- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- C Exercises - Practice Questions with Solutions for C Programming
- C Programming Interview Questions (2024)
- Top 25 C Projects with Source Code in 2023
- Top 50 C Coding Interview Questions and Answers (2024)
- C | Functions | Question 6
- C | Functions | Question 2
- C | Pointer Basics | Question 13
- C Programming For Beginners - A 20 Day Curriculum!
- C | Pointer Basics | Question 1
- C | Functions | Question 7
- C | Data Types | Question 9
- C | Advanced Pointer | Question 10
- C | Loops & Control Structure | Question 4
- C | Loops & Control Structure | Question 1
- C | Loops & Control Structure | Question 3
- C++ Exercises - C++ Practice Set with Solutions
- Zoho Interview Experience | Set 27 (Off-Campus for Software Developer)
- TCS Interview Experience | Set 15 - New 2017 Pattern
- WD-Sandisk Interview Experience
- Zoho Interview Experience | Set 17
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Bitwise Operators in C
- std::sort() in C++ STL
- What is Memory Leak? How can we avoid?
- Substring in C++
- Segmentation Fault in C/C++
- Socket Programming in C
- For Versus While
- Data Types in C
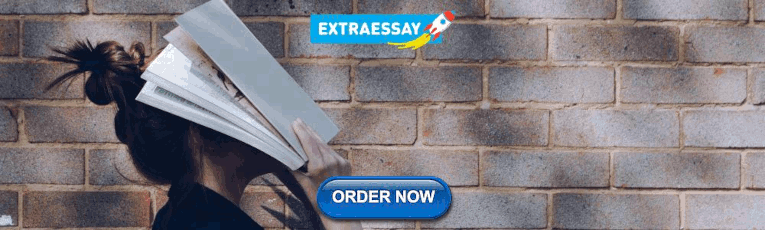
C Exercises – Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
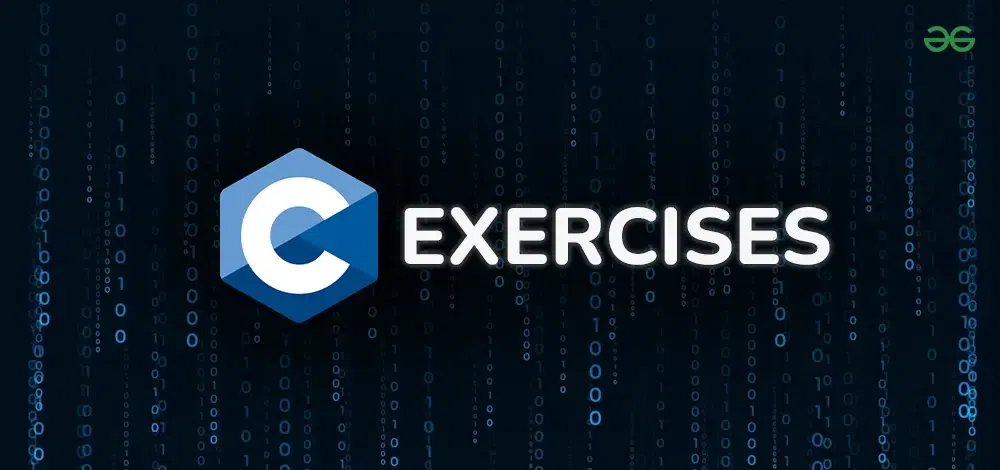
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
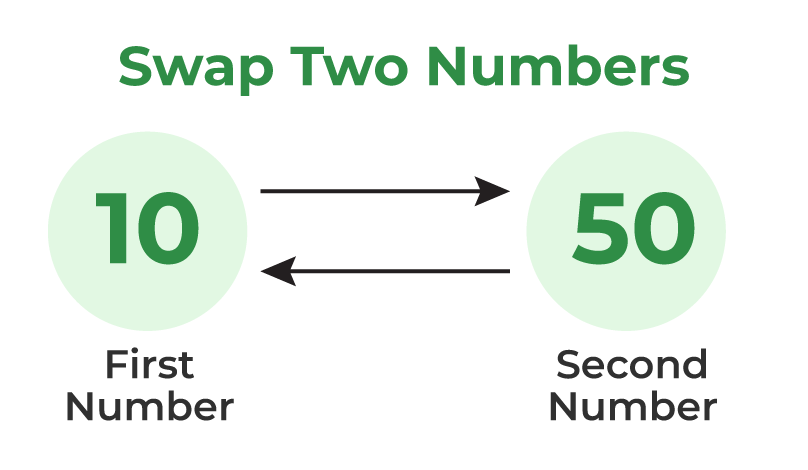
Swap two numbers
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
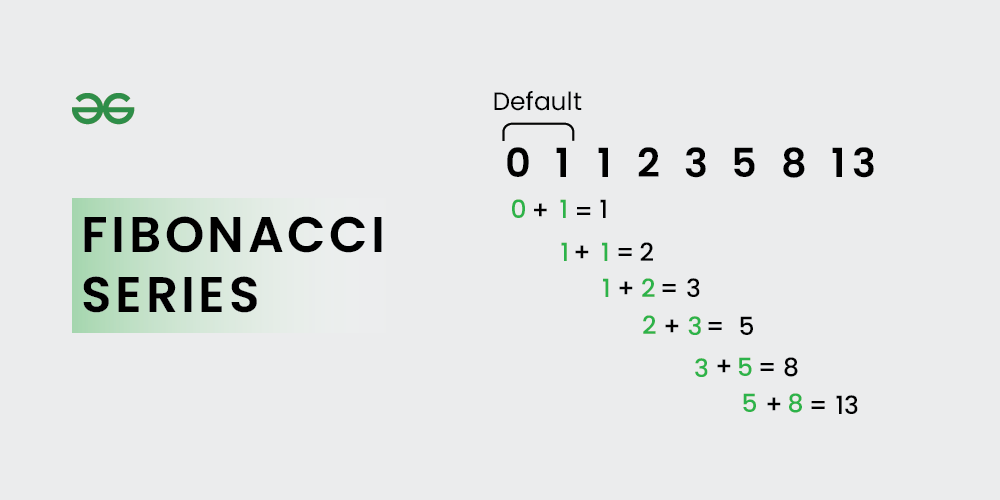
Fibonacci Series
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
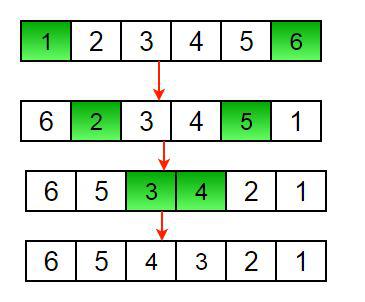
Reverse an array
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array ‘.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
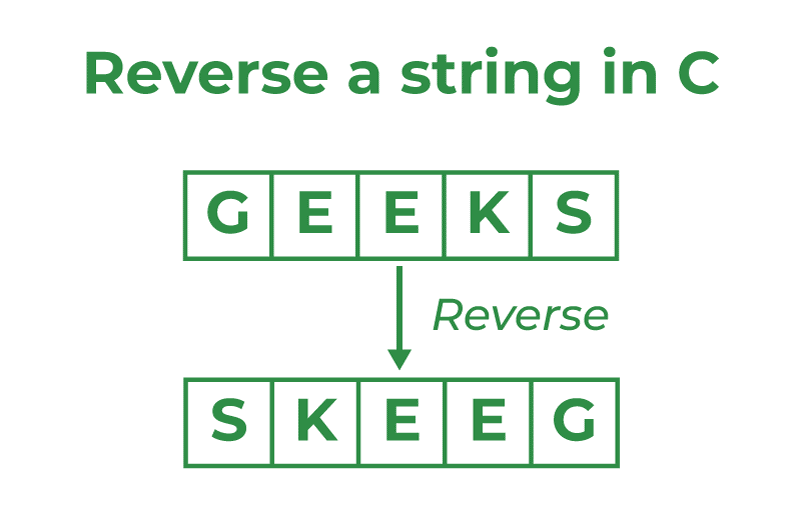
reverse a string
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
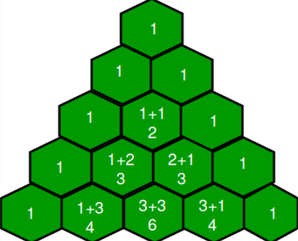
Pascal’s Triangle
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Please Login to comment...
Similar reads.
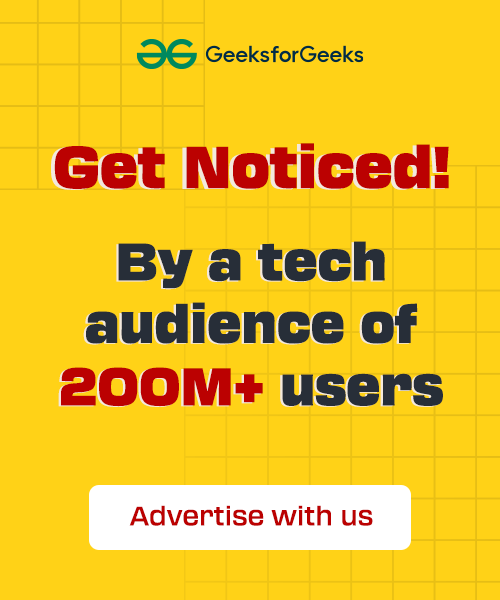
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- C All Exercises & Assignments
Write a C program to check whether a number is even or odd
Description:
Write a C program to check whether a number is even or odd.
Note: Even number is divided by 2 and give the remainder 0 but odd number is not divisible by 2 for eg. 4 is divisible by 2 and 9 is not divisible by 2.
Conditions:
- Create a variable with name of number.
- Take value from user for number variable.
Enter the Number=9 Number is Odd.
Write a C program to swap value of two variables using the third variable.
You need to create a C program to swap values of two variables using the third variable.
You can use a temp variable as a blank variable to swap the value of x and y.
- Take three variables for eg. x, y and temp.
- Swap the value of x and y variable.
Write a C program to check whether a user is eligible to vote or not.
You need to create a C program to check whether a user is eligible to vote or not.
- Minimum age required for voting is 18.
- You can use decision making statement.
Enter your age=28 User is eligible to vote
Write a C program to check whether an alphabet is Vowel or Consonant
You need to create a C program to check whether an alphabet is Vowel or Consonant.
- Create a character type variable with name of alphabet and take the value from the user.
- You can use conditional statements.
Enter an alphabet: O O is a vowel.
Write a C program to find the maximum number between three numbers
You need to write a C program to find the maximum number between three numbers.
- Create three variables in c with name of number1, number2 and number3
- Find out the maximum number using the nested if-else statement
Enter three numbers: 10 20 30 Number3 is max with value of 30
Write a C program to check whether number is positive, negative or zero
You need to write a C program to check whether number is positive, negative or zero
- Create variable with name of number and the value will taken by user or console
- Create this c program code using else if ladder statement
Enter a number : 10 10 is positive
Write a C program to calculate Electricity bill.
You need to write a C program to calculate electricity bill using if-else statements.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements.
Enter the units consumed=278.90 Electricity Bill=1282.84 Rupees
Write a C program to print 1 to 10 numbers using the while loop
You need to create a C program to print 1 to 10 numbers using the while loop
- Create a variable for the loop iteration
- Use increment operator in while loop
1 2 3 4 5 6 7 8 9 10
- C Exercises Categories
- C Top Exercises
- C Decision Making
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively.
The best way to learn C programming is by practicing examples. The page contains examples on basic concepts of C programming. You are advised to take the references from these examples and try them on your own.
All the programs on this page are tested and should work on all platforms.
Want to learn C Programming by writing code yourself? Enroll in our Interactive C Course for FREE.
- C Program to Create Pyramids and Patterns
- C Program to Check Prime Number
- C Program to Check Palindrome Number
- C Program to Print Hello World
- C "Hello, World!" Program
- C Program to Print an Integer (Entered by the User)
- C Program to Add Two Integers
- C Program to Multiply Two Floating-Point Numbers
- C Program to Find ASCII Value of a Character
- C Program to Compute Quotient and Remainder
- C Program to Find the Size of int, float, double and char
- C Program to Demonstrate the Working of Keyword long
- C Program to Swap Two Numbers
- C Program to Check Whether a Number is Even or Odd
- C Program to Check Whether a Character is a Vowel or Consonant
- C Program to Find the Largest Number Among Three Numbers
- C Program to Find the Roots of a Quadratic Equation
- C Program to Check Leap Year
- C Program to Check Whether a Number is Positive or Negative
- C Program to Check Whether a Character is an Alphabet or not
- C Program to Calculate the Sum of Natural Numbers
- C Program to Find Factorial of a Number
- C Program to Generate Multiplication Table
- C Program to Display Fibonacci Sequence
- C Program to Find GCD of two Numbers
- C Program to Find LCM of two Numbers
- C Program to Display Characters from A to Z Using Loop
- C Program to Count Number of Digits in an Integer
- C Program to Reverse a Number
- C Program to Calculate the Power of a Number
- C Program to Check Whether a Number is Palindrome or Not
- C Program to Check Whether a Number is Prime or Not
- C Program to Display Prime Numbers Between Two Intervals
- C Program to Check Armstrong Number
- C Program to Display Armstrong Number Between Two Intervals
- C Program to Display Factors of a Number
- C Program to Make a Simple Calculator Using switch...case
- C Program to Display Prime Numbers Between Intervals Using Function
- C Program to Check Prime or Armstrong Number Using User-defined Function
- C Program to Check Whether a Number can be Expressed as Sum of Two Prime Numbers
- C Program to Find the Sum of Natural Numbers using Recursion
- C Program to Find Factorial of a Number Using Recursion
- C Program to Find G.C.D Using Recursion
- C Program to Convert Binary Number to Decimal and vice-versa
- C Program to Convert Octal Number to Decimal and vice-versa
- C Program to Convert Binary Number to Octal and vice-versa
- C Program to Reverse a Sentence Using Recursion
- C program to calculate the power using recursion
- C Program to Calculate Average Using Arrays
- C Program to Find Largest Element in an Array
- C Program to Calculate Standard Deviation
- C Program to Add Two Matrices Using Multi-dimensional Arrays
- C Program to Multiply Two Matrices Using Multi-dimensional Arrays
- C Program to Find Transpose of a Matrix
- C Program to Multiply two Matrices by Passing Matrix to a Function
- C Program to Access Array Elements Using Pointer
- C Program Swap Numbers in Cyclic Order Using Call by Reference
- C Program to Find Largest Number Using Dynamic Memory Allocation
- C Program to Find the Frequency of Characters in a String
- C Program to Count the Number of Vowels, Consonants and so on
- C Program to Remove all Characters in a String Except Alphabets
- C Program to Find the Length of a String
- C Program to Concatenate Two Strings
- C Program to Copy String Without Using strcpy()
- C Program to Sort Elements in Lexicographical Order (Dictionary Order)
- C Program to Store Information of a Student Using Structure
- C Program to Add Two Distances (in inch-feet system) using Structures
- C Program to Add Two Complex Numbers by Passing Structure to a Function
- C Program to Calculate Difference Between Two Time Periods
- C Program to Store Information of Students Using Structure
- C Program to Store Data in Structures Dynamically
- C Program to Write a Sentence to a File
- C Program to Read the First Line From a File
- C Program to Display its own Source Code as Output
- C Program to Print Pyramids and Patterns
"Hello World!" in C Easy C (Basic) Max Score: 5 Success Rate: 85.68%
Playing with characters easy c (basic) max score: 5 success rate: 84.24%, sum and difference of two numbers easy c (basic) max score: 5 success rate: 94.58%, functions in c easy c (basic) max score: 10 success rate: 95.95%, pointers in c easy c (basic) max score: 10 success rate: 96.54%, conditional statements in c easy c (basic) max score: 10 success rate: 96.94%, for loop in c easy c (basic) max score: 10 success rate: 93.66%, sum of digits of a five digit number easy c (basic) max score: 15 success rate: 98.65%, bitwise operators easy c (basic) max score: 15 success rate: 94.87%, printing pattern using loops medium c (basic) max score: 30 success rate: 95.90%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
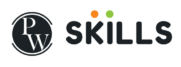
C Programming Examples for Beginners With Solutions & Output
C Programming Examples include many programs, from beginner-level examples like "Hello World" and "Sum of Two Numbers" to more advanced programs like the Fibonacci series and Prime Numbers.
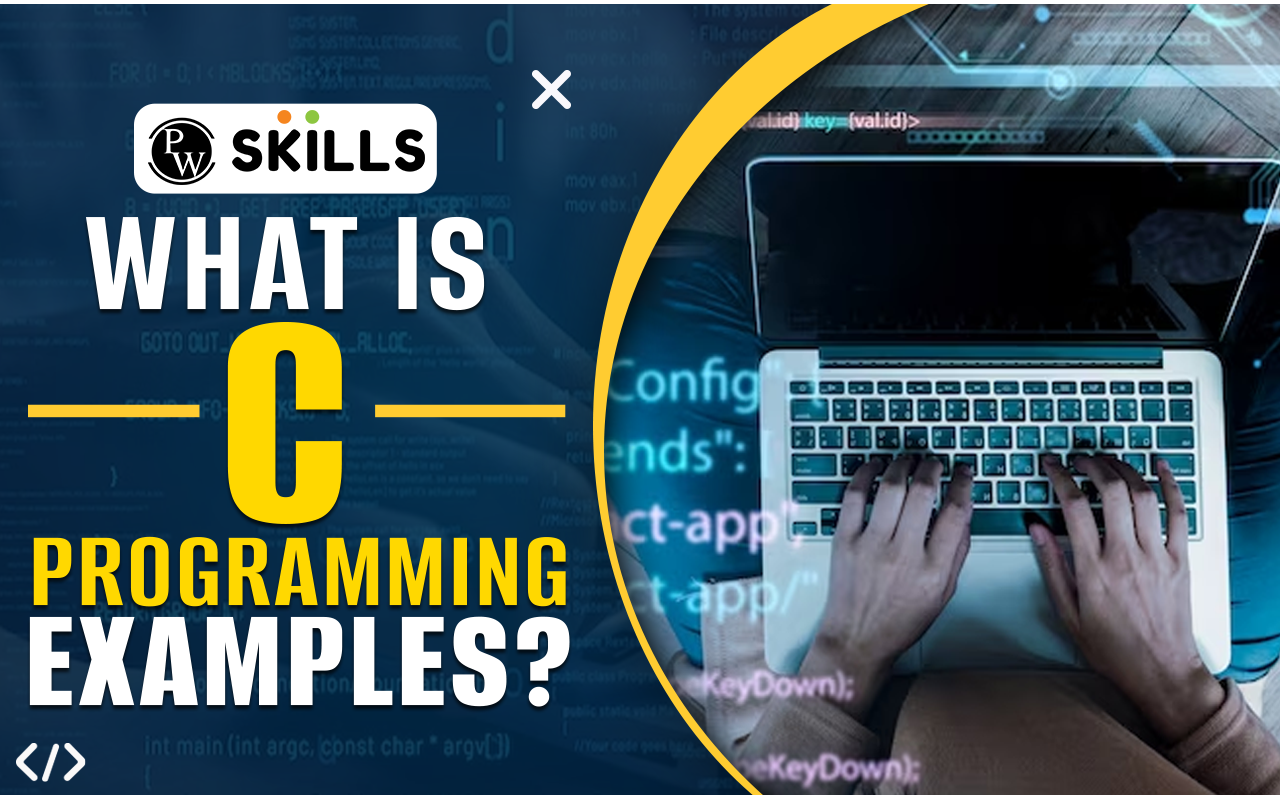
C Programming Examples: Are you just starting out in C programming but feeling overwhelmed? Maybe you’ve been working through the basics of C programming for a while, but are now looking to take your skills to the next level. If so, we can definitely relate!
Here, we share C programs covering various topics in C Programming, including arrays, strings, series, area & volume of geometrical figures, mathematical calculations, sorting & searching algorithms, and many more. Our goal is to provide comprehensive solutions to all C programming questions you may encounter, whether in interviews or class assignments.
Each program in this article is accompanied by its working code and output. The programs are organized into categories, with related programs grouped together. It is recommended to grasp the fundamentals of the C language through our C tutorial before delving into these C programs. You can also download our C programming examples PDF to get instant access to C programming examples . So, whether you’re just setting out or have already made some progress in your learning adventure, this will be the perfect resource guide for honing your C coding skills!
If you find learning C language challenging, consider exploring our new C course from Physics Wallah . This interactive learning experience involves understanding a concept, completing small coding exercises, and progressing to the next lesson. Use “READER” coupon to get instant discounts on PW courses.
Also read: C Programming Language History, Invention, Timeline & More
Table of Contents
C Programming Examples for Beginners
Below, we will share C programming examples for beginners. You can also download C language programs PDF .
1. C Hello World Program
#include <stdio.h>
int main() {
printf(“Hello, World!\n”);
2. C Program to Print Your Own Name
printf(“Your Name\n”);
3. C Program to Print an Integer Entered By the User
printf(“Enter an integer: “);
scanf(“%d”, &num);
printf(“You entered: %d\n”, num);
4. C Program to Check Whether a Number is Prime or Not
int num, i, flag = 0;
printf(“Enter a number: “);
for (i = 2; i <= num / 2; ++i) {
if (num % i == 0) {
if (flag == 0)
printf(“%d is a prime number.\n”, num);
printf(“%d is not a prime number.\n”, num);
Also read: Top 30 Most Asked Basic Programming Questions Asked During Interviews
C Programming Examples With Solutions
Here are some C programming examples with solutions and codes:
1. C Program to Multiply two Floating-Point Numbers
float num1, num2, product;
printf(“Enter two floating-point numbers: “);
scanf(“%f %f”, &num1, &num2);
product = num1 * num2;
printf(“Product: %f\n”, product);
2. C Program to Print the ASCII Value of a Character
printf(“Enter a character: “);
scanf(“%c”, &ch);
printf(“ASCII value of %c = %d\n”, ch, ch);
3. C Program to Swap Two Numbers
int num1, num2, temp;
printf(“Enter two numbers: “);
scanf(“%d %d”, &num1, &num2);
temp = num1;
num1 = num2;
num2 = temp;
printf(“After swapping: num1 = %d, num2 = %d\n”, num1, num2);
4. C Program to Calculate Fahrenheit to Celsius
float fahrenheit, celsius;
printf(“Enter temperature in Fahrenheit: “);
scanf(“%f”, &fahrenheit);
celsius = (fahrenheit – 32) * 5 / 9;
printf(“Temperature in Celsius: %f\n”, celsius);
5. C Program to Find the Size of int, float, double, and char
printf(“Size of int: %d bytes\n”, sizeof(int));
printf(“Size of float: %d bytes\n”, sizeof(float));
printf(“Size of double: %d bytes\n”, sizeof(double));
printf(“Size of char: %d byte\n”, sizeof(char));
6. C Program to Print Prime Numbers From 1 to N
int i, j, n;
printf(“Enter a number (N): “);
scanf(“%d”, &n);
printf(“Prime numbers between 1 and %d are: “, n);
for (i = 2; i <= n; ++i) {
int isPrime = 1;
for (j = 2; j <= i / 2; ++j) {
if (i % j == 0) {
isPrime = 0;
if (isPrime)
printf(“%d “, i);
Also read: 10 Best Programming Languages for Game Development in 2024
C Programming Examples With Output
Learners can also download c programming examples with output PDF to start their C language journey. But practice is the key to learning C language properly. Here are some of the best C programming examples with output :
1. C Program to Check Whether a Number is Positive, Negative, or Zero
if (num > 0)
printf(“Positive number\n”);
else if (num < 0)
printf(“Negative number\n”);
printf(“Zero\n”);
Enter a number: 7
Positive number
2. C Program to Check Whether Number is Even or Odd
if (num % 2 == 0)
printf(“Even number\n”);
printf(“Odd number\n”);
Enter a number: 15
3. C Program to Calculate Sum of Natural Numbers
int n, sum = 0;
printf(“Enter a positive integer: “);
for (int i = 1; i <= n; ++i) {
printf(“Sum of natural numbers from 1 to %d: %d\n”, n, sum);
Enter a positive integer: 5
Sum of natural numbers from 1 to 5: 15
4. C Program to Print Alphabets From A to Z Using Loop
printf(“Alphabets from A to Z:\n”);
for (ch = ‘A’; ch <= ‘Z’; ++ch) {
printf(“%c “, ch);
Alphabets from A to Z:
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Recommended Technical Course
- Full Stack Development Course
- Generative AI Course
- DSA C++ Course
- Data Analytics Course
- Python DSA Course
- DSA Java Course
C Programs for Practice
Below table shows the C programming code that you can use for practice:
Why Choose C Language for Learning?
C language is an excellent entry point into the programming world due to its simplicity and ease of learning. While certain concepts may pose challenges, overall, learners find C language accessible. It introduces fundamental programming concepts like data types, variables, functions, arrays, strings, conditional statements, loops, input/output, and data structures—all foundational principles applicable to various modern programming languages.
For newcomers, acquiring proficiency in C/C++ is crucial for success in college placement interviews, especially with service-based companies such as TCS, Accenture, IBM, etc., which actively seek C developers.
Highly Efficient:
C language is renowned for its efficiency and performance. Programs written in C execute swiftly and optimize system resources effectively.
System-level Programming:
Offering low-level control over a computer’s hardware and memory, C language is well-suited for system-level programming and the development of operating systems.
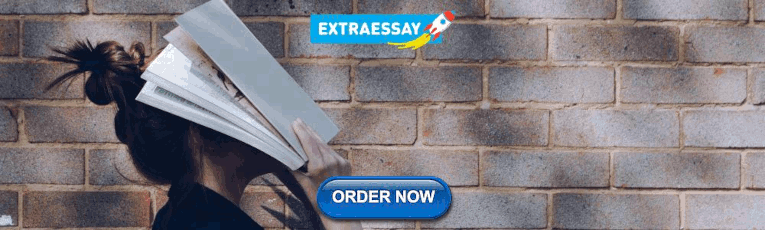
Portability:
C language is favored for its portability; its code can be compiled and executed on various platforms with minimal modifications. This makes C an ideal choice to run programs on diverse platforms.
Easy to Learn:
With a small learning curve, C language involves fewer keywords and concepts. Its syntax is easy to remember and apply. The C compiler provides descriptive errors, simplifying the debugging process and making it particularly beginner-friendly.
Versatility:
C language’s versatility allows for creating both small utility software and large-scale enterprise applications. Its broad applicability makes it a valuable language to master in programming.
Benefits of Practicing C Programming Examples
Practicing C Programming Examples offers several benefits for learners and aspiring programmers:
- Concept Reinforcement: By working on C programming examples, individuals reinforce fundamental programming concepts such as variables, loops, conditionals, and functions. This hands-on practice helps solidify theoretical knowledge.
- Preparation for Interviews: Many technical interviews, especially for entry-level programming positions, involve solving coding problems. Practicing C programming examples prepares individuals for such interviews, helping them perform well and showcase their coding proficiency.
- Application of Knowledge: C programming examples provide a practical platform for applying theoretical knowledge gained from textbooks or tutorials. This application-oriented learning approach ensures a deeper understanding of programming concepts.
- Syntax Mastery: Working on examples allows learners to master the syntax of the C programming language. Writing and debugging code regularly contributes to becoming fluent in C syntax, which is essential for effective programming.
- Understanding Algorithms and Logic: Examples often involve implementing algorithms and logical reasoning. Working through these examples improves algorithmic thinking and logic building, essential skills for writing efficient and optimized code.
- Skill Development: Solving a variety of programming problems enhances problem-solving skills. Each example presents a unique challenge, requiring learners to devise efficient solutions and improve their analytical and coding abilities.
- Builds Confidence: Successfully solving programming problems instills confidence in learners. As they tackle increasingly complex examples, individuals become more comfortable handling challenging coding tasks, boosting their overall confidence in programming.
- Diverse Problem-Solving: C programming examples cover a wide range of problems, including mathematical calculations, string manipulations, array operations, and more. This diversity exposes learners to various problem-solving approaches, enriching their programming toolkit.
- Portfolio Building: Individuals can use the solutions to these examples to build a portfolio showcasing their coding skills. A well-documented portfolio becomes a valuable asset when seeking internships, jobs, or contributing to open-source projects.
Also read: C++ For Kids- Learn Programming in The Fun Way
Why is it essential to practice C programming examples?
Practicing C programming examples is crucial for several reasons. It helps reinforce theoretical knowledge, enhances problem-solving skills, and provides hands-on experience with the language. Through practice, programmers become familiar with common syntax, logic building, and debugging techniques, contributing to overall proficiency in C programming.
What is the purpose of the "Swap Two Numbers" program?
The "Swap Two Numbers" program is designed to interchange the values of two variables. It is a fundamental programming exercise that helps users understand the concept of swapping values using a temporary variable or without using one. Swapping is a common operation in programming, and mastering it is essential.
Where can I find more C programming examples for practice?
You can find more C programming examples for practice on online platforms, programming websites, and educational resources.
How can I use C programming examples for learning?
Start with simple examples like Hello World and gradually progress to more complex ones. Analyze the code, understand how each line works, and experiment with modifications. This hands-on approach is effective for learning programming.
Can I modify the examples to create my programs?
Absolutely! Modifying existing examples is an excellent way to practice. Experiment with changing variables, adding features, or solving similar problems. This process fosters creativity and a deeper understanding of coding.
- Which is The Best Course for Full Stack Development?
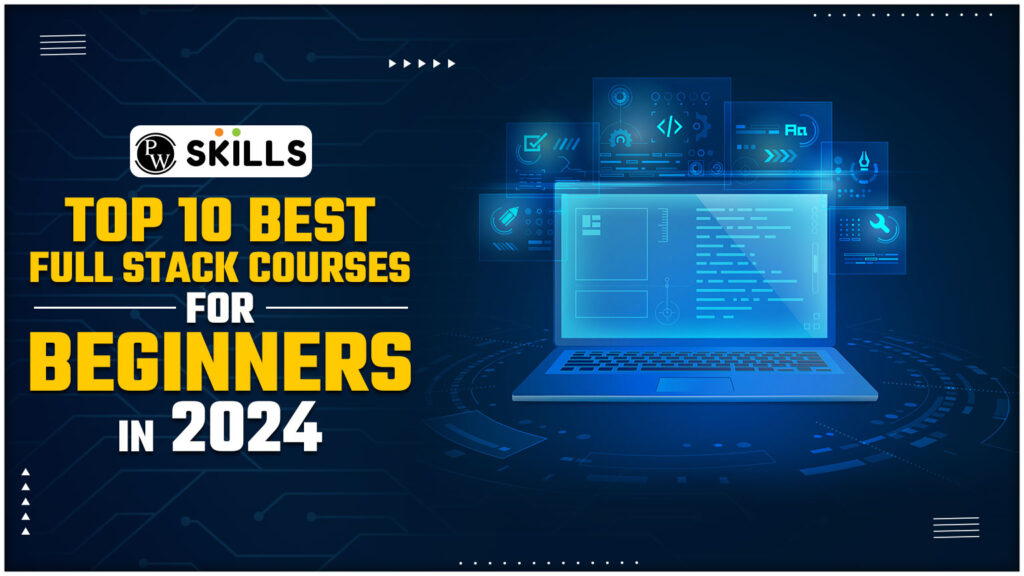
Confused about which course to choose to start your journey in Full Stack development? Don’t worry this article will clear…
- What is a Div Tag in HTML?
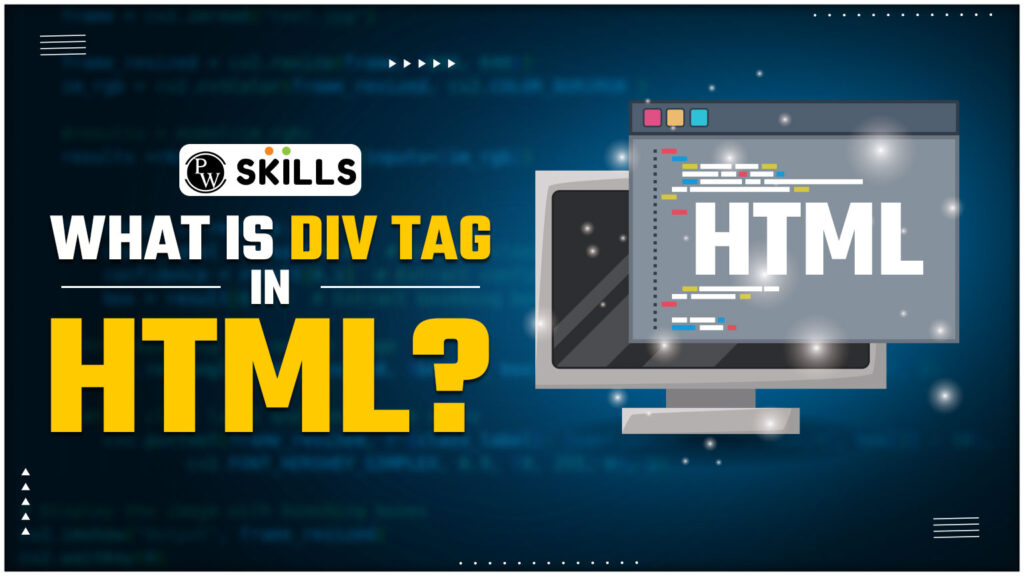
In this article, we will understand everything about the Div tag in HTML including its usage, syntax, and everything. Reading…
- What is C Language Basics?
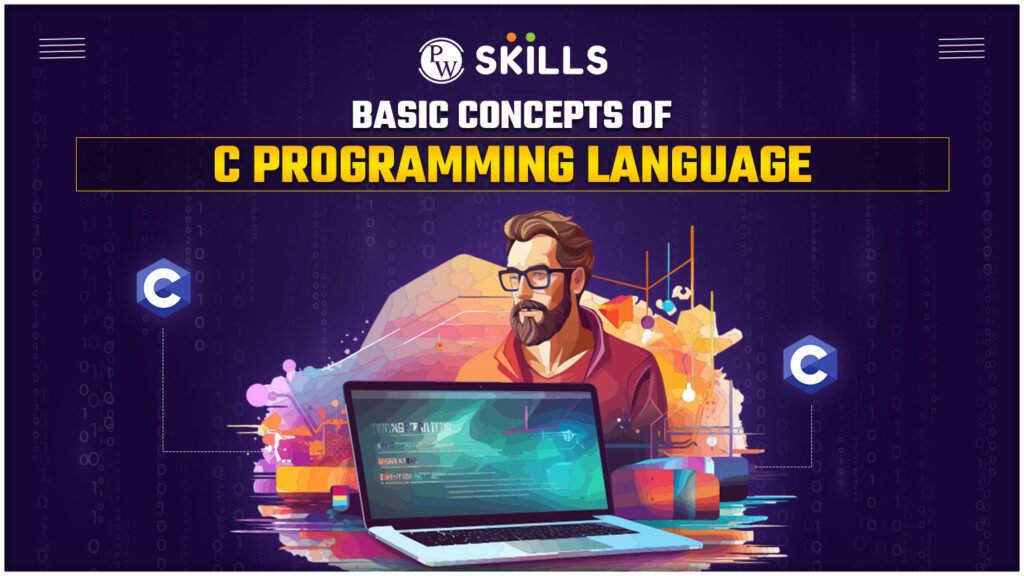
Basic of C language: C language is a widely used programming language used for application development and embedded systems. Learn…
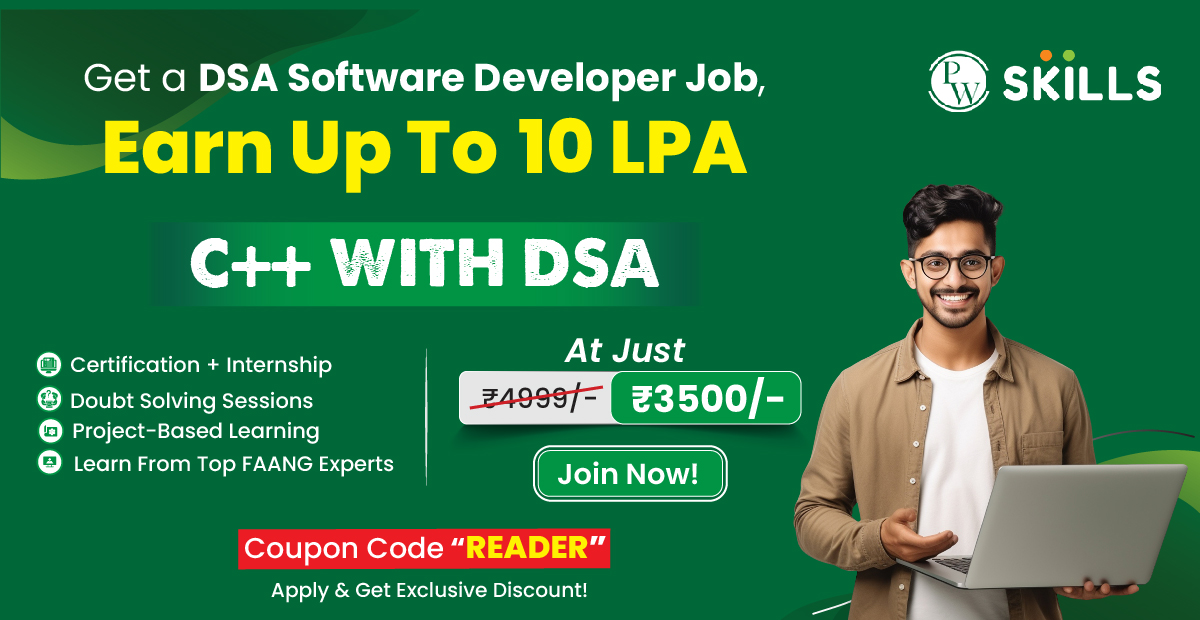
Related Articles
- What CSS Does in HTML?
- What is The HTML Tag?
- What is the Program of C?
- Is Azure DevOps Exam Easy?
- How Do You Type DOCTYPE?
- What is an Attribute in HTML?
- What do HTML Means?
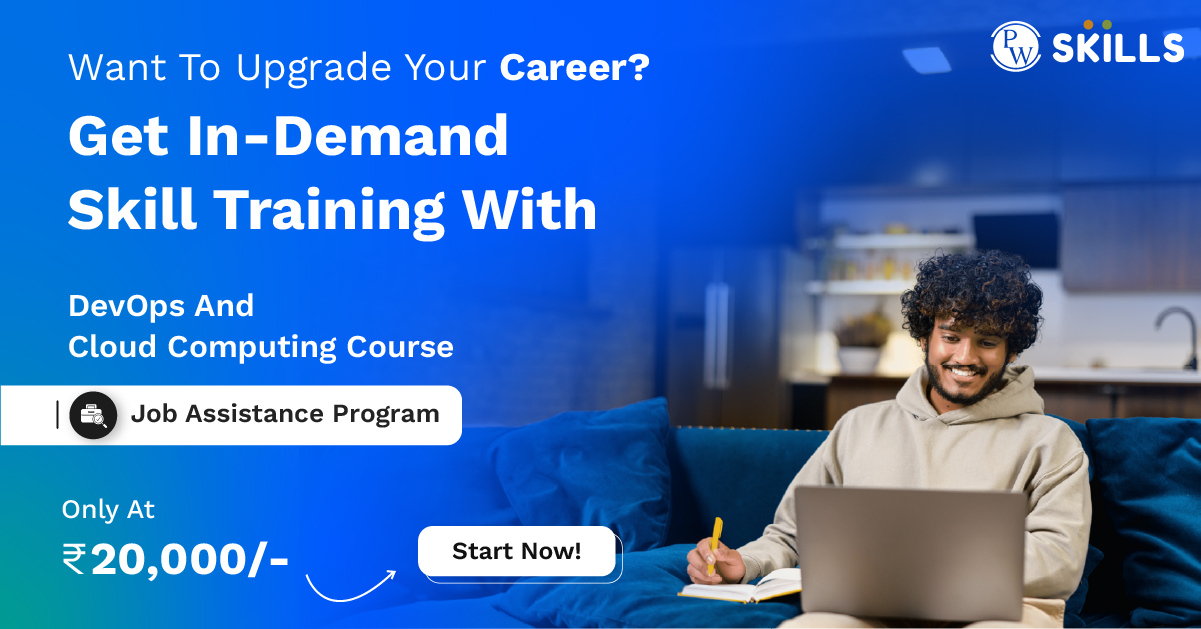
Browse Course Material
Course info, instructors.
- Daniel Weller
- Sharat Chikkerur
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Practical programming in c, course description.
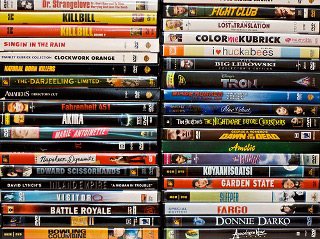
You are leaving MIT OpenCourseWare
- Online Degree Explore Bachelor’s & Master’s degrees
- MasterTrack™ Earn credit towards a Master’s degree
- University Certificates Advance your career with graduate-level learning
- Top Courses
- Join for Free

C Programming: Getting Started - 1
This course is part of C Programming with Linux Specialization
Taught in English
Some content may not be translated

Instructors: Rémi SHARROCK +1 more
Instructors
Instructor ratings.
We asked all learners to give feedback on our instructors based on the quality of their teaching style.
Financial aid available
14,016 already enrolled

(193 reviews)
Recommended experience
Beginner level
None. A course made for complete begininers in programmation.
What you'll learn
Define, distinguish and give examples of hardware/software, computer programs/algorithms
Explain the concept of a variable and declare, initialize and modify variables of data types int, double and char
Create and comment simple C-programs that may print text, special characters and variables to the screen with controlled formatting
Create simple C-programs that utilize for-loops to repeat blocks of instructions
Skills you'll gain
- computer programs/algorithms
- Computer Programming
- C Programming
Details to know

Add to your LinkedIn profile
See how employees at top companies are mastering in-demand skills
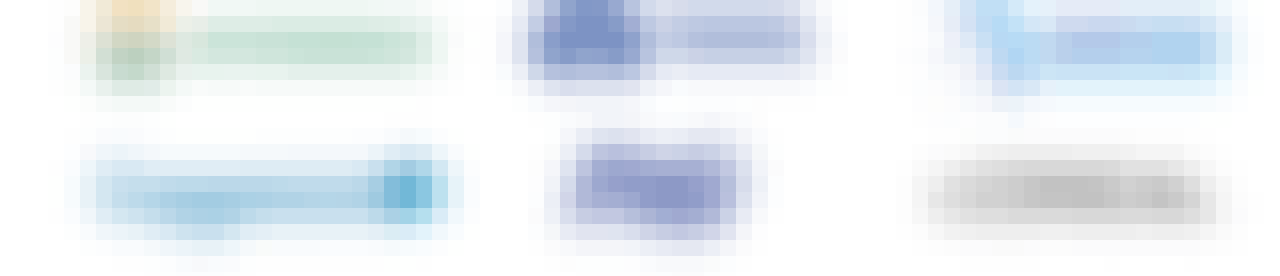
Build your subject-matter expertise
- Learn new concepts from industry experts
- Gain a foundational understanding of a subject or tool
- Develop job-relevant skills with hands-on projects
- Earn a shareable career certificate
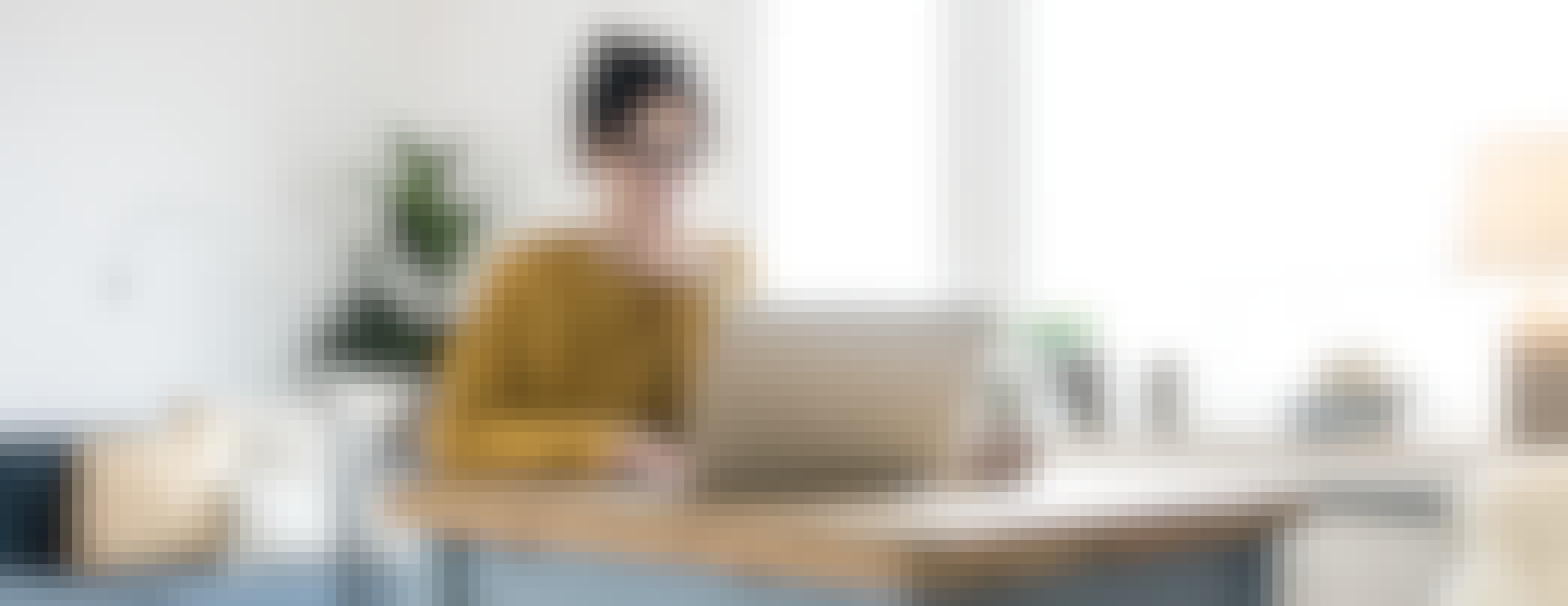
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
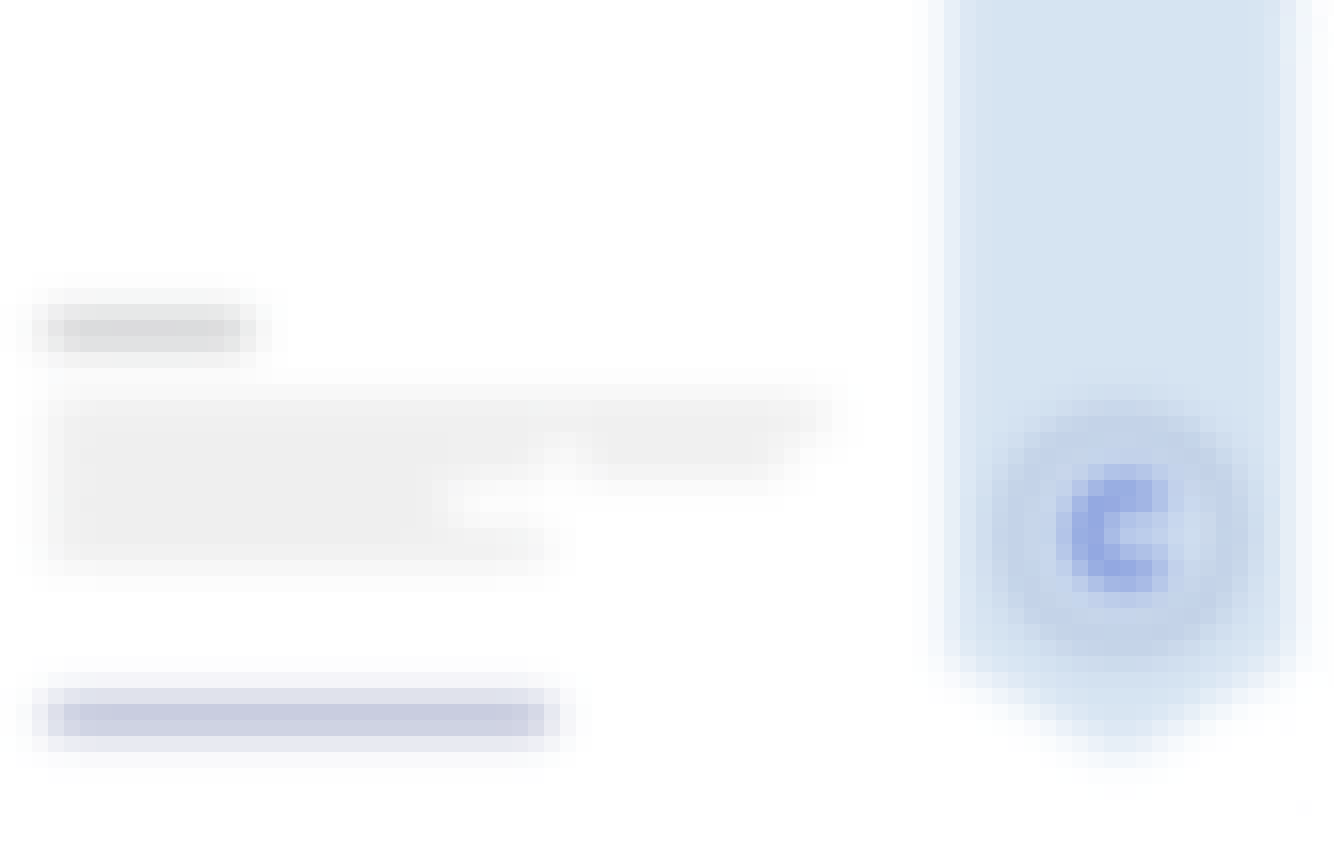
There are 5 modules in this course
Start learning one of the most powerful and widely used programming languages: C. Within moments you will be coding hands-on in a browser tool that will provide instant feedback on your code.
The C programming language is one of the most stable and popular programming languages in the world. It helps to power your smartphone, your car's navigation system, robots, drones, trains, and almost all electronic devices. C is used in any circumstances where speed and flexibility are important, such as in embedded systems or high-performance computing. In this course, you will get started with C and learn how to write your first programs, how to make simple computations and print the results to the screen, how to store values in variables and how to repeat instructions using loops. Beginners, even those without any programming experience, will be able to immediately start coding in C with the help of powerful yet simple coding tools right within the web browser. No need to install anything! Why learn C and not another programming language? Did you know that smartphones, your car’s navigation system, robots, drones, trains, and almost all electronic devices have some C-code running under the hood? C is used in any circumstance where speed and flexibility are important, such as in embedded systems or high-performance computing. C is a foundational programming language taught at engineering schools around the world, and represents one of the building blocks of modern computer information technology. Invented in the 1970’s. It is still one of the most stable and popular programming languages in the world. We are excited to introduce you to the world of coding and launch you along your path to becoming a skilled C programmer! This is the first course in the C Programming with Linux Specialization. This series of seven courses will establish your programming skills and unlock doors to careers in computer engineering. This course has received financial support from the Patrick & Lina Drahi Foundation.
Week 1: Welcome and the History of C
This week you will write your first line of code and become familiar with our learning tools (without installing anything!).
What's included
6 videos 10 readings 2 quizzes 4 app items 3 discussion prompts
6 videos • Total 16 minutes
- Welcome • 2 minutes • Preview module
- Get to work with Codecast • 4 minutes
- Learn about Taskgrader • 3 minutes
- How to navigate the third party tool? • 2 minutes
- History of the C programming language and its inventors, history of ‘hello world’ • 1 minute
- Hardware, software, algorithms, ... • 2 minutes
10 readings • Total 51 minutes
- Course Syllabus • 5 minutes
- Two universities teamed up to offer a new specialization in C Programming with Linux • 3 minutes
- The course team • 5 minutes
- Collaborative MOOC • 1 minute
- Earn a Specialization from Dartmouth and IMT • 5 minutes
- Get the most out of this course • 10 minutes
- Pre-course survey • 10 minutes
- Learn how to use Codecast and Taskgrader • 1 minute
- Codecast Sandbox • 1 minute
- Learn how to post code on the Coursera forum • 10 minutes
2 quizzes • Total 10 minutes
- Are you ready to start? • 5 minutes
- Self-assess your learning in Welcome and History of C • 5 minutes
4 app items • Total 38 minutes
- Get comfortable with your keyboard • 10 minutes
- Define key terms • 15 minutes
- "Hello world" • 5 minutes
- Syntax highlighting in Codecast • 8 minutes
3 discussion prompts • Total 21 minutes
- Introduce yourself • 10 minutes
- Learn how to post your code • 10 minutes
- Technical, general, organizational, and other questions • 1 minute
Week 2: Printing, loops, and comments
This week you will to print text to the screen, utilize for-loops, explain their code by adding comments in various ways, and recognize the different sections of a simple C-program and their purposes (e.g. variable declaration, main function).
1 quiz 10 app items
1 quiz • Total 10 minutes
- Self-assess your learning in Printing, loops, and comments • 10 minutes
10 app items • Total 155 minutes
- "Hello world!" – write and compile • 10 minutes
- Correct simple syntax errors • 10 minutes
- Print text and new lines • 15 minutes
- Print multiple lines with one printf statement • 15 minutes
- Print quotation mark and escape special characters • 15 minutes
- Repeat one instruction with a for loop • 15 minutes
- Repeat a block of instructions with a for loop • 15 minutes
- Discover the effect of simple looping errors • 15 minutes
- Comment your code • 30 minutes
- Structure of a simple C program • 15 minutes
Week 3: Integers, variables and user input
This week, you will explore the concept of variables and use integer-type variables (of format specifier %d) through declarations, assignments, and reassignments. You will also utilize these integer-type variables in for-loops and with user input.
1 quiz 6 app items
1 quiz • Total 5 minutes
- Self-assess your learning in Integers, variables and user input • 5 minutes
6 app items • Total 140 minutes
- Print and compute with integers • 15 minutes
- Use variables • 45 minutes
- Declare and name variables • 30 minutes
- Use variables in loops • 15 minutes
- Read user input • 20 minutes
- Use scanf() inside a loop to read multiple user inputs • 15 minutes
Week 4: Characters and doubles
This week you will utilize variables of type integer, double, and character through declarations, assignments, reassignments, printing, converting between types, casting, etc.. You will also create C-programs that perform tasks involving user input, integer and floating point arithmetic operations, and output to the screen.
1 quiz 8 app items
- Self-assess your learning in Characters and doubles • 5 minutes
8 app items • Total 200 minutes
- Declare, assign and print characters with the %c format specifier • 20 minutes
- Read characters from the user input • 20 minutes
- Use decimals • 20 minutes
- Divide in C • 20 minutes
- Find the remainder in integer division • 15 minutes
- Convert integers to double • 15 minutes
- Convert double to integers • 60 minutes
- Practice division • 30 minutes
Concluding the course
1 video 2 readings
- Concluding Programming in C: Getting Started • 0 minutes • Preview module
2 readings • Total 11 minutes
- Congratulations • 1 minute
- End of course survey • 10 minutes

Founded in 1769, Dartmouth is a member of the Ivy League and consistently ranks among the world’s greatest academic institutions. Dartmouth has forged a singular identity for combining its deep commitment to outstanding undergraduate liberal arts and graduate education with distinguished research and scholarship in the Arts and Sciences and its four leading graduate schools—the Geisel School of Medicine, the Guarini School of Graduate and Advanced Studies, Thayer School of Engineering, and the Tuck School of Business.

Institut Mines-Télécom is a public institution dedicated to higher education, research and innovation in engineering and digital technologies. Always attentive to the economic world, IMT combines strong academic legitimacy, close corporate relations. It focuses on key transformations in Digital Technologies, Production, Energy and Ecology and trains the engineers, managers and PhDs who will be tomorrow’s players in these key changes of the 21st century. Its activities are conducted in Mines and Télécom graduate schools under the aegis of the Minister for Industry and Electronic Communication, one subsidiary school and three strategic partners. The IMT’s schools rank among the leading graduate schools in France.
Recommended if you're interested in Software Development
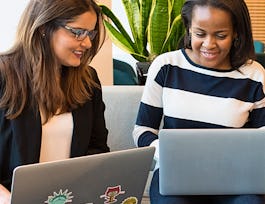
Dartmouth College
C Programming: Modular Programming and Memory Management - 3
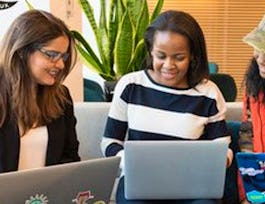
C Programming: Pointers and Memory Management - 4
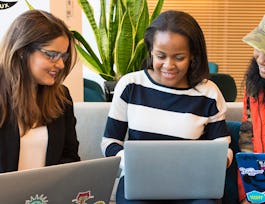
C Programming: Language Foundations - 2
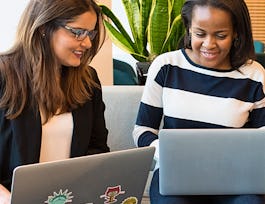
C Programming: Advanced Data Types - 5
Why people choose coursera for their career.

Learner reviews
Showing 3 of 193
193 reviews
Reviewed on Apr 18, 2023
Incredibly, I had an extraordinary experience. I genuinely feel that I am on the right track in learning C and grasping its fundamental concepts.
Reviewed on Dec 24, 2022
Great course for absolute beginner. Interactive learning methods are higly efective both learning and practicing.
Reviewed on May 9, 2023
This course has the best interactive tools for learning C!
New to Software Development? Start here.

Open new doors with Coursera Plus
Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
When will i have access to the lectures and assignments.
Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. If you don't see the audit option:
The course may not offer an audit option. You can try a Free Trial instead, or apply for Financial Aid.
The course may offer 'Full Course, No Certificate' instead. This option lets you see all course materials, submit required assessments, and get a final grade. This also means that you will not be able to purchase a Certificate experience.
What will I get if I subscribe to this Specialization?
When you enroll in the course, you get access to all of the courses in the Specialization, and you earn a certificate when you complete the work. Your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. If you only want to read and view the course content, you can audit the course for free.
What is the refund policy?
If you subscribed, you get a 7-day free trial during which you can cancel at no penalty. After that, we don’t give refunds, but you can cancel your subscription at any time. See our full refund policy Opens in a new tab .
Is financial aid available?
Yes. In select learning programs, you can apply for financial aid or a scholarship if you can’t afford the enrollment fee. If fin aid or scholarship is available for your learning program selection, you’ll find a link to apply on the description page.
More questions
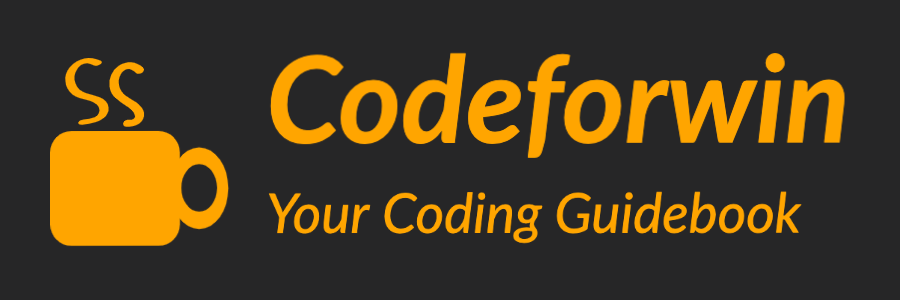
Loop programming exercises and solutions in C
In programming, there exists situations when you need to repeat single or a group of statements till some condition is met. Such as – read all files of a directory, send mail to all employees one after another etc. These task in C programming is handled by looping statements .
Looping statement defines a set of repetitive statements. These statements are repeated with same or different parameters for a number of times. Looping statement is also known as iterative or repetitive statement .
C supports three looping statements.
- do…while loop
In this exercise we will practice lots of looping problems to get a strong grip on loop. This is most recommended C programming exercise for beginners.
Always feel free to drop your queries, suggestions, hugs or bugs down below in the comments section . I always look forward to hear from you.
Required knowledge
Basic C programming , Relational operators , Logical operators , If else , For loop
List of loop programming exercises
- Write a C program to print all natural numbers from 1 to n. – using while loop
- Write a C program to print all natural numbers in reverse (from n to 1) . – using while loop
- Write a C program to print all alphabets from a to z. – using while loop
- Write a C program to print all even numbers between 1 to 100. – using while loop
- Write a C program to print all odd number between 1 to 100.
- Write a C program to find sum of all natural numbers between 1 to n.
- Write a C program to find sum of all even numbers between 1 to n .
- Write a C program to find sum of all odd numbers between 1 to n .
- Write a C program to print multiplication table of any number .
- Write a C program to count number of digits in a number .
- Write a C program to find first and last digit of a number .
- Write a C program to find sum of first and last digit of a number.
- Write a C program to swap first and last digits of a number .
- Write a C program to calculate sum of digits of a number .
- Write a C program to calculate product of digits of a number .
- Write a C program to enter a number and print its reverse .
- Write a C program to check whether a number is palindrome or not.
- Write a C program to find frequency of each digit in a given integer .
- Write a C program to enter a number and print it in words.
- Write a C program to print all ASCII character with their values .
- Write a C program to find power of a number using for loop .
- Write a C program to find all factors of a number .
- Write a C program to calculate factorial of a number .
- Write a C program to find HCF (GCD) of two numbers .
- Write a C program to find LCM of two numbers .
- Write a C program to check whether a number is Prime number or not.
- Write a C program to print all Prime numbers between 1 to n.
- Write a C program to find sum of all prime numbers between 1 to n .
- Write a C program to find all prime factors of a number .
- Write a C program to check whether a number is Armstrong number or not.
- Write a C program to print all Armstrong numbers between 1 to n.
- Write a C program to check whether a number is Perfect number or not .
- Write a C program to print all Perfect numbers between 1 to n .
- Write a C program to check whether a number is Strong number or not .
- Write a C program to print all Strong numbers between 1 to n .
- Write a C program to print Fibonacci series up to n terms .
- Write a C program to find one’s complement of a binary number .
- Write a C program to find two’s complement of a binary number .
- Write a C program to convert Binary to Octal number system .
- Write a C program to convert Binary to Decimal number system .
- Write a C program to convert Binary to Hexadecimal number system .
- Write a C program to convert Octal to Binary number system .
- Write a C program to convert Octal to Decimal number system .
- Write a C program to convert Octal to Hexadecimal number system .
- Write a C program to convert Decimal to Binary number system .
- Write a C program to convert Decimal to Octal number system .
- Write a C program to convert Decimal to Hexadecimal number system .
- Write a C program to convert Hexadecimal to Binary number system .
- Write a C program to convert Hexadecimal to Octal number system .
- Write a C program to convert Hexadecimal to Decimal number system .
- Write a C program to print Pascal triangle upto n rows .
- Star pattern programs – Write a C program to print the given star patterns.
- Number pattern programs – Write a C program to print the given number patterns .
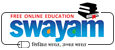
Problem Solving Through Programming In C
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
Note: This exam date is subjected to change based on seat availability. You can check final exam date on your hall ticket.
Page Visits
Course layout, books and references, instructor bio.
Prof. Anupam Basu
Course certificate.
- Assignment score = 25% of average of best 8 assignments out of the total 12 assignments given in the course.
- ( All assignments in a particular week will be counted towards final scoring - quizzes and programming assignments).
- Unproctored programming exam score = 25% of the average scores obtained as part of Unproctored programming exam - out of 100
- Proctored Exam score =50% of the proctored certification exam score out of 100

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :

C Programming Exercises With Solutions (PDF)
Here you will get C Programming Exercises With Solutions.
Here I am going to provide you a document of these, which you can download and make changes according to your own.

Download C Programming Practical Assignments Questions
Download Now
Download C Programming Exercises With Solutions PDF (2020)
Download c programming exercises with solutions pdf (2021), download 100+ c programming exercises pdf.
If you face any problem in downloading C Programming Practical Assignments Questions and Solutions PDFs, then tell us in the comment below and you will definitely share this post with your friends so that they can also be of some help.
Also Read -:
- Database Management System Lab Assignment Exercise Question and There Solutions
- C++ Lab Exercises And Solutions (PDF) – Master Programming
- Web Technology Lab Assignment Question And Solutions
- Pc Software Practical Assignment Question And Solutions
- Operating System with Linux Lab Assignment Question And Solutions (PDF)
Jeetu Sahu is A Web Developer | Computer Engineer | Passionate about Coding and Competitive Programming
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Possible solutions for assignments of Programming Fundamentals at XJTU.
craigkerui/Programming-Fundamentals_XJTU
Folders and files, repository files navigation, programming-fundamentals_xjtu.
Relatives of those who died waiting for livers at now halted Houston transplant program seek answers
DALLAS — Several relatives of patients who died while waiting for a new liver said Wednesday they want to know if their loved ones were wrongfully denied a transplant by a Houston doctor accused of manipulating the waitlist to make some patients ineligible to receive a new organ.
Officials at Memorial Hermann-Texas Medical Center have said they are investigating after finding that a doctor had made “inappropriate changes” in the national database for people awaiting liver transplants. Earlier this month, the hospital halted its liver and kidney programs.
Susie Garcia’s son, Richard Mostacci, died in February 2023 after being told he was too sick for a transplant. He was 43. “We saw him slipping away, slipping away and there was nothing that we could do, and we trusted, we trusted the doctors,” Garcia said at a news conference.
She’s among family members of three patients who retained attorneys with a Houston law firm that filed for a temporary restraining order Tuesday to prevent Dr. Steve Bynon from deleting or destroying evidence. Attorney Tommy Hastings said that some interactions with Bynon had caused “concerns about maybe some personal animosities and that maybe he may have taken it out on patients.”
“Again, we’re very early in this investigation,” Hastings said.
Hermann-Memorial’s statement didn’t name the doctor, but the University of Texas Health Science Center at Houston, or UTHealth Houston, issued a statement defending Bynon, calling him ”an exceptionally talented and caring physician” with survival rates that are “among the best in the nation.”
Bynon is an employee of UTHealth Houston who is contracted to Memorial Hermann. He did not respond to an email inquiry Wednesday.
The hospital has said the inappropriate changes were only made to the liver transplant program, but since he shared leadership over both the liver and kidney transplant programs, they inactivated both.
The U.S. Department of Health and Human Services also said it’s conducting an investigation, adding it is “working across the department to address this matter.”
Neither Hermann Memorial nor UTHealth or HHS had additional comments Wednesday.
Meanwhile, a woman using a different law firm filed a lawsuit last week in Harris County against Memorial Hermann and UTHealth alleging negligence in the death of her husband, John Montgomery, who died in May 2023 at age 66 while on the waitlist for a liver transplant. The lawsuit says that Montgomery was told he wasn’t sick enough, and subsequently, that he was too sick before ultimately being taken off the list.
The death rate for people waiting for a liver transplant at Memorial Hermann was higher than expected in recent years, according to publicly available data from the Scientific Registry of Transplant Recipients, which evaluates U.S. organ transplant programs. The group found that in the two-year period from July 2021 through June 2023, there were 19 deaths on the waitlist, while models would have predicted about 14 deaths.
While the hospital’s waitlist mortality rate of 28% was higher than expected “there were many liver programs with more extreme outcomes during the same period,” Jon Snyder, the registry’s director, said in an email.
He said that the hospital’s first-year success rates for the 56 adults who received transplants between July 2020 through December 2022 was 35% better than expected based on national outcomes.

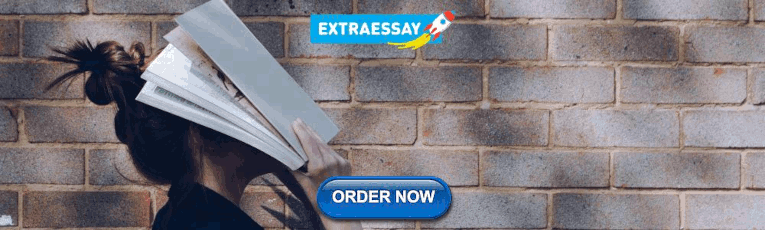
IMAGES
VIDEO
COMMENTS
This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more. So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
Matrix (2D array) Add two matrices. Scalar matrix multiplication. Multiply two matrices. Check if two matrices are equal. Sum of diagonal elements of matrix. Interchange diagonal of matrix. Find upper triangular matrix. Find sum of lower triangular matrix.
ASSIGNMENTS SOLUTIONS 1 Writing, compiling, and debugging programs; preprocessor macros; C file structure; variables; functions and problem statements; returning from functions ... assignment_turned_in Programming Assignments with Examples. Download Course. Over 2,500 courses & materials Freely sharing knowledge with learners and educators ...
Write a C program to check whether number is positive, negative or zero . Description: You need to write a C program to check whether number is positive, negative or zero. Conditions: Create variable with name of number and the value will taken by user or console; Create this c program code using else if ladder statement
List of basic programming exercises. Write a C program to perform input/output of all basic data types. Write a C program to enter two numbers and find their sum. Write a C program to enter two numbers and perform all arithmetic operations. Write a C program to enter length and breadth of a rectangle and find its perimeter.
Last update on May 19 2023 12:52:26 (UTC/GMT +8 hours) C Programming Basic Algorithm [75 exercises with solution] [ An editor is available at the bottom of the page to write and execute the scripts. Go to the editor] 1. Write a C program to compute the sum of the two input values. If the two values are the same, then return triple their sum.
Practice C. Practice C problems, a great starting point if you really want to understand fundamental programming constructs. 4.4 (1758 reviews) 33 Problems Beginner level. 29.2k Learners.
Program. C Program to Print an Integer (Entered by the User) C Program to Add Two Integers. C Program to Multiply Two Floating-Point Numbers. C Program to Find ASCII Value of a Character. C Program to Compute Quotient and Remainder. C Program to Find the Size of int, float, double and char. C Program to Demonstrate the Working of Keyword long.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. We use cookies to ensure you have the best browsing experience on our website. ... For Loop in C. Easy C (Basic) Max Score: 10 Success Rate: 93.65%. Solve Challenge. Sum of Digits of a Five Digit Number. Easy C ...
C Programming Examples With Output. Learners can also download c programming examples with output PDF to start their C language journey. But practice is the key to learning C language properly. Here are some of the best C programming examples with output: 1. C Program to Check Whether a Number is Positive, Negative, or Zero. #include <stdio.h ...
Course Description. This course provides a thorough introduction to the C programming language, the workhorse of the UNIX operating system and lingua franca of embedded processors and micro-controllers. The first two weeks will cover basic syntax and grammar, and expose students to practical programming techniques. The remaining lectures ….
Click me to see the solution. 2. Write a C program to get the C version you are using. Expected Output: We are using C18! Click me to see the solution. 3. Write a C program to print a block F using the hash (#), where the F has a height of six characters and width of five and four characters. And also print a very large 'C'. Expected Output:
Course Syllabus • 5 minutes. Two universities teamed up to offer a new specialization in C Programming with Linux • 3 minutes. The course team • 5 minutes. Collaborative MOOC • 1 minute. Earn a Specialization from Dartmouth and IMT • 5 minutes. Get the most out of this course • 10 minutes. Pre-course survey • 10 minutes.
The C Programming Language is a very popular book and sometimes people refer to it as K&R.The authors Brian W. Kernighan and Dennis M. Ritchie did a very good job of explaining the core concepts of programming. The focus of the book is the C programming language, however, the approach is general, so it can be extrapolated to other programming languages.
List of loop programming exercises. Write a C program to print all natural numbers from 1 to n. - using while loop. Write a C program to print all natural numbers in reverse (from n to 1). - using while loop. Write a C program to print all alphabets from a to z. - using while loop.
Learners enrolled: 29073. ABOUT THE COURSE : This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
Our resource for C Programming: A Modern Approach includes answers to chapter exercises, as well as detailed information to walk you through the process step by step. With Expert Solutions for thousands of practice problems, you can take the guesswork out of studying and move forward with confidence. Find step-by-step solutions and answers to C ...
This course is part of the Coding for Everyone: C and C++ Specialization Coding for Everyone: C and C++ Specialization which is available on coursera offered by University of Califormia, Santa Cruz. Here you will find my solutions of the assignments. 😄 Happy Learning!!!
Solutions to the Assignments for the Algorithmic Toolbox course offered by UCSanDiego on Coursera. - prantosky/coursera-algorithmic-toolbox ... Approach 2 (Dynamic Programming) Maximum Value of an Arithmetic Expression; About. Solutions to the Assignments for the Algorithmic Toolbox course offered by UCSanDiego on Coursera.
C For Loop [61 exercises with solution] [ An editor is available at the bottom of the page to write and execute the scripts. Go to the editor] 1. Write a program in C to display the first 10 natural numbers. 2. Write a C program to compute the sum of the first 10 natural numbers.
Here you will get C Programming Exercises With Solutions. Here I am going to provide you a document of these, which you can download and make changes according to your own. Contents hide. 1 Download C Programming Practical Assignments Questions. 2 Download C Programming Exercises With Solutions PDF (2020)
Possible solutions for assignments of Programming Fundamentals at XJTU. - craigkerui/Programming-Fundamentals_XJTU
Now, with expert-verified solutions from C++ Programming 8th Edition, you'll learn how to solve your toughest homework problems. Our resource for C++ Programming includes answers to chapter exercises, as well as detailed information to walk you through the process step by step. With Expert Solutions for thousands of practice problems, you can ...
C programming related to structures [9 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.Go to the editor]. From Wikipedia - A struct (Structures) in the C programming language (and many derivatives) is a composite data type (or record) declaration that defines a physically grouped list of variables under one name in a block of memory ...
Several relatives of patients who died while awaiting a new liver say they want to know if their loved ones were wrongfully denied a transplant by a Houston doctor ...