Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
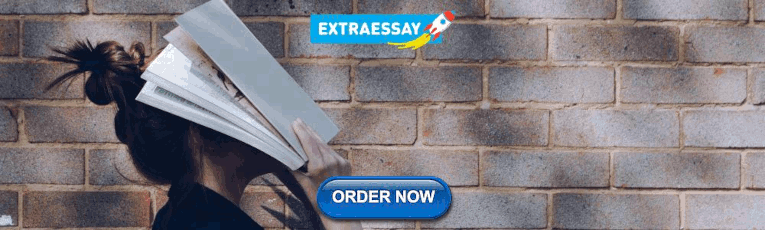
Implement Graph Data Structure in C
This post will cover graph data structure implementation in C using an adjacency list. The post will cover both weighted and unweighted implementation of directed and undirected graphs.
In the graph’s adjacency list representation, each vertex in the graph is associated with the collection of its neighboring vertices or edges, i.e., every vertex stores a list of adjacent vertices.

For example, for the above graph, below is its adjacency list pictorial representation:
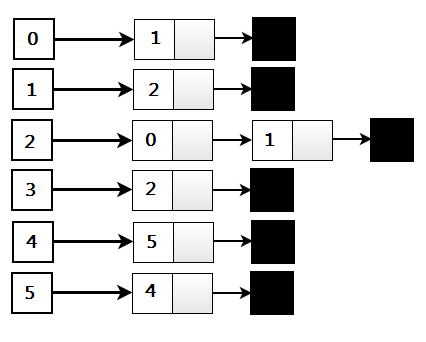
1. Directed Graph Implementation
Following is the C implementation of a directed graph using an adjacency list:
Download Run Code
Output: (0 —> 1) (1 —> 2) (2 —> 1) (2 —> 0) (3 —> 2) (4 —> 5) (5 —> 4)
As evident from the above code, in a directed graph, we only create an edge from src to dest in the adjacency list. Now, if the graph is undirected, we also need to create an edge from dest to src in the adjacency list, as shown below:
Output: (0 —> 2) (0 —> 1) (1 —> 2) (1 —> 2) (1 —> 0) (2 —> 3) (2 —> 1) (2 —> 0) (2 —> 1) (3 —> 2) (4 —> 5) (4 —> 5) (5 —> 4) (5 —> 4)
2. Weighted Directed Graph Implementation
In a weighted graph, each edge will have weight (or cost) associated with it, as shown below:

Following is the implementation of a weighted directed graph in C using the adjacency list. The implementation is similar to that of an unweighted directed graph, except we are also storing weight info along with every edge.
Output: 0 —> 1 (6) 1 —> 2 (7) 2 —> 1 (4) 2 —> 0 (5) 3 —> 2 (10) 4 —> 5 (1) 5 —> 4 (3)
For weighted undirected graphs (as seen before for unweighted undirected graphs), create a path from dest to src as well in the adjacency list. The complete implementation can be seen here .
Also see:
Graph Implementation in C++ (without using STL)
Graph Implementation in C++ using STL
Graph Implementation in Java using Collections
Graph Implementation in Python
Rate this post
Average rating 4.72 /5. Vote count: 278
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Note: You are looking at a static copy of the former PineWiki site, used for class notes by James Aspnes from 2003 to 2012. Many mathematical formulas are broken, and there are likely to be other bugs as well. These will most likely not be fixed. You may be able to find more up-to-date versions of some of these notes at http://www.cs.yale.edu/homes/aspnes/#classes .
2. Why graphs are useful
3. operations on graphs, 4. representations of graphs, 4.1. adjacency matrices, 4.2. adjacency lists, 4.2.1. an implementation, 4.3. implicit representations, 5. searching for paths in a graph.
- Start with a tree consisting of just s.
- If there is at least one edge that leaves the tree (i.e. goes from a node in the current tree to a node outside the current tree), pick the "best" such edge and add it and its sink to the tree.
- Repeat step 2 until no edges leave the tree.
5.1. Depth-first and breadth-first search
5.2. other variations on the basic algorithm.

Graph Data Structure
In this tutorial, you will learn an important data structure, Graph. You will also discover basic terminology, types of graphs, how graphs are represented in memory and their applications.
A graph is a non-linear data structure consisting of vertices and edges that connect these vertices.
A graph is an ordered set G = (V, E) consist of two sets: V and E, where
- V is the set of nodes (vertices, points or nodes)
- E is the set of edges , identified with a unique pair of nodes in V, denoted by e=(u, v) .
The following figure shows an example of a graph where V(G) = {A, B, C, D, E} and E(G) = { (A,B), (B,C), (C,D), (D,E), (E,A), (A,D) }

Graph Terminology
Let e = [u, v]. Then nodes u and v are referred to as endpoints of e, and u and v are referred to as adjacent nodes or neighbours.
The number of edges containing u is the degree of node u.
If u does not belong to any edge (degree of u = 0), then u is referred to as an isolated edge .
A finite sequence of edges that connects a series of vertices is called a path .
The number of edges in a path is the length of the path.

A path is said to be closed if it starts and ends at the same node.
A path is considered to be simple if all of its nodes are distinct, except the initial and last node.

A cycle is a closed simple path with a length of 3 or more.

A graph is considered to be connected if and only if any two of its nodes have a path between them.
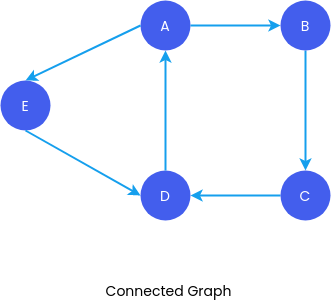
If every node u in a graph is adjacent to every other node v in the graph, then the graph is said to be complete .

An edge e is called a loop if it has identical endpoints, that is if e = [u,u].
When multiple edges in a graph connect the same pair of nodes, the edges are referred to as parallel edges .

A multigraph is a graph that contains a self-loop, parallel edges, or both.
Types of Graphs
Directed and undirected graph.
A graph with edges that don’t have direction is called an undirected graph . The edges in an undirected graph indicate a two-way relationship.
A Directed graph (digraph) is a graph that has edges with direction. These edges represent a one-way relationship. That is edges can be only traversed in one direction.

If a digraph has a directed edge e = [u, v], then
- e begins at u, and ends at v.
- u is the origin or initial point of e, and v is the destination or terminal point of e.
The number of edges beginning at a node u is its outdegree . The number of edges that finish at a node u is its indegree . If a node u has a positive outdegree but a negative indegree, it is referred to as a source . If a node u has a zero outdegree but a positive indegree, it is referred to as a sink .
If there is a directed path from v to u, then node v is said to be reacha ble from u. A directed graph G is said to be connected (strongly connected) if there is a path from u to v and also a path from v to u for each pair u, v of nodes in the graph.
Weighted Graph
A weighted graph is a graph that has a number associated with each of its edges. The number is called the weight of the edge. A weighted graph can be undirected or directed. The weight of a path P is the sum of the weights of the edges along the path.

Representation of Graphs
Graphs are typically represented in one of three ways:
Sequential Representation : Adjacency Matrix
A graph with N nodes can be represented by an NxN square matrix with row and column numbers representing the vertices. A cell at the cross-section of the vertices can only have the values 0 or 1. An entry in the adjacency matrix [V i , V j ] = 1 only if there exists an edge from V i to V j . A graph and its corresponding adjacency matrix are illustrated below:

In the case of a weighted graph, the weight of the edge is saved instead of the value 1. Since the adjacency matrix will be sparse, a lot of space will be wasted. Adding or removing new nodes may be difficult.
Linked Representation
The linked representation of a graph contains two lists, a NODE list and an EDGE (Adjacency) list.
Each element in the NODE list will correspond to a node in the graph. It contains:
- Name of the Node.
- Pointer to adjacent nodes .
- Pointer to next node .
Each element in the edge list will represent an edge of the graph. It contains:
- Destination node of the edge.
- Link that link together the edges with the same initial node.
A graph and its corresponding linked representation are illustrated below.

Because we only need to keep the values for the edges, an adjacency list is efficient in terms of storage.
Applications of Graph
- In circuit networks: connection points as vertices and wires as edges.
- In transport network: stations as vertices and routes as edges.
- For finding shortest paths.

- In state diagrams: states as vertices and transition between states as edges.
- For scientific computations.
- In recommendation algorithms.

Welcome.please sign up.
By signing up or logging in, you agree to our Terms of service and confirm that you have read our Privacy Policy .
Already a member? Go to Log In
Welcome.please login.
Forgot your password
Not registered yet? Go to Sign Up
- Introduction
- Analyze Your Algorithm
- Growth of a Function
- Analyze Iterative Algorithms
- Recurrences
- Let's Iterate
- Now the Recursion
- Master's Theorem
- Sort It Out
- Bubble Sort
- Count and Sort
- Divide and Conquer
- Binary Search
- Dynamic Programming
- Knapsack Problem
- Rod Cutting
- Coin Change
- Backtracking
- Knight's Tour Problem
- Greedy Algorithm
- Activity Selection
- Egyptian Fraction
- Huffman Codes
- Minimum Spanning Tree
- Prim's Algorithm
In simplest terms, a graph is a combination of vertices (or nodes) and edges.

In the above picture, we have 4 nodes and 4 edges and it is a graph.
Graph is a very important data structure to store data which are connected to each other. The simplest example is the network of roads to connect different cities.

We can see how different cities and roads are mapped into different nodes and edges to form a graph.
There are many other relationships which are stored using graphs efficiently. For example, electrical circuits, people network on social media like Facebook, etc.

We represent a graph as G=(V, E), where V is the set of all of its vertices and E is the set of all of its edges.
We are going to use |V| and |E| to represent the number of vertices and number of edges respectively.
Let's have a look at different types of graphs which are generally used.
Types of Graphs
Undirected graph and directed graph.
A graph in which if there is an edge connecting two vertices A and B, implies that B is also connected back to A is an undirected graph .

In the above graph, 1 is connected to 2 and 2 is connected back to 1 and this is true for every edge of the graph. So, the graph is an undirected graph.
In other words, we can say that a graph G=(V,E) is undirected if an edge (i, j) (edge from i to j) $\in$ E implies that the edge (j, i) $\in$ E also.
Two lane roads on which vehicles can go in either direction are the example of undirected graphs.

In a directed graph , the edges are directed i.e., they have a direction. So, if the edge is an edge from i to j i.e., (i, j) $\in$ E, then this doesn't necessarily mean that there will be also an edge from j to i.

This is a directed graph as there is a path from 1 to 2 but there isn't any path from 2 to 1.
Suppose a person is following someone on Twitter but may or may not be followed back. This can be represented by directed graphs.
Cyclic and Acyclic Graph
In a cyclic graph, there are cycles or loops formed by the edges but this doesn't happen with an acyclic graph.

Weighted and Unweighted Graph
Sometimes weights are given to the edges of a graph and these are called weighted graphs. For example, in a graph representing roads and cities, giving the length of the road as weight is a logical choice.

Dense and Sparse Graph
When the number of edges (|E|) is close to the square of the number of vertices (|V| 2 ), then the graph is a dense graph. To visualize, you can imagine a graph with few vertices and lots of edges between them.
If |E| is much less than |V| 2 , then it is a sparse graph.
Simple and Non-simple Graph
The graph which has self-loops or an edge (i, j) occurs more than once (also called multiedge and graph is called multigraph) is a non-simple graph.

Connected and Disconnected Graph
If every node of a graph is connected to some other nodes is a connected graph. However, if there is at least one node which is not connected to any other node, then it is a disconnected graph.
Complete Graph
When each node of a graph is connected to every other node, then it is called a complete graph.

Now, we have an idea of what basically is a graph. Now, we need to know how to use a graph in our program and for that we need to learn how to represent a graph.
Representation of Graphs
- Adjacency-list representation
- Adjacency-matrix representation
According to their names, we use lists in the case of adjacency-list representation and a matrix (2D array) in the case of adjacency matrix representation.
The way in which we are going to represent our graph depends on the task we have to perform. This will be clear after studying these representations in detail.
Adjacency-matrix Representation
In the adjacency-matrix representation, we use a |V|x|V| matrix to represent a graph.

Here, we are assuming that the vertices of the graph are numbered from 1 to |V|. Now for the cell (i, j) of this matrix, we set its value 1 if we have an edge from i to j (or (i, j) $\in$ E), otherwise 0.

In the above picture, we have an edge from 1 to 2, so the cell (1, 2) is 1, but there is no edge connecting 2 back to 1, so the cell (2, 1) is 0.
Similarly, there is no edge from the vertex 4 to any other vertices, so (4, 1), (4, 2), ... , (4, 5) all are 0.
It is quite clear that we are using a |V|x|V| matrix to represent a graph G=(V, E), so the space required to store this would be $\Theta(|V|^2)$.
Now imagine a social media site like Facebook, where there are billions of users. Using a matrix will take quite a large amount of space but the real problem is that even among these billions of people, a person is not connected most of the other person i.e., if each person on average has 1000 friends, then most of the cells are going to be empty (or 0). In this case (and most of the cases), we use adjaceny-list representation.
However, there is also one advantage of this representation - we can tell if one node is connected to another node in no time ($\Theta(1)$ to be appropriate).
We can say that using an adjacency-list for a sparse graph and adjacency-matrix for a dense graph is a general choice.
In most of the applications, the number of nodes which are connected from a node is much less than the total number of nodes. So, we move to adjacency-list representation.
Adjacency-list Representation
In adjacency-list representation, we have a list of all the nodes and for each node, we have a list of nodes for which the node has an edge.

For example, for the above graph, we will have a list of all the nodes.

Now, for each of these nodes, we will store a list (array, hash or linked list) of other nodes which have an edge from these nodes (or adjacent nodes). So, node 1 will have a list containing nodes 2 and 3, node 2 will have a list containing node 4, etc.

Thus, in an adjency-list representation, we have an array (Adj) of |V| lists and each element of this array Adj i.e., Adj[i] consists all the vertices adjacent to it.
The total number of items in all these lists is the number of edges in the graph i.e., |E|, if the graph is directed. But if the graph is undirected, then the total number of items in these adjacency lists will be 2|E| because for any edge (i, j), i will appear in adjacency list j and vice-versa.

Now, the total space taken to store this graph will be space needed to store all adjacency list + space needed to store the lists of vertices i.e., |V|.
So, it requires $\Theta(|V| + |E|)$ space.
We can also add a new vertex in an adjacency -list in $O(1)$ time but doing the same will require $O(|V|^2)$ time in the case of an adjacency-matrix representation because a |V+1|x|V+1| matrix is needed to be reconstructed.
You can learn about linked lists from this chapter .
Now, we know what is a graph and how to use it. So, let's move ahead and study some algorithms related to the graphs.
- Linked lists in C (Singly linked list)
- C++ : Linked lists in C++ (Singly linked list)
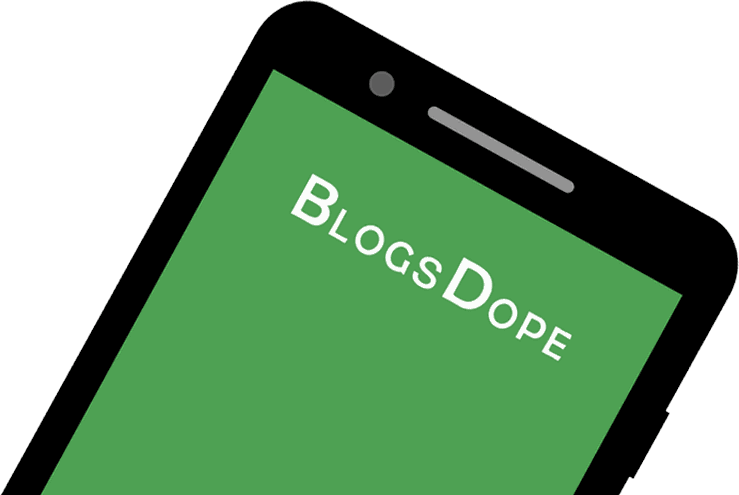
- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Graph Data Structure And Algorithms
- Introduction to Graphs - Data Structure and Algorithm Tutorials
Graph and its representations
- Types of Graphs with Examples
- Basic Properties of a Graph
- Applications, Advantages and Disadvantages of Graph
- Transpose graph
- Difference Between Graph and Tree
BFS and DFS on Graph
- Breadth First Search or BFS for a Graph
- Depth First Search or DFS for a Graph
- Applications, Advantages and Disadvantages of Depth First Search (DFS)
- Applications, Advantages and Disadvantages of Breadth First Search (BFS)
- Iterative Depth First Traversal of Graph
- BFS for Disconnected Graph
- Transitive Closure of a Graph using DFS
- Difference between BFS and DFS
Cycle in a Graph
- Detect Cycle in a Directed Graph
- Detect cycle in an undirected graph
- Detect Cycle in a directed graph using colors
- Detect a negative cycle in a Graph | (Bellman Ford)
- Cycles of length n in an undirected and connected graph
- Detecting negative cycle using Floyd Warshall
- Clone a Directed Acyclic Graph
Shortest Paths in Graph
- How to find Shortest Paths from Source to all Vertices using Dijkstra's Algorithm
- Bellman–Ford Algorithm
- Floyd Warshall Algorithm
- Johnson's algorithm for All-pairs shortest paths
- Shortest Path in Directed Acyclic Graph
- Multistage Graph (Shortest Path)
- Shortest path in an unweighted graph
- Karp's minimum mean (or average) weight cycle algorithm
- 0-1 BFS (Shortest Path in a Binary Weight Graph)
- Find minimum weight cycle in an undirected graph
Minimum Spanning Tree in Graph
- Kruskal’s Minimum Spanning Tree (MST) Algorithm
- Difference between Prim's and Kruskal's algorithm for MST
- Applications of Minimum Spanning Tree
- Total number of Spanning Trees in a Graph
- Minimum Product Spanning Tree
- Reverse Delete Algorithm for Minimum Spanning Tree
Topological Sorting in Graph
- Topological Sorting
- All Topological Sorts of a Directed Acyclic Graph
- Kahn's algorithm for Topological Sorting
- Maximum edges that can be added to DAG so that it remains DAG
- Longest Path in a Directed Acyclic Graph
- Topological Sort of a graph using departure time of vertex
Connectivity of Graph
- Articulation Points (or Cut Vertices) in a Graph
- Biconnected Components
- Bridges in a graph
- Eulerian path and circuit for undirected graph
- Fleury's Algorithm for printing Eulerian Path or Circuit
- Strongly Connected Components
- Count all possible walks from a source to a destination with exactly k edges
- Euler Circuit in a Directed Graph
- Word Ladder (Length of shortest chain to reach a target word)
- Find if an array of strings can be chained to form a circle | Set 1
- Tarjan's Algorithm to find Strongly Connected Components
- Paths to travel each nodes using each edge (Seven Bridges of Königsberg)
- Dynamic Connectivity | Set 1 (Incremental)
Maximum flow in a Graph
- Max Flow Problem Introduction
- Ford-Fulkerson Algorithm for Maximum Flow Problem
- Find maximum number of edge disjoint paths between two vertices
- Find minimum s-t cut in a flow network
- Maximum Bipartite Matching
- Channel Assignment Problem
- Introduction to Push Relabel Algorithm
- Introduction and implementation of Karger's algorithm for Minimum Cut
- Dinic's algorithm for Maximum Flow
Some must do problems on Graph
- Find size of the largest region in Boolean Matrix
- Count number of trees in a forest
- A Peterson Graph Problem
- Clone an Undirected Graph
- Introduction to Graph Coloring
- Traveling Salesman Problem (TSP) Implementation
- Introduction and Approximate Solution for Vertex Cover Problem
- Erdos Renyl Model (for generating Random Graphs)
- Chinese Postman or Route Inspection | Set 1 (introduction)
- Hierholzer's Algorithm for directed graph
- Boggle (Find all possible words in a board of characters) | Set 1
- Hopcroft–Karp Algorithm for Maximum Matching | Set 1 (Introduction)
- Construct a graph from given degrees of all vertices
- Determine whether a universal sink exists in a directed graph
- Number of sink nodes in a graph
- Two Clique Problem (Check if Graph can be divided in two Cliques)
What is Graph Data Structure?
A Graph is a non-linear data structure consisting of vertices and edges. The vertices are sometimes also referred to as nodes and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph is composed of a set of vertices( V ) and a set of edges( E ). The graph is denoted by G(V, E) .
Representations of Graph
Here are the two most common ways to represent a graph :
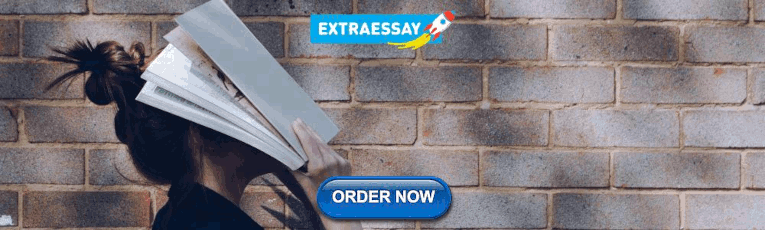
Adjacency Matrix
Adjacency list.
An adjacency matrix is a way of representing a graph as a matrix of boolean (0’s and 1’s).
Let’s assume there are n vertices in the graph So, create a 2D matrix adjMat[n][n] having dimension n x n.
If there is an edge from vertex i to j , mark adjMat[i][j] as 1 . If there is no edge from vertex i to j , mark adjMat[i][j] as 0 .
Representation of Undirected Graph to Adjacency Matrix:
The below figure shows an undirected graph. Initially, the entire Matrix is initialized to 0 . If there is an edge from source to destination, we insert 1 to both cases ( adjMat[destination] and adjMat [ destination]) because we can go either way.
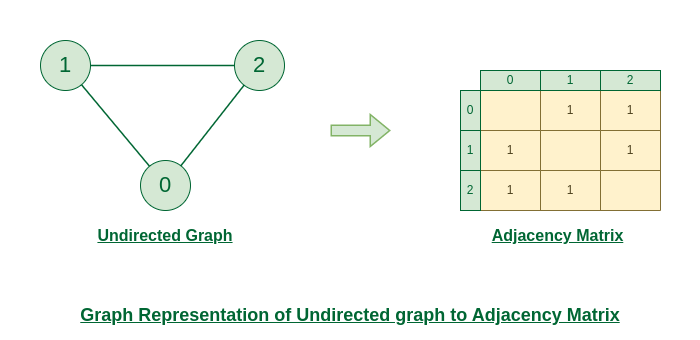
Undirected Graph to Adjacency Matrix
Representation of Directed Graph to Adjacency Matrix:
The below figure shows a directed graph. Initially, the entire Matrix is initialized to 0 . If there is an edge from source to destination, we insert 1 for that particular adjMat[destination] .
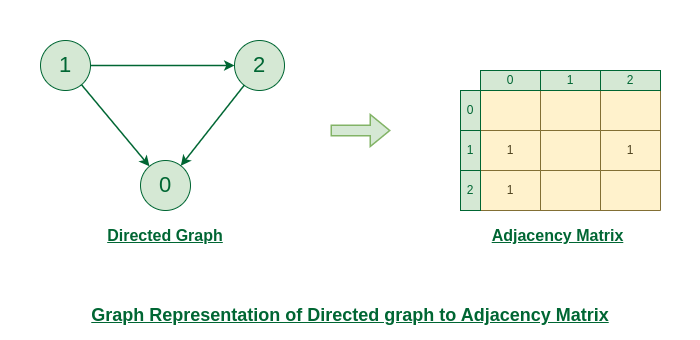
Directed Graph to Adjacency Matrix
An array of Lists is used to store edges between two vertices. The size of array is equal to the number of vertices (i.e, n) . Each index in this array represents a specific vertex in the graph. The entry at the index i of the array contains a linked list containing the vertices that are adjacent to vertex i .
Let’s assume there are n vertices in the graph So, create an array of list of size n as adjList[n].
adjList[0] will have all the nodes which are connected (neighbour) to vertex 0 . adjList[1] will have all the nodes which are connected (neighbour) to vertex 1 and so on.
Representation of Undirected Graph to Adjacency list:
The below undirected graph has 3 vertices. So, an array of list will be created of size 3, where each indices represent the vertices. Now, vertex 0 has two neighbours (i.e, 1 and 2). So, insert vertex 1 and 2 at indices 0 of array. Similarly, For vertex 1, it has two neighbour (i.e, 2 and 0) So, insert vertices 2 and 0 at indices 1 of array. Similarly, for vertex 2, insert its neighbours in array of list.
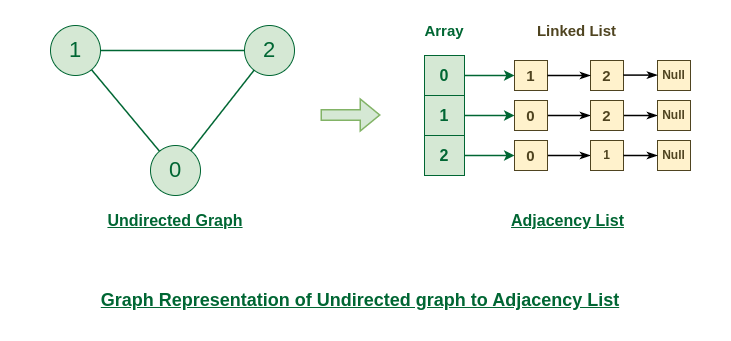
Undirected Graph to Adjacency list
Representation of Directed Graph to Adjacency list:
The below directed graph has 3 vertices. So, an array of list will be created of size 3, where each indices represent the vertices. Now, vertex 0 has no neighbours. For vertex 1, it has two neighbour (i.e, 0 and 2) So, insert vertices 0 and 2 at indices 1 of array. Similarly, for vertex 2, insert its neighbours in array of list.
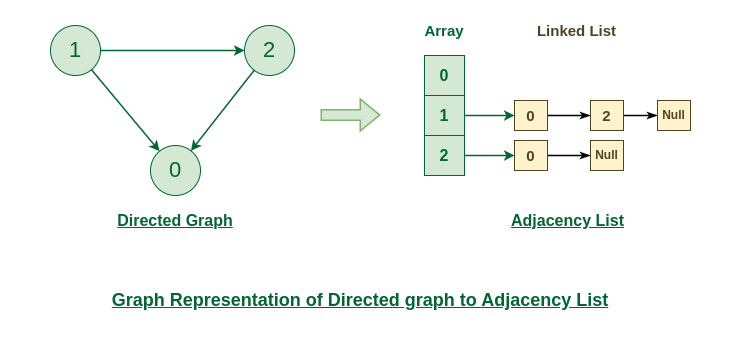
Directed Graph to Adjacency list
Please Login to comment...
- graph-basics
- 10 Best Free Social Media Management and Marketing Apps for Android - 2024
- 10 Best Customer Database Software of 2024
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, dsa introduction.
- What is an algorithm?
- Data Structure and Types
- Why learn DSA?
- Asymptotic Notations
- Master Theorem
- Divide and Conquer Algorithm
Data Structures (I)
- Types of Queue
- Circular Queue
- Priority Queue
Data Structures (II)
- Linked List
- Linked List Operations
- Types of Linked List
- Heap Data Structure
- Fibonacci Heap
- Decrease Key and Delete Node Operations on a Fibonacci Heap
Tree based DSA (I)
- Tree Data Structure
- Tree Traversal
- Binary Tree
- Full Binary Tree
- Perfect Binary Tree
- Complete Binary Tree
- Balanced Binary Tree
- Binary Search Tree
Tree based DSA (II)
- Insertion in a B-tree
- Deletion from a B-tree
- Insertion on a B+ Tree
- Deletion from a B+ Tree
- Red-Black Tree
- Red-Black Tree Insertion
- Red-Black Tree Deletion
Graph based DSA
- Graph Data Structure
- Spanning Tree
- Strongly Connected Components
Adjacency Matrix
Adjacency List
- DFS Algorithm
- Breadth-first Search
- Bellman Ford's Algorithm
Sorting and Searching Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Counting Sort
- Bucket Sort
- Linear Search
- Binary Search
Greedy Algorithms
- Greedy Algorithm
- Ford-Fulkerson Algorithm
- Dijkstra's Algorithm
- Kruskal's Algorithm
- Prim's Algorithm
- Huffman Coding
- Dynamic Programming
- Floyd-Warshall Algorithm
- Longest Common Sequence
Other Algorithms
- Backtracking Algorithm
- Rabin-Karp Algorithm
DSA Tutorials
Graph Data Stucture
Depth First Search (DFS)
Breadth first search
An adjacency list represents a graph as an array of linked lists. The index of the array represents a vertex and each element in its linked list represents the other vertices that form an edge with the vertex.
For example, we have a graph below.
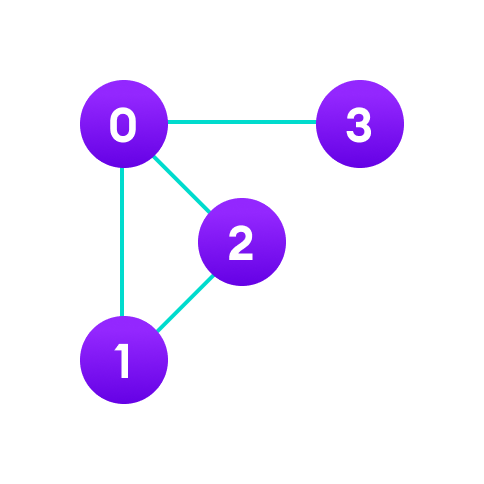
We can represent this graph in the form of a linked list on a computer as shown below.
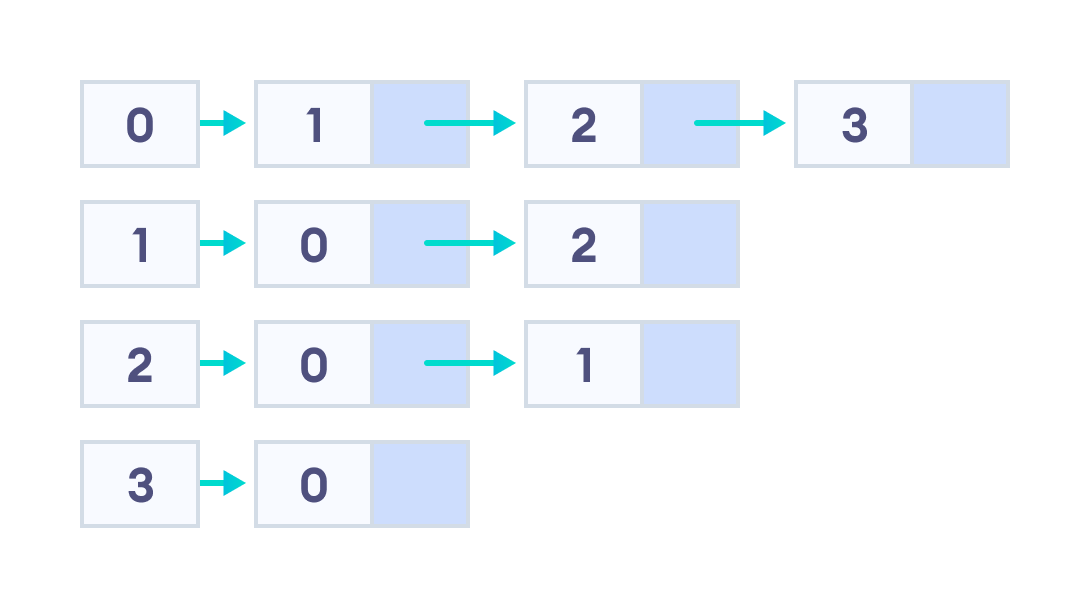
Here, 0 , 1 , 2 , 3 are the vertices and each of them forms a linked list with all of its adjacent vertices. For instance, vertex 1 has two adjacent vertices 0 and 2. Therefore, 1 is linked with 0 and 2 in the figure above.
- Pros of Adjacency List
- An adjacency list is efficient in terms of storage because we only need to store the values for the edges. For a sparse graph with millions of vertices and edges, this can mean a lot of saved space.
- It also helps to find all the vertices adjacent to a vertex easily.
- Cons of Adjacency List
- Finding the adjacent list is not quicker than the adjacency matrix because all the connected nodes must be first explored to find them.
- Adjacency List Structure
The simplest adjacency list needs a node data structure to store a vertex and a graph data structure to organize the nodes.
We stay close to the basic definition of a graph - a collection of vertices and edges {V, E} . For simplicity, we use an unlabeled graph as opposed to a labeled one i.e. the vertices are identified by their indices 0,1,2,3.
Let's dig into the data structures at play here.
Don't let the struct node** adjLists overwhelm you.
All we are saying is we want to store a pointer to struct node* . This is because we don't know how many vertices the graph will have and so we cannot create an array of Linked Lists at compile time.
- Adjacency List C++
It is the same structure but by using the in-built list STL data structures of C++, we make the structure a bit cleaner. We are also able to abstract the details of the implementation.
- Adjacency List Java
We use Java Collections to store the Array of Linked Lists.
The type of LinkedList is determined by what data you want to store in it. For a labeled graph, you could store a dictionary instead of an Integer
- Adjacency List Python
There is a reason Python gets so much love. A simple dictionary of vertices and its edges is a sufficient representation of a graph. You can make the vertex itself as complex as you want.
- Adjacency List Code in Python, Java, and C/C++
- Applications of Adjacency List
- It is faster to use adjacency lists for graphs having less number of edges.
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
DS & Algorithms
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Graphics program in c.
Last Updated on January 8, 2024 by Ankit Kochar
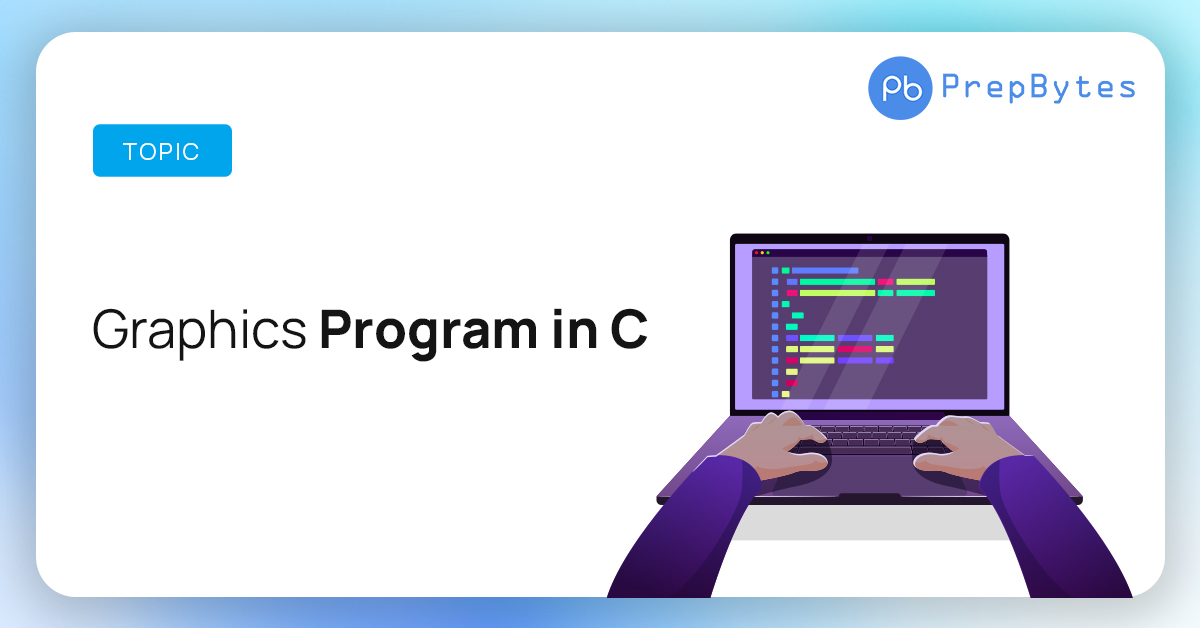
Graphics programming in C opens up a realm of creative possibilities, allowing developers to generate visual representations, animations, and interactive applications. Whether creating simple graphics or sophisticated user interfaces, mastering the principles of graphics programming is essential. This introduction explores the foundational aspects of graphics programming in C, highlighting its significance and potential applications.
What is Graphics Program in C
Graphics program in C involves libraries such as OpenGL, GDI, or Allegro to create 2D and 3D graphics, multimedia applications, and games. A basic understanding of programming concepts is required, along with a development environment such as Code::Blocks or Visual Studio. Applications of graphics programming in C include game development, computer-aided design, visualization and data analysis, multimedia applications, user interfaces, and animation and special effects.
Libraries of Graphic Program in C
Here are some popular graphic libraries for C programming:
- OpenGL: It provides a powerful set of tools for developing 3D graphics applications.
- Simple Direct Media Layer: It is a multimedia library created to offer Direct3D and OpenGL low-level access to hardware for audio, keyboard, mouse, joystick, and graphics.
- Allegro: It is a popular multimedia library for C/C++ programming that provides basic functionality for graphics, sound, and input devices.
- GTK+ (GIMP Toolkit): It is a popular open-source widget toolkit for creating graphical user interfaces (GUIs) that are often used for developing desktop applications.
- Cairo: This is a vector graphics library that provides support for a wide range of output devices including PNG, PDF, and SVG.
- SFML (Simple and Fast Multimedia Library): This is a multimedia library that provides a simple interface for creating 2D and 3D games and multimedia applications.
- GLUT (OpenGL Utility Toolkit): It is a utility library for OpenGL that provides a simple API for creating windows, handling input, and drawing 3D graphics.
- Qt: It is a GUI toolkit that provides a comprehensive set of tools for creating desktop and mobile applications.
Colors of Graphics Program in C
Here, are some of the colors of the graphics program in C
Syntax of Graphics Program in C
Explanation of Syntax This function initializes the graphics driver and sets the graphics mode. The first parameter (gd) is a pointer to the graphics driver, which is set to DETECT to detect the graphics driver automatically. The second parameter (gm) is the graphics mode, which specifies the resolution and color depth of the screen. The last parameter is a string that can be used to pass additional arguments to the graphics driver, but in this case, it’s left empty.
Example of Graphics Program in C
Note: Make sure to install the libraries before running the examples.
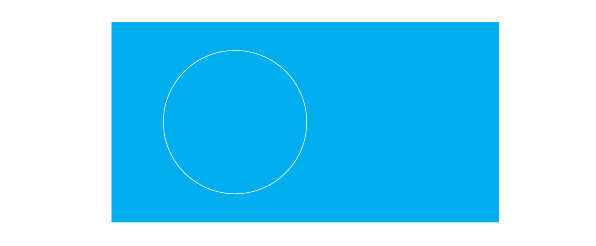
Applications of Graphics Program in C
Graphics programming in C has a wide range of applications. Here are some examples:
- Game development: Graphics program is widely used in game development. C-based graphics libraries such as SDL and Allegro can be used to create 2D and 3D games.
- Computer-aided design (CAD): It is used extensively in CAD software to create and manipulate 2D and 3D designs.
- Visualisation and data analysis: It is used to create visualizations of data in fields such as science, engineering, and finance. This might require producing charts, graphs, and other kinds of visualizations.
- Multimedia applications: It is used in multimedia applications such as video and audio players, image viewers, and video editors.
- User interfaces: It is used to create graphical user interfaces (GUIs) for software applications. This can include creating buttons, menus, and other interface elements.
- Animation and special effects: It is used to create animation and special effects in movies, TV shows, and other media.
Advantages of Graphics Program in C
- Efficient memory management
- Cross-platform compatibility
- Visually appealing applications
- Availability of graphics libraries
- Low-level control for advanced graphics.
Disadvantages of Graphics Program in C
- Performance optimization.
- Limited GUI capabilities.
- Lack of built-in features.
- Steep learning curve.
- Platform-specific issues.
Conclusion In conclusion, graphics programming in C empowers developers to breathe life into their applications through visual elements. From basic shapes to intricate designs, understanding the principles of graphics programming provides the tools to create engaging and dynamic user experiences. As technology advances, the role of graphics programming remains pivotal in shaping the visual aspects of software, making it a valuable skill for C programmers.
Frequently Asked Questions(FAQs) related to Graphics Program in C
Below are some of the FAQs related to the Graphics Program in C:
Q1: What is graphics programming in C? A1: Graphics programming in C involves creating visual elements, images, and animations using the C programming language. It often includes utilizing graphics libraries, such as OpenGL or graphics.h in the case of simple graphics programming in C.
Q2: Which libraries are commonly used for graphics programming in C? A2: Commonly used graphics libraries for C programming include graphics.h (a library for simple graphics operations), OpenGL (a cross-platform graphics API), and SDL (Simple DirectMedia Layer) for multimedia applications.
Q3: How can one draw basic shapes in a C graphics program? A3: Drawing basic shapes in a C graphics program involves using functions provided by the graphics library. For example, in graphics.h, functions like line(), circle(), and rectangle() can be used to draw lines, circles, and rectangles, respectively.
Q4: What are some applications of graphics programming in C? A4: Graphics programming in C finds applications in various domains, including game development, computer-aided design (CAD), simulation software, data visualization, and graphical user interface (GUI) development.
Q5: Is graphics programming in C limited to 2D graphics? A5: While graphics programming in C can be used for 2D graphics, it is not limited to it. With advanced graphics libraries like OpenGL, developers can create sophisticated 3D graphics and animations in C. The capabilities depend on the chosen graphics library and the developer’s expertise.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, assignment operator in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c.
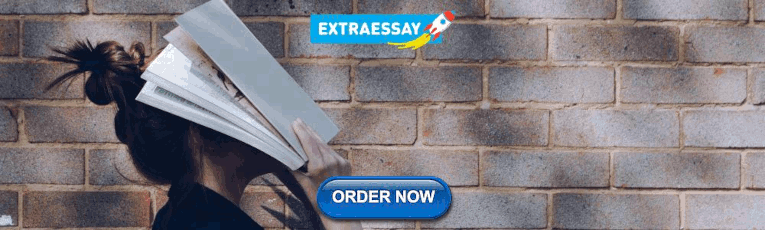
IMAGES
VIDEO
COMMENTS
Directed Graph Implementation. Following is the C implementation of a directed graph using an adjacency list: As evident from the above code, in a directed graph, we only create an edge from src to dest in the adjacency list. Now, if the graph is undirected, we also need to create an edge from dest to src in the adjacency list, as shown below: 2.
The following is the list of C/C++ programs based on the level of difficulty: Unmute. Easy. Depth First Search or DFS for a Graph. Breadth First Search or BFS for a Graph. Find Whether there is Path Between two Cells in Matrix. Shortest Path in a Binary Maze. Print All Paths from a Given Source to a Destination.
graph.c. And here is some test code: test_graph.c. 4.3. Implicit representations. For some graphs, it may not make sense to represent them explicitly. An example might be the word-search graph from CS223/2005/Assignments/HW10, which consists of all words in a dictionary with an edge between any two words that differ only by one letter.
A graph data structure is a collection of nodes that have data and are connected to other nodes. Let's try to understand this through an example. On facebook, everything is a node. That includes User, Photo, Album, Event, Group, Page, Comment, Story, Video, Link, Note...anything that has data is a node. Every relationship is an edge from one ...
Linked Representation. Applications of Graph. In this tutorial, you will learn an important data structure, Graph. You will also discover basic terminology, types of graphs, how graphs are represented in memory and their applications. A graph is a non-linear data structure consisting of vertices and edges that connect these vertices.
Representation of Graphs. There are two ways of representing a graph: Adjacency-list representation. Adjacency-matrix representation. According to their names, we use lists in the case of adjacency-list representation and a matrix (2D array) in the case of adjacency matrix representation.
A Graph is a non-linear data structure consisting of vertices and edges. The vertices are sometimes also referred to as nodes and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph is composed of a set of vertices ( V ) and a set of edges ( E ). The graph is denoted by G (V, E).
An adjacency list represents a graph as an array of linked lists. The index of the array represents a vertex and each element in its linked list represents the other vertices that form an edge with the vertex. For example, we have a graph below. We can represent this graph in the form of a linked list on a computer as shown below. Here, 0, 1, 2 ...
Some situations, or algorithms that we want to run with graphs as input, call for one representation, and others call for a different representation. Here, we'll see three ways to represent graphs. We'll look at three criteria. One is how much memory, or space, we need in each representation. We'll use asymptotic notation for that.
What is Graphics Program in C. Graphics program in C involves libraries such as OpenGL, GDI, or Allegro to create 2D and 3D graphics, multimedia applications, and games. A basic understanding of programming concepts is required, along with a development environment such as Code::Blocks or Visual Studio. Applications of graphics programming in C ...