ method supplies a length guess for structures which do not support __len__ so that lists or other structures can be pre-sized more efficiently.
Also see: the iterator protocol , implementing __len__ , and implementing __getitem__ .
Callability ☎️
Functions, classes, and all other callable objects rely on the __call__ method.
When a class is called, its metaclass 's __call__ method is used. When a class instance is called, the class's __call__ method is used.
For more on callability, see Callables: Python's "functions" are sometimes classes .
Arithmetic operators ➗
Python's dunder methods are often described as a tool for "operator overloading". Most of this "operator overloading" comes in the form of Python's various arithmetic operators.
There are two ways to break down the arithmetic operators:
- Mathematical (e.g. + , - , * , / , % ) versus bitwise (e.g. & , | , ^ , >> , ~ )
- Binary (between 2 values, like x + y ) versus unary (before 1 value, like +x )
The mathematical operators are much more common than the bitwise ones and the binary ones are a bit more common than the unary ones.
These are the binary mathematical arithmetic operators:
Each of these operators includes left-hand and right-hand methods. If x.__add__(y) returns NotImplemented , then y.__radd__(x) will be attempted. See arithmetic dunder methods for more.
These are the binary bitwise arithmetic operators:
These are Python's unary arithmetic operators:
The unary + operator typically has no effect , though some objects use it for a specific operation. For example using + on collections.Counter objects will remove non-positive values.
Python's arithmetic operators are often used for non-arithmetic ends: sequences use + to concatenate and * to self-concatenate and sets use & for intersection, | for union, - for asymmetric difference, and ^ for symmetric difference. Arithmetic operators are sometimes overloaded for more creative uses too. For example, pathlib.Path objects use / to create child paths .
In-place arithmetic operations ♻️
Python includes many dunder methods for in-place operations. If you're making a mutable object that supports any of the arithmetic operations, you'll want to implement the related in-place dunder method(s) as well.
All of Python's binary arithmetic operators work in augmented assignment statements , which involve using an operator followed by the = sign to assign to an object while performing an operation on it.
Augmented assignments on mutable objects are expected to mutate the original object , thanks to the mutable object implementing the appropriate dunder method for in-place arithmetic.
When no dunder method is found for an in-place operation, Python performs the operation followed by an assignment. Immutable objects typically do not implement dunder methods for in-place operations , since they should return a new object instead of changing the original.
Built-in math functions 🧮
Python also includes dunder methods for many math-related functions, both built-in functions and some functions in the math library.
Python's divmod function performs integer division ( // ) and a modulo operation ( % ) at the same time. Note that, just like the many binary arithmetic operators, divmod will also check for an __rvidmod__ method if it needs to ask the second argument to handle the operation.
The __index__ method is for making integer-like objects. This method losslessly converts to an integer, unlike __int__ which may perform a "lossy" integer conversion (e.g. from float to int ). It's used by operations that require true integers, such as slicing , indexing, and bin , hex , and oct functions ( example ).
Attribute access 📜
Python even includes dunder methods for controlling what happens when you access, delete, or assign any attribute on an object!
The __getattribute__ method is called for every attribute access, while the __getattr__ method is only called after Python fails to find a given attribute. All method calls and attribute accesses call __getattribute__ so implementing it correctly is challenging (due to accidental recursion ).
The __dir__ method should return an iterable of attribute names (as strings). When the dir function calls __dir__ , it converts the returned iterable into a sorted list (like sorted does).
The built-in getattr , setattr , and delattr functions correspond to the dunder methods of the same name, but they're only intended for dynamic attribute access (not all attribute accesses).
Metaprogramming 🪄
Now we're getting into the really unusual dunder methods. Python includes many dunder methods for metaprogramming-related features.
The __prepare__ method customizes the dictionary that's used for a class's initial namespace. This is used to pre-populate dictionary values or customize the dictionary type ( silly example ).
The __instancecheck__ and __subclasscheck__ methods override the functionality of isinstance and issubclass . Python's ABCs use these to practice goose typing ( duck typing while type checking).
The __init_subclass__ method allows classes to hook into subclass initialization ( example ). Classes also have a __subclasses__ method (on their metaclass ) but it's not typically overridden.
Python calls __mro_entries__ during class inheritance for any base classes that are not actually classes. The typing.NamedTuple function uses this to pretend it's a class ( see here ).
The __class_getitem__ method allows a class to be subscriptable ( without its metaclass needing a __getitem__ method). This is typically used for enabling fancy type annotations (e.g. list[int] ).
Descriptors 🏷️
Descriptors are objects that, when attached to a class, can hook into the access of the attribute name they're attached to on that class.
The descriptor protocol is mostly a feature that exists to make Python's property decorator work, though it is also used by a number of third-party libraries.
Implementing a low-level memory array? You need Python's buffer protocol .
The __release_buffer__ method is called when the buffer that's returned from __buffer__ is deleted.
Python's buffer protocol is typically implemented in C, since it's meant for low level objects.
Asynchronous operations 🤹
Want to implement an asynchronous context manager? You need these dunder methods:
- __aenter__ : just like __enter__ , but it returns an awaitable object
- __aexit__ : just like __exit__ , but it returns an awaitable object
Need to support asynchronous iteration? You need these dunder methods:
- __aiter__ : must return an asynchronous iterator
- __anext__ : like __next__ or non-async iterators, but this must return an awaitable object and this should raise StopAsyncIteration instead of StopIteration
Need to make your own awaitable object? You need this dunder method:
- __await__ : returns an iterator
I have little experience with custom asynchronous objects, so look elsewhere for more details.
Construction and finalizing 🏭
The last few dunder methods are related to object creation and destruction.
Calling a class returns a new class instance thanks to the __new__ method. The __new__ method is Python's constructor method , though unlike constructors in many programming languages, you should almost never define your own __new__ method. To control object creation, prefer the initializer ( __init__ ), not the constructor ( __new__ ). Here's an odd __new__ example .
You could think of __del__ as a "destructor" method, though it's actually called the finalizer method . Just before an object is deleted, its __del__ method is called ( example ). Files implement a __del__ method that closes the file and any binary file buffer that it may be linked to.
Library-specific dunder methods 🧰
Some standard library modules define custom dunder methods that aren't used anywhere else:
- dataclasses support a __post_init__ method
- abc.ABC classes have a __subclasshook__ method which abc.ABCMeta calls in its __subclasscheck__ method (more in goose typing )
- Path-like objects have a __fspath__ method, which returns the file path as a string
- Python's copy module will use the __copy__ and __deepcopy__ methods if present
- Pickling relies on __getnewargs_ex__ or __getargs__ , though __getstate__ and __setstate__ can customize further and __reduce__ or __reduce_ex__ are even lower-level
- sys.getsizeof relies on the __sizeof__ method to get an object's size (in bytes)
Dunder attributes 📇
In addition to dunder methods, Python has many non-method dunder attributes .
Here are some of the more common dunder attributes you'll see:
- __name__ : name of a function, classes, or module
- __module__ : module name for a function or class
- __doc__ : docstring for a function, class, or module
- __class__ : an object's class (call Python's type function instead)
- __dict__ : most objects store their attributes here (see where are attributes stored? )
- __slots__ : classes using this are more memory efficient than classes using __dict__
- __match_args__ : classes can define a tuple noting the significance of positional attributes when the class is used in structural pattern matching ( match - case )
- __mro__ : a class's method resolution order used when for attribute lookups and super() calls
- __bases__ : the direct parent classes of a class
- __file__ : the file that defined the module object (though not always present!)
- __wrapped__ : functions decorated with functools.wraps use this to point to the original function
- __version__ : commonly used for noting the version of a package
- __all__ : modules can use this to customize the behavior of from my_module import *
- __debug__ : running Python with -O sets this to False and disables Python's assert statements
Those are only the more commonly seen dunder attributes. Here are some more:
- Functions have __defaults__ , __kwdefaults__ , __code__ , __globals__ , and __closure__
- Both functions and classes have __qualname__ , __annotations__ , and __type_params__
- Instance methods have __func__ and __self__
- Modules may also have __loader__ , __package__ , __spec__ , and __cached__ attributes
- Packages have a __path__ attribute
- Exceptions have __traceback__ , __notes__ , __context__ , __cause__ , and __suppress_context__
- Descriptors use __objclass__
- Metaclasses use __classcell__
- Python's weakref module uses __weakref__
- Generic aliases have __origin__ , __args__ , __parameters__ , and __unpacked__
- The sys module has __stdout__ and __stderr__ which point to the original stdout and stderr versions
Additionally, these dunder attributes are used by various standard library modules: __covariant__ , __contravariant__ , __infer_variance__ , __bound__ , __constraints__ . And Python includes a built-in __import__ function which you're not supposed to use ( importlib.import_module is preferred) and CPython has a __builtins__ variable that points to the builtins module (but this is an implementation detail and builtins should be explicitly imported when needed instead). Also importing from the __future__ module can enable specific Python feature flags and Python will look for a __main__ module within packages to make them runnable as CLI scripts.
And that's just most of the dunder attribute names you'll find floating around in Python. 😵
Every dunder method: a cheat sheet ⭐
This is every Python dunder method organized in categories and ordered very roughly by the most commonly seen methods first. Some caveats are noted below.
The above table has a slight but consistent untruth . Most of these dunder methods are not actually called on an object directly but are instead called on the type of that object: type(x).__add__(x, y) instead of x.__add__(y) . This distinction mostly matters with metaclass methods.
I've also purposely excluded library-specific dunder methods (like __post_init__ ) and dunder methods you're unlikely to ever define (like __subclasses__ ). See those below.
So, Python includes 103 "normal" dunder methods, 12 library-specific dunder methods, and at least 52 other dunder attributes of various types. That's over 150 unique __dunder__ names! I do not recommend memorizing these: let Python do its job and look up the dunder method or attribute that you need to implement/find whenever you need it.
Keep in mind that you're not meant to invent your own dunder methods . Sometimes you'll see third-party libraries that do invent their own dunder method, but this isn't encouraged and it can be quite confusing for users who run across such methods and assume they're " real " dunder methods.
A Python tip every week
Need to fill-in gaps in your Python skills?
Sign up for my Python newsletter where I share one of my favorite Python tips every week .
Need to fill-in gaps in your Python skills ? I send weekly emails designed to do just that.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Web Browser
- How to Use SQL DISTINCT and TOP in Same Query?
- How to Convert Epoch Time to Date in SQL?
- SQL Query to Make Month Wise Report
- SQL Query to Convert UTC to Local Time Zone
- SQL Query to Check Given Format of a Date
- SQL Query to Add an Agent Parameter in a Database
- SQL Query to Add Email Validation Using Only One Query
- SQL Query to List All Databases
- SQL Query to Select all Records From Employee Table Where Name is Not Specified
- SQL Query to Get First and Last Day of a Week in a Database
- SQL Query to Calculate Total Number of Weeks Between Two Specific Dates
- SQL Query to Calculate Total Number of Days Between Two Specific Dates
- SQL Query to Convert FLOAT to NVARCHAR
- Compare and Find Differences Between Two Tables in SQL
- SQL Full Outer Join Using Left and Right Outer Join and Union Clause
- Querying Multiple Tables in SQL
- SQL Query to Find Number of Employees According to Gender Whose DOB is Between a Given Range
- SQL Query to Print Name of Distinct Employee Whose DOB is Between a Given Range
- SQL Query to Find Monthly Salary of Employee If Annual Salary is Given
How to Declare a Variable in SQL?
Variables in SQL is a fundamental step towards building efficient and powerful database applications. It enhances the flexibility and efficiency of our database queries. Understanding how to declare variables in SQL is very important to write scalable code. Variables act as placeholders for data which enable us to manipulate and store information within our SQL programs.
In this article, we’ll explore the various methods and best practices for declaring variables in SQL along with the syntax and examples, which help us to write more dynamic and effective queries.
How to Declare a Variable
When working with SQL we may encounter various situations where we need to store and manipulate temporary data within our queries effectively. Variables act as containers for values and enable various operations on the stored data. SQL provides several methods to declare and use variables including the use of the WITH clause, SET command, enhances, and various methods. Below are the methods that are used to declare variables in SQL effectively. The methods are as follows:
Using SET Command
Using WITH Clause
- Using Scalar Subqueries ,
- Using Temporary Tables
1. Using SET Command
Although SQL does not have a built-in SET command like some other database systems, we can use variable assignment using user-defined functions.
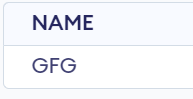
Explanation: The query selects the string “ GFG ” and renames it as “ NAME “. Consequently, the output consists of a single column labeled “ NAME ” containing the string “ GFG ” in each row.
2. Using With Clause
The WITH clause is a powerful feature in SQL that allows defining temporary result sets within a query. Variables can be declared and assigned values within the WITH clause.
Explanation: The output shows a virtual table named “ Customers ” created using a Common Table Expression (CTE) . It consists of a single column labeled “name”, containing the value “ GFG “. The SELECT statement retrieves all rows from this “ Customers ” table, displaying the single row with “ GFG ” as the name.
3. Using Temporary Variables
Temporary tables can be used to store and manipulate data within a session. We can take advantage of temporary tables to mimic variable declaration.

Using Temporary Variable
Explanation: The output displays a temporary table named “ Temp_Table” created with two columns: “ num ” of type INTEGER and “ name ” of type STRING . One row is inserted with values (23, “GFG”). The subsequent SELECT statement retrieves and displays the values from the “ num ” and “ name ” columns of “ Temp_Table “.
4. Using Subqueries
Subqueries in SQL provide a versatile tool for embedding queries within queries. This article delves into their application in assigning values to variables within SQLite databases, offering insights and examples for effective database manipulation.
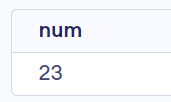
Using Subqueries
Explanation: The output simply returns the value 23 as “ num ” using a subquery within the SELECT statement . It assigns the result of the inner query directly to the column “num” in the outer query’s result set.
In this article, we went through multiple methods to declare variables in SQL , ranging from using with clause to user-defined functions and temporary tables. Each method has its advantages and use cases, depending on the complexity and requirements of our database operations. Understanding these techniques will help you with the flexibility to efficiently manage variables and execute queries in SQL databases. These methods help in flexibility of code.
Please Login to comment...
- WhatsApp To Launch New App Lock Feature
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 10 Best Skillshare Alternatives in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Online Degree Explore Bachelor’s & Master’s degrees
- MasterTrack™ Earn credit towards a Master’s degree
- University Certificates Advance your career with graduate-level learning
- Top Courses
- Join for Free
Operators: What Role Do They Play in Programming?
Learn about different types of operators and why they’re essential in computer programming, along with the different operator types in three popular languages.
![variable assignment in python example [Featured Image] A programmer wearing headphones is working on her laptop with types of operators.](https://d3njjcbhbojbot.cloudfront.net/api/utilities/v1/imageproxy/https://images.ctfassets.net/wp1lcwdav1p1/3Jjm45VPEENtqBBVTGNiRU/3f911f8e86c5e616cd0cada3e91b41bb/GettyImages-1032708132.jpg?w=1500&h=680&q=60&fit=fill&f=faces&fm=jpg&fl=progressive&auto=format%2Ccompress&dpr=1&w=1000)
Operators are symbols with defined functions. Programmers use these symbols to tell the interpreter or compiler in high-level computer languages, such as C++, Java, and Python, to perform a particular action. These symbols form the program's foundation, allowing you to perform various actions ranging from simple maths to complex encryption.
Operators are essential for performing calculations, assigning specific values to variables, and making condition-based decisions. You can learn more about operator types and how they work within three popular computer languages.
Types of operators
Different types perform different tasks within the programme. You can choose from three main types of operators, each with unique functions and capabilities.
Arithmetic operator
Arithmetic operators allow computers to perform mathematical calculations on specific values. For example, you might write ‘A+B = 40’ or ‘A*B = 100’. Common arithmetic operators include:
Addition: +
Subtraction: -
Multiplication: *
Division: /
Integer division: DIV
Remainder: MOD
Relational operator
Relational operators facilitate condition testing, allowing you to create variables and assign them values. For example, if A equals 45 and B equals 50, you might write A < B or A is less than B. That < symbol is a relational operator that produces true or false results. Common relational operators include:
Assignment: =
Equivalence: ==
Less than: <
Greater than: >
Less than or equal to: <=
Greater than or equal to: >=
Does not equal: !=
Logical operator
Logical operators allow programmers to gain increasingly complex computer-based decisions by combining relational operators. For example, with the logical AND operator, if A equals 10 and B equals zero, and you write ‘A AND B is false,’ the condition is true if both variables are the same. If you use the logical OR operator and just one of the variables is something other than zero, ‘A OR B is true’ would be true.
You can reverse the variable’s logical state with the local NOT operator. So, what would otherwise be true becomes false and vice versa.
Common operators include:
AND (&&)
Types of operators in C++
C++ uses more than five types of operators to carry out different functions. You may also use multiple operators in one expression. In these cases, C++ has established operator precedence to determine how things get evaluated. For example, postfix operators work left to right. So do additive, multiplicative, and shift operators. Unary, conditional, and assignment operators have precedence from right to left. Types of C++ operators include:
Assignment: These operators assign the left-hand side operand the right-hand side operand's value and are variations on = , including += (addition assignment), -= (subtraction assignment), *= (multiplication assignment), /= (division assignment), and %= (modulus assignment)
Arithmetic: These operators allow you to perform calculations between two values using symbols such as + (addition), - (subtraction), * (multiplication), / (division), % (modulus), ++ (increment), and — (decrement)
Relational: These operators perform comparisons between operands and include symbols like == equal to), > (greater than), < (less than), != (not equal to), >= (greater than or equal), and <= (less than or equal to)
Logical: These C++ operators check conditions and expressions to determine whether they are true or false to aid decision-making. They include symbols like && (logical AND operator if all operands are true), || (logical OR operator if at least one operand is true), and ! (logical NOT operator that reverses the logical state from true to false or false to true)
Bitwise: These C++ operators allow you to perform operations by treating operands like a string of bits for output in decimals. Symbols include & (logical AND operator performs AND on the bits of two operands), | (logical OR operator performs OR on the bits of two operands), ^ (bitwise XOR operator performs XOR on all bits of two numbers), ~ (bitwise one complement inverts all an operand's bits), << (binary left shift shifts the bits in one operand using the second operand as a determinant), >> (binary right shift shifts the bits in one operand using the second operand as a determinant)
Other: C++ also has other operators, including:
Size of , which determines a variable's size
Conditional , a ternary operator that evaluates test conditions and puts a code block into place based on test results
Comma, which triggers the start of an operational sequence
Types of Operators in Java
In Java, you use operators to perform local operations and mathematical tasks. You can break them into three categories based on the number of operands or the entities they operate upon and use. Unary operators work on one operand, binary operators work on two operands, and ternary operators utilise three operands to carry out the task. Java’s operators include:
Assignment: This binary operator uses the symbol = to assign the value of the operand on the right of an expression to the one on the left.
Arithmetic: These operators may be unary and binary and get placed in three categories, including primary, increment and decrement, and shorthand. Symbols include + (addition), - (subtraction), * (multiplication), / (division), % (modulus), + (unary plus to indicate a positive number), or - (unary minus to indicate a negative number)
Increment and decrement include ++ (increment) and — (decrement) to increment or decrement operands' values
Shorthand operators pair with assignment operators, making it possible to write less code by including symbols such as += (addition assignment), -= (subtraction assignment), and *= (multiplication assignment)
Relational: These binary operators work as comparison or equality operators and include symbols such as > (greater than), < (less than), >= (greater than equal to), <= (less than equal to), == (equal equal to), and != (not equal to)
Logical: Java operators in this category work on boolean operands to perform functions using symbols like & (logical AND), | (logical OR), ! (logical NOT), ^ (logical XOR), == (equal equal to), != (not equal to), && (conditional AND), and || (conditional OR)
Bitwise: To manipulate an operand's bits, you can use these operators, which include symbols like & (bitwise AND), | (bitwise OR), ~ (bitwise NOT), and ^ (bitwise XOR)
Types of Operators in Python
In Python, operators are symbols that perform specific tasks. The operator you choose for any operation changes the results, allowing you to manipulate operands' values. Python’s operators include:
Arithmetic: These operators include symbols that perform mathematical functions, including addition ( + ), subtraction ( - ), multiplication ( * ), division ( / ), and modulus ( % )
Assignment: These operators allow you to assign values and include various symbols, including = (X=2), -= (X-=2), += (X+=2), and *= (X*=2)
Comparison: When you want to compare two values, you will use symbols such as = (equal), != (not equal), > (greater than), and < (less than)
Logical: When you want to compare conditional statements, you can use operators such as x>2 and x>3 , x>2 or x>3 , or not (x>2 and x>3)
Membership: These are operators that allow you to calculate and figure out if an object contains a sequence, including in , which comes back true in the presence of the sequence, or not in , which comes back as true if the sequence doesn't exist
Bitwise: These operators compare binary values using symbols such as & (sets all bits to 1 if all bits are 1), | (sets all bits to 1 if one of the bits is 1), or ^ (sets all bits for 1 if one of the bits is 1). To invert all bits, you could use ~ (Bitwise NOT), or you can use operators to shift left ( << ) or shift right ( >> )
Identity: The two identity operators include is (comes back true if both variables are the same objects) and is not (comes back true if the variables are different objects)
What careers use operators
Operators are commonly used in computer programming, making programmers the primary career that uses them. You may use operators in other professions that require coding, including roles such as data analysts or data scientists.
Software engineers, data scientists, and data analysts are among India's most in-demand jobs [ 1 ]. Many applicants find positions in cities like Bangalore, sometimes called India’s Silicon Valley. Mumbai, Kolkata, and Delhi are common locations for these types of jobs. According to Glassdoor India, as of March 2024, the average base salaries for these careers are as follows:
Programmers: ₹4,00,000
Data scientists: ₹12,59,927
Data analysts: ₹8,47,000
Now that you’re more familiar with the types of operators and how they work in different languages, continue learning to increase your skills and achieve your goals. For example, if you’re new to programming, consider earning a Professional Certificate, such as Google IT with Python , or completing a Specialisation like Learn SQL Basics for Data Science offered by the University of California Davis on Coursera.
If you have programming experience and want to brush up on operators, complete a Guided Project like JavaScript Variables and Assignment Operators on Coursera Project Network or Looker Functions and Operators , a Project that's part of Google Cloud Training—both on Coursera.
Article sources
The Hindu. “ With a Placement Rate of 93.5% Software Engineers have Witnessed a 120% Increase in Salary in 2021-2022, https://www.thehindu.com/brandhub/with-a-placement-rate-of-935-software-engineers-have-witnessed-120-increase-in-salary-in-2021-2022/article65370576.ece.” Accessed March 22, 2024.
Keep reading
Coursera staff.
Editorial Team
Coursera’s editorial team is comprised of highly experienced professional editors, writers, and fact...
This content has been made available for informational purposes only. Learners are advised to conduct additional research to ensure that courses and other credentials pursued meet their personal, professional, and financial goals.
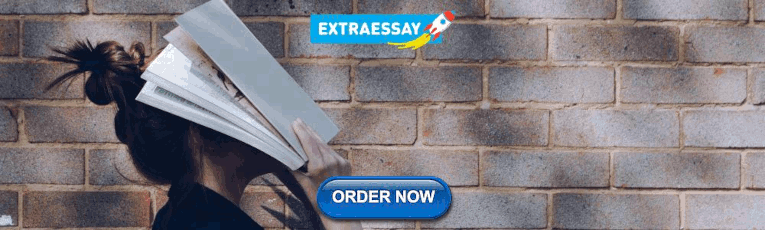
IMAGES
VIDEO
COMMENTS
To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .". Once this is done, n can be used in a statement or expression, and its value will be substituted: Python.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Declare And Assign Value To Variable. Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You" Assigning a value is known as binding in Python. In the example above, we have assigned the value of 2 to mySecondVariable.
The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name "x". After executing this line, this number will be stored into this variable. Until the value is changed or the variable ...
Variable Assignment. Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ).
Just assign a value to a variable using the = operator e.g. variable_name = value. That's it. The following creates a variable with the integer value. Example: Declare a Variable in Python. Copy. num = 10. Try it. In the above example, we declared a variable named num and assigned an integer value 10 to it.
Variable Assignment in Python. Python variables are created when a value is assigned to them using the equals sign (=) operator. For example, ... In the above example, the variable 'x' is a local variable that is defined within the function 'my_function()'. It cannot be accessed outside of that function, which is why the second print ...
A variable is a name for a value. An assignment statement associates a variable name on the left of the equal sign with the value of an expression calculated from the right of the equal sign. Enter. width. Once a variable is assigned a value, the variable can be used in place of that value. The response to the expression width is the same as if ...
As such, we can utilize variables, say name and grade, to serve as placeholders for this information. In this subsection, we will demonstrate how to define variables in Python. In Python, the = symbol represents the "assignment" operator. The variable goes to the left of =, and the object that is being assigned to the variable goes to the ...
A Python variable is a named bit of computer memory, keeping track of a value as the code runs. A variable is created with an "assignment" equal sign =, with the variable's name on the left and the value it should store on the right: x = 42. In the computer's memory, each variable is like a box, identified by the name of the variable.
Assigning a value to a variable in Python is an easy process. You simply use the equal sign = as an assignment operator, followed by the value you want to assign to the variable. Here's an example: country = "United States". year_founded = 1776. In this example, we've created two variables: country and year_founded.
An Example of a Variable in Python is a representational name that serves as a pointer to an object. Once an object is assigned to a variable, it can be referred to by that name. ... Variables Assignment in Python. Here, we will define a variable in python. Here, clearly we have assigned a number, a floating point number, and a string to a ...
As soon as you assign a value to a variable, the old value is lost. x: int = 42. print(x) x = 43. print(x) The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well. a: int = 42. b: int = a # a and b have now the same value. print('a =', a)
As we can see from the above example, we use the assignment operator = to assign a value to a variable. ... Rules for Naming Python Variables. Constant and variable names should have a combination of letters in lowercase ... So num and Num are different variables. For example, var num = 5 var Num = 55 print(num) # 5 print(Num) # 55 ...
The assignment expression (directive := input ("Enter text: ")) binds the value of directive to the value retrieved from the user via the input function. You bind the return value to the variable directive, which you print out in the body of the while loop. The while loop exits whenever the you type stop.
Python has no command for declaring a variable. A variable is created the moment you first assign a value to it. Example. x = 5. y = "John". print (x) print (y) Try it Yourself ». Variables do not need to be declared with any particular type, and can even change type after they have been set.
The "good practice" example clarifies the purpose of the variables, making the code more understandable. Use snake_case for Variable Names Best Practice: Follow the snake_case convention for ...
This method employs the ternary operator, a concise way to assign values based on a condition's truth value. The syntax for using the conditional operator in Python follows the pattern: variable = value_if_true if condition else value_if_false. For example, status = 'Adult' if age >= 18 else 'Minor' assigns 'Adult' to status if age is 18 or ...
Variable Assignment. In Python, a variable allows you to refer to a value with a name. To create a variable x with a value of 5, you use =, like this example: x = 5. You can now use the name of this variable, x, instead of the actual value, 5. Remember, = in Python means assignment, it doesn't test equality!
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables. Operator. Description. Syntax = Assign value of right side of expression to left side operand: ... Example: Python3. a = 3. b = 5 # a = a + b . a += b # Output . print(a)
Starting Python 3.8, and the introduction of assignment expressions (PEP 572) ( := operator), it's now possible to capture the condition value ( isBig (y)) as a variable ( x) in order to re-use it within the body of the condition: if x := isBig(y): return x. Share. Improve this answer. Follow.
The Python 3.8 documentation also includes some good examples of assignment expressions. Here are a few resources for more info on using bpython, the REPL (Read-Eval-Print Loop) tool used in most of these videos: Discover bpython: A Python REPL With IDE-Like Features; A better Python REPL: bpython vs python; bpython Homepage; bpython Docs
The real difference between your two snippets is in the first one where you have: n = 2. It is only the variable n that you are changing and not what it used to refer to. You are changing it to refer to something else. This affects nothing else, unless you are concerned with reference counting. - quamrana.
4. Writing functions that Return Values#. The previous three videos focused on defining the input variables for function, giving them defaults, and walking you through different ways to feed your input values into your functions, but they didn't talk about returning variables from functions. In Python, we commonly want to write functions that not only take in a variety of input values, but ...
All of Python's binary arithmetic operators work in augmented assignment statements, which involve using an operator followed by the = sign to assign to an object while performing an operation on it. Augmented assignments on mutable objects are expected to mutate the original object , thanks to the mutable object implementing the appropriate ...
Explanation: The output simply returns the value 23 as " num " using a subquery within the SELECT statement.It assigns the result of the inner query directly to the column "num" in the outer query's result set. Conclusion. In this article, we went through multiple methods to declare variables in SQL, ranging from using with clause to user-defined functions and temporary tables.
Operators are symbols with defined functions. Programmers use these symbols to tell the interpreter or compiler in high-level computer languages, such as C++, Java, and Python, to perform a particular action. These symbols form the program's foundation, allowing you to perform various actions ranging from simple maths to complex encryption.