- Read Tutorial
- Watch Guide Video
- Complete the Exercise
Now that we've talked about operators. Let's talk about something called the compound assignment operator and I'm going make one little change here in case you're wondering if you ever want to have your console take up the entire window you come up to the top right-hand side here you can undock it into a separate window and you can see that it takes up the entire window.
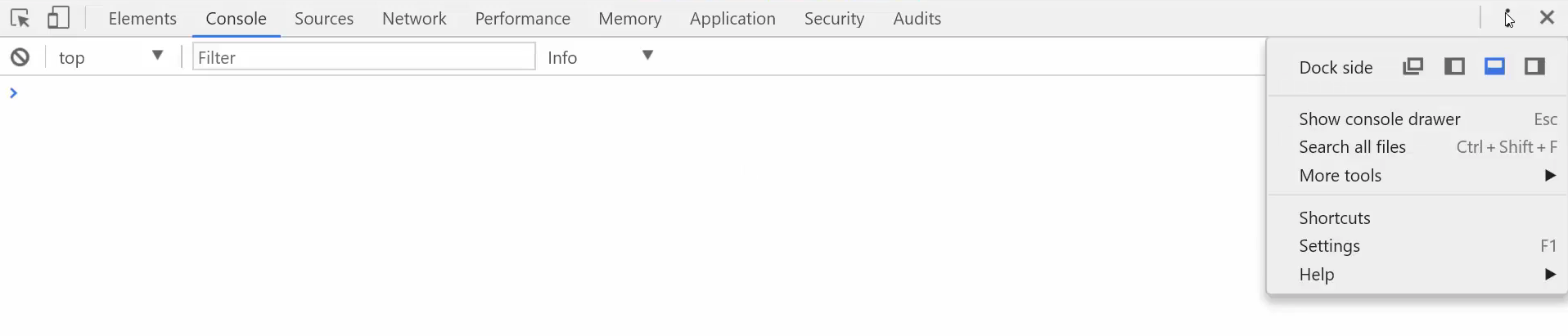
So just a little bit more room now.
Additionally, I have one other thing I'm going to show you in the show notes. I'm going to give you access to this entire set of assignment operators but we'll go through a few examples here. I'm going to use the entire window just to make it a little bit easier to see.
Let's talk about what assignment is. Now we've been using assignment ever since we started writing javascript code. You're probably pretty used to it. Assignment is saying something like var name and then setting up a name
And that is assignment the equals represents assignment.
Now javascript gives us the ability to have the regular assignment but also to have that assignment perform tasks. So for example say that you want to add items up so say that we want to add up a total set of grades to see the total number of scores. I can say var sum and assign it equal to zero.
And now let's create some grades.
I'm going to say var gradeOne = 100.
and then var gradeTwo = 80.
Now with both of these items in place say that we wanted to add these if you wanted to just add both of them together you definitely could do something like sum = (gradeOne + gradeTwo); and that would work.
However, one thing I want to show you is, there are many times where you don't have gradeOne or gradeTwo in a variable. You may have those stored in a database and then you're going to loop through that full set of records. And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do.
Let's use one of the more basic ones which is to have the addition assignment.
Now you can see that sum is equal to 100.
Then if I do
If we had 100 grades we could simply add them just like that.
Essentially what this is equal to is it's a shorthand for saying something like
sum = sum + whatever the next one is say, that we had a gradeThree, it would be the same as doing that. So it's performing assignment, but it also is performing an operation. That's the reason why it's called a compound assignment operator.
Now in addition to having the ability to sum items up, you could also do the same thing with the other operators. In fact literally, every one of the operators that we just went through you can use those in order to do this compound assignment. Say that you wanted to do multiplication you could do sum astrix equals and then gradeTwo and now you can see it equals fourteen thousand four hundred.
This is that was the exact same as doing sum = whatever the value of sum was times gradeTwo. That gives you the exact same type of process so that is how you can use the compound assignment operators. And if you reference the guide that is included in the show notes. You can see that we have them for each one of these from regular equals all the way through using exponents.
Then for right now don't worry about the bottom items. These are getting into much more advanced kinds of fields like bitwise operators and right and left shift assignments. So everything you need to focus on is actually right at the top for how we're going to be doing this. This is something that you will see in a javascript code. I wanted to include it, so when you see it you're not curious about exactly what's happening.
It's a great shorthand syntax for whenever you want to do assignment but also perform an operation at the same time.
- Documentation for Compound Assignment Operators
- Source code
devCamp does not support ancient browsers. Install a modern version for best experience.
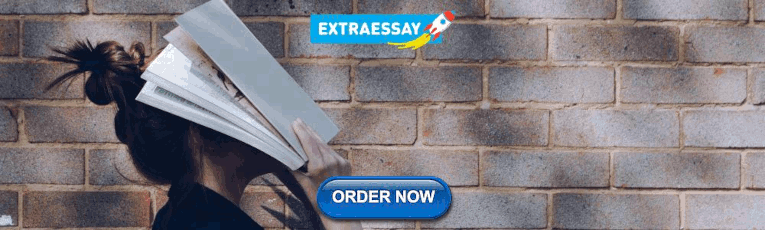
IMAGES
VIDEO
COMMENTS
And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do. Let's use one of the more basic ones which is to have the addition assignment. sum += gradeOne; // 100. Now you can see that sum is equal to 100. Then if I do. sum += gradeTwo; // 180.