C Functions
C structures, c structures (structs).
Structures (also called structs) are a way to group several related variables into one place. Each variable in the structure is known as a member of the structure.
Unlike an array , a structure can contain many different data types (int, float, char, etc.).
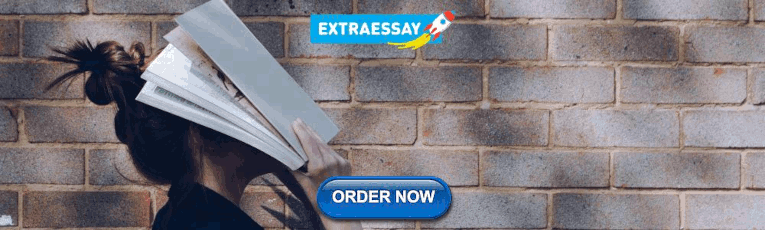
Create a Structure
You can create a structure by using the struct keyword and declare each of its members inside curly braces:
To access the structure, you must create a variable of it.
Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable:
Create a struct variable with the name "s1":
Access Structure Members
To access members of a structure, use the dot syntax ( . ):
Now you can easily create multiple structure variables with different values, using just one structure:
What About Strings in Structures?
Remember that strings in C are actually an array of characters, and unfortunately, you can't assign a value to an array like this:
An error will occur:
However, there is a solution for this! You can use the strcpy() function and assign the value to s1.myString , like this:
Simpler Syntax
You can also assign values to members of a structure variable at declaration time, in a single line.
Just insert the values in a comma-separated list inside curly braces {} . Note that you don't have to use the strcpy() function for string values with this technique:
Note: The order of the inserted values must match the order of the variable types declared in the structure (13 for int, 'B' for char, etc).
Copy Structures
You can also assign one structure to another.
In the following example, the values of s1 are copied to s2:
Modify Values
If you want to change/modify a value, you can use the dot syntax ( . ).
And to modify a string value, the strcpy() function is useful again:
Modifying values are especially useful when you copy structure values:
Ok, so, how are structures useful?
Imagine you have to write a program to store different information about Cars, such as brand, model, and year. What's great about structures is that you can create a single "Car template" and use it for every cars you make. See below for a real life example.
Real-Life Example
Use a structure to store different information about Cars:
C Exercises
Test yourself with exercises.
Fill in the missing part to create a Car structure:
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
- Course List
- Introduction to C
- Environment Setup
- First C Application
- Basic Syntax
- Variables & Constants
- if, else, switch, ternary
- while, do while & for loops
- Jump statement goto
- Intermediate
- Dynamic Memory Management
- Preprocessor directives & macros
C Structs (structures) Tutorial
In this C tutorial we learn how to define our own types in C with structs.
We cover how to define a struct, declare a struct variable, assign data to a struct member and how to assign data to a struct pointer member.
- What is a struct
How to define a struct
How to declare a struct variable, how to access and assign data to a struct property member, how to access and assign data to a struct pointer.
A struct is a user-defined type that can store one or more variables of different types. We use structs to model our application after entities in the real world, grouping related code.
As an example, let’s consider a player character in an first person shooter game. Each player has a list of properties associated with them.
- Player name
- Primary weapon
- Secondary weapon
Our first thought would be to throw all these properties into an array, however, the properties have different types.
We could create individual variables, but what if the player wants to play a co-op match where more than one player’s data needs to be stored.
This is where a struct is useful. A struct allows us to group all of these properties together as a single unit, and then create multiple units.
For example, we group the player properties into a struct called Player. We can then create multiple players of type Player, each with different values.
To define a struct, we use the keyword struct , followed by a name and a code block. Inside the code block we define the struct properties.
Note that a struct’s code block is terminated with a semicolon.
In the example above, we define the struct above the main() function. If we define it below the main() function the compiler will raise an error.
The C languages is organized around one pass compilation, which means it scans the document only once from top to bottom before compiling.
If we define a struct below a point where it’s used, the main() function for example, the compiler will see an undefined type because it doesn’t know about the struct yet.
In functions we get around this problem with a prototype at the top of the document. But, structs don’t have prototypes so we define them above the point they would be used.
Typically, a struct would go into an external file, something like player.h and be included at the top of the document like we do with the stdio.h file.
When we define a structure, it becomes a type, like int or char. Therefore, we need a variable that is of the struct’s type.
There are two ways to create a struct variable:
- After the structure definition.
- With the structure definition.
Let’s take a look at declaring a struct variable the struct’s definition first.
We write the struct keyword, followed by the struct name as the type. Lastly, we give our variable a name.
In the example above, we create a new struct variable with the name p1 of type Player . We will be able to access the struct’s properties with the p1 variable.
We can also declare a variable for the struct when we define the struct itself.
To do this we add the variable name after the closing curly brace of the struct, but before the semicolon terminator.
In the example above, we declare three variables after the struct’s closing curly brace. When declaring multiple struct variables, we can just separate them with a comma.
If we’ve declared a struct variable, we can use it to access any property inside the struct with dot notation.
Dot notation is separating the variable name and property name with a dot operator ( . ).
To assign data we use the assignment operator followed by the data we want to store in the property.
In the example above, we add ammuntion to our ammo property and then print it to the console.
When we assign data to a property, it doesn’t affect the struct itself, only the p1 instance of it .
Since we can create pointers to any type, and a structure is a type, we can also create pointers to structs.
However, we are not allowed to access the structure pointer member by using the dot operator. Instead, we use arrow notation ( -> ) to access the structure member.
Before we are able to access the property, we will need to initialize the pointer to the struct through dynamic memory allocation .
In the example above, we create a struct pointer p1 . Then we initialize the pointer as a struct pointer with the appropriate size. Finally, we access the members of p1 with arrow notation ( -> ).
Remember to release the memory afterwards with the free() function .
- Summary: Points to remember
- A struct is a user-defined type that can hold multiple different types of variables.
- A struct variable can be declared either on its own or as part of the struct definition.
- We access a struct property member with dot notation ( . ).
- We access a struct pointer member with arrow notation ( -> ).
- Define a struct
- Define a struct variable
- Access struct members
- Access struct pointer member
Structured data types in C - Struct and Typedef Explained with Examples
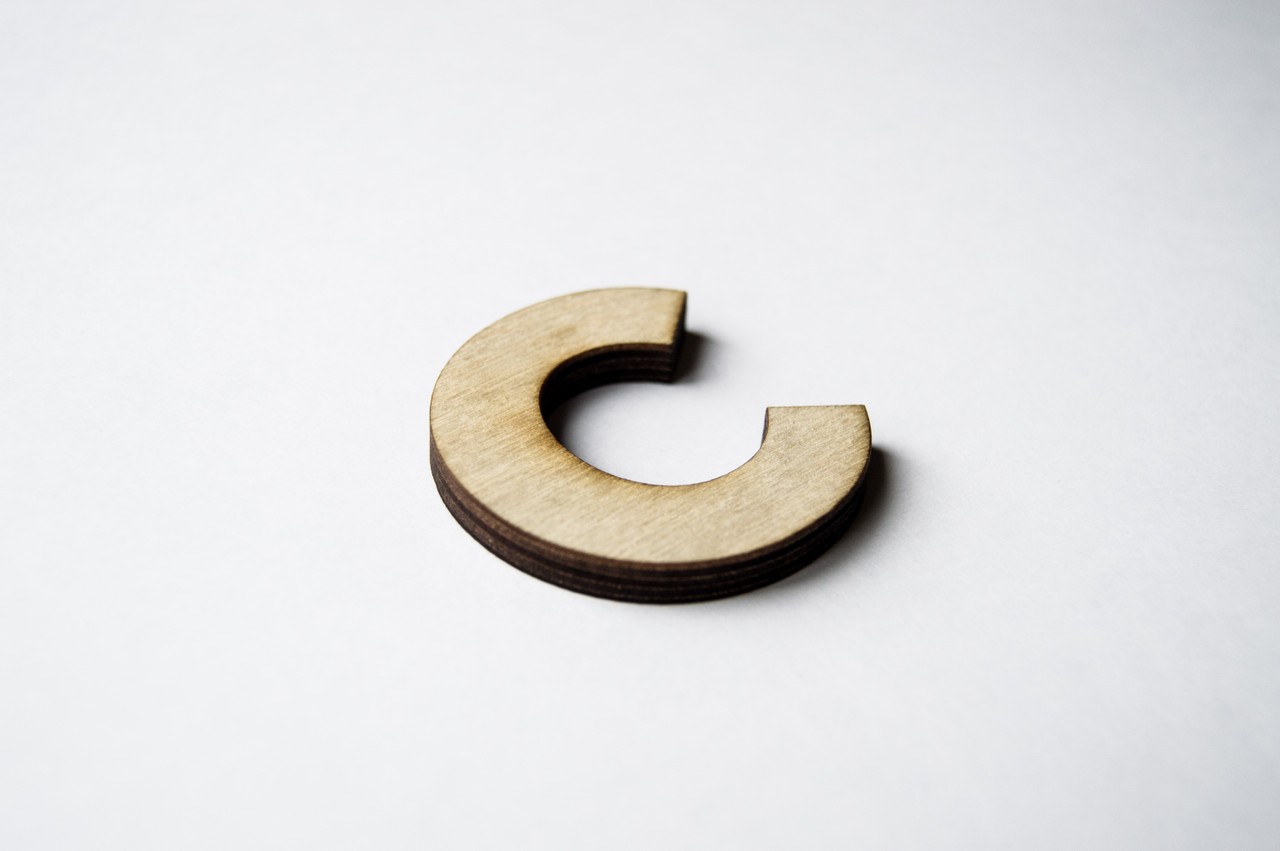
During your programming experience you may feel the need to define your own type of data. In C this is done using two keywords: struct and typedef . Structures and unions will give you the chance to store non-homogenous data types into a single collection.
Declaring a new data type
After this little code student will be a new reserved keyword and you will be able to create variables of type student . Please mind that this new kind of variable is going to be structured which means that defines a physically grouped list of variables to be placed under one name in a block of memory.
New data type usage
Let’s now create a new student variable and initialize its attributes:
As you can see in this example you are required to assign a value to all variables contained in your new data type. To access a structure variable you can use the point like in stu.name . There is also a shorter way to assign values to a structure:
Or if you prefer to set it’s values following a different order:
Unions vs Structures
Unions are declared in the same was as structs, but are different because only one item within the union can be used at any time.
You should use union in such case where only one condition will be applied and only one variable will be used. Please do not forget that we can use our brand new data type too:
A few more tricks
- When you create a pointer to a structure using the & operator you can use the special -> infix operator to deference it. This is very used for example when working with linked lists in C
- The new defined type can be used just as other basic types for almost everything. Try for example to create an array of type student and see how it works.
- Structs can be copied or assigned but you can not compare them!
More Information:
- The C Beginner's Handbook: Learn C Programming Language basics in just a few hours
- Data Types in C - Integer, Floating Point, and Void Explained
- malloc in C: Dynamic Memory Allocation in C Explained
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
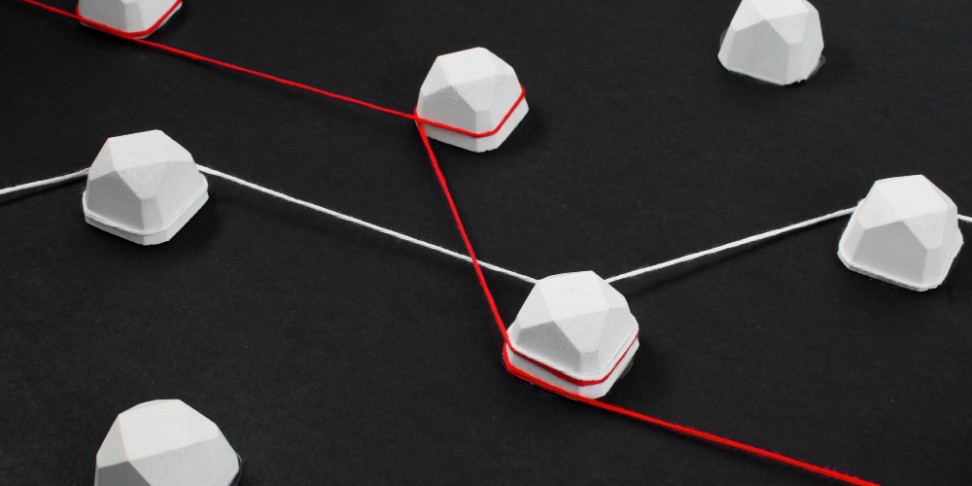
Autor: Codenga 12.05.2023
How to use Structures (Struct) in C
Structures (Structs) are a great way to group related information together. If you have a set of variables in your code that store logically connected data, consider combining them into a single entity - that is, a Structure. In this article, you will learn the most important techniques for using Structures in the C language.
A basic Structure
Let’s start with a simple example:
Pay attention to the keyword "struct". Our variables describing a mechanical vehicle (i.e., Car ) should be enclosed in curly braces, and the entire structure should be terminated with a semicolon. Inside the structure, there are variables of various types: integer types ( int ) and a pointer (char*). This will be our very basic structure, grouping information about a car.
Access the values
You need to imagine that a structure is actually a new data type. In the example below, we will show you how to define types for structures and how to set values:
And here is the result:
Let's analyze what happened in the main() function. At the beginning, we define a variable named "audi" which is of type struct Car. With the variable "audi," we can modify the elements of the structure. To access a structure element, we use the dot operator:
So we got this simple syntax:
As you can see, there's nothing difficult here. A structure (Struct) is simply a set of related data. Access to a field within a structure can be obtained using the dot operator.
Structures and functions
Now it's time for a more advanced technique. Sometimes there is a need to pass a structure to a function. Passing a structure to a function involves making a copy of it and passing it as an argument. Here's a code snippet to demonstrate this:
In this example, the structure "Person" is passed to the function "displayPerson()" as an argument by value, which means it is copied into a local variable "person" (the function parameter). Inside the function, the fields "name" and "age" can be accessed using the dot operator ".".
It's important to note that for large structures, passing them by value can be inefficient in terms of memory consumption and time. In such cases, it is recommended to use pointers.
You already know another useful technique for working with structures. It's time for the next example. Our previous function had the ability to print information from a structure. Of course, there is nothing stopping us from returning a structure from a function. Here is an example that demonstrates such behavior.
In this example, the function "updatePerson()" takes the structure "Person" as an argument, named "person" (by value). It modifies the fields of the structure and then returns the modified structure as the return value.
In the "main()" function, we initialize a structure "p1" and then call the "updatePerson()" function, passing "p1" as an argument. After calling the function, we read the fields of the returned structure "p2" and display them on the screen.
The result is as follows:
These examples illustrate the potential that lies in using structures and functions. As you can see, structures can be passed as arguments and can also be returned by functions.
Structures and pointers
Pointers are an essential part of the C language , and they can also be used to work with structures. Take a look at the example below:
In the above code, we have a crucial line of code where the pointer "ptr" is assigned the address of the "point" object:
After such an assignment, using the pointer "ptr" , we can access the fields of the structure using the "->" operator:
Structures allow you to easily group related data together. You can pass them as parameters to functions, return them from functions, create pointers to structures, and more. With structures, you can achieve clean and organized code where data is logically grouped together.
If you want to master Structures and many other practical techniques in C, enlist for the C Developer Career Path. With this career path, you will learn the C language from the basics to an intermediate-advanced level. The path concludes with an exam that grants you the Specialist Certificate, enabling you to demonstrate your proficiency with the C language.
You may also like:
Flutter and Dart - What You Need to Know to Start
Everything you need to know about Flutter and Dart to make a decision about the profitability of lea...
5 Programming Languages with Rapidly Growing Popularity
Here are five programming languages whose popularity is continually on the rise. Each of them has a ...
IT Industry - Complete Guide for Beginners
Everything you need to start your adventure in the IT industry....
- How it works?
- Career paths
- AI Enhanced
- Frequently asked questions
- Terms of service
- Privacy Policy
- Cookie settings
Intro to C for CS31 Students
Part 2: structs & pointers.
- Pointers and Functions C style "pass by referece"
- Dynamic Memory Allocation (malloc and free)
- Pointers to Structs
Defining a struct type
Declaring variables of struct types, accessing field values, passing structs to functions, rules for using pointer variables.
- Next, initialize the pointer variable (make it point to something). Pointer variables store addresses . Initialize a pointer to the address of a storage location of the type to which it points. One way to do this is to use the ampersand operator on regular variable to get its address value: int x; char ch; ptr = &x; // ptr get the address of x, pointer "points to" x ------------ ------ ptr | addr of x|--------->| ?? | x ------------ ------ cptr = &ch; // ptr get the address of ch, pointer "points to" ch cptr = &x; // ERROR! cptr can hold a char address only (it's NOT a pointer to an int) All pointer variable can be set to a special value NULL . NULL is not a valid address but it is useful for testing a pointer variable to see if it points to a valid memory address before we access what it points to: ptr = NULL; ------ ------ cptr = NULL; ptr | NULL |-----| cptr | NULL |----| ------ ------
- Use *var_name to dereference the pointer to access the value in the location that it points to. Some examples: int *ptr1, *ptr2, x, y; x = 8; ptr1 = NULL; ptr2 = &x; ------------ ------ ptr2 | addr of x|--------->| 8 | x ------------ ------ *ptr2 = 10; // dereference ptr2: "what ptr2 points to gets 10" ------------ ----- ptr2 | addr of x|--------->| 10 | x ------------ ----- y = *ptr2 + 3; // dereference ptr2: "y gets what ptr2 points to plus 3" ----- ----- ptr1 = ptr2; // ptr1 gets address value stored in ptr2 ------------ ----- ptr2 | addr of x |--------->| 10 | x ------------ ----- /\ ------------ | ptr1 | addr of x |-------------- ------------ // TODO: finish tracing through this code and show what is printed *ptr1 = 100; ptr1 = &y; *ptr1 = 80; printf("x = %d y = %d\n", x, y); printf("x = %d y = %d\n", *ptr2, *ptr1);
- and what does this mean with respect to the value of each argument after the call?
- Implement a program with a swap function that swaps the values stored in its two arguments. Make some calls to it in main to test it out.
malloc and free
Pointers, the heap, and functions, linked lists in c.
5.3. Structures
- Declaration and definition
- Stack And Heap
- new And delete
- Dot And Arrow
Programmers often use the term "structure" to name two related concepts. They form structure specifications with the struct keyword and create structure objects with a variable definition or the new operator. We typically call both specifications and objects structures, relying on context to clarify the the specific usage.
Structures are an aggregate data type whose contained data items are called fields or members . Once specified, the structure name becomes a new data type or type specifier in the program. After the program creates or instantiates a structure object, it accesses the fields with one of the selection operators . Referring to the basket analogy suggested previously, a structure object is a basket, and the fields or members are the items in the basket. Although structures are more complex than the fundamental types, programmers use the same pattern when defining variables of either type.
Structure Specifications: Fields And Members
A program must specify or declare a structure before using it. The specification determines the number, the type, the name, and the order of the data items contained in the structure.
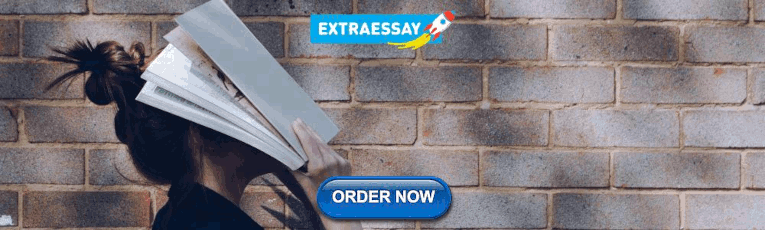
Structure Definitions And Objects
We can use a blueprint as another analogy for a structure. Blueprints show essential details like the number and size of bedrooms, the location of the kitchen, etc. Similarly, structure specifications show the structure fields' number, type, name, and order. Nevertheless, a blueprint isn't a house; you can't live in it. However, given the blueprint, we can make as many identical houses as we wish, and while each one looks like the others, it has a different, distinct set of occupants and a distinct street address. Similarly, each object created from a structure specification looks like the others, but each can store distinct values and has a distinct memory address.
- C++ code that defines three new student structure variables or objects named s1 , s2 , and s3 . The program allocates the memory for the three objects on the stack.
- The pointer variables s4 , s5 , and s6 are defined and allocated automatically on the stack. However, the structure objects they point to are allocated dynamically on the heap.
- An abstract representation of three student objects on the stack. Each object has three fields.
- An abstract representation of three student pointer variables pointing to three student objects allocated dynamically on the heap with the new operator.
Initializing Structures And Fields
Figure 3 demonstrates that we are not required to initialize structure objects when we create them - perhaps the program will read the data from the console or a data file. However, we can initialize structures when convenient. C++ inherited an aggregate field initialization syntax from the C programming language, and the 2011 ANSI standard extends that syntax, making it conform to other major programming languages. More recently, the ANSI 2020 standard added a variety of default field initializations. The following figures illustrate a few of these options.
- The field order within the structure and the data order within the initialization list are significant and must match. The program saves the first list value in the first structure field until it saves the last list value in the last structure field. If there are fewer initializers (the elements inside the braces) than there are fields, the excess fields are initialized to their zero-equivalents . Having more initializers than fields is a compile-time error.
- Similar to (a), but newer standards no longer require the = operator.
- Historically, en block structure initialization was only allowed in the structure definition, but the ANSI 2015 standard removed that restriction, allowing en bloc assignment to previously defined variables.
- Abstract representations of the structure objects or variables created and initialized in (a), (b), and (c). Each object has three fields. Although every student must have these fields, the values stored in them may be different.
- Another way to conceptualize structures and variables is as the rows and columns of a simple database table. Each column represents one field, and each row represents one structure object. The database schema fixes the number of columns, but the program can add any number of rows to the table.
- Initializing a structure object when defining it. The = operator is now optional.
- Designated initializers are more flexible than the non-designated initializers illustrated in Figure 4, which are matched to structure fields by position (first initializer to the first field, second initializer to the second field, etc.). Designated initializers may skip a field - the program does not initialize name in this example.
- Programmers may save data to previously defined structure objects, possibly skipping some fields, with designators.
The next step is accessing the individual fields within a structure object.
Field Selection: The Dot And Arrow Operators
A basket is potentially beneficial, but so far, we have only seen how to put items into it, and then only en masse . To achieve its full potential, we need some way to put items into the basket one at a time, and there must be some way to retrieve them. C++ provides two selection operators that join a specific structure object with a named field. The combination of an object and a field form a variable whose type matches the field type in the structure specification.
The Dot Operator
The C++ dot operator looks and behaves just like Java's dot operator and performs the same tasks in both languages.
- The general dot operator syntax. The left-hand operand is a structure object, and the right-hand operator is the name of one of its fields.
- Examples illustrating the dot operator based on the student examples in Figure 3(a).
- The dot operator's left operand names a specific basket or object, and the right-hand operand names the field. If we view the structure as a table, the left-hand operand selects the row, and the right-hand operand selects the column. So, we can visualize s2 . name as the intersection of a row and column.
The Arrow Operator
Java only has one way of creating objects and only needs one operator to access its fields. C++ can create objects in two ways (see Figure 3 above) and needs an additional field access operator, the arrow: -> .
- The general arrow operator syntax. The left-hand operand is a pointer to a structure object, and the right-hand operator is the name of one of the object's fields.
- Examples illustrate the arrow operator based on the student examples in Figure 3(b).
Choosing the correct selection operator

- Choose the arrow operator if the left-hand operand is a pointer.
- Otherwise, choose the dot operator.
Moving Structures Within A Program
A basket is a convenient way to carry and move many items by holding its handle. Similarly, a structure object is convenient for a program to "hold" and move many data items with a single (variable) name. Specifically, a program can assign one structure to another (as long as they are instances of the same structure specification), pass them as arguments to functions, and return them from functions as the function's return value.
Assignment is a fundamental operation regardless of the kind of data involved. The C++ code to assign one structure to another is simple and should look familiar:
- C++ code defining a new structure object and copying an existing object to it by assignment.
- An abstract representation of a new, empty structure.
- An abstract representation of how a program copies the contents of an existing structure to another structure.
Function Argument
Once the program creates a structure object, passing it to a function looks and behaves like passing a fundamental-type variable. If we view the function argument as a new, empty structure object, we see that argument passing copies one structure object to another, behaving like an assignment operation.
- The first part of the C++ code fragment defines a function (similar to a Java method). The argument is a student structure. The last statement (highlighted) passes a previously created and initialized structure as a function argument.
- In this abstract representation, a program copies the data stored in s2 to the function argument temp . The braces forming the body of the print function create a new scope distinct from the calling scope where the program defines s2 . The italicized variable names label and distinguish the illustrated objects.
Function Return Value
Just as a program can pass a structure into a function as an argument, so it can return a structure as the function's return value, as demonstrated by the following example:
- C++ code defining a function named read that returns a structure. The program defines and initializes the structure object, temp , in local or function scope with a series of console read operations and returns it as the function return value. The assignment operator saves the returned structure in s3 .
- A graphical representation of the return process. Returning an object with the return operator copies the local object, temp , to the calling scope, where the program saves it in s1 .
cppreference.com
Struct and union initialization.
When initializing an object of struct or union type, the initializer must be a non-empty, (until C23) brace-enclosed, comma-separated list of initializers for the members:
where the designator is a sequence (whitespace-separated or adjacent) of individual member designators of the form . member and array designators of the form [ index ] .
All members that are not initialized explicitly are empty-initialized .
[ edit ] Explanation
When initializing a union , the initializer list must have only one member, which initializes the first member of the union unless a designated initializer is used (since C99) .
When initializing a struct , the first initializer in the list initializes the first declared member (unless a designator is specified) (since C99) , and all subsequent initializers without designators (since C99) initialize the struct members declared after the one initialized by the previous expression.
It's an error to provide more initializers than members.
[ edit ] Nested initialization
If the members of the struct or union are arrays, structs, or unions, the corresponding initializers in the brace-enclosed list of initializers are any initializers that are valid for those members, except that their braces may be omitted as follows:
If the nested initializer begins with an opening brace, the entire nested initializer up to its closing brace initializes the corresponding member object. Each left opening brace establishes a new current object . The members of the current object are initialized in their natural order , unless designators are used (since C99) : array elements in subscript order, struct members in declaration order, only the first declared member of any union. The subobjects within the current object that are not explicitly initialized by the closing brace are empty-initialized .
If the nested initializer does not begin with an opening brace, only enough initializers from the list are taken to account for the elements or members of the member array, struct or union; any remaining initializers are left to initialize the next struct member:
[ edit ] Notes
The initializer list may have a trailing comma, which is ignored.
[ edit ] Example
Possible output:
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.7.9/12-39 Initialization (p: 101-105)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.9/12-38 Initialization (p: 140-144)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.8/12-38 Initialization (p: 126-130)
- C89/C90 standard (ISO/IEC 9899:1990):
- 6.5.7 Initialization
[ edit ] See also
- Todo with reason
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 26 January 2023, at 03:21.
- This page has been accessed 1,202,222 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
Operations on struct variables in C
- Static Variables in C
- Initialization of static variables in C
- How to print a variable name in C?
- Redeclaration of global variable in C
- Structure Pointer in C
- C | Variable Declaration and Scope | Question 4
- C | Variable Declaration and Scope | Question 2
- C | Variable Declaration and Scope | Question 6
- C | Variable Declaration and Scope | Question 7
- C | Variable Declaration and Scope | Question 3
- C | Variable Declaration and Scope | Question 1
- Local Variable in C
- Printing Struct Variables in Golang
- Printing structure variables in console in Golang
- How to print struct variables data in Golang?
- Scope of Variables in Go
- Structures in Objective-C
- C | Variable Declaration and Scope | Question 5
- Pointers to Structures in Objective C
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Bitwise Operators in C
- std::sort() in C++ STL
- What is Memory Leak? How can we avoid?
- Substring in C++
- Segmentation Fault in C/C++
- Socket Programming in C
- For Versus While
- Data Types in C
In C, the only operation that can be applied to struct variables is assignment. Any other operation (e.g. equality check) is not allowed on struct variables. For example, program 1 works without any error and program 2 fails in compilation.
Please Login to comment...
Similar reads.
- C-Struct-Union-Enum
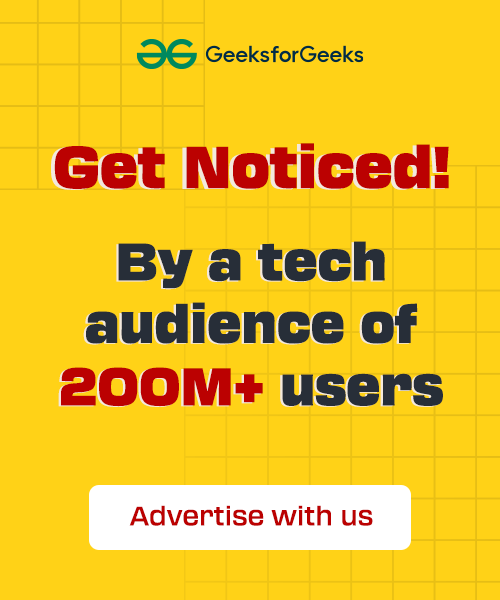
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, introduction.
- Getting Started with C
- Your First C Program
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
Programming Arrays
- C Multidimensional Arrays
Pass arrays to a function in C
Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
Programming Strings
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
Structure and Union
C structs and Pointers
C Structure and Function
Programmimg files.
- C File Handling
C Files Examples
Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
- Add Two Complex Numbers by Passing Structure to a Function
- Store Information of Students Using Structure
- Store Information of a Student Using Structure
- Add Two Distances (in inch-feet system) using Structures
Similar to variables of built-in types, you can also pass structure variables to a function.
Passing structs to functions
We recommended you to learn these tutorials before you learn how to pass structs to functions.
- C structures
- C functions
- User-defined Function
Here's how you can pass structures to a function
Here, a struct variable s1 of type struct student is created. The variable is passed to the display() function using display(s1); statement.
Return struct from a function
Here's how you can return structure from a function:
Here, the getInformation() function is called using s = getInformation(); statement. The function returns a structure of type struct student . The returned structure is displayed from the main() function.
Notice that, the return type of getInformation() is also struct student .
Passing struct by reference
You can also pass structs by reference (in a similar way like you pass variables of built-in type by reference). We suggest you to read pass by reference tutorial before you proceed.
During pass by reference, the memory addresses of struct variables are passed to the function.
In the above program, three structure variables c1 , c2 and the address of result is passed to the addNumbers() function. Here, result is passed by reference.
When the result variable inside the addNumbers() is altered, the result variable inside the main() function is also altered accordingly.
Table of Contents
- Passing structure to a function
- Return struct from a function
- Passing struct by reference
Sorry about that.
Related Tutorials
A man is valued by his works, not his words!
Structure assignment and its pitfall in c language.
Jan 28 th , 2013 9:47 pm
There is a structure type defined as below:
If we want to assign map_t type variable struct2 to sturct1 , we usually have below 3 ways:
Consider above ways, most of programmer won’t use way #1, since it’s so stupid ways compare to other twos, only if we are defining an structure assignment function. So, what’s the difference between way #2 and way #3? And what’s the pitfall of the structure assignment once there is array or pointer member existed? Coming sections maybe helpful for your understanding.
The difference between ‘=’ straight assignment and memcpy
The struct1=struct2; notation is not only more concise , but also shorter and leaves more optimization opportunities to the compiler . The semantic meaning of = is an assignment, while memcpy just copies memory. That’s a huge difference in readability as well, although memcpy does the same in this case.
Copying by straight assignment is probably best, since it’s shorter, easier to read, and has a higher level of abstraction. Instead of saying (to the human reader of the code) “copy these bits from here to there”, and requiring the reader to think about the size argument to the copy, you’re just doing a straight assignment (“copy this value from here to here”). There can be no hesitation about whether or not the size is correct.
Consider that, above source code also has pitfall about the pointer alias, it will lead dangling pointer problem ( It will be introduced below section ). If we use straight structure assignment ‘=’ in C++, we can consider to overload the operator= function , that can dissolve the problem, and the structure assignment usage does not need to do any changes, but structure memcpy does not have such opportunity.
The pitfall of structure assignment:
Beware though, that copying structs that contain pointers to heap-allocated memory can be a bit dangerous, since by doing so you’re aliasing the pointer, and typically making it ambiguous who owns the pointer after the copying operation.
If the structures are of compatible types, yes, you can, with something like:
} The only thing you need to be aware of is that this is a shallow copy. In other words, if you have a char * pointing to a specific string, both structures will point to the same string.
And changing the contents of one of those string fields (the data that the char points to, not the char itself) will change the other as well. For these situations a “deep copy” is really the only choice, and that needs to go in a function. If you want a easy copy without having to manually do each field but with the added bonus of non-shallow string copies, use strdup:
This will copy the entire contents of the structure, then deep-copy the string, effectively giving a separate string to each structure. And, if your C implementation doesn’t have a strdup (it’s not part of the ISO standard), you have to allocate new memory for dest_struct pointer member, and copy the data to memory address.
Example of trap:
Below diagram illustrates above source memory layout, if there is a pointer field member, either the straight assignment or memcpy , that will be alias of pointer to point same address. For example, b.alias and c.alias both points to address of a.alias . Once one of them free the pointed address, it will cause another pointer as dangling pointer. It’s dangerous!!
- Recommend use straight assignment ‘=’ instead of memcpy.
- If structure has pointer or array member, please consider the pointer alias problem, it will lead dangling pointer once incorrect use. Better way is implement structure assignment function in C, and overload the operator= function in C++.
- stackoverflow.com: structure assignment or memcpy
- stackoverflow.com: assign one struct to another in C
- bytes.com: structures assignment
- wikipedia: struct in C programming language
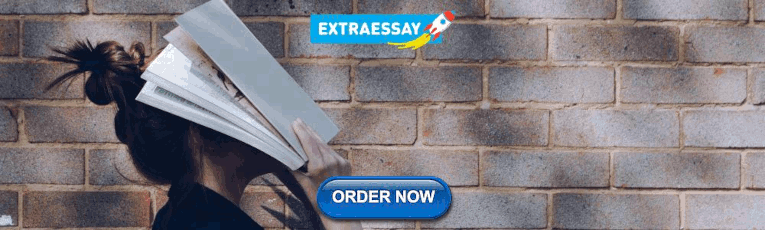
IMAGES
VIDEO
COMMENTS
You can if the values are already part of a similar struct, that is, you can do this: Student s1 = {.id = id, .name = name, .score = score}; which creates an instance of Student and initializes the fields you specify. This probably isn't really any more efficient than assigning values individually, but it does keep the code concise.
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
To access the structure, you must create a variable of it. Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable: Create a struct variable with the name "s1": struct myStructure {. int myNum; char myLetter; }; int main () {. struct myStructure s1;
Create struct Variables. When a struct type is declared, no storage or memory is allocated. To allocate memory of a given structure type and work with it, we need to create variables. Here's how we create structure variables: struct Person { // code }; int main() { struct Person person1, person2, p[20]; return 0; }
C Structures. The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
If a struct defines at least one named member, it is allowed to additionally declare its last member with incomplete array type. When an element of the flexible array member is accessed (in an expression that uses operator . or -> with the flexible array member's name as the right-hand-side operand), then the struct behaves as if the array member had the longest size fitting in the memory ...
In the example above, we declare three variables after the struct's closing curly brace. When declaring multiple struct variables, we can just separate them with a comma. How to access and assign data to a struct property member If we've declared a struct variable, we can use it to access any property inside the struct with dot notation.
As you can see in this example you are required to assign a value to all variables contained in your new data type. To access a structure variable you can use the point like in stu.name. There is also a shorter way to assign values to a structure: typedef struct{ int x; int y; }point; point image_dimension = {640,480};
At the beginning, we define a variable named "audi" which is of type struct Car. With the variable "audi," we can modify the elements of the structure. To access a structure element, we use the dot operator: audi.productionYear = 2017; So we got this simple syntax: [ name of the struct variable ] [ . ] [ name of the value ] = [ value ] [ ; ]
C Pointers to struct. Here's how you can create pointers to structs. struct name { member1; member2; . . }; int main() { struct name *ptr, Harry; } Here, ptr is a ... Sometimes, the number of struct variables you declared may be insufficient. You may need to allocate memory during run-time. Here's how you can achieve this in C programming.
C Stucts and Pointers. This is the second part of a two part introduction to the C programming language. It is written specifically for CS31 students. The first part covers C programs, compiling and running, variables, types, operators, loops, functions, arrays, parameter passing (basic types and arrays), standard I/O (printf, scanf), and file ...
Instead of using the struct home_address every time you need to declare struct variable, you can simply use addr, the typedef that we have defined. You can read the typedef in detail here. Array of Structures in C. An array of structures is an array with structure as elements. For example: Here, stu[5] is an array of structures.
Defining structure variables. Both statements define a variable: int and student are both type specifiers, ... The C++ code to assign one structure to another is simple and should look familiar: student s4; s4 = s1; (a) (b) (c) The assignment operator works with structures. Used with structure operands, the assignment operator copies a block of ...
A designator causes the following initializer to initialize the struct member described by the designator. Initialization then continues forward in order of declaration, beginning with the next element declared after the one described by the designator.
Flexible Array Members in a structure in C; Struct Hack; Designated Initializers in C; C program to store Student records as Structures and Sort them by Name; Enumeration (or enum) in C; C Programming Interview Questions (2024) fabs() Function in C; Function Call Stack in C; Why variable name does not start with numbers in C ? Keywords in C
A struct in the C programming language (and many derivatives) is a composite data type (or record) declaration that defines a physically grouped list of variables under one name in a block of memory, allowing the different variables to be accessed via a single pointer or by the struct declared name which returns the same address. The struct data type can contain other data types so is used for ...
Here, a struct variable s1 of type struct student is created. The variable is passed to the display() function using display(s1); statement. Return struct from a function. Here's how you can return structure from a function:
The pitfall of structure assignment: Beware though, that copying structs that contain pointers to heap-allocated memory can be a bit dangerous, since by doing so you're aliasing the pointer, and typically making it ambiguous who owns the pointer after the copying operation. If the structures are of compatible types, yes, you can, with ...
Assignment Operator; Destructor; Move Constructor (C++11) Move Assignment (C++11) If you want to know why? It is to maintain backward compatibility with C (because C structs are copyable using = and in declaration). But it also makes writing simple classes easier. Some would argue that it adds problems because of the "shallow copy problem".
So you are trying to assign a pointer to a non-existent element of the array. Pay attention to that arrays do not have the assignment operator. You could initialize the object of the structure type when it is defined like. struct Student s1= { .name = "Tom", .age = 20, .gpa = 4.3 };