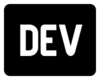
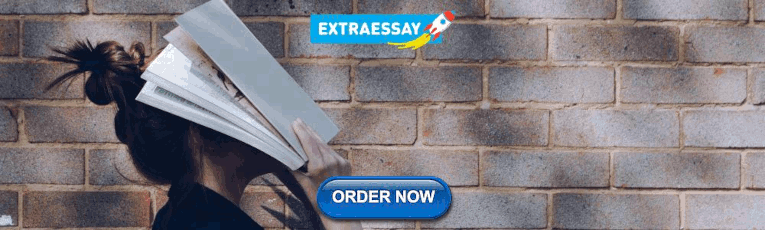
DEV Community

Posted on Aug 29, 2021 • Updated on Aug 30, 2021
Complete Data Structures and Algorithms Roadmap for Placements (Part-1)
Mastering of DSA are always sought by big product-based companies because it ensures good handling of their data and provides a good measure of a candidate’s problem-solving skills.
If you will follow this roadmap, then your preparation will be organized. Here are Topics and most imp problems from listed sub-topics
Very Very IMP Topics
Arrays & Strings
- Basic Array And Strings Questions
- Kadane’s Algorithm
- Dutch National Flag Algorithm
Multidimensional Arrays
- Rotation based problems.
- Traversal Based Problems
Sorting Algorithms & Searching
- Insertion Sort
- Selection Sort.
- Binary Search on Arrays and matrices
Linked Lists
- Reversal Problems
- Sorting Problems.
- Slow And Fast Pointers
- Modify In Linked list
Stacks and Queues
- Implementation Problems
- Application Problems.
Binary Trees
- Construction Of BST
- Conversion Based Problems
- Modification in BST
- Standard Problems
Recursion and Backtracking
- Basic Recursion Questions
- Divide And Conquer
Priority Queues and Heaps
- Implementation Based problems
- Conversion based problems
- K Based Problems
- Graph Traversals – BFS And DFS
- Shortest Path Algorithms
- Topological Sort
- Graphs in Matrix
Dynamic Programming on study material, daily ques, company wise Leetcode problems.
- DP with Arrays
- DP With Strings
- DP With Maths
- DP With Trees
- Breaking And Partition Based Problems
- Counting Based Problems
After completing them, do these!!
- Bit Manipulation
- Circular Queues
- String Algorithms like KMP and Z Algorithm
- Number Theory
Stay tuned for the next post-
💖 from sakshamceo
Top comments (0)
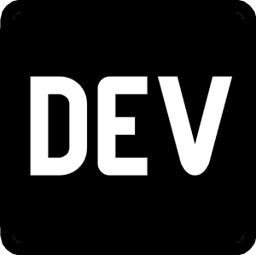
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
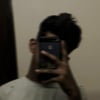
Middlewares = Magic? 3 Reasons Every Dev Should Adopt This Magic! ✨🔥
Arjun Vijay Prakash - Mar 30
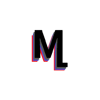
HOW I MADE CITEAL 2.0 MY VERY OWN APP. 😆
Mince - Apr 16
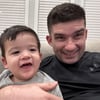
Scaling App Data in DynamoDB With Vertical Partitioning
RyTheTurtle - Mar 26

React.memo - Optimize React Functional Components
Ashfiquzzaman Sajal - Apr 17
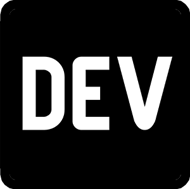
We're a place where coders share, stay up-to-date and grow their careers.
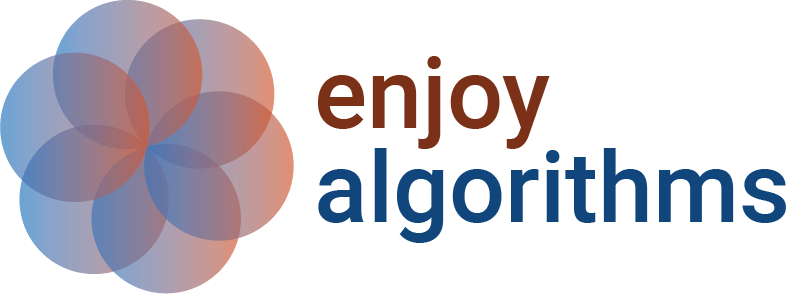
EnjoyMathematics
Guide to Learn Data Structures and Algorithms for Interviews
Introduction.
A solid understanding of data structures and algorithms is crucial for programmers to enhance problem-solving skills in computer science. On other side, interviewers often use DSA questions to evaluate coding skills, problem-solving skill, and clarity of thought.
So one idea is clear: Knowledge of algorithms and data structures is not only important for growing as a smart programmer but also for achieving dream success in tech industry. If you want to master DSA, following a well-defined guided learning plan is important, especially if you are a beginner with basic programming skills.
At the start of the learning journey, you may have questions like this:
- How can I develop a long-term interest in DSA?
- How do I create a continuous learning plan?
- Which topics are good to start the learning journey?
- What are the most crucial DSA concepts and problem-solving approaches?
- What are some key activities that will help me learn effectively?
- How can I prepare for a coding interview in a short time?
If you are looking for answers to these questions, then you have come to the right place. Let's start step by step.
Step 1: Developing long-term motivation
Learning and mastering data structures and algorithms require motivation and perseverance. Here are some thoughts that can keep you motivated on your journey:
- The ability to design efficient algorithms is a highly valued skill in programming, and mastering it can lead to great career opportunities.
- Never get discouraged by setbacks. Once you grasp the basics, your DSA problem-solving skills will improve tremendously.
- Never give up! If you encounter a difficult problem, challenge yourself to give it one more try. These moments can be defining points in your DSA journey.
- Mastering coding and problem-solving is a continuous process that involves learning from mistakes. Each error or bug presents an opportunity to gain new insights and improve your skills.
Blog to explore: Why should we learn Data Structures and Algorithms?
Step 2: Understanding data structures and algorithms syllabus
Now, our goal should be to prepare a list of important topics. To help you with this, we are sharing here a comprehensive list of DSA topics to learn in a defined order. Note: Never forget to explore real-world applications to understand how algorithms are used in practice.
1. Algorithmic and mathematical thinking
Important topics: Algorithmic puzzles, Brainteaser puzzles, Counting, Summation, Numbers theory, Permutation, Mathematical induction, Recurrence relations, Bit manipulation, Probability, etc.
Blog to explore: How to develop algorithmic thinking?
2. Fundamentals of programming
Important topics: Data types, Variables, Operators, Expressions, Control statements, Loop, Function, Pointers and References, Array, String, Memory management, Fundamental of OOPS, etc.
3. Fundamental of algorithm
- Algorithm introduction: Properties and Real-Life Applications of Algorithms.
- Complexity analysis: Input size, Rate of growth, Time complexity, Big-O notations, Worst-case analysis, Best-case analysis, Average-case analysis, Space complexity analysis, etc.
- Iteration: Properties of iteration, Analysis of loop, Patterns of iterative problem-solving such as building partial solutions using single loop, Nested loop, Two pointers, Sliding window, etc.
- Recursion: Properties of recursion, Analysis of recursion, Patterns of recursive problem-solving such as decrease and conquer, Divide and conquer, etc.
- Sorting: Bubble sort, Selection sort, Insertion sort, Merge sort, Quick sort, Heap sort, Counting sort, Radix sort, Properties and comparisons of sorting algorithms, etc.
- Searching: Binary search, Exponential search, Interpolation search, etc.
- Introduction to data structures: Properties, Types, Key operations, Concepts of abstract data types, Real-life applications.
4. Linear data structures
- Array: 1D array, 2D array, string, dynamic array
- Linked list: Singly linked list, doubly linked list, circular linked list.
- Stack , queue , and dequeue .
5. Non-linear data structures
- Hashing: Direct address table, Hash table, Properties and design of a good hash function, Basic operations (insert, delete, and search), Patterns of problem-solving using hash table, Collision resolution techniques like chaining and open addressing, etc.
- Binary tree: Properties and structure, Recursive DFS traversals, Iterative DFS traversal, BFS traversal, Basic operations, Patterns of problem-solving using BFS and DFS traversals, etc.
- Binary search tree: Properties and structure, BST building, BST operations, BST sort, Patterns of problem-solving in BST, Self-balancing BST, Real-life applications, Comparison with hash table.
- Heap: Properties and structure, Heap building, Basic heap operations, Heap sort, Patterns of problem solving, Real-life applications.
- Dictionary and Priority queue: Implementation using array, linked list, BST, Heap (priority queue) and Hash Table (Dictionary), Time complexity comparisons of basic operations, and Real-life applications.
- Advanced data structures (Properties, structure, basic operations, patterns of problem-solving and Real-life applications): Trie, Segment tree, Binary indexed tree, K-dimensional tree, n-ary tree, Suffix tree.
- Graph: Properties, Structure, Types, Adjacency matrix representation, Adjacency list representation, BFS traversal and its properties, DFS traversal and its properties, Problem-solving patterns using DFS and BFS, Topological sorting, Shortest path algorithms, Minimum spanning tree, etc.
6. Algorithm design techniques
- Dynamic programming: Time memory tradeoff, optimization and combinatorial problems, Designing recursive solution of a DP problem, Top-down approach, Bottom-up approach, Problem-solving patterns, Divide and conquer vs Dynamic programming, etc.
- Greedy algorithms: Greedy choice property, Problem solving patterns, DP vs Greedy algorithms, etc.
- Backtracking: Exhaustive search, Recursive and iterative backtracking, Branch and bound, Bit-masking, etc.
- String algorithms: String matching, Patterns of problem solving, etc.
- Mathematical algorithms: Numbers theoretic algorithms, Bitwise algorithms, Randomised algorithms, etc.
7. Coding interview preparation
- Continuous practice of coding questions
- Profile and resume building
- Preparation via mock Interview
- Company-specific research of interview process
Behavioral interview preparation
Step 3: Collecting the best resources for learning and practice
Our next step should be to gather one of the best learning resources: books, blogs, talks, PDFs, courses, etc. The internet can be a great tool for this. Note: It's important to follow authentic sources and organize them according to the syllabus we've outlined. Here is the list of some best learning resources:
Data structures and algorithms resources for beginners
- Book: Algorithms Unlocked by Thomas Coreman, MIT Press
- Book: Algorithmic Puzzles by Anany and Maria Levitin, Oxford University Press
- Course: Mathematics for computer science by MIT Open Courseware
Resources for learning basic and advanced programming concepts
- Book: Think Python (How to Think Like a Computer Scientist)
- Book: C++ Primer
- Book: Introduction to Programming in Java
- Course: Programming Paradigms by Stanford
- Course: Programming Abstraction by Stanford
- Course: Programming Methodology by Stanford
- Book: Clean Code by Robert Martin
Data structures and algorithms resources for advanced learners
- Algorithms by CLRS (Book): Complexity analysis, Divide and conquer, Sorting, Linear data structures, Hash table, Heap, BST, Graph, Dynamic programming, Greedy algorithms, String algorithms.
- The Algorithm Design Manual by Steven Skiena (Book): Complexity analysis, Sorting and Searching, Linear and Non-linear data structures, Backtracking and Dynamic programming.
- Algorithms by Robert Sedgewick (Book): Complexity analysis, Sorting, Stack, Queue, Priority queue, Hash table, Binary search tree, Graph, String algorithms.
- Course: Introduction to Algorithms by MIT Open Courseware.
For competitive programming
- Project Euler
For data structures and algorithms interview
- EnjoyAlgorithms DSA Course
- Cracking the Coding Interview by Gayle Laakmann McDowell
- Leetcode for coding problems practice
Step 4: Finding good mentors and communities
If you want to take your DSA skills to the next level, having a good mentor can be invaluable. An experienced mentor can guide you in building a strong foundation in data structures, mastering problem-solving strategies, clarifying doubts, and preparing for coding interviews.
Additionally, forming a study group with like-minded individuals can be a great idea. This provides an excellent opportunity to learn from each other, support one another, and work towards a common DSA goals. You can also join online communities or groups to learn from experiences of others and seek help with coding questions.
Step 5: Preparing a continuous learning plan for coding Interview
This is one of the critical steps in learning data structures and algorithms. We recommend dedicating minimum of 10 hours per week and designing your plan based on your personal learning goals. Finding a balance that works for you is key, so make sure to stick to your plan consistently.
4-Week quick revision and coding interview preparation plan
This is recommended for learners who want to refresh their concepts and problem-solving for an incoming coding interview. Such learners should have basic problem-solving skills in data structure and algorithms and good knowledge of programming concepts.
- Minimum estimated time = 15 x 4 = 60 hours (Such learners should try to give atleast 15 hours per week).
- Minimum coding problems to practise = 50 (These questions should cover all popular approaches in DSA).
- Concept revision and problem-solving research = 20 hours.
- Problem-solving on paper with pseudocode = 20 Hours (At least 40 coding questions).
- Problem-solving with coding practice = 10 hours (At least 10 coding questions).
- Behavioural interview preparation + Mock interviews = 10 Hours.
8-Week revision and coding interview preparation plan
This is recommended for learners who want to revise DSA concepts and sharpen problem-solving skills for an incoming coding interview. Such learners should have basic problem-solving skills in data structure and algorithms and good knowledge of programming concepts.
- Minimum estimated time = 10 x 8 = 80 hours.
- Minimum coding problems to practise = 75.
- Problem-solving on paper with pseudocode = 25 Hours (at least 50 coding questions).
- Problem-solving with coding practice = 25 Hours (at least 25 coding questions).
16-Week learning and coding interview preparation plan
This is recommended for intermediate learners who want to learn DSA concepts and sharpen problem-solving skills. Such learners should have a basic understanding of programming concepts.
- Minimum estimated time = 10 x 16 = 160 hours.
- Minimum coding problems to practise = 150. (This list of questions should cover problems with various difficulties level).
- Concept learning/revision and problem-solving research = 40 hours.
- Problem-solving on paper with pseudocode = 50 hours (at least 100 coding questions).
- Problem-solving with coding practice = 50 hours (at least 50 coding questions).
- Behavioural interview preparation + Mock interviews = 20 hours.
24-Week learning and coding interview preparation plan
This is recommended for beginners who want to learn DSA from scratch and prepare for interview.
- Minimum estimated time = 10 x 24 = 240 hours.
- Minimum coding problems to practise = 150.
- Learning basic programming and math for DSA = 20 hours.
- Concept learning and problem-solving research = 80 hours.
- Problem-solving on paper with pseudocode = 60 hours (at least 100 coding questions).
- Problem-solving with coding practice = 60 hours (at least 50 coding questions)
Step 6: Starting continuous learning activities
Concept learning and revision.
To fully understand DSA concepts, it's crucial to learn them in the right order. You should create self-notes and use visuals to organize your ideas. This will help you in revising the concepts later. It's also a good idea to brainstorm with a friend to discuss each topic and to seek guidance from a mentor when needed.
Problem-solving research
Improving your DSA skills can be achieved by exploring patterns through various coding questions. It's important to identify important problem-solving strategies and their use cases, just like reading solutions and making notes about the thought process.
Before diving into problem-solving, we should explore various examples to understand the application of concepts. So we recommend taking the time to thoroughly analyze different approaches by going through the solution of at least 3-4 coding questions. This will help you gain a better understanding of different techniques.
Blog to explore: Popular Problem-Solving Approaches in Data Structures and Algorithms
Problem-solving on paper and coding practice
Practicing DSA problem-solving skills on paper or a whiteboard can be really helpful. Visualizing solutions, creating pseudo-codes, and outlining the steps of your algorithms can help you design more efficient and effective solutions. Using paper or a whiteboard is great because it allows you to easily sketch out your ideas and make changes as needed.
One more thing: There are a lot of problems available on the internet, so we need to choose some of the popular interview questions that can be solved using various approaches.
After designing pseudo-code, we should work to translate it into actual code using the programming language of your choice. It's also important to thoroughly test your code using a variety of input and output data, edge cases, and boundary conditions to make sure it's working correctly. We need to think:
- Does the code produce correct output for all possible inputs?
- Can we optimize the code further? It's always a good idea to improve efficiency of your code. If you have time, take another look at the solution and see if there's a better way to implement it.
- How can we improve the code readability? Making sure your code is well-organized and easy to understand is important for you and anyone else who might need to read and maintain it in the future.
Blog to explore: Steps of problem-solving in Data Structure and Algorithms
Resolving critical doubts
It's totally normal to have questions and doubts when you're learning algorithms and data structures. But don't let these doubts hold you back! Use them as opportunities to deepen your understanding and take your learning to the next level. If you're struggling to resolve a doubt, there are few ways to get some help:
- Talk to a friend or colleague about it.
- Ask a mentor or course instructor for guidance.
- Post your question in a coding or learning community.
- Make a note of the doubt and try it later with a fresh perspective. Sometimes, doubts may be related to concepts that you haven't learned yet, and it can be helpful to revisit them once you have more context.
Don't be afraid to ask for help. We all have to start somewhere, and seeking guidance is a great way to move forward and continue learning.
Interview preparation via mock interviews
Mock interviews are one of the best ways to get ready for the big day. Find a partner who shares similar career goals and conduct mock interviews with each other. This will help you get feedback on your performance and identify areas where you can improve.
- When selecting a partner, ensure they have a basic understanding of data structures and algorithms.
- During the mock interview, use a timer to keep the session to about 1 hour or 45 minutes, which is the average length of a real coding interview.
- To make the mock interview as realistic as possible, properly prepare your partner and follow all necessary steps. Don't forget to switch roles after the mock interview and take on the role of the interviewer. This will give you some insight into what companies are looking for in a candidate.
Mock interviews aren't just helpful for feedback, but they can also improve your body language and communication skills. Consider recording the session and reviewing the footage to identify areas for improvement.
Behavioral interviews are a critical part of the job interview process, particularly for programmers aspiring to secure their dream job. Surprisingly, many programmers tend to overlook the importance of behavioral interviews and fail to prepare for them adequately. It's vital to understand that behavioral interviews hold just as much significance as technical interviews.
In a behavioral interview, the interviewer assesses how you approach decision-making and handle challenging situations. This evaluation is crucial as it helps the interviewer determine whether you're the right fit for the company and the position. Therefore, investing time and effort into preparing for behavioral interviews is essential. Demonstrate your skills and abilities, and you'll be one step closer to securing your dream job!
The idea of behavioral interviews can vary from company to company, depending on their values and culture. Here are some tips to keep in mind:
- Practice good communication skills
- Read the job description more than once (the more the better!)
- Research the industry and company
- Prepare for common questions
- Make your sales pitch clear
- Pay attention to your body language
- Come up with smart questions for the interviewers
- Be positive, authentic, and truthful in your answers
- Stay optimistic and embrace the challenge
It's also a good idea to have at least one strong example of:
- An interesting technical problem you solved
- An interpersonal conflict you overcame
- A unique execution story about a past project
- Leadership or ownership
- A question about the company's product/business
- A question about the company's engineering strategy
Tips for Learning Data Structure and Algorithms
Here are some tips to help you get the most out of your data structures and algorithms journey:
- Aim to cover at least 80% of the syllabus and prioritize topics based on your strengths and weaknesses. If you find a topic challenging, don't be afraid to spend more time on it.
- Conduct a weekly critical review to keep track of your progress. Evaluate each topic and ask yourself what you need to do next to improve. You can rate your understanding of each topic on a scale of 1 to 10 for a more objective view.
- Take note of any unique or essential patterns you encounter as you learn DSA, such as brute force and efficient solutions, boundary conditions, time and space complexity analysis, and coding style.
- Instead of trying to solve a lot of problems, focus on the top 150 coding interview questions in the beginning. Choose some well-known coding problems and try to solve them using different techniques.
- Visualize critical operations, implementations, and use cases for each data structure to improve your understanding.
- Don't hesitate to revisit the same concepts and problems multiple times. This can help you uncover hidden ideas. Focus on understanding the reasoning behind things rather than trying to memorize everything.
Tips for cracking the coding interview
To ace your coding interviews, having a well-defined strategy is crucial. It not only boosts your confidence but also gives you an edge over the competition. Here are some key tips to remember:
- Write down your thoughts and visualize the problem on paper. Create test cases and write pseudocode to map out your solution.
- Don't focus on designing the optimal solution right away. Start with a brute force approach and refine it.
- Communication is key. Keep the interviewer in the loop on your approach and any challenges you face.
- Remember that you're working as part of a team to solve the problem. Collaboration skills are just as important as technical skills.
- Don't get discouraged if you get stuck. The interviewer wants to see how you approach problem-solving.
- Think out loud and explain your thought process. The interviewer may provide hints based on your approach.
- Look for popular problem-solving patterns and apply them as needed.
- Use descriptive variable names and follow good coding practices.
- Test your solution thoroughly, including edge cases.
A final motivation!
Mastering data structures and algorithms require a long-term vision, hard work, and consistency. Keep in mind this inspiring quote: "If the mind is weak, then the situation becomes a problem; if the mind is balanced, then the situation becomes a challenge. But if the mind is strong, then the situation becomes an opportunity."
Learning and coding can be enjoyable, so it's essential to embrace the process and have fun! With the right mindset and dedication, you can succeed in your DSA learning journey and make the most of the opportunities that come your way. Best of luck!
If you have any queries/doubts/feedback, please write us at [email protected] . Enjoy learning, enjoy coding, enjoy algorithms!
Share Your Insights
Don’t fill this out if you’re human:
More from EnjoyAlgorithms
Self-paced courses and blogs, coding interview, machine learning, system design, oop concepts, our newsletter.
Subscribe to get well designed content on data structure and algorithms, machine learning, system design, object orientd programming and math.
©2023 Code Algorithms Pvt. Ltd.
All rights reserved.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, learn ds & algorithms.
A computer program is a collection of instructions to perform a specific task. For this, a computer program may need to store data, retrieve data, and perform computations on the data.
A data structure is a named location that can be used to store and organize data. And, an algorithm is a collection of steps to solve a particular problem. Learning data structures and algorithms allow us to write efficient and optimized computer programs.
Our DSA tutorial will guide you to learn different types of data structures and algorithms and their implementations in Python, C, C++, and Java.
Do you want to learn DSA the right way? Enroll in our Interactive DSA Course for FREE.
- Introduction
Data Structures (I)
Data structures (ii), tree based dsa (i), tree based dsa (ii), graph based dsa.
- Sorting and Searching
Greedy Algorithms
- Dynamic Programming
Other Algorithms
- How to learn DSA?
DSA Introduction
- What is an algorithm?
- Data Structure and Types
- Why learn algorithms?
- Asymptotic Notations
- Master Theorem
- Divide and Conquer Algorithm
- Types of Queue
- Circular Queue
- Priority Queue
- Linked List
- Linked List Operations
- Types of Linked List
- Heap Data Structure
- Fibonacci Heap
- Decrease Key and Delete node from Fibonacci Heap
- Tree Data Structure
- Tree Traversal
- Binary Tree
- Full Binary Tree
- Perfect Binary Tree
- Complete Binary Tree
- Balanced Binary Tree
- Binary Search Tree
- Insertion into B-tree
- Deletion from B-tree
- Insertion on a B+ Tree
- Deletion from a B+ Tree
- Red Black Tree
- Insertion in Red Black Tree
- Deletion from Red Black Tree
- Graph Data Structure
- Spanning Tree
- Strongly Connected Components
- Adjacency Matrix
- Adjacency List
- DFS Algorithm
- Breadth-first Search
- Bellman Ford's Algorithm
Sorting and Searching Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Counting Sort
- Bucket Sort
- Linear Search
- Binary Search
- Greedy Algorithm
- Ford-Fulkerson Algorithm
- Dijkstra's Algorithm
- Kruskal's Algorithm
- Prim's Algorithm
- Huffman Code
- Floyd Warshall Algorithm
- Longest Common Subsequence
- Backtracking Algorithm
- Rabin-Karp Algorithm
Why Learn DSA?
- Write optimized and scalable code - Once you have knowledge about different data structures and algorithms, you can determine which data structure and algorithm to choose in various conditions.
- Effective use of time and memory - Having knowledge about data structures and algorithms will help you write codes that run faster and require less storage.
- Better job opportunities - Data structures and algorithms questions are frequently asked in job interviews of various organizations including Google, Facebook, and so on.
How you can learn data structure and algorithms?
Interactive dsa course.
Want to learn DSA with Python by solving quizzes and challenges after learning each concept? Enroll in our DSA Interactive Course for FREE.
Learn DSA from Programiz
Programiz offers a complete series of easy to follow DSA tutorials along with suitable examples. These tutorials are targeted for absolute beginners who want to dive into the field of computer programming.
Learn DSA from Books
Learning from books is always a good practice. You will get the big picture of programming concepts in the book which you may not find elsewhere.
Here are some books we personally recommend.
- Introduction to Algorithms, Thomas H. Cormen - it is one of the best books in algorithms and covers a broad range of algorithms in-depth
- Algorithms, Robert Sedgewick - it is the leading textbook on algorithms and is widely used in colleges and universities
- The Art of Computer Programming, Donald E. Knuth - this book is considered best if you know the subject and are looking for deeper understanding
Learn DSA through visualization
Once you have some idea about data structure and algorithms, there is a great resource at Data Structure Visualizations that lets you learn through animation.
Data structures and algorithms study cheatsheets for coding interviews
What is this .
This section dives deep into practical knowledge and techniques for algorithms and data structures which appear frequently in algorithm interviews. The more techniques you have in your arsenal, the higher the chances of passing the interview. They may lead you to discover corner cases you might have missed out or even lead you towards the optimal approach!
Contents of each study guide
For each topic, you can expect to find:
- A brief overview
- Learning resources
- Language-specific libraries to use
- Time complexities cheatsheet
- Things to look out for during interviews
- Corner cases
- Useful techniques with recommended questions to practice
Study guides list
Here is the list of data structures and algorithms you should prepare for coding interviews and their corresponding study guides:
General interview tips
Clarify any assumptions you made subconsciously. Many questions are under-specified on purpose.
Always validate input first. Check for invalid/empty/negative/different type input. Never assume you are given the valid parameters. Alternatively, clarify with the interviewer whether you can assume valid input (usually yes), which can save you time from writing code that does input validation.
Are there any time/space complexity requirements/constraints?
Check for off-by-one errors.
In languages where there are no automatic type coercion, check that concatenation of values are of the same type: int / str / list .
After finishing your code, use a few example inputs to test your solution.
Is the algorithm meant to be run multiple times, for example in a web server? If yes, the input is likely to be preprocess-able to improve the efficiency in each call.
Use a mix of functional and imperative programming paradigms:
- Write pure functions as much as possible.
- Pure functions are easier to reason about and can help to reduce bugs in your implementation.
- Avoid mutating the parameters passed into your function especially if they are passed by reference unless you are sure of what you are doing.
- However, functional programming is usually expensive in terms of space complexity because of non-mutation and the repeated allocation of new objects. On the other hand, imperative code is faster because you operate on existing objects. Hence you will need to achieve a balance between accuracy vs efficiency, by using the right amount of functional and imperative code where appropriate.
- Avoid relying on and mutating global variables. Global variables introduce state.
- If you have to rely on global variables, make sure that you do not mutate it by accident.
Generally, to improve the speed of a program, we can either: (1) choose a more appropriate data structure/algorithm; or (2) use more memory. The latter demonstrates a classic space vs. time tradeoff, but it is not necessarily the case that you can only achieve better speed at the expense of space. Also, note that there is often a theoretical limit to how fast your program can run (in terms of time complexity). For instance, a question that requires you to find the smallest/largest element in an unsorted array cannot run faster than O(N).
Data structures are your weapons. Choosing the right weapon for the right battle is the key to victory. Be very familiar about the strengths of each data structure and the time complexities for its various operations.
Data structures can be augmented to achieve efficient time complexities across different operations. For example, a hash map can be used together with a doubly-linked list to achieve O(1) time complexity for both the get and put operation in an LRU cache .
Hash table is probably the most commonly used data structure for algorithm questions. If you are stuck on a question, your last resort can be to enumerate through the common possible data structures (thankfully there aren't that many of them) and consider whether each of them can be applied to the problem. This has worked for me sometimes.
If you are cutting corners in your code, state that out loud to your interviewer and say what you would do in a non-interview setting (no time constraints). E.g., I would write a regex to parse this string rather than using split() which may not cover all cases.
Recommended courses
Algomonster .
AlgoMonster aims to help you ace the technical interview in the shortest time possible . By Google engineers, AlgoMonster uses a data-driven approach to teach you the most useful key question patterns and has contents to help you quickly revise basic data structures and algorithms. Best of all, AlgoMonster is not subscription-based - pay a one-time fee and get lifetime access . Join today for a 70% discount →
Grokking the Coding Interview: Patterns for Coding Questions
This course on by Design Gurus expands upon the questions on the recommended practice questions but approaches the practicing from a questions pattern perspective, which is an approach I also agree with for learning and have personally used to get better at coding interviews. The course allows you to practice selected questions in Java, Python, C++, JavaScript and also provides sample solutions in those languages along with step-by-step visualizations. Learn and understand patterns, not memorize answers! Get lifetime access now →
Master the Coding Interview: Data Structures + Algorithms
This Udemy bestseller is one of the highest-rated interview preparation course (4.6 stars, 21.5k ratings, 135k students) and packs 19 hours worth of contents into it. Like Tech Interview Handbook, it goes beyond coding interviews and covers resume, non-technical interviews, negotiations. It's an all-in-one package! Note that JavaScript is being used for the coding demos. Check it out →
Table of Contents
- What is this
- Contents of each study guide
- Study guides list
- General interview tips
- Recommended courses

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
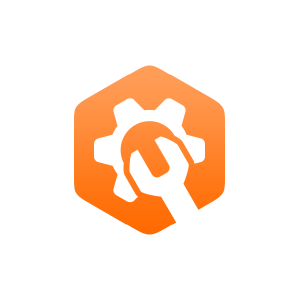
13.2: Random numbers
- Last updated
- Save as PDF
- Page ID 40802
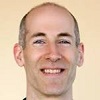
- Allen B. Downey
- Olin College via Green Tea Press
Given the same inputs, most computer programs generate the same outputs every time, so they are said to be deterministic . Determinism is usually a good thing, since we expect the same calculation to yield the same result. For some applications, though, we want the computer to be unpredictable. Games are an obvious example, but there are more.
Making a program truly nondeterministic turns out to be difficult, but there are ways to make it at least seem nondeterministic. One of them is to use algorithms that generate pseudorandom numbers. Pseudorandom numbers are not truly random because they are generated by a deterministic computation, but just by looking at the numbers it is all but impossible to distinguish them from random.
The random module provides functions that generate pseudorandom numbers (which I will simply call “random” from here on).
The function random returns a random float between 0.0 and 1.0 (including 0.0 but not 1.0). Each time you call random , you get the next number in a long series. To see a sample, run this loop:
The function randint takes parameters low and high and returns an integer between low and high (including both).
To choose an element from a sequence at random, you can use choice :
The random module also provides functions to generate random values from continuous distributions including Gaussian, exponential, gamma, and a few more.
Exercise \(\PageIndex{1}\)
Write a function named choose_from_hist that takes a histogram as defined in Section 11.2 and returns a random value from the histogram, chosen with probability in proportion to frequency. For example, for this histogram:
your function should return 'a' with probability 2/3 and 'b' with probability 1/3.

Important DSA Topics for Interviews: A Comprehensive Guide

Data Structures and Algorithms (DSA) are the building blocks of computer science and play a pivotal role in technical interviews for software development positions. A strong grasp of DSA concepts is essential for solving complex problems efficiently and effectively. In this article, we will delve into the most important DSA topics that you should be well-prepared for when facing technical interviews.
Data Structures
Arrays are one of the simplest and most fundamental data structures. They consist of a collection of elements, each identified by an index or a key. Understanding array manipulation, searching, and sorting algorithms is crucial.
2. Linked Lists
Linked lists are a linear data structure consisting of nodes connected by pointers. There are various types of linked lists, such as singly linked, doubly linked, and circular linked lists. Knowledge of linked list operations, reversal, and traversal is vital.
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. You should understand stack operations, including push, pop, and peek, and their applications in solving problems.
Queues are another linear data structure but follow the First-In-First-Out (FIFO) principle. Mastery over queue operations, such as enqueue and dequeue, is essential.
5. Trees (Binary Trees, Binary Search Trees)
Trees are hierarchical data structures with nodes connected by edges. Binary trees and binary search trees (BSTs) are particularly important. Understanding tree traversal, insertion, and deletion operations is critical.
Graphs are versatile data structures with nodes and edges that model various real-world scenarios. You should be familiar with graph representations and algorithms like Breadth-First Search (BFS) and Depth-First Search (DFS).
7. Hash Tables
Hash tables are used for efficient data retrieval. Knowledge of hash functions, collision handling, and the time complexity of operations is essential.
8. Heaps (Priority Queues)
Heaps are specialized trees used for priority-based operations. Understanding heap properties, operations, and their applications in sorting algorithms is crucial.
9. Hash Sets and Hash Maps
Hash sets and hash maps provide fast data access. Comprehending their internal workings and use cases is vital.
Tries are tree-like structures used for efficient string operations. Understanding trie construction, insertion, and search is important, especially in text-processing applications.
11. Segment Trees
Segment trees are used for efficient range queries on arrays. Mastery of construction and query operations is necessary for solving problems involving intervals.
12. Fenwick Trees (Binary Indexed Trees)
Fenwick trees are used for efficient prefix sum calculations. You should know how to construct and update Fenwick trees for solving problems like range sum queries.
13. Disjoint-Set (Union-Find) Data Structure
Disjoint-set data structures are used for solving problems involving connected components and dynamic connectivity.
1. Sorting Algorithms (e.g., QuickSort, MergeSort)
Sorting algorithms are fundamental. Understanding QuickSort, MergeSort, and their time complexities is crucial.
2. Searching Algorithms (e.g., Binary Search)
Binary search is an efficient searching algorithm. You should know how to apply it to sorted arrays.
3. Breadth-First Search (BFS) and Depth-First Search (DFS)
BFS and DFS are graph traversal algorithms with various applications, such as finding connected components and shortest paths.
4. Dynamic Programming
Dynamic programming is a problem-solving technique used for optimization problems. You should be able to recognize and formulate problems that can be solved using dynamic programming.
5. Greedy Algorithms
Greedy algorithms make locally optimal choices to find a global optimum. Understanding greedy strategies and their applications is essential.
6. Shortest Path Algorithms (e.g., Dijkstra’s Algorithm)
Shortest path algorithms are crucial for finding the shortest route in a graph. Dijkstra’s algorithm is a commonly used method.
7. Minimum Spanning Tree Algorithms (e.g., Kruskal’s Algorithm)
Minimum spanning tree algorithms are used for network design and optimization problems. Kruskal’s algorithm is an important choice.
8. Topological Sorting
Topological sorting is used in directed acyclic graphs (DAGs) to find a linear ordering of nodes.
9. Divide and Conquer
Divide and conquer is a problem-solving technique that divides a problem into subproblems, solves them independently, and combines their solutions.
10. Backtracking
Backtracking is a technique for finding all possible solutions to a problem through systematic exploration of different paths.
11. Sliding Window Technique
The sliding window technique is used to efficiently process arrays or strings in a window of fixed size.
12. Two-Pointer Technique
The two-pointer technique involves using two pointers to traverse data structures like arrays or linked lists efficiently.
13. Bit Manipulation
Bit manipulation is essential for handling binary representations of data and optimizing code.
14. Morris Traversal for Trees
Morris traversal is an efficient way to traverse binary trees without using extra memory.
15. KMP (Knuth-Morris-Pratt) Algorithm for Pattern Matching
The KMP algorithm is used for efficient pattern searching in strings.
16. Rabin-Karp Algorithm for String Matching
Rabin-Karp is another string matching algorithm that uses hashing techniques for pattern matching.
17. Floyd-Warshall Algorithm for All-Pairs Shortest Paths
The Floyd-Warshall algorithm is used to find the shortest paths between all pairs of nodes in a graph.
In conclusion, a solid understanding of these DSA topics is essential for excelling in technical interviews for software development positions. Practice, problem-solving, and continuous learning are key to mastering these concepts and becoming a successful software engineer. Remember that interviews often require not only knowledge but also the ability to apply these concepts to real-world problems effectively.
What's your reaction?
Magnificent beat ! I wish to apprentice at the same time as you amend your website, how could i subscribe for a weblog web site? The account helped me a appropriate deal. I were a little bit acquainted of this your broadcast provided shiny transparent idea
Leave a comment Annuler la réponse
Enregistrer mon nom, mon e-mail et mon site dans le navigateur pour mon prochain commentaire.
By using this form you agree with the storage and handling of your data by this website. *
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
dsa-practice
Here are 950 public repositories matching this topic..., prince-1501 / complete-dsa-preparation.
This is A complete DSA preparation Course. A DSA self-paced course for ultimate Interview and Placement Preparation
- Updated Dec 26, 2023
Abhay5855 / Mission-frontend
A curated list of Javascript, React, Machine coding problems, Pattern questions, basic backend knowledge and fundamentals questions all in one.
- Updated Apr 14, 2024
Mugdha-Hazra / 180-Questions-Striver-SDE-sheet-in-CPP
If you are a newbie in coding and wanna do CP then first solve this DSA question then move to cp..it will surely gonna help u clear the concepts. and get better at cp.
- Updated Oct 19, 2023
hiimvikash / DSA-EndGame
I have started Data structures and Algorithms on April 1, 2021, and this repository will be containing my resources, tutorial, codes, and my approach to Qs, for future reference. As I'm in the learning process, this repository will be refreshed daily with my new bits of knowledge.
- Updated Oct 3, 2023
Liopun / leet-chatgpt-extension
AI-powered browser extension that enhances your leetcode and hacker-rank experience.
- Updated Mar 30, 2024
Supsource / 90DaysDSA
Nishant bhaiya's 90DaysDSA challenge.
- Updated Jan 12, 2024
Jatin-Shihora / LeetCode-Solutions
This are the Collections of LeetCode , GFG questions that I have solved till now!! [Note: This repository gets updated daily with new questions]
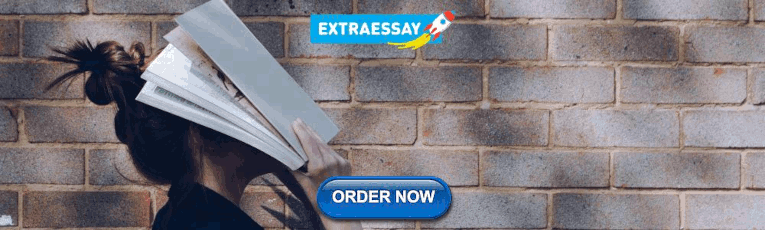
piyush-eon / dsa-with-javascript-course
Course Files for Data Structures and Algorithm Course with Javascript - By RoadsideCoder
- Updated Jan 21, 2024
avinash201199 / DSA-Resources
Data Structures and Algorithms Resources
- Updated Jun 15, 2023
Codechef-WCE-Chapter / 30-Days-6-Companies
30 Days 6 Companies Challenge
- Updated Mar 14, 2023
utkarsh006 / LeetCode-Grind
This Repo is a one way solution to ace your Technical Interviews and crack your dream Job by upsolving LeetCode standard problems.
- Updated Feb 10, 2024
kishanrajput23 / Love-Babbar-CPP-DSA-Course
This repository consists of the code samples, assignments, and notes for the C++ DSA Course of CodeHelp - Love Babbar.
- Updated Apr 23, 2024
radojicic23 / dsa-for-absolute-dummies
This repository is place where you can learn about data structures and algorithms if you want, but don't play to much with it because it's too hard.
- Updated Jul 24, 2023
rajeevrpandey / Coding-Ninjas-Solutions-CPP
Solutions of the Practice problems, Assignment problems and Test problems in DSA course in C++ of Coding Ninjas
- Updated Feb 21, 2024
akshat-fsociety / Data-Structures-Algorithms
Data Structures & Algorithms 💥
- Updated Sep 1, 2022
david-kariuki / ace-the-java-coding-interview
Java project to learn advanced Data Structures and Algorithms
- Updated Apr 16, 2023
shivaamm / Coding-Practice
Learning DSA - From 100DaysOfCode challenge to Placement Preparation:) [ C++]
- Updated Apr 19, 2024
hoshiyarjyani / Masai-School-Assignments
Essential for fresher developers and job seekers! This repository contains 2000+ Masai School DSA questions and Java Related Important Concepts. Master these to excel in interviews and boost your skills. Don't forget to star this repository!
- Updated Sep 1, 2023
DitijTanwar29 / DSA_SUPREME_2.0_BATCH_HANDWRITTEN_NOTES
You will get all live lecture handwritten notes of supreme 2.0 batch. Star this repository for future reference! You can connect with me through my LinkedIn -> https://www.linkedin.com/in/ditij-tanwar-99b881250/
- Updated Nov 5, 2023
abhiiishek07 / 180DSA
180dsa is a web app tracker based on sde sheet by Striver.
- Updated May 10, 2023
Improve this page
Add a description, image, and links to the dsa-practice topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the dsa-practice topic, visit your repo's landing page and select "manage topics."
For enquiries call:
+1-469-442-0620

- Programming
Data Structures and Algorithms (DSA) with Java
Home Blog Programming Data Structures and Algorithms (DSA) with Java

Data structures and algorithms are the building blocks of effective software in computer science and programming. Understanding these foundational concepts is crucial for solving complex problems and creating high-performance applications.
In this blog, we delve into the world of data structures and algorithms, exploring their importance, discussing various applications and implementation methods, and demonstrating how they can be used to create exceptional software solutions. You can go for the best programming certification course to enhance your programming skills.
What is Data Structure?
A data structure is a method for organizing and storing data in a computer's memory to enable quick access to and effective data manipulation. The link between the data, the actions that may be carried out on the data, and the algorithms that can be applied to carry out those operations are all specified by a data structure.
Among other famous data structures, there are arrays, linked lists, stacks, queues, trees, and graphs. Understanding data structures is crucial for creating compelling and optimized software since choosing the right data structure for a given task can have a big impact on how well a code performs.
What is an Algorithm?
An algorithm is a detailed set of instructions or processes that describe how to carry out a given activity or solve a particular problem in computer science. Calculations, data processing, and automated reasoning are just a few of the tasks that algorithms are used for.
They offer a methodical, logical approach to problem-solving that makes it possible to develop effective, optimal solutions. An algorithm needs to be able to handle a variety of input data and provide the correct result in a variety of situations to be effective.
Sorting algorithms, search algorithms, and graph algorithms are a few examples of algorithms that are frequently used. Software engineers need to understand algorithms to design dependable and effective code.
Learn different Data Structures and understand all the well-known algorithms and their implementation with Data Structure online certificate course and set a strong base for programming to solve complex problems faced in software industries, efficiently and optimally.
Data Structure and Algorithm Projects
Here are some of the best projects for data structures and algorithms that may be used to practice programming and gain practical expertise. These projects offer an engaging journey of learning and problem-solving, covering a wide range of subjects, from basic data structures to cutting-edge algorithms.
Cash Flow Minimizer (Graphs, Heaps, and Multisets)
The Cash Flow Minimizer projects based on data structures and algorithms to the problem of cash flow optimization by using graphs, heaps, and multisets. The project's objective is to determine the smallest number of transactions necessary to settle the debts between a group of people.
The project employs a graph data format to represent both the vertices—persons themselves—and the edges—debts between individuals. The program then uses the idea of heaps to determine which people have the highest and lowest debt loads. The procedure then generates transactions between these two parties, updates the debts, and continues until all debts are paid.
To keep track of transactions and their sums, the project also uses multisets, a data structure that enables storing numerous instances of the same information. The multisets assist in determining the least number of transactions necessary to pay off the debts.
The Cash Flow Minimizer project serves as an example of the power and adaptability of DSA in dealing with practical issues. The project is especially helpful in situations where it is difficult to determine the minimum number of transactions necessary to settle the debts between a group of people.
Cash Flow Minimizer Code Snippets
Map Navigator (Dijkstra’s Algorithm)
The Map Navigator project, based on data structures and algorithms, is an advanced mapping application that utilizes an improved version of Dijkstra's algorithm to determine the shortest route between two points on a map. By employing cutting-edge technology and innovative approaches, the Map Navigator ensures fast and accurate navigation results for users.
In this project, the map is initially visualized as a graph, with each place acting as a node and the distance between them as an edge. The graph is then subjected to Dijkstra's algorithm to determine the shortest route between the two points.
As it moves along the graph, the algorithm continuously updates the distances it has tentatively assigned to each site on the map. The computer then chooses the site with the shortest tentative distance and examines the areas around it, adjusting the tentative distances of those areas as necessary. This step is repeated by the algorithm until it reaches the desired place, and the shortest path is found.
Map Navigator Code Snippet
Sudoku Solver (Backtracking)
Sudoku solver mini projects on data structures and algorithms use the backtracking algorithm to resolve Sudoku puzzles. Sudoku is a logic-based number puzzle game that requires you to fill in a 9x9 grid with numbers from 1 to 9, with no repetitions allowed in the rows, columns, or 3x3 subgrids.
The first step in the Sudoku Solver project is to draw the puzzle as a 9x9 grid and fill it in with the specified numbers. The puzzle is then solved using the backtracking approach by iteratively trying out various values in each cell until a solution is discovered.
When the backtracking algorithm runs into a dead end, that is, when it comes into a contradiction that renders the problem impossible to solve, it will turn around and try another possible solution for each cell in the grid. Once a viable answer is found, the algorithm goes back and tests different values in the earlier cells.
The Sudoku Solver data structures and algorithms projects in Python show how the backtracking algorithm can be used in real-world situations to solve challenging puzzles like Sudoku. It is beneficial for puzzle fans, game creators, and anybody else interested in logic-based problem-solving.
Sudoku Solver Code Snippet
Snakes Game (Arrays)
The project "Snake's Game" is an excellent example of a project for data structures and algorithms. It utilizes arrays to implement the well-known Snake game, where a user-controlled snake moves around a grid, consuming food to grow while avoiding collisions with walls and its own body. This project not only offers an enjoyable gaming experience but also demonstrates the application of data structures and algorithms in game development.
The grid is first represented as a 2D array in the Snakes Game project, along with the snake's beginning position and direction. The user can then use the arrow keys to direct the snake during the game's looping portion. In the following loop iterations, the snake's movement is updated, and arrays are used to check for collisions with the walls and the snake's body.
The arrays are used to maintain track of the locations of the food and the placements of the snake's body parts. The snake expands by one segment as it consumes the meal, updating the array of its body segments. The game ends when the snake slams into the walls or its own body.
The Snakes Game project shows how arrays can be used in real-world settings to design a well-known game. It is helpful for those who want to learn how to use arrays in game creation as well as for game creators themselves.
Snake Game Code Snippet
File Zipper (Greedy Huffman Encoder)
The File Zipper project, specifically developed for data structures and algorithms projects in C++ source code, utilizes the well-known lossless data compression algorithm Greedy Huffman Encoder technique. This project aims to compress files by minimizing their size.
The Greedy Huffman Encoder technique works by allocating shorter codes to characters that are used more frequently in a file and longer codes to characters that are used less frequently. By implementing this algorithm in C++, the project showcases how data structures and algorithms can be utilized to optimize file compression and achieve efficient storage.
The first step in the File Zipper project is to read data from a file and make a frequency table of the appearances of each character. The Huffman tree is created using the frequency table, and each leaf node represents a character. The code for each leaf node is obtained from the path from the root to the leaf node.
The data is then encoded using the Huffman tree by swapping out each character with its appropriate code, creating a compressed file. The decoded data is then written to a new file along with the necessary data from the Huffman tree.
File Zipper Code Snippet
Graphical Calculator
The Graphical Calculator project makes use of a variety of data structures and algorithms to construct a calculator that can handle mathematical expressions and display the results in a graphical user interface (GUI).
The Shunting Yard Algorithm is used to first parse the user's mathematical expression in the Graphical Calculator project. The approach changes the expression's notation from infix to postfix, which can be quickly evaluated using a stack data structure.
The postfix expression is then evaluated by the project using a binary expression tree. The postfix expression is parsed to create a tree where each operator is a node, and its operands are its children. This creates the binary expression tree. The expression is then evaluated by moving up the tree starting at the root node.
The user-entered mathematical expression and the outcome of the expression assessment are displayed in the Graphical User Interface (GUI) of the Graphical Calculator project. Additionally, it has a number of buttons for addition, subtraction, multiplication, and division in mathematics.
Graphical Calculator Code Snippet
Terminal Shell
A project called Terminal Shell implements a command-line interface for an operating system on a computer project using data structures and algorithms. Through a text-based interface, the Terminal Shell project enables users to carry out numerous commands, explore the file system, and carry out other tasks.
Implementing a command parser that can recognize and carry out user-inputted commands is the first step in the Terminal Shell project. To quickly find and carry out commands, the command parser employs a variety of data structures and methods, including hash tables and binary trees.
A file system navigator is also implemented in the project, enabling users to move around the file system and carry out operations on files like creating, reading, writing, and deleting files and directories. The file system navigator effectively searches and navigates the file system using a variety of data structures and techniques, including linked lists and trees.
The Terminal Shell project also has features that improve the user experience and boost productivity, like command history, command autocompletion, and input/output redirection.
Terminal Shell Code Snippet
Web Crawler
This project automates the process of gathering data from the World Wide Web by using data structures and algorithms. The goal of the Web Crawler project, specifically designed for data structures and algorithms projects in Java, is to create a complete library of information by crawling through websites and extracting pertinent data, such as links, photos, and text.
The implementation showcases how Java, combined with the right data structures and algorithms, can efficiently handle web scraping tasks and organize the collected data.
The project begins by locating the root URL and storing the URLs of the pages to be viewed in a queue data structure. It then uses a variety of data structures and techniques, including graphs and depth-first search, to systematically browse the web and crawl through pages.
For the project's acquired data to be accurate, timely, and simple to process, other capabilities like web page filtering, duplicate identification, and database storage are also included.
Web Crawler Code Snippet
Benefits of DSA Projects
Hands-on Experience: data structure and algorithm projects give applicants the chance to put their theoretical understanding of data structures and algorithms to use in real-world situations. Candidates improve their comprehension and competency of these ideas by developing and optimizing data structures and algorithms while working on real-world projects.
Problem-solving Abilities: Candidates for DSA projects must be able to analyze difficult issues and come up with workable solutions. Their ability to think critically, dissect issues into smaller parts, and create efficient algorithms is strengthened as a result of this process. These abilities are transferable and extremely helpful in a variety of software development fields.
Code Optimization: Candidates frequently run into performance issues with DSA projects that can be fixed with code optimization. They gain knowledge of methods for evaluating and enhancing the performance of their algorithms and data structures, leading to quicker and more resource-effective solutions. The industry values code optimization as a highly specialized expertise.
Practical Application of Concepts: DSA projects give students a realistic setting in which to apply the theories they have acquired from books or online courses . Candidates get a first-hand look at how various data structures and algorithms work to solve specific issues or boost system performance. Their knowledge is strengthened, and the significance of these ideas is emphasized by this applied understanding.
Language Proficiency: DSA projects give applicants the chance to advance their knowledge of a particular programming language. Candidates gain a deeper understanding of a chosen language's grammar, libraries, and best practices by implementing data structures and algorithms in it. Their general programming skills are improved, and they become more adaptable developers as a result of this proficiency.
Collaboration and Teamwork: DSA projects frequently entail teamwork while replicating actual development settings. Candidates can develop their teamwork, communication, and idea-sharing skills. This encourages cooperation abilities and exposes applicants to various viewpoints and problem-solving techniques, improving their capacity to work well in a team.
Portfolio Enhancement: DSA projects give applicants concrete proof of their abilities and accomplishments. These projects, showcasing their use of data structures and algorithms in practice, might be displayed in a portfolio or presented during interviews. A candidate's reputation and competitiveness in the job market can be greatly improved by a solid portfolio of DSA initiatives.
Self-confidence and Motivation: Candidates get a sense of satisfaction and an increase in self-confidence after finishing DSA projects successfully. It confirms their enthusiasm for programming and proves their capacity to solve challenging challenges. This drive promotes further education and professional development in the area of data structures and algorithms.
In computer science, data structures and algorithms (DSA) are crucial for methodical problem-solving and program optimization. They comprise a variety of methods and structures, including sorting algorithms and graphs, heaps, and trees. Projects for data structures and algorithms help you learn its usage in real-world situations.
Learning DSA gives you a competitive edge in the job market and technical interviews by enhancing your problem-solving, programming performance, code management , and debugging skills. Developers must learn and apply DSA because it is necessary for creating an efficient, scalable, and ideal program. KnowledgeHut Java Programming certification will help you supplement theoretical learning with practical exercises.
Frequently Asked Questions (FAQs)
Image compression, text editors, network routing, social network analysis, and many other project ideas can all be produced using DSA. The greatest idea of projects on algorithms and data structures will rely on your interests, level of expertise, and the issue you wish to address.
In many facets of our daily life, including search engines, GPS navigation, online commerce, and social media, algorithms and data structures are used. For instance, search engines use algorithms like binary search and data structures like hash tables to deliver swift and precise results. Dijkstra's shortest path method and other algorithms are used by GPS navigation systems to identify the fastest path between two sites. Online stores utilize algorithms like sorting and searching to make it simple and quick for shoppers to find things.
There are many well-known data structure algorithms, such as the following:
- Sorting algorithms: bubble sort, insertion sort, merge sort, quicksort, and heapsort.
- Search techniques include depth-first search, binary search, and linear search.
- Dijkstra's algorithm, the Bellman-Ford method, the Kruskal algorithm, and the Prim algorithm are examples of graph algorithms.
- Tree algorithms: red-black tree, binary search tree, and AVL tree.

KnowledgeHut .
KnowledgeHut is an outcome-focused global ed-tech company. We help organizations and professionals unlock excellence through skills development. We offer training solutions under the people and process, data science, full-stack development, cybersecurity, future technologies and digital transformation verticals.
Avail your free 1:1 mentorship session.
Something went wrong
Upcoming Programming Batches & Dates

Browser does not support script.
- Working paper series
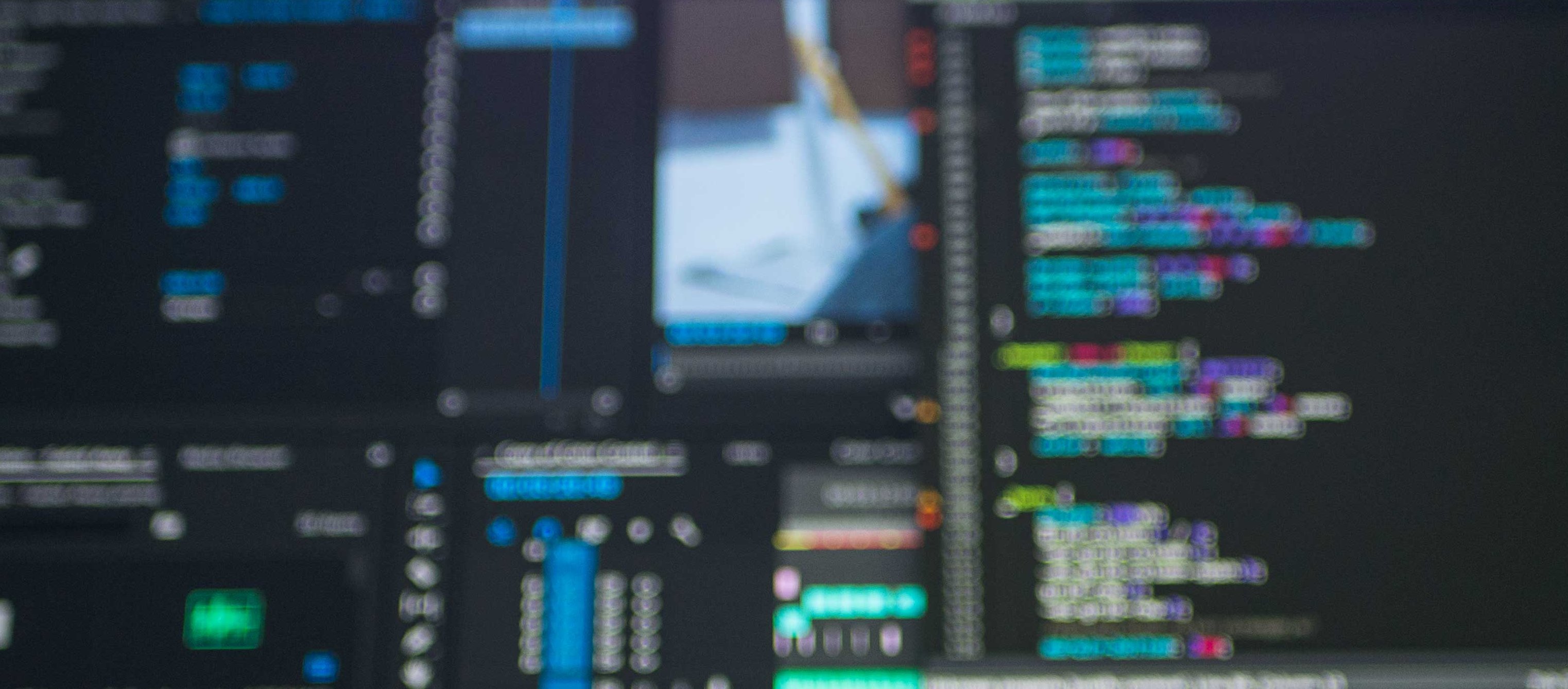
Short Course on the EU Digital Services Act (12, 15, 16, 18 April 2024)
The Short Course on the EU Digital Services Act i s a 16-hour executive education course, which was most recently delivered in four online sessions in September 2023. Past participants were from national regulators, technology companies, law firms, funding agencies, and civil society organisations.
The next course will run in April 2024.
Course organiser : Dr Martin Husovec, LSE Law School, Email: [email protected] .
Course manager: Amanda Tinnams, LSE Law School, Email: [email protected] .
Aims, target audience, structure and timing
- To provide a systematic overview of the main components and individual obligations in the EU Digital Service Act (DSA).
- To explore in-depth case studies of the most relevant and cutting-edge issues of the DSA, including those that are likely to be litigated.
- To discuss practical strategies for compliance with DSA’s content moderation rules and other due diligence obligations.
Target audience
- Practitioners, in-house lawyers, and civil servants across the world who work on the regulation of digital services, including content moderation, and want to prepare for the DSA’s entry into force.
- Professionals, scholars, and students across the world who are familiar with the regulation of digital services and wish to gain in-depth knowledge of the key DSA issues.
Structure and delivery
- Four 4-hour sessions in April 2024 (12 April, 15 April, 16 April, 18 April), all 1.30pm-5.30pm GMT
- Capacity: the short course will be capped at 30 students to maximise interaction.
- Location: the course will be delivered on Zoom.
- Format: lectures with in-class discussions and case studies
- DSA’s scope, components, and broader regulatory context
- DSA’s liability chapter (liability exemptions and injunctions)
- DSA’s due diligence obligations chapter (content moderation and fairness, transparency, advertising, and compliance)
- DSA’s enforcement chapter
Academic staff
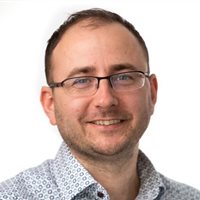
Dr Martin Husovec is an Associate Professor of Law at the London School of Economics and Political Science (LSE). His scholarship deals with questions of innovation policy and digital liberties, in particular, regulation of intellectual property and freedom of expression.
He is the author of an upcoming book, The Principles of Digital Services Act (Oxford University Press, forthcoming in August 2024).
He is also the author of the book, Injunctions against intermediaries in the European Union (Cambridge University Press, 2017) and many articles on the regulation of intermediaries, intellectual property enforcement, and digital freedom of expression.
Martin is a member of the European Copyright Society (ECS), a group of prominent European copyright scholars. He is also a co-founder of a think-tank, European Information Society Institute, which acts as amicus curia before the European Court of Human Rights and operates a domain name dispute resolution system for skTLD. He was an advisor to the President of the Slovak Constitutional Court, national ministries across Europe and Asia, and various EU institutions in the areas of intellectual property, freedom of expression and privacy. His work was repeatedly cited by Advocate Generals at the Court of Justice of the European Union.
Day 1 | 12 April 2024 | 1:30pm-5:30pm GMT
Scope and Context
- Digital ecosystem
- DSA’s basic goals and basic outline
- DSA’s regulated services: infrastructure services, platforms, search engines, etc.
- DSA’s basic components: liability chapter, due diligence obligations, and enforcement
- Regulatory context: DSA vs EU law (copyright, P2B regulation, terrorist content); DSA vs national law
Day 2 | 15 April 2024 | 1:30pm-5:30pm GMT
- Liability exemptions: hosting, mere conduit, and caching;
- The situation of search engines.
- Passive and active hosting and platform services.
- Prohibition of general monitoring obligations.
- Liability case studies: online marketplaces, social media, cyber-lockers, search engines, webhosting, services content delivery networks, internet access providers, and others.
- Injunctions and orders of authorities: filtering and other preventive obligations.
- DSA’s enforcement of liability vs accountability obligations
Day 3 | 16 April 2024 | 1:30pm-5:30pm GMT
Accountability
- Accountability under DSA’s due diligence obligations: active vs passive providers, thresholds, and various types of borderline services.
- Basic areas of due diligence obligations: content moderation, fair design, transparency, advertising, and compliance.
- Content moderation rules for hosting services: from notification to statements of reasons, internal appeals, and external out-of-court dispute resolution.
- Content moderation case studies: (1) social networks & hate speech; (2) webhosting & terrorist content; (3) marketplaces & consumer protection; (4) search engines & right to be delisted
- Fairness and transparency, including advertising due diligence obligations.
Day 4 | 18 April 2024 | 1:30pm-5:30pm GMT
Compliance, VLOPs and Enforcement
- Oversight obligations & special rules for VLOPs/VLOSEs
- Competence of national DSCs and the European Commission
- Standardisation and Codes of Conduct
- Case studies for enforcement: (1) social network & hate speech content moderation; (2) video-sharing platform as a VLOP & copyright content moderation, (3) web hosting & terrorist content; (4) app store as a VLOP & child abuse material; (5) search engine as a VLOSE & right to be delisted
- Private enforcement of the DSA obligations
Registration and fees
- For further information, including registration and fees, please contact Amanda Tinnams, Course Manager. Email: [email protected] .
- The registration form is available here.
- On completion of registration and transfer of payment, you will immediately receive a Zoom link and a set of pre-course readings. The readings will consist of curated materials, including legislative text, excerpts from cases, journal articles, and Husovec's unpublished writing on the DSA."
- Fees: £2,500 for companies, and £2,000 for civil society and public authorities. An early booking discount of 20% will be available for those who register and pay until 15 March 2024. If you have any special requirements, please contact Amanda Tinnams.
- The places are allocated on a first come first served basis. If the registration is full, you can ask to be put on the waiting list.
Certificate and CPD points
- A course certificate — “LSE Short Course on the EU Digital Services Act” — will be provided upon completion of the course.
- CPD points can be applied for.
Cancellation policy
Cancellations requested after the participant receives the reading materials are subject to a £300 cancellation fee. Cancellations requested less than four weeks before the commencement of the course incur the following penalties: two to four weeks 50% of the course fee, less than two weeks 100% of the course fee. If written notification is not received and you do not attend, the full course fee will be retained as a cancellation charge.
- DSA Tutorial
- Data Structures
- Linked List
- Dynamic Programming
- Binary Tree
- Binary Search Tree
- Divide & Conquer
- Mathematical
- Backtracking
- Branch and Bound
- Pattern Searching
DSA Guide for GATE CS Exam | Notes, Syllabus, Preparation Strategy
- Asymptotic Analysis of Algorithms Notes for GATE Exam [2024]
- Recurrence Relations Notes for GATE Exam [2024]
- Array Notes for GATE Exam [2024]
- Linked List Notes for GATE Exam [2024]
- Queue Notes for GATE Exam [2024]
- Stack Notes for GATE Exam [2024]
- Hashing Notes for GATE Exam [2024]
- Trees Notes for GATE Exam [2024]
- Graph Data Structure Notes for GATE Exam [2024]
- Binary Heap Notes for GATE Exam [2024]
- Searching and Sorting Algorithm Notes for GATE Exam [2024]
- Recursion Notes for GATE Exam [2024]
- Divide and Conquer Notes for GATE Exam [2024]
- Greedy Algorithm Notes for GATE Exam [2024]
- Dynamic Programming (DP) Notes for GATE Exam [2024]
- Graph-Based Algorithms for GATE Exam [2024]
- Tips to Clear GATE CS Exam [2024]: Road to Success
Previous Year GATE DSA Questions
- Data Structures and Algorithms | Set 1
- Data Structures and Algorithms | Set 2
- Data Structures and Algorithms | Set 3
- Data Structures and Algorithms | Set 4
- Data Structures and Algorithms | Set 6
- Data Structures and Algorithms | Set 7
- Data Structures and Algorithms | Set 8
- Data Structures and Algorithms | Set 9
- Data Structures and Algorithms | Set 10
- Data Structures and Algorithms | Set 11
- Data Structures and Algorithms | Set 12
- Data Structures and Algorithms | Set 13
- Data Structures and Algorithms | Set 14
- Data Structures and Algorithms | Set 15
- Data Structures and Algorithms | Set 16
- Data Structures and Algorithms | Set 17
- Data Structures and Algorithms | Set 18
- Data Structures and Algorithms | Set 19
- Data Structures and Algorithms | Set 20
- Data Structures and Algorithms | Set 21
- Data Structures and Algorithms | Set 22
- Data Structures and Algorithms | Set 23
- Data Structures and Algorithms | Set 24
- Data Structures and Algorithms | Set 25
- Data Structures and Algorithms | Set 26
- Data Structures and Algorithms | Set 27
- Data Structures and Algorithms | Set 28
- Data Structures and Algorithms | Set 29
- Data Structures and Algorithms | Set 30
- Data Structures and Algorithms | Set 31
- Data Structures and Algorithms | Set 32
- Data Structures and Algorithms | Set 33
- Data Structures and Algorithms | Set 34
- Data Structures and Algorithms | Set 35
- Data Structures and Algorithms | Set 36
- Data Structures and Algorithms | Set 37
- Data Structures and Algorithms | Set 38
The GATE (Graduate Aptitude Test in Engineering) Exam is a critical milestone for computer science enthusiasts seeking advanced education or career opportunities. A solid understanding of Data Structures and Algorithms (DSA) is indispensable for success in this exam, as it forms the core of computer science. In this short guide, we’ll explore key aspects to consider while preparing for the GATE Exam in the realm of DSA.
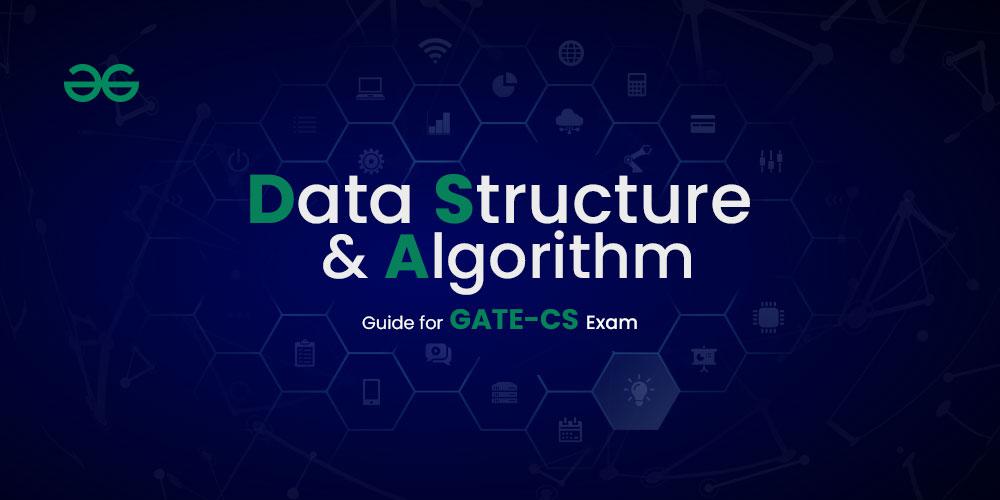
Data Structures and Algorithms (DSA) Guide for GATE-CS Exam
- Why is DSA important for GATE-CS Exam?
Data Structures and Algorithms serve as the backbone of computer science and programming. They are pivotal in designing efficient solutions, optimizing code, and solving complex computational problems. A strong foundation in DSA is not only beneficial for acing the GATE Exam but is also essential for any computer science professional.
Table of Content
- Asymptotic Analysis of Algorithms
- Recurrence Relations
- Graph Data Structure
- Binary Heap
- Searching and Sorting Algorithm
- Divide and Conquer
- Greedy Algorithm
- Dynamic Programming (DP)
- Graph-Based Algorithms
Preparation Strategies for GATE-CS Exam
Gate-cs exam syllabus, faqs related to gate-cs exam, tips for clearing gate-cs exam, key topics to focus on dsa for gate-cs exam.
Here are some key topics to prioritize when preparing for the DSA section in the GATE-CS Exam:
1. Arrays and Strings :
- Understand array manipulation, searching, and sorting.
- Master string manipulation techniques and pattern matching.
2. Linked Lists :
- Learn the concepts of singly and doubly linked lists.
- Practice insertion, deletion, and traversal techniques.
3. Stacks and Queues :
- Grasp the principles of Last In, First Out (LIFO) and First In, First Out (FIFO) structures.
- Understand applications in problem-solving.
4. Trees and Graphs :
- Master binary trees, AVL trees, and graph representations.
- Learn algorithms like DFS (Depth-First Search) and BFS (Breadth-First Search).
5. Sorting and Searching Algorithms :
- Explore various sorting algorithms (QuickSort, MergeSort).
- Understand searching techniques, including Binary Search.
6. Dynamic Programming :
- Develop problem-solving skills using dynamic programming.
- Grasp the concept of breaking down complex problems into overlapping subproblems.
7. Hashing :
- Learn the fundamentals of hashing, hash functions, and collision resolution techniques.
- Understand how hashing is used in solving real-world problems.
8. Graph Algorithms :
- Master algorithms like Dijkstra’s, Kruskal’s, and Prim’s for solving graph-related problems.
- Understand applications in various scenarios.
- Understand the properties of heaps (min heap, max heap).
- Learn how heaps are used in priority queues.
10. Divide and Conquer :
- Grasp the divide-and-conquer paradigm.
- Understand algorithms like MergeSort and QuickSort.
11. Greedy Algorithms :
- Learn the greedy approach for problem-solving.
- Understand how to make locally optimal choices to find a global optimum.
12. Time and Space Complexity :
- Understand Big O notation and analyze the time and space complexity of algorithms.
- Practice evaluating and comparing the efficiency of algorithms.
13. Bit Manipulation :
- Learn bitwise operators and their applications.
- Understand how to perform common operations using bit manipulation.
- Understand the trie data structure.
- Learn its applications, especially in string-related problems.
15. Segment Tree :
- Learn the segment tree data structure.
- Understand its applications in range query problems.
16. Graph Traversal Techniques :
- Master DFS and BFS techniques for traversing graphs.
- Understand their applications in various scenarios.
These key topics cover a broad spectrum of DSA concepts that are commonly tested in the GATE-CS Exam. A thorough understanding of these topics, coupled with consistent practice, will significantly enhance your preparation and performance in the exam. Additionally, solving previous year question papers and participating in mock tests will help you become familiar with the exam pattern and improve your time management skills.
- Utilize standard textbooks and study materials recommended for DSA. Build a strong conceptual foundation through clear explanations and examples.
- Practice solving a variety of problems. Leverage online coding platforms, participate in coding contests, and solve GATE-specific problems.
- Take mock tests and solve previous years’ GATE papers to understand the exam pattern and time management. Analyze your performance to identify weak areas.
- Regularly revise important concepts, algorithms, and problem-solving techniques. Create concise notes for quick reference.
The syllabus is divided into several sections, each covering specific topics. Here’s an overview of the GATE CS syllabus:
1. Engineering Mathematics:
- Mathematical Logic
- Probability
- Set Theory & Algebra
- Combinatorics
- Graph Theory
- Linear Algebra
- Numerical Methods
2. Digital Logic:
- Boolean Algebra
- Minimization
- Combinational and Sequential Circuits
- Number Representation and Computer Arithmetic
3. Computer Organization and Architecture:
- Machine Instructions and Addressing Modes
- Memory Hierarchy: Cache, Main Memory, and Secondary Storage
- Programming Concepts: Control Unit, ALU, Data Path
- Assembly Language Programming and Macros
4. Programming and Data Structures:
- Programming in C
- Arrays, Stacks, Queues, Linked Lists, Trees, Graphs
- Hashing, Heaps
- Searching and Sorting
5. Algorithms:
- Analysis, Asymptotic Notation
- Sorting, Searching, Greedy Algorithms
- Graph Algorithms: BFS, DFS, Shortest Paths
- Minimum Spanning Trees
- NP-Completeness
6. Theory of Computation:
- Regular Languages and Finite Automata
- Context-Free Languages and Pushdown Automata
- Turing Machines
- Undecidability
7. Compiler Design:
- Lexical Analysis
- Syntax Analysis
- Semantic Analysis
- Code Generation
- Optimization Techniques
8. Operating System:
- Processes, Threads, CPU Scheduling
- Memory Management and Virtual Memory
- File Systems, I/O Systems
- Deadlocks, Synchronization
9. Databases:
- Relational Model
- ER Diagrams, Normalization
- SQL, Transactions, Concurrency Control
- Indexing and Hashing
10. Computer Networks:
- OSI and TCP/IP Models
- Network Types, Protocols
- IP Addressing, Subnetting
- Routing Algorithms
- Transport Layer: TCP and UDP
- DNS, HTTP, Email Protocols
11. Web Technologies:
- HTML, CSS, JavaScript
- PHP, XML, Servlets, JSP
- Basics of Cloud Computing and RESTful Web Services
12. Digital Marketing:
- Basics of SEO, SEM, Social Media Marketing
- E-commerce, Analytics
Here are some frequently asked questions (FAQs) about the GATE (Graduate Aptitude Test in Engineering) Computer Science (CS) Exam:
What is GATE CS?
GATE CS is an entrance exam for postgraduate programs in computer science and related fields. It assesses candidates’ understanding of various subjects in computer science and engineering.
Who conducts the GATE CS Exam?
The GATE Exam is conducted jointly by the Indian Institute of Science (IISc) and seven Indian Institutes of Technology (IITs) on behalf of the National Coordination Board (NCB).
What is the eligibility criteria for GATE CS?
Candidates must have a bachelor’s degree in engineering, technology, or science, or be in the final year of their program. There is no age limit.
What is the exam pattern for GATE CS?
GATE CS is a computer-based test consisting of 65 questions for a total of 100 marks. The exam includes multiple-choice questions (MCQs) and numerical answer type questions (NATs).
How is the GATE CS score calculated?
The GATE score is calculated based on the normalized marks obtained in the exam. The score is used for admissions and recruitment in various institutes and organizations.
What are the important topics in GATE CS?
Key topics include algorithms, data structures, computer organization and architecture, programming, operating systems, databases, and discrete mathematics.
Can I use a calculator in the GATE CS Exam?
No, candidates are not allowed to carry calculators. An online virtual calculator is provided within the GATE exam interface.
How should I prepare for GATE CS?
Prepare a comprehensive study plan, focus on understanding fundamental concepts, practice problem-solving, solve previous year papers, and take mock tests for time management.
Can I appear for multiple GATE papers?
Yes, candidates can appear for multiple GATE papers, but they need to choose papers from pre-defined combinations.
What is the validity of the GATE score?
The GATE score is valid for three years from the date of announcement of results.
Can I get a job through GATE CS?
Yes, several public sector undertakings (PSUs) and research organizations use GATE scores for recruitment. Additionally, the score is crucial for admissions to postgraduate programs.
Can foreign nationals apply for GATE CS?
Yes, candidates from Bangladesh, Nepal, Sri Lanka, Ethiopia, and the United Arab Emirates are also eligible to apply for GATE.
Is there negative marking in GATE CS?
Yes, there is negative marking for incorrect answers in multiple-choice questions, while numerical answer type questions do not have negative marking.
How can I download the GATE CS admit card?
The admit card can be downloaded from the official GATE website during the specified period by logging in with the registration credentials.
Can I change my GATE examination center after submitting the application?
No, the examination center once chosen and allotted cannot be changed.
Here are some tips and tricks to help you perform well in the GATE-CS exam:
1. Understand the Syllabus:
- Familiarize yourself with the GATE-CS syllabus. Focus on high-weightage topics and allocate more time to areas where you feel less confident.
2. Create a Study Plan:
- Develop a realistic and well-organized study plan. Break down the syllabus into smaller sections and allocate specific time slots for each.
3. Focus on Fundamentals:
- Strengthen your understanding of fundamental concepts, especially in areas like algorithms, data structures, and programming languages.
4. Practice Regularly:
- Solve a variety of problems regularly to enhance your problem-solving skills. Use online platforms, books, and previous year question papers for practice.
5. Mock Tests and Previous Papers:
- Take mock tests and solve previous year GATE-CS papers to familiarize yourself with the exam pattern and improve time management. Analyze your performance after each test.
6. Time Management:
- Develop good time management skills. Allocate specific time to each question during practice tests and exams.
7. Learn Shortcut Methods:
- While practicing, focus on learning shortcut methods for calculations and problem-solving. This can save valuable time during the exam.
8. Use Virtual Calculator Efficiently:
- Familiarize yourself with the virtual calculator provided in the exam. Practice using it to perform calculations quickly and accurately.
9. Revision is Key:
- Regularly revise important concepts, formulas, and shortcut methods. Create concise notes for quick review before the exam.
10. Stay Healthy:
- Maintain a healthy lifestyle. Get enough sleep, eat well, and exercise regularly. A healthy mind and body contribute to better concentration and performance.
11. Time for Relaxation:
- Take short breaks during study sessions to avoid burnout. Engage in activities you enjoy to refresh your mind.
12. Identify Strengths and Weaknesses:
- Regularly assess your strengths and weaknesses. Allocate more time to topics where you face challenges.
13. Join Online Communities:
- Participate in online forums and communities where GATE aspirants share tips, discuss problems, and provide support. Engaging with peers can offer valuable insights.
14. Simulate Exam Conditions:
- During the last phase of your preparation, simulate exam conditions as closely as possible. Practice solving full-length mock tests to build endurance.
15. Stay Positive and Confident:
- Maintain a positive mindset throughout your preparation. Believe in your abilities and stay confident. Positive thinking can significantly impact your performance.
16. Review Incorrect Answers:
- After each mock test or practice session, thoroughly review the questions you answered incorrectly. Understand the mistakes and learn from them.
17. Seek Guidance:
- If you encounter challenges in understanding certain topics, seek guidance from teachers, mentors, or online resources.
18. Time for Revision Before Exam:
- Reserve the last few days before the exam for revision. Focus on key formulas, concepts, and high-priority topics.
Remember that consistent effort, smart preparation, and a positive attitude are key to success in the GATE-CS exam. Best of luck!
Please Login to comment...
Similar reads.
- GATE-CS-DS-&-Algo
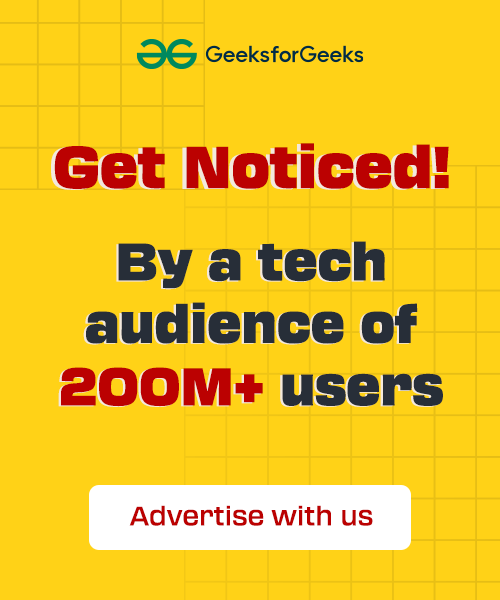
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
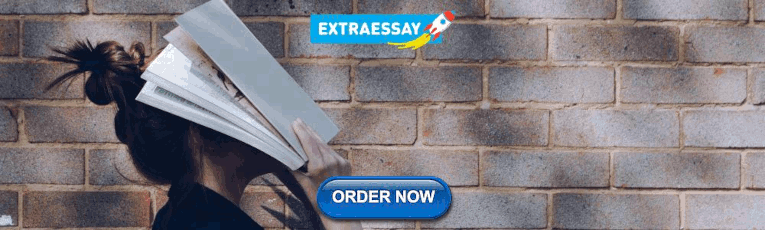
IMAGES
VIDEO
COMMENTS
This chapter presents a case study with exercises that let you think about choosing data structures and practice using them. 13.1: Word frequency analysis. 13.2: Random numbers. 13.3: Word histogram. 13.4: Most common words. 13.5: Optional parameters. 13.6: Dictionary subtraction. 13.7: Random words. 13.8: Markov analysis.
Application of Algorithms: Algorithms are well-defined sets of instructions designed that are used to solve problems or perform a task. To explain in simpler terms, it is a set of operations performed in a step-by-step manner to execute a task. The real-life applications of algorithms are discussed below.
The complete process to learn DSA from scratch can be broken into 5 parts: Learn a programming language of your choice. Learn about Time and Space complexities. Learn the basics of individual Data Structures and Algorithms. Practice, Practice, and Practice more. Compete and Become a Pro.
Data Structures and Algorithms (DSA) refer to the study of methods for organizing and storing data and the design of procedures (algorithms) for solving problems, which operate on these data structures. DSA is one of the most important skills that every computer science student must have. It is often seen that people with good knowledge of these technologies are better programmers than others ...
DSA has great importance in the recruitment process of software companies as well. Recruiters use DSA to test the ability of the programmer because it shows the problem-solving capability of the candidate. As you can see from the above examples, we are able to relate DSA with our day to day life and make it more fun to study.
Part- (1/4) Mastering of DSA are always sought by big product-based companies because it ensures good handling of their data and provides a good measure of a candidate's problem-solving skills. If you will follow this roadmap, then your preparation will be organized. Here are Topics and most imp problems from listed sub-topics.
16-Week learning and coding interview preparation plan. This is recommended for intermediate learners who want to learn DSA concepts and sharpen problem-solving skills. Such learners should have a basic understanding of programming concepts. Minimum estimated time = 10 x 16 = 160 hours.
And, an algorithm is a collection of steps to solve a particular problem. Learning data structures and algorithms allow us to write efficient and optimized computer programs. Our DSA tutorial will guide you to learn different types of data structures and algorithms and their implementations in Python, C, C++, and Java.
and algorithms. We shall study the general ideas concerning e ciency in Chapter 5, and then apply them throughout the remainder of these notes. 1.3 Data structures, abstract data types, design patterns For many problems, the ability to formulate an e cient algorithm depends on being able to organize the data in an appropriate manner.
Study and practice advanced topics in algorithms such as graph theory, computational geometry, and string algorithms. Participate in online coding competitions or contests to test your skills. Day 30:
Master the Coding Interview: Data Structures + Algorithms. This Udemy bestseller is one of the highest-rated interview preparation course (4.6 stars, 21.5k ratings, 135k students) and packs 19 hours worth of contents into it. Like Tech Interview Handbook, it goes beyond coding interviews and covers resume, non-technical interviews, negotiations.
This DSA Crash Course not only helps you brush up on key DSA topics but also includes valuable insights for acing technical interviews. Elevate your technical skills and enhance your interview performance with this essential DSA Crash Course. This comprehensive resource offers a meticulous review of crucial Data Structures and Algorithms ...
How To Prepare For DSA. Hello, Tushar here. Recently, I shared my journey of getting into Cisco from Tier-3 college and Non-CS branch. Data Structures & Algorithms (DSA) played a huge role in my ...
This page titled 13.2: Random numbers is shared under a CC BY-NC 3.0 license and was authored, remixed, and/or curated by Allen B. Downey (Green Tea Press) via source content that was edited to the style and standards of the LibreTexts platform; a detailed edit history is available upon request.
Data Structures and Algorithms (DSA) are the building blocks of computer science and play a pivotal role in technical interviews for software development positions. A strong grasp of DSA concepts is essential for solving complex problems efficiently and effectively. In this article, we will delve into the most important DSA topics that you should be well-prepared for when facing technical ...
Add this topic to your repo. To associate your repository with the dsa-practice topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
Things to do: Binary Search on 1D Arrays. → Binary Search to find X in Sorted Array. → Lower Bound and Upper Bound. → Search Insert Position. → Check if Input array is sorted. → Find the ...
In computer science, data structures and algorithms (DSA) are crucial for methodical problem-solving and program optimization. They comprise a variety of methods and structures, including sorting algorithms and graphs, heaps, and trees. Projects for data structures and algorithms help you learn its usage in real-world situations.
Four 4-hour sessions in April 2024 (12 April, 15 April, 16 April, 18 April), all 1.30pm-5.30pm GMT. Capacity: the short course will be capped at 30 students to maximise interaction. Location: the course will be delivered on Zoom. Format: lectures with in-class discussions and case studies. Topics.
Data structures are essential components that help organize and store data efficiently in computer memory. They provide a way to manage and manipulate data effectively, enabling faster access, insertion, and deletion operations. Common data structures include arrays, linked lists, stacks, queues, trees, and graphs , each serving specific purposes based on the requirements of the problem.
The perception of danger in different cultures. The origins of bipolar disorder through the prism of domestic violence. Covid-19 and related anxiety cases among college students. The dangers of advertisements on children's TV networks. The negative influence of Instagram and distorted body image.
Case Study Locked. Reference Rating 4.7 / 5.0. Customer References 12 total. About. Ackermann Marketing & PR, ranked by PR Week and the Council of PR Firms as one of the top 100 firms in the United States for three consecutive years, is a nationally focused, full-service agency. Since its beginning, the firm has focused on delivering integrated ...
Familiarize yourself with the GATE-CS syllabus. Focus on high-weightage topics and allocate more time to areas where you feel less confident. 2. Create a Study Plan: Develop a realistic and well-organized study plan. Break down the syllabus into smaller sections and allocate specific time slots for each. 3.