- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Creating First REST API with FastAPI
- How to Learn Python in 21 Days
- Avoiding elif and ELSE IF Ladder and Stairs Problem
- Python | Decimal same_quantum() method
- SymPy | Subset.superset() in Python
- SymPy | Subset.subset() in Python
- File Objects in Python
- What makes Python a slow language ?
- Python | cmath.pi constant
- Python | Decimal radix() method
- How to Install dlib Library for python in windows 10
- Python Nested Loops
- Python | sympy.Add() method
- Python - Multi-Line Statements
- Python | Decimal scaleb() method
- Python | Decimal shift() method
- Single and Double Quotes | Python
- Python | Decimal sqrt() method
- Python | Decimal to_eng_string() method
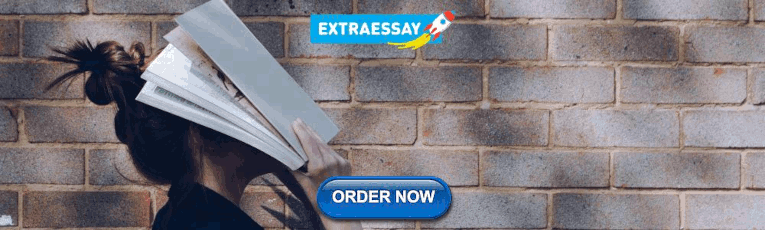
Walrus Operator in Python 3.8
The Walrus Operator is a new addition to Python 3.8 and higher. In this article, we’re going to discuss the Walrus operator and explain it with an example.
Introduction
Walrus Operator allows you to assign a value to a variable within an expression. This can be useful when you need to use a value multiple times in a loop, but don’t want to repeat the calculation.
The Walrus Operator is represented by the := syntax and can be used in a variety of contexts including while loops and if statements. The Assignment expressions allow a value to be assigned to a variable, even a variable that doesn’t exist yet, in the context of expression rather than as a stand-alone statement.
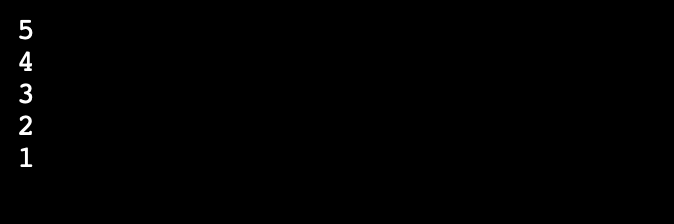
In this example, the length of the numbers list is assigned to the variable n using the Walrus Operator. The value of n is then used in the condition of the while loop, so that the loop will continue to execute until the numbers list is empty.
Example –
Let’s try to understand Assignment Expressions more clearly with the help of an example using both Python 3.7 and Python 3.8. Here we have a list of dictionaries called “sample_data”, which contains the userId, name and a boolean called completed.
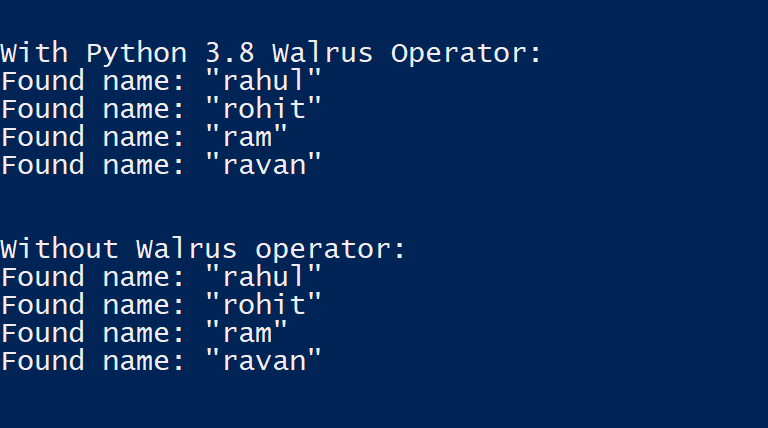
Here is another example:
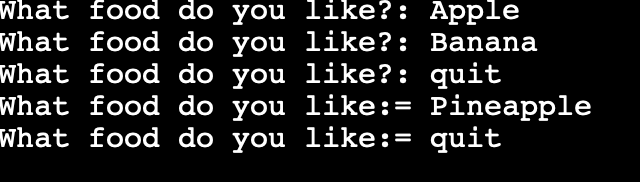
Note: This example, the user input is assigned to the variable name using the Walrus Operator. The value of name is then used in the if statement to determine whether it is in the names list. If it is, the corresponding message is printed, otherwise, a different message is printed.
Please Login to comment...
Similar reads.
- Programming Language
- Google Releases ‘Prompting Guide’ With Tips For Gemini In Workspace
- Google Cloud Next 24 | Gmail Voice Input, Gemini for Google Chat, Meet ‘Translate for me,’ & More
- 10 Best Viber Alternatives for Better Communication
- 12 Best Database Management Software in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Python Walrus Operator: An Introduction to the Assignment Expression in Python
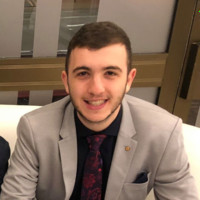
What is the Walrus Operator?
- In a Conditional Statement
- Lists and Dictionaries
Benefits of the Walrus Operator
- Python Version Compatibility
As a Python developer, you are always looking for ways to write more concise and expressive code. One of the exciting features introduced in Python 3.8 is the Walrus Operator, also known as the Assignment Expression. In this blog post, we'll explore the walrus operator, its usage, benefits, drawbacks, and best practices for using assignment expressions in your Python code.
The walrus operator ( := ) is a new syntax introduced in Python 3.8 through PEP 572 that allows you to assign a value to a variable as part of an expression. The operator gets its name from the eyes and tusks of a walrus, resembling the shape of := .
Before the introduction of the walrus operator, you would need to assign a value to a variable before using it in an expression. With the walrus operator, you can perform the assignment and expression evaluation in a single step. This simplifies your code and makes it more concise and readable.
Benefits and Usage of the Walrus Operator
The walrus operator offers several benefits and can be used in various scenarios to improve code clarity and efficiency.
Examples of How to Use the Walrus Operator
The walrus operator can be used in different contexts, such as loops, conditional statements, and dictionary construction. Here are a few examples:
In this example, the walrus operator is used to simultaneously read a line from the user and check if it's empty. The loop continues until the user enters an empty line, and each non-empty line is added to the lines list.
In a Conditional Statement:
In this case, the walrus operator is used to compute the length of the string and store it in the length variable. The length is then compared to max_length to determine if it exceeds the limit. If it does, a message is printed.
Lists and Dictionaries:
This section demonstrates the utilization of the walrus operator to efficiently compute statistical measures for a list of numbers and construct a dictionary. By employing the walrus operator, the code succinctly assigns the sum and length of the numbers list to variables num_sum and num_length respectively, while simultaneously creating the corresponding key-value pairs in the data dictionary. This concise approach simplifies the code structure, enhances readability, and eliminates the need for separate variable assignments.
The walrus operator provides several benefits:
Concise and Readable Code : With the walrus operator, you can perform assignments within expressions, reducing the need for separate lines of code. This results in more concise and readable code, especially in scenarios where you need to use a variable immediately after assigning a value to it.
Improved Performance : By combining assignment and expression evaluation, you avoid redundant computations. This can be particularly useful when working with expensive function calls or complex expressions.
Despite the value provided by the walrus operator, it is important to carefully consider its usage due to a factors like Python version compatibility and support for type hints.
Python version Compatibility
The walrus operator was introduced in Python 3.8. If you are working on a project that needs to support earlier versions of Python, it's crucial to consider compatibility and provide fallback solutions when necessary.
When using the walrus operator, the assigned value is determined dynamically at runtime, making it challenging to provide type hints directly for the assigned value. The walrus operator is primarily designed to improve code readability and simplify expressions by allowing variable assignment within an expression itself.
However, it's worth noting that you can still use type hints for the variables that are assigned using the walrus operator. For example, you can annotate the variable before the assignment statement, and the type hint will be applicable to the variable:
In the above example, the type hint int is provided for the variable result before the assignment statement using the walrus operator. Although the walrus operator itself doesn't directly support type hints, you can apply type hints to variables in the surrounding context.
The walrus operator, or assignment expression, introduced in Python 3.8, provides a powerful and concise way to assign values to variables as part of an expression. By using the walrus operator, you can simplify your code, improve its readability, and eliminate redundant computations.
Python's walrus operator offers a valuable tool for streamlining your code and making it more expressive. By using it judiciously and in alignment with best practices, you can take advantage of its benefits and enhance your Python programming experience.

Nick McCullum
Software Developer & Professional Explainer
How to Use the Walrus Operator in Python
In this article, I will talk about the walrus operator in Python.
The biggest change in Python is the introduction of assignment expressions, also known as walrus operator.
Assignment expression can be defined as a Python expression that allows you to assign and return a value in the same expression.
Table of Contents
You can skip to a specific section of this walrus operator tutorial using the table of contents below:
Strength of Walrus Operator – Demonstrated in While Loop
Final thoughts.
Walrus operator looks like this:
It is called the walrus operator because it looks like the eyes and tusks of a walrus on its side (:=). Now, if you want to assign a value to a variable and return it within the same expression, you can use assignment expression. Here is an example of how that is done typically in Python:
This program will return True .
However, these two statements can be combined to form one statement using the walrus operator. Here’s how to do that:
This program again will return True . You can clearly see that using assignment expression you are able to assign True to walrus and print it immediately. You do not need a separate statement to do that. However, you must understand that you cannot use the walrus operator to do something that is not possible without it.
However, you must understand that you cannot use the walrus operator to do something that is not possible without it. The whole idea behind a walrus operator is only to make some constructs more convenient or to communicate the intent of your code with greater clarity.
I will now demonstrate the use of assignment expressions in a while loop . In a while loop, a variable is initialized and updated. Look at the program below. Here the code requires the user to keep inputting data until they type end .
This code is not perfect and it can be made more efficient by setting up an infinite while loop and using break to stop it.
However, using walrus operator this can be done quite easily and it also simplifies the code to a large extent:
Assignment expressions are more about making the code simple by condensing it so that you do not have to use four lines for what can be achieved in two. There is no specific function that it performs, and therefore, only the code that runs without a walrus operator can be run using it.
If you're interested in learning more about the functionality (and controversy) of the walrus operator, I would highly recommend this fascinating video from Lex Fridman:
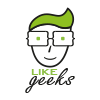
Python walrus operator (Python 3.8 assignment expression)
The “Python walrus operator”, officially known as the assignment expression operator, was introduced in Python 3.8. It’s symbolized by a colon followed by an equal sign := .
The Python community refers to it as the “walrus operator” due to its resemblance to a pair of eyes and tusks, like that of a walrus.
- 1 The Need for the Walrus Operator
- 2 Syntax of the Walrus Operator
- 3 Using in If Statements and While Loops
- 4 Using in List Comprehensions
- 5 Walrus Operator with Data Structures
- 6 When to Use and When Not
- 7 Compatibility and Version Support
- 8 Tips for Transitioning from Traditional Python Syntax
The Need for the Walrus Operator
Before the introduction of the walrus operator, Python developers had to assign values to variables in one line and use them in comparisons in the next.
This often resulted in multiple lines of code for simple operations.
In this code snippet, we had to write two lines to assign the value and then use it for comparison.
The walrus operator allows developers to assign and use variables within the same expression.
Syntax of the Walrus Operator
The syntax of the walrus operator is relatively straightforward. It involves a variable, a walrus operator := , and an expression.
Remember to enclose the assignment expression in parentheses.
In this case, the walrus operator assigns the value 10 to value and also returns the value 10 . However, remember that you can’t use this operator in a stand-alone statement, unlike the standard assignment operator = .
Using in If Statements and While Loops
The walrus operator can be used in if statements and while loops to make the code more concise. Here’s how to use the walrus operator in if statements.
In this example, the walrus operator is used to assign the length of input_value to value and compare it with 4 in the same line.
As the length of the string “Hello” is 5 , which is greater than 4 , the message is printed.
You can also use the walrus operator in while loops to make the code more concise:
In this example, the walrus operator is used to assign the value of input("Enter a non-empty string: ") to value and compare it to an empty string "" .
The loop continues to execute as long as the user enters an empty string.
As soon as a non-empty string is entered, it breaks out of the loop and prints the non-empty string.
Using in List Comprehensions
The walrus operator is handy when working with list comprehensions in Python. This allows for more complex calculations within list comprehensions without calling a function multiple times.
In this example, the walrus operator assigns the value of random.randint(1, 20) to number and checks if it’s even. If it is, it adds the number to the list. This results in a list of random even numbers.
Walrus Operator with Data Structures
The walrus operator can be effectively used with Python’s data structures like lists, sets, and dictionaries, as well as in comprehensions for these data structures.
In this example, the walrus operator is used to assign the maximum and minimum values of the numbers list to the variables num_max and num_min within the dictionary comprehension.
When to Use and When Not
While the walrus operator offers many advantages, it should be used judiciously. It’s best suited to situations where using it can make the code more concise without sacrificing readability.
For example, it’s beneficial when a variable needs to be assigned and used in the same line, such as within conditions or list comprehensions. On the other hand, it may not be suitable for complex expressions, as it can make the code difficult to read and understand.
Similarly, in situations where a stand-alone assignment is needed, the traditional assignment operator = should be used instead of the walrus operator.
Compatibility and Version Support
The walrus operator is a new operator introduced in Python 3.8. As such, it is not available in Python versions prior to 3.8.
For new projects or projects that are guaranteed to run on Python 3.8 or later, feel free to use the walrus operator whenever it improves your code’s clarity and conciseness.
If you’re working on a project that needs to support older Python versions, you should avoid using the walrus operator or rewrite your code in the new syntax.
Tips for Transitioning from Traditional Python Syntax
Transitioning to use the walrus operator from traditional Python syntax can be straightforward with the following tips:
- Start using the walrus operator in simple use cases like if conditions or while loops.
- Gradually move to more advanced uses like list comprehensions and function calls.
- Always consider the readability of your code. If the use of the walrus operator makes your code hard to read, it might be better to stick with traditional syntax.
Resource : https://docs.python.org/3/whatsnew/3.8.html
- Share on Facebook
- Tweet on Twitter
Mokhtar is the founder of LikeGeeks.com. He is a seasoned technologist and accomplished author, with expertise in Linux system administration and Python development. Since 2010, Mokhtar has built an impressive career, transitioning from system administration to Python development in 2015. His work spans large corporations to freelance clients around the globe. Alongside his technical work, Mokhtar has authored some insightful books in his field. Known for his innovative solutions, meticulous attention to detail, and high-quality work, Mokhtar continually seeks new challenges within the dynamic field of technology.
Related posts
- Modulo Operator (%) In Python (Real-World Examples)
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
Python 3.8 Walrus Operator (Assignment Expression)
The release of Python 3.8 came with an exciting new feature: the Walrus Operator . It’s an assignment expression , or in simpler terms an assignment inside an expression.

Let’s dive into practice immediately and look at the following code:
We have a list of users whose data is represented in a dictionary. We want to count the number of approved users. The entry 'approved' is set to True if the user was approved. If the user wasn’t approved, the entry 'approved' can be False or absent.
Inside the for-loop we retrieve the value of 'approved' from the user dictionary first and assign it to the variable appr . Second, we use the if-statement to check if the value of appr is either True or False / None . Using the Walrus Operator we can merge these two steps into one so that our loop would look like this:
Try It Yourself:
Now you can see how we the assignment and the if-expression have become one single step in our code. And this is why it’s called assignment expression .
Applications
The Walrus Operator has a wide range of applications which we want to examine in the following paragraphs.
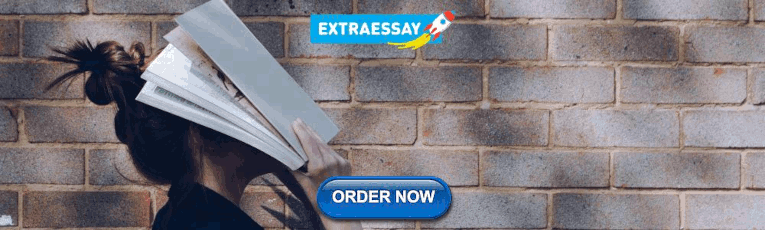
Walrus Operator with Regex
Regular Expressions are a powerful tool in programming. We even wrote an entire book about them! (Check out “The Smartest Way to Learn Python Regex” .)
Together with Regular Expressions the Walrus Operator can be used to check and assign a match in one line.
Here is the code:
I suggest, as an exercise, you try to rewrite the code from above without the walrus operator.
If you need a hint, check the first paragraph of this article.
Read File byte-wise Using the Walrus Operator
The Walrus Operator can be nicely used for reading chunks of data byte-wise from a file.
In this case it would be more complicated to rewrite the code without the Walrus Operator since it would require a while True loop and inside the loop you’d have to check what you read. If the file.read() method returns None you break out of the for-loop, else you process the chunk. So, here the Walrus Operator comes in very handy.
Walrus Operator in List Comprehensions
The Walrus Operator can be used in list comprehensions so that a function doesn’t have to be called multiple times.
In the if-statement of the list comprehension we call function f and assign the value to y if the result of f(x) makes the condition become True .
If we want to add the result of f(x) to the list we’d have to call f(x) again without the Walrus Operator or write a for-loop where we can use a temporary variable.
By using the Walrus Operator in a list comprehension we can avoid multiple function calls and reduce the lines of code .
Reuse a Value That Is Expensive to Compute
I consider this application of the Walrus Operator rather a curiosity but for the sake of completeness I’d like to mention it. In this case it reduces the number of lines of code that you have to write by one.
Instead of writing:
you can shorten the code by using the Walrus Operator to one single line:
I don’t recommend this use of the Walrus Operator since it reduces readability. It’s very easy now to miss the point where the initial value of y gets computed.
The Walrus Operator is a cool new feature but don’t over-use it. As we have seen in the examples above, it helps to write code faster because we need less code. However, readability is also very important since code is written only once but read multiple times. If you save a minute writing your code but then spend two hours debugging it, it’s clearly not worth it.
This ambiguity has also sparked controversy around the Walrus Operand and in my opinion, as many times in life, the middle way is the way to go.
Where to Go From Here?
Want to start earning a full-time income with Python—while working only part-time hours? Then join our free Python Freelancer Webinar .
It shows you exactly how you can grow your business and Python skills to a point where you can work comfortable for 3-4 hours from home and enjoy the rest of the day (=20 hours) spending time with the persons you love doing things you enjoy to do.
Become a Python freelancer now!
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
Python Walrus Operator: Syntax, Usage, Examples
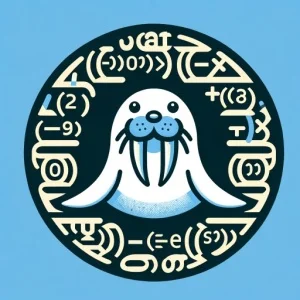
Are you finding the walrus operator in Python a bit puzzling? You’re not alone. Many developers find themselves scratching their heads when it comes to this unique operator. But, just like a walrus uses its tusks to break through ice, Python’s walrus operator can break through complex expressions, making your code more concise and readable.
Think of Python’s walrus operator as a multitasking hero – it allows you to assign values to variables as part of an expression, thereby reducing the lines of code and enhancing readability.
In this guide, we’ll walk you through the ins and outs of the walrus operator in Python , from its basic usage to more advanced applications. We’ll cover everything from the fundamentals of the walrus operator to its practical use cases, as well as alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering the Python walrus operator!
TL;DR: What is the Walrus Operator in Python?
The walrus operator := , also known as the assignment expression operator, is a new operator introduced in Python 3.8. It allows you to assign values to variables as part of an expression, thereby simplifying your code and making it more readable.
Here’s a simple example:
In this example, we’re using the walrus operator := to assign the length of some_list to the variable n as part of the if statement. This allows us to use n in the print statement that follows, thereby reducing the need for an extra line of code to assign n beforehand.
This is just a basic introduction to the walrus operator in Python. Continue reading for a more in-depth exploration of its usage and benefits.
Table of Contents
Simplifying Code with the Walrus Operator: A Beginner’s Guide
Advanced python walrus operator: list comprehensions and while loops, alternative approaches to the python walrus operator, troubleshooting the python walrus operator, python operators: the building blocks, the walrus operator: a unique addition to python, advanced python: walrus operator in larger projects, wrapping up: mastering the python walrus operator.
The walrus operator, also known as the assignment expression operator, is a powerful tool that can simplify your code by combining assignment and comparison in a single line. It’s like a two-in-one tool that not only assigns values to variables but also evaluates the expression at the same time.
Let’s look at a basic example:
In this example, n := len(my_list) is the walrus operator in action. It assigns the length of my_list to the variable n and then evaluates whether n is greater than 10. If the condition is true, it prints ‘The list has {n} elements.’
This is a simple but powerful way to streamline your code, making it more concise and readable. However, like any tool, the walrus operator should be used with care. Overuse can lead to code that is difficult to understand and maintain. So, while the walrus operator is a powerful tool, it’s important to use it judiciously and appropriately.
The walrus operator is not just for simple assignments and conditions. It can also be used in more complex scenarios, such as list comprehensions and while loops. Let’s dive deeper into its advanced usage.
Walrus Operator in List Comprehensions
List comprehensions are a powerful feature of Python that allow you to create lists in a very concise way. The walrus operator can be used in list comprehensions to make them even more efficient.
Consider the following example:
In this example, we’re using the walrus operator to assign the value of x to n and then check if n is even. If it is, n is added to the new list filtered_list . This allows us to create a filtered list in just one line of code.
Walrus Operator in While Loops
The walrus operator can also be used in while loops to both assign and check a condition in the same line. Let’s see an example:
In this example, we’re using the walrus operator to assign a random number between 0 and 10 to the variable number and then check if number is not equal to 5. If number is not 5, the loop continues and prints ‘The number {number} is not 5’. The loop stops when number is 5.
These examples show how the walrus operator can be used in more complex scenarios to make your code more efficient and readable. However, it’s important to remember that the walrus operator should be used judiciously. Overuse can lead to code that is difficult to understand and maintain.
While the walrus operator is a powerful tool, it’s not the only way to assign values to variables as part of an expression. There are several other techniques and operators that can accomplish the same task. Understanding these alternatives can help you make more informed decisions about which approach is best for your specific situation.
Traditional Assignment and Comparison
Before the introduction of the walrus operator, developers would typically use separate lines for assignment and comparison. Let’s look at an example:
In this example, we first assign the length of my_list to n , and then we check if n is greater than 10. Although this approach takes an extra line of code, it can be easier to understand for beginners and is less prone to errors.
Using Ternary Operator
The ternary operator is another approach that can be used to assign values to variables as part of an expression. Here’s how it works:
In this example, we’re using the ternary operator to assign the length of my_list to n if the length of my_list is greater than 10. Otherwise, n is assigned 0. This approach is more concise than the traditional assignment and comparison, but it can be more difficult to understand for beginners.
Each of these alternatives has its own benefits and drawbacks. The traditional assignment and comparison is easier to understand but takes more lines of code. The ternary operator is more concise but can be more difficult to understand. The walrus operator strikes a balance between these two, offering conciseness and readability. However, it’s important to remember that the best approach depends on your specific situation and personal preference.
Like any other programming feature, the Python walrus operator can sometimes lead to errors or obstacles. Understanding these common issues and their solutions can help you write more efficient and error-free code.
Unparenthesized Assignment
One common error when using the walrus operator is forgetting to enclose the assignment in parentheses. This can lead to unexpected results. Let’s look at an example:
In this example, we forgot to enclose n := len(my_list) in parentheses. As a result, n is assigned the result of the comparison len(my_list) > 10 , which is a boolean value. To avoid this error, always enclose the assignment in parentheses:
Using the Walrus Operator in Older Python Versions
The walrus operator was introduced in Python 3.8. If you’re using an older version of Python, you’ll get a syntax error when trying to use the walrus operator. To avoid this error, make sure to update to Python 3.8 or later.
Overusing the Walrus Operator
While the walrus operator can simplify your code, overusing it can lead to code that is difficult to understand and maintain. It’s important to use the walrus operator judiciously and only when it improves the readability of your code.
In conclusion, understanding these common issues and their solutions can help you avoid errors and write more efficient code with the Python walrus operator. Always remember to enclose assignments in parentheses, use Python 3.8 or later, and use the walrus operator judiciously.
Before we dive deeper into the walrus operator, let’s take a step back and understand the concept of operators in Python. Operators are special symbols in Python that carry out arithmetic or logical computation. They are the building blocks of any Python program and are used to manipulate data and variables.
There are several types of operators in Python, including arithmetic operators (like + , - , * , / ), comparison operators (like == , != , < , > ), logical operators (like and , or , not ), and assignment operators (like = , += , -= ). The walrus operator ( := ) is a type of assignment operator, also known as the assignment expression operator.
The walrus operator was introduced in Python 3.8 as a new type of assignment operator. Unlike other assignment operators, the walrus operator allows you to assign values to variables as part of an expression. This means you can assign a value to a variable and evaluate an expression in the same line of code.
This unique ability of the walrus operator to combine assignment and evaluation in a single expression makes it a powerful tool for simplifying and streamlining your Python code.
The walrus operator can be a powerful tool in larger scripts or projects. Its ability to simplify expressions and reduce lines of code can make your code more efficient and easier to read. However, it’s important to remember that the walrus operator is just one tool in the Python toolbox. There are many other operators and functions that often accompany the walrus operator in typical use cases.
For example, the walrus operator can be used in conjunction with the for loop and the if statement to filter elements in a list. Here’s an example:
Further Resources for Mastering Python Operators
If you’re interested in learning more about the walrus operator and other Python operators, here are a few resources that might help:
- Python If Statements – Learn how to troubleshoot and debug code with if statements for error resolution.
Using “if not” in Python – A guide to negation and cheking absence of values in conditionals using “if not”.
Python’s Boolean Operations – An overview on using boolean variables and expressions to create logic in Python programs.
Python’s official documentation on assignment expressions provides a detailed explanation of the walrus operator’s syntax and usage.
Real Python’s Guide to Python operators offers a comprehensive overview of all types of operators in Python.
Python’s official tutorial on control flow tools discusses how the walrus operator can be used in conjunction with other control flow tools.
In this comprehensive guide, we’ve delved into the depths of the Python walrus operator, a powerful tool for simplifying and streamlining your code.
We began with the basics, understanding the purpose and functionality of the walrus operator. We then explored its basic usage, learning how it can combine assignment and comparison in a single line of code. We ventured into more advanced territory, examining how the walrus operator can be used in complex scenarios like list comprehensions and while loops.
Along the way, we tackled common challenges that you might encounter when using the walrus operator, such as unparenthesized assignments and compatibility with older Python versions, providing you with solutions to overcome these hurdles.
We also looked at alternative approaches to the walrus operator, comparing it with traditional assignment and comparison methods, and the ternary operator.
Here’s a quick comparison of these methods:
Whether you’re just starting out with the Python walrus operator or you’re looking to level up your Python skills, we hope this guide has given you a deeper understanding of the walrus operator and its capabilities.
With its unique ability to combine assignment and evaluation in a single expression, the Python walrus operator is a powerful tool for efficient and readable coding. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
Related Posts
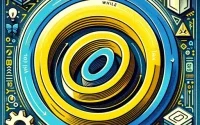
Python's walrus operator

Sign in to change your settings
Sign in to your Python Morsels account to save your screencast settings.
Don't have an account yet? Sign up here .
Let's talk about Python's walrus operator .
An assignment followed by a conditional check
We have a function called get_quantitiy that accepts a string argument which represents a number and a unit (either kilograms or grams):
When we call this function it returns a tuple with 2 items: the number (converted to an integer) and the unit.
If we give this function a string representing just a number (no units), it will give us that number back converted to an integer:
This get_quantitiy function assumes that whatever we give to it is either the pattern (number and unit) or just an integer.
We're doing this using regular expressions , which are a form of pattern matching:
We're not going to get into regular expressions right now.
Instead we're going to focus on these two lines of code (from get_quantity ):
On the first line, the match variable stores either a match object or None .
Then if match asks the question "did we get something that it truthy ?"
A match object is truthy and None is falsey, so we're basically checking whether we got a match object to work with.
Those two lines above (the assignment to match and the conditional check based on match ) can actually be combined into one line of code .
Embedding an assignment into another line with assignment expressions
We can take these two lines of code:
And combine them into one line of code using an assignment expression ( new in Python 3.8 ):
Before we had an assignment statement and a condition (that were checking in our if statement ). Now we have both in one line of code .
We're using the walrus operator , which is the thing that powers assignment expressions.
Assignment expressions allow us to embed an assignment statement inside of another line of code. They use walrus operator ( := ):
Which is different from a plain assignment statement ( = ) because an assignment statement has to be on a line all on its own:
The := is called the walrus operator because it looks kind of like a walrus on its side: the colon looks sort of like eyes and the equal sign looks kind of like tusks.
Checking to see if we got a match object when using regular expressions in Python is a very common use of the walrus operator.
A common use case for the walrus operator
Another common use case for the walrus operator is in a while loop.
Specifically it's common to see a walrus operator used in a while loop that repeatedly:
- Stores a value based on an expression
- Checks a condition based on that value
With the walrus operator we can perform both of those actions at the same time.
We have a function called compute_md5 :
This function takes a file name and gives us back the MD5 checksum of that file:
We might use a function like this if we were trying to check for duplicate files or verify that a large file downloaded accurately.
We're not going to focus on the details of this function though. We care about what is the walrus operator doing in this compute_md5 function and what's the alternative of the walrus operator here?
We're repeatedly reading eight kilobytes (8192 bytes) into the chunk variable:
The alternative to this is to assign to the chunk variable before our loop, check the value of chunk in our loop condition, and also assign to chunk at the end of each loop iteration:
I would argue that the using an assignment expression makes this code more readable than the alternative because we've taken what was three lines of code and turned it into just one line.
In each iteration of our loop we're grabbing a chunk, checking its truthiness (to see whether we've reached the end of the file) and assigning that chunk to the chunk variable. And we're doing all of this in just one line of code:
Assignment expressions use the walrus operator ( := ).
Assignment expressions are a way of taking an assignment statement and embedding it in another line of code . I don't recommend using them unless they make your code more readable.
A Python tip every week
Need to fill-in gaps in your Python skills?
Sign up for my Python newsletter where I share one of my favorite Python tips every week .
Series: Assignment and Mutation
Python's variables aren't buckets that contain things; they're pointers that reference objects.
The way Python's variables work can often confuse folks new to Python, both new programmers and folks moving from other languages like C++ or Java.
To track your progress on this Python Morsels topic trail, sign in or sign up .
Need to fill-in gaps in your Python skills ? I send weekly emails designed to do just that.
Python's for loops do an assignment for each iteration of the loop, import statements do an assignment, and function definitions even do an assignment.
- [email protected]
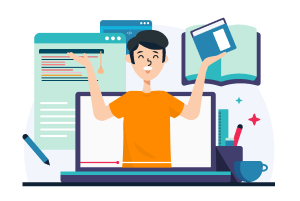
What’s New ?
The Top 10 favtutor Features You Might Have Overlooked

- Don’t have an account Yet? Sign Up
Remember me Forgot your password?
- Already have an Account? Sign In
Lost your password? Please enter your email address. You will receive a link to create a new password.
Back to log-in
By Signing up for Favtutor, you agree to our Terms of Service & Privacy Policy.
Walrus Operator in Python: When to Use It? (with Examples)
- Feb 15, 2023
- 6 Minute Read
- Why Trust Us We uphold a strict editorial policy that emphasizes factual accuracy, relevance, and impartiality. Our content is crafted by top technical writers with deep knowledge in the fields of computer science and data science, ensuring each piece is meticulously reviewed by a team of seasoned editors to guarantee compliance with the highest standards in educational content creation and publishing.
- By Komal Gupta
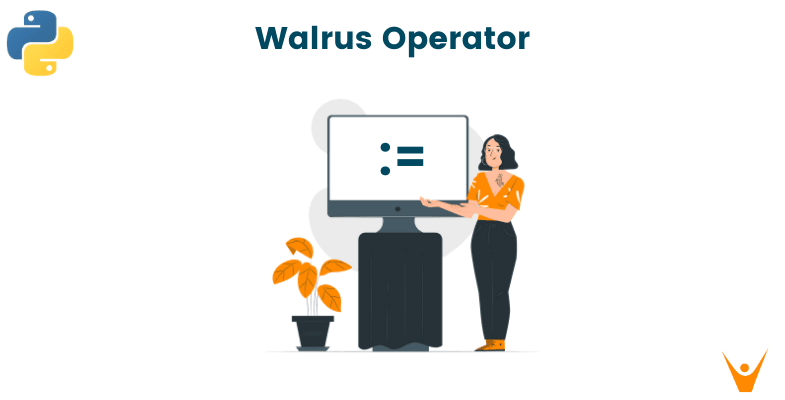
Greetings, Pythonolic, Are you eager to discover something new about python today? We have all used mathematical operators and functions that are familiar to us all, such as logical operators, identical operators, etc. Today, we will talk about a new Operator known as Walrus Operator and how does it work?
What is Walrus Operator in Python?
Walrus Operator in Python allows you to assign values to variables as part of an expression. It was first made available in Python 3..8 and later. In layman's terms, it combines Python's Assignment and Equality operators in a single line of code.
Here is the syntax:
variable := expression
It got its name from the operator symbol (:=) mimicking the eyes and tusks of a sideways walrus (colon equals operator).
The biggest advantage of the walrus operator is to make writing codes easier. In the past, you had to collect each user input into a distinct variable before passing it to the for loop to check its value or apply a condition. The walrus operator cannot be used as a stand-alone statement; this is a crucial distinction to make.
First, we will print a list of numbers until its value is equal to 0, with a simple while loop.
We can perform the same task with the use of a walrus operator:
The value of the integer is reported and then updated using the walrus operator (:=) on each iteration of the loop. When a number is updated by the walrus operator (:=), it is decremented by 1. The number is updated and given a new value using the phrase (number:=number - 1). The expression is not required to be enclosed in parentheses, and doing so has no impact on the code.
The cycle repeats until the value of the number drops to or equals 0. The loop then ends, and the program is finished.
You might be wondering why we enclosed the walrus operator line of code in parenthesis. This is because python will give you a syntax error if you try to use the walrus operator as the assignment operator. It is designed in a way that prevents you from using it as an assignment operator, but you can if you add parentheses.
The visual similarity between the assignment operator (=) and the equality comparison operator (==) might potentially result in defects, which is one of the primary reasons assignments were never considered expressions in python from the start. To prevent problems with the walrus operator, much thought went into the introduction of assignment expressions.
The fact that the (:=) is a crucial component is that the walrus operator is never allowed as a direct replacement for the = operator, and vice versa.
When to use the Walrus Operator?
You have two options either going with the assignment operator or the walrus. But both are used in different scenarios. The assignment operator (=) just assigns a value to a variable for future use, whereas the walrus operator (:=) evaluates the walrus operator expression and assigns the result to the variable name. Also returning will be that value.
As we mentioned, it is great for simplifying the code in the following situations:
- Repeated function calls can make your code slower than necessary.
- Repeated statements can make your code hard to maintain.
- Repeated calls that exhaust iterators can make your code overly complex
It also simplifies the process of iterating over a list or a dictionary in python. Check the code below:
Output:
In this code, the walrus operator is used in the if statement to both assign the value of i + n to the variable j and test the condition j > 3 in the same line. If the condition is true, the code prints the value of j.
Situations where it can’t be used
The walrus operator is not supported inside lambda functions, as lambda functions are limited in scope and do not allow assignments. Check the example:
Another thing to note about the walrus operator has not supported inside list or dictionary comprehensions, as these are expressions that generate new lists or dictionaries, rather than statements that execute arbitrary code. Check the example:
Just remember before using this operator that it only works in python 3.8 or above versions. There are many times it’s possible for you to use the walrus operator, but where it won’t necessarily improve the readability or efficiency of your code. In those cases, you’re better off writing your code in a more traditional manner.
We learned about what is python's walrus operator and where it should and shouldn't be used. It is a valuable addition to the language that can make your code more concise. It is especially useful for tasks such as updating variables within loops and iterating over lists and dictionaries. Happy Learning :)
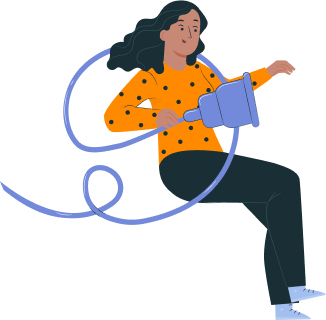
FavTutor - 24x7 Live Coding Help from Expert Tutors!
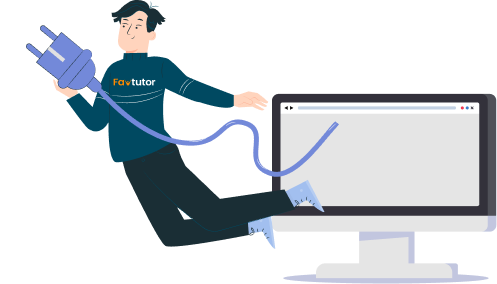
About The Author
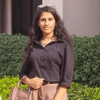
Komal Gupta
More by favtutor blogs, monte carlo simulations in r (with examples), aarthi juryala.
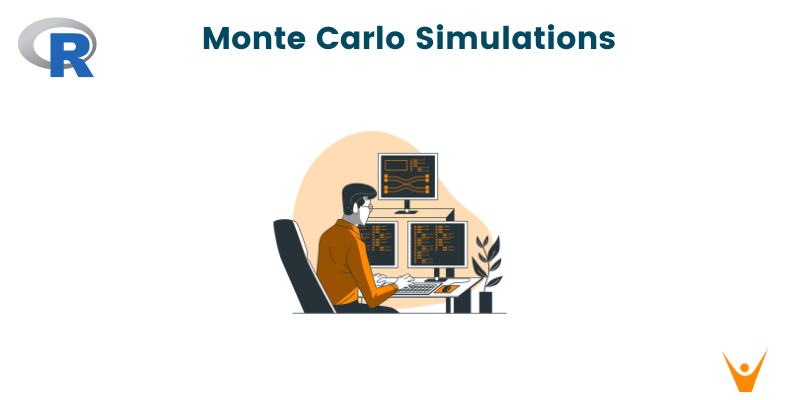
The unlist() Function in R (with Examples)
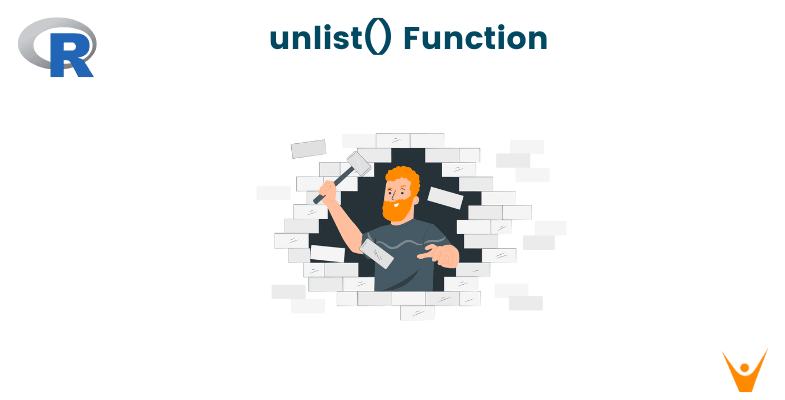
Paired Sample T-Test using R (with Examples)
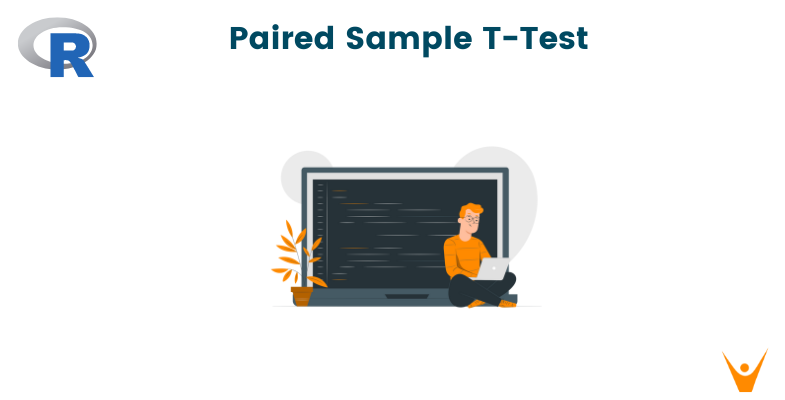
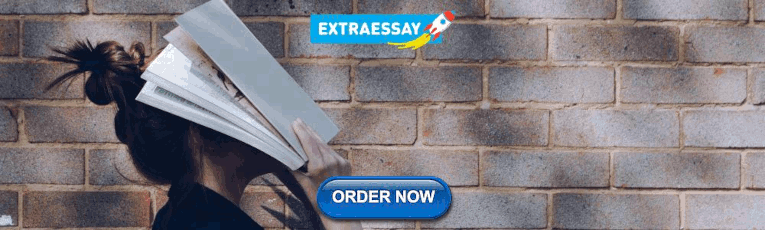
IMAGES
VIDEO
COMMENTS
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This tutorial is an in-depth introduction to the walrus operator.
In this lesson, you'll learn about the biggest change in Python 3.8: the introduction of assignment expressions.Assignment expression are written with a new notation (:=).This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.. Assignment expressions allow you to assign and return a value in the same expression.
Since Python 3.8, code can use the so-called "walrus" operator (:=), documented in PEP 572, for assignment expressions.This seems like a really substantial new feature, since it allows this form of assignment within comprehensions and lambdas.. What exactly are the syntax, semantics, and grammar specifications of assignment expressions?
The Walrus Operator is a new addition to Python 3.8 and higher. In this article, we're going to discuss the Walrus operator and explain it with an example. Introduction. Walrus Operator allows you to assign a value to a variable within an expression. This can be useful when you need to use a value multiple times in a loop, but don't want to ...
enter the walrus operator. Introduced in python 3.8, the walrus operator, (:=), formally known as the assignment expression operator, offers a way to assign to variables within an expression, including variables that do not exist yet.As seen above, with the simple assignment operator (=), we assigned num = 15 in the context of a stand-alone statement.
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions. Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator. This course is an in-depth introduction ...
The walrus operator, or assignment expression, introduced in Python 3.8, provides a powerful and concise way to assign values to variables as part of an expression. By using the walrus operator, you can simplify your code, improve its readability, and eliminate redundant computations.
The walrus operator := (assignment operator) is a feature that helps Python programmers: avoid repeated lines of code. reuse a value that is computationally expensive to compute. improve readability with compact code (It depends. Don't overuse it) Thanks for taking the time to read my article. You can connect with me on LinkedIn.
In this article, I will talk about the walrus operator in Python. The biggest change in Python is the introduction of assignment expressions, also known as walrus operator. Assignment expression can be defined as a Python expression that allows you to assign and return a value in the same expression. Table of Contents
The "Python walrus operator", officially known as the assignment expression operator, was introduced in Python 3.8. It's symbolized by a colon followed by an equal sign :=. The Python community refers to it as the "walrus operator" due to its resemblance to a pair of eyes and tusks, like that of a walrus. Table of Contents hide.
Unparenthesized assignment expressions are prohibited for the value of a keyword argument in a call. Example: foo(x = y := f(x)) # INVALID foo(x=(y := f(x))) # Valid, though probably confusing. This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
Python 3.8, which was released sometime in the Fall of 2019, has added a feature that we call assignment expression. This allows us to assign values to variables in the middle of expressions. Assignment expressions were made possible with the help of the walrus operator. What's this walrus operator?
The release of Python 3.8 came with an exciting new feature: the Walrus Operator. It's an assignment expression, or in simpler terms an assignment inside an expression. Python 3.8 Walrus Operator (Assignment Expression) Let's dive into practice immediately and look at the following code: users = [. {'name': 'Alice', 'uid': 123, 'approved ...
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This course is an in-depth introduction to the walrus operator.
With the release of Python 3.8, the assignment-expression operator—also known as the walrus operator—was released. The operator enables the assignment of a value to be passed into an expression. This generally reduces the number of statements by one. For example:
Python 3.8 introduces a new feature called assignment expressions, which is represented by the `:=` operator. This operator is commonly referred to as the "walrus operator." In this tutorial ...
The walrus operator :=, also known as the assignment expression operator, is a new operator introduced in Python 3.8. It allows you to assign values to variables as part of an expression, thereby simplifying your code and making it more readable.
We're using the walrus operator, which is the thing that powers assignment expressions. Assignment expressions allow us to embed an assignment statement inside of another line of code. They use walrus operator ( := ): if match := UNITS_RE.search(string): Which is different from a plain assignment statement ( =) because an assignment statement ...
In layman's terms, it combines Python's Assignment and Equality operators in a single line of code. Here is the syntax: variable := expression. It got its name from the operator symbol (:=) mimicking the eyes and tusks of a sideways walrus (colon equals operator). The biggest advantage of the walrus operator is to make writing codes easier.
This will result in a SyntaxError: cannot use assignment expressions with tuple. And that's the whole point: Currently the walrus operator doesn't allow unpacking assignment. I also have no information about whether this is planned for the future or not. (Maybe there is even a logical reason I couldn't figure out why this isn't possible at all.)
105. This symbol := is an assignment operator in Python (mostly called as the Walrus Operator ). In a nutshell, the walrus operator compresses our code to make it a little shorter. Here's a very simple example: # without walrus. n = 30. if n > 10: print(f"{n} is greater than 10") # with walrus.
In this course, you learned how to: Convert between code using the walrus operator and code using other assignment methods. Understand the impacts on backward compatibility when using the walrus operator. To learn more about the details of assignment expressions, see PEP 572. You can also check out the PyCon 2019 talk PEP 572: The Walrus ...