C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
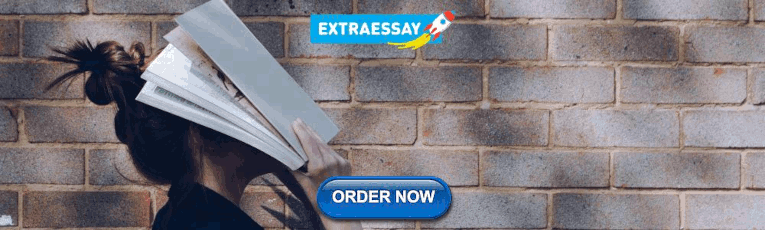
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
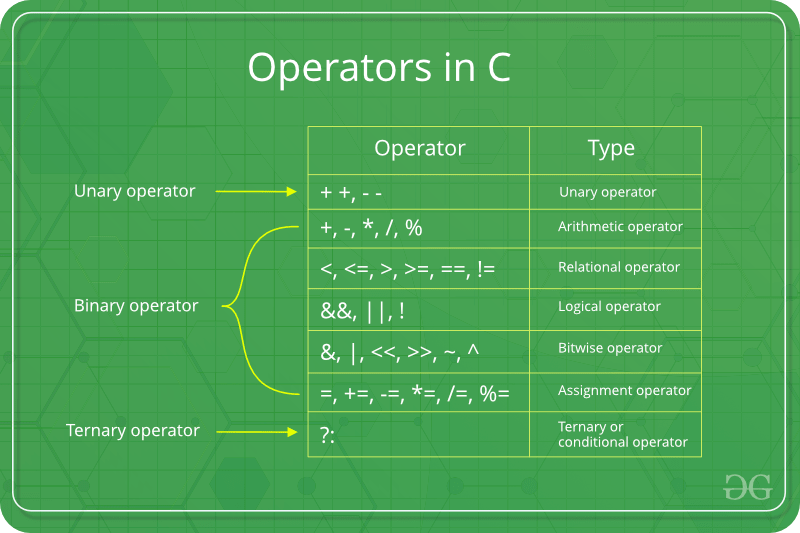
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
- C-Operators
- cpp-operator
- 10 Best Todoist Alternatives in 2024 (Free)
- How to Get Spotify Premium Free Forever on iOS/Android
- Yahoo Acquires Instagram Co-Founders' AI News Platform Artifact
- OpenAI Introduces DALL-E Editor Interface
- Top 10 R Project Ideas for Beginners in 2024
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
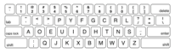
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 08:36.
- This page has been accessed 54,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- Read Tutorial
- Watch Guide Video
If that is about as clear as mud don't worry we're going to walk through a number of examples. And one very nice thing about the syntax for assignment operators is that it is nearly identical to a standard type of operator. So if you memorize the list of all the python operators then you're going to be able to use each one of these assignment operators quite easily.
The very first thing I'm going to do is let's first make sure that we can print out the total. So right here we have a total and it's an integer that equals 100. Now if we wanted to add say 10 to 100 how would we go about doing that? We could reassign the value total and we could say total and then just add 10. So let's see if this works right here. I'm going to run it and you can see we have a hundred and ten. So that works.

However, whenever you find yourself performing this type of calculation what you can do is use an assignment operator. And so the syntax for that is going to get rid of everything here in the middle and say plus equals and then whatever value. In this case I want to add onto it.
So you can see we have our operator and then right afterward you have an equal sign. And this is going to do is exactly like what we had before. So if I run this again you can see total is a hundred and ten

I'm going to just so you have a reference in the show notes I'm going to say that total equals total plus 10. This is exactly the same as what we're doing right here we're simply using assignment in order to do it.
I'm going to quickly go through each one of the other elements that you can use assignment for. And if you go back and you reference the show notes or your own notes for whenever you kept track of all of the different operators you're going to notice a trend. And that is because they're all exactly the same. So here if I want to subtract 10 from the total I can simply use the subtraction operator here run it again. And now you can see we have 90. Now don't be confused because we only temporarily change the value to 1 10. So when I commented this out and I ran it from scratch it took the total and it subtracted 10 from that total and that's what got printed out.
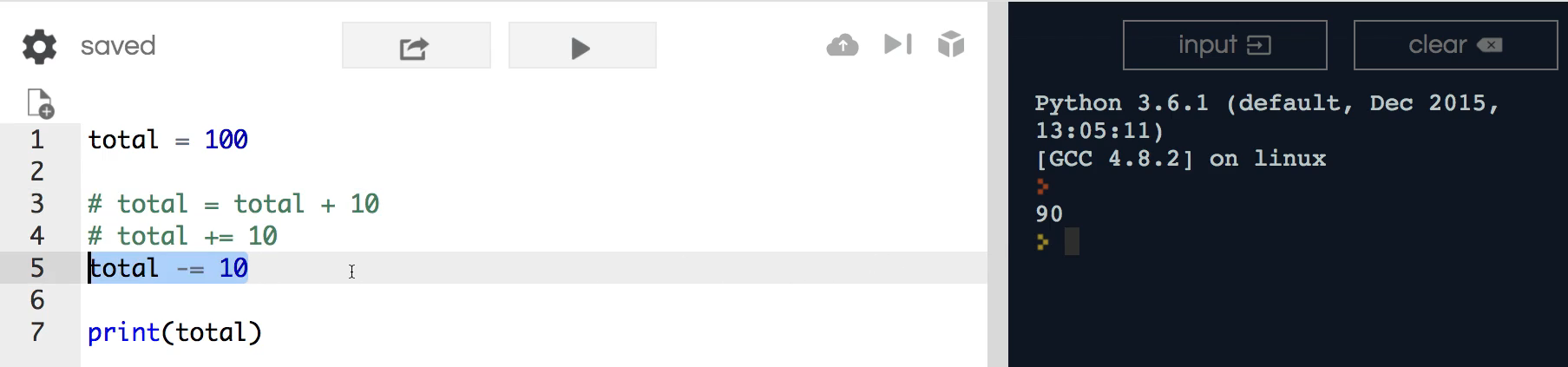
I'm going to copy this and the next one down the line is going to be multiplication. So in this case I'm going to say multiply with the asterisk the total and I'm just going to say times two just so we can see exactly what the value is going to be. And now we can see that's 200 which makes sense.
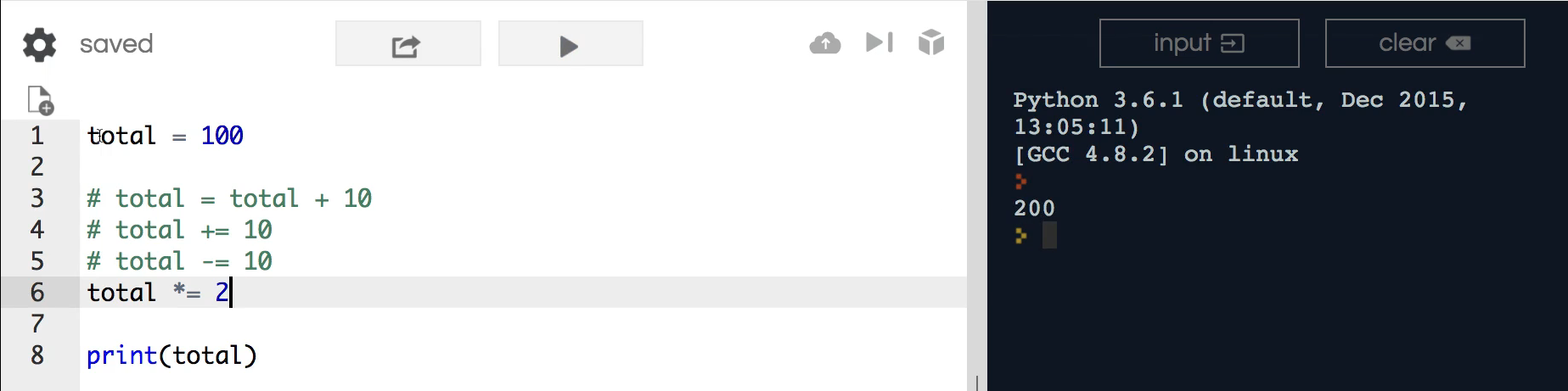
So we've taken total we have multiplied it by two and we have piped the entire thing into the total variable. So far so good. As you may have guessed next when we're going to do is division. So now I'm going to say total and then we're going to perform this division assignment and we're going to say divide this by 10 run it and you can see it gives us the value and it converts it to a float of ten point zero.
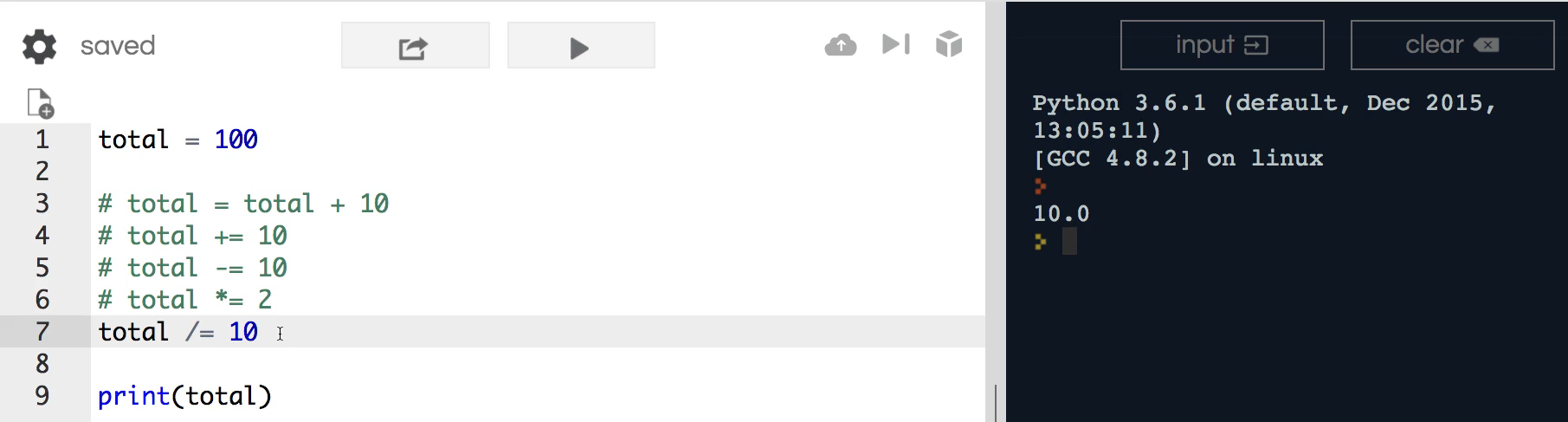
Now if this is starting to get a little bit much. Let's take a quick pause and see exactly what this is doing. Remember that all we're doing here is it's a shortcut. You could still perform it the same way we have in number 3 I could say total is equal to the total divided by 10. And if I run this you'll see we get ten point zero. And let's see what this warning is it says redefinition of total type from int to float. So we don't have to worry about this and this for if you're building Python programs you're very rarely ever going to see the syntax and it's because we have this assignment operator right here. So that is for division. And we also have the ability to use floor division as well. So if I run this you're going to see it's 10. But one thing you may notice is it's 10 it's not ten point zero. So remember that our floor division returns an integer it doesn't return a floating-point number. So if that is what you want then you can perform that task just like we did there.
Next one on the list is our exponents. I'm going to say the total and we're going to say we're going to assign that to the total squared. So going to run this and we get ten thousand. Just like you'd expect. And we have one more which is the modulus operator. So here remember it is the percent equals 2. And this is going to return zero because 100 is even if we changed 100 to be 101. This is going to return one because remember the typical purpose of the modulus operator is to let you know if you're working with an event or an odd value.
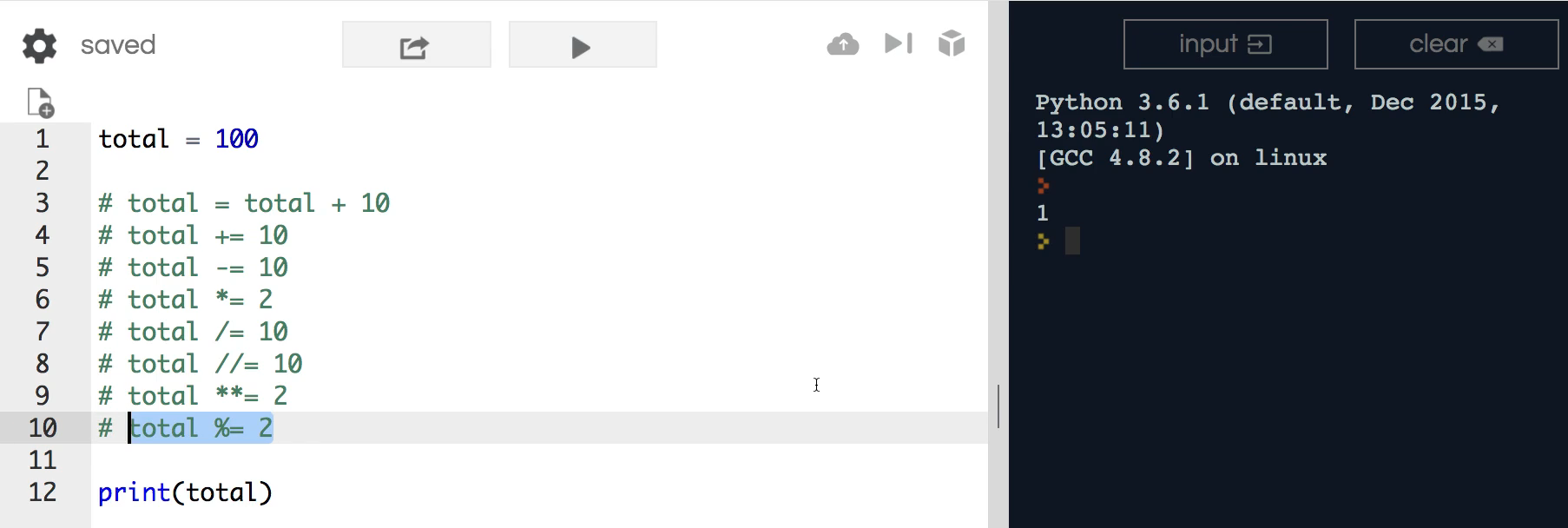
Now with all this being said, I wanted to show you every different option that you could use the assignment operator on. But I want to say that the most common way that you're going to use this or the most common one is going to be this one right here where we're adding or subtracting. So those are going to be the two most common. And what usually you're going to use that for is when you're incrementing or decrementing values so a very common way to do this would actually be like we have our total right here. So we have a total of 100 and you could imagine it being a shopping cart and it's 100 dollars and you could say product 2 and set this equal to 120. And then if I say product 3 and set this equal to 10. And so what I could do here is I could say total plus equals product to and then we could take the value and say product 3 and now if I run this you can see the value is 230.
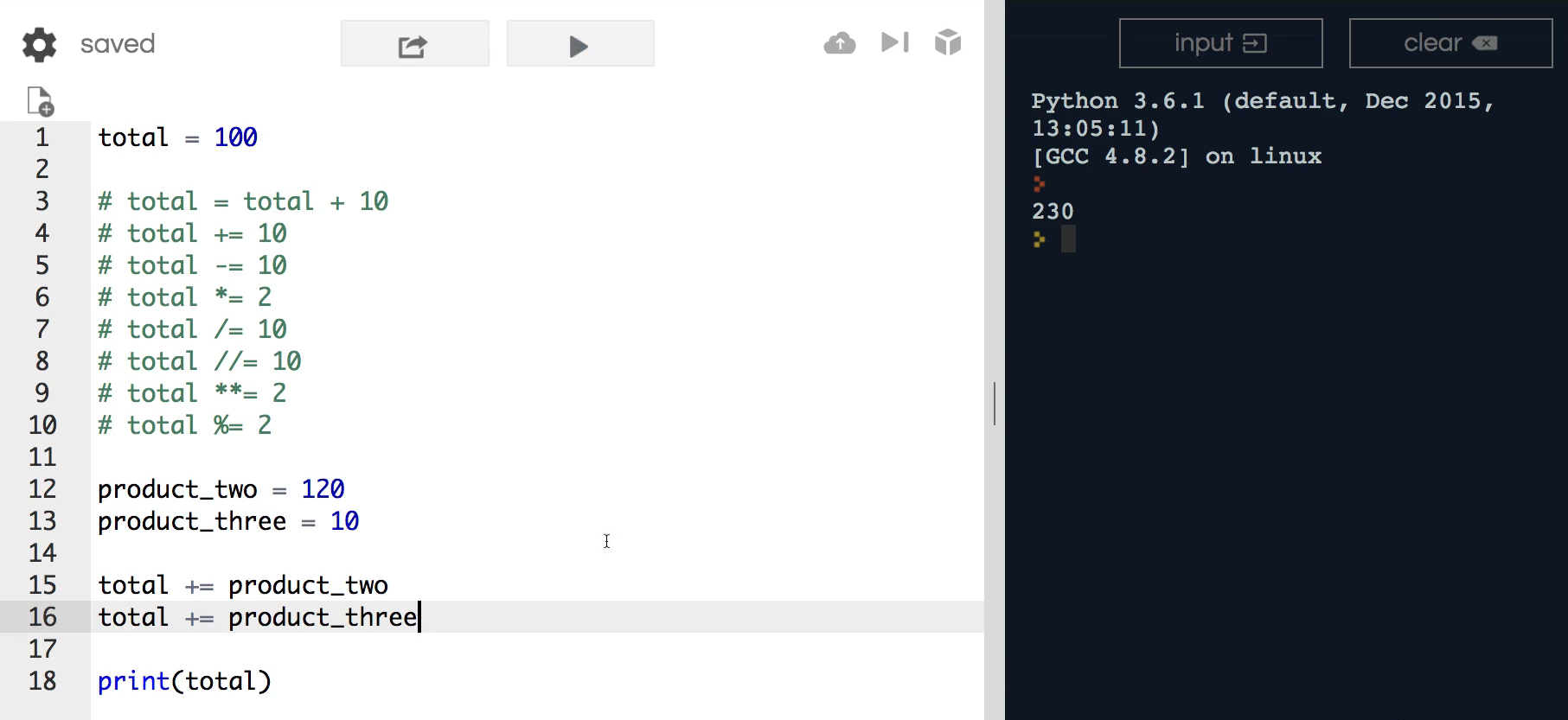
So that's a very common way whenever you want to generate a sum you can use this type of syntax which is much faster and it's also going to be a more pythonic way it's going to be the way you're going to see in standard Python programs whenever you're wanting to generate a sum and then reset and reassign the value.
So in review, that is how you can use assignment operators in Python.
devCamp does not support ancient browsers. Install a modern version for best experience.

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
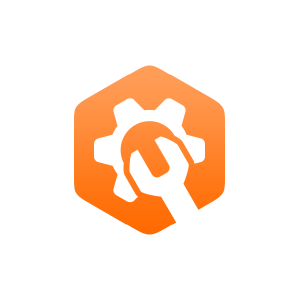
4.5: Assignment Operator
- Last updated
- Save as PDF
- Page ID 10258
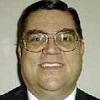
- Kenneth Leroy Busbee
- Houston Community College via OpenStax CNX
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
Definitions
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
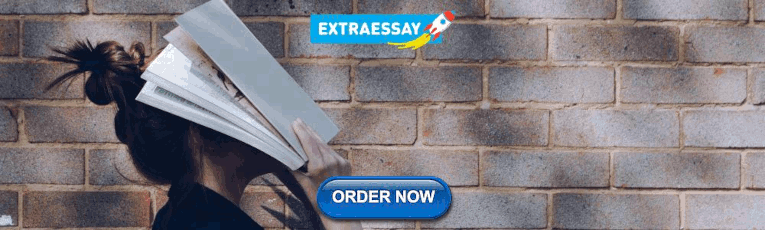
Bitwise AND assignment (&=)
The bitwise AND assignment ( &= ) operator performs bitwise AND on the two operands and assigns the result to the left operand.
Description
x &= y is equivalent to x = x & y , except that the expression x is only evaluated once.
Using bitwise AND assignment
Specifications, browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Bitwise AND ( & )
PHYSICS CALCULATOR AREA CALCULATOR
Arithmetic operators calculator, assignment operators calculator, comparison operators calculator, logical operators calculator, bitwise operators calculator, absalom equation.
ABSALOM'S EQUATION PDF files. Download the file instead
- TutorialKart
- SAP Tutorials
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Bash Script
- Julia Tutorial
- CouchDB Tutorial
- MongoDB Tutorial
- PostgreSQL Tutorial
- Android Compose
- Flutter Tutorial
- Kotlin Android
Web & Server
- Selenium Java
- C++ Tutorials
- C++ Tutorial
- C++ Hello World Program
- C++ Comments
- C++ Variables
- Print datatype of variable
- C++ If Else
- Addition Operator
- Subtraction Operator
- Multiplication Operator
- Division Operator
- Modulus Operator
- Increment Operator
- Decrement Operator
- Simple Assignment
- Addition Assignment
- Subtraction Assignment
- Multiplication Assignment
- Division Assignment
- Remainder Assignment
- Bitwise AND Assignment
- Bitwise OR Assignment
- Bitwise XOR Assignment
- Left Shift Assignment
- Right Shift Assignment
- Bitwise AND
- ADVERTISEMENT
- Bitwise XOR
- Bitwise Complement
- Bitwise Left shift
- Bitwise Right shift
- Logical AND
- Logical NOT
- Not Equal to
- Greater than
- Greater than or Equal to
- Less than or Equal to
- Ternary Operator
- Do-while loop
- Infinite While loop
- Infinite For loop
- C++ Recursion
- Create empty string
- String length
- String comparison
- Get character at specific index
- Get first character
- Get last character
- Find index of substring
- Iterate over characters
- Print unique characters
- Check if strings are equal
- Append string
- Concatenate strings
- String reverse
- String replace
- Swap strings
- Convert string to uppercase
- Convert string to lowercase
- Append character at end of string
- Append character to beginning of string
- Insert character at specific index
- Remove last character
- Replace specific character
- Convert string to integer
- Convert integer to string
- Convert string to char array
- Convert char array to string
- Initialize array
- Array length
- Print array
- Loop through array
- Basic array operations
- Array of objects
- Array of arrays
- Convert array to vector
- Sum of elements in array
- Average of elements in array
- Find largest number in array
- Find smallest number in array
- Sort integer array
- Find string with least length in array
- Create an empty vector
- Create vector of specific size
- Create vector with initial values
- Copy a vector to another
- Vector length or size
- Vector of vectors
- Vector print elements
- Vector iterate using For loop
- Vector iterate using While loop
- Vector Foreach
- Get reference to element at specific index
- Check if vector is empty
- Check if vectors are equal
- Check if vector contains element
- Update/Transform
- Add element(s) to vector
- Append element to vector
- Append vector
- Insert element at the beginning of vector
- Remove first element from vector
- Remove last element from vector
- Remove element at specific index from vector
- Remove duplicates from vector
- Remove elements from vector based on condition
- Resize vector
- Swap elements of two vectors
- Remove all elements from vector
- Reverse a vector
- Sort a vector
- Conversions
- Convert vector to map
- Join vector elements to a string
- Filter even numbers in vector
- Filter odd numbers in vector
- Get unique elements of a vector
- Remove empty strings from vector
- Sort integer vector in ascending order
- Sort integer vector in descending order
- Sort string vector based on length
- Sort string vector lexicographically
- Split vector into two equal halves
- Declare tuple
- Initialise tuple
- Swap tuples
- Unpack tuple elements into variables
- Concatenate tuples
- Constructor
- Copy constructor
- Virtual destructor
- Friend class
- Friend function
- Inheritance
- Multiple inheritance
- Virtual inheritance
- Function overloading
- Operator overloading
- Function overriding
- C++ Try Catch
- Math acos()
- Math acosh()
- Math asin()
- Math asinh()
- Math atan()
- Math atan2()
- Math atanh()
- Math cbrt()
- Math ceil()
- Math copysign()
- Math cosh()
- Math exp2()
- Math expm1()
- Math fabs()
- Math fdim()
- Math floor()
- Math fmax()
- Math fmin()
- Math fmod()
- Math frexp()
- Math hypot()
- Math ilogb()
- Math ldexp()
- Math llrint()
- Math llround()
- Math log10()
- Math log1p()
- Math log2()
- Math logb()
- Math lrint()
- Math lround()
- Math modf()
- Math nearbyint()
- Math nextafter()
- Math nexttoward()
- Math remainder()
- Math remquo()
- Math rint()
- Math round()
- Math scalbln()
- Math scalbn()
- Math sinh()
- Math sqrt()
- Math tanh()
- Math trunc()
- Armstrong number
- Average of three numbers
- Convert decimal to binary
- Factorial of a number
- Factors of a number
- Fibonacci series
- Find largest of three numbers
- Find quotient and remainder
- HCF/GCD of two numbers
- Hello World
- LCM of two numbers
- Maximum of three numbers
- Multiply two numbers
- Sum of two numbers
- Sum of three numbers
- Sum of natural numbers
- Sum of digits in a number
- Swap two numbers
- Palindrome number
- Palindrome string
- Pattern printing
- Power of a number
- Prime number
- Prime Numbers between two numbers
- Print number entered by User
- Reverse a Number
- Read input from user
- ❯ C++ Tutorial
- ❯ C++ Operators
- ❯ Assignment Operators
- ❯ Right Shift Assignment
C++ Right-shift Assignment (>>=) Operator
In this C++ tutorial, you shall learn about Right-shift Assignment operator, its syntax, and how to use this operator, with examples.
C++ Right-shift Assignment
In C++, Right-shift Assignment Operator is used to right shift value in the variable (left operand) by a value (right operand) and assign the result back to this variable (left operand).
The syntax to right shift a value in variable x by 2 places and assign the result to x using Right-shift Assignment Operator is
In the following example, we take a variable x with an initial value of 5 , add right shift by 2 places, and assign the result to x , using Right-shift Assignment Operator.
In this C++ Tutorial , we learned about Right-shift Assignment Operator in C++, with examples.
Popular Courses by TutorialKart
App developement, web development, online tools.
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in python.
Last Updated on June 8, 2023 by Prepbytes
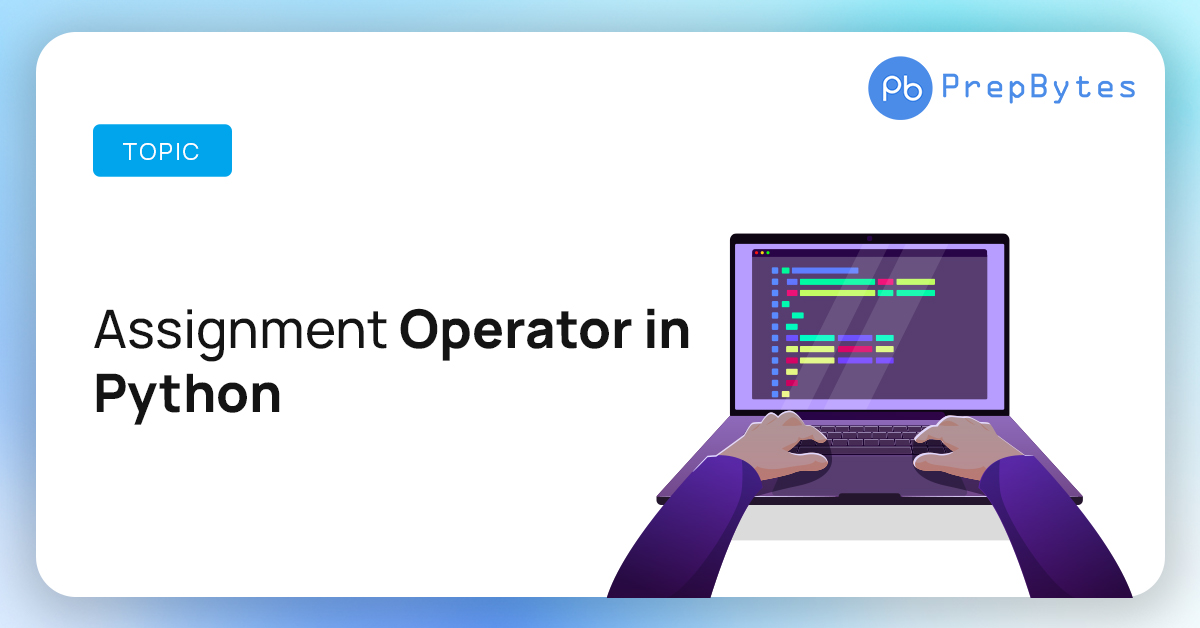
To fully comprehend the assignment operators in Python, it is important to have a basic understanding of what operators are. Operators are utilized to carry out a variety of operations, including mathematical, bitwise, and logical operations, among others, by connecting operands. Operands are the values that are acted upon by operators. In Python, the assignment operator is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is the most commonly used operator in Python. In this article, we will explore the assignment operator in Python, how it works, and its different types.
What is an Assignment Operator in Python?
The assignment operator in Python is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is used to assign a value to a variable. When an assignment operator is used, the value on the right-hand side is assigned to the variable on the left-hand side. This is a fundamental operation in programming, as it allows developers to store data in variables that can be used throughout their code.
For example, consider the following line of code:
Explanation: In this case, the value 10 is assigned to the variable a using the assignment operator. The variable a now holds the value 10, and this value can be used in other parts of the code. This simple example illustrates the basic usage and importance of assignment operators in Python programming.
Types of Assignment Operator in Python
There are several types of assignment operator in Python that are used to perform different operations. Let’s explore each type of assignment operator in Python in detail with the help of some code examples.
1. Simple Assignment Operator (=)
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is:
Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example
Explanation: In this case, the value 25 is assigned to the variable a using the simple assignment operator. The variable a now holds the value 25.
2. Addition Assignment Operator (+=)
The addition assignment operator is used to add a value to a variable and store the result in the same variable. The syntax for the addition assignment operator is:
Here, the value on the right-hand side is added to the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is incremented by 5 using the addition assignment operator. The result, 15, is then printed to the console.
3. Subtraction Assignment Operator (-=)
The subtraction assignment operator is used to subtract a value from a variable and store the result in the same variable. The syntax for the subtraction assignment operator is
Here, the value on the right-hand side is subtracted from the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is decremented by 5 using the subtraction assignment operator. The result, 5, is then printed to the console.
4. Multiplication Assignment Operator (*=)
The multiplication assignment operator is used to multiply a variable by a value and store the result in the same variable. The syntax for the multiplication assignment operator is:
Here, the value on the right-hand side is multiplied by the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is multiplied by 5 using the multiplication assignment operator. The result, 50, is then printed to the console.
5. Division Assignment Operator (/=)
The division assignment operator is used to divide a variable by a value and store the result in the same variable. The syntax for the division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 5 using the division assignment operator. The result, 2.0, is then printed to the console.
6. Modulus Assignment Operator (%=)
The modulus assignment operator is used to find the remainder of the division of a variable by a value and store the result in the same variable. The syntax for the modulus assignment operator is
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the remainder is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the modulus assignment operator. The remainder, 1, is then printed to the console.
7. Floor Division Assignment Operator (//=)
The floor division assignment operator is used to divide a variable by a value and round the result down to the nearest integer, and store the result in the same variable. The syntax for the floor division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is rounded down to the nearest integer. The rounded result is then stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the floor division assignment operator. The result, 3, is then printed to the console.
8. Exponentiation Assignment Operator (**=)
The exponentiation assignment operator is used to raise a variable to the power of a value and store the result in the same variable. The syntax for the exponentiation assignment operator is:
Here, the variable on the left-hand side is raised to the power of the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is raised to the power of 3 using the exponentiation assignment operator. The result, 8, is then printed to the console.
9. Bitwise AND Assignment Operator (&=)
The bitwise AND assignment operator is used to perform a bitwise AND operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise AND assignment operator is:
Here, the variable on the left-hand side is ANDed with the value on the right-hand side using the bitwise AND operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ANDed with 3 using the bitwise AND assignment operator. The result, 2, is then printed to the console.
10. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator is used to perform a bitwise OR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise OR assignment operator is:
Here, the variable on the left-hand side is ORed with the value on the right-hand side using the bitwise OR operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ORed with 3 using the bitwise OR assignment operator. The result, 7, is then printed to the console.
11. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator is used to perform a bitwise XOR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise XOR assignment operator is:
Here, the variable on the left-hand side is XORed with the value on the right-hand side using the bitwise XOR operator, and the result are stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is XORed with 3 using the bitwise XOR assignment operator. The result, 5, is then printed to the console.
12. Bitwise Right Shift Assignment Operator (>>=)
The bitwise right shift assignment operator is used to shift the bits of a variable to the right by a specified number of positions, and store the result in the same variable. The syntax for the bitwise right shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the right by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is shifted 2 positions to the right using the bitwise right shift assignment operator. The result, 2, is then printed to the console.
13. Bitwise Left Shift Assignment Operator (<<=)
The bitwise left shift assignment operator is used to shift the bits of a variable to the left by a specified number of positions, and store the result in the same variable. The syntax for the bitwise left shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the left by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Conclusion Assignment operator in Python is used to assign values to variables, and it comes in different types. The simple assignment operator (=) assigns a value to a variable. The augmented assignment operators (+=, -=, *=, /=, %=, &=, |=, ^=, >>=, <<=) perform a specified operation and assign the result to the same variable in one step. The modulus assignment operator (%) calculates the remainder of a division operation and assigns the result to the same variable. The bitwise assignment operators (&=, |=, ^=, >>=, <<=) perform bitwise operations and assign the result to the same variable. The bitwise right shift assignment operator (>>=) shifts the bits of a variable to the right by a specified number of positions and stores the result in the same variable. The bitwise left shift assignment operator (<<=) shifts the bits of a variable to the left by a specified number of positions and stores the result in the same variable. These operators are useful in simplifying and shortening code that involves assigning and manipulating values in a single step.
Here are some Frequently Asked Questions on Assignment Operator in Python:
Q1 – Can I use the assignment operator to assign multiple values to multiple variables at once? Ans – Yes, you can use the assignment operator to assign multiple values to multiple variables at once, separated by commas. For example, "x, y, z = 1, 2, 3" would assign the value 1 to x, 2 to y, and 3 to z.
Q2 – Is it possible to chain assignment operators in Python? Ans – Yes, you can chain assignment operators in Python to perform multiple operations in one line of code. For example, "x = y = z = 1" would assign the value 1 to all three variables.
Q3 – How do I perform a conditional assignment in Python? Ans – To perform a conditional assignment in Python, you can use the ternary operator. For example, "x = a (if a > b) else b" would assign the value of a to x if a is greater than b, otherwise it would assign the value of b to x.
Q4 – What happens if I use an undefined variable in an assignment operation in Python? Ans – If you use an undefined variable in an assignment operation in Python, you will get a NameError. Make sure you have defined the variable before trying to assign a value to it.
Q5 – Can I use assignment operators with non-numeric data types in Python? Ans – Yes, you can use assignment operators with non-numeric data types in Python, such as strings or lists. For example, "my_list += [4, 5, 6]" would append the values 4, 5, and 6 to the end of the list named my_list.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Solver Title

Generating PDF...
- Pre Algebra Order of Operations Factors & Primes Fractions Long Arithmetic Decimals Exponents & Radicals Ratios & Proportions Percent Modulo Number Line Mean, Median & Mode
- Algebra Equations Inequalities System of Equations System of Inequalities Basic Operations Algebraic Properties Partial Fractions Polynomials Rational Expressions Sequences Power Sums Interval Notation Pi (Product) Notation Induction Logical Sets Word Problems
- Pre Calculus Equations Inequalities Scientific Calculator Scientific Notation Arithmetics Complex Numbers Polar/Cartesian Simultaneous Equations System of Inequalities Polynomials Rationales Functions Arithmetic & Comp. Coordinate Geometry Plane Geometry Solid Geometry Conic Sections Trigonometry
- Calculus Derivatives Derivative Applications Limits Integrals Integral Applications Integral Approximation Series ODE Multivariable Calculus Laplace Transform Taylor/Maclaurin Series Fourier Series Fourier Transform
- Functions Line Equations Functions Arithmetic & Comp. Conic Sections Transformation
- Linear Algebra Matrices Vectors
- Trigonometry Identities Proving Identities Trig Equations Trig Inequalities Evaluate Functions Simplify
- Statistics Mean Geometric Mean Quadratic Mean Average Median Mode Order Minimum Maximum Probability Mid-Range Range Standard Deviation Variance Lower Quartile Upper Quartile Interquartile Range Midhinge Standard Normal Distribution
- Physics Mechanics
- Chemistry Chemical Reactions Chemical Properties
- Finance Simple Interest Compound Interest Present Value Future Value
- Economics Point of Diminishing Return
- Conversions Roman Numerals Radical to Exponent Exponent to Radical To Fraction To Decimal To Mixed Number To Improper Fraction Radians to Degrees Degrees to Radians Hexadecimal Scientific Notation Distance Weight Time Volume
- Pre Algebra
- One-Step Addition
- One-Step Subtraction
- One-Step Multiplication
- One-Step Division
- One-Step Decimals
- Two-Step Integers
- Two-Step Add/Subtract
- Two-Step Multiply/Divide
- Two-Step Fractions
- Two-Step Decimals
- Multi-Step Integers
- Multi-Step with Parentheses
- Multi-Step Rational
- Multi-Step Fractions
- Multi-Step Decimals
- Solve by Factoring
- Completing the Square
- Quadratic Formula
- Biquadratic
- Logarithmic
- Exponential
- Rational Roots
- Floor/Ceiling
- Equation Given Roots
- Newton Raphson
- Substitution
- Elimination
- Cramer's Rule
- Gaussian Elimination
- System of Inequalities
- Perfect Squares
- Difference of Squares
- Difference of Cubes
- Sum of Cubes
- Polynomials
- Distributive Property
- FOIL method
- Perfect Cubes
- Binomial Expansion
- Negative Rule
- Product Rule
- Quotient Rule
- Expand Power Rule
- Fraction Exponent
- Exponent Rules
- Exponential Form
- Logarithmic Form
- Absolute Value
- Rational Number
- Powers of i
- Partial Fractions
- Is Polynomial
- Leading Coefficient
- Leading Term
- Standard Form
- Complete the Square
- Synthetic Division
- Linear Factors
- Rationalize Denominator
- Rationalize Numerator
- Identify Type
- Convergence
- Interval Notation
- Pi (Product) Notation
- Boolean Algebra
- Truth Table
- Mutual Exclusive
- Cardinality
- Caretesian Product
- Age Problems
- Distance Problems
- Cost Problems
- Investment Problems
- Number Problems
- Percent Problems
- Addition/Subtraction
- Multiplication/Division
- Dice Problems
- Coin Problems
- Card Problems
- Pre Calculus
- Linear Algebra
- Trigonometry
- Conversions

Most Used Actions
Number line.
- simplify\:4+(2+1)^2
- simplify\:\frac{1}{x+1}\cdot \frac{x^2}{5}
- factor\:x^{2}-5x+6
- factor\:2x^2+13x+15
- expand\:3(x+6)
- expand\:2x(x-a)
basic-operations-calculator
- Middle School Math Solutions – Polynomials Calculator, Factoring Quadratics Just like numbers have factors (2×3=6), expressions have factors ((x+2)(x+3)=x^2+5x+6). Factoring is the process...
Please add a message.
Message received. Thanks for the feedback.
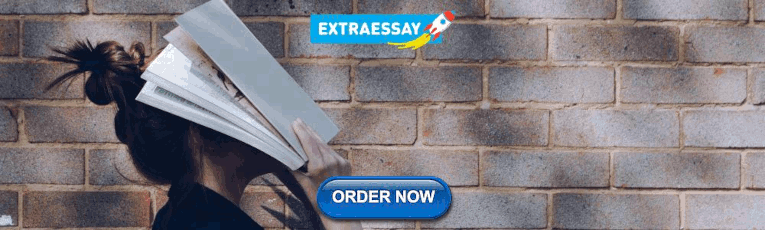
IMAGES
VIDEO
COMMENTS
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: 2. "+=": This operator is combination of '+' and '=' operators.
Solution Help. Hungarian method calculator. 1. A computer centre has 3expert programmers. The centre wants 3 application programmes to be developed. The head of thecomputer centre, after studying carefully the programmes to be developed, estimates the computer time in minutes required by the experts for the application programmes as follows.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left. Example: (a -= b) can be written as (a = a - b) If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Assignment operators have right-to-left associativity, meaning they evaluate expressions from right to left, which allows for chaining assignments like "a = b = c = 5;". ... For example, a program may require multiple variables with different values to calculate the user's age or to store various data results for later analysis. By ...
Earlier on in this section we talked about how we could use mathematical operators to work with numbers in python and in this guide. I'm going to talk about the assignment operator and this is going to give us the ability to perform a calculation while we're performing assignment.
Discussion. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
There is already a special instance of overloading = in place that the designers deemed ok: property setters. Let X be a property of foo. In foo.X = 3, the = symbol is replaced by the compiler by a call to foo.set_X(3). You can already define a public static T op_Assign(ref T assigned, T assignee) method. All that is left is for the compiler to ...
To demonstrate this formula, you can calculate the distance between Oslo (59.9°N 10.8°E) and Vancouver (49.3°N 123.1°W) ... In the examples you've seen so far, the := assignment expression operator does essentially the same job as the = assignment operator in your old code. You've seen how to simplify code, and now you'll learn about ...
Assignment operators (C# reference) The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the ...
The bitwise AND assignment (&=) operator performs bitwise AND on the two operands and assigns the result to the left operand. Try it. Syntax. js. x &= y Description. x &= y is equivalent to x = x & y, except that the expression x is only evaluated once. Examples. Using bitwise AND assignment. js.
not equal value or not equal types ! = = = logical operators calculator. logical not ! = logical and && =
In C++, Right-shift Assignment Operator is used to right shift value in the variable (left operand) by a value (right operand) and assign the result back to this variable (left operand). The syntax to right shift a value in variable x by 2 places and assign the result to x using Right-shift Assignment Operator is. x >>= 2.
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is: variable = value. Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example.
Middle School Math Solutions - Polynomials Calculator, Factoring Quadratics. Just like numbers have factors (2×3=6), expressions have factors ((x+2)(x+3)=x^2+5x+6). Factoring is the process... Enter a problem. Cooking Calculators. Cooking Measurement Converter Cooking Ingredient Converter Cake Pan Converter More calculators.
Codecademy is the easiest way to learn how to code. It's interactive, fun, and you can do it with your friends.