
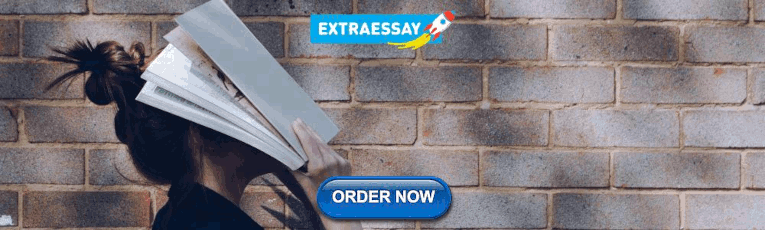
1.4 — Variable assignment and initialization
In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we’ll explore how to actually put values into variables and use those values.
As a reminder, here’s a short snippet that first allocates a single integer variable named x , then allocates two more integer variables named y and z :
Variable assignment
After a variable has been defined, you can give it a value (in a separate statement) using the = operator . This process is called assignment , and the = operator is called the assignment operator .
By default, assignment copies the value on the right-hand side of the = operator to the variable on the left-hand side of the operator. This is called copy assignment .
Here’s an example where we use assignment twice:
This prints:
When we assign value 7 to variable width , the value 5 that was there previously is overwritten. Normal variables can only hold one value at a time.
One of the most common mistakes that new programmers make is to confuse the assignment operator ( = ) with the equality operator ( == ). Assignment ( = ) is used to assign a value to a variable. Equality ( == ) is used to test whether two operands are equal in value.
Initialization
One downside of assignment is that it requires at least two statements: one to define the variable, and another to assign the value.
These two steps can be combined. When an object is defined, you can optionally give it an initial value. The process of specifying an initial value for an object is called initialization , and the syntax used to initialize an object is called an initializer .
In the above initialization of variable width , { 5 } is the initializer, and 5 is the initial value.
Different forms of initialization
Initialization in C++ is surprisingly complex, so we’ll present a simplified view here.
There are 6 basic ways to initialize variables in C++:
You may see the above forms written with different spacing (e.g. int d{7}; ). Whether you use extra spaces for readability or not is a matter of personal preference.
Default initialization
When no initializer is provided (such as for variable a above), this is called default initialization . In most cases, default initialization performs no initialization, and leaves a variable with an indeterminate value.
We’ll discuss this case further in lesson ( 1.6 -- Uninitialized variables and undefined behavior ).
Copy initialization
When an initial value is provided after an equals sign, this is called copy initialization . This form of initialization was inherited from C.
Much like copy assignment, this copies the value on the right-hand side of the equals into the variable being created on the left-hand side. In the above snippet, variable width will be initialized with value 5 .
Copy initialization had fallen out of favor in modern C++ due to being less efficient than other forms of initialization for some complex types. However, C++17 remedied the bulk of these issues, and copy initialization is now finding new advocates. You will also find it used in older code (especially code ported from C), or by developers who simply think it looks more natural and is easier to read.
For advanced readers
Copy initialization is also used whenever values are implicitly copied or converted, such as when passing arguments to a function by value, returning from a function by value, or catching exceptions by value.
Direct initialization
When an initial value is provided inside parenthesis, this is called direct initialization .
Direct initialization was initially introduced to allow for more efficient initialization of complex objects (those with class types, which we’ll cover in a future chapter). Just like copy initialization, direct initialization had fallen out of favor in modern C++, largely due to being superseded by list initialization. However, we now know that list initialization has a few quirks of its own, and so direct initialization is once again finding use in certain cases.
Direct initialization is also used when values are explicitly cast to another type.
One of the reasons direct initialization had fallen out of favor is because it makes it hard to differentiate variables from functions. For example:
List initialization
The modern way to initialize objects in C++ is to use a form of initialization that makes use of curly braces. This is called list initialization (or uniform initialization or brace initialization ).
List initialization comes in three forms:
As an aside…
Prior to the introduction of list initialization, some types of initialization required using copy initialization, and other types of initialization required using direct initialization. List initialization was introduced to provide a more consistent initialization syntax (which is why it is sometimes called “uniform initialization”) that works in most cases.
Additionally, list initialization provides a way to initialize objects with a list of values (which is why it is called “list initialization”). We show an example of this in lesson 16.2 -- Introduction to std::vector and list constructors .
List initialization has an added benefit: “narrowing conversions” in list initialization are ill-formed. This means that if you try to brace initialize a variable using a value that the variable can not safely hold, the compiler is required to produce a diagnostic (usually an error). For example:
In the above snippet, we’re trying to assign a number (4.5) that has a fractional part (the .5 part) to an integer variable (which can only hold numbers without fractional parts).
Copy and direct initialization would simply drop the fractional part, resulting in the initialization of value 4 into variable width . Your compiler may optionally warn you about this, since losing data is rarely desired. However, with list initialization, your compiler is required to generate a diagnostic in such cases.
Conversions that can be done without potential data loss are allowed.
To summarize, list initialization is generally preferred over the other initialization forms because it works in most cases (and is therefore most consistent), it disallows narrowing conversions, and it supports initialization with lists of values (something we’ll cover in a future lesson). While you are learning, we recommend sticking with list initialization (or value initialization).
Best practice
Prefer direct list initialization (or value initialization) for initializing your variables.
Author’s note
Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) also recommend using list initialization to initialize your variables.
In modern C++, there are some cases where list initialization does not work as expected. We cover one such case in lesson 16.2 -- Introduction to std::vector and list constructors .
Because of such quirks, some experienced developers now advocate for using a mix of copy, direct, and list initialization, depending on the circumstance. Once you are familiar enough with the language to understand the nuances of each initialization type and the reasoning behind such recommendations, you can evaluate on your own whether you find these arguments persuasive.
Value initialization and zero initialization
When a variable is initialized using empty braces, value initialization takes place. In most cases, value initialization will initialize the variable to zero (or empty, if that’s more appropriate for a given type). In such cases where zeroing occurs, this is called zero initialization .
Q: When should I initialize with { 0 } vs {}?
Use an explicit initialization value if you’re actually using that value.
Use value initialization if the value is temporary and will be replaced.
Initialize your variables
Initialize your variables upon creation. You may eventually find cases where you want to ignore this advice for a specific reason (e.g. a performance critical section of code that uses a lot of variables), and that’s okay, as long the choice is made deliberately.
Related content
For more discussion on this topic, Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) make this recommendation themselves here .
We explore what happens if you try to use a variable that doesn’t have a well-defined value in lesson 1.6 -- Uninitialized variables and undefined behavior .
Initialize your variables upon creation.
Initializing multiple variables
In the last section, we noted that it is possible to define multiple variables of the same type in a single statement by separating the names with a comma:
We also noted that best practice is to avoid this syntax altogether. However, since you may encounter other code that uses this style, it’s still useful to talk a little bit more about it, if for no other reason than to reinforce some of the reasons you should be avoiding it.
You can initialize multiple variables defined on the same line:
Unfortunately, there’s a common pitfall here that can occur when the programmer mistakenly tries to initialize both variables by using one initialization statement:
In the top statement, variable “a” will be left uninitialized, and the compiler may or may not complain. If it doesn’t, this is a great way to have your program intermittently crash or produce sporadic results. We’ll talk more about what happens if you use uninitialized variables shortly.
The best way to remember that this is wrong is to consider the case of direct initialization or brace initialization:
Because the parenthesis or braces are typically placed right next to the variable name, this makes it seem a little more clear that the value 5 is only being used to initialize variable b and d , not a or c .
Unused initialized variables warnings
Modern compilers will typically generate warnings if a variable is initialized but not used (since this is rarely desirable). And if “treat warnings as errors” is enabled, these warnings will be promoted to errors and cause the compilation to fail.
Consider the following innocent looking program:
When compiling this with the g++ compiler, the following error is generated:
and the program fails to compile.
There are a few easy ways to fix this.
- If the variable really is unused, then the easiest option is to remove the defintion of x (or comment it out). After all, if it’s not used, then removing it won’t affect anything.
- Another option is to simply use the variable somewhere:
But this requires some effort to write code that uses it, and has the downside of potentially changing your program’s behavior.
The [[maybe_unused]] attribute C++17
In some cases, neither of the above options are desirable. Consider the case where we have a bunch of math/physics values that we use in many different programs:
If we use these a lot, we probably have these saved somewhere and copy/paste/import them all together.
However, in any program where we don’t use all of these values, the compiler will complain about each variable that isn’t actually used. While we could go through and remove/comment out the unused ones for each program, this takes time and energy. And later if we need one that we’ve previously removed, we’ll have to go back and re-add it.
To address such cases, C++17 introduced the [[maybe_unused]] attribute, which allows us to tell the compiler that we’re okay with a variable being unused. The compiler will not generate unused variable warnings for such variables.
The following program should generate no warnings/errors:
Additionally, the compiler will likely optimize these variables out of the program, so they have no performance impact.
In future lessons, we’ll often define variables we don’t use again, in order to demonstrate certain concepts. Making use of [[maybe_unused]] allows us to do so without compilation warnings/errors.
Question #1
What is the difference between initialization and assignment?
Show Solution
Initialization gives a variable an initial value at the point when it is created. Assignment gives a variable a value at some point after the variable is created.
Question #2
What form of initialization should you prefer when you want to initialize a variable with a specific value?
Direct list initialization (aka. direct brace initialization).
Question #3
What are default initialization and value initialization? What is the behavior of each? Which should you prefer?
Default initialization is when a variable initialization has no initializer (e.g. int x; ). In most cases, the variable is left with an indeterminate value.
Value initialization is when a variable initialization has an empty brace (e.g. int x{}; ). In most cases this will perform zero-initialization.
You should prefer value initialization to default initialization.
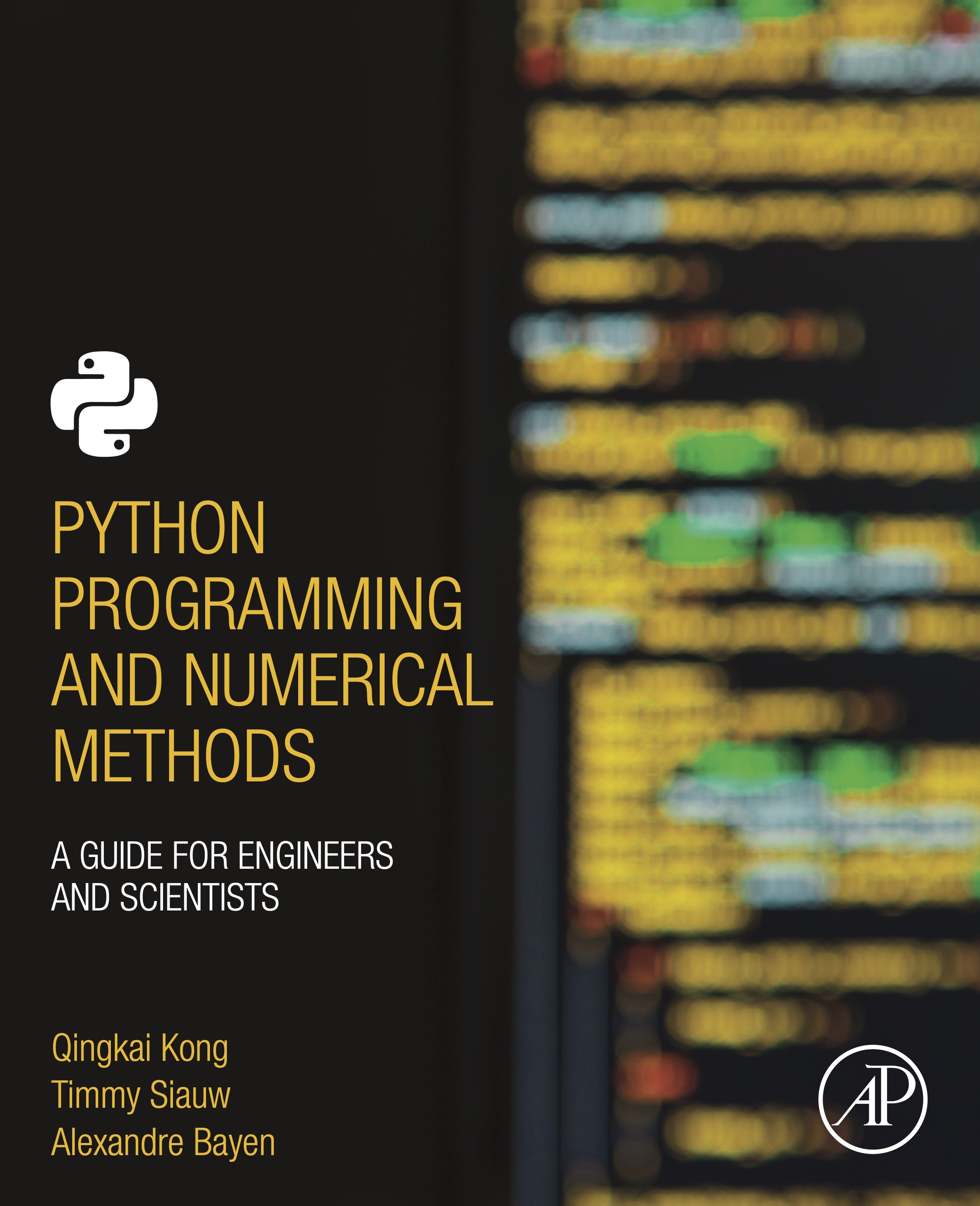
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
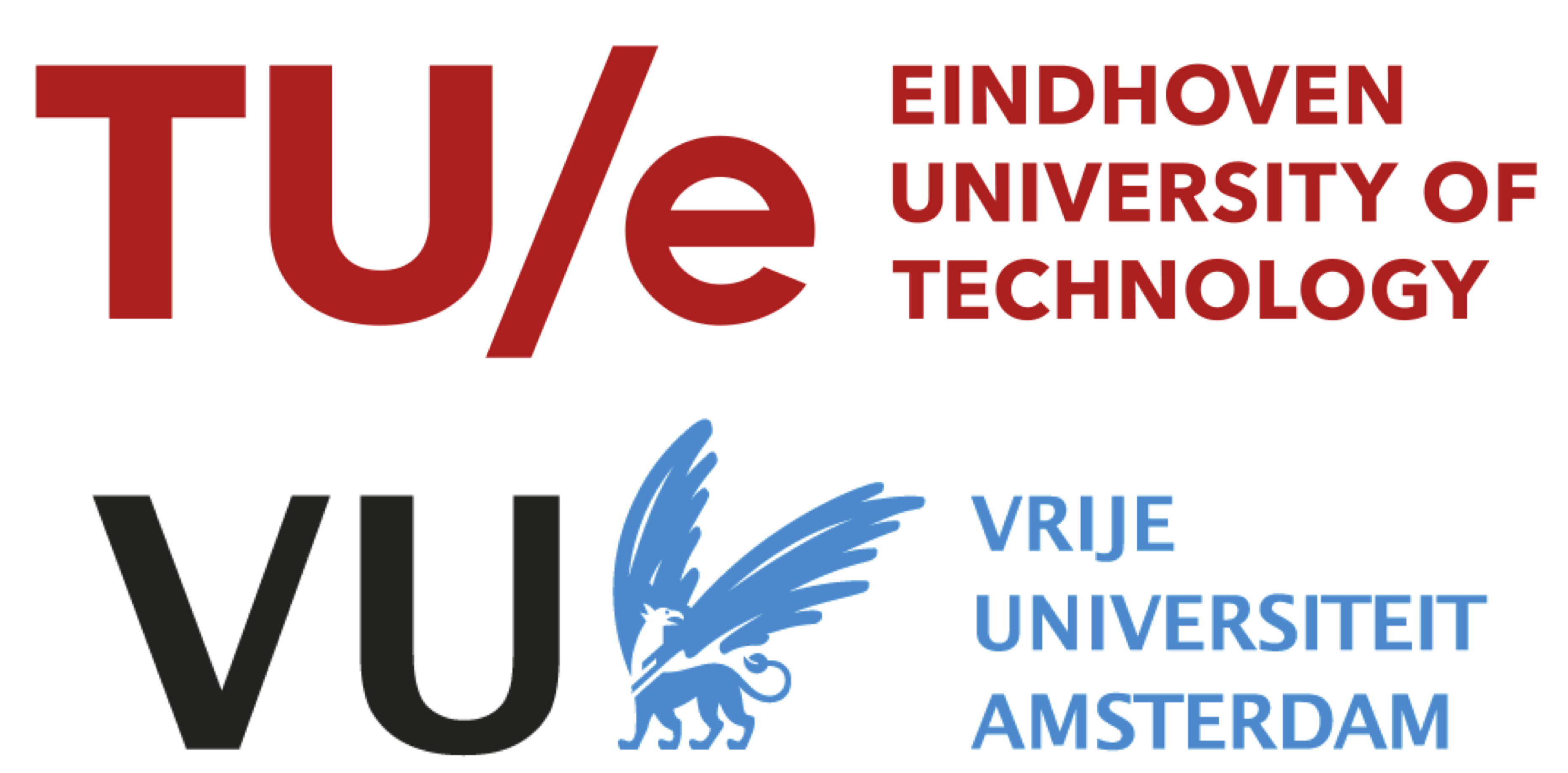
Learning Python by doing
- suggest edit
Variables, Expressions, and Assignments
Variables, expressions, and assignments 1 #, introduction #.
In this chapter, we introduce some of the main building blocks needed to create programs–that is, variables, expressions, and assignments. Programming related variables can be intepret in the same way that we interpret mathematical variables, as elements that store values that can later be changed. Usually, variables and values are used within the so-called expressions. Once again, just as in mathematics, an expression is a construct of values and variables connected with operators that result in a new value. Lastly, an assignment is a language construct know as an statement that assign a value (either as a constant or expression) to a variable. The rest of this notebook will dive into the main concepts that we need to fully understand these three language constructs.
Values and Types #
A value is the basic unit used in a program. It may be, for instance, a number respresenting temperature. It may be a string representing a word. Some values are 42, 42.0, and ‘Hello, Data Scientists!’.
Each value has its own type : 42 is an integer ( int in Python), 42.0 is a floating-point number ( float in Python), and ‘Hello, Data Scientists!’ is a string ( str in Python).
The Python interpreter can tell you the type of a value: the function type takes a value as argument and returns its corresponding type.
Observe the difference between type(42) and type('42') !
Expressions and Statements #
On the one hand, an expression is a combination of values, variables, and operators.
A value all by itself is considered an expression, and so is a variable.
When you type an expression at the prompt, the interpreter evaluates it, which means that it calculates the value of the expression and displays it.
In boxes above, m has the value 27 and m + 25 has the value 52 . m + 25 is said to be an expression.
On the other hand, a statement is an instruction that has an effect, like creating a variable or displaying a value.
The first statement initializes the variable n with the value 17 , this is a so-called assignment statement .
The second statement is a print statement that prints the value of the variable n .
The effect is not always visible. Assigning a value to a variable is not visible, but printing the value of a variable is.
Assignment Statements #
We have already seen that Python allows you to evaluate expressions, for instance 40 + 2 . It is very convenient if we are able to store the calculated value in some variable for future use. The latter can be done via an assignment statement. An assignment statement creates a new variable with a given name and assigns it a value.
The example in the previous code contains three assignments. The first one assigns the value of the expression 40 + 2 to a new variable called magicnumber ; the second one assigns the value of π to the variable pi , and; the last assignment assigns the string value 'Data is eatig the world' to the variable message .
Programmers generally choose names for their variables that are meaningful. In this way, they document what the variable is used for.
Do It Yourself!
Let’s compute the volume of a cube with side \(s = 5\) . Remember that the volume of a cube is defined as \(v = s^3\) . Assign the value to a variable called volume .
Well done! Now, why don’t you print the result in a message? It can say something like “The volume of the cube with side 5 is \(volume\) ”.
Beware that there is no checking of types ( type checking ) in Python, so a variable to which you have assigned an integer may be re-used as a float, even if we provide type-hints .
Names and Keywords #
Names of variable and other language constructs such as functions (we will cover this topic later), should be meaningful and reflect the purpose of the construct.
In general, Python names should adhere to the following rules:
It should start with a letter or underscore.
It cannot start with a number.
It must only contain alpha-numeric (i.e., letters a-z A-Z and digits 0-9) characters and underscores.
They cannot share the name of a Python keyword.
If you use illegal variable names you will get a syntax error.
By choosing the right variables names you make the code self-documenting, what is better the variable v or velocity ?
The following are examples of invalid variable names.
These basic development principles are sometimes called architectural rules . By defining and agreeing upon architectural rules you make it easier for you and your fellow developers to understand and modify your code.
If you want to read more on this, please have a look at Code complete a book by Steven McConnell [ McC04 ] .
Every programming language has a collection of reserved keywords . They are used in predefined language constructs, such as loops and conditionals . These language concepts and their usage will be explained later.
The interpreter uses keywords to recognize these language constructs in a program. Python 3 has the following keywords:
False class finally is return
None continue for lambda try
True def from nonlocal while
and del global not with
as elif if or yield
assert else import pass break
except in raise
Reassignments #
It is allowed to assign a new value to an existing variable. This process is called reassignment . As soon as you assign a value to a variable, the old value is lost.
The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well.
You have a variable salary that shows the weekly salary of an employee. However, you want to compute the monthly salary. Can you reassign the value to the salary variable according to the instruction?
Updating Variables #
A frequently used reassignment is for updating puposes: the value of a variable depends on the previous value of the variable.
This statement expresses “get the current value of x , add one, and then update x with the new value.”
Beware, that the variable should be initialized first, usually with a simple assignment.
Do you remember the salary excercise of the previous section (cf. 13. Reassignments)? Well, if you have not done it yet, update the salary variable by using its previous value.
Updating a variable by adding 1 is called an increment ; subtracting 1 is called a decrement . A shorthand way of doing is using += and -= , which stands for x = x + ... and x = x - ... respectively.
Order of Operations #
Expressions may contain multiple operators. The order of evaluation depends on the priorities of the operators also known as rules of precedence .
For mathematical operators, Python follows mathematical convention. The acronym PEMDAS is a useful way to remember the rules:
Parentheses have the highest precedence and can be used to force an expression to evaluate in the order you want. Since expressions in parentheses are evaluated first, 2 * (3 - 1) is 4 , and (1 + 1)**(5 - 2) is 8 . You can also use parentheses to make an expression easier to read, even if it does not change the result.
Exponentiation has the next highest precedence, so 1 + 2**3 is 9 , not 27 , and 2 * 3**2 is 18 , not 36 .
Multiplication and division have higher precedence than addition and subtraction . So 2 * 3 - 1 is 5 , not 4 , and 6 + 4 / 2 is 8 , not 5 .
Operators with the same precedence are evaluated from left to right (except exponentiation). So in the expression degrees / 2 * pi , the division happens first and the result is multiplied by pi . To divide by 2π, you can use parentheses or write: degrees / 2 / pi .
In case of doubt, use parentheses!
Let’s see what happens when we evaluate the following expressions. Just run the cell to check the resulting value.
Floor Division and Modulus Operators #
The floor division operator // divides two numbers and rounds down to an integer.
For example, suppose that driving to the south of France takes 555 minutes. You might want to know how long that is in hours.
Conventional division returns a floating-point number.
Hours are normally not represented with decimal points. Floor division returns the integer number of hours, dropping the fraction part.
You spend around 225 minutes every week on programming activities. You want to know around how many hours you invest to this activity during a month. Use the \(//\) operator to give the answer.
The modulus operator % works on integer values. It computes the remainder when dividing the first integer by the second one.
The modulus operator is more useful than it seems.
For example, you can check whether one number is divisible by another—if x % y is zero, then x is divisible by y .
String Operations #
In general, you cannot perform mathematical operations on strings, even if the strings look like numbers, so the following operations are illegal: '2'-'1' 'eggs'/'easy' 'third'*'a charm'
But there are two exceptions, + and * .
The + operator performs string concatenation, which means it joins the strings by linking them end-to-end.
The * operator also works on strings; it performs repetition.
Speedy Gonzales is a cartoon known to be the fastest mouse in all Mexico . He is also famous for saying “Arriba Arriba Andale Arriba Arriba Yepa”. Can you use the following variables, namely arriba , andale and yepa to print the mentioned expression? Don’t forget to use the string operators.
Asking the User for Input #
The programs we have written so far accept no input from the user.
To get data from the user through the Python prompt, we can use the built-in function input .
When input is called your whole program stops and waits for the user to enter the required data. Once the user types the value and presses Return or Enter , the function returns the input value as a string and the program continues with its execution.
Try it out!
You can also print a message to clarify the purpose of the required input as follows.
The resulting string can later be translated to a different type, like an integer or a float. To do so, you use the functions int and float , respectively. But be careful, the user might introduce a value that cannot be converted to the type you required.
We want to know the name of a user so we can display a welcome message in our program. The message should say something like “Hello \(name\) , welcome to our hello world program!”.
Script Mode #
So far we have run Python in interactive mode in these Jupyter notebooks, which means that you interact directly with the interpreter in the code cells . The interactive mode is a good way to get started, but if you are working with more than a few lines of code, it can be clumsy. The alternative is to save code in a file called a script and then run the interpreter in script mode to execute the script. By convention, Python scripts have names that end with .py .
Use the PyCharm icon in Anaconda Navigator to create and execute stand-alone Python scripts. Later in the course, you will have to work with Python projects for the assignments, in order to get acquainted with another way of interacing with Python code.
This Jupyter Notebook is based on Chapter 2 of the books Python for Everybody [ Sev16 ] and Think Python (Sections 5.1, 7.1, 7.2, and 5.12) [ Dow15 ] .
Python Variables and Assignment
Python variables, variable assignment rules, every value has a type, memory and the garbage collector, variable swap, variable names are superficial labels, assignment = is shallow, decomp by var.
- Module 2: The Essentials of Python »
- Variables & Assignment
- View page source
Variables & Assignment
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
Variables permit us to write code that is flexible and amendable to repurpose. Suppose we want to write code that logs a student’s grade on an exam. The logic behind this process should not depend on whether we are logging Brian’s score of 92% versus Ashley’s score of 94%. As such, we can utilize variables, say name and grade , to serve as placeholders for this information. In this subsection, we will demonstrate how to define variables in Python.
In Python, the = symbol represents the “assignment” operator. The variable goes to the left of = , and the object that is being assigned to the variable goes to the right:
Attempting to reverse the assignment order (e.g. 92 = name ) will result in a syntax error. When a variable is assigned an object (like a number or a string), it is common to say that the variable is a reference to that object. For example, the variable name references the string "Brian" . This means that, once a variable is assigned an object, it can be used elsewhere in your code as a reference to (or placeholder for) that object:
Valid Names for Variables
A variable name may consist of alphanumeric characters ( a-z , A-Z , 0-9 ) and the underscore symbol ( _ ); a valid name cannot begin with a numerical value.
var : valid
_var2 : valid
ApplePie_Yum_Yum : valid
2cool : invalid (begins with a numerical character)
I.am.the.best : invalid (contains . )
They also cannot conflict with character sequences that are reserved by the Python language. As such, the following cannot be used as variable names:
for , while , break , pass , continue
in , is , not
if , else , elif
def , class , return , yield , raises
import , from , as , with
try , except , finally
There are other unicode characters that are permitted as valid characters in a Python variable name, but it is not worthwhile to delve into those details here.
Mutable and Immutable Objects
The mutability of an object refers to its ability to have its state changed. A mutable object can have its state changed, whereas an immutable object cannot. For instance, a list is an example of a mutable object. Once formed, we are able to update the contents of a list - replacing, adding to, and removing its elements.
To spell out what is transpiring here, we:
Create (initialize) a list with the state [1, 2, 3] .
Assign this list to the variable x ; x is now a reference to that list.
Using our referencing variable, x , update element-0 of the list to store the integer -4 .
This does not create a new list object, rather it mutates our original list. This is why printing x in the console displays [-4, 2, 3] and not [1, 2, 3] .
A tuple is an example of an immutable object. Once formed, there is no mechanism by which one can change of the state of a tuple; and any code that appears to be updating a tuple is in fact creating an entirely new tuple.
Mutable & Immutable Types of Objects
The following are some common immutable and mutable objects in Python. These will be important to have in mind as we start to work with dictionaries and sets.
Some immutable objects
numbers (integers, floating-point numbers, complex numbers)
“frozen”-sets
Some mutable objects
dictionaries
NumPy arrays
Referencing a Mutable Object with Multiple Variables
It is possible to assign variables to other, existing variables. Doing so will cause the variables to reference the same object:
What this entails is that these common variables will reference the same instance of the list. Meaning that if the list changes, all of the variables referencing that list will reflect this change:
We can see that list2 is still assigned to reference the same, updated list as list1 :
In general, assigning a variable b to a variable a will cause the variables to reference the same object in the system’s memory, and assigning c to a or b will simply have a third variable reference this same object. Then any change (a.k.a mutation ) of the object will be reflected in all of the variables that reference it ( a , b , and c ).
Of course, assigning two variables to identical but distinct lists means that a change to one list will not affect the other:
Reading Comprehension: Does slicing a list produce a reference to that list?
Suppose x is assigned a list, and that y is assigned a “slice” of x . Do x and y reference the same list? That is, if you update part of the subsequence common to x and y , does that change show up in both of them? Write some simple code to investigate this.
Reading Comprehension: Understanding References
Based on our discussion of mutable and immutable objects, predict what the value of y will be in the following circumstance:
Reading Comprehension Exercise Solutions:
Does slicing a list produce a reference to that list?: Solution
Based on the following behavior, we can conclude that slicing a list does not produce a reference to the original list. Rather, slicing a list produces a copy of the appropriate subsequence of the list:
Understanding References: Solutions
Integers are immutable, thus x must reference an entirely new object ( 9 ), and y still references 3 .
CS105: Introduction to Python
Variables and assignment statements.
Computers must be able to remember and store data. This can be accomplished by creating a variable to house a given value. The assignment operator = is used to associate a variable name with a given value. For example, type the command:
in the command line window. This command assigns the value 3.45 to the variable named a . Next, type the command:
in the command window and hit the enter key. You should see the value contained in the variable a echoed to the screen. This variable will remember the value 3.45 until it is assigned a different value. To see this, type these two commands:
You should see the new value contained in the variable a echoed to the screen. The new value has "overwritten" the old value. We must be careful since once an old value has been overwritten, it is no longer remembered. The new value is now what is being remembered.
Although we will not discuss arithmetic operations in detail until the next unit, you can at least be equipped with the syntax for basic operations: + (addition), - (subtraction), * (multiplication), / (division)
For example, entering these command sequentially into the command line window:
would result in 12.32 being echoed to the screen (just as you would expect from a calculator). The syntax for multiplication works similarly. For example:
would result in 35 being echoed to the screen because the variable b has been assigned the value a * 5 where, at the time of execution, the variable a contains a value of 7.
After you read, you should be able to execute simple assignment commands using integer and float values in the command window of the Repl.it IDE. Try typing some more of the examples from this web page to convince yourself that a variable has been assigned a specific value.
In programming, we associate names with values so that we can remember and use them later. Recall Example 1. The repeated computation in that algorithm relied on remembering the intermediate sum and the integer to be added to that sum to get the new sum. In expressing the algorithm, we used th e names current and sum .
In programming, a name that refers to a value in this fashion is called a variable . When we think of values as data stored somewhere i n the computer, we can have a mental image such as the one below for the value 10 stored in the computer and the variable x , which is the name we give to 10. What is most important is to see that there is a binding between x and 10.
The term variable comes from the fact that values that are bound to variables can change throughout computation. Bindings as shown above are created, and changed by assignment statements . An assignment statement associates the name to the left of the symbol = with the value denoted by the expression on the right of =. The binding in the picture is created using an assignment statemen t of the form x = 10 . We usually read such an assignment statement as "10 is assigned to x" or "x is set to 10".
If we want to change the value that x refers to, we can use another assignment statement to do that. Suppose we execute x = 25 in the state where x is bound to 10.Then our image becomes as follows:
Choosing variable names
Suppose that we u sed the variables x and y in place of the variables side and area in the examples above. Now, if we were to compute some other value for the square that depends on the length of the side , such as the perimeter or length of the diagonal, we would have to remember which of x and y , referred to the length of the side because x and y are not as descriptive as side and area . In choosing variable names, we have to keep in mind that programs are read and maintained by human beings, not only executed by machines.
Note about syntax
In Python, variable identifiers can contain uppercase and lowercase letters, digits (provided they don't start with a digit) and the special character _ (underscore). Although it is legal to use uppercase letters in variable identifiers, we typically do not use them by convention. Variable identifiers are also case-sensitive. For example, side and Side are two different variable identifiers.
There is a collection of words, called reserved words (also known as keywords), in Python that have built-in meanings and therefore cannot be used as variable names. For the list of Python's keywords See 2.3.1 of the Python Language Reference.
Syntax and Sema ntic Errors
Now that we know how to write arithmetic expressions and assignment statements in Python, we can pause and think about what Python does if we write something that the Python interpreter cannot interpret. Python informs us about such problems by giving an error message. Broadly speaking there are two categories for Python errors:
- Syntax errors: These occur when we write Python expressions or statements that are not well-formed according to Python's syntax. For example, if we attempt to write an assignment statement such as 13 = age , Python gives a syntax error. This is because Python syntax says that for an assignment statement to be well-formed it must contain a variable on the left hand side (LHS) of the assignment operator "=" and a well-formed expression on the right hand side (RHS), and 13 is not a variable.
- Semantic errors: These occur when the Python interpreter cannot evaluate expressions or execute statements because they cannot be associated with a "meaning" that the interpreter can use. For example, the expression age + 1 is well-formed but it has a meaning only when age is already bound to a value. If we attempt to evaluate this expression before age is bound to some value by a prior assignment then Python gives a semantic error.
Even though we have used numerical expressions in all of our examples so far, assignments are not confined to numerical types. They could involve expressions built from any defined type. Recall the table that summarizes the basic types in Python.
The following video shows execution of assignment statements involving strings. It also introduces some commonly used operators on strings. For more information see the online documentation. In the video below, you see the Python shell displaying "=> None" after the assignment statements. This is unique to the Python shell presented in the video. In most Python programming environments, nothing is displayed after an assignment statement. The difference in behavior stems from version differences between the programming environment used in the video and in the activities, and can be safely ignored.
Distinguishing Expressions and Assignments
So far in the module, we have been careful to keep the distinction between the terms expression and statement because there is a conceptual difference between them, which is sometimes overlooked. Expressions denote values; they are evaluated to yield a value. On the other hand, statements are commands (instructions) that change the state of the computer. You can think of state here as some representation of computer memory and the binding of variables and values in the memory. In a state where the variable side is bound to the integer 3, and the variable area is yet unbound, the value of the expression side + 2 is 5. The assignment statement side = side + 2 , changes the state so that value 5 is bound to side in the new state. Note that when you type an expression in the Python shell, Python evaluates the expression and you get a value in return. On the other hand, if you type an assignment statement nothing is returned. Assignment statements do not return a value. Try, for example, typing x = 100 + 50 . Python adds 100 to 50, gets the value 150, and binds x to 150. However, we only see the prompt >>> after Python does the assignment. We don't see the change in the state until we inspect the value of x , by invoking x .
What we have learned so far can be summarized as using the Python interpreter to manipulate values of some primitive data types such as integers, real numbers, and character strings by evaluating expressions that involve built-in operators on these types. Assignments statements let us name the values that appear in expressions. While what we have learned so far allows us to do some computations conveniently, they are limited in their generality and reusability. Next, we introduce functions as a means to make computations more general and reusable.

- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- CBSE Class 11 Informatics Practices Syllabus 2023-24
- CBSE Class 11 Information Practices Syllabus 2023-24 Distribution of Marks
- CBSE Class 11 Informatics Practices Unit-wise Syllabus
- Unit 1:Introduction to Computer System
- Introduction to Computer System
- Evolution of Computer
- Computer Memory
- Unit 2:Introduction to Python
- Python Keywords
- Identifiers
- Expressions
- Input and Output
- if else Statements
- Nested Loops
- Working with Lists and Dictionaries
- Introduction to List
- List Operations
- Traversing a List
- List Methods and Built in Functions
- Introduction to Dictionaries
- Traversing a Dictionary
- Dictionary Methods and Built-in Functions
- Unit 3 Data Handling using NumPy
- Introduction
- NumPy Array
- Indexing and Slicing
- Operations on Arrays
- Concatenating Arrays
- Reshaping Arrays
- Splitting Arrays
- Saving NumPy Arrays in Files on Disk
- Unit 4: Database Concepts and the Structured Query Language
- Understanding Data
- Introduction to Data
- Data Collection
- Data Storage
- Data Processing
- Statistical Techniques for Data Processing
- Measures of Central Tendency
- Database Concepts
- Introduction to Structured Query Language
- Structured Query Language
- Data Types and Constraints in MySQL
- CREATE Database
- CREATE Table
- DESCRIBE Table
- ALTER Table
- SQL for Data Manipulation
- SQL for Data Query
- Data Updation and Deletion
- Unit 5 Introduction to Emerging Trends
- Artificial Intelligence
- Internet of Things
- Cloud Computing
- Grid Computing
- Blockchains
- Class 11 IP Syllabus Practical and Theory Components
Python Variables
Python Variable is containers that store values. Python is not “statically typed”. We do not need to declare variables before using them or declare their type. A variable is created the moment we first assign a value to it. A Python variable is a name given to a memory location. It is the basic unit of storage in a program. In this article, we will see how to define a variable in Python .
Example of Variable in Python
An Example of a Variable in Python is a representational name that serves as a pointer to an object. Once an object is assigned to a variable, it can be referred to by that name. In layman’s terms, we can say that Variable in Python is containers that store values.
Here we have stored “ Geeksforgeeks ” in a variable var , and when we call its name the stored information will get printed.
Notes: The value stored in a variable can be changed during program execution. A Variables in Python is only a name given to a memory location, all the operations done on the variable effects that memory location.
Rules for Python variables
- A Python variable name must start with a letter or the underscore character.
- A Python variable name cannot start with a number.
- A Python variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ).
- Variable in Python names are case-sensitive (name, Name, and NAME are three different variables).
- The reserved words(keywords) in Python cannot be used to name the variable in Python.
Variables Assignment in Python
Here, we will define a variable in python. Here, clearly we have assigned a number, a floating point number, and a string to a variable such as age, salary, and name.
Declaration and Initialization of Variables
Let’s see how to declare a variable and how to define a variable and print the variable.
Redeclaring variables in Python
We can re-declare the Python variable once we have declared the variable and define variable in python already.
Python Assign Values to Multiple Variables
Also, Python allows assigning a single value to several variables simultaneously with “=” operators. For example:
Assigning different values to multiple variables
Python allows adding different values in a single line with “,” operators.
Can We Use the S ame Name for Different Types?
If we use the same name, the variable starts referring to a new value and type.
How does + operator work with variables?
The Python plus operator + provides a convenient way to add a value if it is a number and concatenate if it is a string. If a variable is already created it assigns the new value back to the same variable.
Can we use + for different Datatypes also?
No use for different types would produce an error.
Global and Local Python Variables
Local variables in Python are the ones that are defined and declared inside a function. We can not call this variable outside the function.
Global variables in Python are the ones that are defined and declared outside a function, and we need to use them inside a function.
Global keyword in Python
Python global is a keyword that allows a user to modify a variable outside of the current scope. It is used to create global variables from a non-global scope i.e inside a function. Global keyword is used inside a function only when we want to do assignments or when we want to change a variable. Global is not needed for printing and accessing.
Rules of global keyword
- If a variable is assigned a value anywhere within the function’s body, it’s assumed to be local unless explicitly declared as global.
- Variables that are only referenced inside a function are implicitly global.
- We use a global in Python to use a global variable inside a function.
- There is no need to use a global keyword in Python outside a function.
Python program to modify a global value inside a function.
Variable Types in Python
Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data. Since everything is an object in Python programming, data types are actually classes and variables are instances (object) of these classes.
Built-in Python Data types are:
- Sequence Type ( Python list , Python tuple , Python range )
In this example, we have shown different examples of Built-in data types in Python.
Object Reference in Python
Let us assign a variable x to value 5.
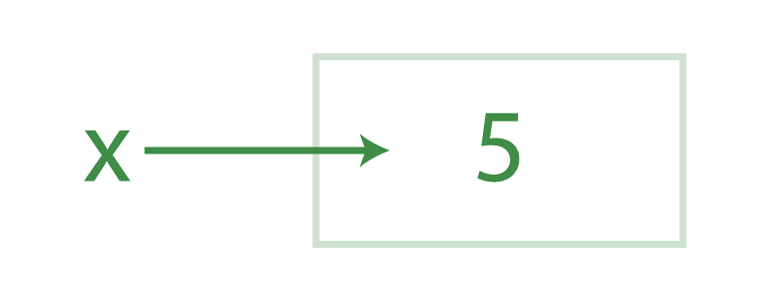
Another variable is y to the variable x.
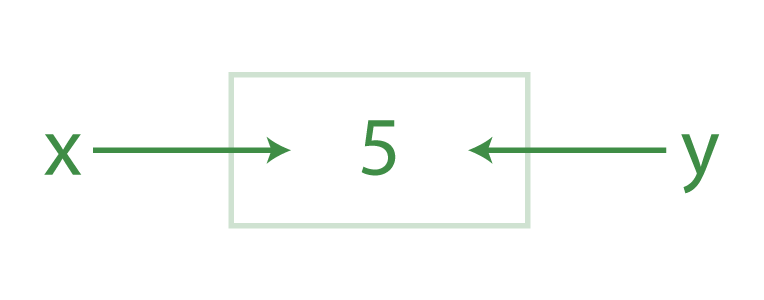
When Python looks at the first statement, what it does is that, first, it creates an object to represent the value 5. Then, it creates the variable x if it doesn’t exist and made it a reference to this new object 5. The second line causes Python to create the variable y, and it is not assigned with x, rather it is made to reference that object that x does. The net effect is that the variables x and y wind up referencing the same object. This situation, with multiple names referencing the same object, is called a Shared Reference in Python. Now, if we write:
This statement makes a new object to represent ‘Geeks’ and makes x reference this new object.
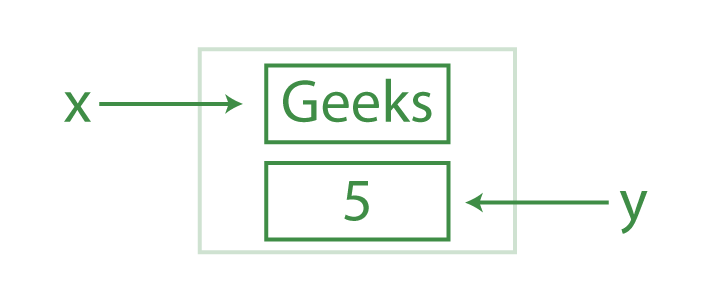
Now if we assign the new value in Y, then the previous object refers to the garbage values.
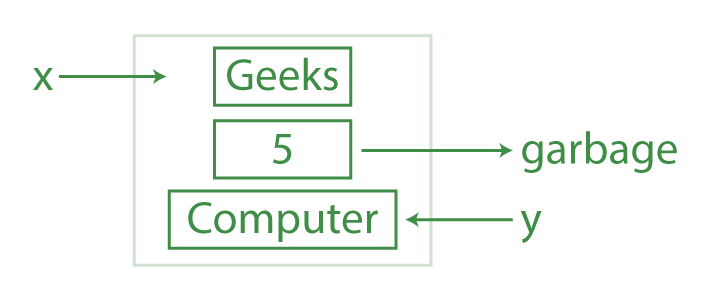
Creating objects (or variables of a class type)
Please refer to Class, Object, and Members for more details.
Frequently Asked Questions
1. how to define a variable in python.
In Python, we can define a variable by assigning a value to a name. Variable names must start with a letter (a-z, A-Z) or an underscore (_) and can be followed by letters, underscores, or digits (0-9). Python is dynamically typed, meaning we don’t need to declare the variable type explicitly; it will be inferred based on the assigned value.
2. Are there naming conventions for Python variables?
Yes, Python follows the snake_case convention for variable names (e.g., my_variable ). They should start with a letter or underscore, followed by letters, underscores, or numbers. Constants are usually named in ALL_CAPS.
3. Can I change the type of a Python variable?
Yes, Python is dynamically typed, meaning you can change the type of a variable by assigning a new value of a different type. For instance:
Please Login to comment...
Similar reads.
- python-basics
- School Programming
- 10 Ways to Use Microsoft OneNote for Note-Taking
- 10 Best Yellow.ai Alternatives & Competitors in 2024
- 10 Best Online Collaboration Tools 2024
- 10 Best Autodesk Maya Alternatives for 3D Animation and Modeling in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Hiring? Flexiple helps you build your dream team of developers and designers .
How To Assign Values To Variables In Python?

Harsh Pandey
Last updated on 16 Apr 2024
Assigning values in Python to variables is a fundamental and straightforward process. This action forms the basis for storing and manipulating Python data. The various methods for assigning values to variables in Python are given below.
Assign Values To Variables Direct Initialisation Method
Assigning values to variables in Python using the Direct Initialization Method involves a simple, single-line statement. This method is essential for efficiently setting up variables with initial values.
To use this method, you directly assign the desired value to a variable. The format follows variable_name = value . For instance, age = 21 assigns the integer 21 to the variable age.
This method is not just limited to integers. For example, assigning a string to a variable would be name = "Alice" . An example of this implementation in Python is.
Python Variables – Assign Multiple Values
In Python, assigning multiple values to variables can be done in a single, efficient line of code. This feature streamlines the initializing of several variables at once, making the code more concise and readable.
Python allows you to assign values to multiple variables simultaneously by separating each variable and value with commas. For example, x, y, z = 1, 2, 3 simultaneously assigns 1 to x, 2 to y, and 3 to z.
Additionally, Python supports unpacking a collection of values into variables. For example, if you have a list values = [1, 2, 3] , you can assign these values to a, b, c by writing a, b, c = values .
Python’s capability to assign multiple values to multiple variables in a single line enhances code efficiency and clarity. This feature is applicable across various data types and includes unpacking collections into multiple variables, as shown in the examples.
Assign Values To Variables Using Conditional Operator
Assigning values to variables in Python using a conditional operator allows for more dynamic and flexible value assignments based on certain conditions. This method employs the ternary operator, a concise way to assign values based on a condition's truth value.
The syntax for using the conditional operator in Python follows the pattern: variable = value_if_true if condition else value_if_false . For example, status = 'Adult' if age >= 18 else 'Minor' assigns 'Adult' to status if age is 18 or more, and 'Minor' otherwise.
This method can also be used with more complex conditions and various data types. For example, you can assign different strings to a variable based on a numerical comparison
Using the conditional operator for variable assignment in Python enables more nuanced and condition-dependent variable initialization. It is particularly useful for creating readable one-liners that eliminate the need for longer if-else statements, as illustrated in the examples.
Python One Liner Conditional Statement Assigning
Python allows for one-liner conditional statements to assign values to variables, providing a compact and efficient way of handling conditional assignments. This approach utilizes the ternary operator for conditional expressions in a single line of code.
The ternary operator syntax in Python is variable = value_if_true if condition else value_if_false . For instance, message = 'High' if temperature > 20 else 'Low' assigns 'High' to message if temperature is greater than 20, and 'Low' otherwise.
This method can also be applied to more complex conditions.
Using one-liner conditional statements in Python for variable assignment streamlines the process, especially when dealing with simple conditions. It replaces the need for multi-line if-else statements, making the code more concise and readable.
Work with top startups & companies. Get paid on time.
Try a top quality developer for 7 days. pay only if satisfied..
// Find jobs by category
You've got the vision, we help you create the best squad. Pick from our highly skilled lineup of the best independent engineers in the world.
- Ruby on Rails
- Elasticsearch
- Google Cloud
- React Native
- Contact Details
- 2093, Philadelphia Pike, DE 19703, Claymont
- [email protected]
- Explore jobs
- We are Hiring!
- Write for us
- 2093, Philadelphia Pike DE 19703, Claymont
Copyright @ 2024 Flexiple Inc
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java variables.
Variables are containers for storing data values.
In Java, there are different types of variables, for example:
- String - stores text, such as "Hello". String values are surrounded by double quotes
- int - stores integers (whole numbers), without decimals, such as 123 or -123
- float - stores floating point numbers, with decimals, such as 19.99 or -19.99
- char - stores single characters, such as 'a' or 'B'. Char values are surrounded by single quotes
- boolean - stores values with two states: true or false
Declaring (Creating) Variables
To create a variable, you must specify the type and assign it a value:
Where type is one of Java's types (such as int or String ), and variableName is the name of the variable (such as x or name ). The equal sign is used to assign values to the variable.
To create a variable that should store text, look at the following example:
Create a variable called name of type String and assign it the value " John ":
Try it Yourself »
To create a variable that should store a number, look at the following example:
Create a variable called myNum of type int and assign it the value 15 :
You can also declare a variable without assigning the value, and assign the value later:
Note that if you assign a new value to an existing variable, it will overwrite the previous value:
Change the value of myNum from 15 to 20 :
Final Variables
If you don't want others (or yourself) to overwrite existing values, use the final keyword (this will declare the variable as "final" or "constant", which means unchangeable and read-only):
Other Types
A demonstration of how to declare variables of other types:
You will learn more about data types in the next section.
Test Yourself With Exercises
Create a variable named carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

Home > Bash Scripting Tutorial > Bash Variables > Variable Declaration and Assignment > How to Assign Variable in Bash Script? [8 Practical Cases]
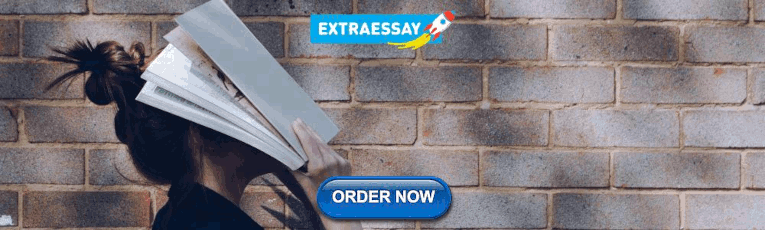
How to Assign Variable in Bash Script? [8 Practical Cases]

Variables allow you to store and manipulate data within your script, making it easier to organize and access information. In Bash scripts , variable assignment follows a straightforward syntax, but it offers a range of options and features that can enhance the flexibility and functionality of your scripts. In this article, I will discuss modes to assign variable in the Bash script . As the Bash script offers a range of methods for assigning variables, I will thoroughly delve into each one.
Key Takeaways
- Getting Familiar With Different Types Of Variables.
- Learning how to assign single or multiple bash variables.
- Understanding the arithmetic operation in Bash Scripting.
Free Downloads
Local vs global variable assignment.
In programming, variables are used to store and manipulate data. There are two main types of variable assignments: local and global .
A. Local Variable Assignment
In programming, a local variable assignment refers to the process of declaring and assigning a variable within a specific scope, such as a function or a block of code. Local variables are temporary and have limited visibility, meaning they can only be accessed within the scope in which they are defined.
Here are some key characteristics of local variable assignment:
- Local variables in bash are created within a function or a block of code.
- By default, variables declared within a function are local to that function.
- They are not accessible outside the function or block in which they are defined.
- Local variables typically store temporary or intermediate values within a specific context.
Here is an example in Bash script.
In this example, the variable x is a local variable within the scope of the my_function function. It can be accessed and used within the function, but accessing it outside the function will result in an error because the variable is not defined in the outer scope.
B. Global Variable Assignment
In Bash scripting, global variables are accessible throughout the entire script, regardless of the scope in which they are declared. Global variables can be accessed and modified from any script part, including within functions.
Here are some key characteristics of global variable assignment:
- Global variables in bash are declared outside of any function or block.
- They are accessible throughout the entire script.
- Any variable declared outside of a function or block is considered global by default.
- Global variables can be accessed and modified from any script part, including within functions.
Here is an example in Bash script given in the context of a global variable .
It’s important to note that in bash, variable assignment without the local keyword within a function will create a global variable even if there is a global variable with the same name. To ensure local scope within a function , using the local keyword explicitly is recommended.
Additionally, it’s worth mentioning that subprocesses spawned by a bash script, such as commands executed with $(…) or backticks , create their own separate environments, and variables assigned within those subprocesses are not accessible in the parent script .
8 Different Cases to Assign Variables in Bash Script
In Bash scripting , there are various cases or scenarios in which you may need to assign variables. Here are some common cases I have described below. These examples cover various scenarios, such as assigning single variables , multiple variable assignments in a single line , extracting values from command-line arguments , obtaining input from the user , utilizing environmental variables, etc . So let’s start.
Case 01: Single Variable Assignment
To assign a value to a single variable in Bash script , you can use the following syntax:
However, replace the variable with the name of the variable you want to assign, and the value with the desired value you want to assign to that variable.
To assign a single value to a variable in Bash , you can go in the following manner:
Steps to Follow >
❶ At first, launch an Ubuntu Terminal .
❷ Write the following command to open a file in Nano :
- nano : Opens a file in the Nano text editor.
- single_variable.sh : Name of the file.
❸ Copy the script mentioned below:
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Next, variable var_int contains an integer value of 23 and displays with the echo command .
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable :
- chmod : changes the permissions of files and directories.
- u+x : Here, u refers to the “ user ” or the owner of the file and +x specifies the permission being added, in this case, the “ execute ” permission. When u+x is added to the file permissions, it grants the user ( owner ) permission to execute ( run ) the file.
- single_variable.sh : File name to which the permissions are being applied.
❻ Run the script by using the following command:

Case 02: Multi-Variable Assignment in a Single Line of a Bash Script
Multi-variable assignment in a single line is a concise and efficient way of assigning values to multiple variables simultaneously in Bash scripts . This method helps reduce the number of lines of code and can enhance readability in certain scenarios. Here’s an example of a multi-variable assignment in a single line.
You can follow the steps of Case 01 , to save & make the script executable.
Script (multi_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Then, three variables x , y , and z are assigned values 1 , 2 , and 3 , respectively. The echo statements are used to print the values of each variable. Following that, two variables var1 and var2 are assigned values “ Hello ” and “ World “, respectively. The semicolon (;) separates the assignment statements within a single line. The echo statement prints the values of both variables with a space in between. Lastly, the read command is used to assign values to var3 and var4. The <<< syntax is known as a here-string , which allows the string “ Hello LinuxSimply ” to be passed as input to the read command . The input string is split into words, and the first word is assigned to var3 , while the remaining words are assigned to var4 . Finally, the echo statement displays the values of both variables.
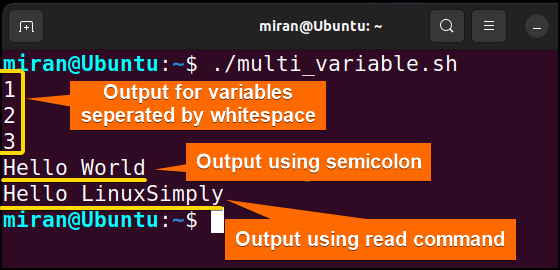
Case 03: Assigning Variables From Command-Line Arguments
In Bash , you can assign variables from command-line arguments using special variables known as positional parameters . Here is a sample code demonstrated below.
Script (var_as_argument.sh) >
The provided Bash script starts with the shebang ( #!/bin/bash ) to use Bash shell. The script assigns the first command-line argument to the variable name , the second argument to age , and the third argument to city . The positional parameters $1 , $2 , and $3 , which represent the values passed as command-line arguments when executing the script. Then, the script uses echo statements to display the values of the assigned variables.
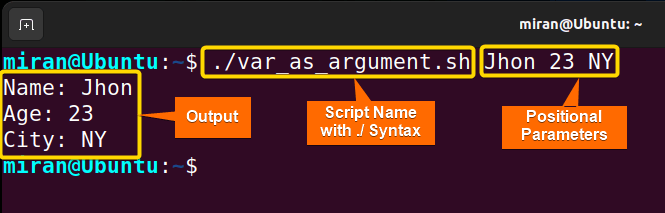
Case 04: Assign Value From Environmental Bash Variable
In Bash , you can also assign the value of an Environmental Variable to a variable. To accomplish the task you can use the following syntax :
However, make sure to replace ENV_VARIABLE_NAME with the actual name of the environment variable you want to assign. Here is a sample code that has been provided for your perusal.
Script (env_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The value of the USER environment variable, which represents the current username, is assigned to the Bash variable username. Then the output is displayed using the echo command.
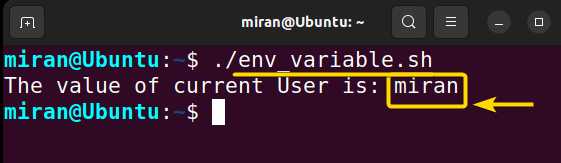
Case 05: Default Value Assignment
In Bash , you can assign default values to variables using the ${variable:-default} syntax . Note that this default value assignment does not change the original value of the variable; it only assigns a default value if the variable is empty or unset . Here’s a script to learn how it works.
Script (default_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The next line stores a null string to the variable . The ${ variable:-Softeko } expression checks if the variable is unset or empty. As the variable is empty, it assigns the default value ( Softeko in this case) to the variable . In the second portion of the code, the LinuxSimply string is stored as a variable. Then the assigned variable is printed using the echo command .
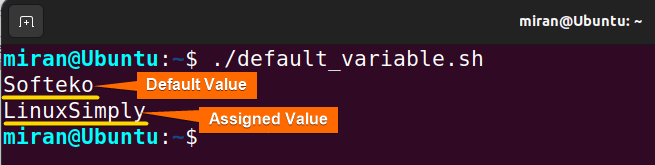
Case 06: Assigning Value by Taking Input From the User
In Bash , you can assign a value from the user by using the read command. Remember we have used this command in Case 2 . Apart from assigning value in a single line, the read command allows you to prompt the user for input and assign it to a variable. Here’s an example given below.
Script (user_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The read command is used to read the input from the user and assign it to the name variable . The user is prompted with the message “ Enter your name: “, and the value they enter is stored in the name variable. Finally, the script displays a message using the entered value.
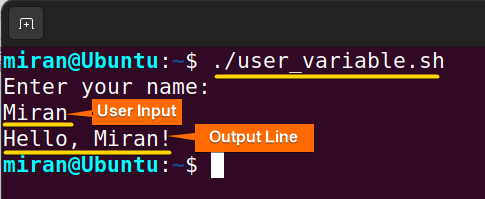
Case 07: Using the “let” Command for Variable Assignment
In Bash , the let command can be used for arithmetic operations and variable assignment. When using let for variable assignment, it allows you to perform arithmetic operations and assign the result to a variable .
Script (let_var_assign.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. then the let command performs arithmetic operations and assigns the results to variables num. Later, the echo command has been used to display the value stored in the num variable.
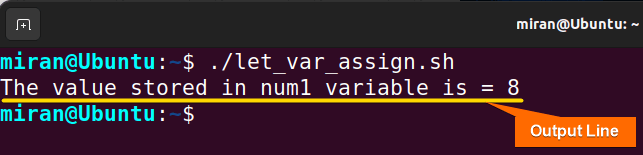
Case 08: Assigning Shell Command Output to a Variable
Lastly, you can assign the output of a shell command to a variable using command substitution . There are two common ways to achieve this: using backticks ( “) or using the $() syntax. Note that $() syntax is generally preferable over backticks as it provides better readability and nesting capability, and it avoids some issues with quoting. Here’s an example that I have provided using both cases.
Script (shell_command_var.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The output of the ls -l command (which lists the contents of the current directory in long format) allocates to the variable output1 using backticks . Similarly, the output of the date command (which displays the current date and time) is assigned to the variable output2 using the $() syntax . The echo command displays both output1 and output2 .
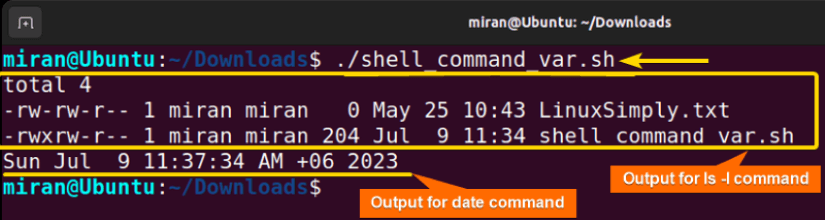
Assignment on Assigning Variables in Bash Scripts
Finally, I have provided two assignments based on today’s discussion. Don’t forget to check this out.
- Difference: ?
- Quotient: ?
- Remainder: ?
- Write a Bash script to find and display the name of the largest file using variables in a specified directory.
In conclusion, assigning variable Bash is a crucial aspect of scripting, allowing developers to store and manipulate data efficiently. This article explored several cases to assign variables in Bash, including single-variable assignments , multi-variable assignments in a single line , assigning values from environmental variables, and so on. Each case has its advantages and limitations, and the choice depends on the specific needs of the script or program. However, if you have any questions regarding this article, feel free to comment below. I will get back to you soon. Thank You!
People Also Ask
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- Bash Variable Naming Conventions in Shell Script [6 Rules]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Default Value in Bash Scripts? [2 Methods]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Variable Declaration and Assignment | Bash Variables | Bash Scripting Tutorial
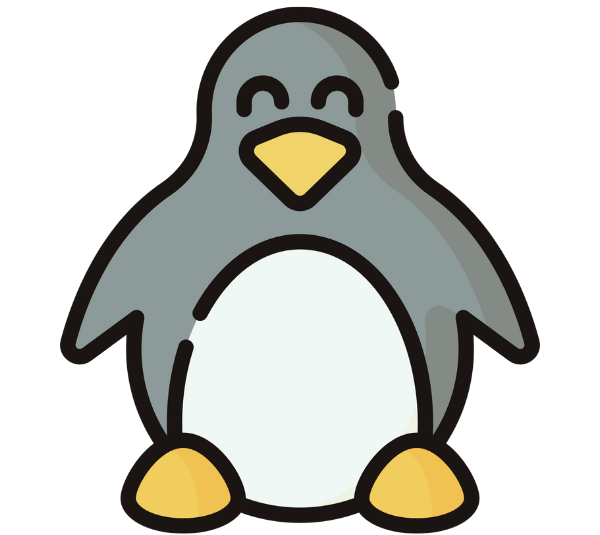
Mohammad Shah Miran
Hey, I'm Mohammad Shah Miran, previously worked as a VBA and Excel Content Developer at SOFTEKO, and for now working as a Linux Content Developer Executive in LinuxSimply Project. I completed my graduation from Bangladesh University of Engineering and Technology (BUET). As a part of my job, i communicate with Linux operating system, without letting the GUI to intervene and try to pass it to our audience.
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Variables (Transact-SQL)
- 14 contributors
A Transact-SQL local variable is an object that can hold a single data value of a specific type. Variables in batches and scripts are typically used:
- As a counter either to count the number of times a loop is performed or to control how many times the loop is performed.
- To hold a data value to be tested by a control-of-flow statement.
- To save a data value to be returned by a stored procedure return code or function return value.
- The names of some Transact-SQL system functions begin with two at signs (@@). Although in earlier versions of SQL Server, the @@functions are referred to as global variables, @@functions aren't variables, and they don't have the same behaviors as variables. The @@functions are system functions, and their syntax usage follows the rules for functions.
- You can't use variables in a view.
- Changes to variables aren't affected by the rollback of a transaction.
The following script creates a small test table and populates it with 26 rows. The script uses a variable to do three things:
- Control how many rows are inserted by controlling how many times the loop is executed.
- Supply the value inserted into the integer column.
- Function as part of the expression that generates letters to be inserted into the character column.
Declaring a Transact-SQL Variable
The DECLARE statement initializes a Transact-SQL variable by:
- Assigning a name. The name must have a single @ as the first character.
- Assigning a system-supplied or user-defined data type and a length. For numeric variables, a precision and scale are also assigned. For variables of type XML, an optional schema collection may be assigned.
- Setting the value to NULL.
For example, the following DECLARE statement creates a local variable named @mycounter with an int data type.
To declare more than one local variable, use a comma after the first local variable defined, and then specify the next local variable name and data type.
For example, the following DECLARE statement creates three local variables named @LastName , @FirstName and @StateProvince , and initializes each to NULL:
The scope of a variable is the range of Transact-SQL statements that can reference the variable. The scope of a variable lasts from the point it is declared until the end of the batch or stored procedure in which it is declared. For example, the following script generates a syntax error because the variable is declared in one batch and referenced in another:
Variables have local scope and are only visible within the batch or procedure where they are defined. In the following example, the nested scope created for execution of sp_executesql does not have access to the variable declared in the higher scope and returns and error.
Setting a Value in a Transact-SQL Variable
When a variable is first declared, its value is set to NULL. To assign a value to a variable, use the SET statement. This is the preferred method of assigning a value to a variable. A variable can also have a value assigned by being referenced in the select list of a SELECT statement.
To assign a variable a value by using the SET statement, include the variable name and the value to assign to the variable. This is the preferred method of assigning a value to a variable. The following batch, for example, declares two variables, assigns values to them, and then uses them in the WHERE clause of a SELECT statement:
A variable can also have a value assigned by being referenced in a select list. If a variable is referenced in a select list, it should be assigned a scalar value or the SELECT statement should only return one row. For example:
If there are multiple assignment clauses in a single SELECT statement, SQL Server does not guarantee the order of evaluation of the expressions. Note that effects are only visible if there are references among the assignments.
If a SELECT statement returns more than one row and the variable references a non-scalar expression, the variable is set to the value returned for the expression in the last row of the result set. For example, in the following batch @EmpIDVariable is set to the BusinessEntityID value of the last row returned, which is 1:
Declare @local_variable SET @local_variable SELECT @local_variable Expressions (Transact-SQL) Compound Operators (Transact-SQL)
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Destructuring assignment
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax but uses it on the left-hand side of the assignment instead. It defines which values to unpack from the sourced variable.
Similarly, you can destructure objects on the left-hand side of the assignment.
This capability is similar to features present in languages such as Perl and Python.
For features specific to array or object destructuring, refer to the individual examples below.
Binding and assignment
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern , with slightly different syntaxes.
In binding patterns, the pattern starts with a declaration keyword ( var , let , or const ). Then, each individual property must either be bound to a variable or further destructured.
All variables share the same declaration, so if you want some variables to be re-assignable but others to be read-only, you may have to destructure twice — once with let , once with const .
In many other syntaxes where the language binds a variable for you, you can use a binding destructuring pattern. These include:
- The looping variable of for...in for...of , and for await...of loops;
- Function parameters;
- The catch binding variable.
In assignment patterns, the pattern does not start with a keyword. Each destructured property is assigned to a target of assignment — which may either be declared beforehand with var or let , or is a property of another object — in general, anything that can appear on the left-hand side of an assignment expression.
Note: The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{ a, b } = { a: 1, b: 2 } is not valid stand-alone syntax, as the { a, b } on the left-hand side is considered a block and not an object literal according to the rules of expression statements . However, ({ a, b } = { a: 1, b: 2 }) is valid, as is const { a, b } = { a: 1, b: 2 } .
If your coding style does not include trailing semicolons, the ( ... ) expression needs to be preceded by a semicolon, or it may be used to execute a function on the previous line.
Note that the equivalent binding pattern of the code above is not valid syntax:
You can only use assignment patterns as the left-hand side of the assignment operator. You cannot use them with compound assignment operators such as += or *= .
Default value
Each destructured property can have a default value . The default value is used when the property is not present, or has value undefined . It is not used if the property has value null .
The default value can be any expression. It will only be evaluated when necessary.
Rest property
You can end a destructuring pattern with a rest property ...rest . This pattern will store all remaining properties of the object or array into a new object or array.
The rest property must be the last in the pattern, and must not have a trailing comma.
Array destructuring
Basic variable assignment, destructuring with more elements than the source.
In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N , only the first N variables are assigned values. The values of the remaining variables will be undefined.
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Using a binding pattern as the rest property
The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after collecting the rest elements, so you cannot access any properties present on the original iterable in this way.
These binding patterns can even be nested, as long as each rest property is the last in the list.
On the other hand, object destructuring can only have an identifier as the rest property.
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Using array destructuring on any iterable
Array destructuring calls the iterable protocol of the right-hand side. Therefore, any iterable, not necessarily arrays, can be destructured.
Non-iterables cannot be destructured as arrays.
Iterables are only iterated until all bindings are assigned.
The rest binding is eagerly evaluated and creates a new array, instead of using the old iterable.
Object destructuring
Basic assignment, assigning to new variable names.
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo .
Assigning to new variable names and providing default values
A property can be both
- Unpacked from an object and assigned to a variable with a different name.
- Assigned a default value in case the unpacked value is undefined .
Unpacking properties from objects passed as a function parameter
Objects passed into function parameters can also be unpacked into variables, which may then be accessed within the function body. As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property.
Consider this object, which contains information about a user.
Here we show how to unpack a property of the passed object into a variable with the same name. The parameter value { id } indicates that the id property of the object passed to the function should be unpacked into a variable with the same name, which can then be used within the function.
You can define the name of the unpacked variable. Here we unpack the property named displayName , and rename it to dname for use within the function body.
Nested objects can also be unpacked. The example below shows the property fullname.firstName being unpacked into a variable called name .
Setting a function parameter's default value
Default values can be specified using = , and will be used as variable values if a specified property does not exist in the passed object.
Below we show a function where the default size is 'big' , default co-ordinates are x: 0, y: 0 and default radius is 25.
In the function signature for drawChart above, the destructured left-hand side has a default value of an empty object = {} .
You could have also written the function without that default. However, if you leave out that default value, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can call drawChart() without supplying any parameters. Otherwise, you need to at least supply an empty object literal.
For more information, see Default parameters > Destructured parameter with default value assignment .
Nested object and array destructuring
For of iteration and destructuring, computed object property names and destructuring.
Computed property names, like on object literals , can be used with destructuring.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifier that is valid.
Destructuring primitive values
Object destructuring is almost equivalent to property accessing . This means if you try to destruct a primitive value, the value will get wrapped into the corresponding wrapper object and the property is accessed on the wrapper object.
Same as accessing properties, destructuring null or undefined throws a TypeError .
This happens even when the pattern is empty.
Combined array and object destructuring
Array and object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
The prototype chain is looked up when the object is deconstructed
When deconstructing an object, if a property is not accessed in itself, it will continue to look up along the prototype chain.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators
- ES6 in Depth: Destructuring on hacks.mozilla.org (2015)
SQL FROM Query Examples and Syntax
By: Joe Gavin | Updated: 2024-04-16 | Comments | Related: > TSQL
You may know the SQL FROM clause is part of every SQL query reading data from a table, view, or function, and as a beginner you would like to learn more about it.
We'll look at several examples of using the FROM clause in the following sections of this SQL tutorial:
Create Table
Alter table, joins across multiple tables, delete from.
The following examples were run in SQL Server Management Studio (SSMS) 19.2 against a Microsoft SQL Server 2022 relational database. The tables and data are a heavily slimmed-down subset of the Wide World Importers Sample Databases for Microsoft SQL Server .
We'll need a couple of populated tables for the examples, so let's use the MyDatabase database.
First, let's create a database table named Customers with two fields, CustomerId of type int and CustomerName of type nvarchar(100). Here is the syntax:
Next, populate the Customers table with some sample data via an INSERT statement with the T-SQL programming language.
Next, create a table called OrderIds with two fields, CustomerId of type int and OrderId of type int.
Populate the OrderIds table with some sample data.
Additional Resources:
- SQL SELECT INTO Examples
- INSERT INTO new SQL table with SELECT INTO
- Creating a Table Using the SQL SELECT INTO Clause - Part 1
We'll add a column called Active of type bit to Customers that will store a 0 if a customer is inactive or a 1 if they're active.
Make them all active except for where the customer is CustomerId = 10.
Now, let's create a view. Views are virtual tables based on the result set of a SELECT query. It can be thought of as a stored SQL query that's treated as a table. The code below will create a view called VCustomersActive over the Customers table that only shows active customers.
SQL SELECT statement with all columns from the view.
The screenshot below shows that only the active customers are returned.
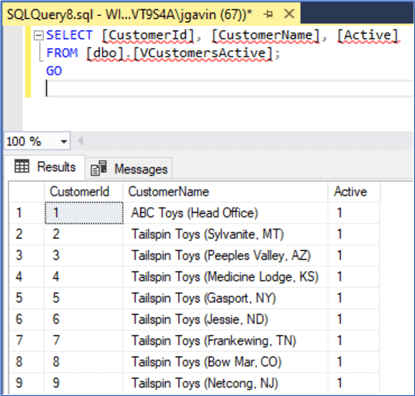
- SQL Server SELECT Examples
- Why You Should Avoid SELECT * in SQL Server T-SQL Code
An alias is an alternative name for something. In SQL, an alias is a temporary name assigned to a column or table. The query below assigns the alias CustName to the CustomerName field in the Customers table.
The following query assigns the alias 'c' to the Customers table. Aliasing a table name here doesn't do much for us, but it becomes apparent that this is useful in a JOIN.
- Aliasing Tables in a SELECT Statement Example
Aliases are very useful when joining tables to combine results from more than one table. Here, we're joining the Customers and OrderIds tables to get the CustomerName and OrderId for any customer who's placed an order. It's perfectly legitimate to write the SELECT in the form TableName.Fieldname for each field we want. But this falls under the 'just because you can doesn't mean you should' category. It's just not that easy to read.
However, by aliasing the table name, we make the SQL simpler, more readable, and less ambiguous. This query replaces the table names Customers with the alias c and OrderIds with the alias oid . This query is easier to read.
- SQL Server Join Example
- Join Tables in a SELECT Statement Example
- Getting Started with SQL INNER JOIN
- SQL RIGHT JOIN Examples
- SQL LEFT JOIN Examples
- Learn SQL FULL OUTER JOIN with Examples
As the name implies, a subquery is a query within a query that returns a result set. A subquery is one way to get a list of customers who have placed an order. This query will return a list of DISTINCT CustomerIDs in the OrderIds table, meaning they have placed an order.
And we get the values 1, 2, 3, 4, 5, 6, and 8.
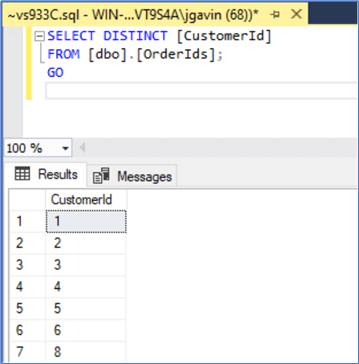
This query creates the result set in a subquery and passes the result set to the WHERE clause.
This SELECT clause is the equivalent of this:
- SQL Server Subquery Example
- SQL Server Derived Table Example
Deleting data from a table is in a SQL database one time where the FROM clause is optional. Both queries delete inactive customers from the Customers table, but including the FROM makes it more intuitive.
- The T-SQL DELETE statement
- Drop Table SQL Server Examples with T-SQL and SQL Server Management Studio
We've seen some simple examples of the SQL FROM clause. Here are some tips with more information:
- SQL Server Window Aggregate Functions SUM, MIN, MAX and AVG
- Group By in SQL Server with CUBE, ROLLUP and GROUPING SETS Examples
- SQL Server String Functions
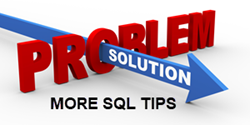
About the author
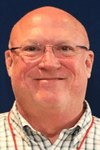
Comments For This Article
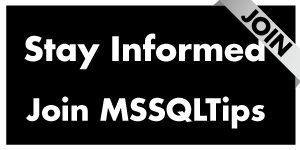
Related Content
SQL Server Cursor Example
SQL CASE Statement in Where Clause to Filter Based on a Condition or Expression
Rolling up multiple rows into a single row and column for SQL Server data
SQL NOT IN Operator
Using MERGE in SQL Server to insert, update and delete at the same time
SQL Server Loop through Table Rows without Cursor
CREATE OR ALTER statement in SQL Server
Related Categories
Change Data Capture
Common Table Expressions
Dynamic SQL
Error Handling
Stored Procedures
Transactions
Development
Date Functions
System Functions
JOIN Tables
SQL Server Management Studio
Database Administration
Performance
Performance Tuning
Locking and Blocking
Data Analytics \ ETL
Microsoft Fabric
Azure Data Factory
Integration Services
Popular Articles
SQL Date Format Options with SQL CONVERT Function
SQL Date Format examples using SQL FORMAT Function
SQL Server CROSS APPLY and OUTER APPLY
DROP TABLE IF EXISTS Examples for SQL Server
SQL Convert Date to YYYYMMDD
Resolving could not open a connection to SQL Server errors
Format numbers in SQL Server
SQL Server PIVOT and UNPIVOT Examples
Script to retrieve SQL Server database backup history and no backups
How to install SQL Server 2022 step by step
An Introduction to SQL Triggers
List SQL Server Login and User Permissions with fn_my_permissions
How to monitor backup and restore progress in SQL Server
SQL Server Database Stuck in Restoring State
Get started with the latest updates for Dockerfile syntax (v1.7.0)
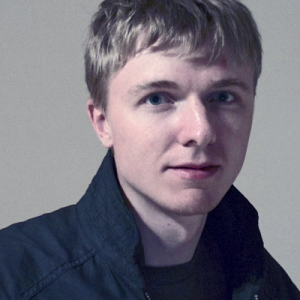
Tonis Tiigi
Dockerfiles are fundamental tools for developers working with Docker, serving as a blueprint for creating Docker images. These text documents contain all the commands a user could call on the command line to assemble an image. Understanding and effectively utilizing Dockerfiles can significantly streamline the development process, allowing for the automation of image creation and ensuring consistent environments across different stages of development. Dockerfiles are pivotal in defining project environments, dependencies, and the configuration of applications within Docker containers.
With new versions of the BuildKit builder toolkit , Docker Buildx CLI , and Dockerfile frontend for BuildKit (v1.7.0) , developers now have access to enhanced Dockerfile capabilities. This blog post delves into these new Dockerfile capabilities and explains how you can can leverage them in your projects to further optimize your Docker workflows.
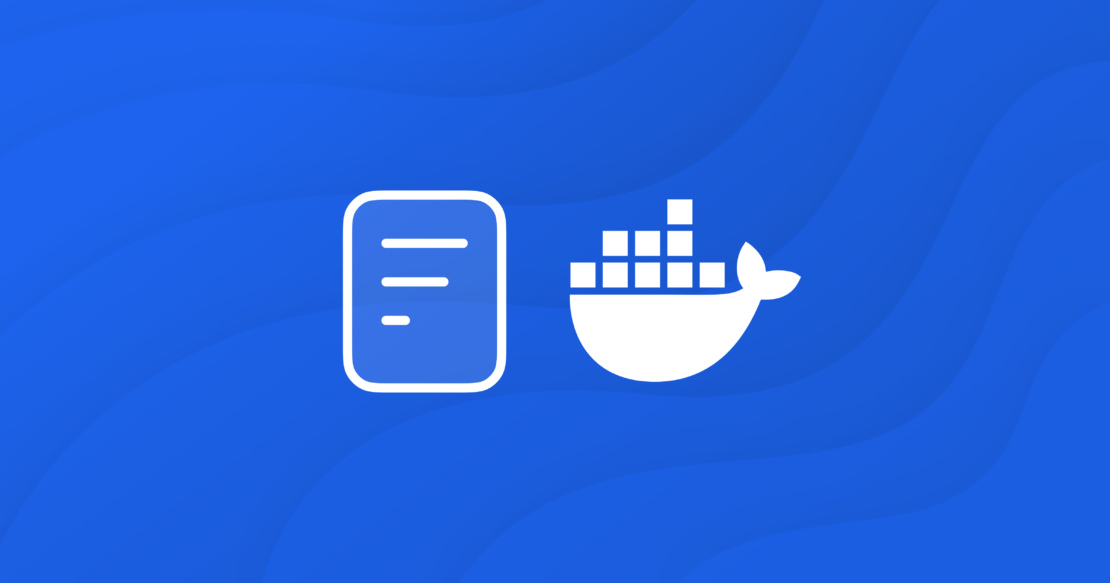
Before we get started, here’s a quick reminder of how Dockerfile is versioned and what you should do to update it.
Although most projects use Dockerfiles to build images, BuildKit is not limited only to that format. BuildKit supports multiple different frontends for defining the build steps for BuildKit to process. Anyone can create these frontends, package them as regular container images, and load them from a registry when you invoke the build.
With the new release, we have published two such images to Docker Hub : docker/dockerfile:1.7.0 and docker/dockerfile:1.7.0-labs .
To use these frontends, you need to specify a #syntax directive at the beginning of the file to tell BuildKit which frontend image to use for the build. Here we have set it to use the latest of the 1.x.x major version. For example:
This means that BuildKit is decoupled from the Dockerfile frontend syntax. You can start using new Dockerfile features right away without worrying about which BuildKit version you’re using. All the examples described in this article will work with any version of Docker that supports BuildKit ( the default builder as of Docker 23 ), as long as you define the correct #syntax directive on the top of your Dockerfile.
You can learn more about Dockerfile frontend versions in the documentation.
Variable expansions
When you write Dockerfiles, build steps can contain variables that are defined using the build arguments ( ARG ) and environment variables ( ENV ) instructions. The difference between build arguments and environment variables is that environment variables are kept in the resulting image and persist when a container is created from it.
When you use such variables, you most likely use ${NAME} or, more simply, $NAME in COPY , RUN , and other commands.
You might not know that Dockerfile supports two forms of Bash-like variable expansion:
- ${variable:-word}: Sets a value to word if the variable is unset
- ${variable:+word}: Sets a value to word if the variable is set
Up to this point, these special forms were not that useful in Dockerfiles because the default value of ARG instructions can be set directly:
If you are an expert in various shell applications, you know that Bash and other tools usually have many additional forms of variable expansion to ease the development of your scripts.
In Dockerfile v1.7, we have added:
- ${variable#pattern} and ${variable##pattern} to remove the shortest or longest prefix from the variable’s value.
- ${variable%pattern} and ${variable%%pattern} to remove the shortest or longest suffix from the variable’s value.
- ${variable/pattern/replacement} to first replace occurrence of a pattern
- ${variable//pattern/replacement} to replace all occurrences of a pattern
How these rules are used might not be completely obvious at first. So, let’s look at a few examples seen in actual Dockerfiles.
For example, projects often can’t agree on whether versions for downloading your dependencies should have a “ v ” prefix or not. The following allows you to get the format you need:
In the next example, multiple variants are used by the same project:
To configure different command behaviors for multi-platform builds, BuildKit provides useful built-in variables like TARGETOS and TARGETARCH . Unfortunately, not all projects use the same values. For example, in containers and the Go ecosystem, we refer to 64-bit ARM architecture as arm64 , but sometimes you need aarch64 instead.
In this case, the URL also uses a custom name for AMD64 architecture. To pass a variable through multiple expansions, use another ARG definition with an expansion from the previous value. You could also write all the definitions on a single line, as ARG allows multiple parameters, which may hurt readability.
Note that the example above is written in a way that if a user passes their own --build-arg ARCH=value , then that value is used as-is.
Now, let’s look at how new expansions can be useful in multi-stage builds.
One of the techniques described in “Advanced multi-stage build patterns” shows how build arguments can be used so that different Dockerfile commands run depending on the build-arg value. For example, you can use that pattern if you build a multi-platform image and want to run additional COPY or RUN commands only for specific platforms. If this method is new to you, you can learn more about it from that post .
In summarized form, the idea is to define a global build argument and then define build stages that use the build argument value in the stage name while pointing to the base of your target stage via the build-arg name.
Old example:
When using this pattern for multi-platform builds, one of the limitations is that all the possible values for the build-arg need to be defined by your Dockerfile. This is problematic as we want Dockerfile to be built in a way that it can build on any platform and not limit it to a specific set.
You can see other examples here and here of Dockerfiles where dummy stage aliases must be defined for all architectures, and no other architecture can be built. Instead, the pattern we would like to use is that there is one architecture that has a special behavior, and everything else shares another common behavior.
With new expansions, we can write this to demonstrate running special commands only on RISC-V, which is still somewhat new and may need custom behavior:
Let’s look at these ARCH definitions more closely.
- The first sets ARCH to TARGETARCH but removes riscv64 from the value.
- Next, as we described previously, we don’t actually want the other architectures to use their own values but instead want them all to share a common value. So, we set ARCH to common except if it was cleared from the previous riscv64 rule.
- Now, if we still have an empty value, we default it back to $TARGETARCH .
- The last definition is optional, as we would already have a unique value for both cases, but it makes the final stage name base-riscv64 nicer to read.
Additional examples of including multiple conditions with shared conditions, or conditions based on architecture variants can be found in this GitHub Gist page.
Comparing this example to the initial example of conditions between stages, the new pattern isn’t limited to just controlling the platform differences of your builds but can be used with any build-arg. If you have used this pattern before, then you can effectively now define an “else” clause, whereas previously, you were limited to only “if” clauses.
Copy with keeping parent directories
The following feature has been released in the “labs” channel. Define the following at the top of your Dockerfile to use this feature.
When you are copying files in your Dockerfile, for example, do this:
This example means the source file is copied directly to the destination directory. If your source path was a directory, all the files inside that directory would be copied directly to the destination path.
What if you have a file structure like the following:
You want to copy only files in app1/src , but so that the final files at the destination would be /to/dest/dir/app1/src/server.go and not just /to/dest/dir/server.go .
With the new COPY --parents flag, you can write:
This will copy the files inside the src directory and recreate the app1/src directory structure for these files.
Things get more powerful when you start to use wildcard paths. To copy the src directories for both apps into their respective locations, you can write:
This will create both /to/dest/dir/app1 and /to/dest/dir/app2 , but it will not copy the docs directory. Previously, this kind of copy was not possible with a single command. You would have needed multiple copies for individual files (as shown in this example ) or used some workaround with the RUN --mount instruction instead.
You can also use double-star wildcard ( ** ) to match files under any directory structure. For example, to copy only the Go source code files anywhere in your build context, you can write:
If you are thinking about why you would need to copy specific files instead of just using COPY ./ to copy all files, remember that your build cache gets invalidated when you include new files in your build. If you copy all files, the cache gets invalidated when any file is added or changed, whereas if you copy only Go files, only changes in these files influence the cache.
The new --parents flag is not only for COPY instructions from your build context, but obviously, you can also use them in multi-stage builds when copying files between stages using COPY --from .
Note that with COPY --from syntax, all source paths are expected to be absolute, meaning that if the --parents flag is used with such paths, they will be fully replicated as they were in the source stage. That may not always be desirable, and instead, you may want to keep some parents but discard and replace others. In that case, you can use a special /./ relative pivot point in your source path to mark which parents you wish to copy and which should be ignored. This special path component resembles how rsync works with the --relative flag.
This example above shows how only bin directories are copied from the collection of files that the intermediate stage generated, but all the directories will keep their paths relative to the out directory.
Exclusion filters
The following feature has been released in the “labs” channel. Define the following at the top of your Dockerfile to use this feature:
Another related case when moving files in your Dockerfile with COPY and ADD instructions is when you want to move a group of files but exclude a specific subset. Previously, your only options were to use RUN --mount or try to define your excluded files inside a .dockerignore file.
.dockerignore files, however, are not a good solution for this problem, because they only list the files excluded from the client-side build context and not from builds from remote Git/HTTP URLs and are limited to one per Dockerfile . You should use them similarly to .gitignore to mark files that are never part of your project but not as a way to define your application-specific build logic.
With the new --exclude=[pattern] flag, you can now define such exclusion filters for your COPY and ADD commands directly in the Dockerfile. The pattern uses the same format as .dockerignore .
The following example copies all the files in a directory except Markdown files:
You can use the flag multiple times to add multiple filters. The next example excludes Markdown files and also a file called README :
Double-star wildcards exclude not only Markdown files in the copied directory but also in any subdirectory:
As in .dockerignore files, you can also define exceptions to the exclusions with ! prefix. The following example excludes all Markdown files in any copied directory, except if the file is called important.md — in that case, it is still copied.
This double negative may be confusing initially, but note that this is a reversal of the previous exclude rule, and “include patterns” are defined by the source parameter of the COPY instruction.
When using --exclude together with previously described --parents copy mode, note that the exclude patterns are relative to the copied parent directories or to the pivot point /./ if one is defined. See the following directory structure for example:
This command would create the directory structure below. Note that only directories with the icons prefix were copied, the root parent directory assets was skipped as it was before the relative pivot point, and additionally, testapp was not copied as it was defined with an exclusion filter.
We hope this post gave you ideas for improving your Dockerfiles and that the patterns shown here will help you describe your build more efficiently. Remember that your Dockerfile can start using all these features today by defining the #syntax line on top, even if you haven’t updated to the latest Docker yet.
For a full list of other features in the new BuildKit, Buildx, and Dockerfile releases, check out the changelogs:
- BuildKit v0.13.0
- Buildx v0.13.0
- Dockerfile v1.7.0 v1.7.0-labs
Thanks to community members @tstenner , @DYefimov , and @leandrosansilva for helping to implement these features!
If you have issues or suggestions you want to share, let us know in the issue tracker.
- Subscribe to the Docker Newsletter .
- Get the latest release of Docker Desktop .
- Vote on what’s next! Check out our public roadmap .
- Have questions? The Docker community is here to help .
- New to Docker? Get started .
0 thoughts on "Get started with the latest updates for Dockerfile syntax (v1.7.0)"
AI Trends Report 2024: AI's Growing Role in Software Development
Next-level error handling: how docker desktop 4.29 aims to simplify developer challenges, docker desktop 4.29: docker socket mount permissions in eci, advanced error management, moby 26, and new beta features .
Apr 9, 2024
- Engineering
Firefox is no longer supported on Windows 8.1 and below.
Please download Firefox ESR (Extended Support Release) to use Firefox.
Download Firefox ESR 64-bit
Download Firefox ESR 32-bit
Firefox is no longer supported on macOS 10.14 and below.
Mozilla Foundation Security Advisory 2024-18
Security vulnerabilities fixed in firefox 125.
- Firefox 125
# CVE-2024-3852: GetBoundName in the JIT returned the wrong object
Description.
GetBoundName could return the wrong version of an object when JIT optimizations were applied.
- Bug 1883542
# CVE-2024-3853: Use-after-free if garbage collection runs during realm initialization
A use-after-free could result if a JavaScript realm was in the process of being initialized when a garbage collection started.
- Bug 1884427
# CVE-2024-3854: Out-of-bounds-read after mis-optimized switch statement
In some code patterns the JIT incorrectly optimized switch statements and generated code with out-of-bounds-reads.
- Bug 1884552
# CVE-2024-3855: Incorrect JIT optimization of MSubstr leads to out-of-bounds reads
In certain cases the JIT incorrectly optimized MSubstr operations, which led to out-of-bounds reads.
- Bug 1885828
# CVE-2024-3856: Use-after-free in WASM garbage collection
A use-after-free could occur during WASM execution if garbage collection ran during the creation of an array.
- Bug 1885829
# CVE-2024-3857: Incorrect JITting of arguments led to use-after-free during garbage collection
The JIT created incorrect code for arguments in certain cases. This led to potential use-after-free crashes during garbage collection.
- Bug 1886683
# CVE-2024-3858: Corrupt pointer dereference in js::CheckTracedThing<js::Shape>
It was possible to mutate a JavaScript object so that the JIT could crash while tracing it.
- Bug 1888892
# CVE-2024-3859: Integer-overflow led to out-of-bounds-read in the OpenType sanitizer
On 32-bit versions there were integer-overflows that led to an out-of-bounds-read that potentially could be triggered by a malformed OpenType font.
- Bug 1874489
# CVE-2024-3860: Crash when tracing empty shape lists
An out-of-memory condition during object initialization could result in an empty shape list. If the JIT subsequently traced the object it would crash.
- Bug 1881417
# CVE-2024-3861: Potential use-after-free due to AlignedBuffer self-move
If an AlignedBuffer were assigned to itself, the subsequent self-move could result in an incorrect reference count and later use-after-free.
- Bug 1883158
# CVE-2024-3862: Potential use of uninitialized memory in MarkStack assignment operator on self-assignment
The MarkStack assignment operator, part of the JavaScript engine, could access uninitialized memory if it were used in a self-assignment.
- Bug 1884457
# CVE-2024-3863: Download Protections were bypassed by .xrm-ms files on Windows
The executable file warning was not presented when downloading .xrm-ms files. Note: This issue only affected Windows operating systems. Other operating systems are unaffected.
- Bug 1885855
# CVE-2024-3302: Denial of Service using HTTP/2 CONTINUATION frames
There was no limit to the number of HTTP/2 CONTINUATION frames that would be processed. A server could abuse this to create an Out of Memory condition in the browser.
- Bug 1881183
- VU#421644 - HTTP/2 CONTINUATION frames can be utilized for DoS attacks
# CVE-2024-3864: Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
Memory safety bug present in Firefox 124, Firefox ESR 115.9, and Thunderbird 115.9. This bug showed evidence of memory corruption and we presume that with enough effort this could have been exploited to run arbitrary code.
- Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
# CVE-2024-3865: Memory safety bugs fixed in Firefox 125
Memory safety bugs present in Firefox 124. Some of these bugs showed evidence of memory corruption and we presume that with enough effort some of these could have been exploited to run arbitrary code.
- Memory safety bugs fixed in Firefox 125
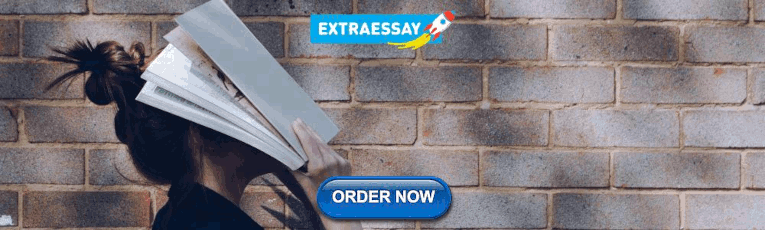
IMAGES
VIDEO
COMMENTS
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
int x; // define an integer variable named x int y, z; // define two integer variables, named y and z. Variable assignment. After a variable has been defined, you can give it a value (in a separate statement) using the = operator. This process is called assignment, and the = operator is called the assignment operator.
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You" Assigning a value is known as binding in Python. In the example above, we have assigned the value of 2 to mySecondVariable. Note how I assigned an integer value of 1 and then a string ...
Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. Assignment expressions allow variable assignments to occur inside of larger expressions.
The example in the previous code contains three assignments. The first one assigns the value of the expression 40 + 2 to a new variable called magicnumber; the second one assigns the value of π to the variable pi, and; the last assignment assigns the string value 'Data is eatig the world' to the variable message.
Python Variables and Assignment Python Variables. A Python variable is a named bit of computer memory, keeping track of a value as the code runs. ... The string 'hello' in the example above is shown in gray. It is not needed by the code after the third line runs — no variable points to it any longer, so it cannot be used. Memory like this ...
Assign this list to the variable x; x is now a reference to that list. Using our referencing variable, x, update element-0 of the list to store the integer -4. This does not create a new list object, rather it mutates our original list. This is why printing x in the console displays [-4, 2, 3] and not [1, 2, 3]. A tuple is an example of an ...
Since Python 3.8, code can use the so-called "walrus" operator (:=), documented in PEP 572, for assignment expressions.This seems like a really substantial new feature, since it allows this form of assignment within comprehensions and lambdas.. What exactly are the syntax, semantics, and grammar specifications of assignment expressions?
Creating Variables. Python has no command for declaring a variable. A variable is created the moment you first assign a value to it. Example. x = 5 y = "John" print(x) print(y) ... Example. This will create two variables: a = 4 A = "Sally" #A will not overwrite a.
The assignment operator = is used to associate a variable name with a given value. For example, type the command: a=3.45. in the command line window. This command assigns the value 3.45 to the variable named a. Next, type the command: a. in the command window and hit the enter key. You should see the value contained in the variable a echoed to ...
An Example of a Variable in Python is a representational name that serves as a pointer to an object. Once an object is assigned to a variable, it can be referred to by that name. ... Variables Assignment in Python. Here, we will define a variable in python. Here, clearly we have assigned a number, a floating point number, and a string to a ...
Assigning values to variables in Python using the Direct Initialization Method involves a simple, single-line statement. This method is essential for efficiently setting up variables with initial values. To use this method, you directly assign the desired value to a variable. The format follows variable_name = value.
Java Variables. Variables are containers for storing data values. In Java, there are different types of variables, for example: String - stores text, such as "Hello". String values are surrounded by double quotes. int - stores integers (whole numbers), without decimals, such as 123 or -123. float - stores floating point numbers, with decimals ...
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
Variables allow you to store and manipulate data within your script, making it easier to organize and access information.In Bash scripts, variable assignment follows a straightforward syntax, but it offers a range of options and features that can enhance the flexibility and functionality of your scripts.In this article, I will discuss modes to assign variable in the Bash script.
The example you've given is making use of this property of JavaScript to perform an assignment. It can be used anywhere where you need to get the first truthy or falsy value among a set of values. This code below will assign the value "Hello" to b as it makes it easier to assign a default value, instead of doing if-else checks.
To assign a variable a value by using the SET statement, include the variable name and the value to assign to the variable. This is the preferred method of assigning a value to a variable. The following batch, for example, declares two variables, assigns values to them, and then uses them in the WHERE clause of a SELECT statement:
If you're interested in having a "switch" expression, you can find the little fluent class that I wrote here in order to have code like this: a = Switch.On(b) .Case(c).Then(d) .Case(e).Then(f) .Default(g); You could go one step further and generalize this even more with function parameters to the Then methods.
As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property. Consider this object, which contains information about a user.
SQL Server Cursor Example. SQL CASE Statement in Where Clause to Filter Based on a Condition or Expression. Rolling up multiple rows into a single row and column for SQL Server data. SQL Convert Date to YYYYMMDD. SQL NOT IN Operator. Resolving could not open a connection to SQL Server errors. Format numbers in SQL Server. SQL Server PIVOT and ...
Variable expansions. When you write Dockerfiles, build steps can contain variables that are defined using the build arguments (ARG) and environment variables (ENV) instructions. The difference between build arguments and environment variables is that environment variables are kept in the resulting image and persist when a container is created ...
# CVE-2024-3862: Potential use of uninitialized memory in MarkStack assignment operator on self-assignment Reporter Ronald Crane Impact moderate Description. The MarkStack assignment operator, part of the JavaScript engine, could access uninitialized memory if it were used in a self-assignment. References. Bug 1884457