- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
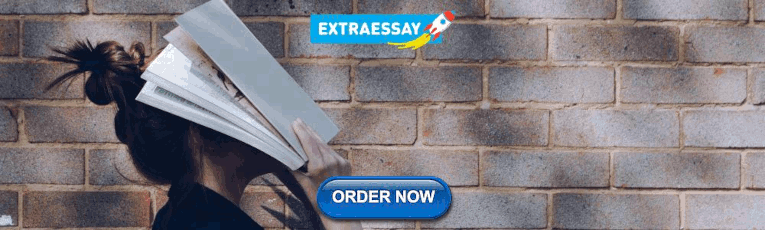
Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python Exercise: Practice makes you perfect in everything. This proverb always proves itself correct. Just like this, if you are a Python learner, then regular practice of Python exercises makes you more confident and sharpens your skills. So, to test your skills, go through these Python exercises with solutions.
Python is a widely used general-purpose high-level language that can be used for many purposes like creating GUI, web Scraping, web development, etc. You might have seen various Python tutorials that explain the concepts in detail but that might not be enough to get hold of this language. The best way to learn is by practising it more and more.
The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains. So if you are at any stage like beginner, intermediate or advanced this Python practice set will help you to boost your programming skills in Python.
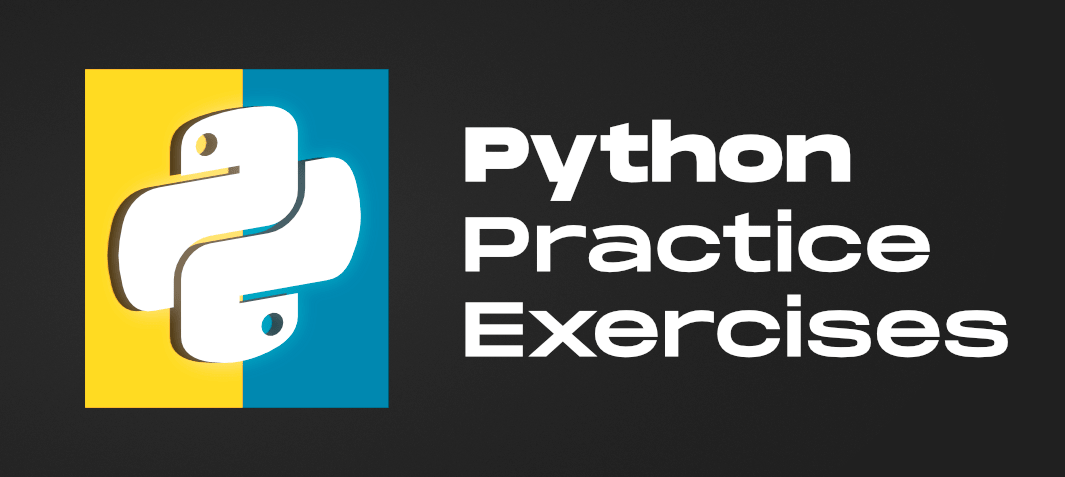
List of Python Programming Exercises
In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set.
Python List Exercises
- Python program to interchange first and last elements in a list
- Python program to swap two elements in a list
- Python | Ways to find length of list
- Maximum of two numbers in Python
- Minimum of two numbers in Python
>> More Programs on List
Python String Exercises
- Python program to check whether the string is Symmetrical or Palindrome
- Reverse words in a given String in Python
- Ways to remove i’th character from string in Python
- Find length of a string in python (4 ways)
- Python program to print even length words in a string
>> More Programs on String
Python Tuple Exercises
- Python program to Find the size of a Tuple
- Python – Maximum and Minimum K elements in Tuple
- Python – Sum of tuple elements
- Python – Row-wise element Addition in Tuple Matrix
- Create a list of tuples from given list having number and its cube in each tuple
>> More Programs on Tuple
Python Dictionary Exercises
- Python | Sort Python Dictionaries by Key or Value
- Handling missing keys in Python dictionaries
- Python dictionary with keys having multiple inputs
- Python program to find the sum of all items in a dictionary
- Python program to find the size of a Dictionary
>> More Programs on Dictionary
Python Set Exercises
- Find the size of a Set in Python
- Iterate over a set in Python
- Python – Maximum and Minimum in a Set
- Python – Remove items from Set
- Python – Check if two lists have atleast one element common
>> More Programs on Sets
- Python – Assigning Subsequent Rows to Matrix first row elements
- Python – Group similar elements into Matrix
>> More Programs on Matrices
>> More Programs on Functions
- Python | Find the Number Occurring Odd Number of Times using Lambda expression and reduce function
>> More Programs on Lambda
- Programs for printing pyramid patterns in Python
>> More Programs on Python Pattern Printing
- Python program to get Current Time
- Get Yesterday’s date using Python
- Python program to print current year, month and day
- Python – Convert day number to date in particular year
- Get Current Time in different Timezone using Python
>> More Programs on DateTime
>> More Programs on Python OOPS
- Python – Check if String Contain Only Defined Characters using Regex
>> More Programs on Python Regex
>> More Programs on Linked Lists
>> More Programs on Python Searching
- Python Program for Bubble Sort
- Python Program for QuickSort
- Python Program for Insertion Sort
- Python Program for Selection Sort
- Python Program for Heap Sort
>> More Programs on Python Sorting
- Program to Calculate the Edge Cover of a Graph
- Python Program for N Queen Problem
>> More Programs on Python DSA
- Read content from one file and write it into another file
- Write a dictionary to a file in Python
- How to check file size in Python?
- Find the most repeated word in a text file
- How to read specific lines from a File in Python?
>> More Programs on Python File Handling
- Update column value of CSV in Python
- How to add a header to a CSV file in Python?
- Get column names from CSV using Python
- Writing data from a Python List to CSV row-wise
>> More Programs on Python CSV
>> More Programs on Python JSON
- Python Script to change name of a file to its timestamp
>> More Programs on OS Module
- Python | Create a GUI Marksheet using Tkinter
- Python | ToDo GUI Application using Tkinter
- Python | GUI Calendar using Tkinter
- File Explorer in Python using Tkinter
- Visiting Card Scanner GUI Application using Python
>> More Programs on Python Tkinter
NumPy Exercises
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Replace NumPy array elements that doesn’t satisfy the given condition
- Get the maximum value from given matrix
>> More Programs on NumPy
Pandas Exercises
- Make a Pandas DataFrame with two-dimensional list | Python
- How to iterate over rows in Pandas Dataframe
- Create a pandas column using for loop
- Create a Pandas Series from array
- Pandas | Basic of Time Series Manipulation
>> More Programs on Python Pandas
>> More Programs on Web Scraping
- Download File in Selenium Using Python
- Bulk Posting on Facebook Pages using Selenium
- Google Maps Selenium automation using Python
- Count total number of Links In Webpage Using Selenium In Python
- Extract Data From JustDial using Selenium
>> More Programs on Python Selenium
- Number guessing game in Python
- 2048 Game in Python
- Get Live Weather Desktop Notifications Using Python
- 8-bit game using pygame
- Tic Tac Toe GUI In Python using PyGame
>> More Projects in Python
In closing, we just want to say that the practice or solving Python problems always helps to clear your core concepts and programming logic. Hence, we have designed this Python exercises after deep research so that one can easily enhance their skills and logic abilities.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Python Practice for Beginners: 15 Hands-On Problems

- online practice
Want to put your Python skills to the test? Challenge yourself with these 15 Python practice exercises taken directly from our Python courses!
There’s no denying that solving Python exercises is one of the best ways to practice and improve your Python skills . Hands-on engagement with the language is essential for effective learning. This is exactly what this article will help you with: we've curated a diverse set of Python practice exercises tailored specifically for beginners seeking to test their programming skills.
These Python practice exercises cover a spectrum of fundamental concepts, all of which are covered in our Python Data Structures in Practice and Built-in Algorithms in Python courses. Together, both courses add up to 39 hours of content. They contain over 180 exercises for you to hone your Python skills. In fact, the exercises in this article were taken directly from these courses!
In these Python practice exercises, we will use a variety of data structures, including lists, dictionaries, and sets. We’ll also practice basic programming features like functions, loops, and conditionals. Every exercise is followed by a solution and explanation. The proposed solution is not necessarily the only possible answer, so try to find your own alternative solutions. Let’s get right into it!
Python Practice Problem 1: Average Expenses for Each Semester
John has a list of his monthly expenses from last year:
He wants to know his average expenses for each semester. Using a for loop, calculate John’s average expenses for the first semester (January to June) and the second semester (July to December).
Explanation
We initialize two variables, first_semester_total and second_semester_total , to store the total expenses for each semester. Then, we iterate through the monthly_spending list using enumerate() , which provides both the index and the corresponding value in each iteration. If you have never heard of enumerate() before – or if you are unsure about how for loops in Python work – take a look at our article How to Write a for Loop in Python .
Within the loop, we check if the index is less than 6 (January to June); if so, we add the expense to first_semester_total . If the index is greater than 6, we add the expense to second_semester_total .
After iterating through all the months, we calculate the average expenses for each semester by dividing the total expenses by 6 (the number of months in each semester). Finally, we print out the average expenses for each semester.
Python Practice Problem 2: Who Spent More?
John has a friend, Sam, who also kept a list of his expenses from last year:
They want to find out how many months John spent more money than Sam. Use a for loop to compare their expenses for each month. Keep track of the number of months where John spent more money.
We initialize the variable months_john_spent_more with the value zero. Then we use a for loop with range(len()) to iterate over the indices of the john_monthly_spending list.
Within the loop, we compare John's expenses with Sam's expenses for the corresponding month using the index i . If John's expenses are greater than Sam's for a particular month, we increment the months_john_spent_more variable. Finally, we print out the total number of months where John spent more money than Sam.
Python Practice Problem 3: All of Our Friends
Paul and Tina each have a list of their respective friends:
Combine both lists into a single list that contains all of their friends. Don’t include duplicate entries in the resulting list.
There are a few different ways to solve this problem. One option is to use the + operator to concatenate Paul and Tina's friend lists ( paul_friends and tina_friends ). Afterwards, we convert the combined list to a set using set() , and then convert it back to a list using list() . Since sets cannot have duplicate entries, this process guarantees that the resulting list does not hold any duplicates. Finally, we print the resulting combined list of friends.
If you need a refresher on Python sets, check out our in-depth guide to working with sets in Python or find out the difference between Python sets, lists, and tuples .
Python Practice Problem 4: Find the Common Friends
Now, let’s try a different operation. We will start from the same lists of Paul’s and Tina’s friends:
In this exercise, we’ll use a for loop to get a list of their common friends.
For this problem, we use a for loop to iterate through each friend in Paul's list ( paul_friends ). Inside the loop, we check if the current friend is also present in Tina's list ( tina_friends ). If it is, it is added to the common_friends list. This approach guarantees that we test each one of Paul’s friends against each one of Tina’s friends. Finally, we print the resulting list of friends that are common to both Paul and Tina.
Python Practice Problem 5: Find the Basketball Players
You work at a sports club. The following sets contain the names of players registered to play different sports:
How can you obtain a set that includes the players that are only registered to play basketball (i.e. not registered for football or volleyball)?
This type of scenario is exactly where set operations shine. Don’t worry if you never heard about them: we have an article on Python set operations with examples to help get you up to speed.
First, we use the | (union) operator to combine the sets of football and volleyball players into a single set. In the same line, we use the - (difference) operator to subtract this combined set from the set of basketball players. The result is a set containing only the players registered for basketball and not for football or volleyball.
If you prefer, you can also reach the same answer using set methods instead of the operators:
It’s essentially the same operation, so use whichever you think is more readable.
Python Practice Problem 6: Count the Votes
Let’s try counting the number of occurrences in a list. The list below represent the results of a poll where students were asked for their favorite programming language:
Use a dictionary to tally up the votes in the poll.
In this exercise, we utilize a dictionary ( vote_tally ) to count the occurrences of each programming language in the poll results. We iterate through the poll_results list using a for loop; for each language, we check if it already is in the dictionary. If it is, we increment the count; otherwise, we add the language to the dictionary with a starting count of 1. This approach effectively tallies up the votes for each programming language.
If you want to learn more about other ways to work with dictionaries in Python, check out our article on 13 dictionary examples for beginners .
Python Practice Problem 7: Sum the Scores
Three friends are playing a game, where each player has three rounds to score. At the end, the player whose total score (i.e. the sum of each round) is the highest wins. Consider the scores below (formatted as a list of tuples):
Create a dictionary where each player is represented by the dictionary key and the corresponding total score is the dictionary value.
This solution is similar to the previous one. We use a dictionary ( total_scores ) to store the total scores for each player in the game. We iterate through the list of scores using a for loop, extracting the player's name and score from each tuple. For each player, we check if they already exist as a key in the dictionary. If they do, we add the current score to the existing total; otherwise, we create a new key in the dictionary with the initial score. At the end of the for loop, the total score of each player will be stored in the total_scores dictionary, which we at last print.
Python Practice Problem 8: Calculate the Statistics
Given any list of numbers in Python, such as …
… write a function that returns a tuple containing the list’s maximum value, sum of values, and mean value.
We create a function called calculate_statistics to calculate the required statistics from a list of numbers. This function utilizes a combination of max() , sum() , and len() to obtain these statistics. The results are then returned as a tuple containing the maximum value, the sum of values, and the mean value.
The function is called with the provided list and the results are printed individually.
Python Practice Problem 9: Longest and Shortest Words
Given the list of words below ..
… find the longest and the shortest word in the list.
To find the longest and shortest word in the list, we initialize the variables longest_word and shortest_word as the first word in the list. Then we use a for loop to iterate through the word list. Within the loop, we compare the length of each word with the length of the current longest and shortest words. If a word is longer than the current longest word, it becomes the new longest word; on the other hand, if it's shorter than the current shortest word, it becomes the new shortest word. After iterating through the entire list, the variables longest_word and shortest_word will hold the corresponding words.
There’s a catch, though: what happens if two or more words are the shortest? In that case, since the logic used is to overwrite the shortest_word only if the current word is shorter – but not of equal length – then shortest_word is set to whichever shortest word appears first. The same logic applies to longest_word , too. If you want to set these variables to the shortest/longest word that appears last in the list, you only need to change the comparisons to <= (less or equal than) and >= (greater or equal than), respectively.
If you want to learn more about Python strings and what you can do with them, be sure to check out this overview on Python string methods .
Python Practice Problem 10: Filter a List by Frequency
Given a list of numbers …
… create a new list containing only the numbers that occur at least three times in the list.
Here, we use a for loop to iterate through the number_list . In the loop, we use the count() method to check if the current number occurs at least three times in the number_list . If the condition is met, the number is appended to the filtered_list .
After the loop, the filtered_list contains only numbers that appear three or more times in the original list.
Python Practice Problem 11: The Second-Best Score
You’re given a list of students’ scores in no particular order:
Find the second-highest score in the list.
This one is a breeze if we know about the sort() method for Python lists – we use it here to sort the list of exam results in ascending order. This way, the highest scores come last. Then we only need to access the second to last element in the list (using the index -2 ) to get the second-highest score.
Python Practice Problem 12: Check If a List Is Symmetrical
Given the lists of numbers below …
… create a function that returns whether a list is symmetrical. In this case, a symmetrical list is a list that remains the same after it is reversed – i.e. it’s the same backwards and forwards.
Reversing a list can be achieved by using the reverse() method. In this solution, this is done inside the is_symmetrical function.
To avoid modifying the original list, a copy is created using the copy() method before using reverse() . The reversed list is then compared with the original list to determine if it’s symmetrical.
The remaining code is responsible for passing each list to the is_symmetrical function and printing out the result.
Python Practice Problem 13: Sort By Number of Vowels
Given this list of strings …
… sort the list by the number of vowels in each word. Words with fewer vowels should come first.
Whenever we need to sort values in a custom order, the easiest approach is to create a helper function. In this approach, we pass the helper function to Python’s sorted() function using the key parameter. The sorting logic is defined in the helper function.
In the solution above, the custom function count_vowels uses a for loop to iterate through each character in the word, checking if it is a vowel in a case-insensitive manner. The loop increments the count variable for each vowel found and then returns it. We then simply pass the list of fruits to sorted() , along with the key=count_vowels argument.
Python Practice Problem 14: Sorting a Mixed List
Imagine you have a list with mixed data types: strings, integers, and floats:
Typically, you wouldn’t be able to sort this list, since Python cannot compare strings to numbers. However, writing a custom sorting function can help you sort this list.
Create a function that sorts the mixed list above using the following logic:
- If the element is a string, the length of the string is used for sorting.
- If the element is a number, the number itself is used.
As proposed in the exercise, a custom sorting function named custom_sort is defined to handle the sorting logic. The function checks whether each element is a string or a number using the isinstance() function. If the element is a string, it returns the length of the string for sorting; if it's a number (integer or float), it returns the number itself.
The sorted() function is then used to sort the mixed_list using the logic defined in the custom sorting function.
If you’re having a hard time wrapping your head around custom sort functions, check out this article that details how to write a custom sort function in Python .
Python Practice Problem 15: Filter and Reorder
Given another list of strings, such as the one below ..
.. create a function that does two things: filters out any words with three or fewer characters and sorts the resulting list alphabetically.
Here, we define filter_and_sort , a function that does both proposed tasks.
First, it uses a for loop to filter out words with three or fewer characters, creating a filtered_list . Then, it sorts the filtered list alphabetically using the sorted() function, producing the final sorted_list .
The function returns this sorted list, which we print out.
Want Even More Python Practice Problems?
We hope these exercises have given you a bit of a coding workout. If you’re after more Python practice content, head straight for our courses on Python Data Structures in Practice and Built-in Algorithms in Python , where you can work on exciting practice exercises similar to the ones in this article.
Additionally, you can check out our articles on Python loop practice exercises , Python list exercises , and Python dictionary exercises . Much like this article, they are all targeted towards beginners, so you should feel right at home!
You may also like
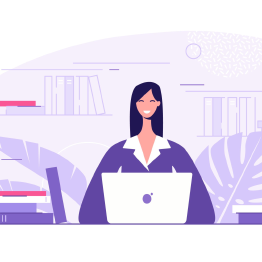
How Do You Write a SELECT Statement in SQL?
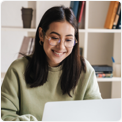
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
- MapReduce Algorithm
- Linear Programming using Pyomo
- Networking and Professional Development for Machine Learning Careers in the USA
- Predicting Employee Churn in Python
- Airflow Operators

Solving Transportation Problem using Linear Programming in Python
Learn how to use Python PuLP to solve transportation problems using Linear Programming.
In this tutorial, we will broaden the horizon of linear programming problems. We will discuss the Transportation problem. It offers various applications involving the optimal transportation of goods. The transportation model is basically a minimization model.
The transportation problem is a type of Linear Programming problem. In this type of problem, the main objective is to transport goods from source warehouses to various destination locations at minimum cost. In order to solve such problems, we should have demand quantities, supply quantities, and the cost of shipping from source and destination. There are m sources or origin and n destinations, each represented by a node. The edges represent the routes linking the sources and the destinations.
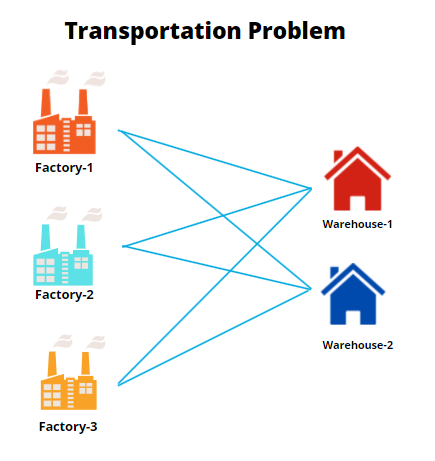
In this tutorial, we are going to cover the following topics:
Transportation Problem
The transportation models deal with a special type of linear programming problem in which the objective is to minimize the cost. Here, we have a homogeneous commodity that needs to be transferred from various origins or factories to different destinations or warehouses.
Types of Transportation problems
- Balanced Transportation Problem : In such type of problem, total supplies and demands are equal.
- Unbalanced Transportation Problem : In such type of problem, total supplies and demands are not equal.
Methods for Solving Transportation Problem:
- NorthWest Corner Method
- Least Cost Method
- Vogel’s Approximation Method (VAM)
Let’s see one example below. A company contacted the three warehouses to provide the raw material for their 3 projects.
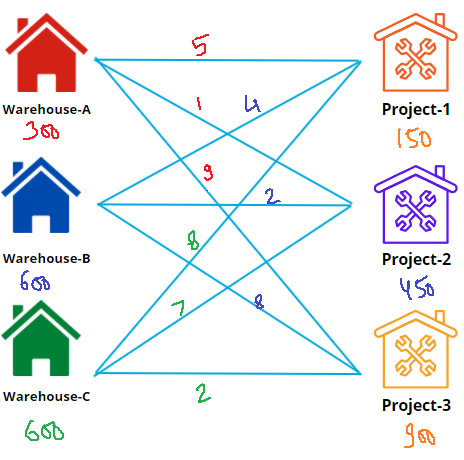
This constitutes the information needed to solve the problem. The next step is to organize the information into a solvable transportation problem.
Formulate Problem
Let’s first formulate the problem. first, we define the warehouse and its supplies, the project and its demands, and the cost matrix.
Initialize LP Model
In this step, we will import all the classes and functions of pulp module and create a Minimization LP problem using LpProblem class.
Define Decision Variable
In this step, we will define the decision variables. In our problem, we have various Route variables. Let’s create them using LpVariable.dicts() class. LpVariable.dicts() used with Python’s list comprehension. LpVariable.dicts() will take the following four values:
- First, prefix name of what this variable represents.
- Second is the list of all the variables.
- Third is the lower bound on this variable.
- Fourth variable is the upper bound.
- Fourth is essentially the type of data (discrete or continuous). The options for the fourth parameter are LpContinuous or LpInteger .
Let’s first create a list route for the route between warehouse and project site and create the decision variables using LpVariable.dicts() the method.
Define Objective Function
In this step, we will define the minimum objective function by adding it to the LpProblem object. lpSum(vector)is used here to define multiple linear expressions. It also used list comprehension to add multiple variables.
In this code, we have summed up the two variables(full-time and part-time) list values in an additive fashion.
Define the Constraints
Here, we are adding two types of constraints: supply maximum constraints and demand minimum constraints. We have added the 4 constraints defined in the problem by adding them to the LpProblem object.
Solve Model
In this step, we will solve the LP problem by calling solve() method. We can print the final value by using the following for loop.
From the above results, we can infer that Warehouse-A supplies the 300 units to Project -2. Warehouse-B supplies 150, 150, and 300 to respective project sites. And finally, Warehouse-C supplies 600 units to Project-3.
In this article, we have learned about Transportation problems, Problem Formulation, and implementation using the python PuLp library. We have solved the transportation problem using a Linear programming problem in Python. Of course, this is just a simple case study, we can add more constraints to it and make it more complicated. In upcoming articles, we will write more on different optimization problems such as transshipment problem, assignment problem, balanced diet problem. You can revise the basics of mathematical concepts in this article and learn about Linear Programming in this article .
- Solving Cargo Loading Problem using Integer Programming in Python
- Solving Blending Problem in Python using Gurobi
You May Also Like
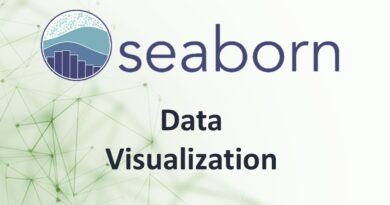
Data Visualization using Seaborn
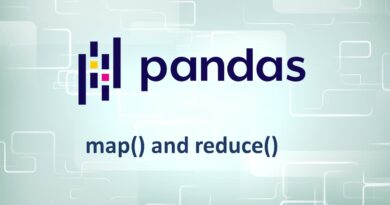
Pandas map() and reduce() Operations
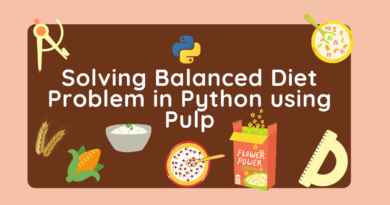
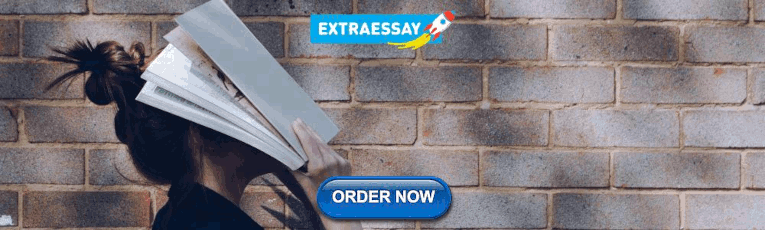
Solving Balanced Diet Problem in Python using PuLP
- [email protected]
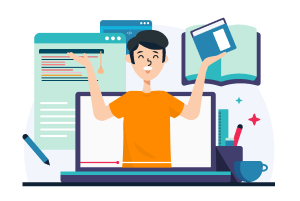
What’s New ?
The Top 10 favtutor Features You Might Have Overlooked

- Don’t have an account Yet? Sign Up
Remember me Forgot your password?
- Already have an Account? Sign In
Lost your password? Please enter your email address. You will receive a link to create a new password.
Back to log-in
By Signing up for Favtutor, you agree to our Terms of Service & Privacy Policy.
Python Assignment Help: Expert Solutions and Guidance
Are Python assignments giving you a hard time? Stop stressing and get the help you need now! Our experts are available 24/7 to provide immediate assistance with all your Python coding questions and projects.
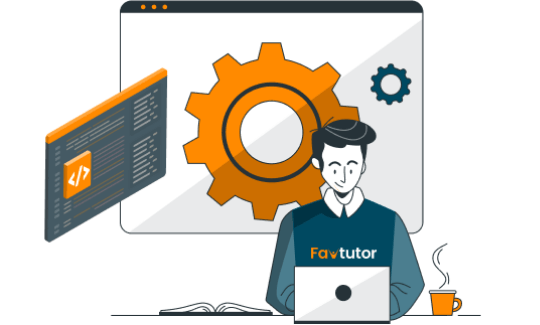
Why Choose FavTutor for Python Help?
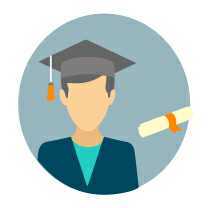
Python experts with 5+ years of experience
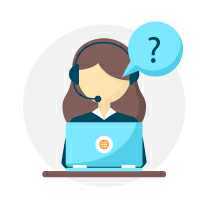
24/7 support for all your Python questions
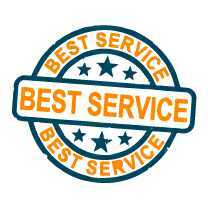
On-time delivery, even for urgent deadlines
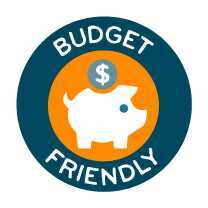
Budget-friendly prices starting at just $35/hour
Python homework and assignment help.
Our expert Python programmers are here to help you with any aspect of your Python learning, from coding assignments and debugging to concept clarification and exam prep. Whatever Python challenge you're facing, we've got you covered. Chat with us now for personalized support!
.jpg)
Do you need Python assignment help online?
If you require immediate Python programming assistance, FavTutor can connect you with Python experts for online help right now. Python, as an object-oriented language, is highly sought after among students. However, with multiple classes, exams, and tight assignment deadlines, it can be challenging to manage everything. If you find yourself struggling with these demands, FavTutor offers a solution with our online Python homework help service, designed to relieve your stress.
Our top-level experts are dedicated to conducting comprehensive research on your assignments and delivering effective solutions. With 24/7 Python online support available, students can confidently work towards completing their assignments and improving their grades. Don't let Python assignments overwhelm you—let FavTutor be your reliable partner in academic success.
About Python
Python is a high-level, interpreted, object-oriented programming language with dynamic semantics. It's a language that's known for its friendliness and ease of use, making it a favorite among both beginners and seasoned developers.
What sets Python apart is its simplicity. You can write code that's clean and easy to understand, which comes in handy when you're working on projects with others or need to revisit your own code later on.
But Python isn't just easy to read; it's also incredibly powerful. It comes with a vast standard library that's like a toolkit filled with pre-built modules and functions for all sorts of tasks. Whether you're doing web development, data analysis, or even diving into machine learning, Python has you covered.
Speaking of web development, Python has some fantastic frameworks like Django and Flask that make building web applications a breeze. And when it comes to data science and artificial intelligence, Python shines with libraries like NumPy, pandas, and TensorFlow.
Perhaps the best thing about Python is its community. No matter where you are in your coding journey, you'll find a warm and welcoming community ready to help. There are tutorials, forums, and a wealth of resources to support you every step of the way. Plus, Python's open-source nature means it's constantly evolving and improving.
Key Topics in Python
Let us understand some of the key topics of Python programming language below:
- Variables and Data Types: Python allows you to store and manipulate data using variables. Common data types include integers (whole numbers), floats (decimal numbers), strings (text), and boolean values (True or False).
- Conditional Statements: You can make decisions in your Python programs using conditional statements like "if," "else," and "elif." These help you execute different code blocks based on specific conditions.
- Loops: Loops allow you to repeat a set of instructions multiple times. Python offers "for" and "while" loops for different types of iterations.
- Functions: Functions are reusable blocks of code that perform specific tasks. You can define your own functions or use built-in ones from Python's standard library.
- Lists and Data Structures: Lists are collections of items that can hold different data types. Python also offers other data structures like dictionaries (key-value pairs) and tuples (immutable lists).
- File Handling: Python provides tools to work with files, including reading from and writing to them. This is essential for tasks like data manipulation and file processing.
- Exception Handling: Exceptions are errors that can occur during program execution. Python allows you to handle these exceptions gracefully, preventing your program from crashing.
- Object-Oriented Programming (OOP): Python supports OOP principles, allowing you to create and use classes and objects. This helps in organizing and structuring code for complex projects.
- Modules and Libraries: Python's extensive standard library and third-party libraries offer a wide range of pre-written code to extend Python's functionality. You can import and use these modules in your projects.
- List Comprehensions: List comprehensions are concise ways to create lists based on existing lists. They simplify operations like filtering and transforming data.
- Error Handling: Properly handling errors is crucial in programming. Python provides mechanisms to catch and manage errors, ensuring your programs run smoothly.
- Regular Expressions: Regular expressions are powerful tools for pattern matching and text manipulation. Python's "re" module allows you to work with regular expressions.
- Web Development with Flask or Django: Python is commonly used for web development, with frameworks like Flask and Django. These frameworks simplify the process of building web applications.
- Data Science with Pandas and NumPy: Python is widely used in data science. Libraries like Pandas and NumPy provide tools for data manipulation, analysis, and scientific computing.
- Machine Learning with TensorFlow or Scikit-Learn: Python is a popular choice for machine learning and artificial intelligence. Libraries like TensorFlow and Scikit-Learn offer machine learning algorithms and tools.
Advantages and Features of Python Programming
Below are some of the features and advantages of python programming:
- It's Free and Open Source: Python won't cost you a dime. You can download it from the official website without opening your wallet. That's a win-win!
- It's Easy to Learn: Python's syntax is simple and easy to understand. It's almost like writing in plain English. If you're new to coding, Python is a fantastic starting point.
- It's Super Versatile: Python can do it all. Whether you're building a website, analyzing data, or even diving into artificial intelligence, Python has your back.
- It's Fast and Flexible: Python might seem easygoing, but it's no slouch in terms of speed. Plus, Python code can run on pretty much any computer, making it super flexible.
- A Library Wonderland: Python's library collection is like a magical forest. There are libraries for just about anything you can think of: web development, data science, and more. It's like having a vast collection of pre-made tools at your disposal.
- Scientific Superpowers: Python isn't just for developers; it's a favorite among scientists too. It has specialized libraries for data analysis and data mining, making it a powerhouse for researchers.
- Code Interpreted in Real Time: Python doesn't wait around. It interprets and runs your code line by line. If it finds an issue, it stops and lets you know what went wrong, which can be a real lifesaver when debugging.
- Dynamic Typing: Python is smart. It figures out the data type of your variables as it goes along, so you don't have to declare them explicitly. It's like a built-in problem solver.
- Object-Oriented Magic: Python supports object-oriented programming. It lets you organize your code into neat, reusable objects, making complex problems more manageable.
Can You Help with my Python Homework or Assignment?
Yes, we provide 24x7 python assignment help online for students all around the globe. If you are struggling to manage your assignment commitments due to any reason, we can help you. Our Python experts are committed to delivering accurate assignments or homework help within the stipulated deadlines. The professional quality of our python assignment help can provide live assistance with your homework and assignments. They will provide plagiarism-free work at affordable rates so that students do not feel any pinch in their pocket. So, get the work delivered on time and carve the way to your dream grades. Chat now for python live help to get rid of all your python queries.
How Do We Help, Exactly?
At FavTutor, we believe that the best way to learn Python is through a combination of expert guidance and hands-on practice. That's why we offer a unique two-pronged approach to Python assignment help: 1) Detailed, Step-by-Step Solutions - Our experienced Python tutors will provide you with carefully crafted, easy-to-follow solutions to your assignments. These solutions not only give you the answers you need but also break down the thought process and logic behind each step, helping you understand the "why" behind the code.
2) Live 1:1 Tutoring Sessions - To cement your understanding, we pair our written solutions with live, one-on-one tutoring sessions. During these personalized sessions, our tutor will:
- Walk you through the solution, explaining each step in detail
- Answer any questions you have and clarify complex concepts
- Help you practice applying the concepts to new problems
- Offer tips, best practices, and insights from real-world Python experience
This powerful combination of detailed solutions and live tutoring ensures that you not only complete your Python assignments successfully but also gain a deep, practical understanding of the language that will serve you well in your future coding endeavors.
Challenges Faced By Students While Working on Python Assignments
While Python is often touted as a beginner-friendly programming language, newcomers can run into a few hurdles that might make it seem a tad tricky. Let's explore some of these challenges:
1) Setting Up Your Workspace
Before you even start coding, you need to set up your development environment just right. Now, for beginners, this can be a bit of a puzzle. Figuring out all the necessary configurations can sometimes feel like a maze, and it might even leave you a bit demotivated at the beginning of your coding journey.
2) Deciding What to Code
Computers are like really obedient but somewhat clueless pets. You have to spell out every single thing for them. So, here's the thing: deciding what to tell your computer in your code can be a head-scratcher. Every line you type has a purpose, and that can get a bit overwhelming. It's like giving really detailed instructions to your pet, but in this case, your pet is a computer.
3) Dealing with Compiler Errors
Now, imagine this: You've written your code, hit that magic "run" button, and... oops! Compiler errors pop up on your screen. For beginners, this can be a heart-sinking moment. But hey, don't worry, it happens to the best of us.
4) Hunting Down Bugs
Making mistakes is perfectly normal, especially when you're just starting out. Syntax errors, in particular, can be a real pain. However, the good news is that with practice and time, these errors become less frequent. Debugging, or finding and fixing these issues, is a crucial part of learning to code. It helps you understand what can go wrong and how to write better code in the future.
If you find yourself grappling with these challenges or any others while working on your Python homework, don't sweat it. Our team of Python programmers is here to lend a helping hand. At Favtutor, we offer top-notch Python assignment help. Our experts, hailing from all around the globe, can provide efficient solutions to address your questions and challenges, all at prices that won't break the bank. So, don't hesitate to reach out for assistance and conquer your Python assignment obstacles.

Reasons to choose FavTutor
- Top rated experts- We pride in our programemrs who are experts in various subjects and provide excellent help to students for all their assignments, and help them secure better grades.
- Specialize in International education- We have programmers who work with students studying in the USA and Canada, and who understand the ins and outs of international education.
- Prompt delivery of assignments- With an extensive research, FavTutor aims to provide a timely delivery of your assignments. You will get adequate time to check your homework before submitting them.
- Student-friendly pricing- We follow an affordable pricing structure, so that students can easily afford it with their pocket money and get value for each penny they spend.
- Round the clock support- Our experts provide uninterrupted support to the students at any time of the day, and help them advance in their career.
3 Steps to Connect-
Get help in your assignment within minutes with these three easy steps:
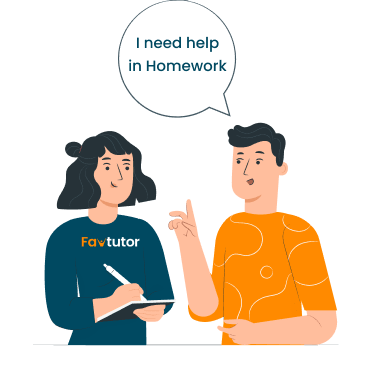
Click on the Signup button below & register your query or assignment.
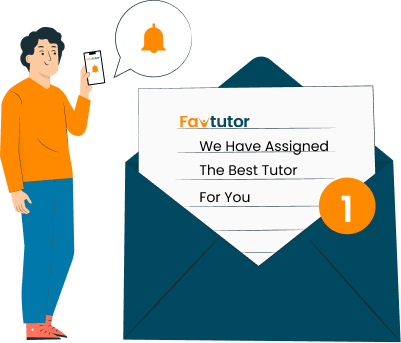
You will be notified when we have assigned the best expert for your query.
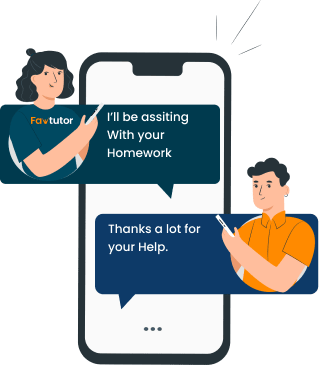
Voila! You can start chatting with python expert and get started with your learning.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Truck assignement problem solved with mixed integer linear programming
krflorian/truck_assignment
Folders and files, repository files navigation, assignment problem.
The assignment problem arises in a number of different industries. The most prominent is assigning Long Haul Trucks to their Loads. This project is heavily influenced by "A Stochastic Formulation of the Dynamic Assignment Problem, with an Application to Truckload Motor Carriers" by Warren B. Powell (Princton University 1996)
- install pipenv
- install all dependencies via pipenv
- choose the kernel named "truck_assignment_*" for the jupyter notebook
Truck Assignment
The algorithm assigns trucks to loads depending on their haversine distance to those Loads. We want to minimize the distance between the truck/load pairs
- Truck and Load Positions

- Assignments

- Jupyter Notebook 98.9%
- Python 1.1%

Build a Fast Food Order Taker in Python
Learn python while making a program that takes your order.
By Matthew Hull on 2021-04-20
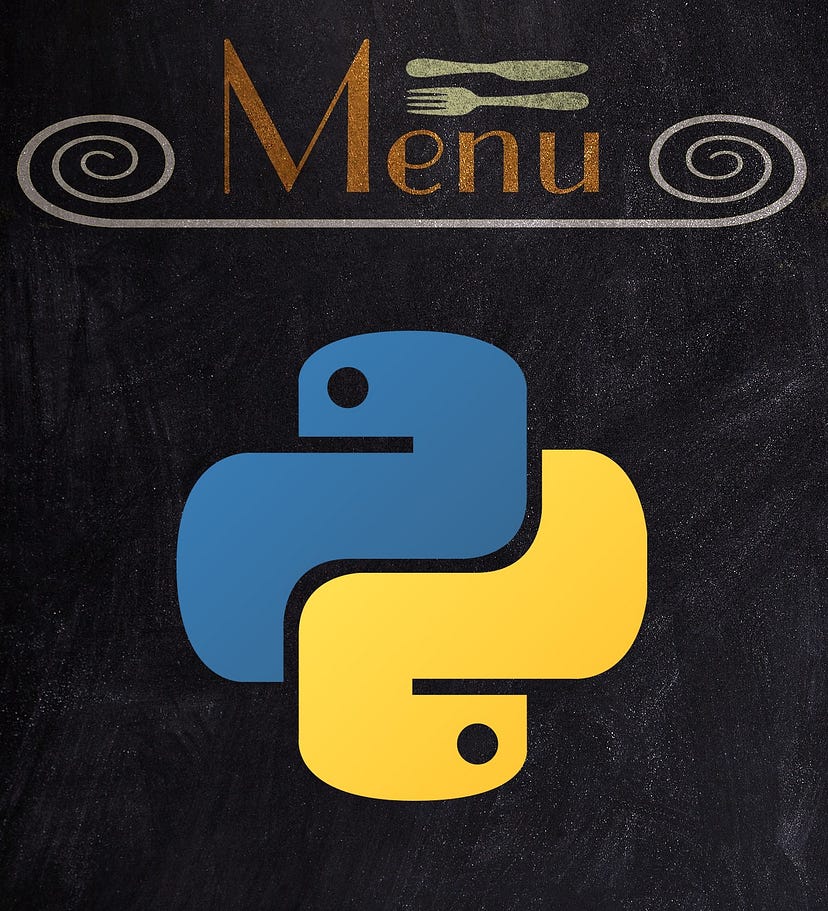
Hello again! Welcome to part 3 in a series for beginning Python! In Part 2 , we covered constants, functions, lists, and string formatting. Today we will be creating a simple order taker using only a few new concepts. If you are completely new to Python, check out Part 1 to start your journey!
Today’s Topic: Order Taker Bot
Concepts we will learn:
Creating multiple functions
raising errors
Just like last time, we are going to start with some introductory code in Programmiz :
Notice something different with our MENU constant? It’s using curly brackets instead of the brackets we used for lists in Part 2! By using curly brackets, and still having comma separated values inside, we are initializing a set. Sets are different from lists in a few ways but most notably, they are faster for searching and can only contain unique values.
Let’s focus on the first difference: searching for a value. With today’s project, we will be taking an order from your input and checking if what you ordered is actually on the menu. If MENU was a list, and we ordered a “shake”, it would go through the list, checking if soda, fries, burger, and finally shake matched what we ordered. Since MENU is a set, it’s able to instead check if the hash, or a unique number, of the value is in the set, finding shake immediately.
In less technical terms, a list is the equivalent of having a deck of playing cards face-down, and needing to flip each card over to see if it’s the card you are looking for. A set is the equivalent of having all the cards spread out and face-up, allowing you to quickly find the card you are looking for. This doesn’t matter much right now with 6 values but knowing the best data structure for a current task is very important, like whether or not you get hired important.
Take a look at the new function, get_order() . The logic is as follows: create an empty list (note the brackets), execute a while loop that is always true, print “what can I get for you?” to the console, wait for an input, check if the order is actually on the menu, and if it is then add it to the current order list. If not, print “I’m sorry, we don’t serve that.” to the console. What happens after that? The logic starts right back at the top of the while loop! The purpose of a while loop is to continue looping through the same bit of code until the condition of the while loop is no longer true. As you can see, while True: will never be not true, so we have to find another way out of this loop or risk looping for eternity! Or at least until we close the program.
Naming Conventions
As a quick side note, notice how my functions more or less state the intent of the function in the title? A function ideally is supposed to handle one to two tasks and then return its result, so naming what it’s doing should be fairly easy. In Python, underscores are used to separate words for functions and variables (we will discuss class and object naming later).
In other languages, there are entire lessons and interview questions devoted to proper searching. Python has them too, just less often due to what you see here: if order_item in MENU: . If what we ordered is in the MENU constant, then the if statement is true and our order is added to the current order. That’s it, we’ve searched the menu and added our order. Alternatively, if we order a banana, we get the response that it isn’t served and nothing is added to the current_order variable.
Escaping the While Loop
In order to escape a while loop, we have 3 options: return which takes us out of the function, set the condition to False (not possible here since it’s True), or use a new command called break . There are two new commands in loops that we haven’t discussed: continue and break . continue is self explanatory: stop where we are and go back to the top of the loop. break cuts us out of the loop and allows us to move on in the function, past the while loop. In this project, we are going to make sure we are finished with the order, and return with our current_order variable. Take a look:
A new function has been added called is_order_complete() and it asks the user if they are finished with their order. If they are, then we return our current order to main() . If not, then we continue within the while loop. If the user inputs anything other than yes/no, then we raise an error, or essentially flip the table on the program, and exit with the message “invalid input”. Our program doesn’t mess around with input handling! Back in get_order() , we check if is_order_complete() returned True or False, and return current_order if so. Notice the continue right above it? If the user orders something not on the menu, we don’t want to ask “is that all?” but instead restart the loop and ask what they want to order.
After our user has ordered what they want from the menu, all that’s needed now is to read back what they ordered and get them to the next window. That means one more function and we are done! Check it out:
Repeating the order
By this point in our program, we have finished getting the order and returned to main() , having placed our current_order list into the order variable. We now have the output_order(order_list) function to pick up that order, print “Okay, so you want”, and print out each item in that order list. If you didn’t notice, the variable names between what goes in the function argument and what is used in the function itself do not have to be the same. What is also new is the for loop. A for loop allows us to go through each value in the data structure (list/set/etc), assign the value to a variable, and perform logic on each one individually. This loop is just like the while loop, except that it is constrained to the data structure being looped through, ending once the last value has been read. As you see here, all we do is print each order item, one per line.
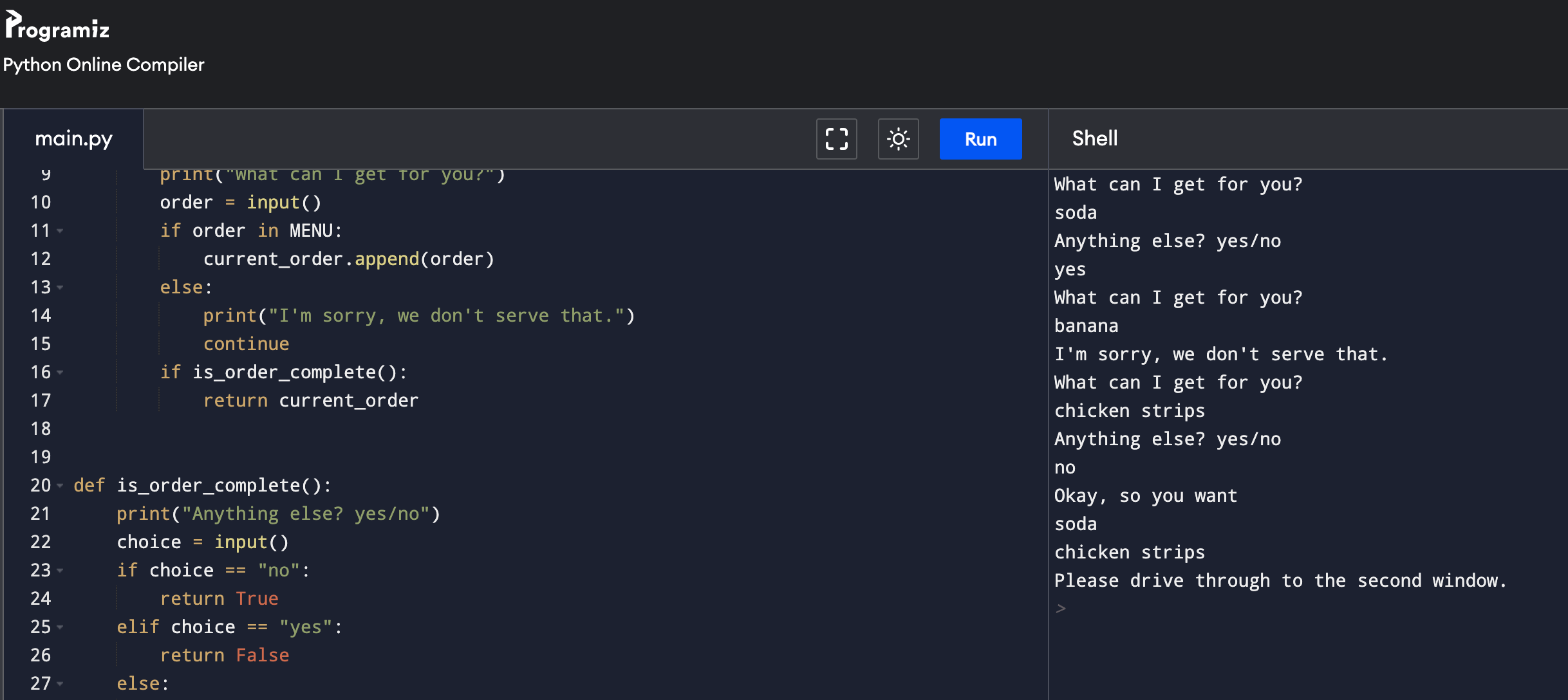
What do you mean you don’t sell bananas!?
Last, we print out to drive to the next window, and we are done! Feel free to play around with different menu items or extend it to include pricing! How? Dictionaries of course! What are dictionaries? Find out in Part 4 . See ya there!
Continue Learning
How to speed up pandas data operations using vectorized operations, how to remove image background using python.
Remove images’ backgrounds using the Python library Rembg.
A solution to boost Python speed 1000x times
People said Python is slow, how slow it can be
Greedy Algorithm in Python
Using the Greedy Algorithm to find a solution to a graph-modeled problem
Unit Conversion in Python
Dimensional analysis and unit conversion of physical quantities with SymPy
How to Find Minimum and Maximum Values in a List Using Python
A tutorial on finding minimum and maximum values in a list using Python.
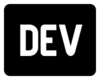
DEV Community

Posted on Jun 14, 2021
Solution: Maximum Units on a Truck
This is part of a series of Leetcode solution explanations ( index ). If you liked this solution or found it useful, please like this post and/or upvote my solution post on Leetcode's forums .
Leetcode Problem #1710 ( Easy ): Maximum Units on a Truck
Description:.
( Jump to : Solution Idea || Code : JavaScript | Python | Java | C++ )
You are assigned to put some amount of boxes onto one truck . You are given a 2D array boxTypes , where boxTypes[i] = [numberOfBoxesi, numberOfUnitsPerBoxi] : numberOfBoxes i is the number of boxes of type i . numberOfUnitsPerBox i is the number of units in each box of the type i . You are also given an integer truckSize , which is the maximum number of boxes that can be put on the truck. You can choose any boxes to put on the truck as long as the number of boxes does not exceed truckSize . Return the maximum total number of units that can be put on the truck .
Example 1: Input: boxTypes = [[1,3],[2,2],[3,1]], truckSize = 4 Output: 8 Explanation: There are: - 1 box of the first type that contains 3 units. - 2 boxes of the second type that contain 2 units each. - 3 boxes of the third type that contain 1 unit each. You can take all the boxes of the first and second types, and one box of the third type. The total number of units will be = (1 * 3) + (2 * 2) + (1 * 1) = 8. Example 2: Input: boxTypes = [[5,10],[2,5],[4,7],[3,9]], truckSize = 10 Output: 91
Constraints:
1 <= boxTypes.length <= 1000 1 <= numberOfBoxesi, numberOfUnitsPerBoxi <= 1000 1 <= truckSize <= 10^6
( Jump to : Problem Description || Code : JavaScript | Python | Java | C++ )
For this problem, we simply need to prioritize the more valuable boxes first. To do this, we should sort the boxtypes array ( B ) in descending order by the number of units per box ( B[i][1] ).
Then we can iterate through B and at each step, we should add as many of the boxes as we can, until we reach the truck size ( T ). We should add the number of boxes added multiplied by the units per box to our answer ( ans ), and decrease T by the same number of boxes .
Once the truck is full ( T == 0 ), or once the iteration is done, we should return ans .
- Time Complexity: O(N log N) where N is the length of B , for the sort
- Space Complexity: O(1)
Javascript Code:
( Jump to : Problem Description || Solution Idea )
Python Code:
Top comments (0).
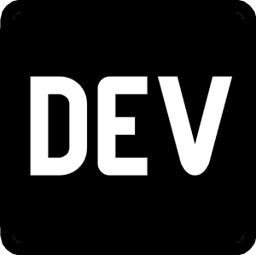
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

How to Become a React Native Superstar
Paulo Messias - May 23
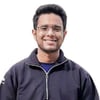
Understanding the Basics of Quantum Programming
Kartik Mehta - May 23

Top ChatGPT Prompts Every Developer Should Know
Abdullah - May 22
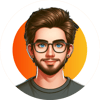
Asymptotic Notations: A Comprehensive Guide
Prince M - May 12
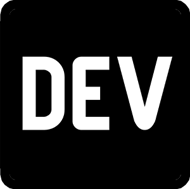
We're a place where coders share, stay up-to-date and grow their careers.
Creating a Four-step Transportation Model in Python
A four-step transportation model predicts the traffic load on a network given data about a region. These models are used to evaluate the impacts of land-use and transportation projects. In this example, we will create a model representing California as if it acted as a city. To get started, first we will import the necessary libraries. All of these can be installed from pip.
Step 0 Gather Data
First we need some data about the study area. Supply data is the transportation network including roads, public transportation schedules, etc. To keep things simple, we are going to assume the transport network is a line connecting the centroid of each zone to the centroid of each other zone.
Supply Data
At this point we can plot our zones and see how they look:
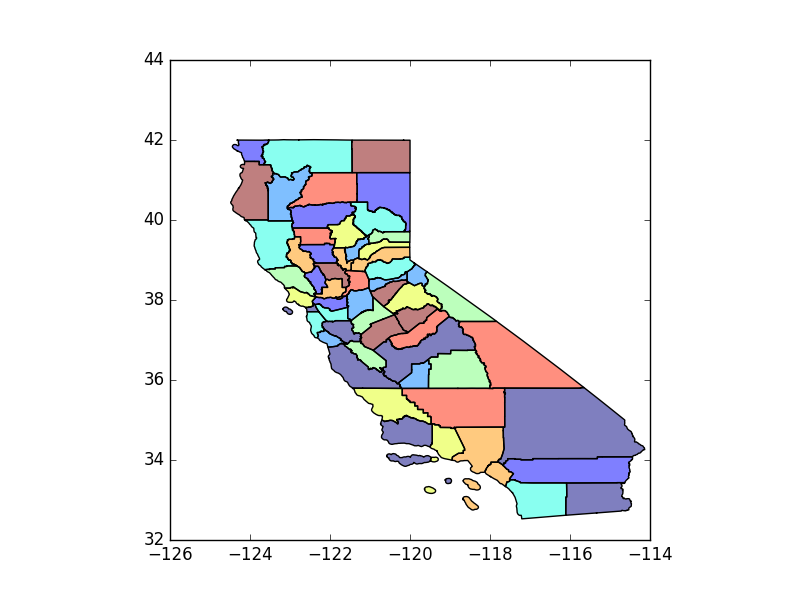
Demand Data
Demand dats is the users of the transportation network. For passenger models, demand data is typically census data including residential locations, work locations, school location, etc. For freight models, demand data could be tons of freight, number of bananas, etc. In this case we will study workers' home locations (from the 2015 American Community Survey (ACS) 5-Year Data) and employees' locations (from the Bureau of Labor Statistics (BLS)). (The BLS API seems to be quite slow...)
The second to last line makes sure the sums of Production and Attraction are equal. Iterative Proportional Fitting in Trip Distribution will fail if they are not. We assume people with multiple jobs are spread thoughout the study area. Now let's take a look at where our commuters live and work:
We can easily see that Alameda and Alpine Counties see an influx of commuters during the day and Butte and Calaveras Counties are the opposite.
Step 1 Trip Generation
Trip Generation is where we compute the numbers for Production and Attraction. We completed this above.
Step 2 Trip Distribution
In Trip Distribution we use a Gravity Model to calculate a cost matrix representing the cost of travel between each pair of zones. First, we create a simple cost function. Then we use that function to calculate our cost matrix by interating through all possible zone pairs. Then we can use our cost matrix to distribute our trips across our study area. Changing the beta parameter adjusts the Friction of Distance . Beta will vary based on the units of distance. We use a Haversine Function to calculate distances in kilometers (or miles) from geographic coordinates. The Trip Distribution function uses Iterative Proportional Fitting to assign trips from our Production and Attraction arrays to our matrix.
If we take a look at the trips table we can see that most trips stay inside each county, but some go quite far. Origin zones are on the left. Destination zones are on the top.
Step 3 Mode Choice
At this point we have a matrix of the number of trips from each zone to each zone. Next we split those trips across the available modes, in this case walking, cycling, and driving. For this we create a Utility Function that describes the utility gained from the trip minus the utility lost due to travel time, cost, and other negative factors associated with the mode. We then use this utility function to determine the probability of taking each mode for each zone pair. Similar to Trip Distribution, we use these probabilities to compute a matrix. We can then multiply our trip matrix by the probability matrices to get the number of trips between each zone pair using a given mode.
Now we can look at the number of driving trips between each zone pair.
Step 4 Route Assignment
At this point we have a matrix of all trips from each zone to each zone by mode. We could use this information to calculate mode share percentages. However, we would also like to see how the trips look on the transportation network. For that, we create a graph to represent the network. Then we calculate the shortest path for each trip and add all the trips to the network ignoring capacity contraints. Last, we can visualize our trips and see how the traffic is distributed. The width of the line between centroids show the volume of traffic.
Here are our trips:
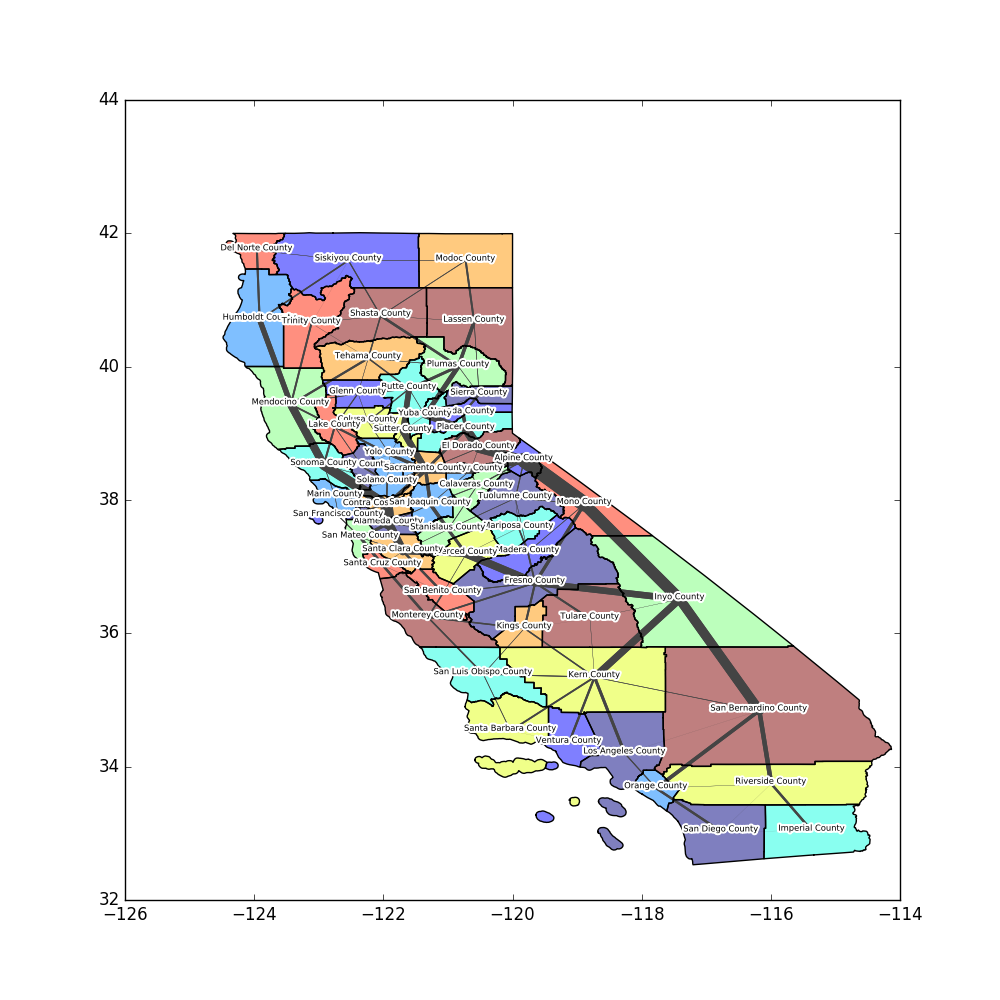
Obviously the scale of this example is quite ridiculous. Because the cost of travel is so low, our model is telling us that there will be many long distance trips. And because we used centroid-to-centroid routes, there is no concept of geography. This makes the route through the east of the state the fastest path north to south. However, this is the simple method used by transportation planners around the world to predict travel patterns. If you'd like to play with the parameters, here are all the functions:
That's all folks. Questions? Improvements?
Reply to this article .


Truck haulage simulation animation in Python
- Linnart Felkl
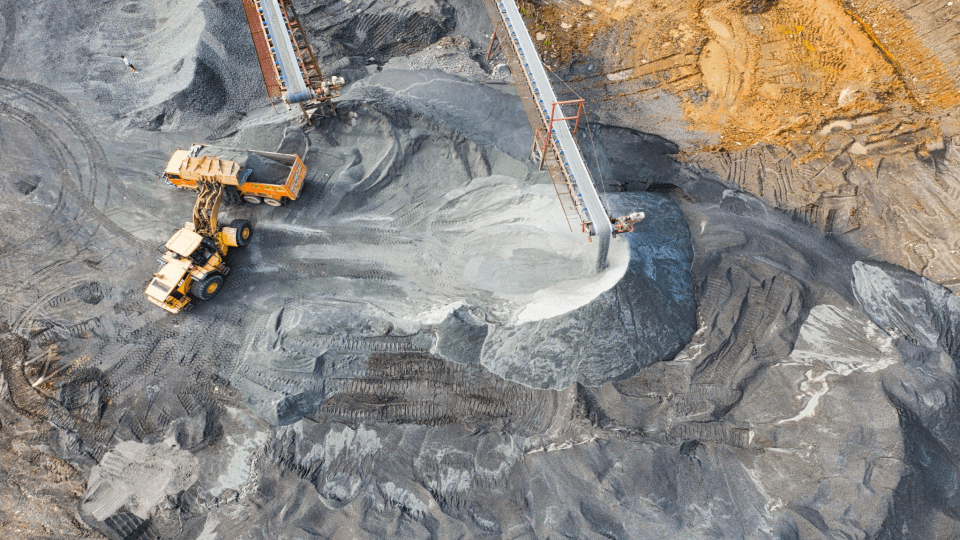
In this article I will share a discrete-event simulation animation example in Python. More specifically a truck haul transport simulation animation for a mine, using SimPy and DesViz in Python. This example, and the DesViz module, was developed by Prof. Paul Corry and his team and I am resharing his example in this post. Using DesViz SimPy model developers can animate their simulation model .
Below is an animation of a SimPy truck haul simulation model for mining operations.
The example is documented and available in Paul Corry’s GitHub repository: https://github.com/corryp/DesViz
How can DesViz be used for simulation animation in Python?
Citing directly from the DesViz documentation:
DesViz is a collection of Python classes and functions facilitating asynchronous animation for discrete event simulation ( DES ) models. It is built on top of the Pyglet package which provides the underlying graphics functionality. DesViz allows a DES model to write a csv file which is later interpreted by DesViz to configure and move sprites representing background and foreground objects in the simulation. Each line of the csv file gives the simulation time, an animation instruction and set of arguments relating to that instruction. These instructions provide a compact method to specify sprite appearance and movements in ways that are useful in a DES context. DesViz documentation, by Paul Corry
SimPy developers can use DesViz to animate their simulation model , in a two-step approach. First, they must use the DesViz library to generate and store animation data. Next, the animation is used for rendering an animation.
Here are some examples of what you can animate with DesViz:
- movements from pixel point to pixel point or along predefined paths, with automatic object orientations
- adjusting object orientations, i.e. animate object rotations
- define master-slave relationships between objects for animation purposes, e.g. truck (master) and trailer (slave)
- progress bars, either static or attached to another object (i.e. moving together with the associated object)
- labeling, annotation, and background images
- defined animation speed (frame interval, i.e. fps – frames per second)
Under the hood, DesViz populates a database (csv-file) with defined animation instructions. These instructions must be implemented into the simulation application itself. The underlying database is populated during simulation execution and is then used for rendering the animation itself. For this, DesViz provides are range of classes, methods, and functions.
Related content
If you are interested in learning more about discrete-event simulation and related model implementation in Python you might be interested in the following blog posts:
- Link: Discrete-event simulation software list
- Link : Simulation methods for SCM analysts
- Link: Discrete-event simulation procedure model
- Link : Job shop SimPy Python simulation
- Link : Visualizing stats with salabim (DES, Python)
If you are interested in learning more about simulation and its use cases in mining industry you may be interested in the following articles:
- Link: Open-cast mine simulation for better planning
- Link : Simulation and its use-cases in mining industry
- Link : Tackling blending problems in mining industry
- Link : Solving the iron ore blending problem
- Link : Analytics in the steel production value chain

Data scientist focusing on simulation, optimization and modeling in R, SQL, VBA and Python
You May Also Like

Service facility allocation – an optimization

Warehouse receiving process simulation

Scheduling a CNC job shop machine park
Leave a reply.
- Default Comments
- Facebook Comments
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
This site uses Akismet to reduce spam. Learn how your comment data is processed .
- Entries feed
- Comments feed
- WordPress.org
Privacy Overview
- How it works
- Homework answers
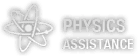
Answer to Question #336261 in Python for sandhya
you are given two strings N &K your goal is to determine the smallest substring of N that contains all the characters in K If no substring present in N print No matches found
note: if a character is repeated multiple time in K your substring should also contain that character repeated the same no.of times
i/p:the 1st line input two strings N&K
stealen lent
tomato tomatho
no matches found
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Discount sale:it is a summer discount sale in mumbai and all the local shops have put up various off
- 2. candies:Rose bought 3 boxes of candies each box contains candies only of one specific flavorbox1:blu
- 3. Write a program to print the following,InputThe first line contains a string representing a scramble
- 4. Number of moves:you are given a nxn square chessboard with one bishop and k number of obstacles plac
- 5. ProgramWrite a program to print the following, Given a word W and pattern P, you need to check wheth
- 6. Elle Joy VasquezPreliminary Test 02Create Python function that checks whether a passed string is PAL
- 7. Elle Joy VasquezPreliminary Test 04Create a Python function that takes a list of n integers and retu
- Programming
- Engineering
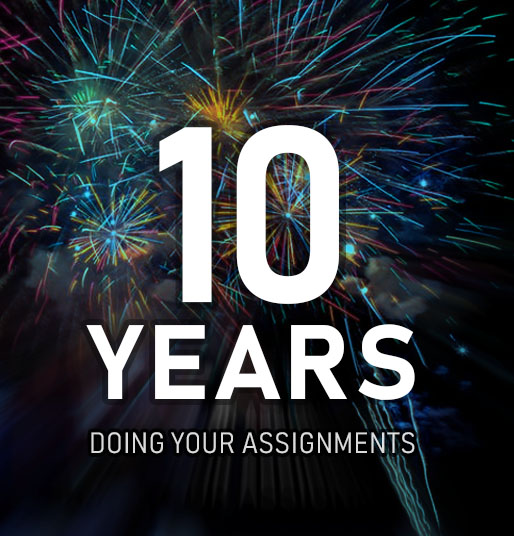
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
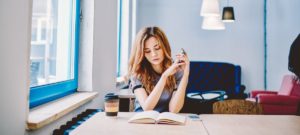
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
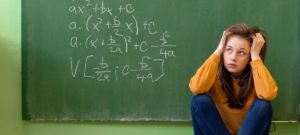
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
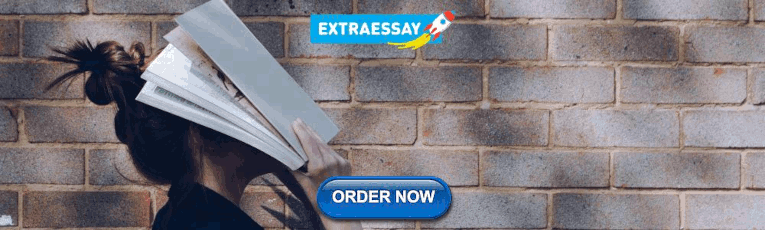
IMAGES
VIDEO
COMMENTS
Question #350992. Write a Python program to demonstrate Polymorphism. 1. Class Vehicle with a parameterized function Fare, that takes input value as fare and. returns it to calling Objects. 2. Create five separate variables Bus, Car, Train, Truck and Ship that call the Fare. function. 3.
The following graph shows the four rooms in the apartment, the truck, how many boxes are initially in which rooms, and how the rooms are connected: On each turn, you can either move or push a box into an adjacent location, in any direction: north, south, east, west. When this story begins, you have just parked the truck.
ALL Answered. Question #350996. Python. Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not. 11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
Python Practice Problem 1: Average Expenses for Each Semester. John has a list of his monthly expenses from last year: He wants to know his average expenses for each semester. Using a for loop, calculate John's average expenses for the first semester (January to June) and the second semester (July to December).
The transportation problem is a type of Linear Programming problem. In this type of problem, the main objective is to transport goods from source warehouses to various destination locations at minimum cost. In order to solve such problems, we should have demand quantities, supply quantities, and the cost of shipping from source and destination.
Python Homework and Assignment Help. Our expert Python programmers are here to help you with any aspect of your Python learning, from coding assignments and debugging to concept clarification and exam prep. Whatever Python challenge you're facing, we've got you covered. Chat with us now for personalized support! Chat With Python Expert
The assignment problem arises in a number of different industries. The most prominent is assigning Long Haul Trucks to their Loads. This project is heavily influenced by "A Stochastic Formulation of the Dynamic Assignment Problem, with an Application to Truckload Motor Carriers" by Warren B. Powell (Princton University 1996)
beginner fast food order taker programming tutorial python. Hello again! Welcome to part 3 in a series for beginning Python! In Part 2, we covered constants, functions, lists, and string formatting. Today we will be creating a simple order taker using only a few new concepts. If you are completely new to Python, check out Part 1 to start your ...
Here's a drive() method that literally implements what you said you wanted:. The Drive method is supposed to take the car and move it a specified amount of miles as a parameter. If the car can achieve all the miles without running out of fuel, then the car makes the trip and outputs the miles while also incrementing the odometer.
Question #194527. 1. Write a class named Car that has the following data attributes: a) __year_model (for the car's year model) __make (for the make of the car) __speed (for the car's current speed) The Car class should have an __init__ method that accepts the car's year model and make as arguments. These values should be assigned to the ...
8. Explanation: There are: - 1 box of the first type that contains 3 units. - 2 boxes of the second type that contain 2 units each. - 3 boxes of the third type that contain 1 unit each. You can take all the boxes of the first and second types, and one box of the third type. The total number of units will be = (1 * 3) + (2 * 2) + (1 * 1) = 8.
A four-step transportation model predicts the traffic load on a network given data about a region. These models are used to evaluate the impacts of land-use and transportation projects. In this example, we will create a model representing California as if it acted as a city. To get started, first we will import the necessary libraries.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Return the Sum of Two Numbers. Create a function that takes two numbers as arguments and returns their sum. Examples addition (3, 2) 5 addition (-3, -6) -9 addition (7, 3) 10 Notes Don't forget to return the result. If you get stuck on a challenge, find help in the Resources tab.
00:00 Since Python's argument passing mechanism relies so much on how Python deals with assignment, the next couple of lessons will go into a bit more depth about how assignment works in Python.. 00:12 Recall some things I've already mentioned: Assignment is the process of binding a name to an object. Parameter names are also bound to objects on function entry in Python.
Question #279821. 1. Demonstrate use of Object Oriented Programming (OOP) principles, data structures and file. have derived classes named GrandSon and GrandDaughter respectively. All child. classes have derived genetic features and skills from Person. Create 5 methods for. of polymorphism. person.txt.
With our help, you can master Python programming and tackle any assignment with confidence. In conclusion, mastering Python programming requires dedication, practice, and expert guidance.
Computer Science questions and answers. For this assignment, you need to submit a Python program that gathers information about cars and trucks according to the rules provided: What type of vehicle we're dealing with (car or truck) Vehicle identification number (VIN). This is required and must be exactly 17 characters [letters and digits] long.
Truck haulage simulation animation in Python. In this article I will share a discrete-event simulation animation example in Python. More specifically a truck haul transport simulation animation for a mine, using SimPy and DesViz in Python. This example, and the DesViz module, was developed by Prof. Paul Corry and his team and I am resharing his ...
Python. Question #336261. substring: you are given two strings N &K your goal is to determine the smallest substring of N that contains all the characters in K If no substring present in N print No matches found. note: if a character is repeated multiple time in K your substring should also contain that character repeated the same no.of times.
Description: Python's built-in function chr() is used for converting an Integer to a Character, while the function ord() is used to do the reverse, i.e, convert a Character to an Integer.; We need to read the string from the user where length of the string should be the even number. Based on the 1st character, we are modifying the second, based on 3rd character we need to modify the 4th ...