- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
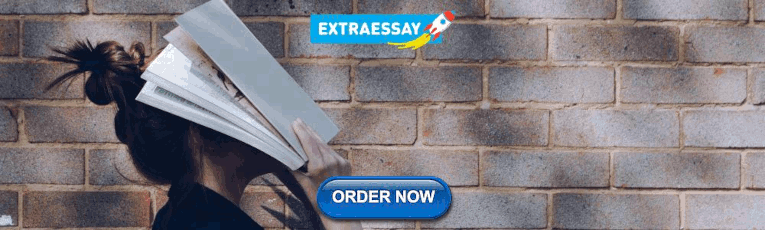
Hungarian Algorithm for Assignment Problem | Set 2 (Implementation)
- Hungarian Algorithm for Assignment Problem | Set 1 (Introduction)
- Implementation of Exhaustive Search Algorithm for Set Packing
- Greedy Approximate Algorithm for Set Cover Problem
- Introduction to Exact Cover Problem and Algorithm X
- Job Assignment Problem using Branch And Bound
- Prim's Algorithm (Simple Implementation for Adjacency Matrix Representation)
- Introduction to Disjoint Set (Union-Find Algorithm)
- Channel Assignment Problem
- Java Program for Counting sets of 1s and 0s in a binary matrix
- Top 20 Greedy Algorithms Interview Questions
- C++ Program for Counting sets of 1s and 0s in a binary matrix
- C# Program for Dijkstra's shortest path algorithm | Greedy Algo-7
- Java Program for Dijkstra's shortest path algorithm | Greedy Algo-7
- C / C++ Program for Dijkstra's shortest path algorithm | Greedy Algo-7
- Self assignment check in assignment operator
- Python Program for Dijkstra's shortest path algorithm | Greedy Algo-7
- Algorithms | Dynamic Programming | Question 7
- Assignment Operators in C
- Assignment Operators in Programming
- Merge Sort - Data Structure and Algorithms Tutorials
- Must Do Coding Questions for Companies like Amazon, Microsoft, Adobe, ...
- QuickSort - Data Structure and Algorithm Tutorials
- Bubble Sort - Data Structure and Algorithm Tutorials
- Tree Traversal Techniques - Data Structure and Algorithm Tutorials
- Binary Search - Data Structure and Algorithm Tutorials
- Insertion Sort - Data Structure and Algorithm Tutorials
- Selection Sort – Data Structure and Algorithm Tutorials
- Understanding the basics of Linked List
- Breadth First Search or BFS for a Graph
Given a 2D array , arr of size N*N where arr[i][j] denotes the cost to complete the j th job by the i th worker. Any worker can be assigned to perform any job. The task is to assign the jobs such that exactly one worker can perform exactly one job in such a way that the total cost of the assignment is minimized.
Input: arr[][] = {{3, 5}, {10, 1}} Output: 4 Explanation: The optimal assignment is to assign job 1 to the 1st worker, job 2 to the 2nd worker. Hence, the optimal cost is 3 + 1 = 4. Input: arr[][] = {{2500, 4000, 3500}, {4000, 6000, 3500}, {2000, 4000, 2500}} Output: 4 Explanation: The optimal assignment is to assign job 2 to the 1st worker, job 3 to the 2nd worker and job 1 to the 3rd worker. Hence, the optimal cost is 4000 + 3500 + 2000 = 9500.
Different approaches to solve this problem are discussed in this article .
Approach: The idea is to use the Hungarian Algorithm to solve this problem. The algorithm is as follows:
- For each row of the matrix, find the smallest element and subtract it from every element in its row.
- Repeat the step 1 for all columns.
- Cover all zeros in the matrix using the minimum number of horizontal and vertical lines.
- Test for Optimality : If the minimum number of covering lines is N , an optimal assignment is possible. Else if lines are lesser than N , an optimal assignment is not found and must proceed to step 5.
- Determine the smallest entry not covered by any line. Subtract this entry from each uncovered row, and then add it to each covered column. Return to step 3.
Consider an example to understand the approach:
Let the 2D array be: 2500 4000 3500 4000 6000 3500 2000 4000 2500 Step 1: Subtract minimum of every row. 2500, 3500 and 2000 are subtracted from rows 1, 2 and 3 respectively. 0 1500 1000 500 2500 0 0 2000 500 Step 2: Subtract minimum of every column. 0, 1500 and 0 are subtracted from columns 1, 2 and 3 respectively. 0 0 1000 500 1000 0 0 500 500 Step 3: Cover all zeroes with minimum number of horizontal and vertical lines. Step 4: Since we need 3 lines to cover all zeroes, the optimal assignment is found. 2500 4000 3500 4000 6000 3500 2000 4000 2500 So the optimal cost is 4000 + 3500 + 2000 = 9500
For implementing the above algorithm, the idea is to use the max_cost_assignment() function defined in the dlib library . This function is an implementation of the Hungarian algorithm (also known as the Kuhn-Munkres algorithm) which runs in O(N 3 ) time. It solves the optimal assignment problem.
Below is the implementation of the above approach:
Time Complexity: O(N 3 ) Auxiliary Space: O(N 2 )
Please Login to comment...
Similar reads.
- Mathematical
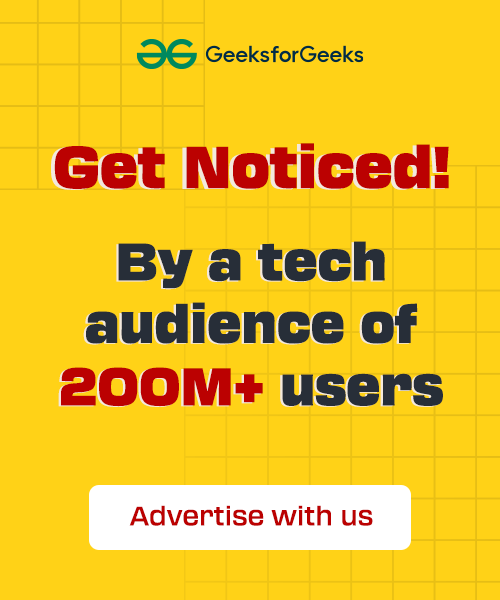
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
AP®︎/College Computer Science Principles
Course: ap®︎/college computer science principles > unit 4, the building blocks of algorithms.
- Expressing an algorithm
Want to join the conversation?
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

The assignment problem revisited
- Original Paper
- Published: 16 August 2021
- Volume 16 , pages 1531–1548, ( 2022 )
Cite this article
- Carlos A. Alfaro ORCID: orcid.org/0000-0001-9783-8587 1 ,
- Sergio L. Perez 2 ,
- Carlos E. Valencia 3 &
- Marcos C. Vargas 1
970 Accesses
4 Citations
4 Altmetric
Explore all metrics
First, we give a detailed review of two algorithms that solve the minimization case of the assignment problem, the Bertsekas auction algorithm and the Goldberg & Kennedy algorithm. It was previously alluded that both algorithms are equivalent. We give a detailed proof that these algorithms are equivalent. Also, we perform experimental results comparing the performance of three algorithms for the assignment problem: the \(\epsilon \) - scaling auction algorithm , the Hungarian algorithm and the FlowAssign algorithm . The experiment shows that the auction algorithm still performs and scales better in practice than the other algorithms which are harder to implement and have better theoretical time complexity.
This is a preview of subscription content, log in via an institution to check access.
Access this article
Price includes VAT (Russian Federation)
Instant access to the full article PDF.
Rent this article via DeepDyve
Institutional subscriptions
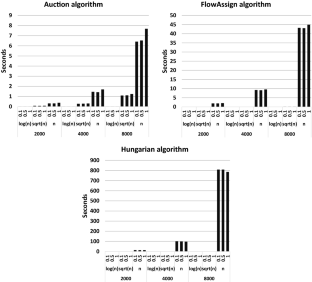
Similar content being viewed by others
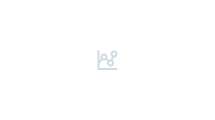
Some results on an assignment problem variant
Integer Programming
A Full Description of Polytopes Related to the Index of the Lowest Nonzero Row of an Assignment Matrix
Bertsekas, D.P.: The auction algorithm: a distributed relaxation method for the assignment problem. Annal Op. Res. 14 , 105–123 (1988)
Article MathSciNet Google Scholar
Bertsekas, D.P., Castañon, D.A.: Parallel synchronous and asynchronous implementations of the auction algorithm. Parallel Comput. 17 , 707–732 (1991)
Article Google Scholar
Bertsekas, D.P.: Linear network optimization: algorithms and codes. MIT Press, Cambridge, MA (1991)
MATH Google Scholar
Bertsekas, D.P.: The auction algorithm for shortest paths. SIAM J. Optim. 1 , 425–477 (1991)
Bertsekas, D.P.: Auction algorithms for network flow problems: a tutorial introduction. Comput. Optim. Appl. 1 , 7–66 (1992)
Bertsekas, D.P., Castañon, D.A., Tsaknakis, H.: Reverse auction and the solution of inequality constrained assignment problems. SIAM J. Optim. 3 , 268–299 (1993)
Bertsekas, D.P., Eckstein, J.: Dual coordinate step methods for linear network flow problems. Math. Progr., Ser. B 42 , 203–243 (1988)
Bertsimas, D., Tsitsiklis, J.N.: Introduction to linear optimization. Athena Scientific, Belmont, MA (1997)
Google Scholar
Burkard, R., Dell’Amico, M., Martello, S.: Assignment Problems. Revised reprint. SIAM, Philadelphia, PA (2011)
Gabow, H.N., Tarjan, R.E.: Faster scaling algorithms for network problems. SIAM J. Comput. 18 (5), 1013–1036 (1989)
Goldberg, A.V., Tarjan, R.E.: A new approach to the maximum flow problem. J. Assoc. Comput. Mach. 35 , 921–940 (1988)
Goldberg, A.V., Tarjan, R.E.: Finding minimum-cost circulations by successive approximation. Math. Op. Res. 15 , 430–466 (1990)
Goldberg, A.V., Kennedy, R.: An efficient cost scaling algorithm for the assignment problem. Math. Programm. 71 , 153–177 (1995)
MathSciNet MATH Google Scholar
Goldberg, A.V., Kennedy, R.: Global price updates help. SIAM J. Discr. Math. 10 (4), 551–572 (1997)
Kuhn, H.W.: The Hungarian method for the assignment problem. Naval Res. Logist. Quart. 2 , 83–97 (1955)
Kuhn, H.W.: Variants of the Hungarian method for the assignment problem. Naval Res. Logist. Quart. 2 , 253–258 (1956)
Lawler, E.L.: Combinatorial optimization: networks and matroids, Holt. Rinehart & Winston, New York (1976)
Orlin, J.B., Ahuja, R.K.: New scaling algorithms for the assignment ad minimum mean cycle problems. Math. Programm. 54 , 41–56 (1992)
Ramshaw, L., Tarjan, R.E., Weight-Scaling Algorithm, A., for Min-Cost Imperfect Matchings in Bipartite Graphs, : IEEE 53rd Annual Symposium on Foundations of Computer Science. New Brunswick, NJ 2012 , 581–590 (2012)
Zaki, H.: A comparison of two algorithms for the assignment problem. Comput. Optim. Appl. 4 , 23–45 (1995)
Download references
Acknowledgements
This research was partially supported by SNI and CONACyT.
Author information
Authors and affiliations.
Banco de México, Mexico City, Mexico
Carlos A. Alfaro & Marcos C. Vargas
Mountain View, CA, 94043, USA
Sergio L. Perez
Departamento de Matemáticas, CINVESTAV del IPN, Apartado postal 14-740, 07000, Mexico City, Mexico
Carlos E. Valencia
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Carlos A. Alfaro .
Ethics declarations
Conflict of interest.
There is no conflict of interest.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
The authors were partially supported by SNI and CONACyT.
Rights and permissions
Reprints and permissions
About this article
Alfaro, C.A., Perez, S.L., Valencia, C.E. et al. The assignment problem revisited. Optim Lett 16 , 1531–1548 (2022). https://doi.org/10.1007/s11590-021-01791-4
Download citation
Received : 26 March 2020
Accepted : 03 August 2021
Published : 16 August 2021
Issue Date : June 2022
DOI : https://doi.org/10.1007/s11590-021-01791-4
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Assignment problem
- Bertsekas auction algorithm
- Combinatorial optimization and matching
- Find a journal
- Publish with us
- Track your research
Solving assignment problem using min-cost-flow ¶
The assignment problem has two equivalent statements:
- Given a square matrix $A[1..N, 1..N]$ , you need to select $N$ elements in it so that exactly one element is selected in each row and column, and the sum of the values of these elements is the smallest.
- There are $N$ orders and $N$ machines. The cost of manufacturing on each machine is known for each order. Only one order can be performed on each machine. It is required to assign all orders to the machines so that the total cost is minimized.
Here we will consider the solution of the problem based on the algorithm for finding the minimum cost flow (min-cost-flow) , solving the assignment problem in $\mathcal{O}(N^3)$ .
Description ¶
Let's build a bipartite network: there is a source $S$ , a drain $T$ , in the first part there are $N$ vertices (corresponding to rows of the matrix, or orders), in the second there are also $N$ vertices (corresponding to the columns of the matrix, or machines). Between each vertex $i$ of the first set and each vertex $j$ of the second set, we draw an edge with bandwidth 1 and cost $A_{ij}$ . From the source $S$ we draw edges to all vertices $i$ of the first set with bandwidth 1 and cost 0. We draw an edge with bandwidth 1 and cost 0 from each vertex of the second set $j$ to the drain $T$ .
We find in the resulting network the maximum flow of the minimum cost. Obviously, the value of the flow will be $N$ . Further, for each vertex $i$ of the first segment there is exactly one vertex $j$ of the second segment, such that the flow $F_{ij}$ = 1. Finally, this is a one-to-one correspondence between the vertices of the first segment and the vertices of the second part, which is the solution to the problem (since the found flow has a minimal cost, then the sum of the costs of the selected edges will be the lowest possible, which is the optimality criterion).
The complexity of this solution of the assignment problem depends on the algorithm by which the search for the maximum flow of the minimum cost is performed. The complexity will be $\mathcal{O}(N^3)$ using Dijkstra or $\mathcal{O}(N^4)$ using Bellman-Ford . This is due to the fact that the flow is of size $O(N)$ and each iteration of Dijkstra algorithm can be performed in $O(N^2)$ , while it is $O(N^3)$ for Bellman-Ford.
Implementation ¶
The implementation given here is long, it can probably be significantly reduced. It uses the SPFA algorithm for finding shortest paths.
- Daili01 (75.89%)
- prprprpony (12.5%)
- adamant-pwn (7.14%)
- dufferzafar (2.68%)
- jakobkogler (0.89%)
- Hasan-Mesbaul-Ali-Taher (0.89%)

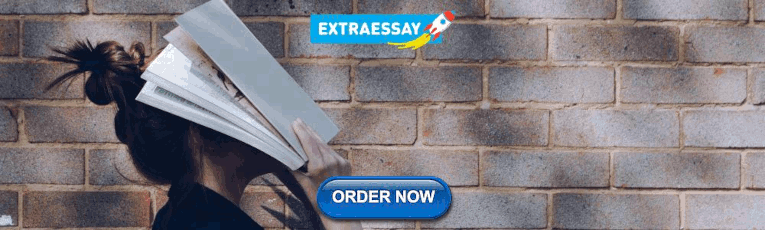
How to Solve the Assignment Problem: A Complete Guide
Table of Contents
Assignment problem is a special type of linear programming problem that deals with assigning a number of resources to an equal number of tasks in the most efficient way. The goal is to minimize the total cost of assignments while ensuring that each task is assigned to only one resource and each resource is assigned to only one task. In this blog, we will discuss the solution of the assignment problem using the Hungarian method, which is a popular algorithm for solving the problem.
Understanding the Assignment Problem
Before we dive into the solution, it is important to understand the problem itself. In the assignment problem, we have a matrix of costs, where each row represents a resource and each column represents a task. The objective is to assign each resource to a task in such a way that the total cost of assignments is minimized. However, there are certain constraints that need to be satisfied – each resource can be assigned to only one task and each task can be assigned to only one resource.
Solving the Assignment Problem
There are various methods for solving the assignment problem, including the Hungarian method, the brute force method, and the auction algorithm. Here, we will focus on the steps involved in solving the assignment problem using the Hungarian method, which is the most commonly used and efficient method.
Step 1: Set up the cost matrix
The first step in solving the assignment problem is to set up the cost matrix, which represents the cost of assigning a task to an agent. The matrix should be square and have the same number of rows and columns as the number of tasks and agents, respectively.
Step 2: Subtract the smallest element from each row and column
To simplify the calculations, we need to reduce the size of the cost matrix by subtracting the smallest element from each row and column. This step is called matrix reduction.
Step 3: Cover all zeros with the minimum number of lines
The next step is to cover all zeros in the matrix with the minimum number of horizontal and vertical lines. This step is called matrix covering.
Step 4: Test for optimality and adjust the matrix
To test for optimality, we need to calculate the minimum number of lines required to cover all zeros in the matrix. If the number of lines equals the number of rows or columns, the solution is optimal. If not, we need to adjust the matrix and repeat steps 3 and 4 until we get an optimal solution.
Step 5: Assign the tasks to the agents
The final step is to assign the tasks to the agents based on the optimal solution obtained in step 4. This will give us the most cost-effective or profit-maximizing assignment.
Solution of the Assignment Problem using the Hungarian Method
The Hungarian method is an algorithm that uses a step-by-step approach to find the optimal assignment. The algorithm consists of the following steps:
- Subtract the smallest entry in each row from all the entries of the row.
- Subtract the smallest entry in each column from all the entries of the column.
- Draw the minimum number of lines to cover all zeros in the matrix. If the number of lines drawn is equal to the number of rows, we have an optimal solution. If not, go to step 4.
- Determine the smallest entry not covered by any line. Subtract it from all uncovered entries and add it to all entries covered by two lines. Go to step 3.
The above steps are repeated until an optimal solution is obtained. The optimal solution will have all zeros covered by the minimum number of lines. The assignments can be made by selecting the rows and columns with a single zero in the final matrix.
Applications of the Assignment Problem
The assignment problem has various applications in different fields, including computer science, economics, logistics, and management. In this section, we will provide some examples of how the assignment problem is used in real-life situations.
Applications in Computer Science
The assignment problem can be used in computer science to allocate resources to different tasks, such as allocating memory to processes or assigning threads to processors.
Applications in Economics
The assignment problem can be used in economics to allocate resources to different agents, such as allocating workers to jobs or assigning projects to contractors.
Applications in Logistics
The assignment problem can be used in logistics to allocate resources to different activities, such as allocating vehicles to routes or assigning warehouses to customers.
Applications in Management
The assignment problem can be used in management to allocate resources to different projects, such as allocating employees to tasks or assigning budgets to departments.
Let’s consider the following scenario: a manager needs to assign three employees to three different tasks. Each employee has different skills, and each task requires specific skills. The manager wants to minimize the total time it takes to complete all the tasks. The skills and the time required for each task are given in the table below:
The assignment problem is to determine which employee should be assigned to which task to minimize the total time required. To solve this problem, we can use the Hungarian method, which we discussed in the previous blog.
Using the Hungarian method, we first subtract the smallest entry in each row from all the entries of the row:
Next, we subtract the smallest entry in each column from all the entries of the column:
We draw the minimum number of lines to cover all the zeros in the matrix, which in this case is three:
Since the number of lines is equal to the number of rows, we have an optimal solution. The assignments can be made by selecting the rows and columns with a single zero in the final matrix. In this case, the optimal assignments are:
- Emp 1 to Task 3
- Emp 2 to Task 2
- Emp 3 to Task 1
This assignment results in a total time of 9 units.
I hope this example helps you better understand the assignment problem and how to solve it using the Hungarian method.
Solving the assignment problem may seem daunting, but with the right approach, it can be a straightforward process. By following the steps outlined in this guide, you can confidently tackle any assignment problem that comes your way.
How useful was this post?
Click on a star to rate it!
Average rating 0 / 5. Vote count: 0
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you! 😔
Let us improve this post!
Tell us how we can improve this post?
Operations Research
1 Operations Research-An Overview
- History of O.R.
- Approach, Techniques and Tools
- Phases and Processes of O.R. Study
- Typical Applications of O.R
- Limitations of Operations Research
- Models in Operations Research
- O.R. in real world
2 Linear Programming: Formulation and Graphical Method
- General formulation of Linear Programming Problem
- Optimisation Models
- Basics of Graphic Method
- Important steps to draw graph
- Multiple, Unbounded Solution and Infeasible Problems
- Solving Linear Programming Graphically Using Computer
- Application of Linear Programming in Business and Industry
3 Linear Programming-Simplex Method
- Principle of Simplex Method
- Computational aspect of Simplex Method
- Simplex Method with several Decision Variables
- Two Phase and M-method
- Multiple Solution, Unbounded Solution and Infeasible Problem
- Sensitivity Analysis
- Dual Linear Programming Problem
4 Transportation Problem
- Basic Feasible Solution of a Transportation Problem
- Modified Distribution Method
- Stepping Stone Method
- Unbalanced Transportation Problem
- Degenerate Transportation Problem
- Transhipment Problem
- Maximisation in a Transportation Problem
5 Assignment Problem
- Solution of the Assignment Problem
- Unbalanced Assignment Problem
- Problem with some Infeasible Assignments
- Maximisation in an Assignment Problem
- Crew Assignment Problem
6 Application of Excel Solver to Solve LPP
- Building Excel model for solving LP: An Illustrative Example
7 Goal Programming
- Concepts of goal programming
- Goal programming model formulation
- Graphical method of goal programming
- The simplex method of goal programming
- Using Excel Solver to Solve Goal Programming Models
- Application areas of goal programming
8 Integer Programming
- Some Integer Programming Formulation Techniques
- Binary Representation of General Integer Variables
- Unimodularity
- Cutting Plane Method
- Branch and Bound Method
- Solver Solution
9 Dynamic Programming
- Dynamic Programming Methodology: An Example
- Definitions and Notations
- Dynamic Programming Applications
10 Non-Linear Programming
- Solution of a Non-linear Programming Problem
- Convex and Concave Functions
- Kuhn-Tucker Conditions for Constrained Optimisation
- Quadratic Programming
- Separable Programming
- NLP Models with Solver
11 Introduction to game theory and its Applications
- Important terms in Game Theory
- Saddle points
- Mixed strategies: Games without saddle points
- 2 x n games
- Exploiting an opponent’s mistakes
12 Monte Carlo Simulation
- Reasons for using simulation
- Monte Carlo simulation
- Limitations of simulation
- Steps in the simulation process
- Some practical applications of simulation
- Two typical examples of hand-computed simulation
- Computer simulation
13 Queueing Models
- Characteristics of a queueing model
- Notations and Symbols
- Statistical methods in queueing
- The M/M/I System
- The M/M/C System
- The M/Ek/I System
- Decision problems in queueing
Browse Course Material
Course info, instructors.
- Prof. Erik Demaine
- Prof. Srini Devadas
- Prof. Nancy Lynch
Departments
- Electrical Engineering and Computer Science
- Mathematics
As Taught In
- Algorithms and Data Structures
- Computer Networks
- Cryptography
- Applied Mathematics
Learning Resource Types
Design and analysis of algorithms, assignments.

You are leaving MIT OpenCourseWare
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Solutions to the Assignments for the Algorithmic Toolbox course offered by UCSanDiego on Coursera.
prantosky/coursera-algorithmic-toolbox
Folders and files, repository files navigation, algorithmic toolbox.
- Sum of Two Digits
- Maximum Pairwise Product
- Fibonacci Number
- Last Digit of a Large Fibonacci Number
- Greatest Common Divisor
- Least Common Multiple
- Fibonacci Number Again
- Last Digit of the Sum of Fibonacci Numbers
- Last Digit of the Sum of Fibonacci Numbers Again
- Last Digit of the Sum of Squares of Fibonacci Numbers
- Money Change
- Maximum Value of the Loot
- Car Fueling
- Maximum Advertisement Revenue
- Collecting Signatures
- Maximum Number of Prizes
- Maximum Salary
- Binary Search
- Majority Element
- Improving Quick Sort
- Number of Inversions
- Organizing a Lottery
- Closest Points
- Money Change Again
- Primitive Calculator
- Edit Distance
- Longest Common Subsequence of Two Sequences
- Longest Common Subsequence of Three Sequences
- Maximum Amount of Gold
- Approach 1 (Brute Force)
- Approach 2 (Dynamic Programming)
- Maximum Value of an Arithmetic Expression
Contributors 2
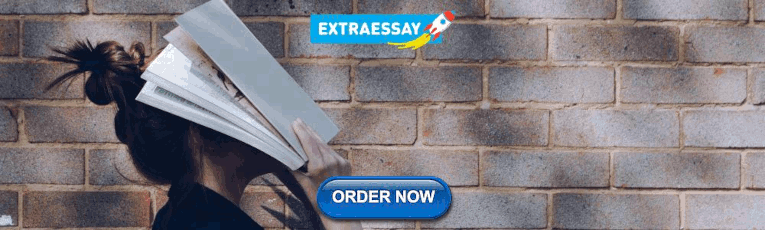
IMAGES
VIDEO
COMMENTS
In the balanced assignment problem, both parts of the bipartite graph have the same number of vertices, denoted by n. One of the first polynomial-time algorithms for balanced assignment was the Hungarian algorithm. It is a global algorithm - it is based on improving a matching along augmenting paths (alternating paths between unmatched vertices).
The Hungarian algorithm, aka Munkres assignment algorithm, utilizes the following theorem for polynomial runtime complexity (worst case O(n 3)) and guaranteed optimality: If a number is added to or subtracted from all of the entries of any one row or column of a cost matrix, then an optimal assignment for the resulting cost matrix is also an ...
Solution 1: Brute Force. We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O (n!). Solution 2: Hungarian Algorithm. The optimal assignment can be found using the Hungarian algorithm.
The Hungarian method is a combinatorial optimization algorithm that solves the assignment problem in polynomial time and which anticipated later primal-dual methods.It was developed and published in 1955 by Harold Kuhn, who gave it the name "Hungarian method" because the algorithm was largely based on the earlier works of two Hungarian mathematicians, Dénes Kőnig and Jenő Egerváry.
Hungarian algorithm steps for minimization problem. Step 1: For each row, subtract the minimum number in that row from all numbers in that row. Step 2: For each column, subtract the minimum number in that column from all numbers in that column. Step 3: Draw the minimum number of lines to cover all zeroes.
General description of the algorithm. This problem is known as the assignment problem. The assignment problem is a special case of the transportation problem, which in turn is a special case of the min-cost flow problem, so it can be solved using algorithms that solve the more general cases. Also, our problem is a special case of binary integer ...
For implementing the above algorithm, the idea is to use the max_cost_assignment() function defined in the dlib library. This function is an implementation of the Hungarian algorithm (also known as the Kuhn-Munkres algorithm) which runs in O(N 3) time. It solves the optimal assignment problem. Below is the implementation of the above approach:
A description of the algorithm in English and, if helpful, pseudocode. At least one worked example or diagram to show more precisely how your algorithm works. A proof (or indication) of the correctness of the algorithm. An analysis of the running time of the algorithm. Remember, your goal is to communicate.
The Hungarian Method: The following algorithm applies the above theorem to a given n × n cost matrix to find an optimal assignment. Step 1. Subtract the smallest entry in each row from all the entries of its row. Step 2. Subtract the smallest entry in each column from all the entries of its column. Step 3.
There are three building blocks of algorithms: sequencing, selection, and iteration. Sequencing is the sequential execution of operations, selection is the decision to execute one operation versus another operation (like a fork in the road), and iteration is repeating the same operations a certain number of times or until something is true.
First, we give a detailed review of two algorithms that solve the minimization case of the assignment problem, the Bertsekas auction algorithm and the Goldberg & Kennedy algorithm. It was previously alluded that both algorithms are equivalent. We give a detailed proof that these algorithms are equivalent. Also, we perform experimental results comparing the performance of three algorithms for ...
The complexity of this solution of the assignment problem depends on the algorithm by which the search for the maximum flow of the minimum cost is performed. The complexity will be $\mathcal{O}(N^3)$ using Dijkstra or $\mathcal{O}(N^4)$ using Bellman-Ford.
Total Cost= 2+8+4+6=20. Approach 3: Greedy Approach In this case, the algorithm will choose the lowest cost worker to be assigned to the task as the first assignment, then choose the next lowest ...
In this lesson we learn what is an assignment problem and how we can solve it using the Hungarian method.
The Hungarian method is an algorithm that uses a step-by-step approach to find the optimal assignment. The algorithm consists of the following steps: Subtract the smallest entry in each row from all the entries of the row. Subtract the smallest entry in each column from all the entries of the column. Draw the minimum number of lines to cover ...
The assignment problem is a problem that takes many forms in optimization and graph theory, and by changing some of the constraints or interpreting them differently and adding other constraints, it can be converted to routing, distribution, and scheduling problems. Showing such correlations is one of the aims of this paper. For some of the derived problems having exponential time complexity ...
The Hungarian method is a combinatorial optimization algorithm which solves the assignment problem in polynomial time . Later it was discovered that it was a primal-dual Simplex method.. It was developed and published by Harold Kuhn in 1955, who gave the name "Hungarian method" because the algorithm was largely based on the earlier works of two Hungarian mathematicians: Denes Konig and Jeno ...
Massachusetts Technology, MA Cambridge, 02139, U.S.A. and. Institute of. Received 15 August 1979 Revised manuscript 1980 received 6 October. Wepropose new algorithm a for the classical assignment problem. in some ways the Hungarian in method o er but respects. The av differs rag computational of an complexity effic ent of implementation the a ...
This is a snippet from some pseudocode for a sorting algorithm. In it, the symbol ← is used to denote assignment, for example for the variable done.However, in the while loop the statement done:= false is written. I would assume it is also an assignment statement but I suspect it means somethings else, or perhaps extra, since if not, the ← would have simple be used again.
An algorithm is a sequence of instructions that a computer must perform to solve a well-defined problem. It essentially defines what the computer needs to do and how to do it. Algorithms can instruct a computer how to perform a calculation, process data, or make a decision. The best way to understand an algorithm is to think of it as a recipe ...
assignment_turned_in Problem Sets with Solutions. grading Exams with Solutions. notes Lecture Notes. co_present Instructor Insights. Download Course. menu. search; Give Now; ... Class on Design and Analysis of Algorithms, Solutions to Problem Set 1. pdf. 181 kB Class on Design and Analysis of Algorithms, Problem Set 2. pdf.
whereby the assignment optimization is viewed as the primal problem and the minimization of the cost (1.2)-(1.3) is the dual problem.y Algorithms for Solving the Assignment Problem There are several iterative algorithms for the solution of the assignment problem, which are described in
1. Well, obviously you have to predict how many children (or simply total load) each process will have on average. Than you can use classic assignment algorithms, they're usually quite simple. The most important issue is of course to decide what you want to minimize. Usually we want to minimize lateness (how much "behind schedule" we get), but ...
Solutions to the Assignments for the Algorithmic Toolbox course offered by UCSanDiego on Coursera. - prantosky/coursera-algorithmic-toolbox