
THE PATH TO SUCCESS IN EXAM...
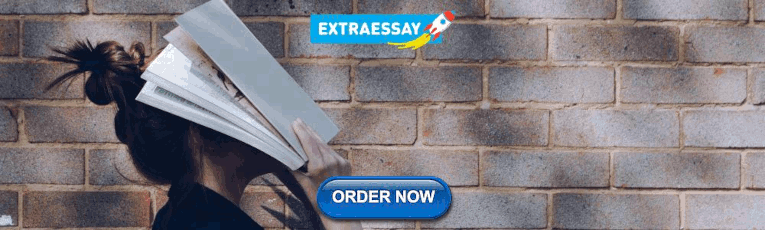
Introduction to Problem Solving – Notes
Introduction to problem solving.
- Steps for problem solving ( analysing the problem, developing an algorithm, coding, testing and debugging).
- flow chart and
- pseudo code,
Decomposition
Introduction
Computers is machine that not only use to develop the software. It is also used for solving various day-to-day problems.
Computers cannot solve a problem by themselves. It solve the problem on basic of the step-by-step instructions given by us.
Thus, the success of a computer in solving a problem depends on how correctly and precisely we –
- Identifying (define) the problem
- Designing & developing an algorithm and
- Implementing the algorithm (solution) do develop a program using any programming language.
Thus problem solving is an essential skill that a computer science student should know.
Steps for Problem Solving-
1. Analysing the problem
Analysing the problems means understand a problem clearly before we begin to find the solution for it. Analysing a problem helps to figure out what are the inputs that our program should accept and the outputs that it should produce.
2. Developing an Algorithm
It is essential to device a solution before writing a program code for a given problem. The solution is represented in natural language and is called an algorithm.
Algorithm: A set of exact steps which when followed, solve the problem or accomplish the required task.
Coding is the process of converting the algorithm into the program which can be understood by the computer to generate the desired solution.
You can use any high level programming languages for writing a program.
4. Testing and Debugging
The program created should be tested on various parameters.
- The program should meet the requirements of the user.
- It must respond within the expected time.
- It should generate correct output for all possible inputs.
- In the presence of syntactical errors, no output will be obtained.
- In case the output generated is incorrect, then the program should be checked for logical errors, if any.
Software Testing methods are
- unit or component testing,
- integration testing,
- system testing, and
- acceptance testing
Debugging – The errors or defects found in the testing phases are debugged or rectified and the program is again tested. This continues till all the errors are removed from the program.
Algorithm is a set of sequence which followed to solve a problem.
Algorithm for an activity ‘riding a bicycle’: 1) remove the bicycle from the stand, 2) sit on the seat of the bicycle, 3) start peddling, 4) use breaks whenever needed and 5) stop on reaching the destination.
Algorithm for Computing GCD of two numbers:
Step 1: Find the numbers (divisors) which can divide the given numbers.
Step 2: Then find the largest common number from these two lists.
A finite sequence of steps required to get the desired output is called an algorithm. Algorithm has a definite beginning and a definite end, and consists of a finite number of steps.
Characteristics of a good algorithm
- Precision — the steps are precisely stated or defined.
- Uniqueness — results of each step are uniquely defined and only depend on the input and the result of the preceding steps.
- Finiteness — the algorithm always stops after a finite number of steps.
- Input — the algorithm receives some input.
- Output — the algorithm produces some output.
While writing an algorithm, it is required to clearly identify the following:
- The input to be taken from the user.
- Processing or computation to be performed to get the desired result.
- The output desired by the user.
Representation of Algorithms
There are two common methods of representing an algorithm —
Flowchart — Visual Representation of Algorithms
A flowchart is a visual representation of an algorithm. A flowchart is a diagram made up of boxes, diamonds and other shapes, connected by arrows. Each shape represents a step of the solution process and the arrow represents the order or link among the steps. There are standardised symbols to draw flowcharts.
Start/End – Also called “Terminator” symbol. It indicates where the flow starts and ends.
Process – Also called “Action Symbol,” it represents a process, action, or a single step. Decision – A decision or branching point, usually a yes/no or true/ false question is asked, and based on the answer, the path gets split into two branches.
Input / Output – Also called data symbol, this parallelogram shape is used to input or output data.
Arrow – Connector to show order of flow between shapes.
Question: Write an algorithm to find the square of a number. Algorithm to find square of a number. Step 1: Input a number and store it to num Step 2: Compute num * num and store it in square Step 3: Print square
The algorithm to find square of a number can be represented pictorially using flowchart

A pseudocode (pronounced Soo-doh-kohd) is another way of representing an algorithm. It is considered as a non-formal language that helps programmers to write algorithm. It is a detailed description of instructions that a computer must follow in a particular order.
- It is intended for human reading and cannot be executed directly by the computer.
- No specific standard for writing a pseudocode exists.
- The word “pseudo” means “not real,” so “pseudocode” means “not real code”.
Keywords are used in pseudocode:
Question : Write an algorithm to calculate area and perimeter of a rectangle, using both pseudocode and flowchart.
Pseudocode for calculating area and perimeter of a rectangle.
INPUT length INPUT breadth COMPUTE Area = length * breadth PRINT Area COMPUTE Perim = 2 * (length + breadth) PRINT Perim The flowchart for this algorithm

Benefits of Pseudocode
- A pseudocode of a program helps in representing the basic functionality of the intended program.
- By writing the code first in a human readable language, the programmer safeguards against leaving out any important step.
- For non-programmers, actual programs are difficult to read and understand, but pseudocode helps them to review the steps to confirm that the proposed implementation is going to achieve the desire output.
Flow of Control :
The flow of control depicts the flow of process as represented in the flow chart. The process can flow in
In a sequence steps of algorithms (i.e. statements) are executed one after the other.
In a selection, steps of algorithm is depend upon the conditions i.e. any one of the alternatives statement is selected based on the outcome of a condition.
Conditionals are used to check possibilities. The program checks one or more conditions and perform operations (sequence of actions) depending on true or false value of the condition.
Conditionals are written in the algorithm as follows: If is true then steps to be taken when the condition is true/fulfilled otherwise steps to be taken when the condition is false/not fulfilled
Question : Write an algorithm to check whether a number is odd or even. • Input: Any number • Process: Check whether the number is even or not • Output: Message “Even” or “Odd” Pseudocode of the algorithm can be written as follows: PRINT “Enter the Number” INPUT number IF number MOD 2 == 0 THEN PRINT “Number is Even” ELSE PRINT “Number is Odd”
The flowchart representation of the algorithm
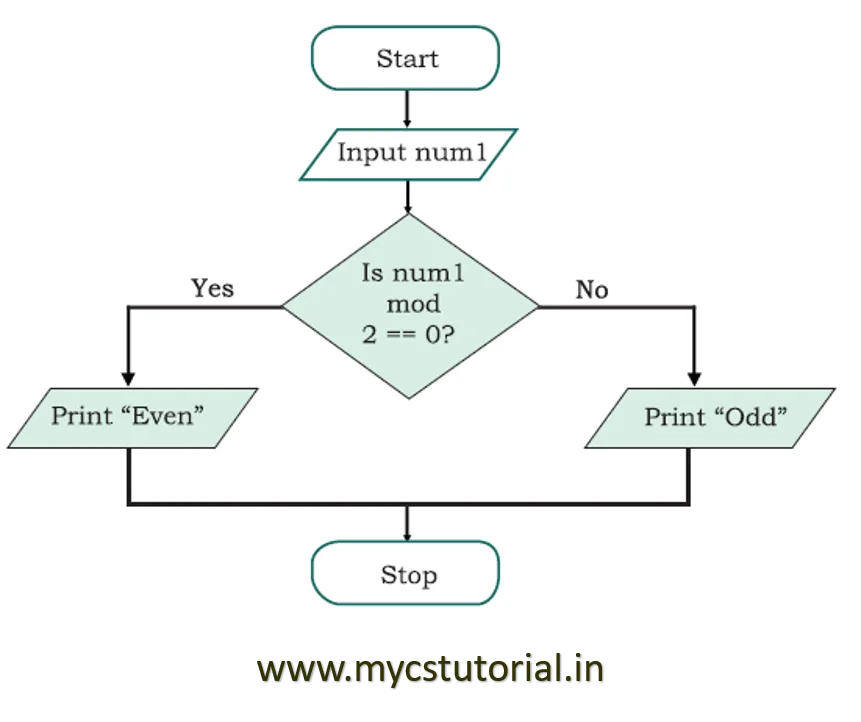
Repetitions are used, when we want to do something repeatedly, for a given number of times.
Question : Write pseudocode and draw flowchart to accept numbers till the user enters 0 and then find their average. Pseudocode is as follows:
Step 1: Set count = 0, sum = 0 Step 2: Input num Step 3: While num is not equal to 0, repeat Steps 4 to 6 Step 4: sum = sum + num Step 5: count = count + 1 Step 6: Input num Step 7: Compute average = sum/count Step 8: Print average The flowchart representation is
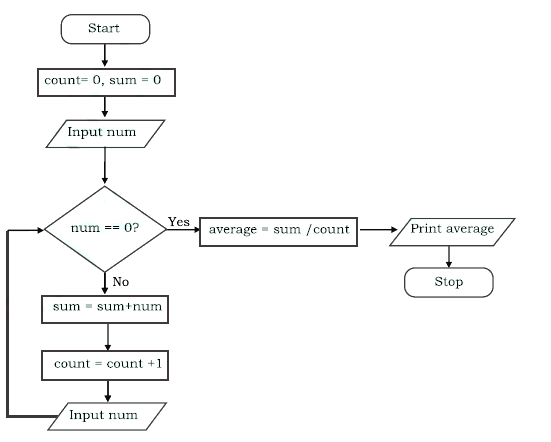
Once an algorithm is finalised, it should be coded in a high-level programming language as selected by the programmer. The ordered set of instructions are written in that programming language by following its syntax.
The syntax is the set of rules or grammar that governs the formulation of the statements in the language, such as spelling, order of words, punctuation, etc.
Source Code: A program written in a high-level language is called source code.
We need to translate the source code into machine language using a compiler or an interpreter so that it can be understood by the computer.
Decomposition is a process to ‘decompose’ or break down a complex problem into smaller subproblems. It is helpful when we have to solve any big or complex problem.
- Breaking down a complex problem into sub problems also means that each subproblem can be examined in detail.
- Each subproblem can be solved independently and by different persons (or teams).
- Having different teams working on different sub-problems can also be advantageous because specific sub-problems can be assigned to teams who are experts in solving such problems.
Once the individual sub-problems are solved, it is necessary to test them for their correctness and integrate them to get the complete solution.
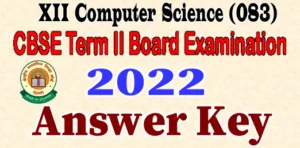
- Input Output in Python

Related Posts

Society, Law, and Ethics: Societal Impacts – Notes
Data structure: stacks – notes.

Python Revision Tour I : Basics of Python – Notes

Introduction to Python Module – Notes

Sorting Techniques in Python – Notes
Dictionary handling in python – notes, tuples manipulation in python notes, list manipulation – notes, leave a comment cancel reply.
You must be logged in to post a comment.
You cannot copy content of this page


Introduction to Problem Solving Class 11 Notes | CBSE Computer Science
Latest Problem Solving Class 11 Notes includes Problem Solving, steps, algorithm and its need, flow chart, pseudo code with lots of examples.
- 1 What is Problem Solving?
- 2 Steps for problem solving
- 3 What is Algorithm?
- 4 Why do we need Algorithm?
- 5.1 Flow chart
- 5.2 Flow Chart Examples
- 5.3 Pseudo code
- 5.4 Pseudo Code Example
- 6.1 Selection
- 6.2 Algorithm, Pseudocode, Flowchart with Selection ( Using if ) Examples
- 6.3 Repetition
- 6.4 Algorithm, Pseudocode, Flowchart with Repetition ( Loop ) Examples
- 7 Decomposition
What is Problem Solving?
Problem solving is the process of identifying a problem, analyze the problem, developing an algorithm for the identified problem and finally implementing the algorithm to develop program.
Steps for problem solving
There are 4 basic steps involved in problem solving
Analyze the problem
- Developing an algorithm
- Testing and debugging
Analyzing the problem is basically understanding a problem very clearly before finding its solution. Analyzing a problem involves
- List the principal components of the problem
- List the core functionality of the problem
- Figure out inputs to be accepted and output to be produced
Developing an Algorithm
- A set of precise and sequential steps written to solve a problem
- The algorithm can be written in natural language
- There can be more than one algorithm for a problem among which we can select the most suitable solution.
Algorithm written in natural language is not understood by computer and hence it has to be converted in machine language. And to do so program based on that algorithm is written using high level programming language for the computer to get the desired solution.
Testing and Debugging
After writing program it has to be tested on various parameters to ensure that program is producing correct output within expected time and meeting the user requirement.
There are many standard software testing methods used in IT industry such as
- Component testing
- Integration testing
- System testing
- Acceptance testing
What is Algorithm?
- A set of precise, finite and sequential set of steps written to solve a problem and get the desired output.
- Algorithm has definite beginning and definite end.
- It lead to desired result in finite amount of time of followed correctly.
Why do we need Algorithm?
- Algorithm helps programmer to visualize the instructions to be written clearly.
- Algorithm enhances the reliability, accuracy and efficiency of obtaining solution.
- Algorithm is the easiest way to describe problem without going into too much details.
- Algorithm lets programmer understand flow of problem concisely.
Characteristics of a good algorithm
- Precision — the steps are precisely stated or defined.
- Uniqueness — results of each step are uniquely defined and only depend on the input and the result of the preceding steps.
- Finiteness — the algorithm always stops after a finite number of steps.
- Input — the algorithm receives some input.
- Output — the algorithm produces some output.
What are the points that should be clearly identified while writing Algorithm?
- The input to be taken from the user
- Processing or computation to be performed to get the desired result
- The output desired by the user
Representation of Algorithm
An algorithm can be represented in two ways:
Pseudo code
- Flow chart is visual representation of an algorithm.
- It’s a diagram made up of boxes, diamonds and other shapes, connected by arrows.
- Each step represents a step of solution process.
- Arrows in the follow chart represents the flow and link among the steps.

Flow Chart Examples
Example 1: Write an algorithm to divide a number by another and display the quotient.
Input: Two Numbers to be divided Process: Divide number1 by number2 to get the quotient Output: Quotient of division
Step 1: Input a two numbers and store them in num1 and num2 Step 2: Compute num1/num2 and store its quotient in num3 Step 3: Print num3

- Pseudo code means ‘not real code’.
- A pseudo code is another way to represent an algorithm. It is an informal language used by programmer to write algorithms.
- It does not require strict syntax and technological support.
- It is a detailed description of what algorithm would do.
- It is intended for human reading and cannot be executed directly by computer.
- There is no specific standard for writing a pseudo code exists.
Keywords used in writing pseudo code
Pseudo Code Example
Example: write an algorithm to display the square of a given number.
Input, Process and Output Identification
Input: Number whose square is required Process: Multiply the number by itself to get its square Output: Square of the number
Step 1: Input a number and store it to num. Step 2: Compute num * num and store it in square. Step 3: Print square.
INPUT num COMPUTE square = num*num PRINT square

Example: Write an algorithm to calculate area and perimeter of a rectangle, using both pseudo code and flowchart.
INPUT L INPUT B COMPUTER Area = L * B PRINT Area COMPUTE Perimeter = 2 * ( L + B ) PRINT Perimeter

Flow of Control
An algorithm is considered as finite set of steps that are executed in a sequence. But sometimes the algorithm may require executing some steps conditionally or repeatedly. In such situations algorithm can be written using
Selection in algorithm refers to Conditionals which means performing operations (sequence of steps) depending on True or False value of given conditions. Conditionals are written in the algorithm as follows:
If <condition> then Steps to be taken when condition is true Otherwise Steps to be taken when condition is false
Algorithm, Pseudocode, Flowchart with Selection ( Using if ) Examples
Example: write an algorithm, pseudocode and flowchart to display larger between two numbers
INPUT: Two numbers to be compared PROCESS: compare two numbers and depending upon True and False value of comparison display result OUTPUT: display larger no
STEP1: read two numbers in num1, num2 STEP 2: if num1 > num2 then STEP 3: display num1 STEP 4: else STEP 5: display num2
INPUT num1 , num2 IF num1 > num2 THEN PRINT “num1 is largest” ELSE PRINT “num2 is largest” ENDIF

Example: write pseudocode and flowchart to display largest among three numbers
INPUT: Three numbers to be compared PROCESS: compare three numbers and depending upon True and False value of comparison display result OUTPUT: display largest number
INPUT num1, num2, num3 PRINT “Enter three numbers” IF num1 > num2 THEN IF num1 > num3 THEN PRINT “num1 is largest” ELSE PRINT “num3 is largest” END IF ELSE IF num2 > num3 THEN PRINT “num2 is largest” ELSE PRINT “num3 is largest” END IF END IF

- Repetition in algorithm refers to performing operations (Set of steps) repeatedly for a given number of times (till the given condition is true).
- Repetition is also known as Iteration or Loop
Repetitions are written in algorithm is as follows:
While <condition>, repeat step numbers Steps to be taken when condition is true End while
Algorithm, Pseudocode, Flowchart with Repetition ( Loop ) Examples
Example: write an algorithm, pseudocode and flow chart to display “Techtipnow” 10 times
Step1: Set count = 0 Step2: while count is less than 10, repeat step 3,4 Step 3: print “techtipnow” Step 4: count = count + 1 Step 5: End while
SET count = 0 WHILE count<10 PRINT “Techtipnow” Count = count + 1 END WHILE

Example: Write pseudocode and flow chart to calculate total of 10 numbers
Step 1: SET count = 0, total = 0 Step 2: WHILE count < 10, REPEAT steps 3 to 5 Step 3: INPUT a number in var Step 4: COMPUTE total = total + var Step 5: count = count + 1 Step 6: END WHILE Step 7: PRINT total
Example: Write pseudo code and flow chart to find factorial of a given number
Step 1: SET fact = 1 Step 2: INPUT a number in num Step 3: WHILE num >=1 REPEAT step 4, 5 Step 4: fact = fact * num Step 5: num = num – 1 Step 6: END WHILE Step 7: PRINT fact

Decomposition
- Decomposition means breaking down a complex problem into smaller sub problems to solve them conveniently and easily.
- Breaking down complex problem into sub problem also means analyzing each sub problem in detail.
- Decomposition also helps in reducing time and effort as different subprograms can be assigned to different experts in solving such problems.
- To get the complete solution, it is necessary to integrate the solution of all the sub problems once done.
Following image depicts the decomposition of a problem

2 thoughts on “Introduction to Problem Solving Class 11 Notes | CBSE Computer Science”
SO HELPFUL AND BEST NOTES ARE AVAILABLE TO GAIN KNOWLEDGE EASILY THANK YOU VERY VERY HEPFUL CONTENTS
THANK YOU SO MUCH FOR THE WONDERFUL NOTES
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
TutorialAICSIP
A best blog for CBSE Class IX to Class XII
Introduction to problem solving Computer Science Class 11 Notes
This article – introduction to problem solving Computer Science Class 11 offers comprehensive notes for Chapter 4 of the CBSE Computer Science Class 11 NCERT textbook.
Topics Covered
Introduction to problem solving Computer Science class 11
Computers, mobiles, the internet, etc. becomes our essentials nowadays for our routine life. We are using the to make our tasks easy and faster.
For example, earlier we were going to banks and standing in long queues for any type of transaction like money deposits or withdrawals. Today we can do these tasks from anywhere without visiting banks through internet banking and mobiles.
Basically, this was a complex problem and solved by a computer. The system was made online with the help of computers and the internet and made our task very easy.
This process is termed “Computerisations”. The problem is solved by using software to make a task easy and comfortable. Problem solving is a key term related to computer science.
The question comes to your mind how to solve a complex problem using computers? Let’s begin the article introduction to problem-solving Computer Science 11.
Introduction to problem solving Computer Science Class 11 – Steps for problem solving
“Computer Science is a science of abstraction -creating the right model for a problem and devising the appropriate mechanizable techniques to solve it.”
Solving any complex problem starts with understanding the problem and identifying the problem.
Suppose you are going to school by your bicycle. While riding on it you hear some noise coming from it. So first you will try to find that from where the noise is coming. So if you couldn’t solve the problem, you need to get it repaired.
The bicycle mechanic identifies the problem like a source of noise, causes of noise etc. then understand them and repair it for you.
So there are multiple steps involved in problem-solving. If the problem is simple and easy, we will find the solution easily. But the complex problem needs a few methods or steps to solve.
So complex problem requires some tools, a system or software in order to provide the solution. So it is a step-by-step process. These steps are as follows:
Analysing the problem
Developing an algorithm, testing and debugging.
The first step in the introduction to problem solving Computer Science Class 11 is analyzing the problem.
When you need to find a solution for a problem, you need to understand the problem in detail. You should identify the reasons and causes of the problem as well as what to be solved.
So this step involves a detailed study of the problem and then you need to follow some principles and core functionality of the solution.
In this step input and output, elements should be produced.
The second step for introduction to problem solving Computer Science class 11 is developing an algorithm.
An algorithm is a step-by-step process of a solution to a complex problem. It is written in natural language. An algorithm consists of various steps and begins from start to end. In between input, process and output will be specified. More details we will cover in the next section.
In short, the algorithm provides all the steps required to solve a problem.
For example:
Finding the simple interest, you need to follow the given steps:
- Gather required information and data such as principle amount, rate of interest and duration.
- Apply the formula for computing simple interest i.e. si=prn/100
- Now store the answer in si
- Display the calculated simple interest
In the above example, I have started and completed a task in a finite number of steps. It is completed in 4 finite steps.
Why algorithm is needed?
The algorithm helps developers in many ways. So it is needed for them for the following reasons:
- It prepares a roadmap of the program to be written before writing code.
- It helps to clearly visualise the instructions to be given in the program.
- When the algorithm is developed, a programmer knows the number of steps required to follow for the particular task.
- Algorithm writing is the initial stage (first step) of programming.
- It makes program writing easy and simple.
- It also ensures the accuracy of data and program output.
- It increases the reliability and efficiency of the solution.
Characteristics of a good algorithm
The characteristics of a good algorithm are as follows:
- It starts and ends with a finite number of steps. Therefore the steps are precisely stated or defined.
- In the algorithm, the result of each step is defined uniquely and based on the given input and process.
- After completion of the task, the algorithm will end.
- The algorithm accepts input and produces the output.
While writing the algorithm the following things should be clearly identified:
- The input required for the task
- The computation formula or processing instructions
After writing the algorithm, it is required to represent it. Once the steps are finalised, it is required to be represented logically. This logical representation of the program clearly does the following:
- Clears the logic of the program
- The execution of the program
The algorithm is steps written in the form of text. So it is difficult to read sometimes. So if it is represented in pictorial form it would be better for analysis of the program.
The flowchart is used to represent the algorithm in visual form.
Flowchart – Visual representation of an algorithm
A flowchart is made of some symbols or shapes like rectangles, squares, and diamonds connected by arrows. Every shape represents each step of an algorithm. The arrow basically represents the order or link of the steps.
The symbols used in the flow chart are as follows:

Coding is an essential part of the introduction to problem solving ComputerScience11.
- It is pronounced as soo-doh-kohd
- It is one of the ways of representing algorithms in a systematic way
- The word pseudo means not real, therefore pseudocode means not real code
- It is non-formal language, that helps programmers to write code
- It is written in human understandable language
- It cannot be directly read by computers
- There is no specific standard or way of writing pseudocode is there
When an algorithm is prepared, the next step is writing code. This code will be written in a specific programming language. The code follows certain rules and regulations of the programing language and provides solutions.
When coding is done you need to maintain it with proper documentation as well. The best practices for coding procedures must be followed. Because this code can be reviewed a number of times for further development and upgradation.
Let’s understand this step with a simple example!!
When your mother prepares a cake at your home, she will give peace of cake to someone before serving it to check the taste of the cake, right!!! If anything is needed like sugar or softness or hardness should be improved she will decide and do the improvement.
Similarly after writing code testing and debugging are required to check the software whether is providing the solution in a good manner not.
Have look at this also: Computer Science Class XI
Share this:
- Click to share on WhatsApp (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on Facebook (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
By Sanjay Parmar
More on this, computer science class 11 sample paper 2023-24 comprehensive guide, split up syllabus computer science class 11 comprehensive guide, comprehensive notes types of software class 11, leave a reply cancel reply.
You must be logged in to post a comment.
- Class 6 Maths
- Class 6 Science
- Class 6 Social Science
- Class 6 English
- Class 7 Maths
- Class 7 Science
- Class 7 Social Science
- Class 7 English
- Class 8 Maths
- Class 8 Science
- Class 8 Social Science
- Class 8 English
- Class 9 Maths
- Class 9 Science
- Class 9 Social Science
- Class 9 English
- Class 10 Maths
- Class 10 Science
- Class 10 Social Science
- Class 10 English
- Class 11 Maths
- Class 11 Computer Science (Python)
- Class 11 English
- Class 12 Maths
- Class 12 English
- Class 12 Economics
- Class 12 Accountancy
- Class 12 Physics
- Class 12 Chemistry
- Class 12 Biology
- Class 12 Computer Science (Python)
- Class 12 Physical Education
- GST and Accounting Course
- Excel Course
- Tally Course
- Finance and CMA Data Course
- Payroll Course
Interesting
- Learn English
- Learn Excel
- Learn Tally
- Learn GST (Goods and Services Tax)
- Learn Accounting and Finance
- GST Tax Invoice Format
- Accounts Tax Practical
- Tally Ledger List
- GSTR 2A - JSON to Excel
Are you in school ? Do you love Teachoo?
We would love to talk to you! Please fill this form so that we can contact you
You are learning...
Chapter 4 Class 11 - Introduction to Problem Solving
Click on any of the links below to start learning from Teachoo ...
Do you want to learn how to solve problems using computers? Do you want to develop your logical thinking and programming skills ? Do you want to explore the fascinating world of algorithms and data structures ? If you answered yes to any of these questions, then this chapter is for you! 🙌
In this chapter, you will learn about the basic concepts and techniques of problem solving using computers. You will learn how to:
- Define a problem and its specifications 📝
- Analyze a problem and identify its inputs, outputs and processing steps 🔎
- Design an algorithm to solve a problem using various methods such as pseudocode, flowcharts and decision tables 📊
- Implement an algorithm using a programming language such as Python 🐍
- Test and debug your program to ensure its correctness and efficiency 🛠️
- Evaluate your solution and compare it with other possible solutions 💯
By the end of this chapter, you will be able to apply your problem solving skills to various domains such as mathematics, science, engineering, games, art and more. You will also be able to appreciate the beauty and elegance of algorithms and data structures, and how they can help you solve complex and challenging problems. 😍
This chapter is designed for students who have some basic knowledge of computers and programming, but want to improve their problem solving abilities. It is also suitable for anyone who is interested in learning more about computer science and its applications. 🚀
MCQ questions (1 mark each)
True or false questions (1 mark each), fill in the blanks questions (1 mark each), very short answer type questions (1 mark each), short answer type questions (2 marks each), long answer type questions (3 marks each).
What's in it?
Hi, it looks like you're using AdBlock :(
Please login to view more pages. it's free :), solve all your doubts with teachoo black.
- Introduction to Algorithms and Flowcharts
Problem Solving
Today, we use computers in every field for various purposes. But, we know that they cannot solve the problems all by themselves. Furthermore, we have to give step by step instructions to the computer for solving the problem. We can define problem-solving as a process of understanding the problem, finding solutions for the problem, and finally implementing the solution to it. We can design the solution before coding in the form of algorithms and flowcharts. Moreover, the identification of arithmetic and logical operations is very important in developing the program.
Therefore, we can say that a successful problem-solving process depends on the following factors:
- understanding the problem and defining it precisely.
- designing proper algorithms and flowcharts of the solution.
- implementing the algorithm successfully.
When the problems are easy we can easily search out a solution. Whereas, complex problems require step by step process to solve. Hence, this means that we have to apply problem-solving techniques to solve the problem. Furthermore, this starts with finding a precise definition of the problem and ends with a successful solution. Moreover, the identification of arithmetic and logical operations plays a vital role while designing the algorithm. Here, we will study the algorithms and flowcharts.
Designing the solution
After understanding the relationship between input and output and the functionalities required we have to design an algorithm or flowchart. Furthermore, the algorithm should contain all the necessary functions to solve the problem. Moreover, it should produce a proper output for every input.
Hence, we can say that before writing the exact code for the problem it is necessary to define a solution. We can do this by starting with an initial plan and improvising it till it gives perfect results. Moreover, there can be more than one possible solution for a problem. Therefore, it is the responsibility of the programmer to choose the best solution.
While designing a problem we can represent it in algorithms and flowcharts. Hence, before writing the program code we can design the solution either in the form of an algorithm or a flowchart.
Browse more Topics Under Problem Solving Methodologies
- Understanding of the Problem
- Solution for the Problem
- Breaking Down Solution into Simple Steps
- Identification of Arithmetic and Logical Operations
- Control Structure
Introduction to Algorithms
An algorithm is basically a procedure of steps that we exactly follow to solve a particular task or problem. We can say that it is a set of rules that we need to follow while developing a program code during problem-solving. Furthermore, if we write an algorithm before actually writing a code, it becomes easy to perform the coding part. Moreover, the algorithm is in simple English language hence, others can also easily understand it and develop the code.
Features of an algorithm
The features of an algorithm are as follows:
The algorithm should be very clear and unambiguous in its meaning. It should be simple so that one can understand it easily.
- Well-defined inputs
A program may require to take input from the user. Therefore, the algorithm should clearly define the inputs.
- Well-defined outputs
The algorithm should clearly specify the output that the program will produce.
The algorithm should have a termination point. This means that the algorithm should not be such that it runs infinite times or end up in loops.
- Feasibility
The algorithm should be such that we can implement it easily. Hence, it should be simple and practical to implement.
- Language independent
It should be in simple English language. Since the code implementation should result in the same output no matter which programming language we use while writing the code.
Introduction to Flowcharts
It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program. Moreover, the programmers use it to depicting the flow of data and instructions while problem-solving. F lowcharting is the process of drawing a flowchart for an algorithm.
Symbols in a flowchart
The flowchart uses various symbols in the representation. These basic symbols are as follows:
It represents the start, stop, or halt in a program’s flow. The flowchart always starts and ends with this symbol. Besides, we represent it using an oval shape.
Input/Output
We represent it using a parallelogram . It indicates any input from the user or output of the program.
We represent it using a rectangle . It indicates any arithmetic operation’s processing such as addition, subtraction, multiplication, division, etc.
We represent it using the diamond symbol. It represents any type of decision in a program that results in true or false. For example. if-conditions.
We represent it using a circle . Whenever a flowchart is too large we can use connectors to avoid confusion.
These are basically arrows that represent the flow of the program.
Frequently Asked Questions (FAQs)
Q1. What are the main steps in problem-solving?
A1. A successful problem-solving process depends on the following factors:
- understanding the problem.
- designing proper algorithms and flowcharts.
- implementing the algorithm.
Q2. What is problem-solving?
A2. We can define problem-solving as a process of understanding the problem, finding solution for the problem, and finally implementing the solution to it.
Q3. What is an algorithm?
A3. An algorithm is basically a procedure of steps that we exactly follow to solve a particular task or problem.
Q4. What is a flowchart?
A4. It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program.
Q5. Name the features of an algorithm.
A5. The features of an algorithm are as follows:
Customize your course in 30 seconds
Which class are you in.

Problem Solving Methodologies
- Control Structures
- Identification of Arithmetic and Logical Operations Required for Solution
- Understanding the Problem
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App


- CBSE Revision Notes
- CBSE Free Video Lectures
- CBSE Important Questions
- CBSE Objective (MCQs)
- Assertation & Reasoning Questions
- CBSE Syllabus
- CBSE Sample paper
- CBSE Extra Sampler Paper
- Question Bank
- Project, Assignments & Activities
- ICSE Free Video Lectures
- Class 6 ICSE Revision Notes
- CLASS 7 ICSE REVISION NOTES
- CLASS 8 ICSE REVISION NOTES
- CLASS 9 ICSE REVISION NOTES
- CLASS 10 ICSE REVISION NOTES
- Class 6 ICSE Solutions
- CLASS 7 ICSE Solutions
- CLASS 8 ICSE Solutions
- CLASS 9 ICSE Solutions
- CLASS 10 ICSE Solutions
- Class 6 ICSE Important Questions
- CLASS 7 ICSE Important Question
- CLASS 8 ICSE IMPORTANT QUESTIONS
- CLASS 9 ICSE Important Questions
- CLASS 10 ICSE Important Question
- Class 6 ICSE Mcqs Question
- Class 7 ICSE Mcqs Question
- CLASS 8 ICSE MCQS QUESTION
- CLASS 9 ICSE Mcqs Questions
- CLASS 10 ICSE Mcqs Question
- ICSE Syllabus
- Class 6th Quick Revision Notes
- Class 7th Quick Revision Notes
- Class 8th Quick Revision Notes
- Class 9th Quick Revision Notes
- Class 10th Quick Revision Notes
- Class 11th Quick revision Notes
- Class 12th Quick Revision Notes
- Class 6th NCERT Solution
- Class 7th NCERT Solution
- Class 8th – NCERT Solution
- Class 9th NCERT Solution
- Class 10th NCERT Solution
- Class 11th NCERT Solution
- Class 12th NCERT Solution
- Class 6th Most Important Questions
- Class 7th Important Questions
- Class 8th Important Questions
- Class 9th Most Important Questions
- Class 10th Most Important Questions
- Class 11th important questions
- Class 12th important questions
- Class 6th MCQs
- Class 7th MCQs
- Class 8th MCQs
- Class 9th MCQs
- Class 10th MCQs
- Class 11th MCQs questions
- Class 12th Important MCQs
- NCERT Sample Paper
- NCERT Books in Pdf
- NCERT Chapter Mind Maps
- OliveTree Books Study Materials
- Forever With Books Study Material
- Rs Aggrawal Solutions
- RD Sharma Solution
- HC Verma Solution
- Lakhmir Singh Solution
- T.R Jain & V.K ohri Solution
- DK Goel Solutions
- TS Grewal Solution
- Our Products
- Online Tuition Services
- Career Advisor Booking
- Skill Development Courses
- Previous Year Board Exam Papers
- Free Video Lectures
Chapter 4 : Introduction to Problem Solving | class 11th | revision notes computer science
- Chapter 4 : Introduction to…
Introduction to Problem Solving
- Steps for problem solving ( analysing the problem, developing an algorithm, coding, testing and debugging).
- flow chart and
- pseudo code,
Decomposition
Introduction
Computers is machine that not only use to develop the software. It is also used for solving various day-to-day problems.
Computers cannot solve a problem by themselves. It solve the problem on basic of the step-by-step instructions given by us.
Thus, the success of a computer in solving a problem depends on how correctly and precisely we –
- Identifying (define) the problem
- Designing & developing an algorithm and
- Implementing the algorithm (solution) do develop a program using any programming language.
Thus problem solving is an essential skill that a computer science student should know.
Steps for Problem Solving-
1. Analysing the problem
Analysing the problems means understand a problem clearly before we begin to find the solution for it. Analysing a problem helps to figure out what are the inputs that our program should accept and the outputs that it should produce.
2. Developing an Algorithm
It is essential to device a solution before writing a program code for a given problem. The solution is represented in natural language and is called an algorithm.
Algorithm: A set of exact steps which when followed, solve the problem or accomplish the required task.
Coding is the process of converting the algorithm into the program which can be understood by the computer to generate the desired solution.
You can use any high level programming languages for writing a program.
4. Testing and Debugging
The program created should be tested on various parameters.
- The program should meet the requirements of the user.
- It must respond within the expected time.
- It should generate correct output for all possible inputs.
- In the presence of syntactical errors, no output will be obtained.
- In case the output generated is incorrect, then the program should be checked for logical errors, if any.
Software Testing methods are
- unit or component testing,
- integration testing,
- system testing, and
- acceptance testing
Debugging – The errors or defects found in the testing phases are debugged or rectified and the program is again tested. This continues till all the errors are removed from the program.
Algorithm is a set of sequence which followed to solve a problem.
Algorithm for an activity ‘riding a bicycle’: 1) remove the bicycle from the stand, 2) sit on the seat of the bicycle, 3) start peddling, 4) use breaks whenever needed and 5) stop on reaching the destination.
Algorithm for Computing GCD of two numbers:
Step 1: Find the numbers (divisors) which can divide the given numbers.
Step 2: Then find the largest common number from these two lists.
A finite sequence of steps required to get the desired output is called an algorithm. Algorithm has a definite beginning and a definite end, and consists of a finite number of steps.
Characteristics of a good algorithm
- Precision — the steps are precisely stated or defined.
- Uniqueness — results of each step are uniquely defined and only depend on the input and the result of the preceding steps.
- Finiteness — the algorithm always stops after a finite number of steps.
- Input — the algorithm receives some input.
- Output — the algorithm produces some output.
While writing an algorithm, it is required to clearly identify the following:
- The input to be taken from the user.
- Processing or computation to be performed to get the desired result.
- The output desired by the user.
Representation of Algorithms
There are two common methods of representing an algorithm —
Flowchart — Visual Representation of Algorithms
A flowchart is a visual representation of an algorithm. A flowchart is a diagram made up of boxes, diamonds and other shapes, connected by arrows. Each shape represents a step of the solution process and the arrow represents the order or link among the steps. There are standardised symbols to draw flowcharts.
Start/End – Also called “Terminator” symbol. It indicates where the flow starts and ends.
Process – Also called “Action Symbol,” it represents a process, action, or a single step. Decision – A decision or branching point, usually a yes/no or true/ false question is asked, and based on the answer, the path gets split into two branches.
Input / Output – Also called data symbol, this parallelogram shape is used to input or output data.
Arrow – Connector to show order of flow between shapes.
Question: Write an algorithm to find the square of a number. Algorithm to find square of a number. Step 1: Input a number and store it to num Step 2: Compute num * num and store it in square Step 3: Print square
The algorithm to find square of a number can be represented pictorially using flowchart

Table of Contents
A pseudocode (pronounced Soo-doh-kohd) is another way of representing an algorithm. It is considered as a non-formal language that helps programmers to write algorithm. It is a detailed description of instructions that a computer must follow in a particular order.
- It is intended for human reading and cannot be executed directly by the computer.
- No specific standard for writing a pseudocode exists.
- The word “pseudo” means “not real,” so “pseudocode” means “not real code”.
Keywords are used in pseudocode:
Question : Write an algorithm to calculate area and perimeter of a rectangle, using both pseudocode and flowchart.
Pseudocode for calculating area and perimeter of a rectangle.
INPUT length INPUT breadth COMPUTE Area = length * breadth PRINT Area COMPUTE Perim = 2 * (length + breadth) PRINT Perim The flowchart for this algorithm
Benefits of Pseudocode
- A pseudocode of a program helps in representing the basic functionality of the intended program.
- By writing the code first in a human readable language, the programmer safeguards against leaving out any important step.
- For non-programmers, actual programs are difficult to read and understand, but pseudocode helps them to review the steps to confirm that the proposed implementation is going to achieve the desire output.
Flow of Control :
The flow of control depicts the flow of process as represented in the flow chart. The process can flow in
In a sequence steps of algorithms (i.e. statements) are executed one after the other.
In a selection, steps of algorithm is depend upon the conditions i.e. any one of the alternatives statement is selected based on the outcome of a condition.
Conditionals are used to check possibilities. The program checks one or more conditions and perform operations (sequence of actions) depending on true or false value of the condition.
Conditionals are written in the algorithm as follows: If is true then steps to be taken when the condition is true/fulfilled otherwise steps to be taken when the condition is false/not fulfilled
Question : Write an algorithm to check whether a number is odd or even. • Input: Any number • Process: Check whether the number is even or not • Output: Message “Even” or “Odd” Pseudocode of the algorithm can be written as follows: PRINT “Enter the Number” INPUT number IF number MOD 2 == 0 THEN PRINT “Number is Even” ELSE PRINT “Number is Odd”
Repetitions are used, when we want to do something repeatedly, for a given number of times.
Question : Write pseudocode and draw flowchart to accept numbers till the user enters 0 and then find their average. Pseudocode is as follows:
Step 1: Set count = 0, sum = 0 Step 2: Input num Step 3: While num is not equal to 0, repeat Steps 4 to 6 Step 4: sum = sum + num Step 5: count = count + 1 Step 6: Input num Step 7: Compute average = sum/count Step 8: Print average The flowchart representation is
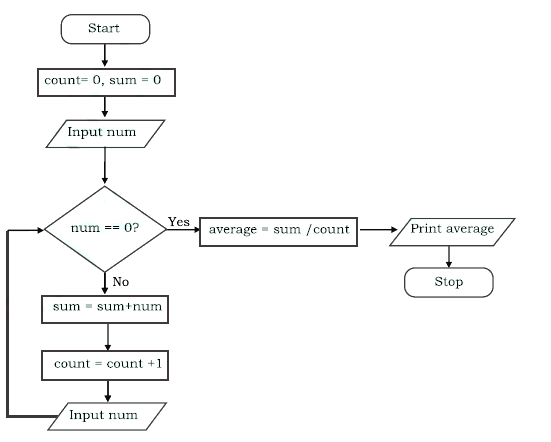
Once an algorithm is finalised, it should be coded in a high-level programming language as selected by the programmer. The ordered set of instructions are written in that programming language by following its syntax.
The syntax is the set of rules or grammar that governs the formulation of the statements in the language, such as spelling, order of words, punctuation, etc.
Source Code: A program written in a high-level language is called source code.
We need to translate the source code into machine language using a compiler or an interpreter so that it can be understood by the computer.
Decomposition is a process to ‘decompose’ or break down a complex problem into smaller subproblems. It is helpful when we have to solve any big or complex problem.
- Breaking down a complex problem into sub problems also means that each subproblem can be examined in detail.
- Each subproblem can be solved independently and by different persons (or teams).
- Having different teams working on different sub-problems can also be advantageous because specific sub-problems can be assigned to teams who are experts in solving such problems.
Once the individual sub-problems are solved, it is necessary to test them for their correctness and integrate them to get the complete solution.

Author: noor arora
Related posts.
Chapter 10 Wave Optics assertation & reasoning Questions assertation & reasoning Questions Class 12th Physics May 24, 2023
Chapter 5 Indian Sociologists | NCERT Important MCQs for Class 11th Sociology : Understanding Society January 17, 2023
Chapter 4 Introducing Western Sociologists | NCERT Important MCQs for Class 11th Sociology : Understanding Society January 17, 2023
Chapter 3 Environment and Society | NCERT Important MCQs for Class 11th Sociology : Understanding Society January 17, 2023
Chapter 2 Social Change and Social Order in Rural and Urban Society | NCERT Important MCQs for Class 11th Sociology : Understanding Society January 17, 2023
Chapter 1 Social Structure, Stratification and Social Processes in Society | NCERT Important MCQs for Class 11th Sociology : Understanding Society January 17, 2023
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Post comment
- Online Degree Explore Bachelor’s & Master’s degrees
- MasterTrack™ Earn credit towards a Master’s degree
- University Certificates Advance your career with graduate-level learning
- Top Courses
- Join for Free

Problem Solving Using Computational Thinking
Taught in English
Some content may not be translated
Financial aid available
85,094 already enrolled
Gain insight into a topic and learn the fundamentals

Instructor: Chris Quintana

Included with Coursera Plus
(1,224 reviews)
What you'll learn
Recognize Computational Thinking concepts in practice through a series of real-world case examples.
Develop solutions through the application of Computational Thinking concepts to real world problems.
Skills you'll gain
- Computer Programming
- Computational Thinking
Details to know

Add to your LinkedIn profile
See how employees at top companies are mastering in-demand skills
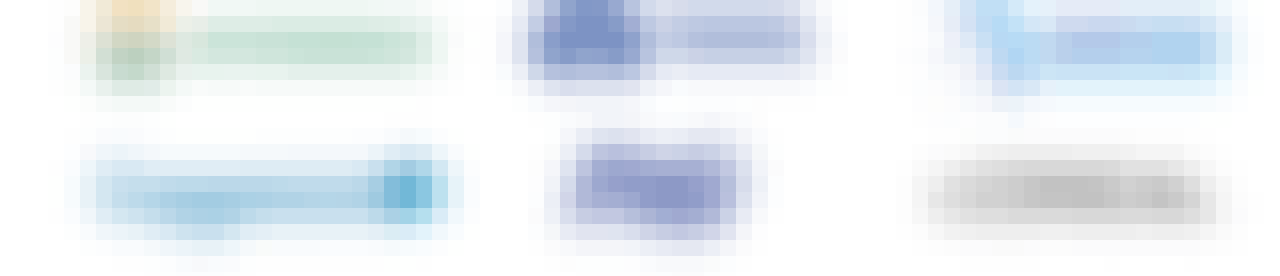
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
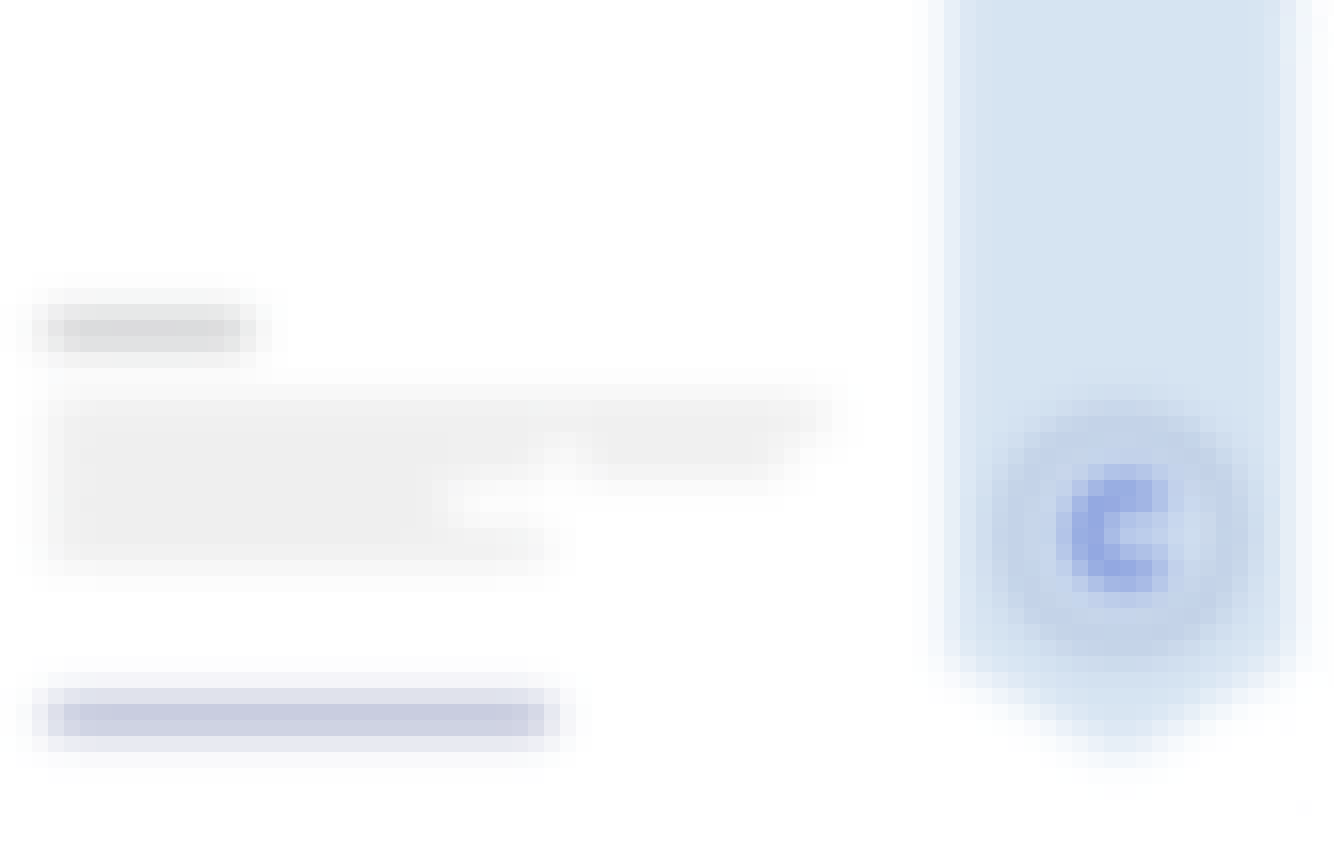
There are 5 modules in this course
Have you ever heard that computers "think"? Believe it or not, computers really do not think. Instead, they do exactly what we tell them to do. Programming is, "telling the computer what to do and how to do it."
Before you can think about programming a computer, you need to work out exactly what it is you want to tell the computer to do. Thinking through problems this way is Computational Thinking. Computational Thinking allows us to take complex problems, understand what the problem is, and develop solutions. We can present these solutions in a way that both computers and people can understand. The course includes an introduction to computational thinking and a broad definition of each concept, a series of real-world cases that illustrate how computational thinking can be used to solve complex problems, and a student project that asks you to apply what they are learning about Computational Thinking in a real-world situation. This project will be completed in stages (and milestones) and will also include a final disaster response plan you'll share with other learners like you. This course is designed for anyone who is just beginning programming, is thinking about programming or simply wants to understand a new way of thinking about problems critically. No prior programming is needed. The examples in this course may feel particularly relevant to a High School audience and were designed to be understandable by anyone. You will learn: -To define Computational Thinking components including abstraction, problem identification, decomposition, pattern recognition, algorithms, and evaluating solutions -To recognize Computational Thinking concepts in practice through a series of real-world case examples -To develop solutions through the application of Computational Thinking concepts to real world problems
Foundations of Computational Thinking
What's included.
3 videos 5 readings 2 quizzes 1 discussion prompt
3 videos • Total 43 minutes
- Welcome to Computational Thinking • 15 minutes • Preview module
- Example: Making a Cake • 16 minutes
- Introduction to the Graphic Organizer • 11 minutes
5 readings • Total 50 minutes
- Welcome and Syllabus • 10 minutes
- Help Us Learn More about You! • 10 minutes
- Contributor Acknowledgements • 10 minutes
- Introduction to the Graphic Organizer • 10 minutes
- Would you like to plan your learning journey with Michigan Online? • 10 minutes
2 quizzes • Total 35 minutes
- Foundations of Computational Thinking Quiz • 25 minutes
- Foundations of Computational Thinking Practice Questions • 10 minutes
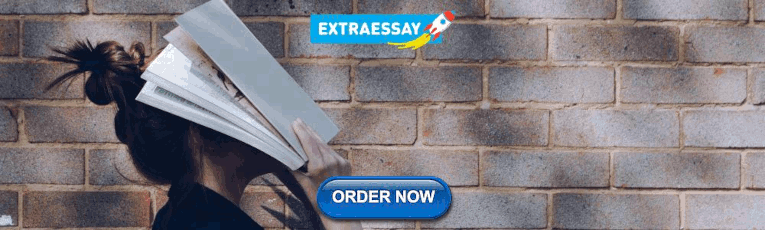
1 discussion prompt • Total 10 minutes
- Real-World Applications of Computational Thinking • 10 minutes
Case Study: Airport Surveillance and Image Analysis
6 videos 3 readings 3 quizzes 2 discussion prompts
6 videos • Total 29 minutes
- Image Analysis: Importance of Computational Thinking - Part 1 • 2 minutes • Preview module
- Image Analysis: Importance of Computational Thinking - Part 2 • 1 minute
- Image Analysis: Abstraction and Algorithms • 10 minutes
- Image Analysis: Algorithms, Optional Advanced Video • 9 minutes
- Image Analysis: Evaluating Solutions • 4 minutes
- Image Analysis: Problem Identification and Decomposition • 0 minutes
3 readings • Total 30 minutes
- Introduction to Airport Surveillance Case-Study • 10 minutes
- Airport Surveillance Case-Study Check-In 1 • 10 minutes
- Airport Surveillance Check-In 2 • 10 minutes
3 quizzes • Total 55 minutes
- Airport Surveillance Case-Study Quiz • 20 minutes
- Airport Surveillance Practice Questions Set 1 • 15 minutes
- Airport Surveillance Practice Questions Set 2 • 20 minutes
2 discussion prompts • Total 20 minutes
- Image Analysis: What Would You Do? • 10 minutes
- Other Applications • 10 minutes
Case Study: Epidemiology
6 videos 5 readings 2 quizzes 2 discussion prompts
6 videos • Total 52 minutes
- Epidemiology: Introduction and Problem Identification • 1 minute • Preview module
- Epidemiology: Problem Identification Part 2 • 8 minutes
- Epidemiology: Abstraction and Decomposition • 14 minutes
- Epidemiology: Algorithms and Evaluating Solutions - Part 1 • 13 minutes
- Epidemiology: Algorithms and Evaluating Solutions - Part 2 • 8 minutes
- Epidemiology: Conclusion • 6 minutes
- Introduction to Epidemiology Case-Study • 10 minutes
- Epidemiology Case-Study Check-In 1 • 10 minutes
- Up Next: Rafael's Algorithm • 10 minutes
- Epidemiology Case-Study Check-In 2 • 10 minutes
- Stay in touch on University of Michigan online courses • 10 minutes
2 quizzes • Total 36 minutes
- Epidemiology Case-Study Quiz • 20 minutes
- Epidemiology Practice Questions • 16 minutes
- Using Computational Thinking in Public Health • 10 minutes
- Understanding the Problem • 10 minutes
Case Study: Human Trafficking
3 videos 2 readings 2 quizzes
3 videos • Total 34 minutes
- Human Trafficking: Importance of Computational Thinking • 10 minutes • Preview module
- Human Trafficking: How Computational Thinking May Apply - Part 1 • 11 minutes
- Human Trafficking: How Computational Thinking May Apply - Part 2 • 12 minutes
2 readings • Total 20 minutes
- Introduction to Human Trafficking Case-Study • 10 minutes
- Human Trafficking Case-Study Check-In • 10 minutes
2 quizzes • Total 50 minutes
- Next Case: Potential Applications of Computational Thinking to Human Trafficking • 30 minutes
- Human Trafficking Practice Questions • 20 minutes
Final Project
8 readings 1 peer review
8 readings • Total 80 minutes
- Introduction to the Final Project • 10 minutes
- Final Project Part 1. Background and Context • 10 minutes
- Final Project Part 2: Graphic Organizer and Project Justification • 10 minutes
- Final Project Part 3: Project Justification • 10 minutes
- Final Project Part 4: Algorithm depiction • 10 minutes
- Course Feedback • 10 minutes
- Create innovative learning environments for students with Introduction to Learning Experience Design • 10 minutes
- Keep Learning with Michigan Online • 10 minutes
1 peer review • Total 60 minutes
- Final Project • 60 minutes
Instructor ratings
We asked all learners to give feedback on our instructors based on the quality of their teaching style.

The mission of the University of Michigan is to serve the people of Michigan and the world through preeminence in creating, communicating, preserving and applying knowledge, art, and academic values, and in developing leaders and citizens who will challenge the present and enrich the future.
Recommended if you're interested in Algorithms
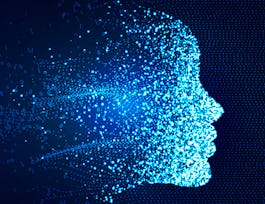
University of Pennsylvania
Computational Thinking for Problem Solving
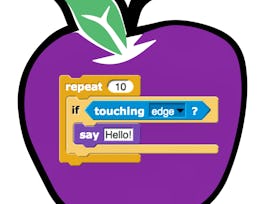
University of California San Diego
Computational Thinking for K-12 Educators: Abstraction, Methods, and Lists
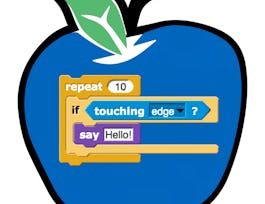
Computational Thinking for K-12 Educators: Nested If Statements and Compound Conditionals
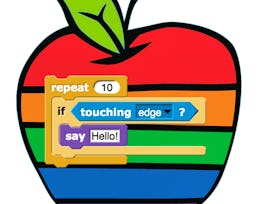
Computational Thinking for K-12 Educators Capstone
Why people choose coursera for their career.

Learner reviews
Showing 3 of 1224
1,224 reviews
Reviewed on May 28, 2021
The course helped me develop problem thinking skills and I appreciate the real life examples used in teaching the course. They made understanding the concepts much easier.
Reviewed on Jul 28, 2021
This course is what I really need to understand what is Computational Thinking. I learned about all aspect of it. To who want to begin your road to Computer Science, this course is my recommend
Reviewed on May 31, 2021
The course is highly enlightening. It has helped me see that a lot of problems can be solved using computational thinking. I will recommend to anyone willing to gain knowledge in this area.
New to Algorithms? Start here.

Open new doors with Coursera Plus
Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
When will i have access to the lectures and assignments.
Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. If you don't see the audit option:
The course may not offer an audit option. You can try a Free Trial instead, or apply for Financial Aid.
The course may offer 'Full Course, No Certificate' instead. This option lets you see all course materials, submit required assessments, and get a final grade. This also means that you will not be able to purchase a Certificate experience.
What will I get if I purchase the Certificate?
When you purchase a Certificate you get access to all course materials, including graded assignments. Upon completing the course, your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. If you only want to read and view the course content, you can audit the course for free.
What is the refund policy?
You will be eligible for a full refund until two weeks after your payment date, or (for courses that have just launched) until two weeks after the first session of the course begins, whichever is later. You cannot receive a refund once you’ve earned a Course Certificate, even if you complete the course within the two-week refund period. See our full refund policy Opens in a new tab .
Is financial aid available?
Yes. In select learning programs, you can apply for financial aid or a scholarship if you can’t afford the enrollment fee. If fin aid or scholarship is available for your learning program selection, you’ll find a link to apply on the description page.
More questions
- Computer Science and Engineering
- Introduction to Problem Solving and Programming (Video)
- Co-ordinated by : IIT Kanpur
- Available from : 2009-12-31
- Watch on YouTube
- Assignments
- Transcripts
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Computer Fundamental Tutorial
What is computer, introduction to computer fundamentals, history and evolution of computers, components of a computer system, computer hardware, computer software, data storage and memory.
- Computer Memory
Basics of Operating System
Computer networks and internet, introduction to programming, computer security and privacy, functionalities of computer, the evolution of computers, applications of computer fundamentals, faqs on computer fundamentals.
This Computer Fundamental Tutorial covers everything from basic to advanced concepts, including computer hardware, software, operating systems, peripherals, etc. Whether you’re a beginner or an experienced professional, this tutorial is designed to enhance your computer skills and take them to the next level.
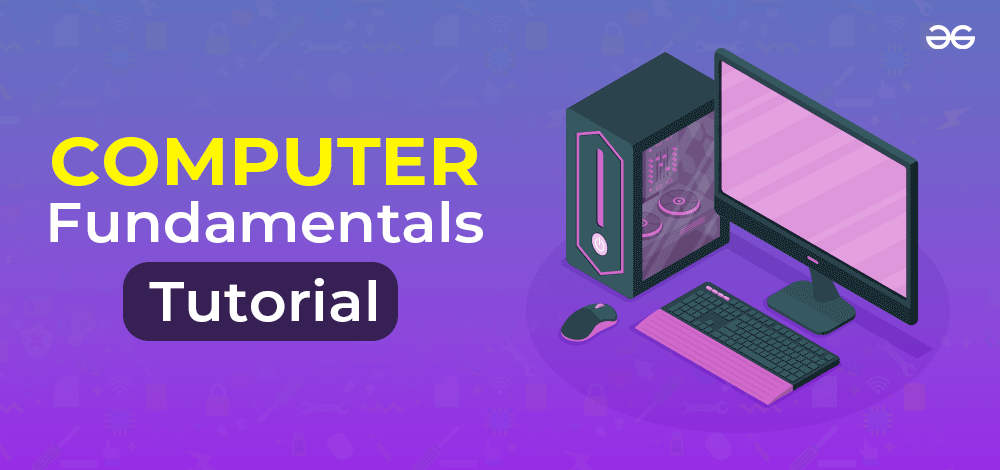
The computer is a super-intelligent electronic device that can perform tasks, process information, and store data. It takes the data as an input and processes that data to perform tasks under the control of a program and produces the output. A computer is like a personal assistant that follows instructions to get things done quickly and accurately. It has memory to store information temporarily so that the computer can quickly access it when needed.
Prerequisites: No prerequisites or prior knowledge required. This article on Computer Fundamentals is designed for absolute beginners.
Computer Fundamentals Index
- What are Computer Fundamentals?
- Importance of Computer Fundamentals in Digital Age
- Advantages and Disadvantages of Computer
- Classification of Computers
- Application area of Computer
- History of Computers
- The Origins of Computing
- Generations of Computer
- Central Processing Unit (CPU)
- Memory Units
- Input Devices
- Output Devices
- Motherboard
- Random Access Memory (RAM)
- Hard Disk Drives (HDD)
- Solid State Drives (SSD)
- Graphics Processing Unit (GPU)
- Power Supply Unit (PSU)
- Computer Peripherals (Keyboard, Mouse, Monitor, etc.)
- Introduction to Software
- Types of Software
- Application Software
- System Software
- What is a Storage Device?
- Types of Data Storage
- Optical Storage ( CDs , DVDs, Blu-rays )
- Flash Drives and Memory Cards
- Cloud Storage
- Register Memory
- Cache Memory
- Primary Memory
- Secondary Memory
- What is Operating System?
- Evolution of Operating System
- Types of Operating Systems
- Operating System Services
- Functions of Operating System
- Introduction to Computer Networks
- Types of Networks (LAN, WAN, MAN)
- Network Topologies (Star, Bus, Ring)
- Network Protocols (TCP/IP, HTTP, FTP)
- Network Devices (Hub, Repeater, Bridge, Switch, Router, Gateways and Brouter)
- World Wide Web
- What is Programming?
- A Categorical List of programming languages
- Language Processors: Assembler, Compiler and Interpreter
- Variables ( C , C++ , Java )
- Data Types ( C , C++ , Java )
- Operators ( C , C++ , Java )
- Control Structures (Conditionals, Loops)
- Functions and Procedures
- Importance of Computer Security
- Common Security Threats
- Malware (Viruses, Worms, Trojans)
- Network Security Measures (Firewalls, Encryption)
- Access Control
- User Authentication
- Privacy Concerns and Data Protection
Any digital computer performs the following five operations:
- Step 1 − Accepts data as input.
- Step 2 − Saves the data/instructions in its memory and utilizes them as and when required.
- Step 3 − Execute the data and convert it into useful information.
- Step 4 − Provides the output.
- Step 5 − Have control over all the above four steps
A journey through the history of computers. We’ll start with the origins of computing and explore the milestones that led to the development of electronic computers.
- Software Development: Computer fundamentals are fundamental to software development. Understanding programming languages, algorithms, data structures, and software design principles are crucial for developing applications, websites, and software systems. It forms the basis for creating efficient and functional software solutions.
- Network Administration : Computer fundamentals are essential for network administrators. They help set up and manage computer networks, configure routers and switches, troubleshoot network issues, and ensure reliable connectivity. Knowledge of computer fundamentals enables network administrators to maintain and optimize network performance.
- Cybersecurity : Computer fundamentals are at the core of cybersecurity. Understanding the basics of computer networks, operating systems, encryption techniques, and security protocols helps professionals protect systems from cyber threats. It enables them to identify vulnerabilities, implement security measures, and respond effectively to security incidents.
- Data Analysis : Computer fundamentals are necessary for data analysis and data science. Knowledge of programming, statistical analysis, and database management is essential to extract insights from large datasets. Understanding computer fundamentals helps in processing and analyzing data efficiently, enabling data-driven decision-making.
- Artificial Intelligence and Machine Learning : Computer fundamentals provide the foundation for AI and machine learning. Concepts such as algorithms, data structures, and statistical modelling are vital in training and developing intelligent systems. Understanding computer fundamentals allows professionals to create AI models, train them on large datasets, and apply machine learning techniques to solve complex problems.
Q.1 How long does it take to learn computer fundamentals?
The time required to learn computer fundamentals can vary depending on your prior knowledge and the depth of understanding you aim to achieve. With consistent effort and dedication, one can grasp the basics within a few weeks or months. However, mastering computer fundamentals is an ongoing process as technology evolves.
Q.2 Are computer fundamentals only for technical professionals?
No, computer fundamentals are not limited to technical professionals. They are beneficial for anyone who uses computers in their personal or professional life. Basic computer skills are increasingly essential in various careers and everyday tasks.
Q.3 Can I learn computer fundamentals without any prior technical knowledge?
Absolutely! Computer fundamentals are designed to be beginner-friendly. You can start learning without any prior technical knowledge. There are numerous online tutorials, courses, and resources available that cater to beginners.
Q.4 How can computer fundamentals improve my job prospects?
Computer skills are highly sought after in today’s job market. Proficiency in computer fundamentals can enhance your employability by opening up job opportunities in various industries. It demonstrates your adaptability, problem-solving abilities, and ability to work with digital tools.
Please Login to comment...
Similar reads.
- Computer Subject
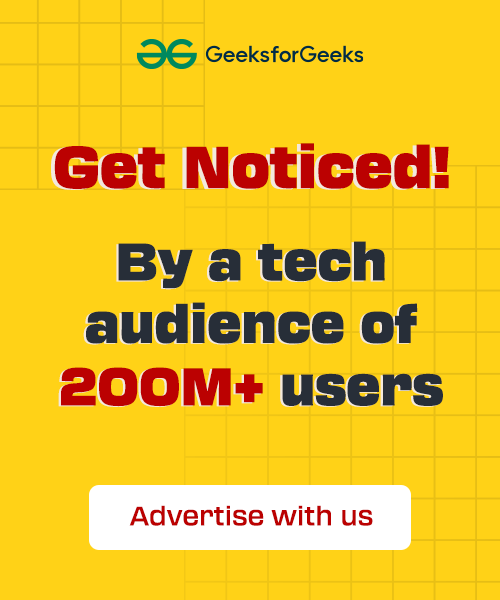
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Introduction to Problem Solving Class 11 Notes
Teachers and Examiners ( CBSESkillEduction ) collaborated to create the Introduction to Problem Solving Class 11 Notes . All the important Information are taken from the NCERT Textbook Computer Science (083) class 11 .
Introduction to Problem Solving
Problems cannot be resolved by computers alone. We must provide clear, step-by-step directions on how to solve the issue. Therefore, the effectiveness of a computer in solving a problem depends on how exactly and correctly we describe the problem, create an algorithm to solve it, and then use a programming language to implement the algorithm to create a programme. So, the process of identifying a problem, creating an algorithm to solve it, and then putting the method into practise to create a computer programme is known as problem solving.
Steps for Problem Solving
To identify the best solution to a difficult problem in a computer system, a Problem Solving methodical approach is necessary. To put it another way, we must use problem-solving strategies to solve the difficult problem in a computer system. Problem fixing starts with the accurate identification of the issue and concludes with a fully functional programme or software application. Program Solving Steps are – 1. Analysing the problem 2. Developing an Algorithm 3. Coding 4. Testing and Debugging
Analyzing the problem – It is important to clearly understand a problem before we begin to find the solution for it. If we are not clear as to what is to be solved, we may end up developing a program which may not solve our purpose.
Developing an Algorithm – Before creating the programme code to solve a particular problem, a solution must be thought out. Algorithm is a step by step process where we write the problem and the steps of the programs.
Coding – After the algorithm is completed, it must be translated into a form that the computer can understand in order to produce the desired outcome. A programme can be written in a number of high level programming languages.
Testing and Debugging – The developed programme needs to pass different parameter tests. The programme needs to fulfil the user’s requirements. It must answer in the anticipated amount of time. For all conceivable inputs, it must produce accurate output.
What is the purpose of Algorithm?
A programme is created by a programmer to tell the computer how to carry out specific activities. Then, the computer executes the instructions contained in the programme code. As a result, before creating any code, the programmer first creates a roadmap for the software. Without a roadmap, a programmer might not be able to visualise the instructions that need to be written clearly and might end up creating a software that might not function as intended. This roadmap is known as algorithm.
Why do we need an Algorithm?
The purpose of using an algorithm is to increase the reliability, accuracy and efficiency of obtaining solutions.
Characteristics of a good algorithm
• Precision — the steps are precisely stated or defined. • Uniqueness — results of each step are uniquely defined and only depend on the input and the result of the preceding steps. • Finiteness — the algorithm always stops after a finite number of steps. • Input — the algorithm receives some input. • Output — the algorithm produces some output.
While writing an algorithm, it is required to clearly identify the following:
• The input to be taken from the user • Processing or computation to be performed to get the desired result • The output desired by the user
Representation of Algorithms
There are two common methods of representing an algorithm —flowchart and pseudocode. Either of the methods can be used to represent an algorithm while keeping in mind the following: • it showcases the logic of the problem solution, excluding any implementational details • it clearly reveals the flow of control during execution of the program
Flowchart — Visual Representation of Algorithms
A flowchart is a visual representation of an algorithm. A flowchart is a diagram made up of boxes, diamonds and other shapes, connected by arrows. Each shape represents a step of the solution process and the arrow represents the order or link among the steps.
There are standardized symbols to draw flowcharts. Some are given below –

Flow Chart Syntax

How to draw flowchart
Q. Draw a flowchart to find the sum of two numbers?

Q. Draw a flowchart to print the number from 1 to 10?

Another way to represent an algorithm is with a pseudocode, which is pronounced Soo-doh-kohd. It is regarded as a non-formal language that aids in the creation of algorithms by programmers. It is a thorough explanation of the steps a computer must take in a specific order.
The word “pseudo” means “not real,” so “pseudocode” means “not real code”. Following are some of the frequently used keywords while writing pseudocode –
Write an algorithm to display the sum of two numbers entered by user, using both pseudocode and flowchart.
Pseudocode for the sum of two numbers will be – input num1 input num2 COMPUTE Result = num1 + num2 PRINT Result
Flowchart for this pseudocode or algorithm –
Flow of Control
The flow of control depicts the flow of events as represented in the flow chart. The events can flow in a sequence, or on branch based on a decision or even repeat some part for a finite number of times.
Sequence – These algorithms are referred to as executing in sequence when each step is carried out one after the other.
Selection – An algorithm may require a question at some point because it has come to a stage when one or more options are available. This type of problem we can solve using If Statement and Switch Statement in algorithm or in the program.
Repetition – We often use phrases like “go 50 steps then turn right” while giving directions. or “Walk to the next intersection and turn right.” These are the kind of statements we use, when we want something to be done repeatedly. This type of problem we can solve using For Statement, While and do-while statement.
Verifying Algorithms
Software is now used in even more important services, such as the medical industry and space missions. Such software must function properly in any circumstance. As a result, the software designer must ensure that every component’s operation is accurately defined, validated, and confirmed in every way.
To verify, we must use several input values and run the algorithm for each one to produce the desired result. We can then tweak or enhance the algorithm as necessary.
Comparison of Algorithm
There may be more than one method to use a computer to solve a problem, If you wish to compare two programmes that were created using two different approaches for resolving the same issue, they should both have been built using the same compiler and executed on the same machine under identical circumstances.
Once an algorithm is decided upon, it should be written in the high-level programming language of the programmer’s choice. By adhering to its grammar, the ordered collection of instructions is written in that programming language. The grammar or set of rules known as syntax controls how sentences are produced in a language, including word order, punctuation, and spelling.
Decomposition
A problem may occasionally be complex, meaning that its solution cannot always be found. In these circumstances, we must break it down into simpler components. Decomposing or breaking down a complicated problem into smaller subproblems is the fundamental concept behind addressing a complex problem by decomposition. These side issues are more straightforward to resolve than the main issue.
Computer Science Class 11 Notes
- Unit 1 : Basic Computer Organisation
- Unit 1 : Encoding Schemes and Number System
- Unit 2 : Introduction to problem solving
- Unit 2 : Getting Started with Python
- Unit 2 : Conditional statement and Iterative statements in Python
- Unit 2 : Function in Python
- Unit 2 : String in Python
- Unit 2 : Lists in Python
- Unit 2 : Tuples in Python
- Unit 2 : Dictionary in Python
- Unit 3 : Society, Law and Ethics
Computer Science Class 11 MCQ
Computer science class 11 ncert solutions.
- Unit 2 : Tuples and Dictionary in Python
Browse Course Material
Course info.
- Dr. Ana Bell
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
Learning Resource Types
Introduction to cs and programming using python.
Lecture 1: Introduction
Topics: Introduction to Python: knowledge, machines, objects, types, variables, bindings, IDEs
- Download video
- Download transcript
Lecture Notes
Lecture 1 Code
Ch 1, Ch 2.1–2.2
Finger Exercise Lecture 1
Assume 3 variables are already defined for you: a , b , and c . Create a variable called total that adds a and b then multiplies the result by c . Include a last line in your code to print the value: print(total)
6.100L Finger Exercises Lecture 1 Solutions
Problem Set 0
Problem Set 0 Code
Recitation 1
Recitations were smaller sections that reviewed the previous lectures.
Recitation 1 Notes

You are leaving MIT OpenCourseWare
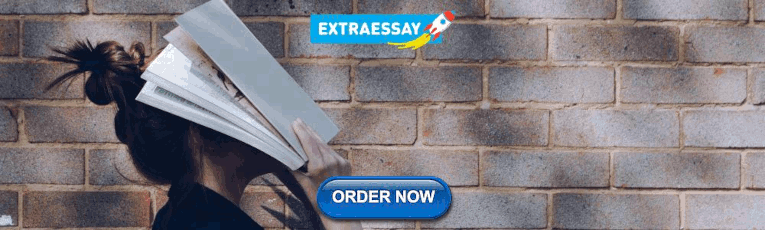
IMAGES
VIDEO
COMMENTS
Step 1: Find the numbers (divisors) which can divide the given numbers. Step 2: Then find the largest common number from these two lists. A finite sequence of steps required to get the desired output is called an algorithm. Algorithm has a definite beginning and a definite end, and consists of a finite number of steps.
Steps for problem solving. There are 4 basic steps involved in problem solving. Analyze the problem. Developing an algorithm. Coding. Testing and debugging. Analyze the problem. Analyzing the problem is basically understanding a problem very clearly before finding its solution. Analyzing a problem involves.
COMP1405/1005 - An Introduction to Computer Science and Problem Solving Fall 2011 - 6-1.2 Writing Programs in Processing It is now time to start writing simple programs to solve simple problems. As mentioned, we will be using the Processing language (available for free from www.Processing.org for your PC, MAC or Linux system).
So it is a step-by-step process. These steps are as follows: Analysing the problem. Developing an algorithm. Coding. Testing and debugging. The first step in the introduction to problem solving Computer Science Class 11 is analyzing the problem.
We can do this in four steps. 1. Identify all of the nouns in the sentence. Given the 3 dimensions of a box (length, width, and height), calculate the volume. The nouns in the problem specification identify descriptions of information that you will need to either identify or keep track of.
This section provides the lecture notes for each session of the course along with supporting code files. ... Introduction to Computers and Engineering Problem Solving. Menu. More Info Syllabus Instructor Insights Readings Lecture Notes ... Introduction to Swing (PDF) Lecture 17 code (ZIP) (This ZIP file contains: 1 .java file.) L 18
1. Problem solving is a skill (it can be learned). It is not an innate ability. 2. Problem solving is fundamentally about attitude and effort (the "problem-solving stance"). 3. The problem-solving stance isn't something that you can just "turn on" when you need it for a test, etc. You have to live it - and successful
You will learn how to: Define a problem and its specifications 📝. Analyze a problem and identify its inputs, outputs and processing steps 🔎. Design an algorithm to solve a problem using various methods such as pseudocode, flowcharts and decision tables 📊. Implement an algorithm using a programming language such as Python 🐍.
MIT OpenCourseWare is a web based publication of virtually all MIT course content. OCW is open and available to the world and is a permanent MIT activity
🚀This video Unlock the Power of Problem Solving! 💡 🔎I'll guide you through the essential Steps for Problem Solving. 💥 Learn Decomposition a break down co...
Computational thinking is a problem-solving process in which the last step is expressing the solution so that it can be executed on a computer. However, before we are able to write a program to implement an algorithm, we must understand what the computer is capable of doing -- in particular, how it executes instructions and how it uses data.
Introduction to Flowcharts. It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program. Moreover, the programmers use it to depicting the flow of data and instructions while problem-solving. Flowcharting is the process of drawing a ...
Introduction to Problem Solving Notes Topics: Introduction Computers is machine that not only use to develop the software. It is also used for solving various day-to-day problems. Computers cannot solve a problem by themselves. It solve the problem on basic of the step-by-step instructions given by us. Thus, the success of a computer in solving…
The audience for 1.00 is non-computer science majors. 1.00 does not focus on writing compilers or parsers or computing tools where the computer is the system; it focuses on engineering problems where the computer is part of the system, or is used to model a physical or logical system. 1.00 teaches the Java programming language, and it focuses ...
Computational Thinking allows us to take complex problems, understand what the problem is, and develop solutions. We can present these solutions in a way that both computers and people can understand. The course includes an introduction to computational thinking and a broad definition of each concept, a series of real-world cases that ...
Introduction to Problem Solving: Problem-solving strategies, Problem identification, Problem understanding, Algorithm development, Solution planning (flowcharts ... Problem solving - Lecture notes 1. Problem Solving and Programming. Lecture notes. 100% (9) Comments. ... Using computer's in problem solving. Software development method (SDM).
NPTEL :: Computer Science and Engineering - Introduction to Problem Solving and Programming. Courses. Computer Science and Engineering. Introduction to Problem Solving and Programming (Video) Syllabus. Co-ordinated by : IIT Kanpur. Available from : 2009-12-31.
Functionalities of Computer. Any digital computer performs the following five operations: Step 1 − Accepts data as input. Step 2 − Saves the data/instructions in its memory and utilizes them as and when required. Step 3 − Execute the data and convert it into useful information. Step 4 − Provides the output.
• To learn to write programs (using structured programming approach) in C to solve problems. UNIT ‐ I Introduction to Computing - Computer Systems-Hardware and Software, Computer Languages, Algorithm, Flowchart, Representation of Algorithm and Flowchart with examples.
CS2104: Introduction to Problem Solving in Computer Science. This course introduces the student to a broad range of heuristics for solving problems in a range of settings. Emphasis on problem-solving techniques that aid programmers and computer scientists.
Developing an Algorithm - Before creating the programme code to solve a particular problem, a solution must be thought out. Algorithm is a step by step process where we write the problem and the steps of the programs. Coding - After the algorithm is completed, it must be translated into a form that the computer can understand in order to produce the desired outcome.
All of the video and note materials for Lecture 1: Introduction. Browse Course Material ... Lecture Notes. Lecture 1: Introduction. Lecture 1 Code. Readings. Ch 1, Ch 2.1-2.2. Finger Exercise Lecture 1. ... Problem Set 0. Problem Set 0 Code. Recitation 1.
NCERT Class 11 Computer Science Chapter 4 | Class 11 Computer Science Notesclass 11 computer science one shot | class 11 computer science pythonHaan bhyii MA...
Programming for problem solving using C Notes ... Algorithm, Flowchart, Pseudo code. Introduction to C Programming: Introduction, Structure of a C Program, Comments, Keywords, Identifiers, Data Types, Variables, Constants, Input/Output Statements, Operators, Type Conversion INTRODUCTION TO PROGRAMMING 1.1 Introduction to Computer Software ...