- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
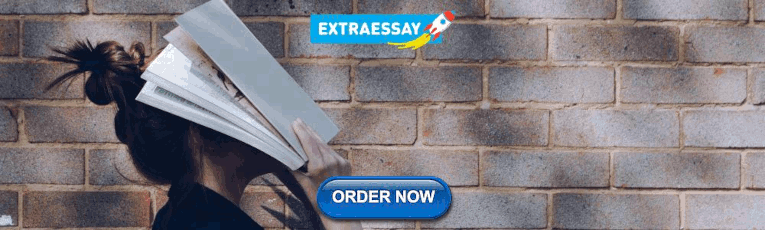
How to Create a MS PowerPoint Presentation in Java with a Maven Project?
- Creating Java Project Without Maven in Apache NetBeans (11 and Higher)
- How to Create a Maven Project in IntelliJ IDEA?
- How to Create a Maven Project in Eclipse IDE?
- Java Program to Create a Blank PPT Document
- Creating Hyperlink on a Slide in a PPT using Java
- Formatting Text on a Slide in a PPT using Java
- How to Edit a Powerpoint Presentation?
- 10 PowerPoint Presentation Tips to Make More Creative Slideshows
- How to Add Audio to Powerpoint Presentation
- How to Save PowerPoint Presentations as PDF Files using MS PowerPoint?
- How to Change Slide Layout in MS PowerPoint ?
- How to Add different Slide Designs in MS PowerPoint?
- Create a New Maven Project from Command Prompt
- How to Convert PNG to JPG using MS PowerPoint?
- How to use Animation Pane in MS PowerPoint?
- How to Use the Animation Painter in MS Powerpoint?
- How to Install Apache Maven on Windows?
- How to Insert Video in Microsoft Powerpoint?
- Applying Transitions to Slides in MS PowerPoint
- Arrays in Java
- Spring Boot - Start/Stop a Kafka Listener Dynamically
- Parse Nested User-Defined Functions using Spring Expression Language (SpEL)
- Split() String method in Java with examples
- Arrays.sort() in Java with examples
- For-each loop in Java
- Object Oriented Programming (OOPs) Concept in Java
- Reverse a string in Java
- HashMap in Java
- How to iterate any Map in Java
In the software industry, presentations play a major role as information can be conveyed easily in a presentable way via presentations. Using Java, with the help of Apache POI, we can create elegant presentations. Let us see in this article how to do that.
Necessary dependencies for using Apache POI:
It has support for both .ppt and .pptx files. i.e. via
- HSLF implementation is used for the Powerpoint 97(-2007) file format
- XSLF implementation for the PowerPoint 2007 OOXML file format.
There is no common interface available for both implementations. Hence for
- .pptx formats, XMLSlideShow, XSLFSlide, and XSLFTextShape classes need to be used.
- .ppt formats, HSLFSlideShow, HSLFSlide, and HSLFTextParagraph classes need to be used.
Let us see the example of creating with .pptx format
Creation of a new presentation:
Next is adding a slide
Now, we can retrieve the XSLFSlideLayout and it has to be used while creating the new slide
Let us cover the whole concept by going through a sample maven project.
Example Maven Project
Project Structure:
As this is the maven project, let us see the necessary dependencies via pom.xml
PowerPointHelper.java
In this file below operations are seen
- A new presentation is created
- New slides are added
- save the presentation as
We can write Text, create hyperlinks, and add images. And also the creation of a list, and table are all possible. In general, we can create a full-fledged presentation easily as well can alter the presentation by adjusting the slides, deleting the slides, etc. Below code is self-explanatory and also added comments to get an understanding of it also.
We can able to get the presentation got created according to the code written and its contents are shown in the image below
We can test the same by means of the below test file as well
PowerPointIntegrationTest.java
Output of JUnit:
We have seen the ways of creating of presentation, adding text, images, lists, etc to the presentation as well as altering the presentation as well. Apache POI API is a very useful and essential API that has to be used in software industries for working with the presentation.
Please Login to comment...
Similar reads.
- Technical Scripter 2022
- Technical Scripter
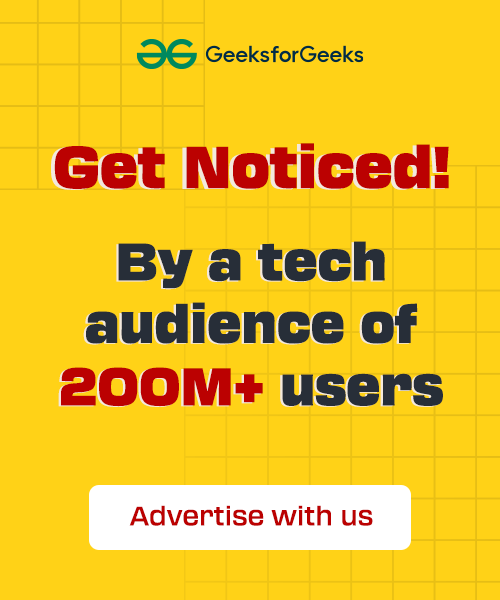
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
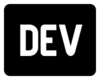
DEV Community

Posted on May 6, 2022
Java - How to Create a PowerPoint Presentation
Creating an attractive PowerPoint presentation is a meticulous task that needs the cooperation of brain, eyes, and hands. In order to achieve a perfect effect, we need to constantly fine tune the details, such as adjusting the size and position of a shape, or changing the color of text. Due to this reason, creating PowerPoint documents manually is typically more efficient than using code. However, in certain cases, we may have to do it programmatically.
In this article, you’ll learn how to create a simple PowerPoint document and insert basic elements (including text shape, image shape, list, and table) into it by using Free Spire.Presentation for Java , which is a free class library for processing PowerPoint documents in Java applications. The main tasks of this tutorial are as follows.
Create a PowerPoint Document
Get the first slide and set background image, insert a text shape, insert an image shape, insert a list, insert a table, save the powerpoint document, add spire.presentation.jar as dependency.
If you are working on a maven project, you can include the dependency in pom.xml file using this:
If you are not using maven, then you can find the required jar files from the zip file available in this location . Include all the jar files into the application lib folder to run the sample code given in this tutorial.
Import Namespaces
By default, there is one blank slide preset in the newly created PowerPoint document.

Top comments (0)
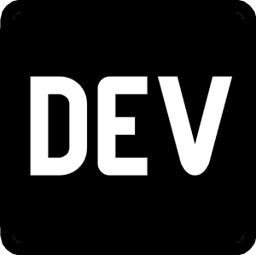
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
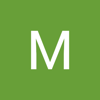
what is Playwright ? Why?
Mangai Ram - Mar 22
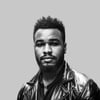
Build an AI-powered blogging platform (Next.js, Langchain & CopilotKit)
Bonnie - Apr 11
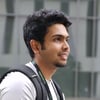
Devil or Devin : The World’s First AI Software Engineer
Dhanush N - Mar 25
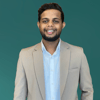
Java Memory Leaks: Find & Conquer
Tharindu Dulshan Fernando - Apr 13
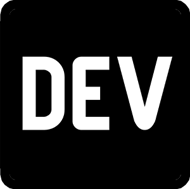
We're a place where coders share, stay up-to-date and grow their careers.
Create PowerPoint PPT PPTX Presentations in Java
PowerPoint presentations let you create attractive slide slows containing text, graphics, charts, animations, and other elements to make your presentations appealing. In this article, you are going to learn how to implement PowerPoint automation features from within Java applications. Particularly, we will cover how to create PowerPoint PPT or PPTX presentations from scratch in Java . In addition, we will demonstrate how to insert various types of elements in the slides programmatically.
- Java API to Create PowerPoint Presentations - Free Download
- Create a PowerPoint PPT in Java
- Open an Existing PowerPoint PPT
- Add Slide to a PowerPoint PPT/PPTX
- Add Text to a Slide in PPT
- Create a Table in PowerPoint PPT
- Add an Image to PPT/PPTX
Java API to Create PowerPoint Presentations - Free Download #
For implementing the PowerPoint automation features, Aspose offers Aspose.Slides for Java . It is a high-speed API that makes it quite easier for you to create, edit, convert, and manipulate PowerPoint PPT/PPTX from within your Java applications. You can either download the API or install it within your Maven-based applications using the following configurations.
Create a PowerPoint Presentation in Java #
To begin with the PowerPoint automation, let’s first create an empty presentation document and save it as a PPTX file. The following are the steps to create a presentation document.
- Create an instance of the Presentation class.
- Save it as PPTX using Presentation.save(String, SaveFormat) method.
The following code sample shows how to create a PowerPoint PPT using Java.
Edit a PowerPoint PPT in Java #
Aspose.Slides for Java also allows you to open existing PowerPoint presentations in order to update their content. The following are the steps to load a PowerPoint PPTX file.
- Create an instance of the Presentation class and provide the PPTX file’s path to its constructor.
- Update the content of the presentation.
- Save the updated presentation using Presentation.save(String, SaveFormat) method.
The following code sample shows how to open an existing PowerPoint presentation using Java.
Add Slide to a PPT in Java #
Let’s now have a look at how to add slides to a presentation document. This can be done either for a new presentation or an existing one. The following are the steps to add slides to a PowerPoint PPT in Java.
- Instantiate ISlideCollection class by setting a reference to the Presentation.getSlides() .
- Add an empty slide to the presentation using ISlideCollection.addEmptySlide(ILayoutSlide) method exposed by ISlideCollection object.
The following code sample shows how to add slides to a PowerPoint PPT in Java.
Create PPT and Add Text in Java #
Once you have created a presentation and added slides to it, you can start inserting different elements into it. First of all, let’s have a look at the steps of adding text to a slide using Aspose.Slides for Java.
- Get the reference of the slide you want to add the text to in the ISlide object.
- Add a rectangle using ISlide.getShapes().addAutoShape() method and get its reference in IAutoShape object.
- Add a TextFrame to the shape containing the default text.
- Set the properties of the text such as fill color, fill type, etc.
The following code sample shows how to add text to a PowerPoint PPTX in Java.
Create Table in PowerPoint PPTX in Java #
Table is an important element that is used to organize the content in the form of rows and columns. For adding table to a slide, you can following the below steps.
- Get the reference of the slide you want to add the text to.
- Create an array of columns’ width.
- Create an array of rows’ height.
- Add a Table to the slide using ISlide.getShapes().addTable() method and get its reference to ITable object.
- Iterate through each cell to apply formatting to the Top, Bottom, Right and Left Borders.
- Add some text to the cell.
The following code sample shows how to create a table in PowerPoint PPTX using Java.
Learn more about working with tables using this article .
Add an Image in PowerPoint PPTX in Java #
The following are the steps to add an image in a PowerPoint presentation using Java.
- Get the reference of the slide in the ISlide object.
- Create an object of IPPImage class.
- Add image to the presentation using Presentation.getImages().addImage(FileInputStream) method.
- Add the image as a picture frame to the slide with the height and width equivalent of the image.
The following code sample shows how to add image to a PowerPoint PPT in Java.
Live example: Want to see a simple implementation of Aspose APIs? Check out this online Viewer app used to open and read presentations.
API to Create PowerPoint PPT in Java - Get a Free License #
You can use Aspose.Slides for Java without evaluation limitations by getting a free temporary license .
Conclusion #
In this article, you have learned how to create PowerPoint PPT or PPTX presentations from scratch in Java. Furthermore, the steps and code samples have demonstrated how to insert slides, text, images, and tables in new or existing PPT/PPTX presentations. Moreover, you can explore about the Java PowerPoint API using documentation .
- Convert PowerPoint to PDF using Java
Create PowerPoint Presentation in Java with Apache POI API
In our previous article, we introduced the Apache POI components for working with PowerPoint presentation files. We had a look at the HSLF (Horrible Slide Layout Format) and XSLF (XML Slide Layout Format) APIs offered by Apache POI. In this article, we’ll see how to create presentations in Java and save these as PPTX files .
System Requirements
Before you begin, make sure that your system meets the following requirements.
- JDK – Java SE 2 JDK 1.5 or above
- Memory – 1 GB Ram
- Operating System – Windows/ Linux/ Mac OS
Setting Up Development Environment for Apache POI
You’ll need a Java development environment such as Eclipse, IntelliJ IDEA, or any other IDE you’re comfortable with to work with the Apache POI library in your application. Next is to add Apache POI Maven dependency in your application’s pom.xml file as shown below.
Creating Empty Presentation in Java
Now that your development is ready, let’s dive into writing the code for creating our first blank PowerPoint Presentation.
When you open the saved file, you will see that the Presentation opens with Microsoft PowerPoint and doesn’t have any slides in it. This is the default behavior when creating a PowerPoint presentation with Apache POI.
Add Slides to PowerPoint Presentation in Java
The above code sample created an empty PowerPoint presentation without any slides. In order to create a new presentation with slides, use the createSlide method of the XMLSlideShow class as shown in the updated code sample below.
The createSlide method of the XMLSlideShow class is used to add a blank slide to the presentation. This method returns an object of the XSLFSlide class that can be further used to add content to the slide and work with its properties.
Apache POI components for working with PowerPoint presentation files let you create and edit PowerPoint PPT and PPTX files from within your Java application. In our upcoming blogs, we’ll further demonstrate the usage of Apache POI Java components for working with PowerPoint presentations. So, stay tuned.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Spire.Presentation for Java is a professional PowerPoint API that enables developers to create, read, write, convert and save PowerPoint documents in Java Applications.
eiceblue/Spire.Presentation-for-Java
Folders and files, repository files navigation, spire.presentation-for-java library for processing powerpoint documents.
Product Page | Tutorials | Examples | Forum | Customized Demo | Temporary License
Spire.Presentation for Java is a professional PowerPoint API that enables developers to create, read , write, convert and save PowerPoint documents in Java Applications. As an independent Java library, Spire.Presentation doesn't need Microsoft PowerPoint to be installed on system.
A rich set of features can be supported by Spire.Presentation for Java, such as add/edit/remove slide , create chart , create table , add bullets , encrypt and decrypt PPT , add watermark , add hyperlink , insert audio and video , paragraph settings, document properties settings , insert/extract image , extract text , set animation , add header and footer , add/delete comment, add note , create SmartArt .
Standalone Java API
Spire.Presentation for Java is a 100% independent Java PowerPoint API which doesn't require Microsoft PowerPoint to be installed on system.
Support PowerPoint Version
- PPT - PowerPoint Presentation 97-2003
- PPS - PowerPoint SlideShow 97-2003
- PPTX - PowerPoint Presentation 2007/2010/2013/2016/2019
- PPSX - PowerPoint SlideShow 2007, 2010
Rich PowerPoint Elements Supported
Spire.Presentation for Java supports to process a variety of PowerPoint elements, such as slide , text , image , shape , table , chart , watermark , animation , header and footer , comment , note , SmartArt , hyperlink , OLE object, audio and video .
High Quality PowerPoint File Conversion
Spire.Presentation for Java allow developers to convert PowerPoint documents to other file formats such as:
Convert PowerPoint to PDF
Convert PowerPoint to Image
Convert PowerPoint to SVG
Convert PowerPoint to XPS
Convert PowerPoint to HTML
Convert PowerPoint to PDF in Java
Convert powerpoint to images in java, convert powerpoint to svg in java.
- Spire.Office for .NET
- Spire.OfficeViewer for .NET
- Spire.Doc for .NET
- Spire.DocViewer for .NET
- Spire.XLS for .NET
- Spire.Spreadsheet for .NET
- Spire.Presentation for .NET
- Spire.PDF for .NET
- Spire.PDFViewer for .NET
- Spire.PDFViewer for ASP.NET
- Spire.DataExport for .NET
- Spire.Barcode for .NET
- Spire.Email for .NET
- Spire.OCR for .NET
- Free Spire.Office for .NET
- Free Spire.Doc for .NET
- Free Spire.DocViewer for .NET
- Free Spire.XLS for .NET
- Free Spire.Presentation for .NET
- Free Spire.PDF for .NET
- Free Spire.PDFViewer for .NET
- Free Spire.PDFConverter for .NET
- Free Spire.DataExport for .NET
- Free Spire.Barcode for .NET
- Spire.Office for WPF
- Spire.Doc for WPF
- Spire.DocViewer for WPF
- Spire.XLS for WPF
- Spire.PDF for WPF
- Spire.PDFViewer for WPF
- Order Online
- Download Centre
- Temporary License
- Purchase Policies
- Renewal Policies
- Find A Reseller
- Purchase FAQS
- Support FAQs
- How to Apply License
- License Agreement
- Privacy Policy
- Customized Demo
- Code Samples
- Unsubscribe
- API Reference
- Spire.Doc for Java
- Spire.XLS for Java
Spire.Presentation for Java
- Spire.PDF for Java
- Become Our Reseller
- Paid Support
- Our Customers
- Login/Register

- Python APIs
- Android APIs
- AI Products
- .NET Libraries
- Free Products
- Free Spire.Email for .NET
- WPF Libraries
- Java Libraries
- Spire.Office for Java
- Spire.Barcode for Java
- Spire.OCR for Java
- Free Spire.Office for Java
- Free Spire.Doc for Java
- Free Spire.XLS for Java
- Free Spire.Presentation for Java
- Free Spire.PDF for Java
- Free Spire.Barcode for Java
- C++ Libraries
- Spire.Office for C++
- Spire.Doc for C++
- Spire.XLS for C++
- Spire.PDF for C++
- Spire.Presentation for C++
- Spire.Barcode for C++
- Python Libraries
- Spire.Office for Python
- Spire.Doc for Python
- Spire.XLS for Python
- Spire.PDF for Python
- Spire.Presentation for Python
- Spire.Barcode for Python
- Android Libraries
- Spire.Office for Android via Java
- Spire.Doc for Android via Java
- Spire.XLS for Android via Java
- Spire.Presentation for Android via Java
- Spire.PDF for Android via Java
- Free Spire.Office for Android via Java
- Free Spire.Doc for Android via Java
- Free Spire.XLS for Android via Java
- Free Spire.Presentation for Android via Java
- Free Spire.PDF for Android via Java
- Cloud Libraries
- Spire.Cloud.Office
- Spire.Cloud.Word
- Spire.Cloud.Excel
- Spire.XLS AI for .NET
- Newsletter Subscribe Unsubscribe
- Spire.Presentation
- Spire.Barcode
- Spire.Email
- Spire.DocViewer
- Spire.PDFViewer
- Spire.SpreadSheet
- Spire.Cloud
Java PowerPoint Library – Create Read Modify Print Convert PowerPoint Documents in Java
A PowerPoint® Compatible Library without Microsoft PowerPoint
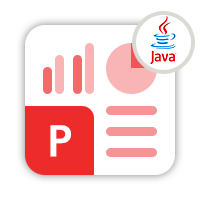
Spire.Presentation for Java is a professional PowerPoint API that enables developers to create, read , write, convert and save PowerPoint documents in Java Applications. As an independent Java library, Spire.Presentation doesn't need Microsoft PowerPoint to be installed on system.
A rich set of features can be supported by Spire.Presentation for Java, such as add/edit/remove slide , create chart , create table , add bullets , encrypt and decrypt PPT , add watermark , add hyperlink , insert audio and video , paragraph settings, document properties settings , insert/extract image , extract text , set animation , add header and footer , add/delete comment, add note , create SmartArt .
Spire.Presentation for Java also supports to convert PowerPoint document to image , PDF , HTML, XPS , PPTX and SVG in high quality.

Convert PowerPoint to PDF
Converting PowerPoint to PDF helps you maintain the layout and formatting of your presentation when viewed on different systems or devices.
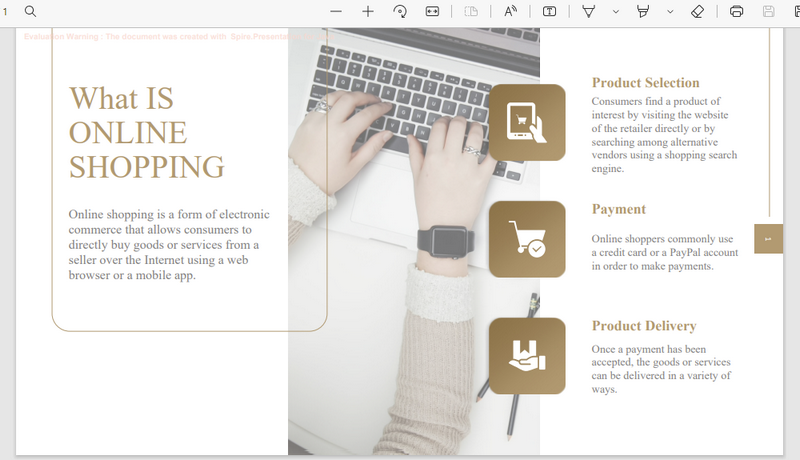
Create Slide Masters
A slide master controls the design of all the slides based on it. Using a slide master makes it easier to create presentations that look consistent and visually appealing.
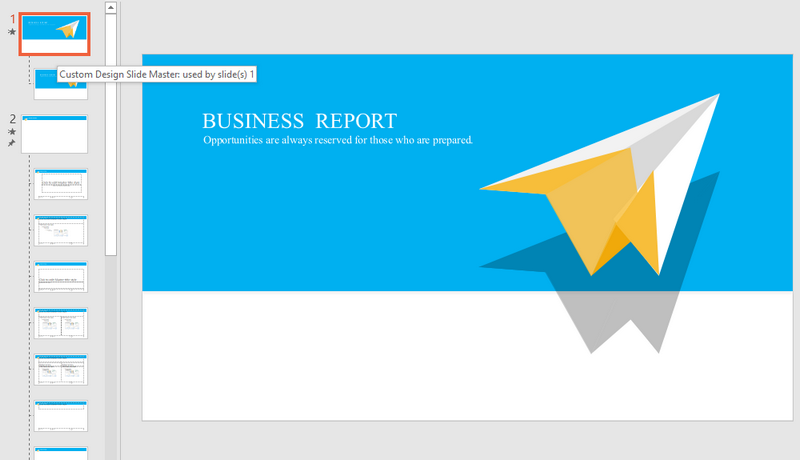
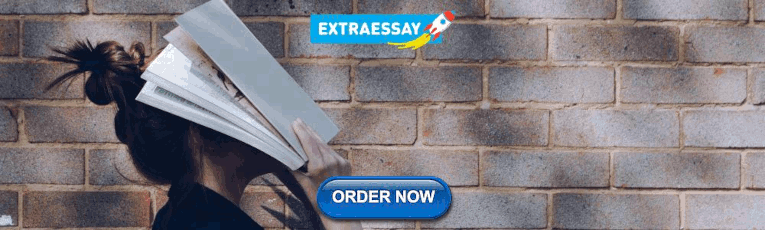
Add a Watermark
Watermarks are used to declare confidentiality, copyright, source, or other attributes of the document, or as a decoration to make the document more attractive. Both text watermarks and image watermarks can be added to presentations.
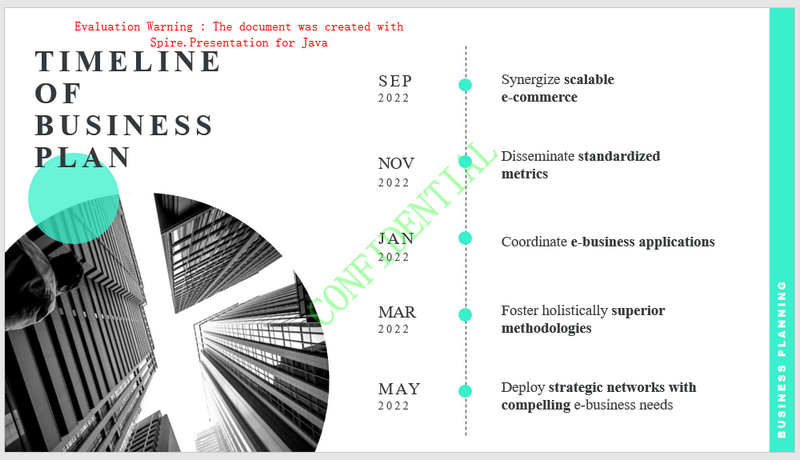
Extract Text and Images
If you only need the text and images of a PowerPoint document regardless of their formatting and layout, you can directly extract them from the document.
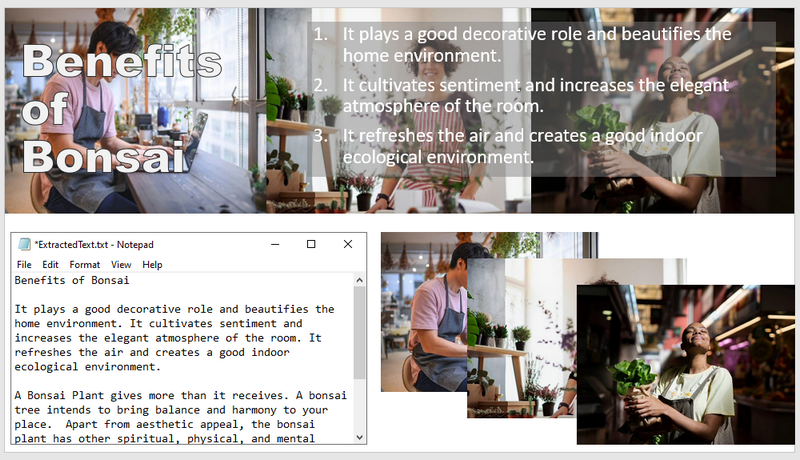
Digitally Sign PowerPoint Documents
A digital signature provides assurances about the validity and authenticity of your presentation. Once a PowerPoint document is digitally signed, any changes to the document will invalidate the signature.
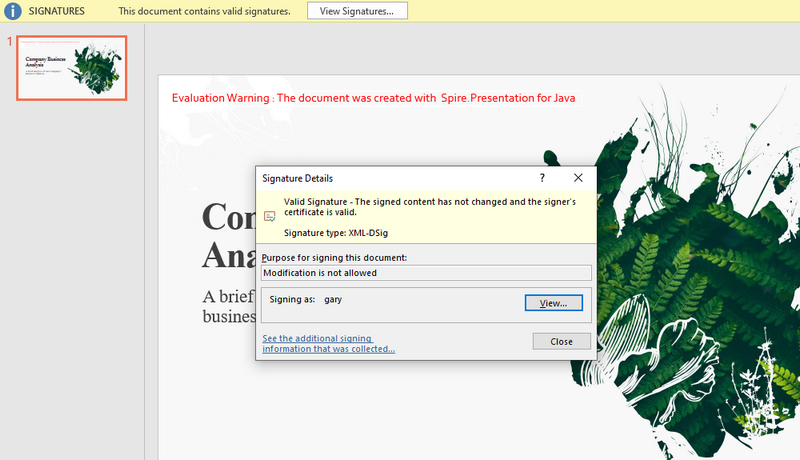
Insert Charts
Charts in PowerPoint can help illustrate data, show trends or changes in data over time, and make the whole document more professional and attractive.
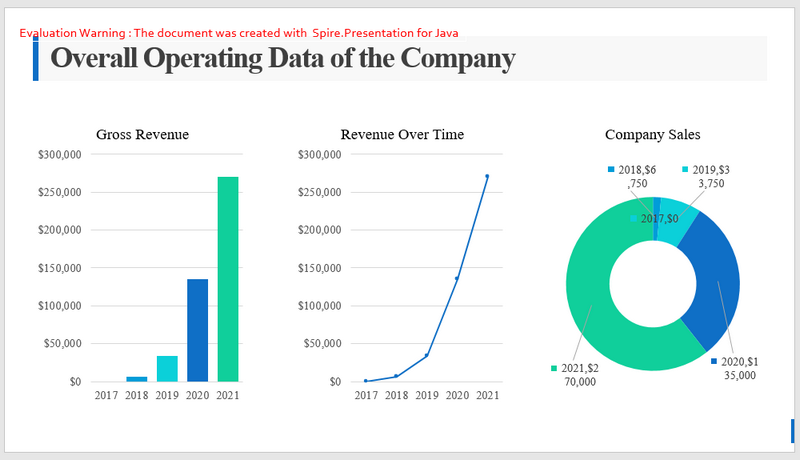
Insert a SmartArt
SmartArt is a way to combine text, shapes and colors into an image or illustration. SmartArt graphics let you easily create a visual representation of your information.
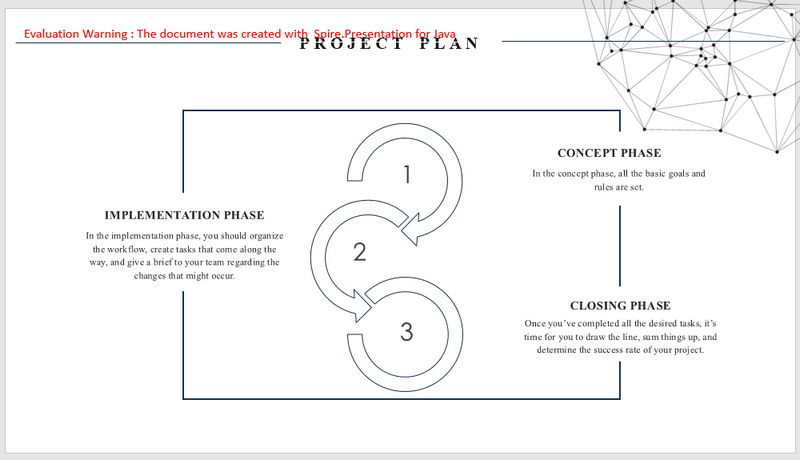
Insert Images and Shapes
Adding pictures and shapes can make your presentations more interesting and engaging. And you can customize your images by cropping, reordering, changing colors or adding other formatting and customize shape according to your own color palette, preferences.
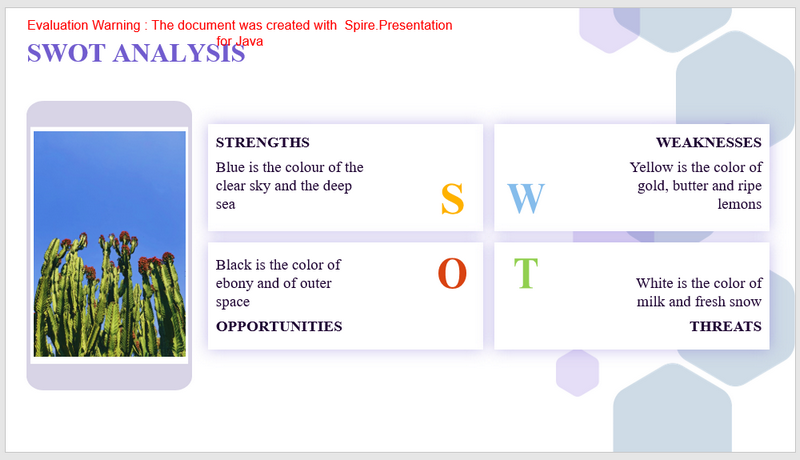
Add Speaker Notes
Adding speaker notes to a PowerPoint presentation provides reference material for the speaker when they’re presenting a slideshow, allowing them to stay on track without forgetting the key points to deliver a flawless presentation.
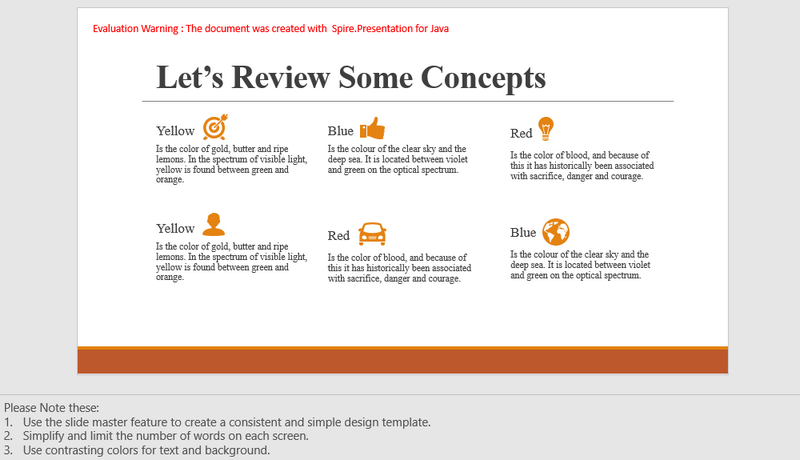
Set Animations on Shapes in PowerPoint
Animation is a great way to emphasize important points, to control the flow of information, and to increase viewer interest in your presentation. You can animate almost every objects in PowerPoint slide to give them visual effects.
Standalone Java API
100% independent Java PowerPoint API which doesn't require Microsoft PowerPoint to be installed on system.
- PPT - PowerPoint Presentation 97-2003
- PPS - PowerPoint SlideShow 97-2003
- PPTX - PowerPoint Presentation 2007/2010/2013/2016/2019
- PPSX - PowerPoint SlideShow 2007, 2010
Powerful Toolset, Multichannel Support

Work with PowerPoint Charts

Print PowerPoint Presentations

Work with SmartArt

Images and Shapes

Audio and Video

Protect Presentation Slides

Text and Image Watermark

Merge Split PowerPoint Document

Comments and Notes

Manage PowerPoint Tables

Set Animations on Shapes

Manage Hyperlink

Extract Text and Image

Replace Text
Conversion File Documents with High Quality

PowerPoint Document
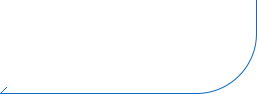
MAIN FUNCTION
Only Spire.Presentation for Java, No Microsoft Office Installed
High Quality PowerPoint File Conversion
Rich PowerPoint Elements Supported
Easy Integration
Commercial Edition $799
Compared with the free version, the commercial edition has no slides limitation and is more comprehensive in processing PowerPoint files.
Free Edition $0
Free version is limited to 10 presentation slides when creating PPT and PPTX. When converting PowerPoint files to PDF, Image or XPS, you can only get the first 10 pages of the generated file.
GET STARTED
Free Trials for All Progress Solutions
Support Environment
Support powerpoint version, paragraph and text, image and shape, table and chart, comment and note.
Here is a brief summary of Spire.Presentation for Java features.
- 100% Written in Java
- Supports 32-bit and 64-bit OS
- Works on Windows, Linux, Unix and Mac OS
- No Need to Install Additional Software
- PPTX - PowerPoint Presentation 2007, 2010, 2013, 2016 and 2019
- Convert PPT/PPTX to Image
- Convert PPT/PPTX to PDF
- Convert PPT to PPTX
- Convert PPT/PPTX to SVG
- Convert PPT/PPTX to HTML
- Convert PPT/PPTX to XPS
- Encrypt Documents
- Remove Encryption
- Set Document to Read Only
- Create, Remove, Hide and Clone Slide
- Change Slide Layout
- Add Master Slide
- Set Background
- Set Transitions
- Set Paragraph Indent
- Add Bullets
- Extract Text
- Insert Hyperlinks
- Add Text Watermark
- Insert Image
- Insert Shape
- Fill Shape (with solid, gradient color or picture)
- Extract Image and Shape
- Set Animations
- Create Table
- Create Combination Chart
- Create Doughnut Chart
- Save Chart as Image
- Merge Table Cell
- Insert Audio
- Insert Video
- Insert SmartArt
- Add and Remove Node
- Add comment
- Delete comment
- Believe The Users
Version: 9.4.5
Version: 9.3.1, version: 9.2.8, version: 9.2.2, version: 8.12.1, version: 8.11.1, version: 8.10.4, version: 8.9.4, version: 8.8.1, version: 8.7.3.
This is the list of changelogs of Spire.Presentation for Java New release and hotfix. You can get the detail information of each version's new features and bug solutions.
- Find and Replace
- Add Text and Image
- Add WaterMark

Set text watermark
Set image watermark
If you don't find the function you want, please fill in a form to request a free demo from us. Make sure the demo you want meets the following requirements:
- It is a small project that implements a particular scenario.
- It relates to our libraries stored on E-iceblue online store.
- It costs less than 2 hours for us to complete it.
- It is not a bug report.
- It is not a feature request.
- Spire.Spreadsheet
- Purchase FAQs
- License Upgrade
- Our Service
Aspose Knowledge Base
Find advice and answers for most commonly faced scenarios.
Find Answers by API
- Aspose.Total Product Family
- Aspose.Words Product Family
- Aspose.PDF Product Family
- Aspose.Cells Product Family
- Aspose.Email Product Family
- Aspose.Slides Product Family
- Aspose.Imaging Product Family
- Aspose.BarCode Product Family
- Aspose.Diagram Product Family
- Aspose.Tasks Product Family
- Aspose.OCR Product Family
- Aspose.Note Product Family
- Aspose.CAD Product Family
- Aspose.3D Product Family
- Aspose.HTML Product Family
- Aspose.GIS Product Family
- Aspose.ZIP Product Family
- Aspose.Page Product Family
- Aspose.PSD Product Family
- Aspose.OMR Product Family
- Aspose.PUB Product Family
- Aspose.SVG Product Family
- Aspose.Finance Product Family
- Aspose.Drawing Product Family
- Aspose.Font Product Family
- Aspose.TeX Product Family
How to Create PowerPoint Presentation using Java
In this simple topic, we will walk you through how to create PowerPoint Presentation using Java in MS Windows, macOS, or Ubuntu operating systems. This topic covers the detailed steps to set up the environment and by using a few lines of easy code in Java PPT presentation can be generated.
Steps to Generate PowerPoint Presentation in Java
- Download and install Aspose.Slides for Java from the Maven repository
- Instantiate the Presentation class object to create an empty presentation
- Create a blank slide and add that to the presentation slides collection
- Using the AddAutoShape method, insert a Rectangle shape in the newly created slide
- Insert a text frame using the addTextFrame method and set the text related properties
- Save the presentation on the disk in PPTX format
The aforementioned steps in Java create PPTX file on the disk using the simple API interface and with no dependence on PowerPoint. Firstly, an empty presentation is created using the Presentation class instance, which is followed by adding a blank slide inside the presentation. Then, a text frame is added inside the shape and its respective textual properties are set before saving the presentation on the disk using the save method.
Code to Create PowerPoint Presentation using Java
In Java presentation can be generated using a few lines of code as given in the above example. You may also save the presentation in the other formats like PPT, PPS, PPSX, ODP, POT and POTX using the SaveFormat enumerator. The text inside the presentation can be customized by using the different options exposed by the ParagraphFormat and PortionFormat classes which include setting the options like text wrapping, text autofit, indentations, margins, bullets, text highlighting and strike-through.
In this topic, we have learned how using Java PowerPoint presentation in different formats can be created. If you are interested in converting presentation slides to SVG, please visit the details mentioned in the article on how to convert PPTX to SVG using Java .
Updated on 11 May 2022
Got any suggestions?
We want to hear from you! Send us a message and help improve Slidesgo
Top searches
Trending searches
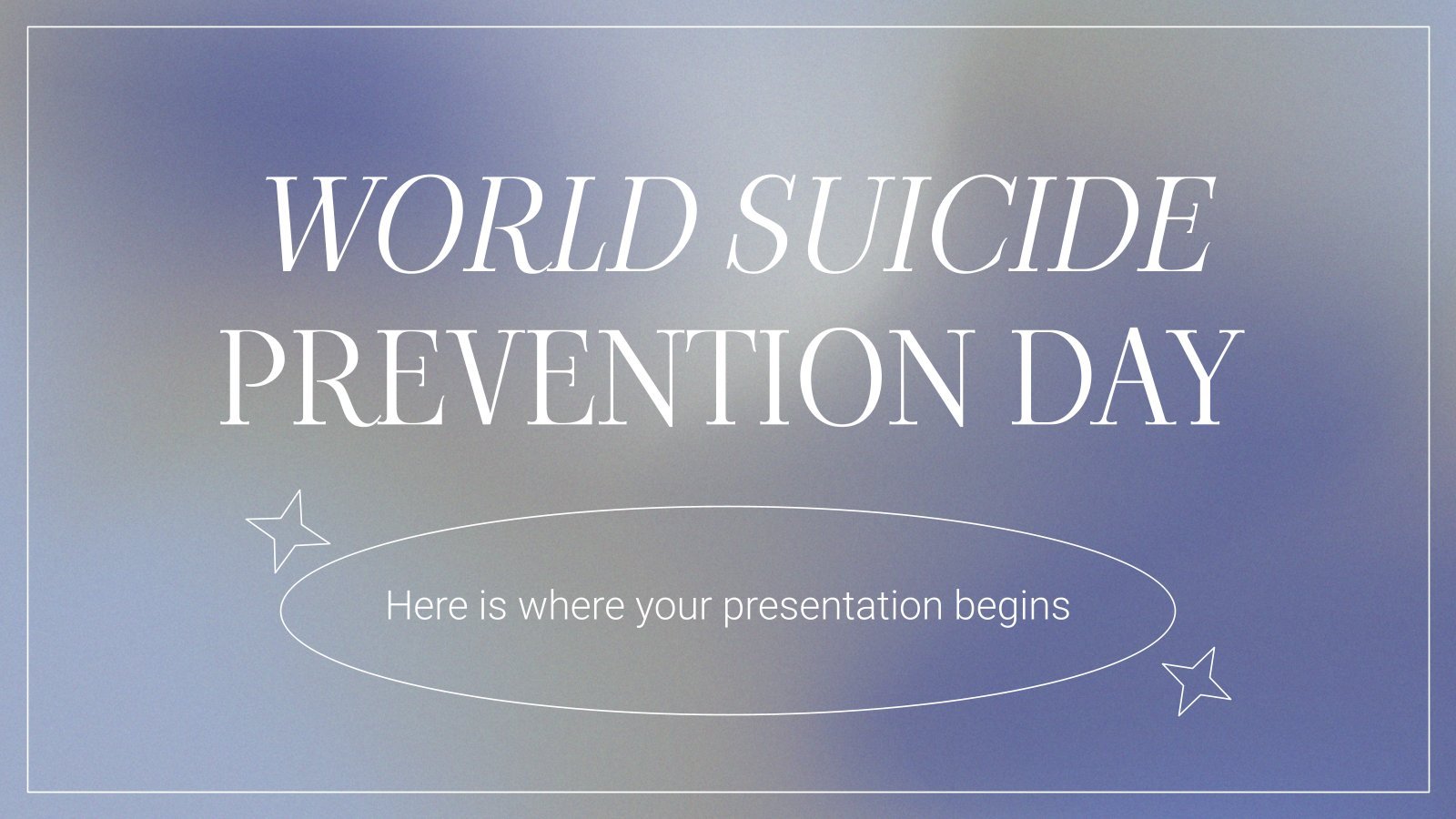
suicide prevention
8 templates
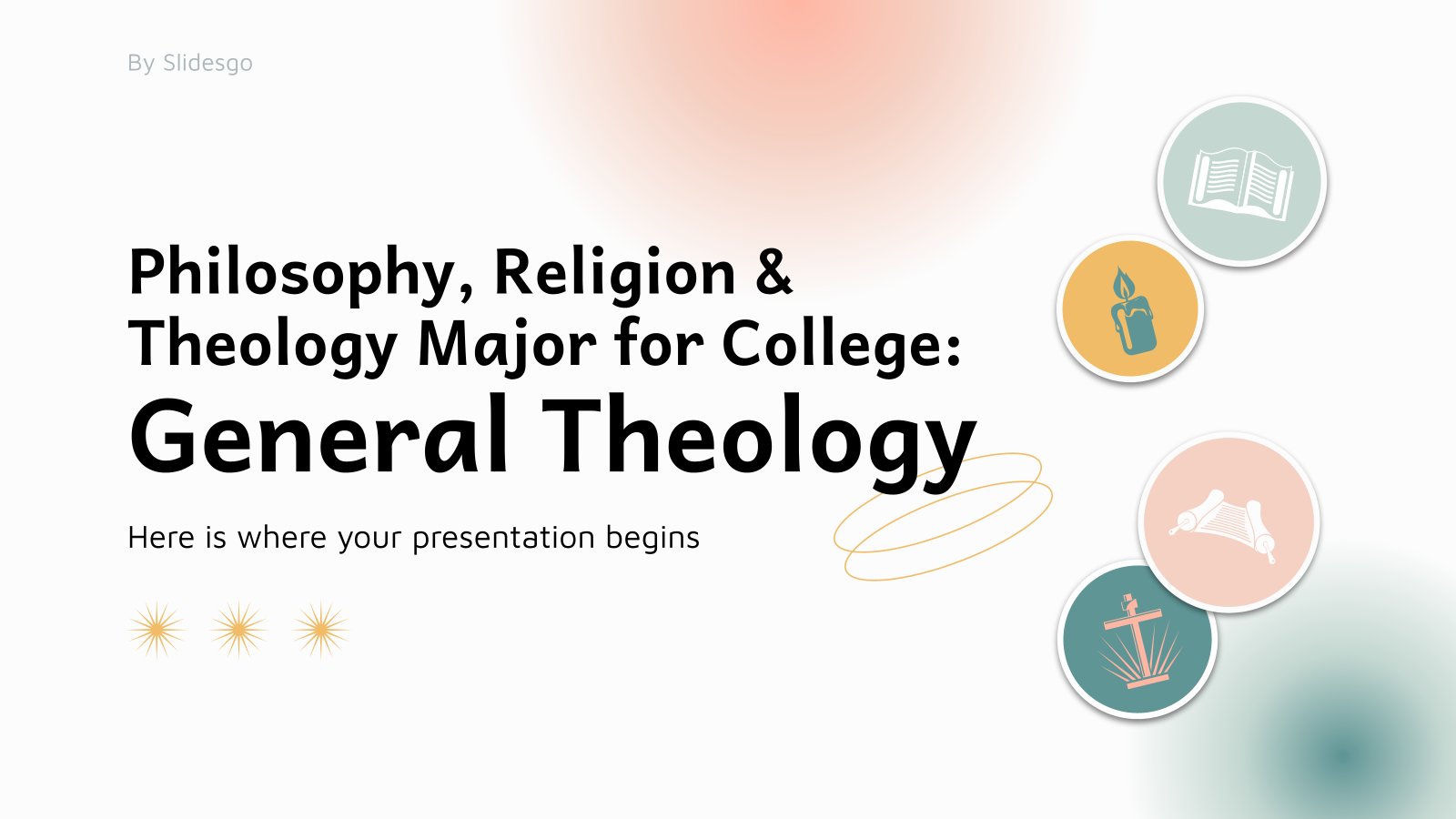
46 templates
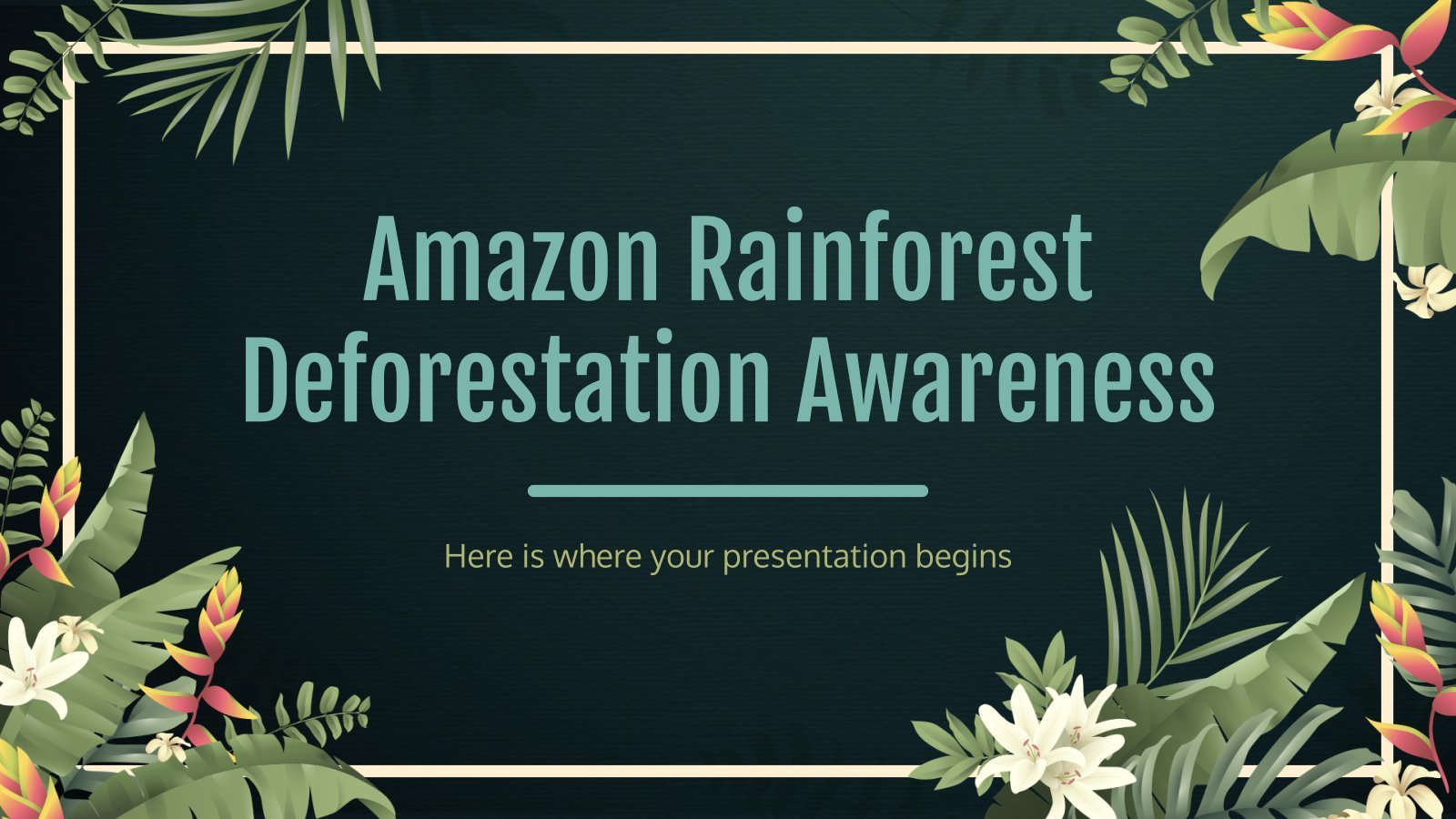
tropical rainforest
29 templates
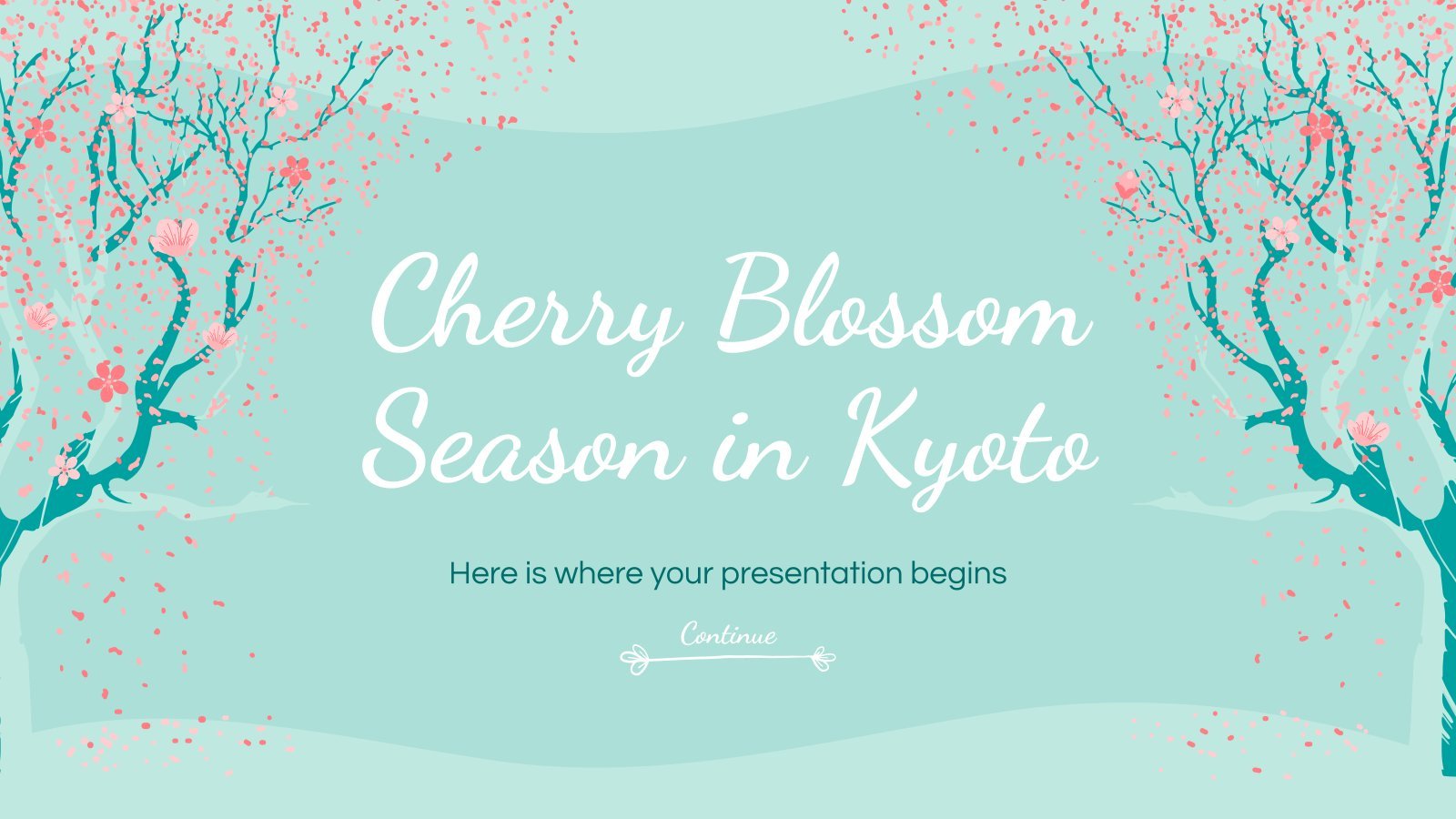
spring season
34 templates
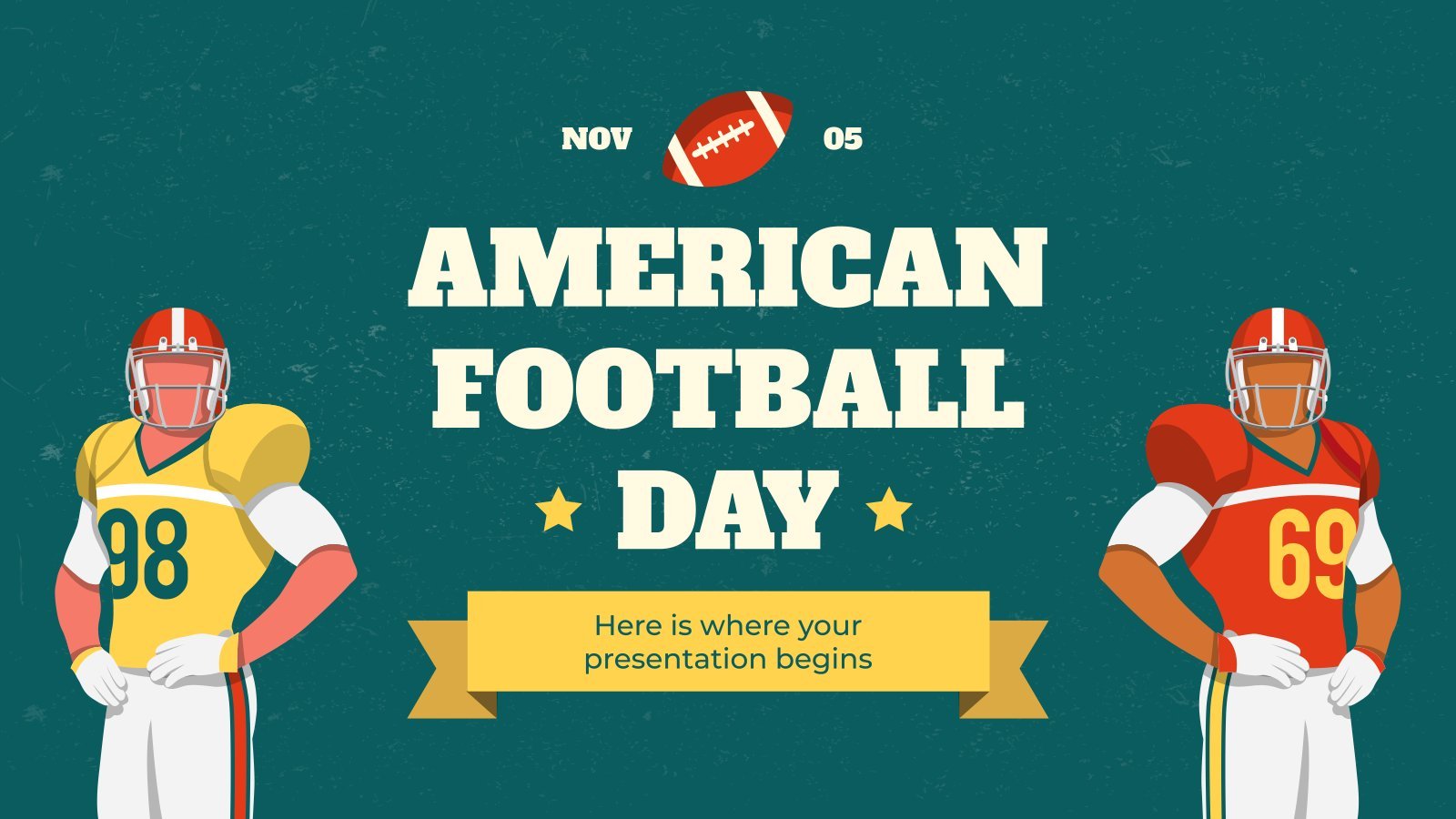
american football
16 templates
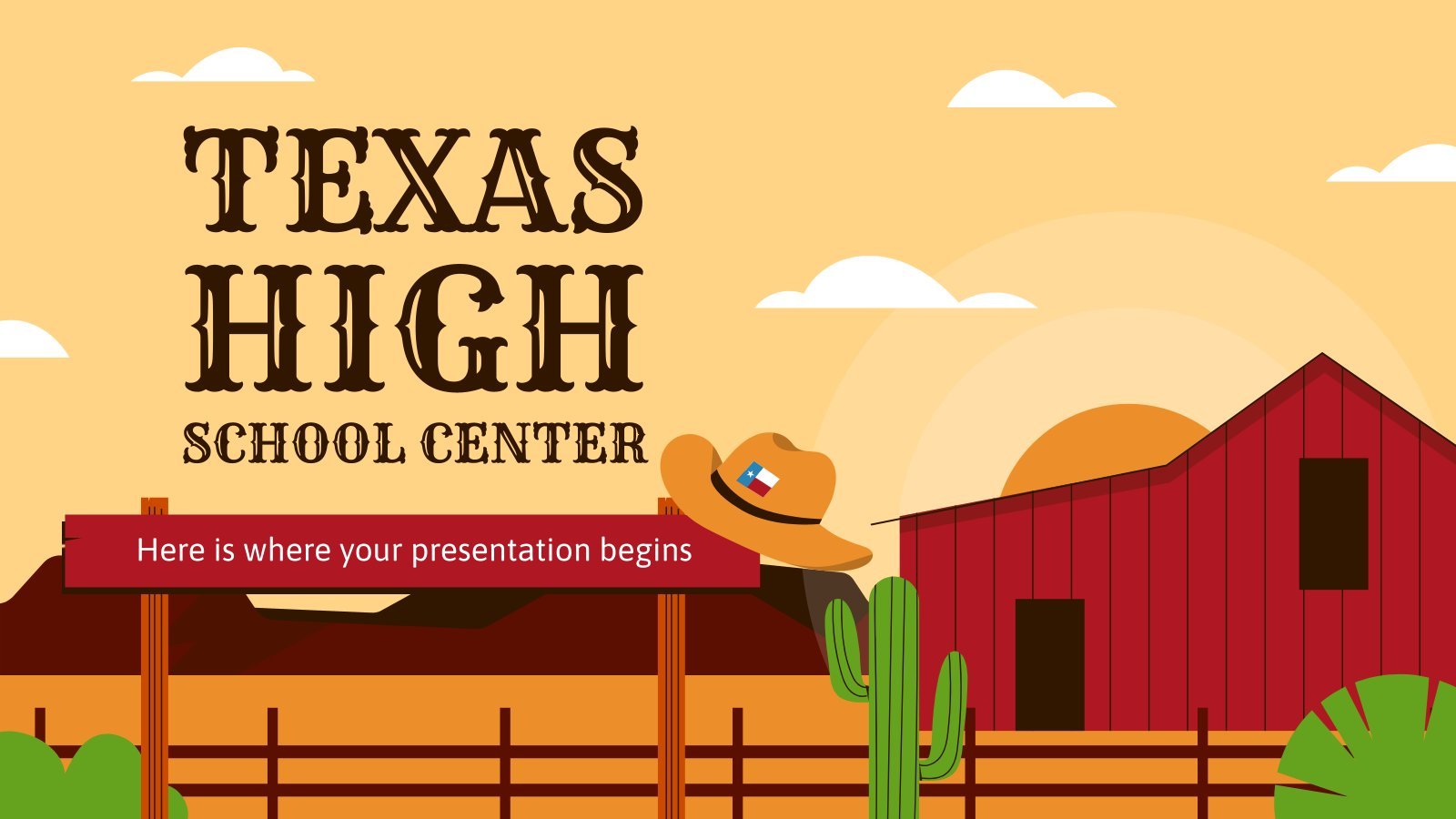
32 templates
Java Programming Workshop
Java programming workshop presentation, free google slides theme, powerpoint template, and canva presentation template.
Programming... it's hard, it must be said! It won't be after you use this presentation! If you are an expert in Java and programming, share your knowledge in the form of a workshop. This template is designed for you to include everything you know about Java and show it to other interested people. The slides feature black backgrounds decorated with gradient lines of pink, blue, and purple — which by words can mean nothing, but if you look at it, it looks like any programming language! Don't wait any longer to download this template and prepare your workshop! People need your knowledge!
Features of this template
- 100% editable and easy to modify
- 32 different slides to impress your audience
- Contains easy-to-edit graphics such as graphs, maps, tables, timelines and mockups
- Includes 500+ icons and Flaticon’s extension for customizing your slides
- Designed to be used in Google Slides, Canva, and Microsoft PowerPoint
- 16:9 widescreen format suitable for all types of screens
- Includes information about fonts, colors, and credits of the resources used
How can I use the template?
Am I free to use the templates?
How to attribute?
Attribution required If you are a free user, you must attribute Slidesgo by keeping the slide where the credits appear. How to attribute?
Related posts on our blog.
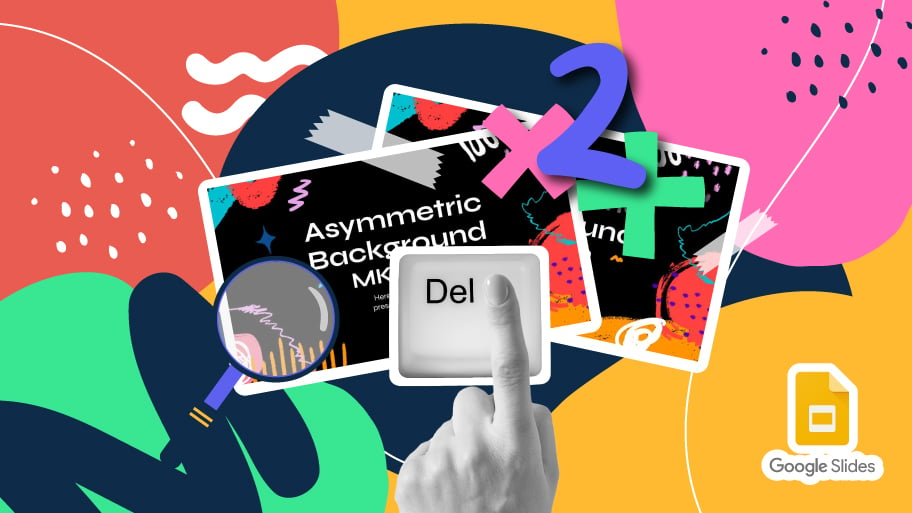
How to Add, Duplicate, Move, Delete or Hide Slides in Google Slides

How to Change Layouts in PowerPoint
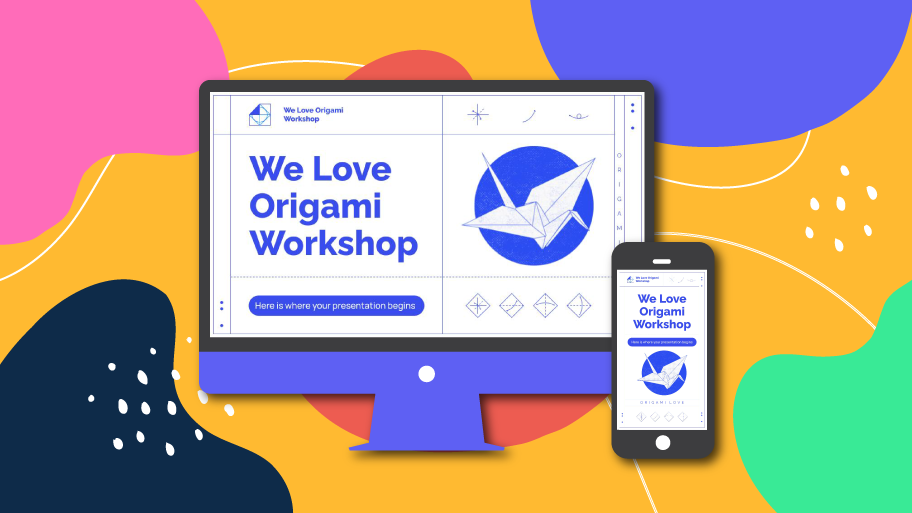
How to Change the Slide Size in Google Slides
Related presentations.
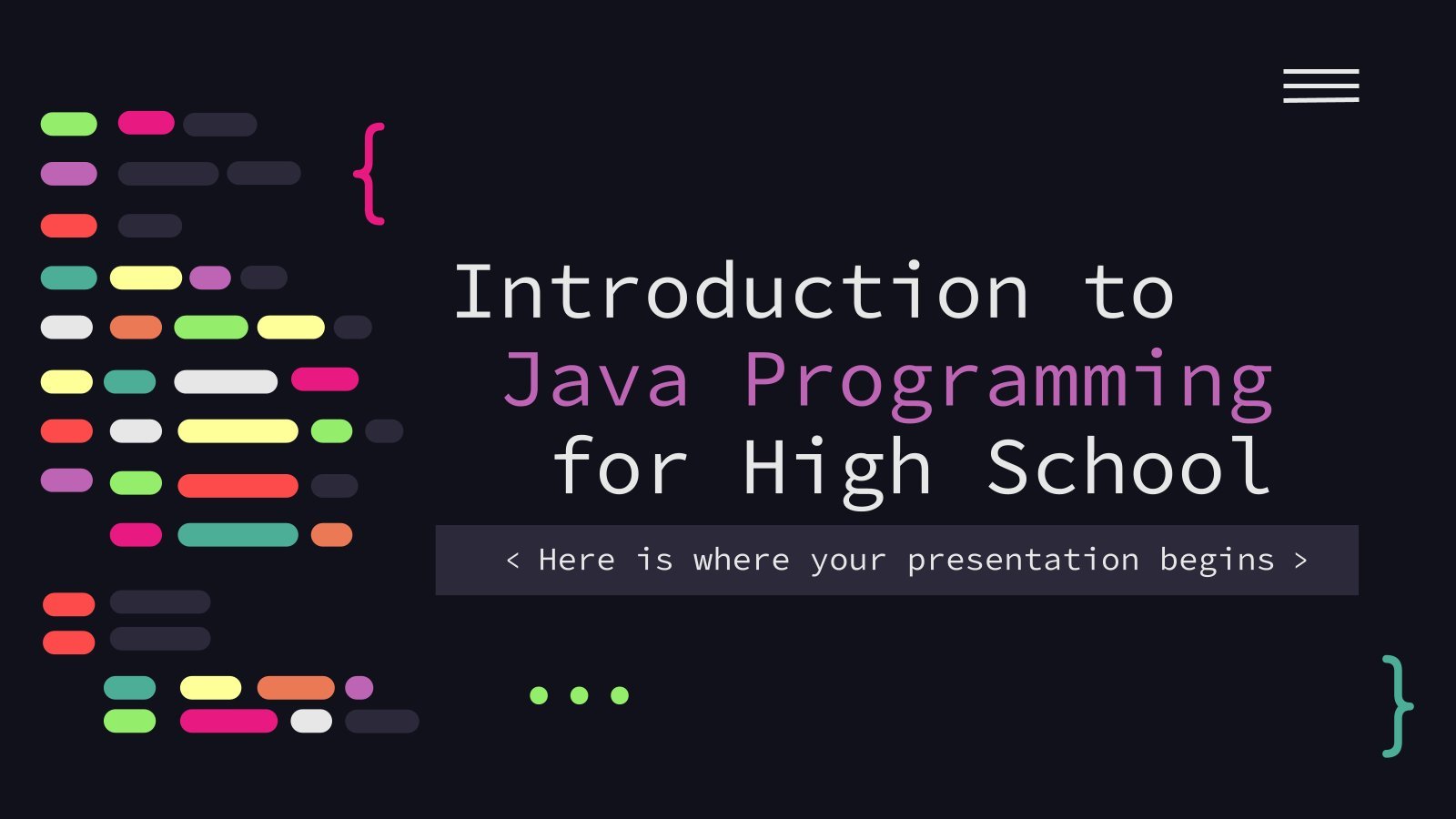
Premium template
Unlock this template and gain unlimited access
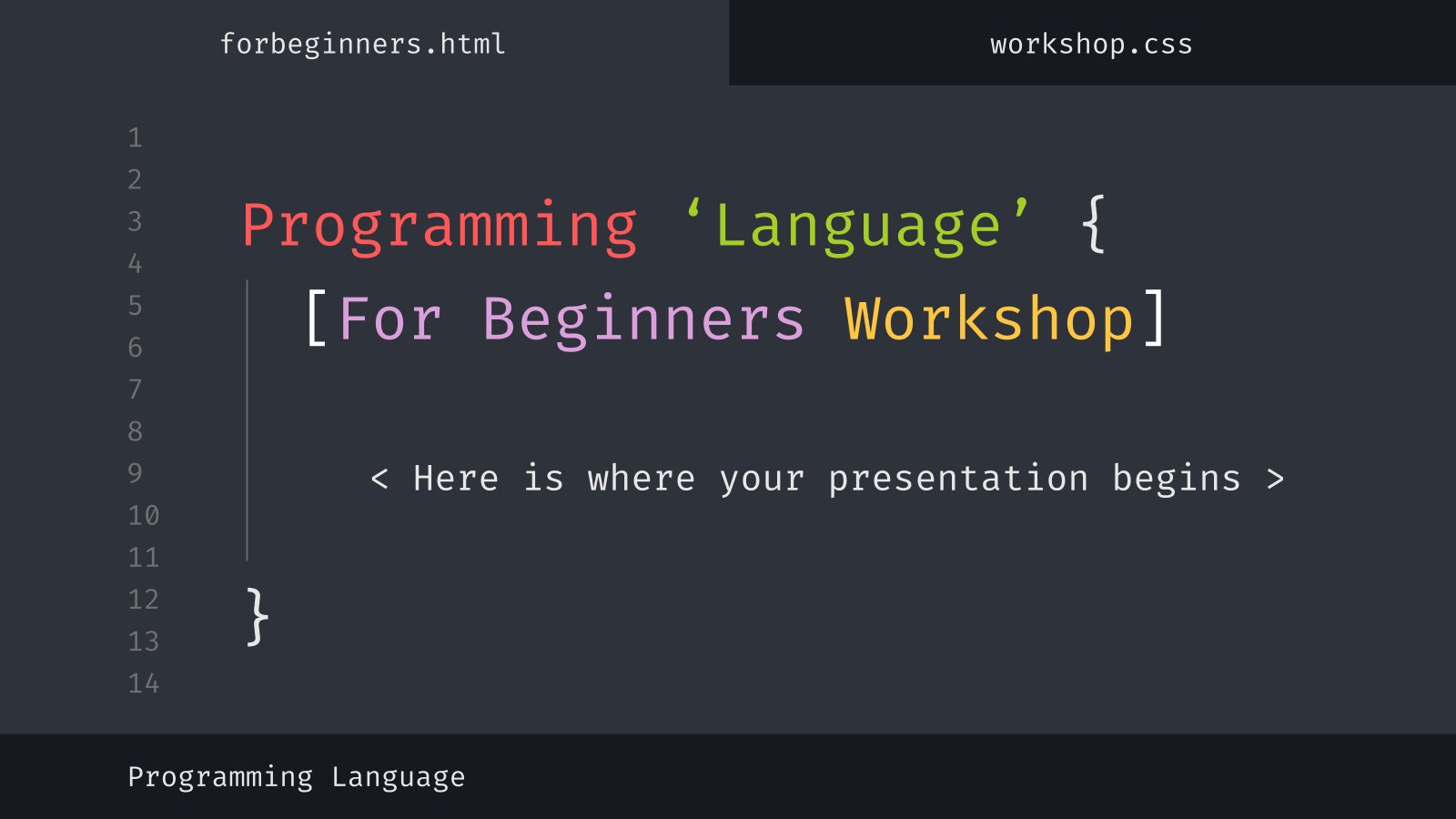
Editing PPT/PPTX Presentations Made Easy with Java

Presentation files come in different formats like PPT , PPTX , and ODP . You must be familiar with softwares like Microsoft PowerPoint, OpenOffice Impress, and Apple Keynote – they all work with these formats, helping us create amazing presentations. As developers, we have the power to programmatically edit these presentations in our applications. This article will guide you on how to edit PPT/PPTX presentations in Java using presentation editing API .
In this article, we’ll cover the following topics:
- Java API - Presentation Slides Editing
- Edit PPT/PPTX Presentations in Java
Java API for Presentations Editing and Automation #
In our examples, we’ll be relying on a powerful GroupDocs.Editor for Java library. This library serves as a presentation editing API, enabling developers to seamlessly load, edit, and save presentations in formats such as PPT, PPTX, and PDF.
Not only does this API handle presentations, but it also supports the editing of various other document types, including word-processing documents, spreadsheets, HTML, XML, JSON, TXT, TSV, and CSV formats.
To get started, you can download the necessary JAR file from the downloads section or incorporate the latest repository and dependency Maven configurations directly into your Java applications.
Editing PPT/PPTX Presentations in Java #
Once you have set up the API, you can swiftly start editing your presentation slides. Here are the steps to edit presentations in PPT/PPTX and other compatible formats:
Step 1: Load the Presentation #
Begin by loading the presentation. Provide the file path and password if the presentation is password-protected.
Step 2: Edit PPT/PPTX Presentation Slides with Java #
After loading, modify the presentation as needed. For instance, in the following Java code, I’m replacing occurrences of the word “documents” with “presentation” in a PPTX presentation.
Step 3: Save the Edited PowerPoint Presentation with Options #
When saving the edited content, you have the flexibility to set various options. These options include setting a password and configuring output format settings. In the code snippet below, I demonstrate how to apply these options and save the edited presentation as a password-protected PPTX file.
Complete Java Code Example #
For your convenience, here’s the complete Java code that was explained above. This code demonstrates how to edit a PowerPoint presentation and save it in PPTX format.
After running the above code, the output presentation will look like the image below. In this edited presentation, all occurrences of the word ‘documents’ have been replaced with ‘presentation’.

Output presentation - ‘documents’ occurrences are replaced by ‘presentation’
Feel free to test out the code and see the changes for yourself! If you have any questions or need further assistance, don’t hesitate to ask .
Conclusion #
In summary, we explored how to edit presentation slides in Java using a presentation editing API. This API allows you to visually edit your presentations using WYSIWYG editors. With this knowledge, you can create your own presentation editor or integrate the editing feature directly into your Java application.
For in-depth information, additional options, and examples, you can refer to the documentation and the GitHub repository . If you have any further questions, feel free to reach out to the support team on the forum .
Related Articles #
- Edit PowerPoint Presentations Online
- Word Documents Editing using Java
- Excel Spreadsheets Editing using Java
- How to Edit XML files in Java
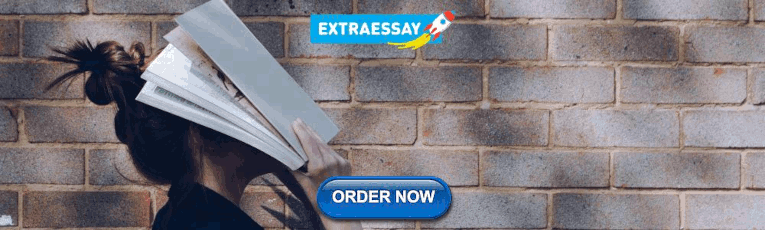
IMAGES
VIDEO
COMMENTS
When working with text inside a presentation, as in MS PowerPoint, we have to create the text box inside a slide, add a paragraph and then add the text to the paragraph: XSLFTextBox shape = slide.createTextBox(); XSLFTextParagraph p = shape.addNewTextParagraph(); XSLFTextRun r = p.addNewTextRun(); r.setText( "Baeldung" );
A new presentation is created. New slides are added. save the presentation as. FileOutputStream outputStream = new FileOutputStream(fileLocation); samplePPT.write(outputStream); outputStream.close(); We can write Text, create hyperlinks, and add images. And also the creation of a list, and table are all possible.
Overview of Free Spire.Presentation for Java Free Spire.Presentation for Java is a free Java PowerPoint API, by which developers can create, read, modify, write, convert and save PowerPoint document in Java applications without installing Microsoft Office. For more information of Free Spire.Presentation for Java, check here.
In this article, you'll learn how to create a simple PowerPoint document and insert basic elements (including text shape, image shape, list, and table) into it by using Free Spire.Presentation for Java, which is a free class library for processing PowerPoint documents in Java applications. The main tasks of this tutorial are as follows.
It's always interesting to be able to generate Microsoft PowerPoint PPTX presentations in an application to propose slideshows to users for example. In that ...
Add an Image in PowerPoint PPTX in Java. The following are the steps to add an image in a PowerPoint presentation using Java. Create an instance of the Presentation class and provide the PPTX file's path to its constructor. Get the reference of the slide in the ISlide object. Create an object of IPPImage class.
When you open the saved file, you will see that the Presentation opens with Microsoft PowerPoint and doesn't have any slides in it. This is the default behavior when creating a PowerPoint presentation with Apache POI. Add Slides to PowerPoint Presentation in Java. The above code sample created an empty PowerPoint presentation without any slides.
Spire.Presentation for Java is a professional PowerPoint API that enables developers to create, read, write, convert and save PowerPoint documents in Java Applications. As an independent Java library, Spire.Presentation doesn't need Microsoft PowerPoint to be installed on system.
DOWNLOAD. Spire.Presentation for Java is a professional PowerPoint API that enables developers to create, read, write, convert and save PowerPoint documents in Java Applications. As an independent Java library, Spire.Presentation doesn't need Microsoft PowerPoint to be installed on system. A rich set of features can be supported by Spire ...
Steps to Generate PowerPoint Presentation in Java. Download and install Aspose.Slides for Java from the Maven repository. Instantiate the Presentation class object to create an empty presentation. Create a blank slide and add that to the presentation slides collection. Using the AddAutoShape method, insert a Rectangle shape in the newly created ...
If you are an expert in Java and programming, share your knowledge in the form of a workshop. This template is designed for you to include everything you know about Java and show it to other interested people. The slides feature black backgrounds decorated with gradient lines of pink, blue, and purple — which by words can mean nothing, but if ...
The following are the steps to do so: Create an instance of Presentation class. Load the first PowerPoint presentation using Presentation.loadFromFile () method. Create an instance of Presentation ...
1. I'm working on a project in Java where I need to display a powerpoint presentation complete with transitions and animations. The Apache POI library provides a nice method of viewing previews of different slides statically, but it seems that any animations or transitions need to be implemented separately which, looking at the library seems to ...
Step 2: Edit PPT/PPTX Presentation Slides with Java. After loading, modify the presentation as needed. For instance, in the following Java code, I'm replacing occurrences of the word "documents" with "presentation" in a PPTX presentation. // Edit Presentation. Editor editor = new Editor( new FileInputStream( "path/presentation.pptx ...
1. Visualization can be done by visualizing each slide as a jpg image. You can use the Jacob java library for the conversion. This library expoits COM bridge and gets Microsoft Office Power Point to do the conversion (through save-as command). I've got PP2007: package jacobSample;
Intelligo Technologies. This document provides an overview of the Java programming language including how it works, its features, syntax, and input/output capabilities. Java allows software to run on any device by compiling code to bytecode that runs on a virtual machine instead of a particular computer architecture. It is an object-oriented ...
Introduction to java. Sep 14, 2011 • Download as PPTX, PDF •. 218 likes • 190,236 views. Veerabadra Badra. This PPT will help the students who were interested to learn java. Technology News & Politics. 1 of 22. Download now. Introduction to java - Download as a PDF or view online for free.
11. Introduction to Java - Bytecode, JIT A Java program is first compiled into the machine code that is understood by JVM. Such a code is called Byte Code. Although the details of the JVM will differ from platform to platform, they all interpret the Java Byte Code into executable native machine code. The Just In Time (JIT) compiler compiles the byte code into executable machine code in real ...
In C, almost everything is in functions In Java, almost everything is in classes There is often only one class per file There must be only one public class per file The file name must be the same as the name of that public class, but with a .java extension Java program layout A typical Java file looks like: import java.util.*; import java ...
ÐÏ à¡± á> þÿ K N ...
Introduction To Java. Java And J2EE (160 Slides) 6076 Views. Unlock a Vast Repository of Java And J2EE PPT Slides, Meticulously Curated by Our Expert Tutors and Institutes. Download Free and Enhance Your Learning!
Java is high-level programming language originally developed by Sun Microsystems and released in 1995. Java runs on a variety of platforms, such as Windows, Mac OS/ and the various versions of UNIX. According to SUN, 3 billi n devices run java. There are many devices where java is currently used. Some of them are as follows: Presentation by Abk ...
Activity : Create and Execute a Java project Writing a simple HelloWorld program in Java using Eclipse. Step l: Start Eclipse. Step 2: Create a new workspace called sample and 01. Project Eun Step 5: Select "Java" in the categ Java EE - Eclipse Eile Edit Navigate Search ti ct • Select "Java Project" in the project list.