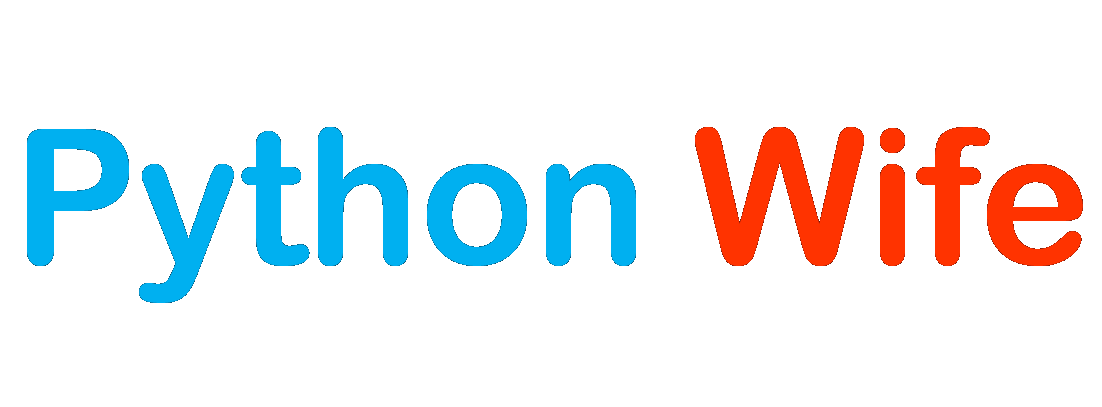
- Computer Vision
- Problem Solving in Python
- Intro to DS and Algo
- Analysis of Algorithm
- Dictionaries
- Linked Lists
- Doubly Linked Lists
- Circular Singly Linked List
- Circular Doubly Linked List
- Tree/Binary Tree
- Binary Search Tree
- Binary Heap
- Sorting Algorithms
- Searching Algorithms
- Single-Source Shortest Path
- Topological Sort
- Dijkstra’s
- Bellman-Ford’s
- All Pair Shortest Path
- Minimum Spanning Tree
- Kruskal & Prim’s
Problem-solving is the process of identifying a problem, creating an algorithm to solve the given problem, and finally implementing the algorithm to develop a computer program .
An algorithm is a process or set of rules to be followed while performing calculations or other problem-solving operations. It is simply a set of steps to accomplish a certain task.
In this article, we will discuss 5 major steps for efficient problem-solving. These steps are:
- Understanding the Problem
- Exploring Examples
- Breaking the Problem Down
- Solving or Simplification
- Looking back and Refactoring
While understanding the problem, we first need to closely examine the language of the question and then proceed further. The following questions can be helpful while understanding the given problem at hand.
- Can the problem be restated in our own words?
- What are the inputs that are needed for the problem?
- What are the outputs that come from the problem?
- Can the outputs be determined from the inputs? In other words, do we have enough information to solve the given problem?
- What should the important pieces of data be labeled?
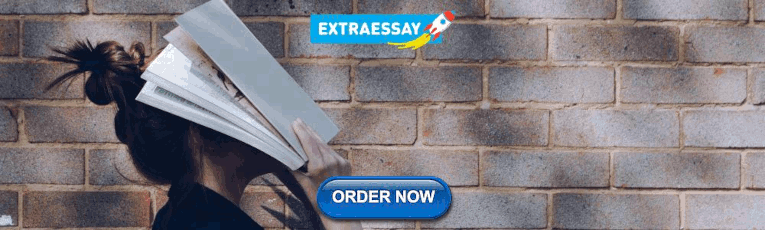
Example : Write a function that takes two numbers and returns their sum.
- Implement addition
- Integer, Float, etc.
Once we have understood the given problem, we can look up various examples related to it. The examples should cover all situations that can be encountered while the implementation.
- Start with simple examples.
- Progress to more complex examples.
- Explore examples with empty inputs.
- Explore examples with invalid inputs.
Example : Write a function that takes a string as input and returns the count of each character
After exploring examples related to the problem, we need to break down the given problem. Before implementation, we write out the steps that need to be taken to solve the question.
Once we have laid out the steps to solve the problem, we try to find the solution to the question. If the solution cannot be found, try to simplify the problem instead.
The steps to simplify a problem are as follows:
- Find the core difficulty
- Temporarily ignore the difficulty
- Write a simplified solution
- Then incorporate that difficulty
Since we have completed the implementation of the problem, we now look back at the code and refactor it if required. It is an important step to refactor the code so as to improve efficiency.
The following questions can be helpful while looking back at the code and refactoring:
- Can we check the result?
- Can we derive the result differently?
- Can we understand it at a glance?
- Can we use the result or mehtod for some other problem?
- Can you improve the performance of the solution?
- How do other people solve the problem?
Trending Posts You Might Like
- File Upload / Download with Streamlit
- Dijkstra’s Algorithm in Python
- Seaborn with STREAMLIT
- Greedy Algorithms in Python
Author : Bhavya
How to Solve Algorithmic Problems in Python
Understanding the Basics of Algorithmic Problems
An algorithm is a step-by-step procedure for solving a problem or accomplishing a specific task. Algorithmic problems are challenges that require an algorithm to solve. These challenges often involve data manipulation or optimization.
Step 1: Understand the Problem
The first step in solving any problem, algorithmic or otherwise, is to understand the problem thoroughly. This involves identifying the problem's inputs, expected outputs, and constraints.
Step 2: Design the Algorithm
Once you understand the problem, the next step is to design an algorithm to solve it. This involves breaking down the problem into smaller, more manageable parts and designing a solution for each.
Example: Solving a Sorting Problem
Let's look at a simple algorithmic problem: sorting a list of numbers in ascending order. Here is a Python function that uses the Bubble Sort algorithm to solve this problem:
The bubble_sort function sorts the list of numbers by repeatedly swapping adjacent numbers if they are in the wrong order.
Step 3: Implement the Algorithm
After designing the algorithm, you must implement it in Python. This involves writing Python code that follows the steps outlined in your algorithm.
The code above creates a list of numbers, sorts it using the bubble_sort function, and then prints the sorted list.
Step 4: Test the Algorithm
After implementing the algorithm, you should test it to ensure it works correctly. This involves running your Python code and checking that it produces the expected output for various input values.
The code snippet above shows the output of running the bubble_sort.py script in a shell. As you can see, it correctly sorts the list of numbers.
Step 5: Analyze the Algorithm
You should analyze your algorithm to understand its efficiency. This involves calculating your algorithm's time and space complexity, which are measures of how the algorithm's resource usage scales with the input size.
For Bubble Sort, the time complexity is O(n^2), and the space complexity is O(1). This means Bubble Sort can be inefficient for large lists but uses a small, constant amount of memory.
In conclusion, solving algorithmic problems in Python involves understanding the problem, designing an algorithm to solve it, implementing it in Python, testing it, and analyzing its efficiency. This process is a fundamental part of Python programming and general software development. If you're looking to hire Python developers who are proficient in solving algorithmic problems, check out Reintech.
If you're interested in enhancing this article or becoming a contributing author, we'd love to hear from you.
Please contact Sasha at [email protected] to discuss the opportunity further or to inquire about adding a direct link to your resource. We welcome your collaboration and contributions!
An algorithm is a step-by-step procedure or set of instructions designed to solve a problem or achieve a specific goal. In computer programming, algorithms are used to perform tasks, manipulate data, and make decisions based on certain conditions. Developing efficient algorithms is a crucial aspect of computer science and software engineering.
To learn more about algorithms, visit the Wikipedia page on the subject.
Bubble Sort
Bubble Sort is a simple comparison-based sorting algorithm. It works by repeatedly swapping adjacent elements if they are in the wrong order. This process continues until no more swaps are needed, indicating that the list is sorted. Although it's straightforward and easy to implement, Bubble Sort is not efficient for large datasets compared to other sorting algorithms like Quick Sort or Merge Sort. More info .
Hire Python Developers with Cloudfront Skill for Your Remote Tech Team
Boost Your Technical Excellence With Python JWT Skilled Remote Developers
Hire Expert Python Developers Skilled in Shopify API

Problem Solving with Python
If you like this book, please consider purchasing a hard copy version on amazon.com .
- You will find the book chapters on the left hand menu
- You will find navigation within a section of a chapter (one webpage) on the righthand menu
- Sources for this text are stored on GitHub at github.com/professorkazarinoff/Problem-Solving-with-Python-37-Edition
If you find the text useful, please consider supporting the work by purchasing a hard copy of the text .
This work is licensed under a GNU General Public License v3.0
- +91-98717 00866 , +91-74286 41159
- Office No - G-20, 2nd Floor, Preet vihar, Near Metro Pillar No 103, Vikas marg main road, Delhi-110092
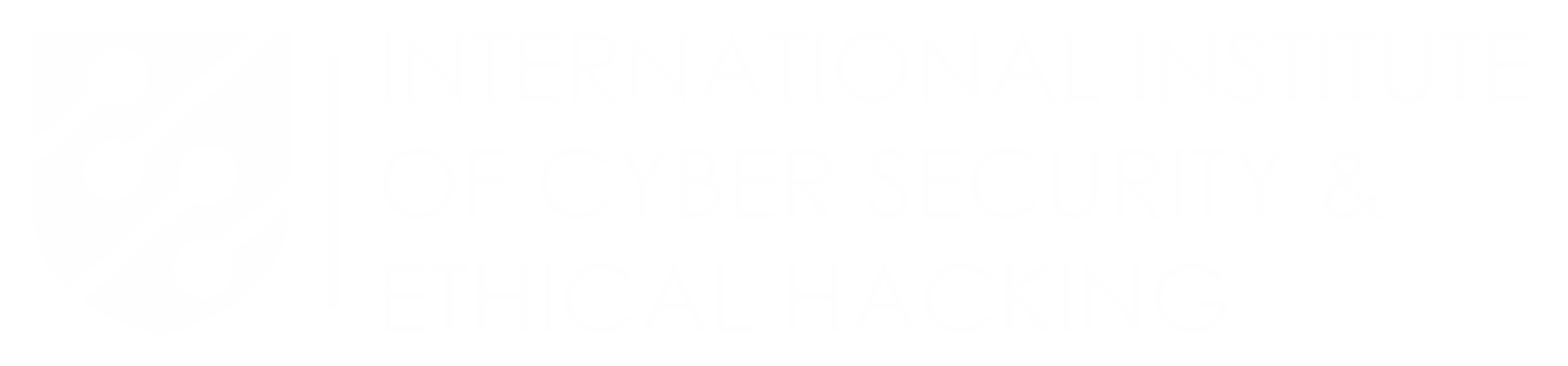
Mastering Python: Tips and Tricks for Efficient Coding and Problem Solving

- Last Updated Aug 21, 2023
Python is a versatile and powerful programming language known for its simplicity and readability. It's a popular choice for beginners and experienced programmers alike. To truly master Python, it's essential to not only understand its syntax but also to adopt efficient coding practices and problem-solving techniques. In this article, we'll explore some tips and tricks to help you become a more proficient Python programmer. 1. Code Readability One of Python's most significant strengths is its readability. PEP 8, the official Python style guide, provides conventions for formatting your code. Following these guidelines will make your code more accessible to others and, more importantly, to yourself in the future. Use meaningful variable and function names, follow consistent indentation, and use comments to explain complex sections of your code. 2. Pythonic Idioms Python has a unique and expressive coding style known as "Pythonic." To write more Pythonic code, embrace list comprehensions, generators, and context managers. These constructs allow you to write concise, readable code that leverages Python's inherent capabilities effectively. 3. Effective Debugging Mastering debugging is crucial for efficient problem-solving. Python provides a range of debugging tools, including the built-in `pdb` module for interactive debugging and third-party libraries like `pdbpp` and `ipdb`. Learn how to set breakpoints, inspect variables, and step through your code to identify and resolve issues quickly. 4. Libraries and Modules Python's extensive standard library and rich ecosystem of third-party packages are some of its most valuable assets. Familiarise yourself with commonly used libraries like NumPy for numerical computing, pandas for data manipulation, and matplotlib for data visualisation. Additionally, explore the Python Package Index (PyPI) to discover community-contributed packages that can streamline your work. 5. Documentation Documenting your code is often overlooked but crucial for maintaining and sharing your projects. Use docstrings to provide information about your functions and modules. Consider using tools like Sphinx to generate comprehensive documentation for your projects. 6. Version Control Version control systems like Git are essential for collaborative programming and code management. Learn the basics of Git to track changes, collaborate with others, and revert to previous versions of your code when necessary. Platforms like GitHub and GitLab offer hosting services for your projects. 7. Python Virtual Environments Virtual environments allow you to isolate project dependencies, ensuring that different projects don't interfere with one another. Tools like `venv` and `virtualenv` make it easy to create and manage these environments. Use them to maintain clean, organised development environments. 8. Testing Implementing tests for your code with libraries like `unittest` or `pytest` helps catch bugs early and ensures that your code functions as expected. Embrace test-driven development (TDD) to improve code quality and maintainability. 9. Problem-Solving Strategies To become a proficient Python programmer, focus on developing problem-solving skills. Break down complex problems into smaller, manageable tasks, and use algorithms and data structures efficiently. Online coding platforms like LeetCode and HackerRank offer a wealth of programming challenges to sharpen your problem-solving abilities. 10. Continuous Learning Finally, Python is an evolving language with frequent updates and improvements. Stay up to date with the latest developments in Python by reading blogs, following Python-related news, and exploring advanced topics like concurrency and parallelism. In conclusion, mastering Python is more than just writing code; it's about adopting efficient coding practices, debugging effectively, leveraging libraries, and becoming a skilled problem solver. By following these tips and tricks, you'll enhance your Python programming skills and unlock the full potential of this versatile language. Happy coding!
- Scientific skills for getting a better result
- Communication skills to getting in touch
- A career overview opportunity available
- A good work environment for work
- Other Courses: Amazon Web Services (AWS) Certified Ethical Hacker (CEHv12) Cisco Certified Network Associate (CCNA) Python Programming Red Hat Certified System Administrator (RHCSA)
- Location: Office No - G-20, 2nd Floor, Preet vihar, Near Metro Pillar No 103, Vikas marg main road, Delhi-110092
- Call: +91-98717 00866 +91-74286 41159
- Email: [email protected]
- Admiral “Amazing Grace” Hopper
Exploring the Intricacies of NP-Completeness in Computer Science
Understanding p vs np problems in computer science: a primer for beginners, understanding key theoretical frameworks in computer science: a beginner’s guide.

Learn Computer Science with Python
CS is a journey, not a destination
- Foundations
Understanding Algorithms: The Key to Problem-Solving Mastery

The world of computer science is a fascinating realm, where intricate concepts and technologies continuously shape the way we interact with machines. Among the vast array of ideas and principles, few are as fundamental and essential as algorithms. These powerful tools serve as the building blocks of computation, enabling computers to solve problems, make decisions, and process vast amounts of data efficiently.
An algorithm can be thought of as a step-by-step procedure or a set of instructions designed to solve a specific problem or accomplish a particular task. It represents a systematic approach to finding solutions and provides a structured way to tackle complex computational challenges. Algorithms are at the heart of various applications, from simple calculations to sophisticated machine learning models and complex data analysis.
Understanding algorithms and their inner workings is crucial for anyone interested in computer science. They serve as the backbone of software development, powering the creation of innovative applications across numerous domains. By comprehending the concept of algorithms, aspiring computer science enthusiasts gain a powerful toolset to approach problem-solving and gain insight into the efficiency and performance of different computational methods.
In this article, we aim to provide a clear and accessible introduction to algorithms, focusing on their importance in problem-solving and exploring common types such as searching, sorting, and recursion. By delving into these topics, readers will gain a solid foundation in algorithmic thinking and discover the underlying principles that drive the functioning of modern computing systems. Whether you’re a beginner in the world of computer science or seeking to deepen your understanding, this article will equip you with the knowledge to navigate the fascinating world of algorithms.
What are Algorithms?
At its core, an algorithm is a systematic, step-by-step procedure or set of rules designed to solve a problem or perform a specific task. It provides clear instructions that, when followed meticulously, lead to the desired outcome.
Consider an algorithm to be akin to a recipe for your favorite dish. When you decide to cook, the recipe is your go-to guide. It lists out the ingredients you need, their exact quantities, and a detailed, step-by-step explanation of the process, from how to prepare the ingredients to how to mix them, and finally, the cooking process. It even provides an order for adding the ingredients and specific times for cooking to ensure the dish turns out perfect.
In the same vein, an algorithm, within the realm of computer science, provides an explicit series of instructions to accomplish a goal. This could be a simple goal like sorting a list of numbers in ascending order, a more complex task such as searching for a specific data point in a massive dataset, or even a highly complicated task like determining the shortest path between two points on a map (think Google Maps). No matter the complexity of the problem at hand, there’s always an algorithm working tirelessly behind the scenes to solve it.
Furthermore, algorithms aren’t limited to specific programming languages. They are universal and can be implemented in any language. This is why understanding the fundamental concept of algorithms can empower you to solve problems across various programming languages.
The Importance of Algorithms
Algorithms are indisputably the backbone of all computational operations. They’re a fundamental part of the digital world that we interact with daily. When you search for something on the web, an algorithm is tirelessly working behind the scenes to sift through millions, possibly billions, of web pages to bring you the most relevant results. When you use a GPS to find the fastest route to a location, an algorithm is computing all possible paths, factoring in variables like traffic and road conditions, to provide you the optimal route.
Consider the world of social media, where algorithms curate personalized feeds based on our previous interactions, or in streaming platforms where they recommend shows and movies based on our viewing habits. Every click, every like, every search, and every interaction is processed by algorithms to serve you a seamless digital experience.
In the realm of computer science and beyond, everything revolves around problem-solving, and algorithms are our most reliable problem-solving tools. They provide a structured approach to problem-solving, breaking down complex problems into manageable steps and ensuring that every eventuality is accounted for.
Moreover, an algorithm’s efficiency is not just a matter of preference but a necessity. Given that computers have finite resources — time, memory, and computational power — the algorithms we use need to be optimized to make the best possible use of these resources. Efficient algorithms are the ones that can perform tasks more quickly, using less memory, and provide solutions to complex problems that might be infeasible with less efficient alternatives.
In the context of massive datasets (the likes of which are common in our data-driven world), the difference between a poorly designed algorithm and an efficient one could be the difference between a solution that takes years to compute and one that takes mere seconds. Therefore, understanding, designing, and implementing efficient algorithms is a critical skill for any computer scientist or software engineer.
Hence, as a computer science beginner, you are starting a journey where algorithms will be your best allies — universal keys capable of unlocking solutions to a myriad of problems, big or small.
Common Types of Algorithms: Searching and Sorting
Two of the most ubiquitous types of algorithms that beginners often encounter are searching and sorting algorithms.
Searching algorithms are designed to retrieve specific information from a data structure, like an array or a database. A simple example is the linear search, which works by checking each element in the array until it finds the one it’s looking for. Although easy to understand, this method isn’t efficient for large datasets, which is where more complex algorithms like binary search come in.
Binary search, on the other hand, is like looking up a word in the dictionary. Instead of checking each word from beginning to end, you open the dictionary in the middle and see if the word you’re looking for should be on the left or right side, thereby reducing the search space by half with each step.
Sorting algorithms, meanwhile, are designed to arrange elements in a particular order. A simple sorting algorithm is bubble sort, which works by repeatedly swapping adjacent elements if they’re in the wrong order. Again, while straightforward, it’s not efficient for larger datasets. More advanced sorting algorithms, such as quicksort or mergesort, have been designed to sort large data collections more efficiently.
Diving Deeper: Graph and Dynamic Programming Algorithms
Building upon our understanding of searching and sorting algorithms, let’s delve into two other families of algorithms often encountered in computer science: graph algorithms and dynamic programming algorithms.
A graph is a mathematical structure that models the relationship between pairs of objects. Graphs consist of vertices (or nodes) and edges (where each edge connects a pair of vertices). Graphs are commonly used to represent real-world systems such as social networks, web pages, biological networks, and more.
Graph algorithms are designed to solve problems centered around these structures. Some common graph algorithms include:
Dynamic programming is a powerful method used in optimization problems, where the main problem is broken down into simpler, overlapping subproblems. The solutions to these subproblems are stored and reused to build up the solution to the main problem, saving computational effort.
Here are two common dynamic programming problems:
Understanding these algorithm families — searching, sorting, graph, and dynamic programming algorithms — not only equips you with powerful tools to solve a variety of complex problems but also serves as a springboard to dive deeper into the rich ocean of algorithms and computer science.
Recursion: A Powerful Technique
While searching and sorting represent specific problem domains, recursion is a broad technique used in a wide range of algorithms. Recursion involves breaking down a problem into smaller, more manageable parts, and a function calling itself to solve these smaller parts.
To visualize recursion, consider the task of calculating factorial of a number. The factorial of a number n (denoted as n! ) is the product of all positive integers less than or equal to n . For instance, the factorial of 5 ( 5! ) is 5 x 4 x 3 x 2 x 1 = 120 . A recursive algorithm for finding factorial of n would involve multiplying n by the factorial of n-1 . The function keeps calling itself with a smaller value of n each time until it reaches a point where n is equal to 1, at which point it starts returning values back up the chain.
Algorithms are truly the heart of computer science, transforming raw data into valuable information and insight. Understanding their functionality and purpose is key to progressing in your computer science journey. As you continue your exploration, remember that each algorithm you encounter, no matter how complex it may seem, is simply a step-by-step procedure to solve a problem.
We’ve just scratched the surface of the fascinating world of algorithms. With time, patience, and practice, you will learn to create your own algorithms and start solving problems with confidence and efficiency.
Related Articles

Three Elegant Algorithms Every Computer Science Beginner Should Know
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Concept of Comments in Computer Programming
- Modular Approach in Programming
- Domain Specific Tools
- CBSE Class 11 | Concepts of Programming Methodology
- Open Source, Freeware and Shareware Softwares
- System Software
- Classification of Computers
- Cyber safety
- Difference between Algorithm, Pseudocode and Program
- Office Tools and Domain Specific Tools
- CBSE Class 11 | Mobile Operating Systems - Symbian, Android and iOS
- CBSE Class 11 | Computer Science - C++ Syllabus
- Interesting Examples of algorithms in everyday life
- ISC- Class 12 Computer Science 2017
- CBSE Class 11 C++ Sample Paper-3
- CBSE Class 11 C++ | Sample Paper -2
- Writing First C++ Program - Hello World Example
- Types of Operating Systems
CBSE Class 11 | Problem Solving Methodologies
Problem solving process.
The process of problem-solving is an activity which has its ingredients as the specification of the program and the served dish is a correct program. This activity comprises of four steps : 1. Understanding the problem: To solve any problem it is very crucial to understand the problem first. What is the desired output of the code and how that output can be generated? The obvious and essential need to generate the output is an input. The input may be singular or it may be a set of inputs. A proper relationship between the input and output must be drawn in order to solve the problem efficiently. The input set should be complete and sufficient enough to draw the output. It means all the necessary inputs required to compute the output should be present at the time of computation. However, it should be kept in mind that the programmer should ensure that the minimum number of inputs should be there. Any irrelevant input only increases the size of and memory overhead of the program. Thus Identifying the minimum number of inputs required for output is a crucial element for understanding the problem.
2. Devising the plan: Once a problem has been understood, a proper action plan has to be devised to solve it. This is called devising the plan. This step usually involves computing the result from the given set of inputs. It uses the relationship drawn between inputs and outputs in the previous step. The complexity of this step depends upon the complexity of the problem at hand.
3. Executing the plan: Once the plan has been defined, it should follow the trajectory of action while ensuring the plan’s integrity at various checkpoints. If any inconsistency is found in between, the plan needs to be revised.
4. Evaluation: The final result so obtained must be evaluated and verified to see if the problem has been solved satisfactorily.
Problem Solving Methodology(The solution for the problem)
The methodology to solve a problem is defined as the most efficient solution to the problem. Although, there can be multiple ways to crack a nut, but a methodology is one where the nut is cracked in the shortest time and with minimum effort. Clearly, a sledgehammer can never be used to crack a nut. Under problem-solving methodology, we will see a step by step solution for a problem. These steps closely resemble the software life cycle . A software life cycle involves several stages in a program’s life cycle. These steps can be used by any tyro programmer to solve a problem in the most efficient way ever. The several steps of this cycle are as follows :
Step by step solution for a problem (Software Life Cycle) 1. Problem Definition/Specification: A computer program is basically a machine language solution to a real-life problem. Because programs are generally made to solve the pragmatic problems of the outside world. In order to solve the problem, it is very necessary to define the problem to get its proper understanding. For example, suppose we are asked to write a code for “ Compute the average of three numbers”. In this case, a proper definition of the problem will include questions like : “What exactly does average mean?” “How to calculate the average?”
Once, questions like these are raised, it helps to formulate the solution of the problem in a better way. Once a problem has been defined, the program’s specifications are then listed. Problem specifications describe what the program for the problem must do. It should definitely include :
what is the input set of the program
What is the desired output of the program and in what form the output is desired?
2. Problem Analysis (Breaking down the solution into simple steps): This step of solving the problem follows a modular approach to crack the nut. The problem is divided into subproblems so that designing a solution to these subproblems gets easier. The solutions to all these individual parts are then merged to get the final solution of the original problem. It is like divide and merge approach.
Modular Approach for Programming :
The process of breaking a large problem into subproblems and then treating these individual parts as different functions is called modular programming. Each function behaves independent of another and there is minimal inter-functional communication. There are two methods to implement modular programming :
- Top Down Design : In this method, the original problem is divided into subparts. These subparts are further divided. The chain continues till we get the very fundamental subpart of the problem which can’t be further divided. Then we draw a solution for each of these fundamental parts.
- Bottom Up Design : In this style of programming, an application is written by using the pre-existing primitives of programming language. These primitives are then amalgamated with more complicated features, till the application is written. This style is just the reverse of the top-down design style.
3. Problem Designing: The design of a problem can be represented in either of the two forms :
The ways to execute any program are of three categories:
- Sequence Statements Here, all the instructions are executed in a sequence, that is, one after the another, till the program is executed.
- Selection Statements As it is self-clear from the name, in these type of statements the whole set of instructions is not executed. A selection has to be made. A selected number of instructions are executed based on some condition. If the condition holds true then some part of the instruction set is executed, otherwise, another part of the set is executed. Since this selection out of the instruction set has to be made, thus these type of instructions are called Selection Statements.
Identification of arithmetic and logical operations required for the solution : While writing the algorithm for a problem, the arithmetic and logical operations required for the solution are also usually identified. They help to write the code in an easier manner because the proper ordering of the arithmetic and logical symbols is necessary to determine the correct output. And when all this has been done in the algorithm writing step, it just makes the coding task a smoother one.
- Flow Chart : Flow charts are diagrammatic representation of the algorithm. It uses some symbols to illustrate the starting and ending of a program along with the flow of instructions involved in the program.
4. Coding: Once an algorithm is formed, it can’t be executed on the computer. Thus in this step, this algorithm has to be translated into the syntax of a particular programming language. This process is often termed as ‘coding’. Coding is one of the most important steps of the software life cycle. It is not only challenging to find a solution to a problem but to write optimized code for a solution is far more challenging.
Writing code for optimizing execution time and memory storage : A programmer writes code on his local computer. Now, suppose he writes a code which takes 5 hours to get executed. Now, this 5 hours of time is actually the idle time for the programmer. Not only it takes longer time, but it also uses the resources during that time. One of the most precious computing resources is memory. A large program is expected to utilize more memory. However, memory utilization is not a fault, but if a program is utilizing unnecessary time or memory, then it is a fault of coding. The optimized code can save both time and memory. For example, as has been discussed earlier, by using the minimum number of inputs to compute the output , one can save unnecessary memory utilization. All such techniques are very necessary to be deployed to write optimized code. The pragmatic world gives reverence not only to the solution of the problem but to the optimized solution. This art of writing the optimized code also called ‘competitive programming’.
5. Program Testing and Debugging: Program testing involves running each and every instruction of the code and check the validity of the output by a sample input. By testing a program one can also check if there’s an error in the program. If an error is detected, then program debugging is done. It is a process to locate the instruction which is causing an error in the program and then rectifying it. There are different types of error in a program : (i) Syntax Error Every programming language has its own set of rules and constructs which need to be followed to form a valid program in that particular language. If at any place in the entire code, this set of rule is violated, it results in a syntax error. Take an example in C Language
In the above program, the syntax error is in the first printf statement since the printf statement doesn’t end with a ‘;’. Now, until and unless this error is not rectified, the program will not get executed.
Once the error is rectified, one gets the desired output. Suppose the input is ‘good’ then the output is : Output:
(ii) Logical Error An error caused due to the implementation of a wrong logic in the program is called logical error. They are usually detected during the runtime. Take an example in C Language:
In the above code, the ‘for’ loop won’t get executed since n has been initialized with the value of 11 while ‘for’ loop can only print values smaller than or equal to 10. Such a code will result in incorrect output and thus errors like these are called logical errors. Once the error is rectified, one gets the desired output. Suppose n is initialised with the value ‘5’ then the output is : Output:
(iii) Runtime Error Any error which causes the unusual termination of the program is called runtime error. They are detected at the run time. Some common examples of runtime errors are : Example 1 :
If during the runtime, the user gives the input value for B as 0 then the program terminates abruptly resulting in a runtime error. The output thus appears is : Output:
Example 2 : If while executing a program, one attempts for opening an unexisting file, that is, a file which is not present in the hard disk, it also results in a runtime error.
6. Documentation : The program documentation involves :
- Problem Definition
- Problem Design
- Documentation of test perform
- History of program development
7. Program Maintenance: Once a program has been formed, to ensure its longevity, maintenance is a must. The maintenance of a program has its own costs associated with it, which may also exceed the development cost of the program in some cases. The maintenance of a program involves the following :
- Detection and Elimination of undetected errors in the existing program.
- Modification of current program to enhance its performance and adaptability.
- Enhancement of user interface
- Enriching the program with new capabilities.
- Updation of the documentation.
Control Structure- Conditional control and looping (finite and infinite)
There are codes which usually involve looping statements. Looping statements are statements in which instruction or a set of instructions is executed multiple times until a particular condition is satisfied. The while loop, for loop, do while loop, etc. form the basis of such looping structure. These statements are also called control structure because they determine or control the flow of instructions in a program. These looping structures are of two kinds :
In the above program, the ‘for’ loop gets executed only until the value of i is less than or equal to 10. As soon as the value of i becomes greater than 10, the while loop is terminated. Output:
In the above code, one can easily see that the value of n is not getting incremented. In such a case, the value of n will always remain 1 and hence the while loop will never get executed. Such loop is called an infinite loop. Output:
Please Login to comment...
- School Programming
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
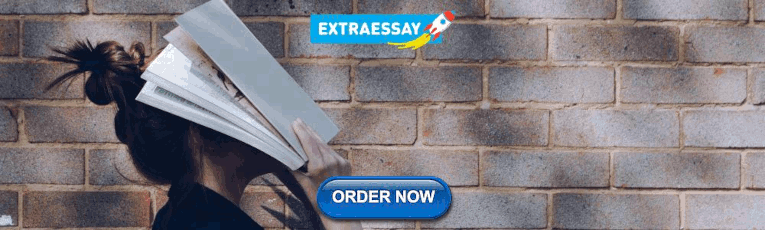
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Code With C
The Way to Programming
- C Tutorials
- Java Tutorials
- Python Tutorials
- PHP Tutorials
- Java Projects
Solving Common Python Programming Problems: Tips and Tricks
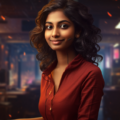
Sure, I’ll get started on crafting a humorous and fun blog post on solving common Python programming problems based on the provided outlines. Let’s dive into the world of Python programming with a touch of humor! 🐍✨
Identifying and Handling Common Python Programming Problems
Python programming can sometimes feel like herding cats 🐱💻—you think you’ve got everything under control until a wild syntax error jumps out of nowhere! 🙀 In this blog post, we will explore some of the most common Python programming problems and equip you with tips and tricks to tackle them head-on. So, grab your coding cape and let’s dive into the wacky world of Python problem-solving! 🦸♀️🚀
Syntax Errors
Understanding common syntax mistakes.
Picture this: you’re cruising through your Python code , feeling like a coding wizard 🧙♂️, when suddenly, a syntax error slaps you in the face! It’s like a sneaky ninja waiting to trip you up. From missing colons to unmatched parentheses, syntax errors can turn your code from hero to zero in seconds. But fear not, fellow coder! We’ve all been there, and with a few tricks up your sleeve, you’ll be dodging syntax errors like Neo dodges bullets! 💥💻
Let’s uncover the mysteries behind common syntax mistakes and how to outsmart them:
- Tip: Always double-check your indentation and sprinkle those colons like confetti! 🎉
- Tip: Pair up those parentheses and brackets like a match made in coding heaven! 💑
Debugging Techniques for Syntax Errors
Now, when it comes to debugging syntax errors, it’s all about channeling your inner detective 🕵️♀️. Sherlock your way through the code, follow the clues, and before you know it, you’ll be cracking the case of the elusive syntax bug! 🔍🐞 Here are some nifty techniques to up your debugging game:
- Print statements are your best friends! Sprinkle them like breadcrumbs through your code to track the elusive bug.
- Use an integrated development environment (IDE) with built-in syntax highlighting and error checking to catch those pesky mistakes before they catch you.
Logical Errors
Identifying logical flaws in code.
Logical errors are the mischievous pranksters of programming 🃏. Your code runs without a hiccup, but the output is as nonsensical as a unicorn in a library! 🦄📚 Identifying these sneaky buggers requires a keen eye for detail and a dash of Sherlock’s logic.
Here are some clues to uncover logical flaws in your code:
- Check your assumptions: Are you sure that variable should be a string and not an integer? Double-check your assumptions to reveal hidden gremlins.
- Walk through your code step by step: Sometimes, a manual run-through of your code can reveal logic leaps that only a trained sloth could make! 🦥💨
Strategies to Debug and Fix Logical Errors
When it’s time to face the music and debug those logical gremlins, arm yourself with these battle-tested strategies:
- Rubber ducky debugging: Yes, you heard that right! Explain your code to a rubber ducky or an unsuspecting friend 🦆. The act of verbalizing can miraculously unearth logical bugs.
- Divide and conquer: Break down your code into smaller chunks and test each part independently. It’s like chopping up a coding puzzle into bite-sized pieces.
Stay tuned for the next part where we’ll tackle performance issues, module import errors, and dive into the fascinating world of exception handling in Python! 🎩✨
Anxiously awaiting Part 2? Stay tuned for more Python problem-solving shenanigans coming your way! 🚀
Thank you for bearing with me through this fun-filled Python programming adventure! Remember, coding is not just about solving problems; it’s about enjoying the journey. Happy coding, fellow Pythonistas! 🐍💻🌟
Program Code – Solving Common Python Programming Problems: Tips and Tricks
Code output:.
- Reversed string: olleh
- First non-repeating character: w
- Convert list to number: 1234
Code Explanation:
The Python code snippet above solves three common programming problems with efficient solutions.
- Reverse a string without using string functions: We define a function reverse_string that takes a string s as input and reverses it without using any built-in string functions. The method iterates through each character in the input string, prepending it to a new string, resulting in a reversed string.
- Find the first non-repeated character in a string: The function first_non_repeating_char finds the first character in a string that does not repeat. It uses a dictionary counts to keep track of character occurrences and a list char_order to remember the order in which characters appear. The function then iterates through the ordered list of characters and returns the first one with a count of 1. If all characters repeat or if the string is empty, it returns ‘None’.
- Convert a list of numbers to a single number: The function convert_list_to_number takes a list of numbers and converts them into a single number by concatenating their digits. This is achieved by first converting each number in the list to a string, using the map function, and then joining them together before converting back to an integer.
Each of these functions showcases a common problem-solving approach in Python, highlighting the language’s capability to implement concise and effective solutions with minimal code. The code is written with clarity and is meticulously commented to ensure understanding and readability, demonstrating how to address some typical Python programming problems in an efficient manner.
Frequently Asked Questions (F&Q) on Solving Common Python Programming Problems: Tips and Tricks
Q1: what are some common python programming problems that developers face.
A: Common Python programming problems include issues with syntax errors, logic errors, and handling exceptions. Understanding these common pitfalls can help developers write more robust code.
Q2: How can I efficiently debug Python programming problems?
A: Use debugging tools like print statements, logging, and Python’s built-in debugger (pdb). Also, consider using IDEs with debugging capabilities such as PyCharm or Visual Studio Code .
Q3: Are there any tips for optimizing Python code to prevent performance problems?
A: Yes, optimizing Python code involves techniques like using appropriate data structures , avoiding unnecessary loops, and utilizing libraries like NumPy for numerical computations.
Q4: What should I do when facing challenges with Python package dependencies?
A: Managing dependencies in Python can be tricky. Utilize virtual environments (e.g., virtualenv or conda), use a package manager like pip , and consider using requirements.txt files to manage dependencies effectively.
Q5: How can I stay updated on best practices for Python programming to avoid common pitfalls?
A: Stay connected with the Python community through forums, blogs (like RealPython and Python.org), attend Python meetups or conferences, and follow influential Python developers on social media for valuable insights.
Q6: Is there a recommended approach for handling file I/O errors in Python programming?
A: When dealing with file I/O errors, use try-except blocks to catch specific exceptions (e.g., FileNotFoundError) and handle them gracefully. It’s also essential to close files properly to prevent resource leaks.
You Might Also Like
Adaptive software development: embracing change in technology projects, python vs. other programming languages: what sets it apart, getting started with the python programming language, the evolution of computer languages: python’s rise to prominence, utilizing python to write data to a file: techniques and tips.
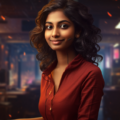
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Latest Posts
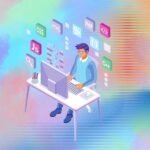
Efficient Group Planning Queries Over Spatial-Social Networks Data Mining Project
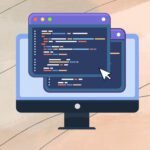
Efficient Network Generation Project for Keyword-Based Database Queries
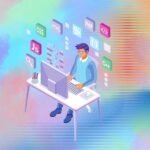
Unveiling Temporal Patterns for Event Sequence Clustering Project
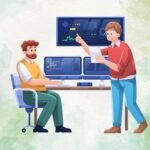
Cutting-Edge DGDFS Project: Predicting Adverse Drug-Drug Interaction
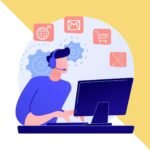
Enhancing Data Mining Projects: Cashless Society Privacy Project
Privacy overview.
Sign in to your account
Username or Email Address
Remember Me
- How to Improve Programming Skills in Python
Powerful, stable and flexible Cloud servers. Try Clouding.io today.
If you have worked in the programming field, or even considered going into programming, you are probably familiar with the famous words of Apple founder Steve Jobs:
“Everyone in this country should learn to program a computer, because it teaches you to think.”
Python is one of the most popular programming languages and can teach us a lot about critical thinking. But if you are in programming, you also know that there are important reasons to keep your programming skills up to date, especially with Python . In this article, we’ll consider some of the most important ways you can update your programming skills—and it isn’t just about learning more Python. Critical thinking is essential to programming and a great way to build your skills.
Why programmers need more than programming skills
According to HackerRank,
“Problem-solving skills are almost unanimously the most important qualification that employers look for […] more than programming languages proficiency, debugging, and system design.”
So how can you apply this to developing Python proficiency?
Obviously, the most important way to build programming skills in Python is to learn Python. Taking Python courses is a great place to start, and building toward more advanced Python learning will help you build technical skills. But programmers need more than just technical skills. You need to understand the best way to solve problems. While most people solve problems through brute force, but, this is not the best way to reach a solution. Instead, Python programmers need to develop a methodology for problem solving that will lead them to a well-crafted solution.
Improving Python Programming Skills in Four Steps
There are a few key steps, and they are listed below. However, it is not enough just to read them — you need to actually make them the part of your programming “life.”
- Evaluate the problem. Understand the programming issue you are attempting to overcome and all of the parts of the problem. In Python programming, a key skill is simply evaluating what needs to be done before you begin the process of programming a solution. Therefore, in any Python challenge, the first step is to study the problem in order to ascertain what you need to research and what skills you need to develop in order to begin to approach a solution. Frequently, if you find that you are able to explain the problem in plain English, it means that you understand it well enough to start to find a solution.
- Make a plan to handle the problem. In Python programming, as with any other type of programming problem, don’t simply launch into your programming without making a plan to handle potential problems logically from beginning to end. You want to begin from a position of strength, not simply start hacking and hoping for the best. Therefore, consider where you are starting and where you want to end up in order to map out the most logical way to arrange steps to reach that point.
- Make the problem manageable by dividing it up. When you are programming Python, it can be intimidating to tackle a major project of a major problem all at once. Instead, try dividing your next programming task into smaller steps that you can easily achieve as you move step by step through the programming or problem-solving process. This will not only make it easier to reach your goals but will also help you to celebrate small victories on your way, giving you the motivation to keep building on your successes. One of the best ways to achieve success is, to begin with, the smallest, easiest division to complete and use that success to build toward increasingly large and complex problems. Doing so will often help to simplify the larger tasks and make the overall project easier. As V. Anton Spraul said, “Reduce the problem to the point where you know how to solve it and write the solution. Then expand the problem slightly and rewrite the solution to match, and keep going until you are back where you started.”
- Practice your skills every day. Lastly, the most important way to develop your Python programming skills and how to troubleshoot Python code is to practice all the time. That doesn’t mean you have to seek out problems just to try to fix them. There are other ways to practice the same skill set in other ways. Elon Musk, for example, plays video games and Peter Thiel plays chess to build problem-solving skills that apply in many areas of life.
When Your Programming Skills Are not Enough
While all the tips above will certainly work if you actually apply them, you can rest assured that you will stumble upon many difficult tasks, which you won’t be able to crack without some assistance. Asking for help is one of the most efficient strategies of problem-solving. You can hire a tutor to help you gradually increase your programming skills, analyze your mistakes, etc. However, if the matter is urgent, you can choose another path — start with delegating your coding assignments to specialized services, and let experts help you with your homework here and now. Later, you can use the assignment done by professionals as tutorial material for other similar assignments. Let’s face it, if studying materials were of better quality and answered the current programming trends more accurately, students would need much less extra assistance.
If you are studying Python programming or trying to problem-solve in Python for a course, your biggest challenge is probably making it through your programming homework. Fortunately, if you have programming challenges, you can pay someone to do a programming assignment for you. Professional homework services like AssignmentCore have programming experts who can help with any type of coding project or Python assignment. There is a team of experts who are on stand-by to leap into action as soon as you have a Python challenge that you need an expert’s eye to complete so you can get ahead of the competition.
Author Bio:
Ted Wilson is a senior programming expert at AssignmentCore , a leading worldwide programming homework service. His main interests are Python, Java, MATLAB languages, and web development. He is responsible for providing customers with top-quality help with programming assignments of any complexity.
You Might Be Interested In
You’ll also like:.
- AWS Invoke One Lambda Function From Another
- AWS Cognito adminSetUserMFAPreference not setting MFA
- Unable to import module 'lambda_function' no module named 'lambda_function' | AWS Cognito | Lambda Function
- Python Try Except Else Finally
- How to Show Progress Bar in Python
- Post JSON to FastAPI
- Send Parameters to POST Request | FastAPI
- Passing Query Parameters in FastAPI
- Python API Using FastAPI
- No matching distribution found for fastapi
- Python Ternary Operator
- Download YouTube Videos Using Python | Source Code
- Python Script To Check Vaccine Availability | Source Code
- Create Login Page Using Python Flask & Bootstrap
- Python, Sorting Object Array
- Python : SyntaxError: Missing parentheses in call to 'print'.
- Python, Capitalize First Letter Of All Sentences
- Python, Capitalize First Letter In A Sentence
- Python, Check String Contains Another String
- Skills That Make You a Successful Python Developer
- Choosing the Right Python Framework in 2020: Django vs Flask
- How To Secure Python Apps
- Secure Coding in Python
- Building Serverless Apps Using Azure Functions and Python
- Development With Python in AWS
- How To Handle 404 Error In Python Flask
- How To Read And Display JSON using Python
- 6 Cool Things You Can Do with PyTorch - the Python-Native Deep Learning Framework
- How To Read Email From GMAIL API Using Python
- How to Implement Matrix Multiplication In Python
- How To Send Email Using Gmail In Python
- How PyMongo Update Document Works
- Python Flask Web Application On GE Predix
- How to Read Email From Gmail Using Python 3
- Understanding Regular expressions in Python
- Writing Error Log in Python Flask Web Application
- How to Create JSON Using Python Flask
- Creating a Web App Using Python Flask, AngularJS & MongoDB
- Insert, Read, Update, Delete in MongoDB using PyMongo
- Python REST API Authentication Using AngularJS App
- Working with JSON in Python Flask
- What does __name__=='__main__' mean in Python ?
- Python Flask jQuery Ajax POST
- Python Web Application Development Using Flask MySQL
- Flask AngularJS app powered by RESTful API - Setting Up the Application
- Creating RESTful API Using Python Flask & MySQL - Part 2
- Creating Flask RESTful API Using Python & MySQL
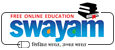
Problem solving Aspects and Python Programming
Page Visits
Course layout, books and references, instructor bio.
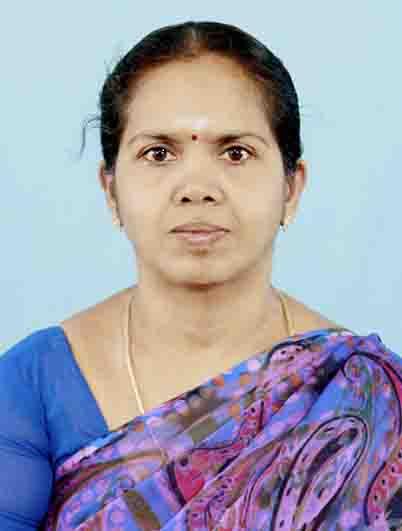
Dr.S.Malliga, Dr.R.Thangarajan, Dr.S.V.Kogilavani
Course certificate.

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :
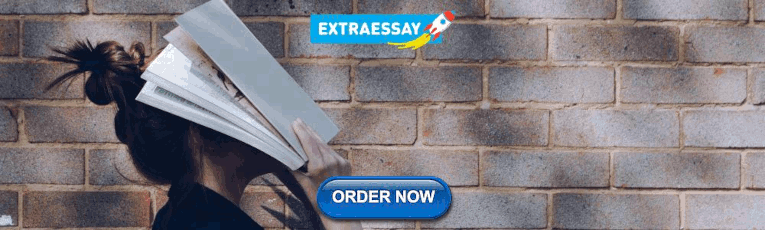
IMAGES
VIDEO
COMMENTS
Problem Solving in Python. Problem-solving is the process of identifying a problem, creating an algorithm to solve the given problem, and finally implementing the algorithm to develop a computer program. An algorithm is a process or set of rules to be followed while performing calculations or other problem-solving operations.
Lesson 3 - Problem solving techniques. In the last lesson, we learned control flow statements such as if-else and for. In this lesson, let us try to write a program for this problem: "Given a day, the program should print if it is a weekday or weekend.". $ python3 day_of_the_week.py monday. weekday. $ python3 day_of_the_week.py sunday. weekend.
Python core algorithms include sorting and searching, each with its unique applications and efficiencies. Quick Sort: A divide-and-conquer algorithm that picks an element as a pivot and partitions the array around the pivot. It's efficient for large datasets. Example: def quicksort(arr): if len(arr) <= 1: return arr.
In this course you will see how to author more complex ideas and capabilities in Python. In technical terms, you will learn dictionaries and how to work with them and nest them, functions, refactoring, and debugging, all of which are also thinking tools for the art of problem solving. We'll use this knowledge to explore our browsing history ...
Course Overview. As a developer, mastering the concepts of algorithms and being proficient in implementing them is essential to improving problem-solving skills. This course aims to equip you with an in-depth understanding of algorithms and how they can be utilized for problem-solving in Python. Starting with the basics, you'll gain a ...
Contents 1 Introduction 1 1.1 Modern Computers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .1 1.2 Computer Languages ...
1.4 Problem-Solving Strategies. 1.4. Problem-Solving Strategies. Problem solving happens on three different levels: Strategy: A high-level idea for finding a solution. Tactics: Methods or patterns that work in many different settings. Tools: Tricks and techniques that are used in specific situations. As you progress through this text, you will ...
Example: Solving a Sorting Problem. Let's look at a simple algorithmic problem: sorting a list of numbers in ascending order. Here is a Python function that uses the Bubble Sort algorithm to solve this problem: numbers[j], numbers[j + 1] = numbers[j + 1], numbers[j] return numbers. The bubble_sort function sorts the list of numbers by ...
Welcome to the world of problem solving with Python! This first Orientation chapter will help you get started by guiding you through the process of installing Python on your computer. By the end of this chapter, you will be able to: Describe why Python is a useful computer language for problem solvers. Describe applications where Python is used.
Website companion for the book Problem Solving with Python by Peter D. Kazarinoff
Linear Programming With Python. The basic method for solving linear programming problems is called the simplex method, which has several variants. Another popular approach is the interior-point method. ... The approach for defining and solving the problem is the same as in the previous example: Python
This method capitalizes on the principle that solving smaller instances of a problem can lead to an efficient solution for the larger, original problem. General Strategy of Divide and Conquer:
9. Problem-Solving Strategies To become a proficient Python programmer, focus on developing problem-solving skills. Break down complex problems into smaller, manageable tasks, and use algorithms and data structures efficiently. Online coding platforms like LeetCode and HackerRank offer a wealth of programming challenges to sharpen your problem ...
At its core, an algorithm is a systematic, step-by-step procedure or set of rules designed to solve a problem or perform a specific task. It provides clear instructions that, when followed meticulously, lead to the desired outcome. Consider an algorithm to be akin to a recipe for your favorite dish.
Problem solving technique is a set of techniques that helps in providing logic for solving a problem. Problem solving can be expressed in the form of 1. Algorithms. 2. Flowcharts. ... ELSE are the conditional structures used in Python language. •CASE is the structure used to select multi way selection control. It is not supported in Python ...
The process of problem-solving is an activity which has its ingredients as the specification of the program and the served dish is a correct program. This activity comprises of four steps : 1. Understanding the problem: To solve any problem it is very crucial to understand the problem first. What is the desired output of the code and how that ...
In this video, I explain some simple problem solving techniques programmers use to break down and solve problems.To learn more Python programming, check out ...
Copy Code. # Solving Common Python Programming Problems: Tips and Tricks. # Problem 1: Reverse a string without using string functions. def reverse_string(s): '''. This function takes a string and returns the reversed string without using built-in functions. '''. reversed_string = ''. for char in s:
As V. Anton Spraul said, "Reduce the problem to the point where you know how to solve it and write the solution. Then expand the problem slightly and rewrite the solution to match, and keep going until you are back where you started.". Practice your skills every day. Lastly, the most important way to develop your Python programming skills ...
While this solution takes a literal approach to solving the Caesar cipher problem, you could also use a different approach modeled after the .translate() solution in practice problem 2. Solution 2. The second solution to this problem mimics the behavior of Python's built-in method .translate(). Instead of shifting each letter by a given ...
Each exercise has 10-20 Questions. The solution is provided for every question. Practice each Exercise in Online Code Editor. These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Example 3: Marketing Budget Optimization solved by Pyomo. Pyomo is an open-source Python modelling language for mathematical optimization that supports the modelling of complex systems with linear ...
This course is designed for use by freshmen students taking their first course in programming. It deals with the techniques needed to practice computational thinking, the art of using computers to solve problems and the ways the computers can be used to solve problems. The Second part covers Python Programming.