- Machine Learning Decision Tree – Solved Problem (ID3 algorithm)
- Poisson Distribution | Probability and Stochastic Process
- Conditional Probability | Joint Probability
- Solved assignment problems in communicaion |online Request
- while Loop in C++
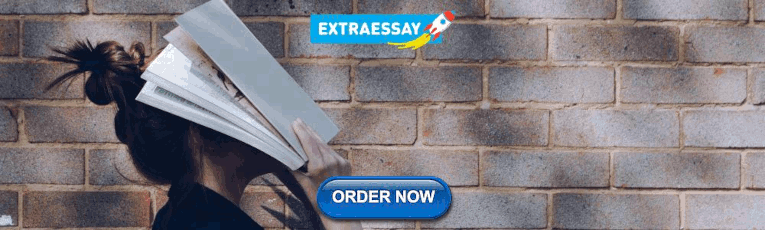
EngineersTutor
Solved assignment problems – algorithms and flowcharts.
An algorithm is defined as sequence of steps to solve a problem (task) . The steps must be finite, well defined and unambiguous. Writing algorithm requires some thinking. Algorithm can also be defined as a plan to solve a problem and represents its logic. Note that an algorithm is of no use if it does not help us arrive at the desired solution
Algorithm characteristics
- It should have finite number of steps . No one can be expected to execute infinite number of steps.
- The steps must be in order and simple
- Each step should be defined clearly i.e. without un-ambiguity (without doubtfulness)
- Must include all required information
- Should exhibit at least one output
A flowchart is a pictorial (graphical) representation of an algorithm . A flowchart is drawn using different kinds of symbols. A symbol is used for a specific purpose. Each symbol has name.
Different algorithms have different performance characteristics to solve the same problem. some algorithms are fast. some are slow. some occupy more memory space. some occupy less memory space. some are complex and some algorithms are simple..
Logically algorithm, flowchart and program are the same.
Q1 . Create a program to compute the volume of a sphere. Use the formula: V = (4/3) *pi*r 3 where pi is equal to 3.1416 approximately. The r is the radius of sphere. Display the result.
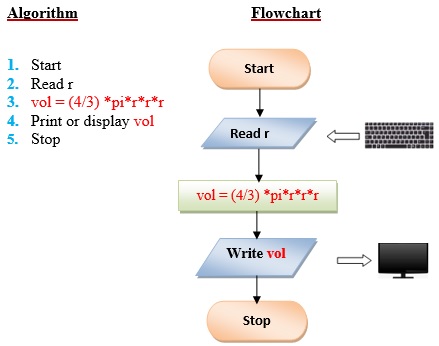
Q2 . Write a program the converts the input Celsius degree into its equivalent Fahrenheit degree. Use the formula: F = (9/5) *C+32.
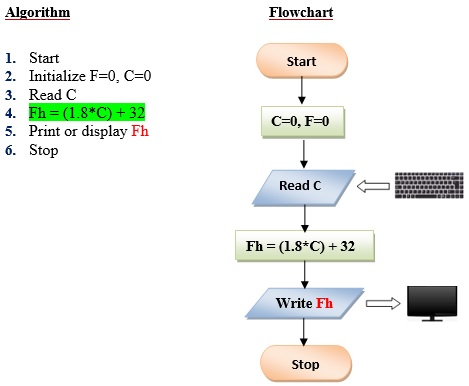
Q3 . Write a program that converts the input dollar to its peso exchange rate equivalent. Assume that the present exchange rate is 51.50 pesos against the dollar. Then display the peso equivalent exchange rate.
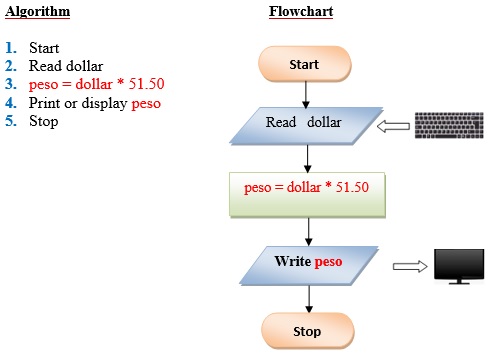
Q4 . Write a program that converts an input inch(es) into its equivalent centimeters. Take note that one inch is equivalent to 2.54cms.
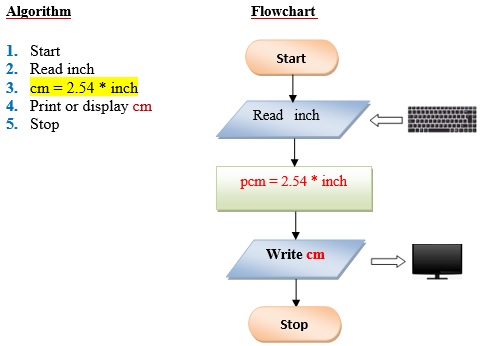
Q5 . Write a program that exchanges the value of two variables: x and y. The output must be: the value of variable y will become the value of variable x, and vice versa.
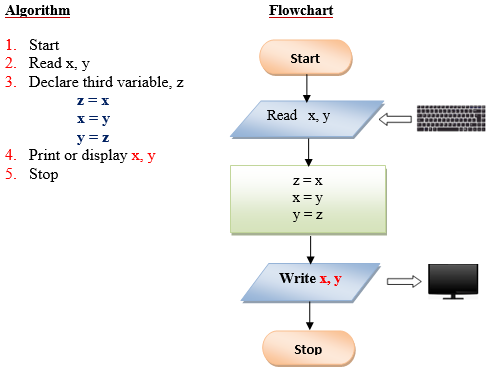
Q6 . Design a program to find the circumference of a circle. Use the formula: C=2πr, where π is approximately equivalent 3.1416.
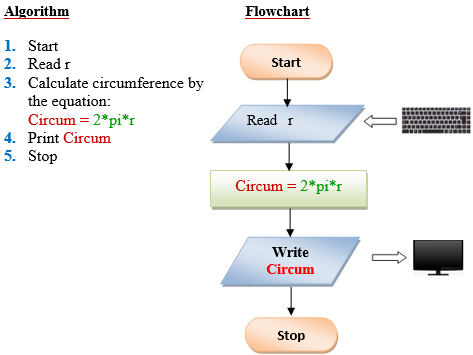
Q7 . Write a program that takes as input the purchase price of an item (P), its expected number of years of service (Y) and its expected salvage value (S). Then outputs the yearly depreciation for the item (D). Use the formula: D = (P – S) Y.
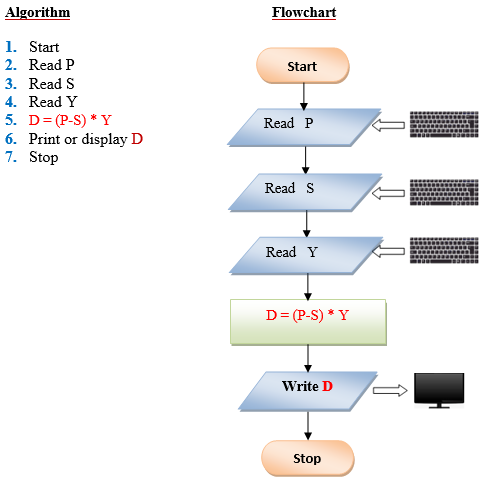
Q8 . Swapping of 2 variables without using temporary (or 3 rd variable).
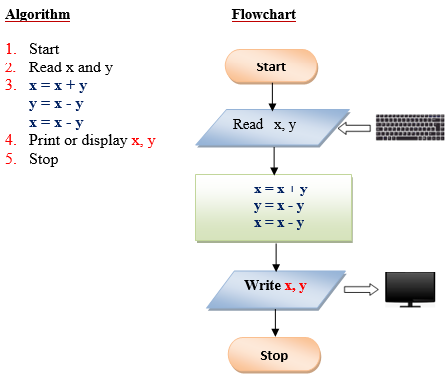
Q9 . Determine the most economical quantity to be stocked for each product that a manufacturing company has in its inventory: This quantity, called economic order quantity (EOQ) is calculated as follows: EOQ=2rs/1 where: R= total yearly production requirement S=set up cost per order I=inventory carrying cost per unit.
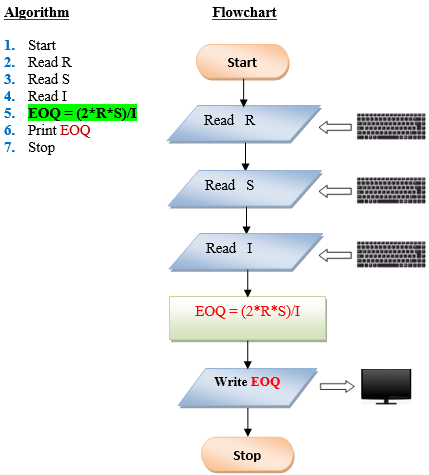
Q10 . Write a program to compute the radius of a circle. Derive your formula from the given equation: A=πr², then display the output.
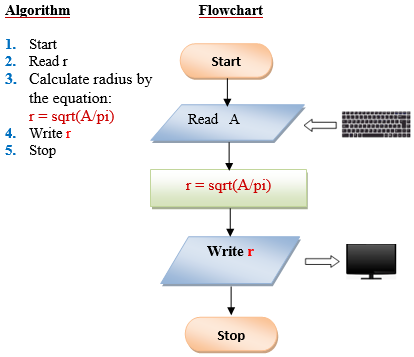
- ← Solved Assignment Problems in Java (with Algorithm and Flowchart)
- Simple if statement in C →
Gopal Krishna
Hey Engineers, welcome to the award-winning blog,Engineers Tutor. I'm Gopal Krishna. a professional engineer & blogger from Andhra Pradesh, India. Notes and Video Materials for Engineering in Electronics, Communications and Computer Science subjects are added. "A blog to support Electronics, Electrical communication and computer students".
You May Also Like
Solved assignment problems in c++ (with algorithm and flowchart), solved assignment problems in c (with algorithm and flowchart), binary search example step by step, leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Computersciencementor | Hardware, Software, Networking and programming
Algorithm and flowchart explained with examples, what is algorithm and flowchart.
Algorithm and flowchart are programming tools. A Programmer uses various programming languages to create programs. But before actually writing a program in a programming language, a programmer first needs to find a procedure for solving the problem which is known as planning the program. The program written without proper pre-planning have higher chances of errors. The tools that are used to plan or design the problem are known as programming tools. Algorithm and flowchart are widely used programming tools.
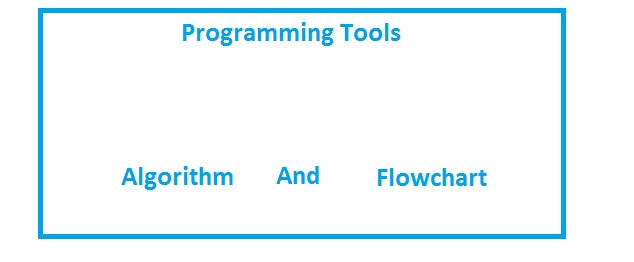
The word “algorithm” relates to the name of the mathematician Al- khowarizmi , which means a procedure or a technique. Programmer commonly uses an algorithm for planning and solving the problems.
An algorithm is a specific set of meaningful instructions written in a specific order for carrying out or solving a specific problem.
Types of Algorithm:
The algorithm and flowchart are classified into three types of control structures.
- Branching(Selection)
- Loop(Repetition)
According to the condition and requirement, these three control structures can be used.
In the sequence structure, statements are placed one after the other and the execution takes place starting from up to down.
Whereas in branch control, there is a condition and according to a condition, a decision of either TRUE or FALSE is achieved. In the case of TRUE, one of the two branches is explored; but in the case of FALSE condition, the other alternative is taken. Generally, the ‘IF-THEN’ is used to represent branch control.
Write an algorithm to find the smallest number between two numbers
Write an algorithm to check odd or even number.
The Loop or Repetition allows a statements or block of statements to be executed repeatedly based on certain loop condition. ‘While’ and ‘for’ construct are used to represent the loop structure in most programming languages. Loops are of two types: Bounded and Unbounded loop. In bounded loop, the number of iterations is fixed while in unbounded loops the condition has to satisfy to end the loop.
An algorithm to calculate even numbers between 20 and 40
Write an algorithm to input a natural number, n, and calculate the odd numbers equal or less than n.
Characteristics of a good algorithm.
- The Finite number of steps:
After starting an algorithm for any problem, it has to terminate at some point.
- Easy Modification.
There can be numbers of steps in an algorithm depending on the type of problem. It supports easy modification of Steps.
- Easy and simple to understand
A Simple English language is used while writing an algorithm. It is not dependent on any particular programming language. People without the knowledge of programming can read and understand the steps in the algorithm.
An algorithm is just a design of a program. Every program needs to display certain output after processing the input data. So one always expects the result as an output from an algorithm. It can give output at different stages. The result obtained at the end of an algorithm is known as an end result and if the result is obtained at an intermediate stage of process or operation then the result is known as an intermediate result. Also, the output has to be as expected having some relation to the inputs.
The first design of flowchart goes back to 1945 which was designed by John Von Neumann . Unlike an algorithm, Flowchart uses different symbols to design a solution to a problem. It is another commonly used programming tool.
In general, a flowchart is a diagram that uses different symbols to visually present the flow of data. By looking at a flow chart one can understand the operations and sequence of operations performed in a system. This is why flowchart is often considered as a blueprint of a design used for solving a specific problem.
A flowchart is defined as a symbolic or a graphical representation of an algorithm that uses different standard symbols.
Flowchart Symbols:
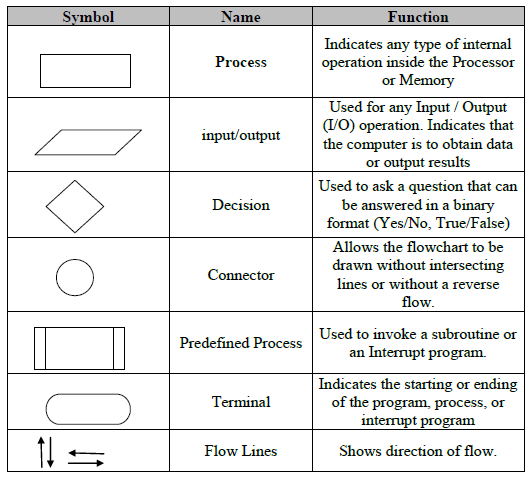
Guidelines for drawing a flowchart.
- The Title for every flowchart is compulsory.
- There must be START and END point for every flowchart.
- The symbols used in flowchart should have only one entry point on the top. The exit point for symbols (except for decision/diamond symbol) is on the button.
- There should be two exit points for decision symbol; exit points can be on the bottom and one side or on the sides.
- The flow of flowchart is generally from top to bottom. But in some cases, it can also flow to upward direction
- The direction of the flow of control should be indicated by arrowheads.
- The operations for every step should be written inside the symbol.
- The language used in flowchart should be simple so that it can be easily understood.
- The flowlines that show the direction of flow of flowchart must not cross each other.
- While connecting different pages of the same flowchart, Connectors must be used.
Some examples of algorithm and flowchart.
Example1: To calculate the area of a circle
Step1: Start
Step2: Input radius of the circle say r
Step3: Use the formula πr 2 and store result in a variable AREA
Step4: Print AREA
Step5: Stop Flowchart:
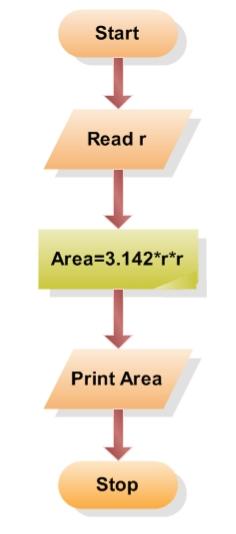
Related Posts
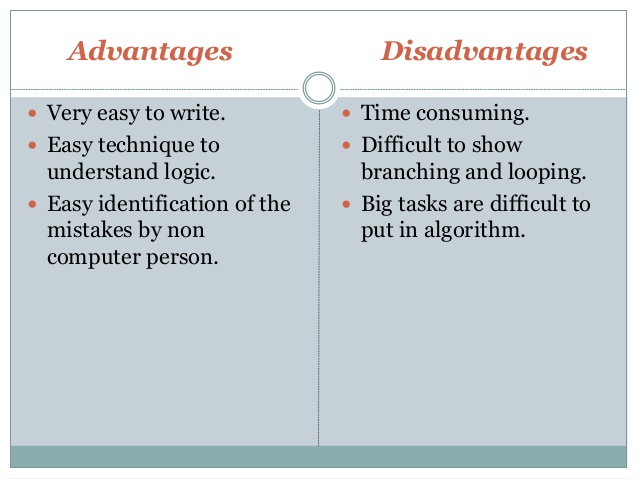
Sampurna shrestha
Save my name, email, and website in this browser for the next time I comment.
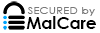
- Introduction to Algorithms and Flowcharts
Problem Solving
Today, we use computers in every field for various purposes. But, we know that they cannot solve the problems all by themselves. Furthermore, we have to give step by step instructions to the computer for solving the problem. We can define problem-solving as a process of understanding the problem, finding solutions for the problem, and finally implementing the solution to it. We can design the solution before coding in the form of algorithms and flowcharts. Moreover, the identification of arithmetic and logical operations is very important in developing the program.
Therefore, we can say that a successful problem-solving process depends on the following factors:
- understanding the problem and defining it precisely.
- designing proper algorithms and flowcharts of the solution.
- implementing the algorithm successfully.
When the problems are easy we can easily search out a solution. Whereas, complex problems require step by step process to solve. Hence, this means that we have to apply problem-solving techniques to solve the problem. Furthermore, this starts with finding a precise definition of the problem and ends with a successful solution. Moreover, the identification of arithmetic and logical operations plays a vital role while designing the algorithm. Here, we will study the algorithms and flowcharts.
Designing the solution
After understanding the relationship between input and output and the functionalities required we have to design an algorithm or flowchart. Furthermore, the algorithm should contain all the necessary functions to solve the problem. Moreover, it should produce a proper output for every input.
Hence, we can say that before writing the exact code for the problem it is necessary to define a solution. We can do this by starting with an initial plan and improvising it till it gives perfect results. Moreover, there can be more than one possible solution for a problem. Therefore, it is the responsibility of the programmer to choose the best solution.
While designing a problem we can represent it in algorithms and flowcharts. Hence, before writing the program code we can design the solution either in the form of an algorithm or a flowchart.
Browse more Topics Under Problem Solving Methodologies
- Understanding of the Problem
- Solution for the Problem
- Breaking Down Solution into Simple Steps
- Identification of Arithmetic and Logical Operations
- Control Structure
Introduction to Algorithms
An algorithm is basically a procedure of steps that we exactly follow to solve a particular task or problem. We can say that it is a set of rules that we need to follow while developing a program code during problem-solving. Furthermore, if we write an algorithm before actually writing a code, it becomes easy to perform the coding part. Moreover, the algorithm is in simple English language hence, others can also easily understand it and develop the code.
Features of an algorithm
The features of an algorithm are as follows:
The algorithm should be very clear and unambiguous in its meaning. It should be simple so that one can understand it easily.
- Well-defined inputs
A program may require to take input from the user. Therefore, the algorithm should clearly define the inputs.
- Well-defined outputs
The algorithm should clearly specify the output that the program will produce.
The algorithm should have a termination point. This means that the algorithm should not be such that it runs infinite times or end up in loops.
- Feasibility
The algorithm should be such that we can implement it easily. Hence, it should be simple and practical to implement.
- Language independent
It should be in simple English language. Since the code implementation should result in the same output no matter which programming language we use while writing the code.
Introduction to Flowcharts
It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program. Moreover, the programmers use it to depicting the flow of data and instructions while problem-solving. F lowcharting is the process of drawing a flowchart for an algorithm.
Symbols in a flowchart
The flowchart uses various symbols in the representation. These basic symbols are as follows:
It represents the start, stop, or halt in a program’s flow. The flowchart always starts and ends with this symbol. Besides, we represent it using an oval shape.
Input/Output
We represent it using a parallelogram . It indicates any input from the user or output of the program.
We represent it using a rectangle . It indicates any arithmetic operation’s processing such as addition, subtraction, multiplication, division, etc.
We represent it using the diamond symbol. It represents any type of decision in a program that results in true or false. For example. if-conditions.
We represent it using a circle . Whenever a flowchart is too large we can use connectors to avoid confusion.
These are basically arrows that represent the flow of the program.
Frequently Asked Questions (FAQs)
Q1. What are the main steps in problem-solving?
A1. A successful problem-solving process depends on the following factors:
- understanding the problem.
- designing proper algorithms and flowcharts.
- implementing the algorithm.
Q2. What is problem-solving?
A2. We can define problem-solving as a process of understanding the problem, finding solution for the problem, and finally implementing the solution to it.
Q3. What is an algorithm?
A3. An algorithm is basically a procedure of steps that we exactly follow to solve a particular task or problem.
Q4. What is a flowchart?
A4. It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program.
Q5. Name the features of an algorithm.
A5. The features of an algorithm are as follows:
Customize your course in 30 seconds
Which class are you in.

Problem Solving Methodologies
- Control Structures
- Identification of Arithmetic and Logical Operations Required for Solution
- Understanding the Problem
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

Problem-Solving Flowchart: A Visual Method to Find Perfect Solutions
Lucid Content
Reading time: about 7 min
“People ask me questions Lost in confusion Well, I tell them there's no problem Only solutions” —John Lennon, “Watching the Wheels”
Despite John Lennon’s lyrics, nobody is free from problems, and that’s especially true in business. Chances are that you encounter some kind of problem at work nearly every day, and maybe you’ve had to “put out a fire” before lunchtime once or twice in your career.
But perhaps what Lennon’s saying is that, no matter what comes our way, we can find solutions. How do you approach problems? Do you have a process in place to ensure that you and your co-workers come to the right solution?
In this article, we will give you some tips on how to find solutions visually through a problem-solving flowchart and other methods.
What is visual problem-solving?
If you are a literal thinker, you may think that visual problem-solving is something that your ophthalmologist does when your vision is blurry. For the rest of us, visual problem-solving involves executing the following steps in a visual way:
- Define the problem.
- Brainstorm solutions.
- Pick a solution.
- Implement solutions.
- Review the results.
How to make your problem-solving process more visual
Words pack a lot of power and are very important to how we communicate on a daily basis. Using words alone, you can brainstorm, organize data, identify problems, and come up with possible solutions. The way you write your ideas may make sense to you, but it may not be as easy for other team members to follow.
When you use flowcharts, diagrams, mind maps, and other visuals, the information is easier to digest. Your eyes dart around the page quickly gathering information, more fully engaging your brain to find patterns and make sense of the data.
Identify the problem with mind maps
So you know there is a problem that needs to be solved. Do you know what that problem is? Is there only one problem? Is the problem sum total of a bunch of smaller problems?
You need to ask these kinds of questions to be sure that you are working on the root of the issue. You don’t want to spend too much time and energy solving the wrong problem.
To help you identify the problem, use a mind map. Mind maps can help you visually brainstorm and collect ideas without a strict organization or structure. A mind map more closely aligns with the way a lot of our brains work—participants can bounce from one thought to the next defining the relationships as they go.
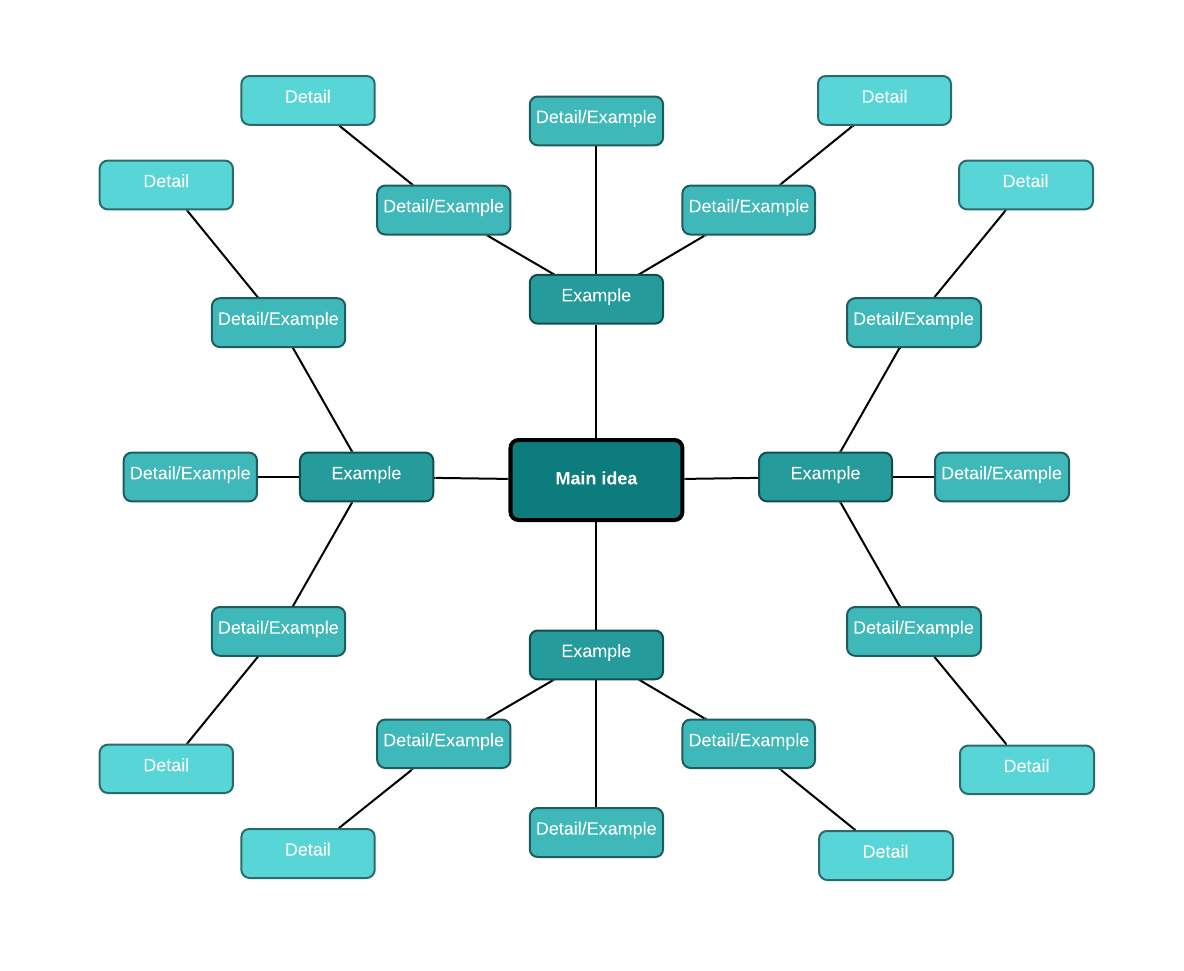
Mind mapping to solve a problem includes, but is not limited to, these relatively easy steps:
- In the center of the page, add your main idea or concept (in this case, the problem).
- Branch out from the center with possible root causes of the issue. Connect each cause to the central idea.
- Branch out from each of the subtopics with examples or additional details about the possible cause. As you add more information, make sure you are keeping the most important ideas closer to the main idea in the center.
- Use different colors, diagrams, and shapes to organize the different levels of thought.
Alternatively, you could use mind maps to brainstorm solutions once you discover the root cause. Search through Lucidchart’s mind maps template library or add the mind map shape library to quickly start your own mind map.
Create a problem-solving flowchart
A mind map is generally a good tool for non-linear thinkers. However, if you are a linear thinker—a person who thinks in terms of step-by-step progression making a flowchart may work better for your problem-solving strategy. A flowchart is a graphical representation of a workflow or process with various shapes connected by arrows representing each step.
Whether you are trying to solve a simple or complex problem, the steps you take to solve that problem with a flowchart are easy and straightforward. Using boxes and other shapes to represent steps, you connect the shapes with arrows that will take you down different paths until you find the logical solution at the end.
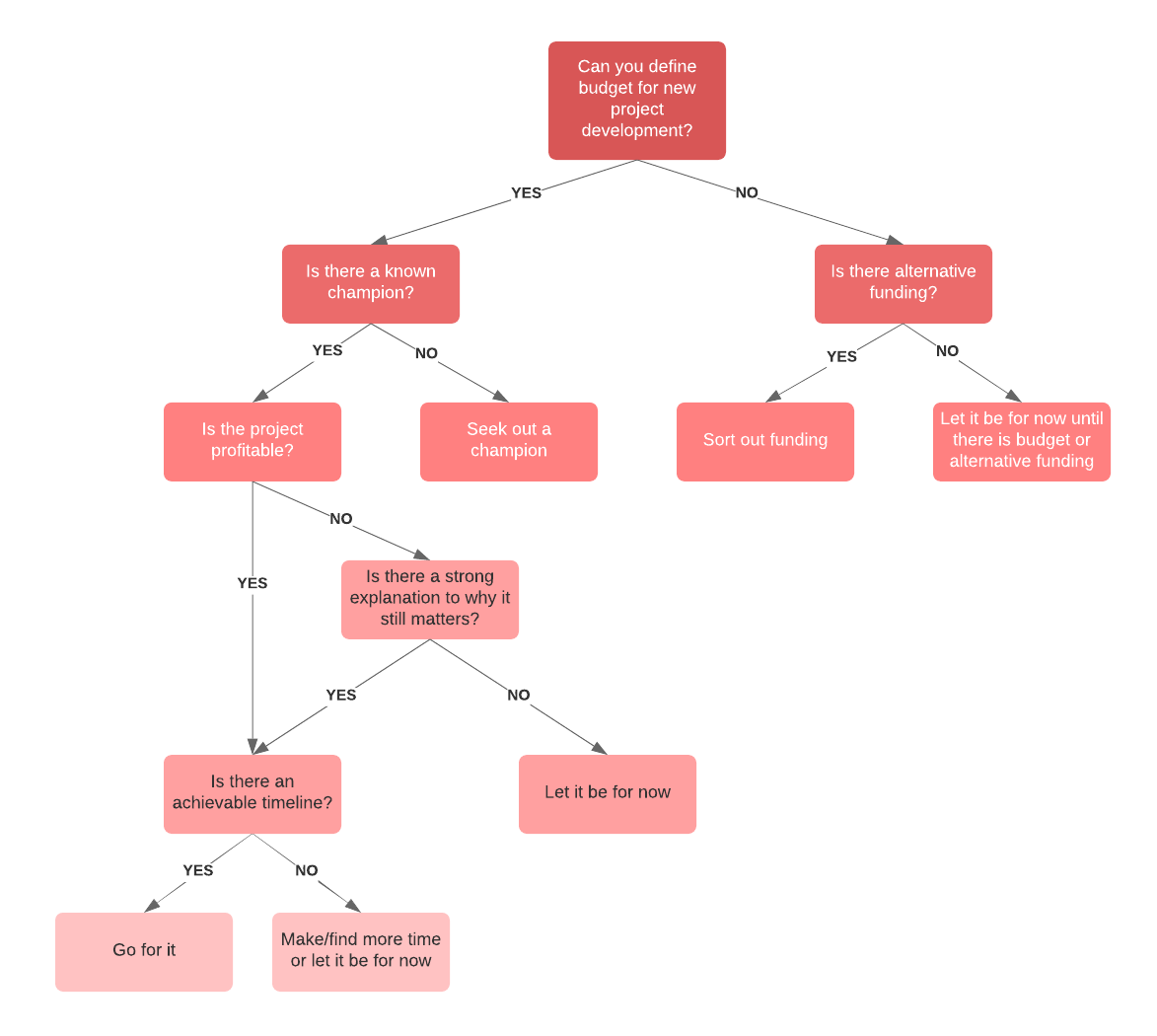
Flowcharts or decision trees are best used to solve problems or answer questions that are likely to come up multiple times. For example, Yoder Lumber , a family-owned hardwood manufacturer, built decision trees in Lucidchart to demonstrate what employees should do in the case of an injury.
To start your problem-solving flowchart, follow these steps:
- Draw a starting shape to state your problem.
- Draw a decision shape where you can ask questions that will give you yes-or-no answers.
- Based on the yes-or-no answers, draw arrows connecting the possible paths you can take to work through the steps and individual processes.
- Continue following paths and asking questions until you reach a logical solution to the stated problem.
- Try the solution. If it works, you’re done. If it doesn’t work, review the flowchart to analyze what may have gone wrong and rework the flowchart until you find the solution that works.
If your problem involves a process or workflow , you can also use flowcharts to visualize the current state of your process to find the bottleneck or problem that’s costing your company time and money.
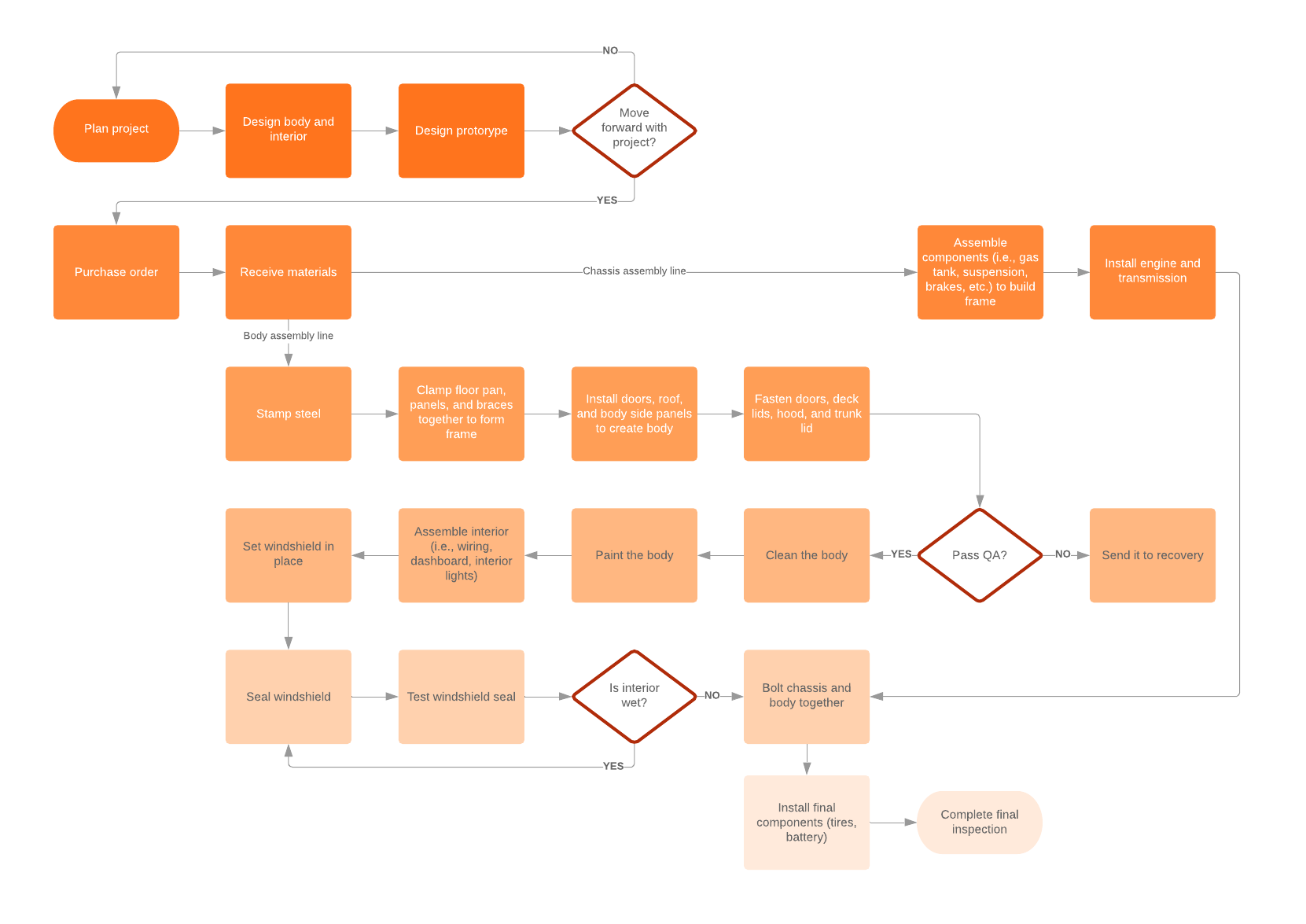
Lucidchart has a large library of flowchart templates to help you analyze, design, and document problem-solving processes or any other type of procedure you can think of.
Draw a cause-and-effect diagram
A cause-and-effect diagram is used to analyze the relationship between an event or problem and the reason it happened. There is not always just one underlying cause of a problem, so this visual method can help you think through different potential causes and pinpoint the actual cause of a stated problem.
Cause-and-effect diagrams, created by Kaoru Ishikawa, are also known as Ishikawa diagrams, fishbone diagrams , or herringbone diagrams (because they resemble a fishbone when completed). By organizing causes and effects into smaller categories, these diagrams can be used to examine why things went wrong or might go wrong.
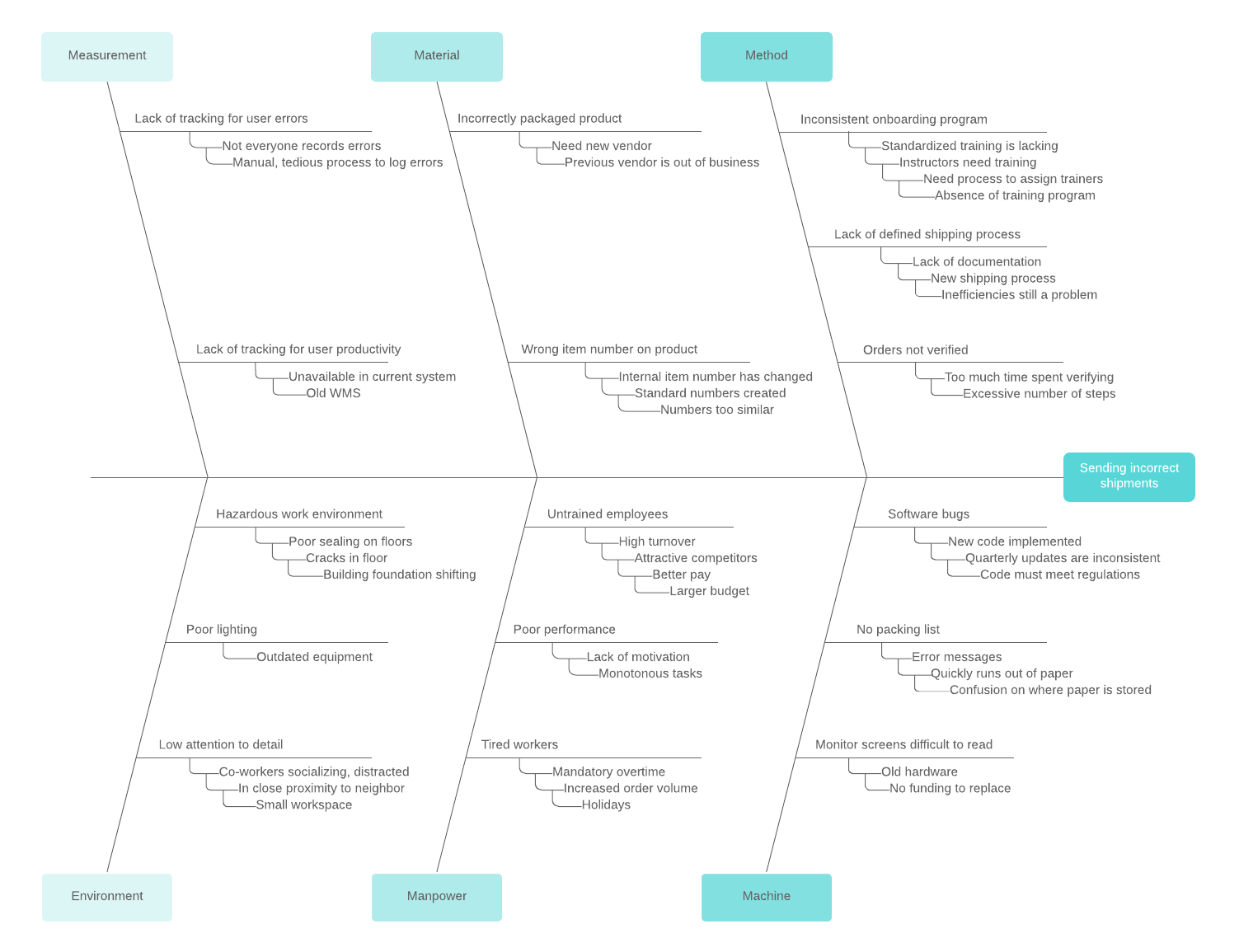
To perform a cause-and-effect analysis, follow these steps.
1. Start with a problem statement.
The problem statement is usually placed in a box or another shape at the far right of your page. Draw a horizontal line, called a “spine” or “backbone,” along the center of the page pointing to your problem statement.
2. Add the categories that represent possible causes.
For example, the category “Materials” may contain causes such as “poor quality,” “too expensive,” and “low inventory.” Draw angled lines (or “bones”) that branch out from the spine to these categories.
3. Add causes to each category.
Draw as many branches as you need to brainstorm the causes that belong in each category.
Like all visuals and diagrams, a cause-and-effect diagram can be as simple or as complex as you need it to be to help you analyze operations and other factors to identify causes related to undesired effects.
Collaborate with Lucidchart
You may have superior problem-solving skills, but that does not mean that you have to solve problems alone. The visual strategies above can help you engage the rest of your team. The more involved the team is in the creation of your visual problem-solving narrative, the more willing they will be to take ownership of the process and the more invested they will be in its outcome.
In Lucidchart, you can simply share the documents with the team members you want to be involved in the problem-solving process. It doesn’t matter where these people are located because Lucidchart documents can be accessed at any time from anywhere in the world.
Whatever method you decide to use to solve problems, work with Lucidchart to create the documents you need. Sign up for a free account today and start diagramming in minutes.
Lucidchart, a cloud-based intelligent diagramming application, is a core component of Lucid Software's Visual Collaboration Suite. This intuitive, cloud-based solution empowers teams to collaborate in real-time to build flowcharts, mockups, UML diagrams, customer journey maps, and more. Lucidchart propels teams forward to build the future faster. Lucid is proud to serve top businesses around the world, including customers such as Google, GE, and NBC Universal, and 99% of the Fortune 500. Lucid partners with industry leaders, including Google, Atlassian, and Microsoft. Since its founding, Lucid has received numerous awards for its products, business, and workplace culture. For more information, visit lucidchart.com.
Related articles
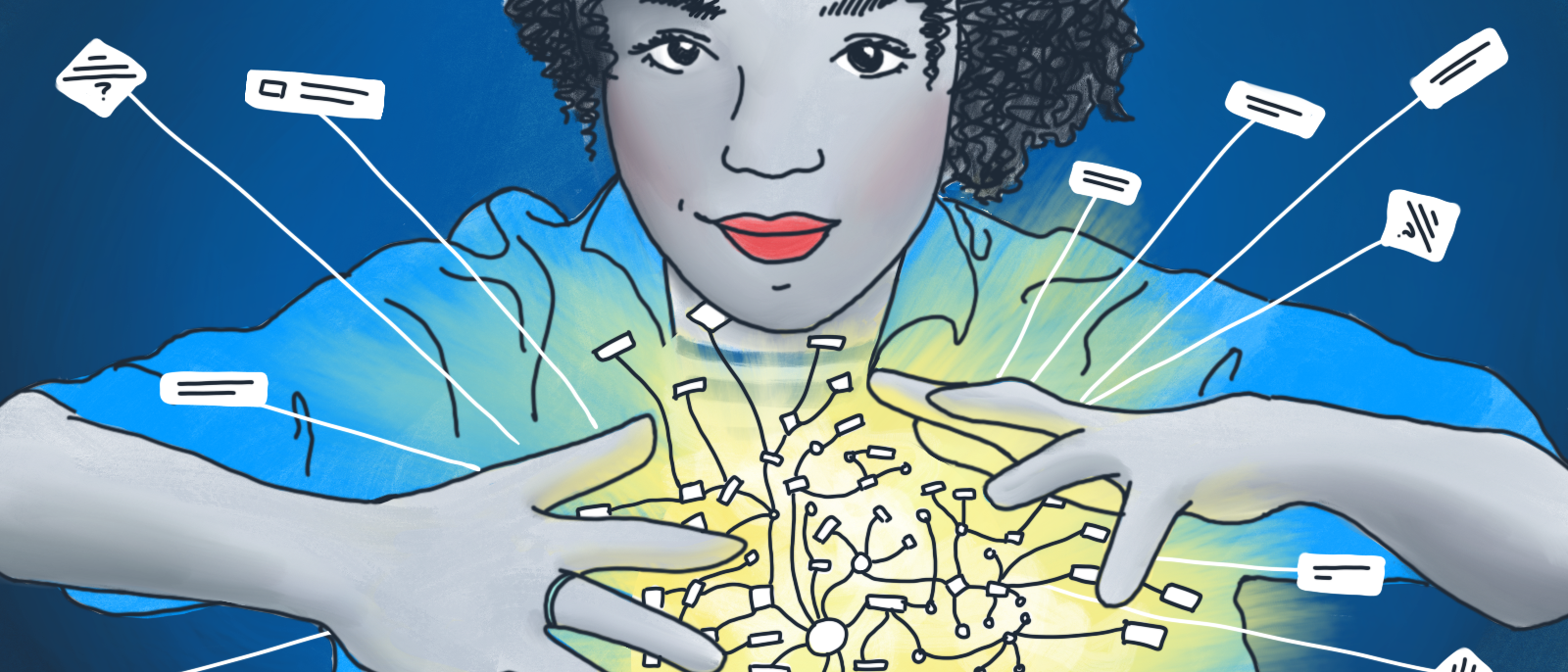
Sometimes you're faced with challenges that traditional problem solving can't fix. Creative problem solving encourages you to find new, creative ways of thinking that can help you overcome the issue at hand more quickly.
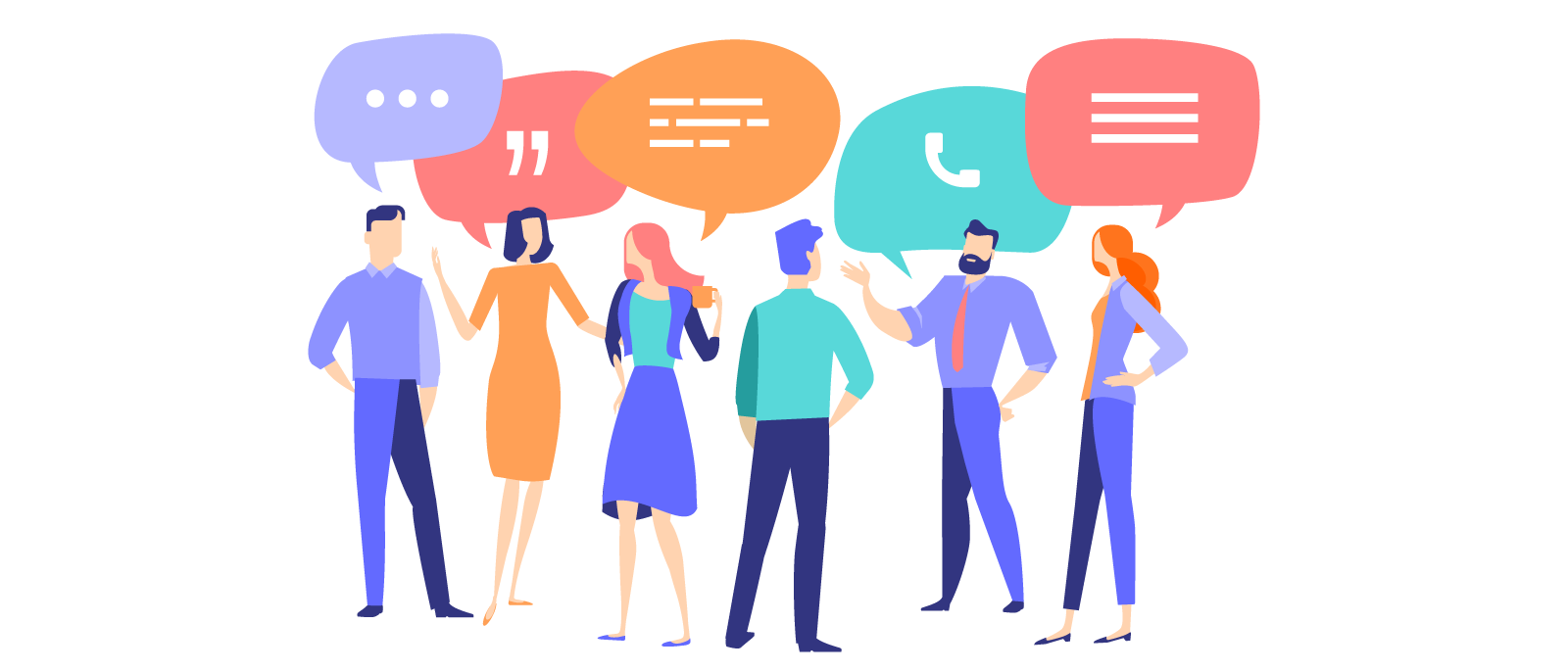
Dialogue mapping is a facilitation technique used to visualize critical thinking as a group. Learn how you and your team can start dialogue mapping today to solve problems and bridge gaps in knowledge and understanding (plus get a free template!).
Bring your bright ideas to life.
or continue with

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
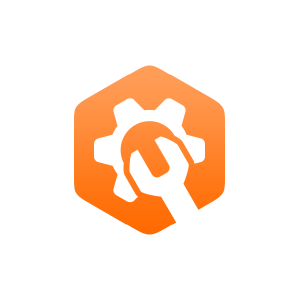
1: Algorithmic Problem Solving
- Last updated
- Save as PDF
- Page ID 46789
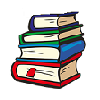
- Harrison Njoroge
- African Virtual University
Unit Objectives
Upon completion of this unit the learner should be able to:
- describe an algorithm
- explain the relationship between data and algorithm
- outline the characteristics of algorithms
- apply pseudo codes and flowcharts to represent algorithms
Unit Introduction
This unit introduces learners to data structures and algorithm course. The unit is on the different data structures and their algorithms that can help implement the different data structures in the computer. The application of the different data structures is presented by using examples of algorithms and which are not confined to a particular computer programming language.
- Data: the structural representation of logical relationships between elements of data
- Algorithm: finite sequence of steps for accomplishing some computational task
- Pseudo code: an informal high-level description of the operating principle of a computer program or other algorithm
- Flow chart: diagrammatic representation illustrates a solution model to a given problem.
Learning Activities
- 1.1: Activity 1 - Introduction to Algorithms and Problem Solving In this learning activity section, the learner will be introduced to algorithms and how to write algorithms to solve tasks faced by learners or everyday problems. Examples of the algorithm are also provided with a specific application to everyday problems that the learner is familiar with. The learners will particularly learn what is an algorithm, the process of developing a solution for a given task, and finally examples of application of the algorithms are given.
- 1.2: Activity 2 - The characteristics of an algorithm This section introduces the learners to the characteristics of algorithms. These characteristics make the learner become aware of what to ensure is basic, present and mandatory for any algorithm to qualify to be one. It also exposes the learner to what to expect from an algorithm to achieve or indicate. Key expectations are: the fact that an algorithm must be exact, terminate, effective, general among others.
- 1.3: Activity 3 - Using pseudo-codes and flowcharts to represent algorithms The student will learn how to design an algorithm using either a pseudo code or flowchart. Pseudo code is a mixture of English like statements, some mathematical notations and selected keywords from a programming language. It is one of the tools used to design and develop the solution to a task or problem. Pseudo codes have different ways of representing the same thing and emphasis is on the clarity and not style.
- 1.4: Unit Summary In this unit, you have seen what an algorithm is. Based on this knowledge, you should now be able to characterize an algorithm by stating its properties. We have explored the different ways of representing an algorithm such as using human language, pseudo codes and flow chart. You should now be able to present solutions to problems in form of an algorithm.
Popular Tutorials
Popular examples, learn python interactively, related articles.
- self in Python, Demystified
- Increment ++ and Decrement -- Operator as Prefix and Postfix
- Interpreter Vs Compiler : Differences Between Interpreter and Compiler
- Algorithm in Programming
Flowchart In Programming
A flowchart is a diagrammatic representation of an algorithm. A flowchart can be helpful for both writing programs and explaining the program to others.
Symbols Used In Flowchart
Examples of flowcharts in programming.
1. Add two numbers entered by the user.
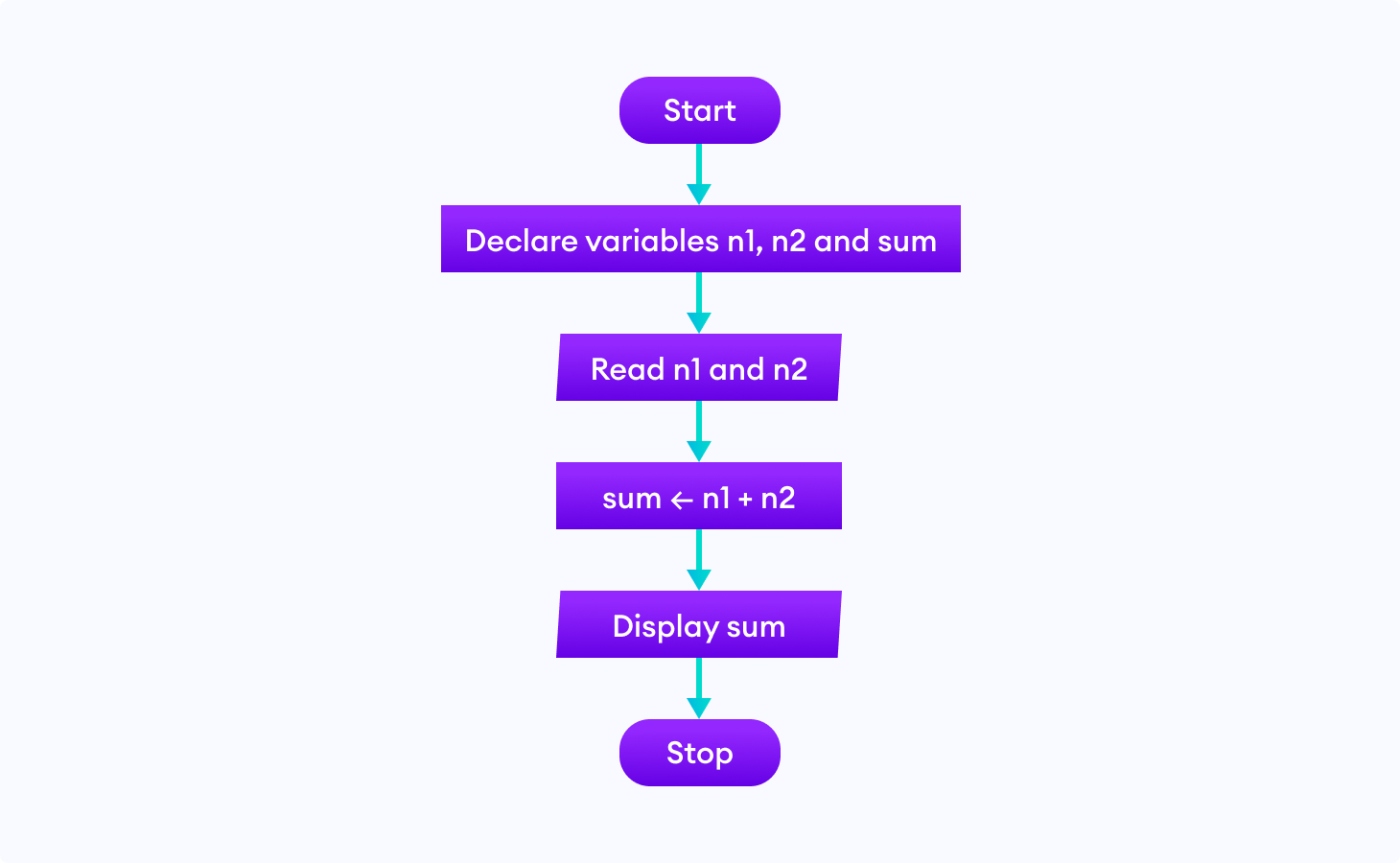
2. Find the largest among three different numbers entered by the user.
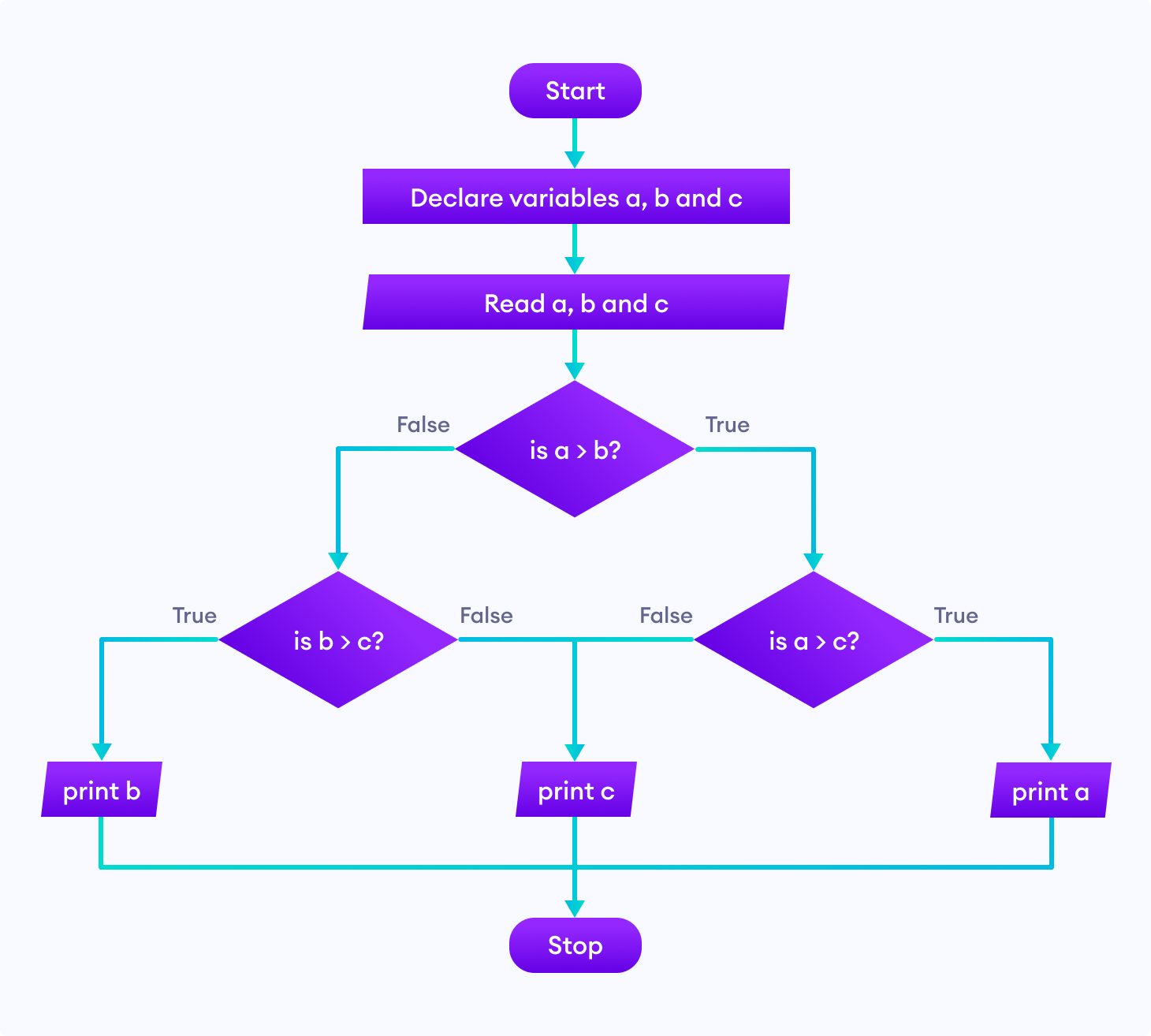
3. Find all the roots of a quadratic equation ax 2 +bx+c=0
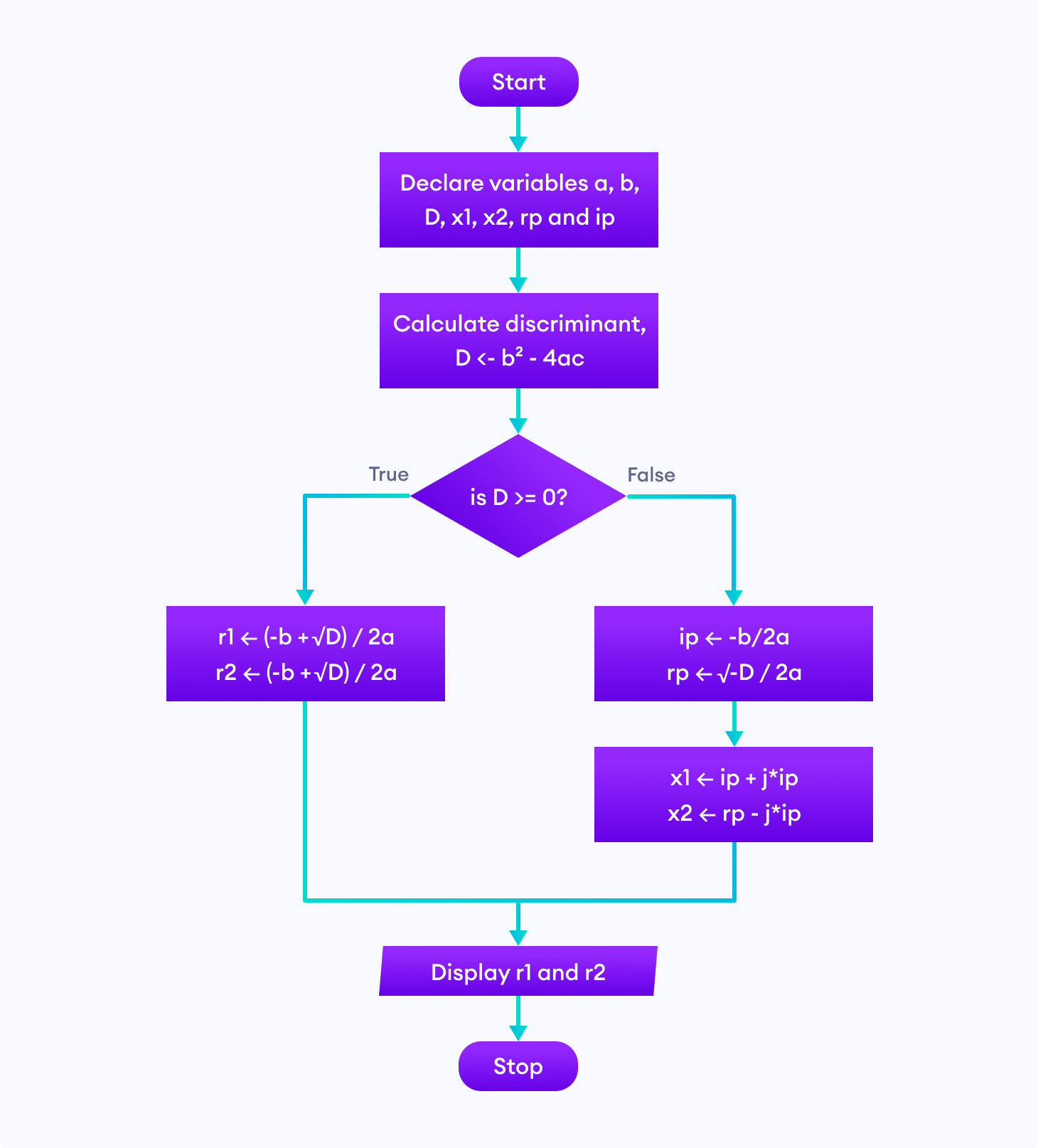
4. Find the Fibonacci series till term≤1000.
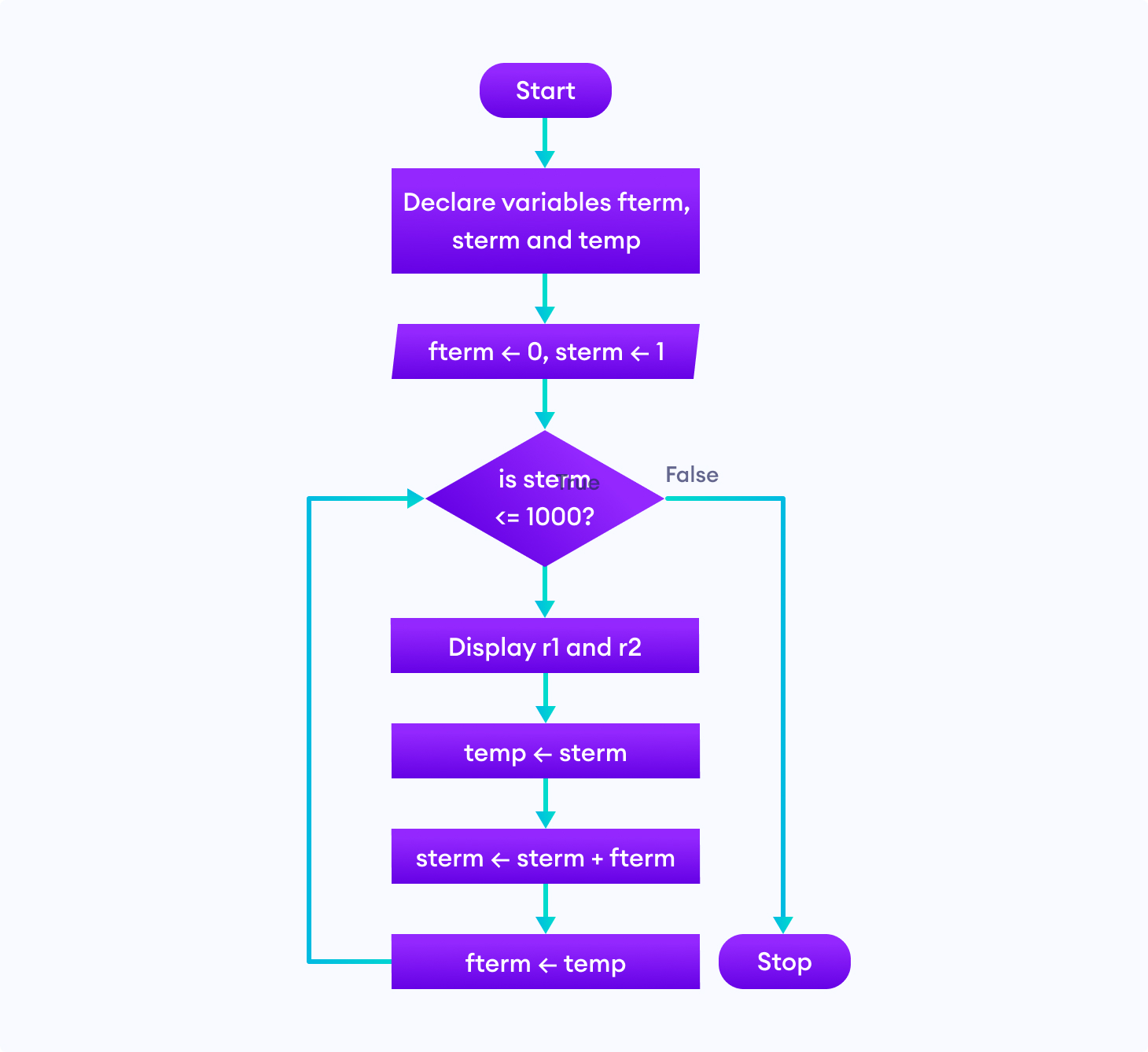
Note: Though flowcharts can be useful for writing and analyzing a program, drawing a flowchart for complex programs can be more complicated than writing the program itself. Hence, creating flowcharts for complex programs is often ignored.
Sorry about that.
Algorithms and Problem Solving
Algorithms and flowcharts (designing a program) .
Reference this presentation for your first homework.
Five Simple Ideas Used to Create All Programs
- A list of instructions performed in order
- If…, then…, else…
- Repeated behavior (i.e., while there are cookies on the plate, keep eating cookies)
- Breaking the problem into sub-problems that can be solved independently.
- A set of instructions that return a single result (answer a question).
Complexity
Most computers only really “understand” about 100 different instructions.
Powerful applications take advantage of the extreme number of possible instruction combinations.
Chess is a good analogy:
- 6 types of pieces; each piece moves in a simple pattern.
- Possible/playable chess games (assuming an avg. game has 30 moves) are 4,670,033.
Algorithms
An algorithm is A step-by-step list of instructions for solving a problem. The solution must be determined in a finite amount of time.
Algorithms can be expressed in many kinds of notations (e.g., natural language, pseudocode, flowcharts, etc.)
Flowcharts
A flowchart is one way to represent an algorithm and uses the following symbols.
Creating Solutions
- Step-by-step problem-solving process
- In a finite amount of time
- Programming is a process of problem-solving.
Programming with the Problem Analysis–Coding–Execution Cycle
Analyze the problem
- Does the program require user interaction?
- Does the program manipulate data?
- What is the output?
- Analyze and design algorithms for each subproblem
- Can test using sample data
- Some mathematical analysis might be required
Implement the algorithm
- Enter the program using a text editor. This is called the implementation of the algorithm.
- Compile code
- Look at the code and remove errors
- Run code again through the compiler
- The compiler guarantees that the program follows the rules of the language. It does not guarantee that the program will run correctly.
- Linker links machine code with system resources
Execution (run the compiles program)
- Once compiled and linked, the loader can place the program into the main memory for execution.
- The final step is to execute the program.
Maintenance
- Use and modify the program if the problem domain changes.
Programming Methods
Two popular approaches to programming design
- Structured (e.g., Procedural)
- Object-oriented
There are many other programming paradigms .
Structured Programming
Procedural design is a subset of structured design:
- Dividing a problem into smaller subproblems
- A solution to a subproblem is a “module” or “procedure” and is simply a series of computation steps to be carried out.
- Top-down (or bottom-up) design
- Stepwise refinement
- Modular programming
Object-Oriented Programming
Object-oriented design :
- Identify components called objects.
- Determine how objects interact with each other
- Specify relevant data and possible operations to be performed on that data.
- Each object consists of data and operations on that data.
- An object combines data and operations on the data into a single unit
- A language that supports object-oriented design is called an object-oriented programming (OOP) language
- Must learn how to represent data in computer memory, how to manipulate data, and how to implement operations.
- C++ was designed to support object-oriented programming.
- Object-oriented design is used with structured design.
Flowcharts and Pseudocode
Lesson objective .
In this lesson we will be learning about flowcharts and pseudocode in detail by drawing and writing them for some example problems. As you already know, both of these are techniques to represent algorithms and programming logic. They are important because they help us to explain our programming logic better and in a clear way to someone else and also it enables us to debug any error in our logic.
Flowcharts
You are already aware that flowcharts are diagrammatic representations of algorithms. You know which symbols are used to draw flowcharts and the basic guidelines to draw them. You also know about their importance and usage.
Guidelines for developing flowcharts
Flowchart should have only one start and one stop symbol
General flow of processes is top to bottom or left to right
Arrows should not cross each other
A step by step guide how to create and use flowcharts and different symbols used in them:
Follow-up question
Q. ___ is a diagrammatic representation of ordered sequences of steps to carry out an operation.
A. Algorithm
B. Flowchart
C. Computer program
D. None of the above
Ans: B) Flowchart
Tools to draw flowcharts
Now, let’s discover some tools you can use to draw flowcharts.
1. Diagrams.net
You can go to website and download the diagram drawing tool to draw flowcharts. It is easy to use and involves simple drag and drop to draw the symbols in the flowchart.
This is a fun way to draw flowcharts as opposed to the conventional pen and paper drawing.
2. Flowgorithm
A more amazing tool is Flowgorithm. Flowgorithm is a free beginner's programming language that is based on simple graphical flowcharts. It is closer to how you actually code to implement your logic in a programming language. In fact, it allows you to convert the flowcharts you create directly into the code of any programming language of your choice. You can convert your flowcharts into pseudo code as well. You can also run your programs directly in Flowgorithm.
For beginners, many programming languages can be frustratingly difficult. Many of them require you to write lines of confusing code just to display the text "Hello, world!". By using flowcharts, you can concentrate on programming concepts rather than all the nuances of a typical programming language.
But, if you don’t have experience with any programming language, it’s better to use diagrams.net for now and just focus on the problem solving aspect. When you’re more familiar with programming languages, Flowgorithm can be a great tool to use.
For windows user the flowgorithm software is directly accessible on website , however for the Mac users please follow the instructions given in the video below:
You can learn the basics of how to make a flowchart using Flowgorithm from this tutorial:
Tuturial Link
Here is a video tutorial as well.
Well!! We are all set to draw a flowchart. Now go out and try a flowchart for the sample problem statement given.
Guidelines to submit your Flowchart
Choose your method of drawing the flowchart.
Once you are done, export it as an image file and save to your local files.
Upload the image to the link attached next to the Problem statement.
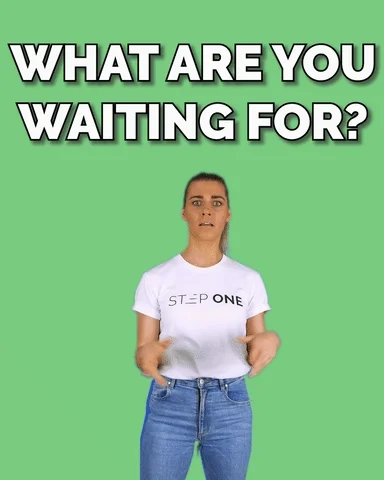
Problem Statement: You have to add two numbers entered by the user.
Step 1: Marking the start of the algorithm (with an oval shaped symbol)
Step 2: Declaring variables n1,n2 and sum to be used (in a rectangular box that is used for processing and writing instructions)
📌 Note: Declaring variables is a stylistic choice and variables can be used directly as well. You will see in future lessons that some programming languages require variable declaration before use and some do not.
Step 3: Taking input of n1 and n2 from the user (in parallelogram shaped box)
Step 4: Performing sum operation for n1 and n2 (in rectangular shaped box)
Step 5: Giving sum as output (in parallelogram shaped box)
Step 6: Marking the end of the algorithm (with an oval shaped symbol)
Submit your Flowchart!
Now, you can check your answer with the solution.
Check the Flowchart!
Pseudocode
Pseudocode is defined as a method of describing a process or writing programming code and algorithms using a natural language such as English. It is not the code itself, but rather a description of what the code should do. In other words, it is used as a detailed yet understandable step-by-step plan or blueprint from which a program can be written. It is like a rough draft of a program or an algorithm before it is implemented in a programming language. It can also be referred to as 'false code' or 'representation of code'.
Guidelines for writing pseudocode
You are already aware of the main constructs used in pseudocode. Now, you will learn about the general guidelines to follow while writing pseudo code.
When writing pseudocode, everyone often has their own style of presenting things out since it’s read by humans and not by a computer; its rules are less rigorous than that of a programming language. However, there are some simple rules that help make pseudocode more universally understood.
Always capitalise the initial word (often one of the main 6 constructs).
Maintain the habit of keeping only one statement per line.
Indent to show hierarchy, improve readability, and show nested constructs.
Always end multi line sections using any of the END keywords (ENDIF, ENDWHILE, etc.).
Keep your statements programming language independent.
Use the naming domain of the problem, not that of the implementation. E.g., "Append the last name to the first name" instead of "name = first or last."
Keep it simple, concise, and readable.
Following these rules help you generate readable pseudocode and give you the ability to recognize an ill-written code.
Tool to write Pseudocode
You can use any text editor for writing pseudocode because it is only written for representation of algorithms and program logic. But, if you want to be able to run the logic you write, even though that might reduce some flexibility of how you write the algorithm, you can do so with an awesome online tool called pseudoeditor.com .
Guidelines to submit your Pseudocode
Choose your method of editor.
Once you are done, save it as a .txt file on your local file.
Upload the txt file to the link attached next to the Problem statement.
Let's try a sample problem now.
Submit your Pseudocode!
Check the solution below, once you are done.
NUMBER n1, n2, sum
INPUT n1, n2
SUM = n1 + n2
Let's try more such Flowchart and pseudocode based problems.
Remember, this is the first step to problem solving!!
Problem Statement: Find the largest among three different numbers entered by the user.
(You can skip to check these steps and try on your own)
Step 1: Mark the start of the algorithm
Step 2: Declare a,b,c the three numbers
Step 3: Take a,b,c as input
Step 4: Write a condition to check if a>b or not (inside a diamond shaped decision box); if above condition is true goto step 5 else goto step 6
Step 5: We come to this step if a>b and now we check if a>c or not. If this condition is true then we will output "a" else we will output "c" according to our logic.
Step 6: We come to this step if a is lesser than b and now we check whether b is greater than c or not. If this condition is true we output "b" else we output "c".
Step 7: We mark the end of algorithm
INPUT a, b, c
IF a > b
Problem Statement: Find the sum of series 1+2+3+…..+N.
Step 2: Declare i and sum with values 1 and 0 respectively.
Step 3: Take N as input
Step 4: Write a condition to check if i <= N or not (inside a diamond shaped decision box); if the condition is true goto step 5 else goto step 6.
Step 5: We come to this step if i is still less than or equal to N. Now, increase the value of sum to sum+i. Then, increment i by 1. Then go to step 4 (this is a loop)
Step 6: We come to this step when i is greater than N. Now, print the value of sum.
sum = 0, i = 1
WHILE i <= n
sum = sum + i
Now, it's time to think steps on your own. 🔥
Problem Statement: Find the roots of a quadratic equation aX^2 + bX + c = 0
INPUT a,b,c
d = b b - 4 a*c
IF (d equals 0)
r1 = r2 = (-b) / 2*a
ELSE IF (d > 0)
r1 = ((-b)+sqrt(d) / 2*a
r2 = ((-b)-sqrt(d) / 2*a
PRINT r1, r2
Problem Statement: Find The LCM (Lowest Common Multiple) of two numbers taken from the user as input.
📌 Note: LCM is the smallest positive integer that is perfectly divisible by both numbers
IF n1 > n2
rem1 = lcm % n1
rem2 = lcm % n2
WHILE (rem1 > 0 OR rem2 > 0)
📌 Note: As you may already know, the remainder is being calculated by the modulus operator(%) which gives us the remainder that is left on dividing the first operand by the second operand.
Problem Statement: Print the Fibonacci Series upto n terms. A series of numbers in which each number is the sum of the two preceding or previous numbers is called Fibonacci Series. The first few terms are as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, … , n.
📌 Note: The first two terms are by default 0 and 1. And their sum forms the third term and similarly the series propagates.
PRINT a, b on separate lines
sum = a + b
PRINT sum on next line
Useful resources for you:
Flowchart examples: https://visme.co/blog/flowchart-examples/
- Lesson Objective
- Guidelines for developing flowcharts
- Tools to draw flowcharts
- Guidelines for writing pseudocode
- Tool to write Pseudocode
- Useful resources for you:
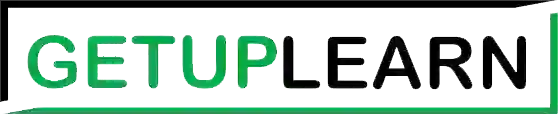
What is Problem Solving Algorithm?, Steps, Representation
- Post author: Disha Singh
- Post published: 6 June 2021
- Post category: Computer Science
- Post comments: 0 Comments
Table of Contents
- 1 What is Problem Solving Algorithm?
- 2 Definition of Problem Solving Algorithm
- 3.1 Analysing the Problem
- 3.2 Developing an Algorithm
- 3.4 Testing and Debugging
- 4.1 Flowchart
- 4.2 Pseudo code
What is Problem Solving Algorithm?
Computers are used for solving various day-to-day problems and thus problem solving is an essential skill that a computer science student should know. It is pertinent to mention that computers themselves cannot solve a problem. Precise step-by-step instructions should be given by us to solve the problem.
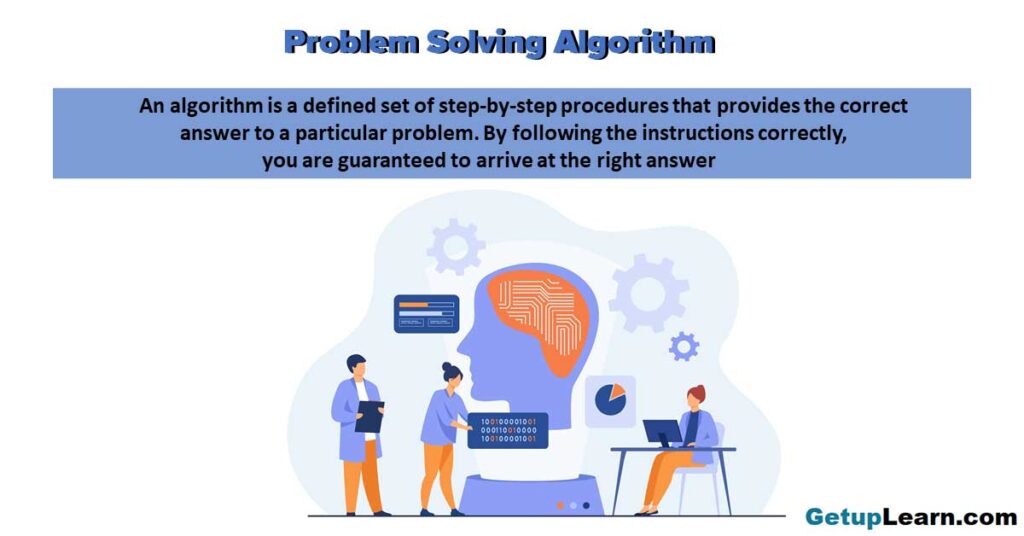
Thus, the success of a computer in solving a problem depends on how correctly and precisely we define the problem, design a solution (algorithm) and implement the solution (program) using a programming language.
Thus, problem solving is the process of identifying a problem, developing an algorithm for the identified problem and finally implementing the algorithm to develop a computer program.
Definition of Problem Solving Algorithm
These are some simple definition of problem solving algorithm which given below:
Steps for Problem Solving
When problems are straightforward and easy, we can easily find the solution. But a complex problem requires a methodical approach to find the right solution. In other words, we have to apply problem solving techniques.
Problem solving begins with the precise identification of the problem and ends with a complete working solution in terms of a program or software. Key steps required for solving a problem using a computer.
For Example: Suppose while driving, a vehicle starts making a strange noise. We might not know how to solve the problem right away. First, we need to identify from where the noise is coming? In case the problem cannot be solved by us, then we need to take the vehicle to a mechanic.
The mechanic will analyse the problem to identify the source of the noise, make a plan about the work to be done and finally repair the vehicle in order to remove the noise. From the example, it is explicit that, finding the solution to a problem might consist of multiple steps.
Following are Steps for Problem Solving :
Analysing the Problem
Developing an algorithm, testing and debugging.
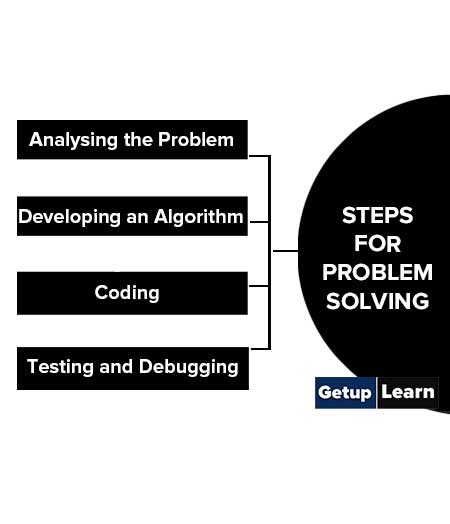
It is important to clearly understand a problem before we begin to find the solution for it. If we are not clear as to what is to be solved, we may end up developing a program which may not solve our purpose.
Thus, we need to read and analyse the problem statement carefully in order to list the principal components of the problem and decide the core functionalities that our solution should have. By analysing a problem, we would be able to figure out what are the inputs that our program should accept and the outputs that it should produce.
It is essential to device a solution before writing a program code for a given problem. The solution is represented in natural language and is called an algorithm. We can imagine an algorithm like a very well-written recipe for a dish, with clearly defined steps that, if followed, one will end up preparing the dish.
We start with a tentative solution plan and keep on refining the algorithm until the algorithm is able to capture all the aspects of the desired solution. For a given problem, more than one algorithm is possible and we have to select the most suitable solution.
After finalising the algorithm, we need to convert the algorithm into the format which can be understood by the computer to generate the desired solution. Different high level programming languages can be used for writing a program. It is equally important to record the details of the coding procedures followed and document the solution. This is helpful when revisiting the programs at a later stage.
The program created should be tested on various parameters. The program should meet the requirements of the user. It must respond within the expected time. It should generate correct output for all possible inputs. In the presence of syntactical errors, no output will be obtained. In case the output generated is incorrect, then the program should be checked for logical errors, if any.
Software industry follows standardised testing methods like unit or component testing, integration testing, system testing, and acceptance testing while developing complex applications. This is to ensure that the software meets all the business and technical requirements and works as expected.
The errors or defects found in the testing phases are debugged or rectified and the program is again tested. This continues till all the errors are removed from the program. Once the software application has been developed, tested and delivered to the user, still problems in terms of functioning can come up and need to be resolved from time to time.
The maintenance of the solution, thus, involves fixing the problems faced by the user, answering the queries of the user and even serving the request for addition or modification of features.
Representation of Algorithms
Using their algorithmic thinking skills, the software designers or programmers analyse the problem and identify the logical steps that need to be followed to reach a solution. Once the steps are identified, the need is to write down these steps along with the required input and desired output.
There are two common methods of representing an algorithm —flowchart and pseudocode. Either of the methods can be used to represent an algorithm while keeping in mind the following:
- It showcases the logic of the problem solution, excluding any implementational details.
- It clearly reveals the flow of control during execution of the program.
A flowchart is a visual representation of an algorithm . A flowchart is a diagram made up of boxes, diamonds and other shapes, connected by arrows. Each shape represents a step of the solution process and the arrow represents the order or link among the steps.
A flow chart is a step by step diagrammatic representation of the logic paths to solve a given problem. Or A flowchart is visual or graphical representation of an algorithm .
The flowcharts are pictorial representation of the methods to b used to solve a given problem and help a great deal to analyze the problem and plan its solution in a systematic and orderly manner. A flowchart when translated in to a proper computer language, results in a complete program.
Advantages of Flowcharts:
- The flowchart shows the logic of a problem displayed in pictorial fashion which felicitates easier checking of an algorithm
- The Flowchart is good means of communication to other users. It is also a compact means of recording an algorithm solution to a problem.
- The flowchart allows the problem solver to break the problem into parts. These parts can be connected to make master chart.
- The flowchart is a permanent record of the solution which can be consulted at a later time.
Differences between Algorithm and Flowchart
Pseudo code.
The Pseudo code is neither an algorithm nor a program. It is an abstract form of a program. It consists of English like statements which perform the specific operations. It is defined for an algorithm. It does not use any graphical representation.
In pseudo code , the program is represented in terms of words and phrases, but the syntax of program is not strictly followed.
Advantages of Pseudocode
- Before writing codes in a high level language, a pseudocode of a program helps in representing the basic functionality of the intended program.
- By writing the code first in a human readable language, the programmer safeguards against leaving out any important step. Besides, for non-programmers, actual programs are difficult to read and understand.
- But pseudocode helps them to review the steps to confirm that the proposed implementation is going to achieve the desire output.
Related posts:
10 Types of Computers | History of Computers, Advantages
What is microprocessor evolution of microprocessor, types, features, types of computer memory, characteristics, primary memory, secondary memory, data and information: definition, characteristics, types, channels, approaches, what is cloud computing classification, characteristics, principles, types of cloud providers, what is debugging types of errors, types of storage devices, advantages, examples, 10 evolution of computing machine, history, what are functions of operating system 6 functions, advantages and disadvantages of operating system.
- Data Representation in Computer: Number Systems, Characters, Audio, Image and Video
- What are Data Types in C++? Types
- What are Operators in C? Different Types of Operators in C
- What are Expressions in C? Types
What are Decision Making Statements in C? Types
You might also like.
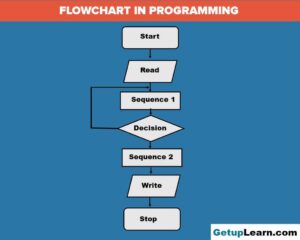
What is Flowchart in Programming? Symbols, Advantages, Preparation
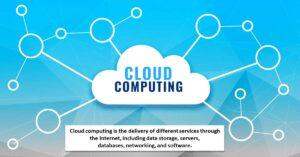
Generations of Computer First To Fifth, Classification, Characteristics, Features, Examples
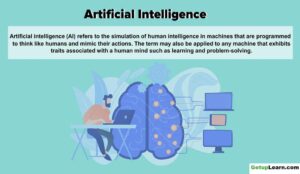
What is Artificial Intelligence? Functions, 6 Benefits, Applications of AI
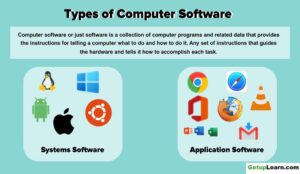
Types of Computer Software: Systems Software, Application Software
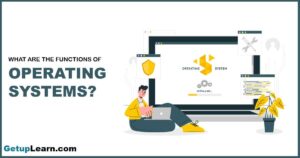
Advantages and Disadvantages of Flowcharts
What are c++ keywords set of 59 keywords in c ++, what is c++ programming language c++ character set, c++ tokens.
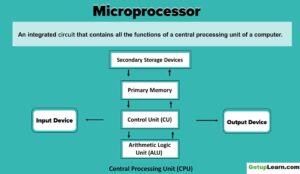
What is Computer System? Definition, Characteristics, Functional Units, Components
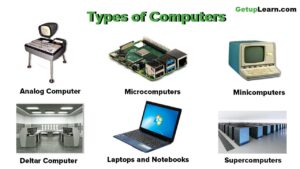
What is Big Data? Characteristics, Tools, Types, Internet of Things (IOT)
- Entrepreneurship
- Organizational Behavior
- Financial Management
- Communication
- Human Resource Management
- Sales Management
- Marketing Management
We use essential cookies to make Venngage work. By clicking “Accept All Cookies”, you agree to the storing of cookies on your device to enhance site navigation, analyze site usage, and assist in our marketing efforts.
Manage Cookies
Cookies and similar technologies collect certain information about how you’re using our website. Some of them are essential, and without them you wouldn’t be able to use Venngage. But others are optional, and you get to choose whether we use them or not.
Strictly Necessary Cookies
These cookies are always on, as they’re essential for making Venngage work, and making it safe. Without these cookies, services you’ve asked for can’t be provided.
Show cookie providers
- Google Login
Functionality Cookies
These cookies help us provide enhanced functionality and personalisation, and remember your settings. They may be set by us or by third party providers.
Performance Cookies
These cookies help us analyze how many people are using Venngage, where they come from and how they're using it. If you opt out of these cookies, we can’t get feedback to make Venngage better for you and all our users.
- Google Analytics
Targeting Cookies
These cookies are set by our advertising partners to track your activity and show you relevant Venngage ads on other sites as you browse the internet.
- Google Tag Manager
- Infographics
- Daily Infographics
- Graphic Design
- Graphs and Charts
- Data Visualization
- Human Resources
- Training and Development
- Beginner Guides
Blog Business
What is a Problem-Solving Flowchart & How to Make One
By Danesh Ramuthi , Aug 10, 2023
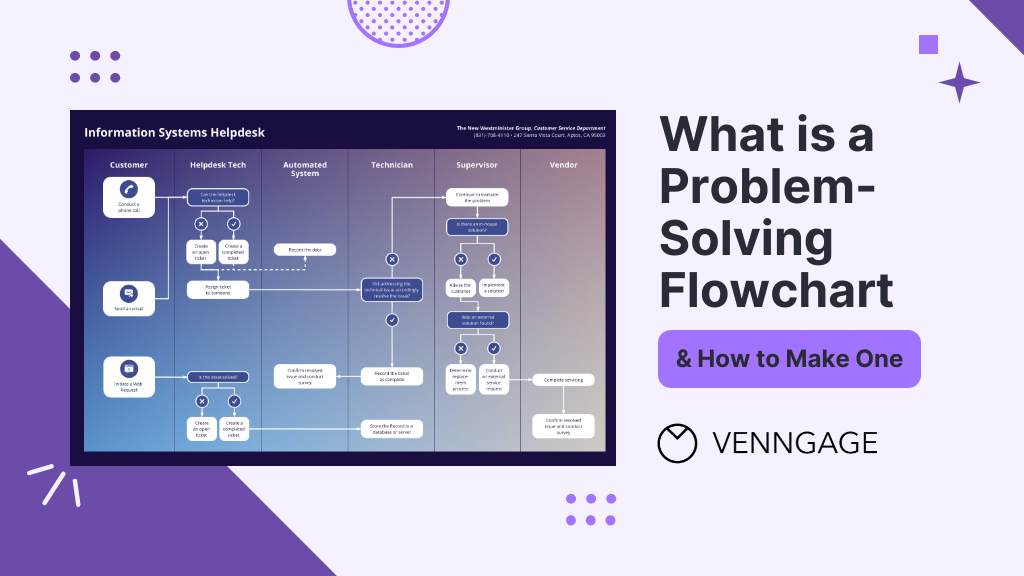
Problem-Solving Flowcharts, contrary to what many believe aren’t just aesthetic wonders — they’re almost like magical blueprints for troubleshooting those pesky problems that many of us face.
Flowcharts take business challenges and turn them into a navigable pathway. In this post, I will guide you on key aspects of problem-solving flowcharts such as what it is, the advantages of problem-solving flowcharts, how to create one and more.
Besides, you’ll also discover how to create problem-solving flowcharts with the help of Venngage’s Flowchart Maker.
And for those of you thinking, “I’m no designer, how can I create one?” worry not! I’ve got you covered. Just hop on Venggage’s Flowchart Templates and you’ll be charting your way to problem-solving glory in no time.
Click to jump ahead:
What are problem-solving flowcharts?
When to use problem-solving flowcharts, what are the advantages of flowcharts in problem-solving, what are the 7 steps of problem-solving flowcharts.
- 5 different types of problem-solving flowcharts
Best practices for designing effective problem-solving flowcharts
How to make a flowchart using venngage , problem-solving flowcharts faqs.
- Final Thoughts
Problem-Solving Flowcharts is a graphical representation used to break down problem or process into smaller, manageable parts, identify the root causes and outline a step-by-step solution.
It helps in visually organizing information and showing the relationships between various parts of the problem.
This type of flowcharts consists of different symbols and arrows, each representing different components or steps in the problem-solving process.
By following the flow of the chart, individuals or teams can methodically approach problem, analyze different aspects of it and come to a well-informed solution.
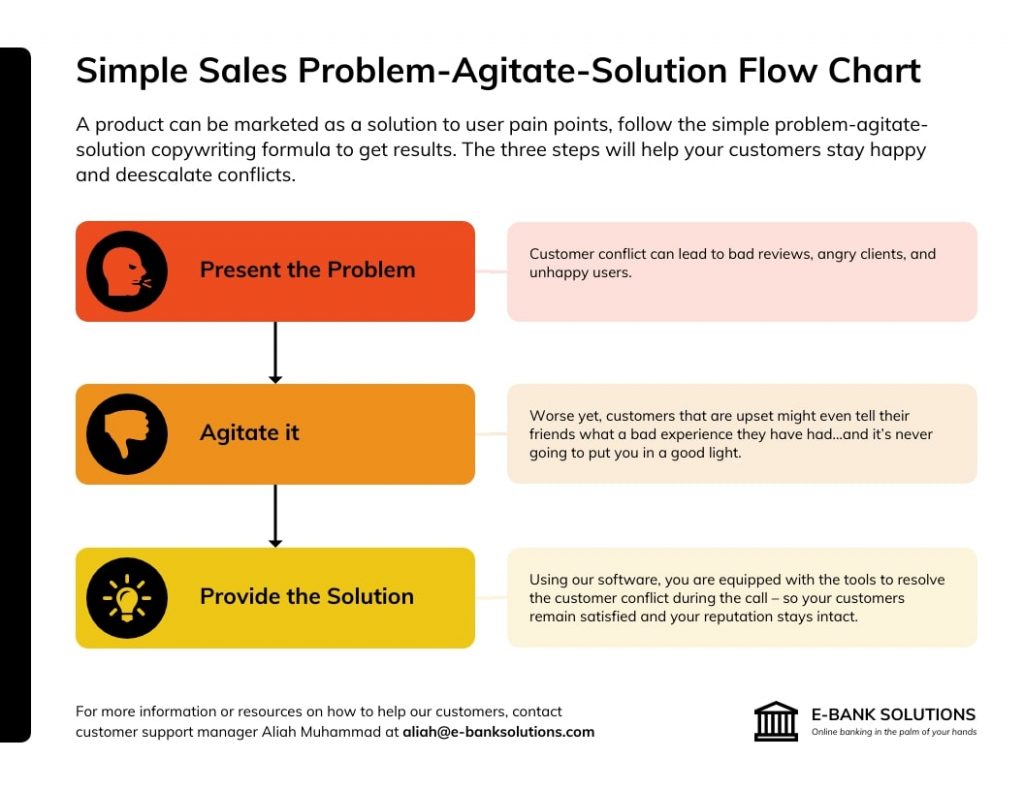
Problem-Solving Flowcharts is a versatile tool that can be used in various scenarios. Here’s when to consider utilizing one:
- Complex Problems: When faced with a multifaceted issue that involves multiple steps or variables, flowcharts can help break down the complexity into digestible parts.
- Team Collaboration: If you’re working with a team and need a common understanding of problem and its potential solutions then a flowchart provides a visual that everyone can refer to.
- Analyzing Processes: In a situation where you need to understand a particular process, whether it’s within a project or a part of regular operations then mapping it out in a flowchart can offer clarity.
- Decision Making: When various paths or decisions might be taken, a flowchart can outline the potential outcomes of each aiding in making an informed choice.
- Training and Onboarding: Flowcharts can be used in training materials to help new employees understand complex processes or procedures which makes the learning curve smoother.
- Identifying Root Causes: If you’re looking to identify the underlying causes of problem then a flowchart can facilitate a systematic approach to reaching the root of the issue.
Related: How to Use Fishbone Diagrams to Solve Complex Problems
Problem-solving flowcharts can offer several benefits to the users who are looking to solve a particular problem. Few advantages of flowcharts in problem solving are:
Visual Clarity
When you’re dealing with multifaceted problems or processes, words alone can make the situation seem even more tangled. Flowcharts distill these complexities into easily understandable visual elements.
By mapping out each phase or component of problem, flowcharts offer a bird’s eye view enabling individuals to grasp the bigger picture and the finer details simultaneously.
Sequential Representation
Flowcharts excel in laying out the sequence of events or actions. By indicating a clear starting point and illustrating each subsequent step, they guide users through a process or solution path methodically.
This linear representation ensures that no step is overlooked and each is executed in the right order.
Collaboration
Problem-solving often requires team effort and flowcharts are instrumental in fostering collaborative environments.
When a team is discussing potential solutions or trying to understand problem’s intricacies, a flowchart serves as a collective reference point.
It aids in synchronizing everyone’s understanding, minimizing miscommunications and promoting constructive discussions.
Read more about: Flowcharts Symbols and Meaning
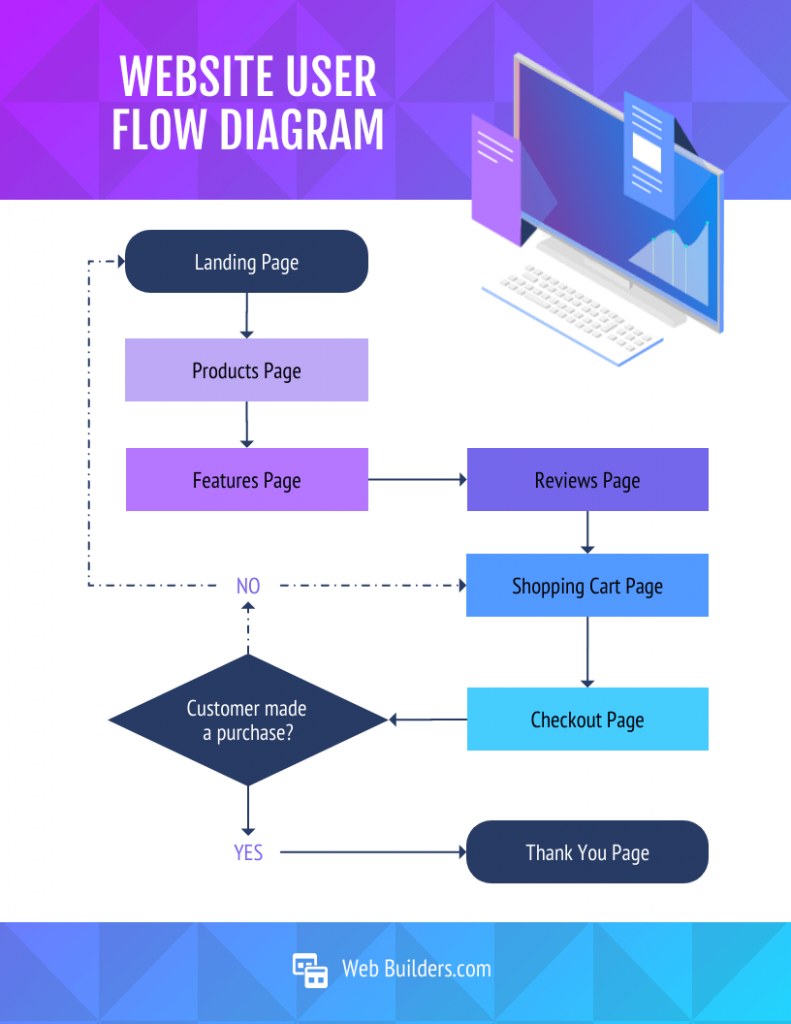
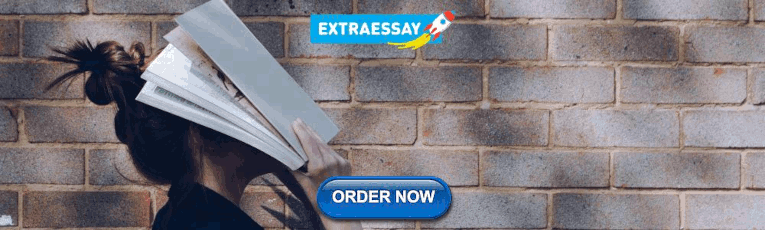
1. Define the Problem
Before anything else, it’s essential to articulate the problem or task you want to solve clearly and accurately. By understanding exactly what needs to be addressed you can ensure that subsequent steps align with the core issue.
2. Identify the Inputs and Outputs
Determine what inputs (such as data, information or resources) will be required to solve the problem and what the desired outputs or outcomes are. Identifying these factors will guide you in structuring the steps needed to reach the end goal and ensure that all necessary resources are at hand.
3. Identify the Main Steps
Break down the problem-solving process into its main steps or subtasks. This involves pinpointing the essential actions or stages necessary to reach the solution. Create a roadmap that helps in understanding how to approach the problem methodically.
4. Use Decision Symbols
In problem-solving, decisions often lead to different paths or outcomes. Using standard symbols to represent these decision points in the flowcharts allows for a clear understanding of these critical junctures. It helps visually present various scenarios and their consequences.
5. Add Descriptions and Details
A well-designed flowcharts is concise but clear in its labeling. Using arrows and short, descriptive phrases to explain what happens at each step or decision point ensures that the flowcharts communicates the process without unnecessary complexity.
6. Revise and Refine
Creating a flowcharts is not always a one-and-done process. It may require revisions to improve its clarity, accuracy or comprehensiveness. Necessary refinement ensures that the flowcharts precisely reflects the problem-solving process and is free from errors or ambiguities.
7. Use Flowchart Tool
While it’s possible to draw a flowcharts manually, using a flowcharts tool like Venngage’s Flowchart Maker and Venngage’s Flowchart Templates can make the process more efficient and flexible. These tools come with pre-designed templates and intuitive interfaces that make it easy to create, modify and share flowcharts.
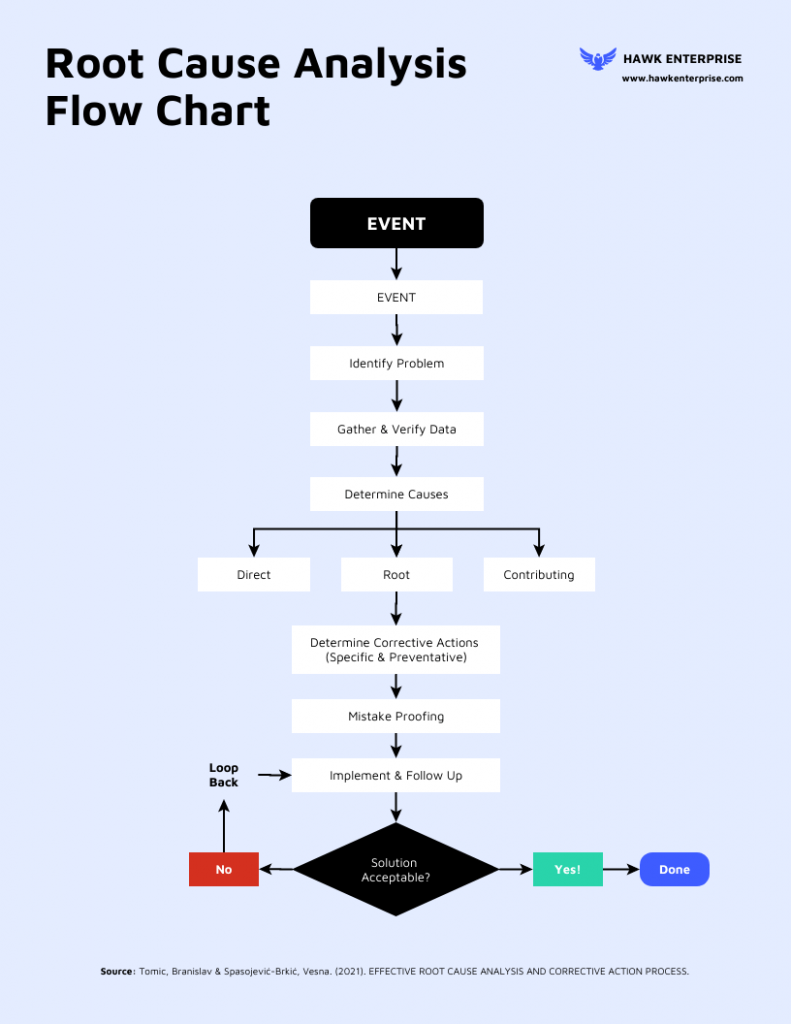
5 different types of problem-solving flowcharts
Let’s have a look at 5 most common types of flowcharts that individuals and organizations often use.
1. Process Flowchart s
A process flowcharts is a visual representation of the sequence of steps and decisions involved in executing a particular process or procedure.
It serves as a blueprint that showcases how different stages or functions are interconnected in a systematic flow and it highlights the direction of the process from its beginning to its end.
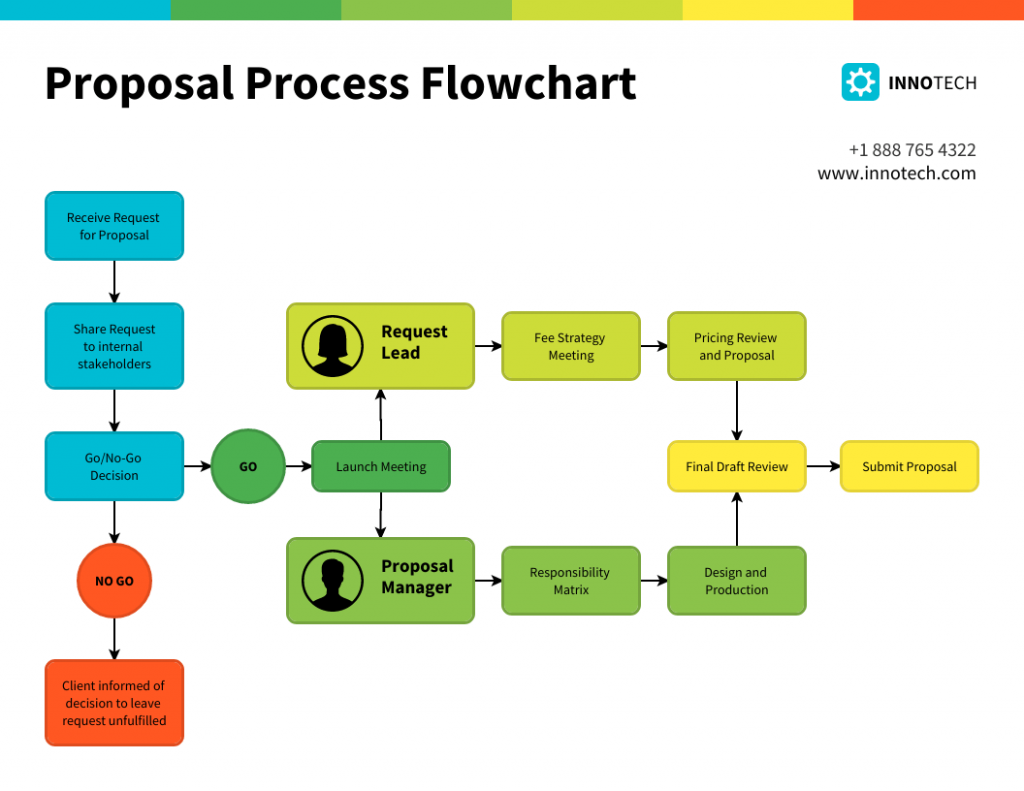
Process flowcharts are instrumental in training and onboarding, sales process , process optimization, documentation, recruitment and in any scenario where clear communication of a process is crucial.
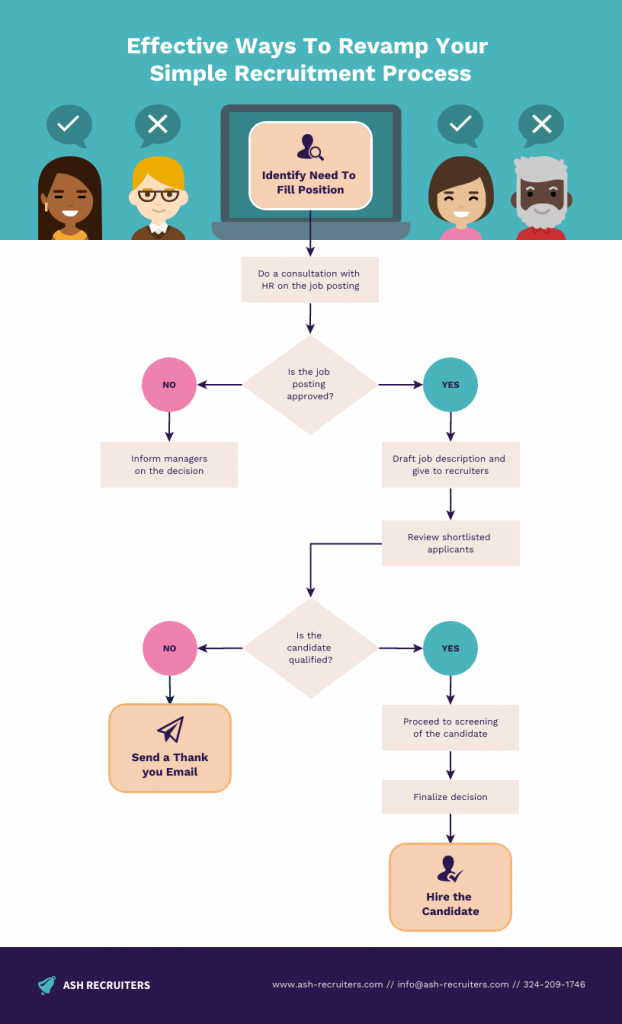
2. Flowcharts Infographic
A flowcharts infographic is a great way to showcase the process or a series of steps using a combination of graphics, icons, symbols and concise text. It aims to communicate complex information in a clear and easy-to-understand manner, making it a popular tool for conveying information, data and instructions in a visually engaging way.
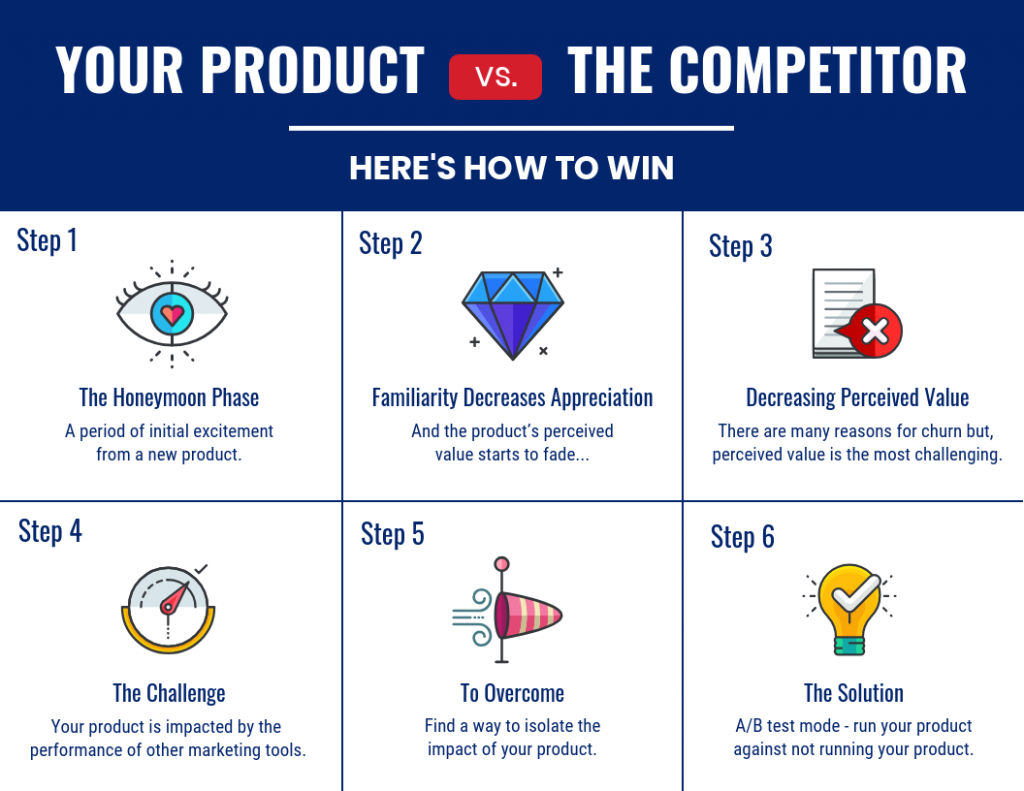
For example, you can use this flowchart to illustrate a health insurance process that visually explains the steps involved from finding a provider to paying for your healthcare provider.
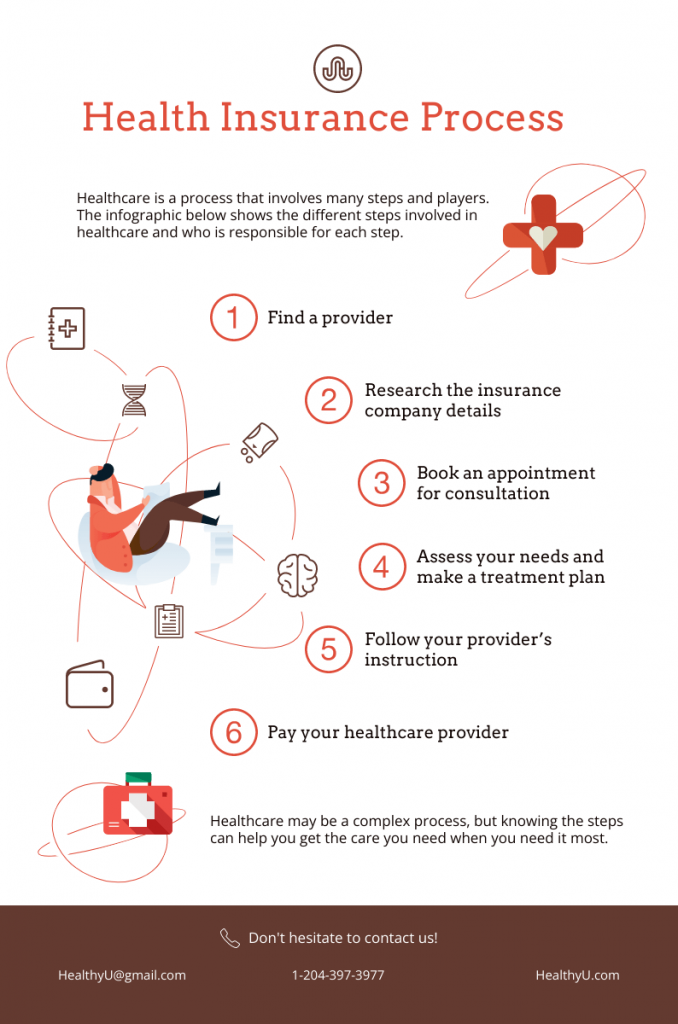
3. Circular Flowcharts
A circular flowcharts is used to illustrate the flow of information, goods, services or money within a closed system or process. It gets its name from its circular shape, which emphasizes the continuous and cyclical nature of the flow.
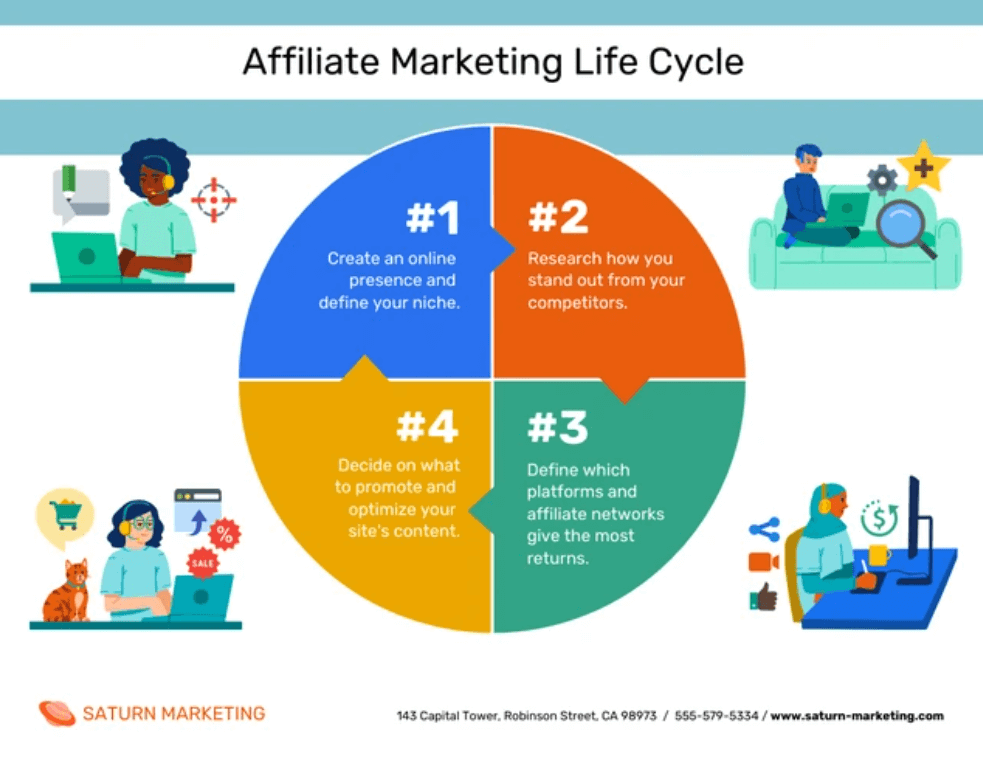
Circular flowcharts are widely used in various fields such as economics, business, engineering and process management to help visualize and understand complex systems.
In a circular flowcharts , elements are represented using various shapes and connected with arrows to indicate the direction of flow. The circular arrangement indicates that the process is ongoing and repeats itself over time.
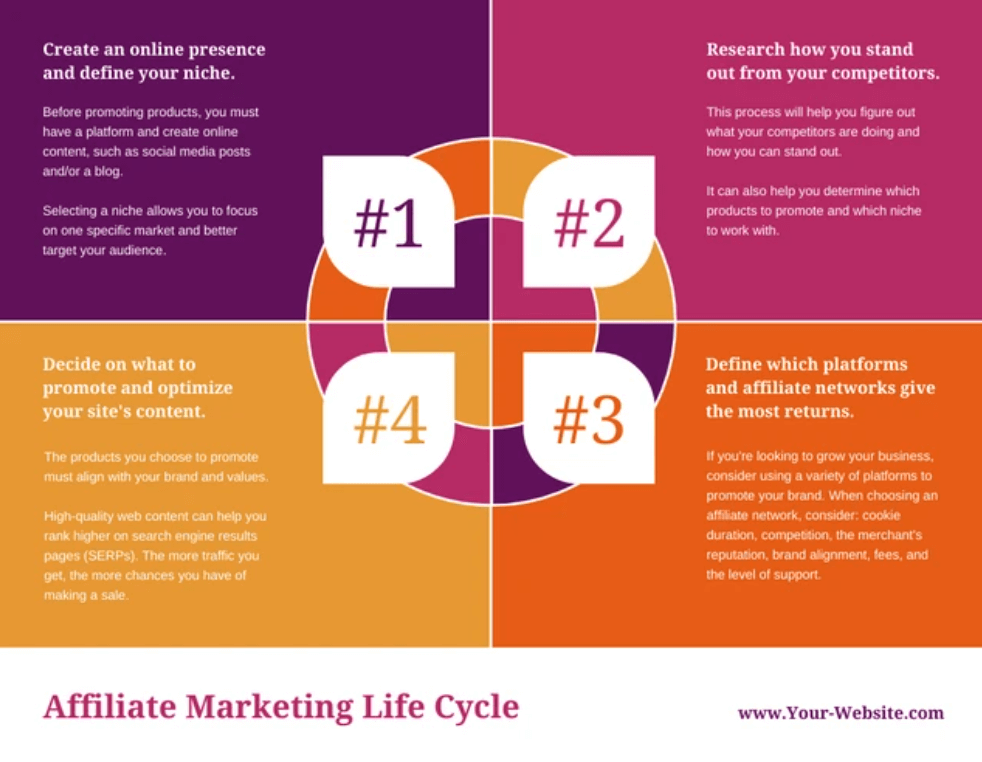
4. Swimlane flowcharts
Swimlane flowcharts , also known as cross-functional flowcharts are a specific type of flowchart that organizes the process flow into lanes or “swimlanes.”
Each lane represents a different participant or functional area involved in the process and the flowchart shows how activities or information move between these participants.
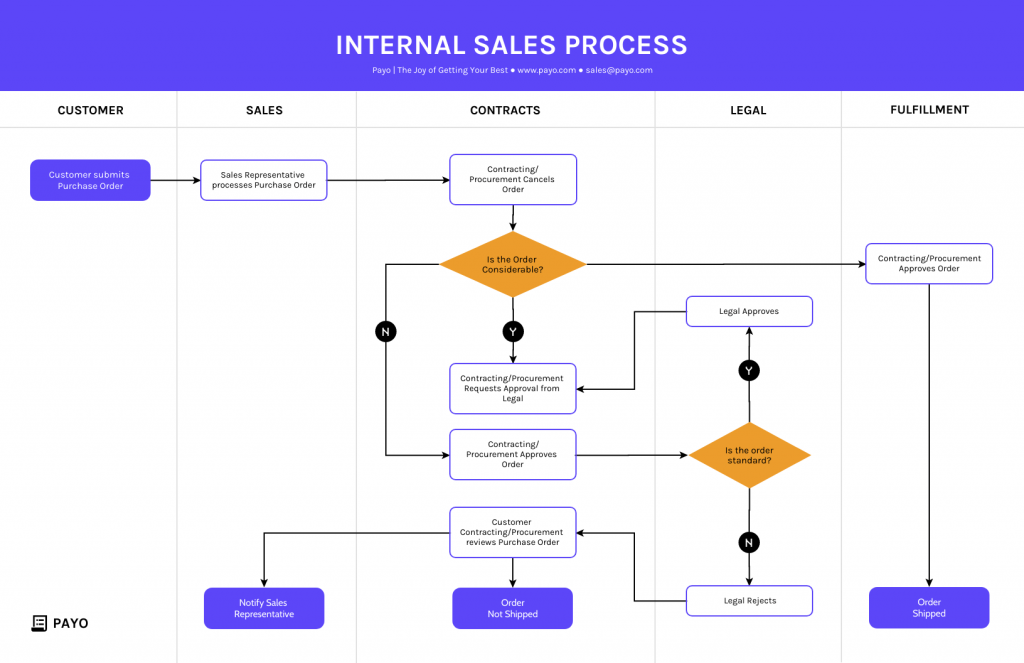
Swimlane flowcharts are particularly useful for illustrating complex processes that involve multiple stakeholders or departments.
In a swimlane flowcharts, the process is divided horizontally into lanes and each lane is labeled with the name of the department, person or role responsible for that part of the process. Vertically, the flowchart displays the sequence of steps or actions taken in the process.
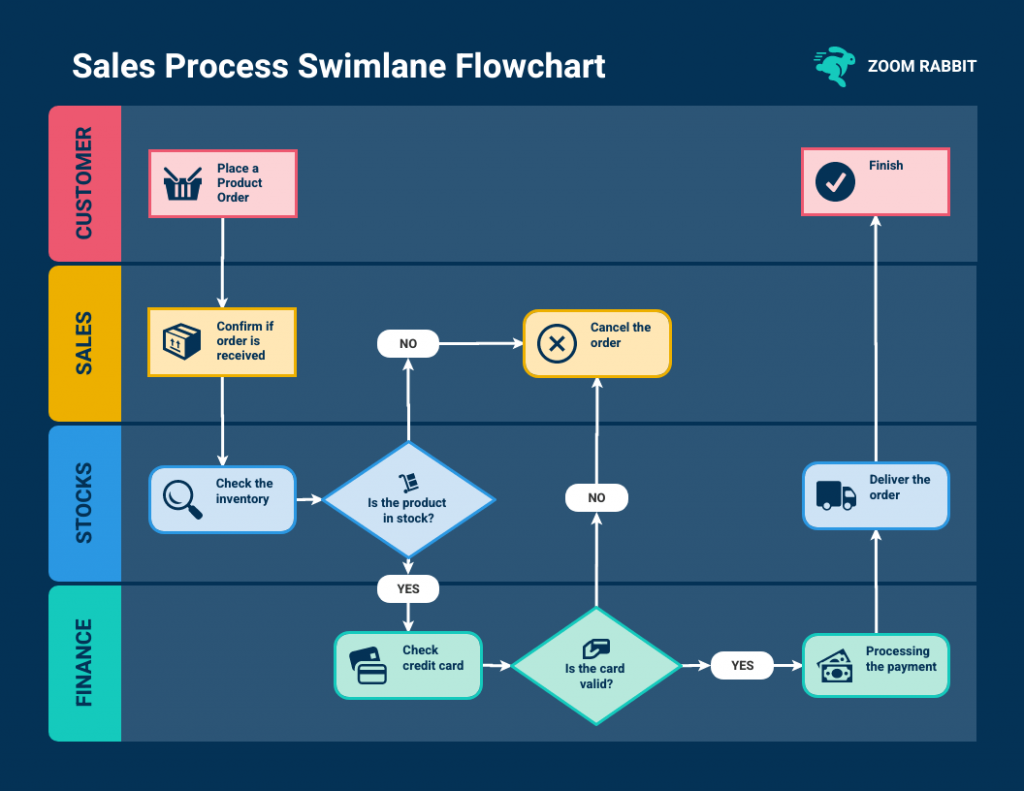
5. Decision Flowchart s
Decision flowcharts, also known as decision trees or flow diagrams are graphical representations that illustrate the process of making decisions or solving problems.
They are widely used in various fields such as computer science, business mapping , engineering and problem-solving scenarios.
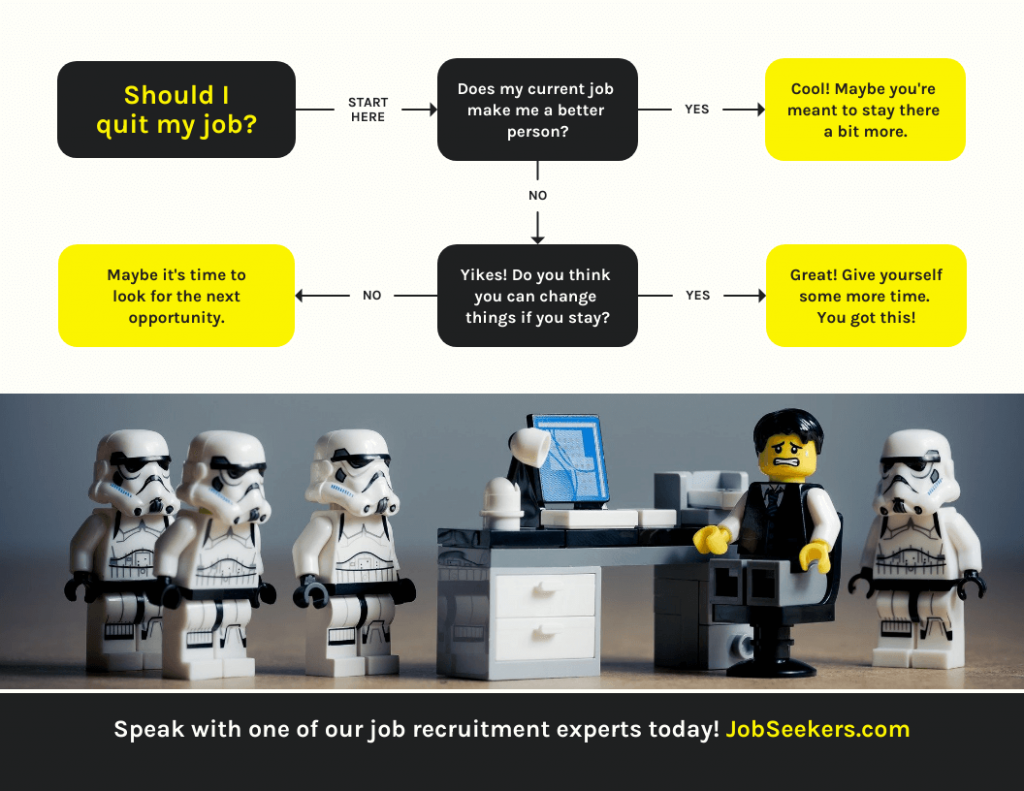
Decision flowcharts help break down complex decision-making processes into simple, sequential steps, making it easier to understand and follow.
A decision tree is a specialized flowchart used to visually represent the process of decision-making.
Businesses and other individuals can employ a decision tree analysis as a tool to aid in evaluating different options and the possible consequences associated with each choice.
Decision trees Infographics can be used to create a more nuanced type of flowchart that is more informative and visually appealing by combining a decision flowchart and the flowchart infographic.
Decision flowcharts are valuable tools for visualizing decision-making processes, analyzing complex problems and communicating them effectively to others.
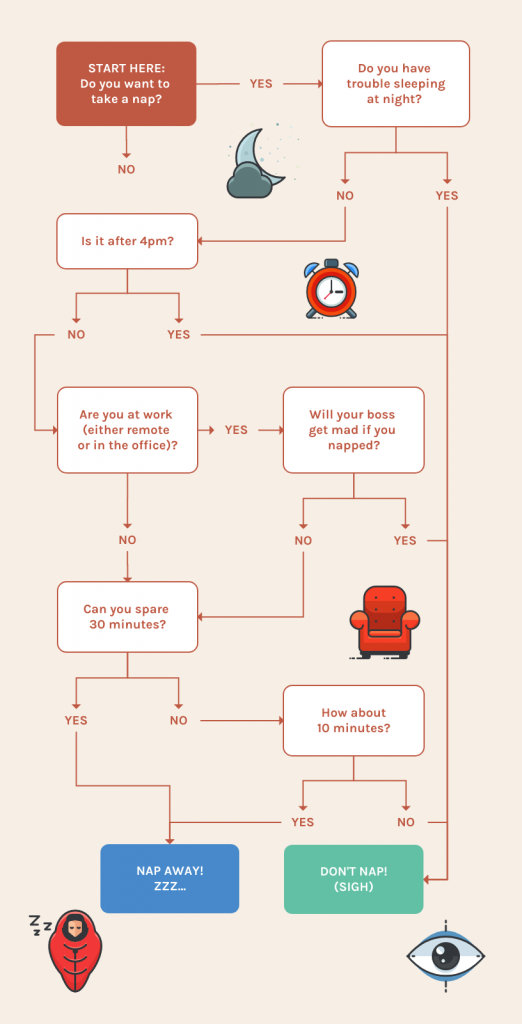
Designing effective problem-solving flowcharts involves careful consideration of various factors to ensure clarity, accuracy and usability. Here are some best practices to create efficient and useful problem-solving flowcharts:
- Understand the problem first & clearly define it
- Keep it simple
- Use standard & recognizable symbols
- Ensure that the flowchart follows a logical and sequential order
- Clearly label each decision point, action and outcome
- Verify the flowchart’s accuracy by testing it
- Clearly state the decision criteria that lead to different branches
- Provide context when the flowchart is part of a larger process or system
- Review and revise the flowchart
Creating problem-solving flowchart on Venngage is incredibly simple. All you have to do is:
- Start by Signing Up and Creating an Account with Venngage
- Choose a flowchart template that best suits your needs from our library.
- Start editing your flowchart by choosing the desired shapes, labels and colors.
- You can also enhance your flowchart by incorporating icons, illustrations or backgrounds all of which are readily available in our library.
- Once done, you will have 2 options to choose from, either sharing it online for free or downloading your flowchart to your desktop by subscribing to the Premium or Business Plan.
Is flowchart the representation of problem solutions?
Flowcharts are not the representation of problem solutions per se; rather, they are a visual representation of processes, decision-making steps and actions taken to arrive at a solution to problem.
What are the 3 basic structures of flowcharts?
3 Basic Structures of Flowcharts are:
- Sequence: Simplify Complexity
- Selection (Decision): Embrace Choices
- Repetition (Loop): Emphasize Iteration
What are the elements of a good flowchart?
A good flowchart should exhibit clarity and simplicity, using consistent symbols and labels to depict a logical sequence of steps. It should be readable, with appropriate white space to avoid clutter while eliminating ambiguity through well-defined decision criteria and paths.
Can flowcharts be used for both simple and complex problem-solving?
Yes, flowcharts can be used for both simple and complex problem-solving scenarios. Flowcharts are versatile visual tools that can effectively represent various processes, decision-making steps and problem-solving approaches regardless of their complexity.
In both cases, flowcharts offer a systematic and visual means of organizing information, identifying potential problems and facilitating collaboration among team members.
Can problem-solving flowcharts be used in any industry or domain?
Problem-solving flowcharts can be used in virtually any industry or domain. The versatility and effectiveness of flowcharts make them applicable to a wide range of fields such as Business and Management, Software Development and IT, Healthcare, Education, Finance, Marketing & Sales and a lot more other industries.
Final thoughts
Problem-solving flowcharts are a valuable and versatile tool that empowers individuals and teams to tackle complex problems with clarity and efficiency.
By visually representing the step-by-step process of identifying, analyzing and resolving issues, flowcharts serve as navigational guides simplifying intricate challenges into digestible parts.
With the aid of modern tools like Venngage’s Flowchart Maker and Venngage’s Flowchart Templates , designing impactful flowcharts becomes accessible to all while revolutionizing the way problems are approached and solved.
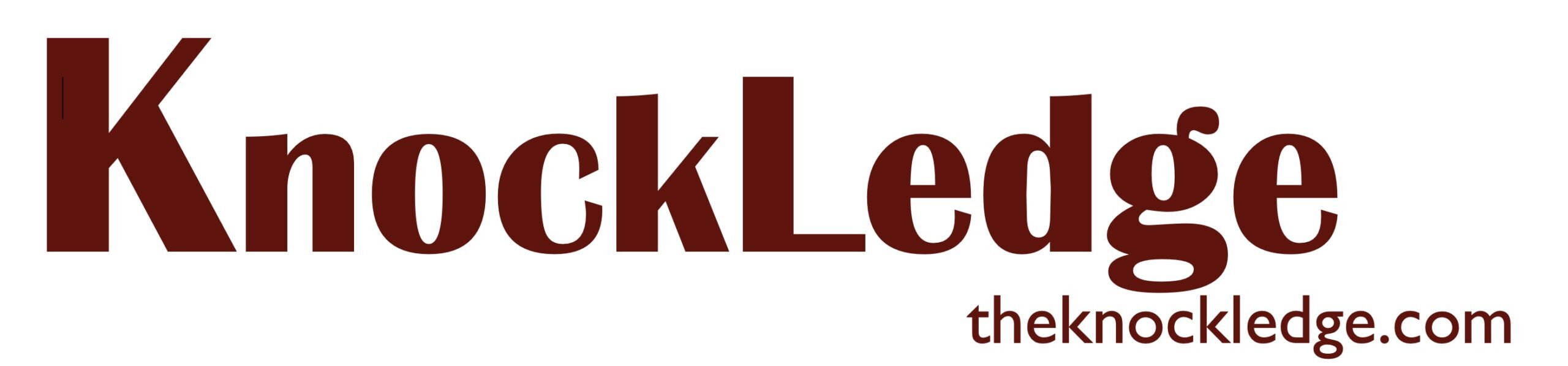
What are Algorithms and Flowcharts?
Hello Friends,
Welcome to the world of problem-solving with Algorithms and Flowcharts! These two essential tools are like a roadmap for computers, guiding them in making decisions and solving puzzles step by step. In this article, we’ll explore what Algorithms and Flowcharts are all about and how they help simplify complex tasks, making them accessible to both machines and humans. So, let’s dive into the fascinating world of Algorithms and Flowcharts to unravel their significance in problem-solving.
WHAT ARE ALGORITHMS?
- It is a series of instructions to perform a task.
- It is the step-by-step solution (process/procedure).
How to solve any problem?
- Know the problem
- Analyse the problem
- Find the best solution, i.e. the easiest solution having less steps, precise and unique.
Finally, algorithm is a step-by-step process to obtain a solution for a specific task when executed. The most efficient algorithm is chosen based on the solution, the solving time and the amount of space used by an algorithm.
Advantages of algorithm
- Doesn’t use programming terminology, so easy to understand
- If any error, it can easily be identified and solved
- Independent of any programming language, and everyone is eligible to make an algorithm
Disadvantages of algorithm
- Solutions of complex problems cannot be made an algorithm easily
- Time consuming
- Difficult to explain loop concepts (repetition)
Criteria of algorithm
- The steps should be in sequence.
- Steps can include mathematical expressions.
- Variable can be used (to store values of input, intermediate and final result).
- The words ‘read’ and ‘print’ are ideally used in place of input and output.
- Any step can be repeated any number of times; or the number of repetitions can be specified to reduce the number of steps.
- Decision is made using a condition, using if-else statements .
- Output can be numerical, Boolean, a line of text, etc.
- The best solution must be selected from the number of solutions to a given problem, and should be stated as an algorithm.
Steps to develop an algorithm
- Problem description – The problem to be solved must be easy to understand.
- Analyse the problem – Decide the type of input, output and problem by listing the characteristics (decide whether: the solution is numerical, etc; the solution is a single value or multiple value; the problem requires a formula or not).
- Develop algorithm (process of solving) – Write the step-by-step solution.
- Test the algorithm – check each step and the final output and determine it has performed the intended operation.
- Review the algorithm – checking for changes, more simplifications, solves problem based on the analysed problem, etc.
CHARACTERISTICS OF ALGORITHMS – IODFU
- I – Input – There can be zero or more quantities as input.
- O – Output – There should be at least one output .
- D – Definiteness – Each instruction should be clear and easy to understand .
- F – Finiteness – There should be finite number of steps producing an output.
- U – Unique – Each step should produce a unique result depending upon the input and the previous steps.
Pseudocode: a notation similar to any programming language but does not involve the complicated syntax of that programming language. Instead, structural conventions for the same are used (no machine-readable code, therefore easy to write and understand).
- HISTORY OF COMPUTERS | KnockLedge
- File Management | Organization of Data
- State legislative assemblies of India
EXAMPLES OF ALGORITHM
Write an algorithm to prepare maggi..
1. Take maggi, bowl, water and salt.
2. Take water in a bowl, add maggi and boil it for 2-5 minutes.
3. Add spices or vegetables according to your taste.
4. Cook it for 4-5 minutes.
5. Your maggi is ready!
Write an algorithm to add 2 numbers.
1. Take two numbers (a, b).
2. Calculate the sum (c = a + b).
3. Print the sum (c).
Write an algorithm to calculate the simple interest.
1. Input Principal, Rate and Time (P, R, T).
2. Calculate the simple interest ·(SI = (P*R*T)/100).
3. Print the Simple Interest (SI).
Write an algorithm to check whether a number is positive on negative.
1. Input a number (n).
2. Check if, n>0 If true, print “Positive no.” If false, print “Negative no.”
3. If n=0, print “zero”.
Write an algorithm to calculate the average of 3 numbers.
1. Input three numbers (a, b, c).
2. Calculate the average (Avg = (a+b+c)/3).
3. Print the average (Avg).
Write an algorithm to calculate and print the area and circumference of a circle.
1. Input the radius of a circle.
2. Calculate the area of the circle (A = 3.14*r*r).
3. Print Area (A).
4. Calculate the circumference of a circle (C = 2* 3.14* r).
5. Print the circumference (C).
Write an algorithm to input a number and check whether it is even or odd.
2. Check if, n%2=0 If true, print “Even no.” If false, print “Odd no.”
Write an algorithm to input three numbers and print the largest one.
1. Input three numbers (a, b, c).
2. Check if, a>b and a>c
If true, print a.. If false, go to step 3.
3. Check if, b>c and b>a.
If true, print b. If false, print c.
Write an algorithm to check whether a number is Buzz or not.
INFO: BUZZ No. ends with 7, e.g. 797 or BUZZ no is divisible by 7, e.g. 70.
1. Read a number (n).
2. Check if, n%7=0 or n%10=7
3. If true, print n is a buzz no. If false, print n is not a buzz no.
WHAT ARE FLOWCHARTS?
- It is the graphical representation of an algorithm.
- Without algorithm, a flowchart cannot exist.
Finally, a flowchart is a pictorial (graphical) representation of problem-solving process. It gives a step-by-step procedure for the solution to a problem.
What are the elements of flowchart?
- Geometrical shapes (ellipse, circle, parallelogram, square, rhombus)
- Directional arrows (up, down, right, left arrows)
Uses of flowchart
- Graphical communication to get the solution and ideas
- Plan the sequence
- Used as a method to solve a problem
Advantages of flowchart
- Logics can be easily understood
- Easy to understand being pictorial
- Errors can be found in no time
Disadvantages of flowchart
- No scope for modifications
- Logic cannot be applied on computer directly
MEANING OF THE SYMBOLS USED IN FLOWCHART
- Ellipse : To begin or start or end or stop the flowchart
- Parallelogram : To read (input) data and print (output) data
- Rectangle : Denotes the processing that takes place in flowchart
- Diamond : It contains a question for which the decision is made. It either gives the answer in ‘Yes’ or ‘No’.
- Arrows : Provides a sequence (flow) to the problem-solving process by connecting the steps.
- Circle : They are connectors that jump directly from one point to another to avoid crosslines between steps. They are denoted using capital letters .
- Pentagon : They are off-page connectors which connect the same flowchart that extends beyond a page. They are denoted using digits .
EXAMPLES OF FLOWCHARTS
Draw a flowchart to find the average of 3 numbers., create a flowchart to input two numbers and print the larger one., draw a flowchart to input a no. and check whether it is positive. if positive, check whether it is even or odd., anju wants to create a flowchart by entering a positive number and she wants to check whether the no. is single digit, double digit or triple digit. draw a flowchart for the same..
To wrap up, algorithms and flowcharts are two distinct tools in problem-solving. Algorithms are step-by-step instructions, while flowcharts are visual diagrams. Understanding these differences will help you in various fields, from programming to everyday problem-solving. Keep exploring, and happy learning! Thank you for reading! 😊
Thank you, dear readers for visiting our website theknockledge.com , and reading our article. Your support and interest mean a lot to us. I appreciate your time and hope you find value in my other articles too. Keep coming back for more knowledge and insights!
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Algorithm Flowcharts Examples
Edraw content team, do you want to make your algorithm flowchart .
EdrawMax specializes in diagramming and visualizing. Learn from this article to know everything about algorithm flowchart examples and templates , and how to use them. Just try it free now!
Algorithms and flowcharts are two different ways of presenting the process of solving a problem. Algorithms consist of steps for solving a particular problem, while in flowcharts, those steps are usually displayed in shapes and process boxes with arrows. So flowcharts can be used for presenting algorithms. EdrawMax will introduce some examples of algorithm flowcharts .
1. Rules of Drawing Flowcharts for Algorithms
Flowcharts usually consist of basic symbols and shapes that have a specific representation. The symbols make the chart more meaningful and easy to understand.
Some of the most standard symbols include shapes like a rectangle representing an operation, a parallelogram showing input and output of data, a cylinder indicating the start/end, while a diamond represents a decision. Read more about flowchart symbols .
However, knowing these symbols well and using them in a flowchart involves critical thinking. Here are some basic rules that need to be considered while drawing a flowchart.
- All the boxes and shapes in a flowchart should be connected with arrows to indicate a logical order and make it easy to follow.
- Make sure the flow direction is consistent and not ambiguous. The flow charts always flow from either left to right or top to bottom.
- Every chart will begin with a Start box and end with a Terminal box, with the latter only having one flow line associated with it.
- All the shapes, symbols, and lines should be consistent. You can also add a symbol key to make it easier to follow the chart.
2. Free Algorithm Flowchart Examples & Templates
There are many algorithm flowchart examples provided for you, you can click the table of contents to get them soon.

Examples List
- Find the Sum of Two Numbers Entered
- Determining the Largest Number Among All the Entered Integers
- Work Out All the Roots of a Quadratic Equation ax² +bx+c=0
- Find the Fibonacci series till term ≤ 1000
- Calculate the Sum of The First 50 Numbers
- Find the largest price among 100 given values and reduce it by 10%
- Arrange the numbers X, Y, Z in descending order
- Determine the Highest Common Factor (HCF) of two integers
- Determine and Output Whether Number N is Even or Odd
- Calculate the Interest of a Bank Deposit
- Determine Whether a Temperature is Below or Above the Freezing Point
- Determine Whether A Student Passed the Exam or Not
Example 1: Find the Sum of Two Numbers Entered
- Step 1: Read the Integer A.
- Step 2: Read Integer B.
- Step 3: Perform the addition by using the formula: C= A + B.
- Step 4: Print the Integer C.
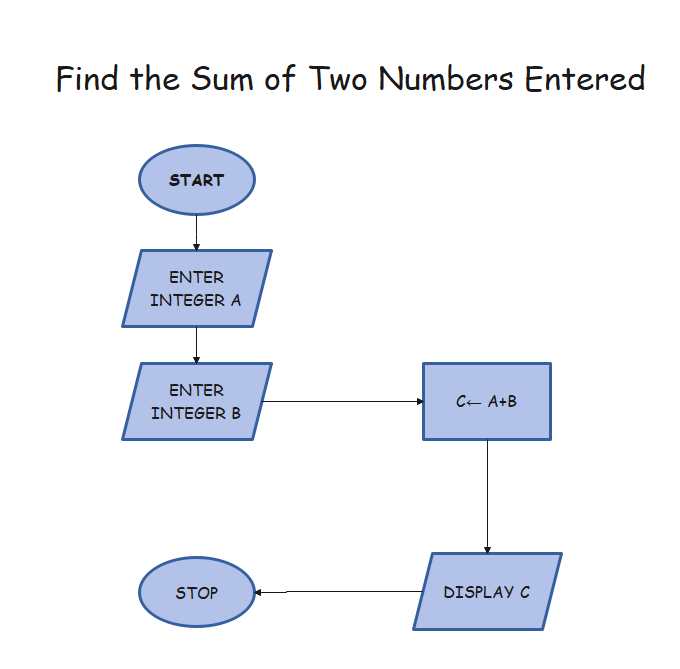
Example 2: Determining the Largest Number Among All the Entered Integers
- Step 3: If B is greater than A, then print B, else A.
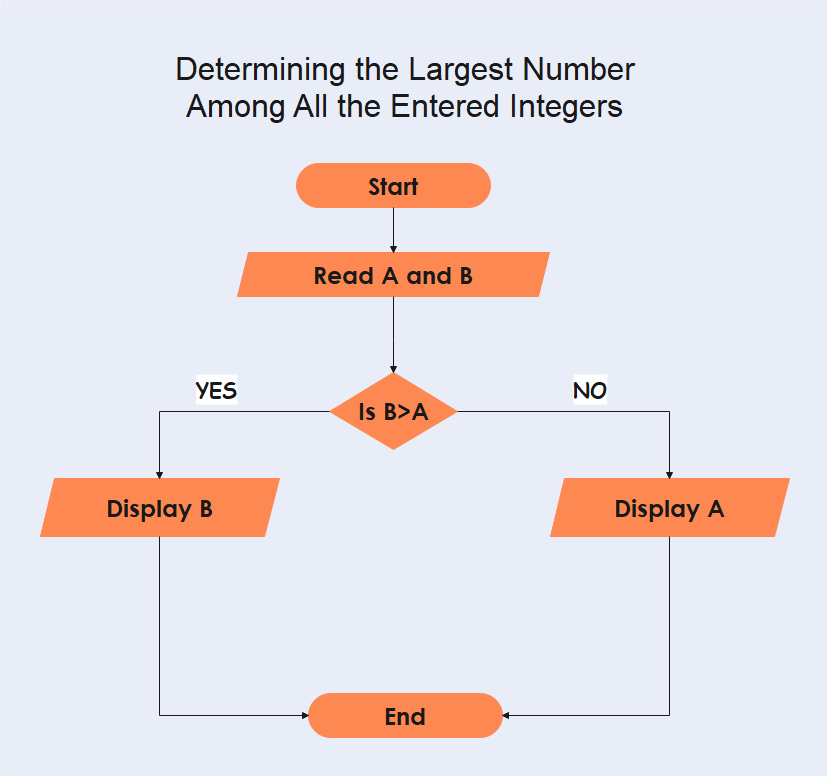
Example 3: Work Out All the Roots of a Quadratic Equation ax² +bx+c=0
- Step 1: Enter the variables a, b, c, D, x1, x2, rp, and ip.
- Step 2: Evaluate the discriminant by using the formula: D= b²- 4ac
- Step 3: Print rp and ip.
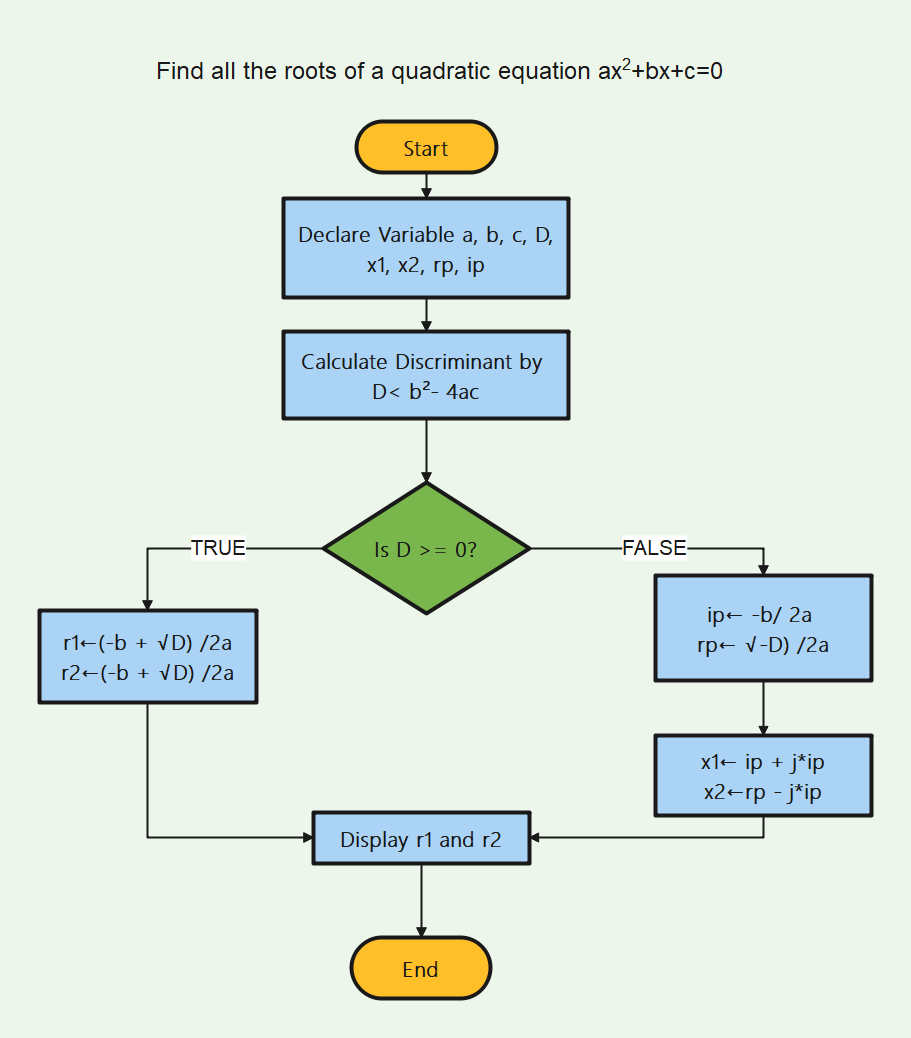
Example 4: Find the Fibonacci series till term ≤ 1000
- Step 1: Declare the variables i, a, b, show.
- Step 2: Enter the values for the variables, a=0, b=1, show=0
- Step 3: Enter the terms of the Fibonacci series to be printed, i.e=, 1000.
- Step 4: Print the first two terms of the series.
- Step 5: Loop the following steps:
- Show = a + b
- Add 1 to the value of i each time.
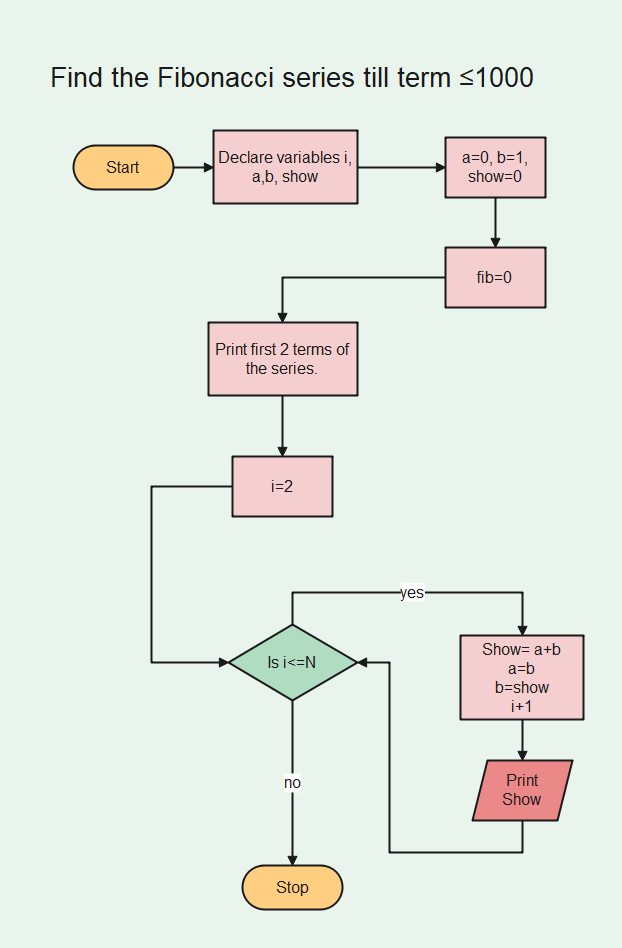
Example 5: Calculate the Sum of The First 50 Numbers
- Step 1: Declare number N= 0 and sum= 0
- Step 2: Determine N by N= N+1
- Step 3: Calculate the sum by the formula: Sum= N + Sum.
- Step 4: Add a loop between steps 2 and 3 until N= 50.
- Step 5: Print Sum.
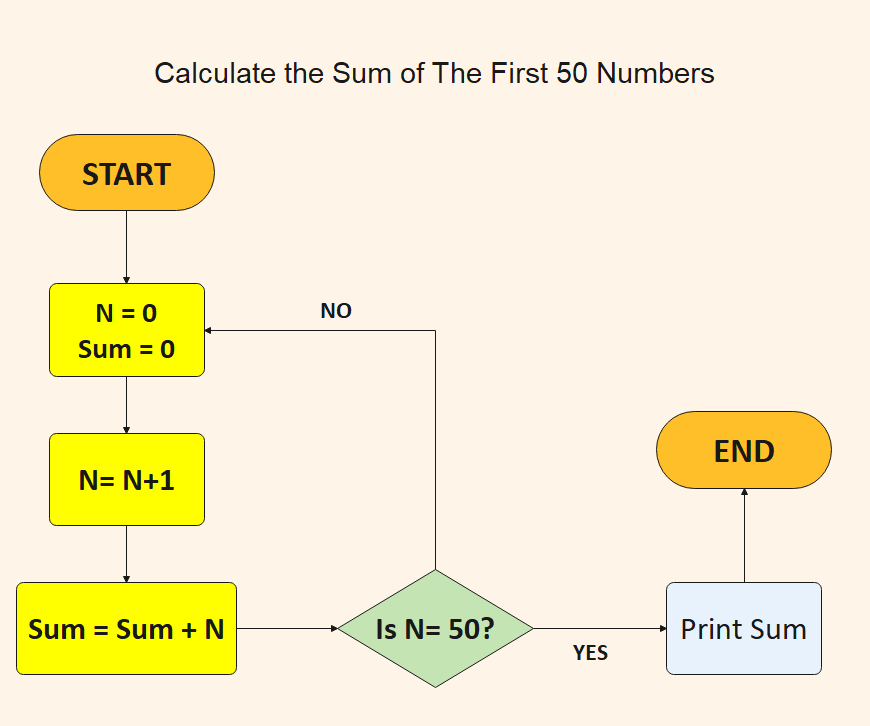
Example 6: Find the largest price among 100 given values and reduce it by 10%
- Step 1: Read the 100 prices.
- Step 2: Compare the first price with the next and let the greater of the two be 'max' in the 'max index.
- Step 3: Loop it until the largest price has been found.
- Step 4: Reduce the 'max' value by 10% using the formula: prices [max index] = prices [max index] x 0.9.
- Step 5: Print.
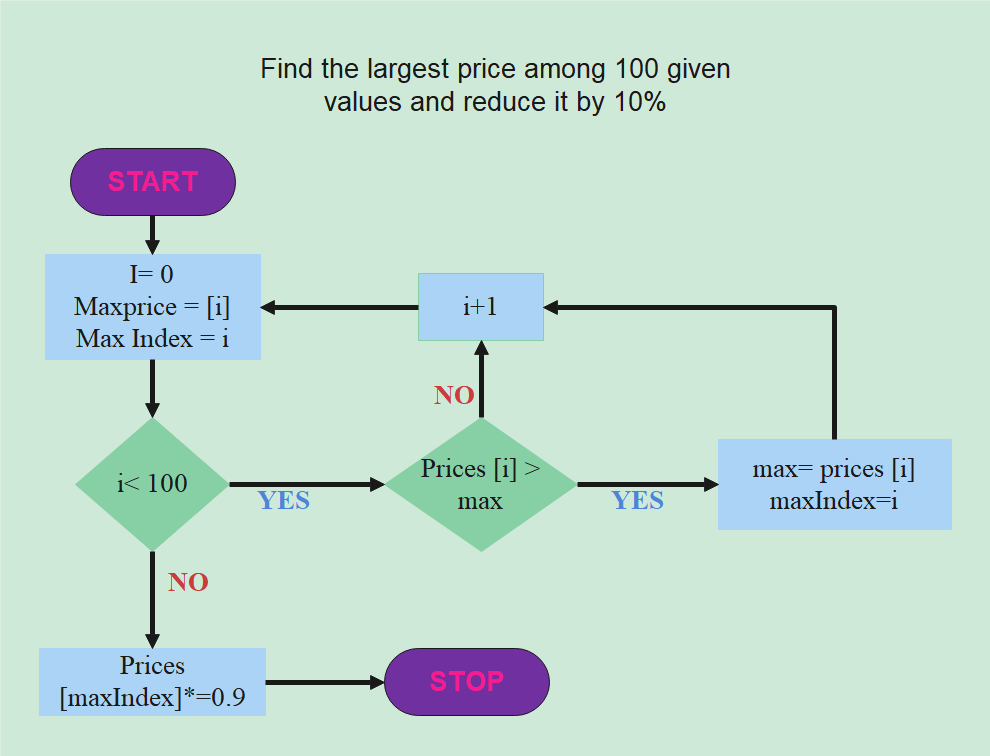
Example 7: Arrange the numbers X, Y, Z in descending order
- Step 1: Input the value for variables X, Y, Z.
- Step 2: Read the integers X, Y, Z.
- Step 3: Check if x>y, x>z, y>z
- Step 4: Print the possible order.
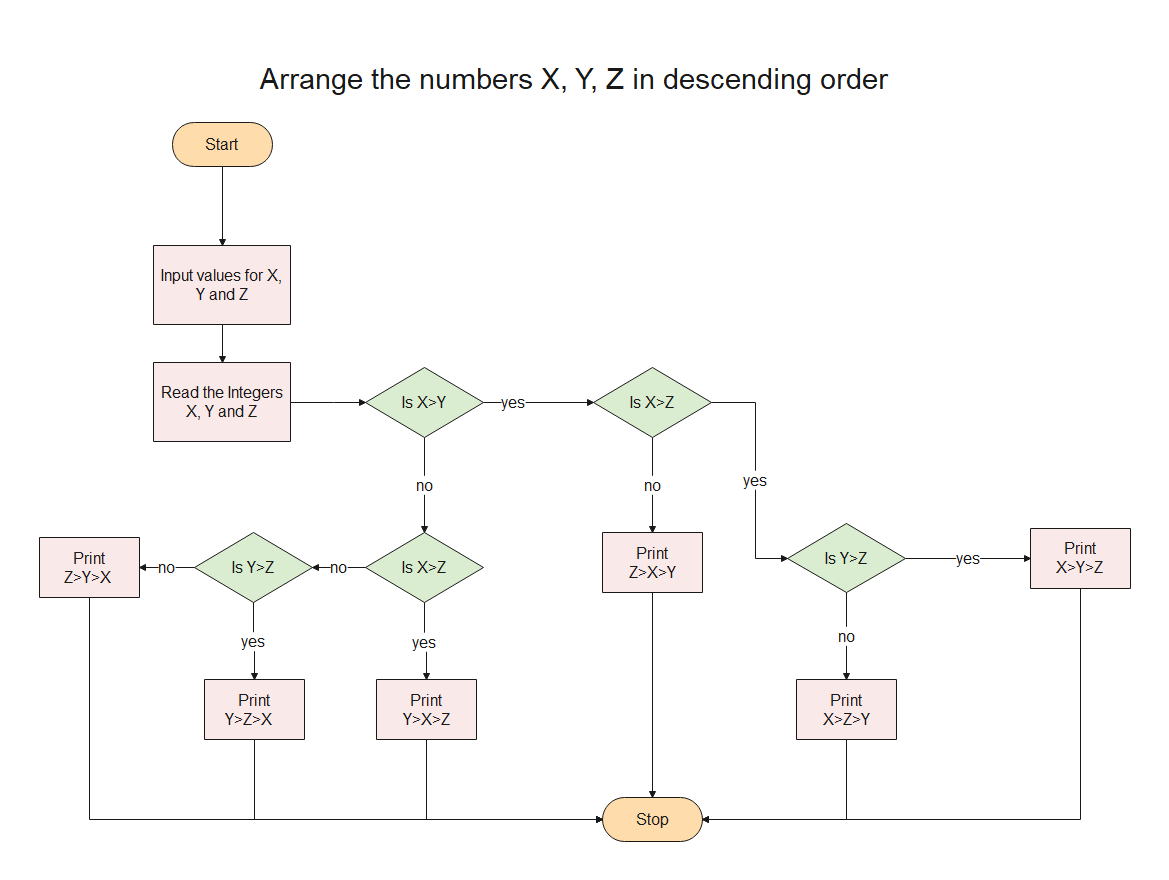
Example 8: Determine the Highest Common Factor (HCF) of two integers
- Step 1: Read the variables a and b.
- Step 2: If a = b, go to step 4.
- Step 3: If a > b, then: a = a - b. Return to step 2.
- Step 4: Print a or b.
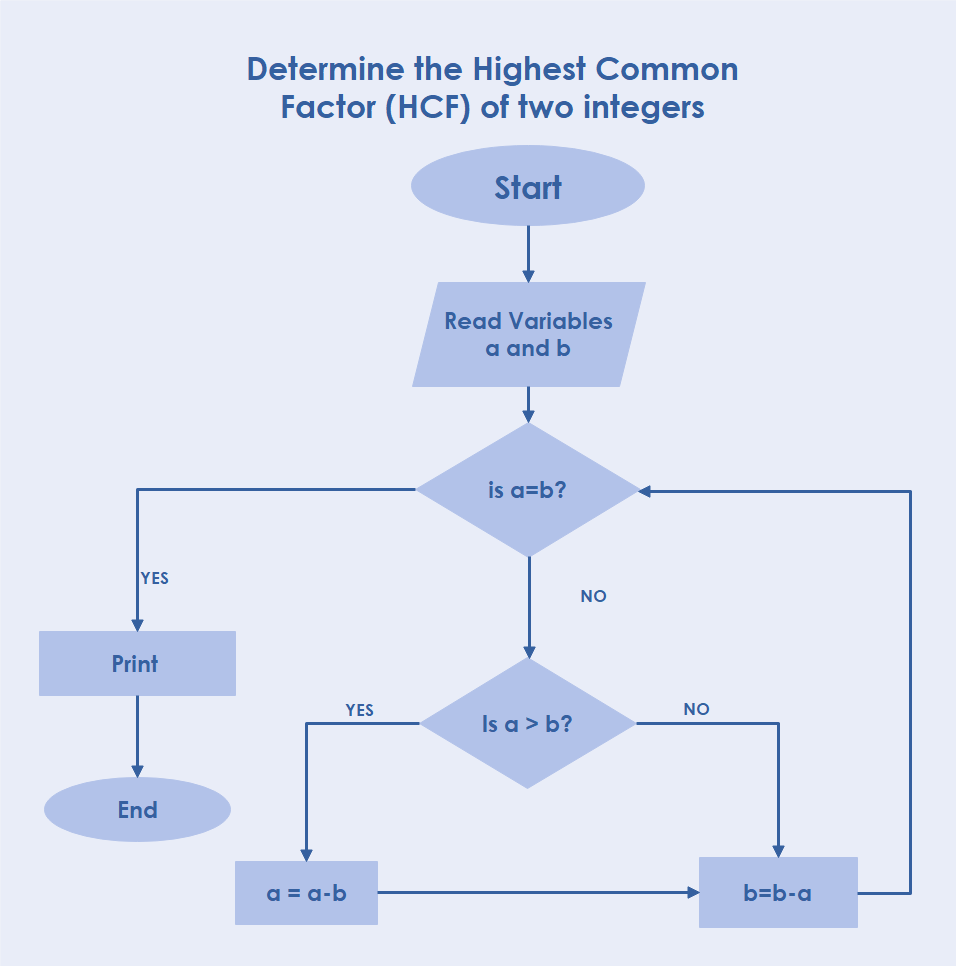
Example 9: Determine and Output Whether Number N is Even or Odd
- Step 1: Read number N.
- Step 2: Set remainder as N modulo 2.
- Step 3: If the remainder is equal to 0, then number N is even, else number N is odd.
- Step 4: Print output.
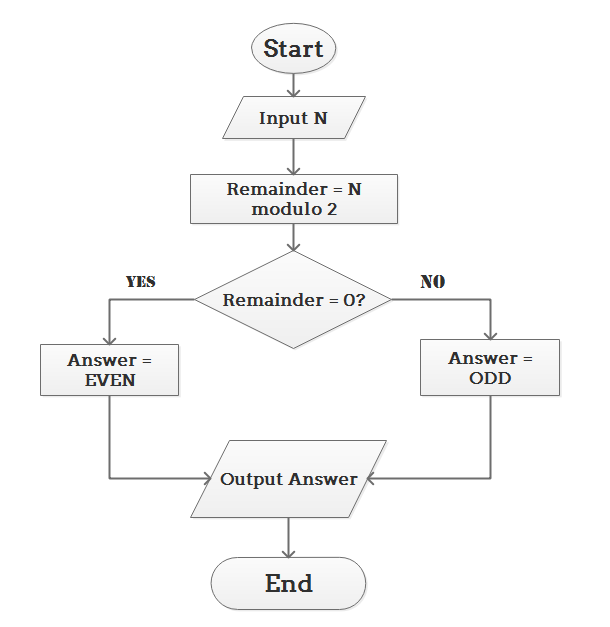
Example 10: Calculate the Interest of a Bank Deposit
- Step 1: Read amount.
- Step 2: Read years.
- Step 3: Read rate.
- Step 4: Calculate the interest with the formula "Interest=Amount*Years*Rate/100.
- Step 5: Print interest.
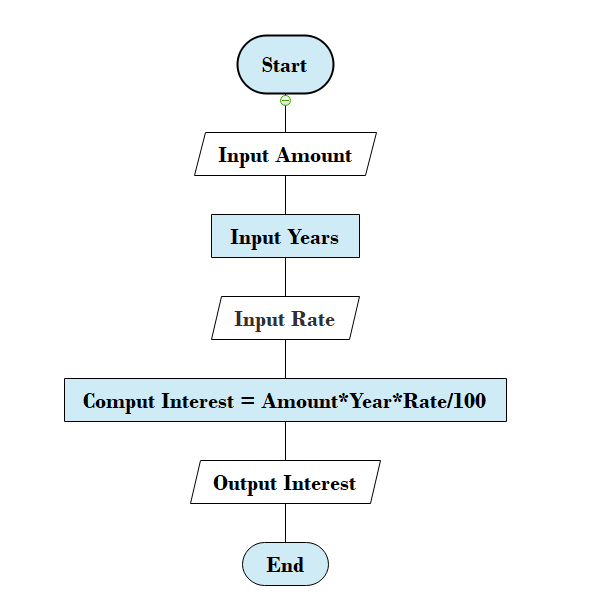
Example 11: Determine Whether a Temperature is Below or Above the Freezing Point
- Step 1: Input temperature.
- Step 2: If it is less than 32, then print "below freezing point", otherwise print "above freezing point".
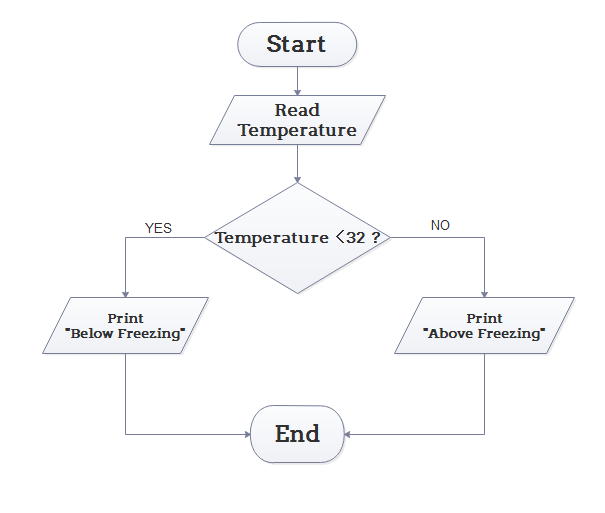
Example 12: Determine Whether A Student Passed the Exam or Not
- Step 1: Input grades of 4 courses M1, M2, M3 and M4.
- Step 2: Calculate the average grade with the formula "Grade=(M1+M2+M3+M4)/4".
- Step 3: If the average grade is less than 60, print "FAIL", else print "PASS".

3. How to Create a Flowchart for Algorithm?
EdrawMax is vector-based software that provides various templates for your algorithm flowchart. It provides an easily understandable interface for its users to draw efficiently and helps users edit existing templates and layouts and make their designs or template. It also provides 26000+ symbols to illustrate your algorithm flowchart. You can learn more about algorithm flowchart in details from this algorithm flowchart guide .
Step1 Install EdrawMax
You can edit the templates directly from this page. First of all, when your mouse click to the templates on this page, you can edit online easily, or you can download EdrawMax here.
Step2 Download the Template
After installing EdrawMax, you can go and download the template by clicking on the bottom right corner or look for a template in the EdrawMax library without going online in desktop.
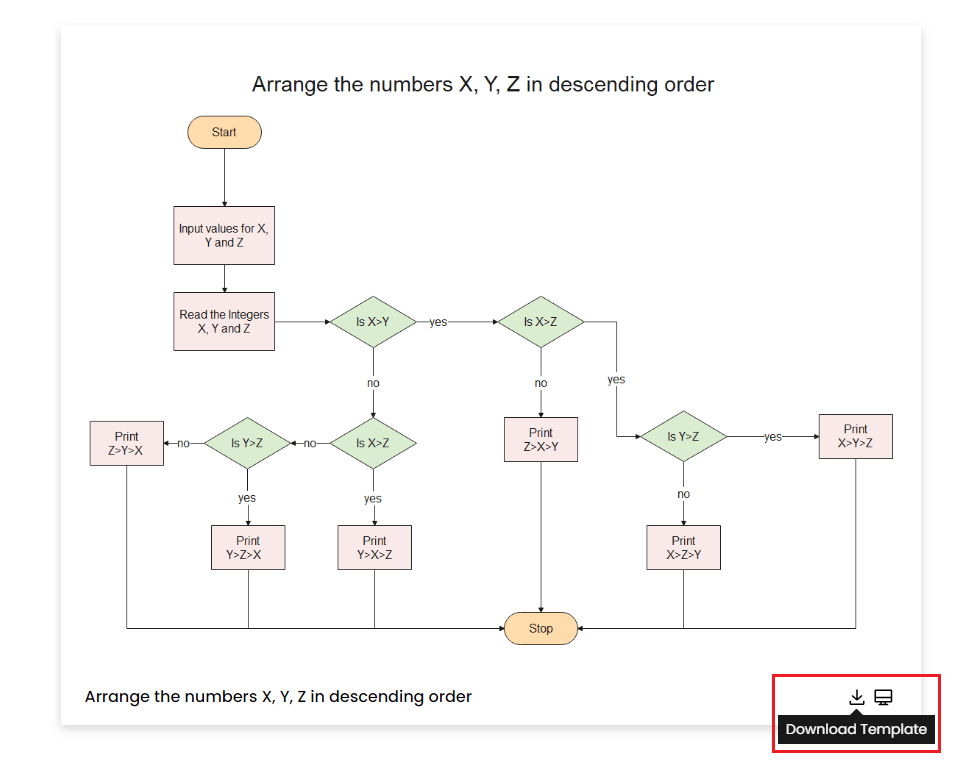
Step3 Open the Template
After downloading the template, you can double click to open it in EdrawMax diagramming software, or you can open EdrawMax beforehand and navigate to the template to open it. Every algorithm flowchart diagram is incomplete without symbols. Head to the 'Symbols' section and click on the 'algorithm flowchart ' and 'Algorithm Flowchart' to import important symbols.
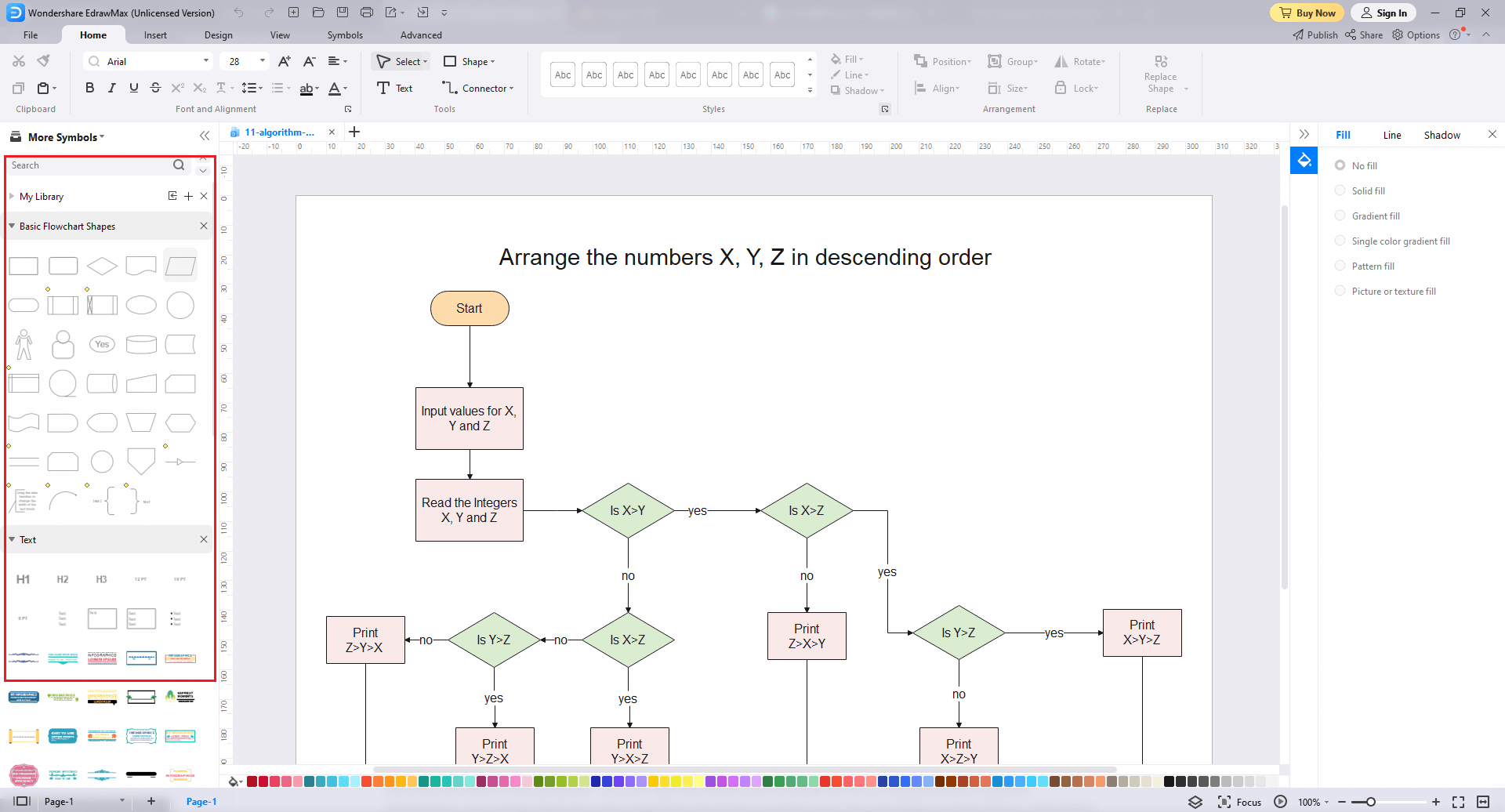
Step4 Customize the Algorithm Flowchart Template
After opening it, the next step is to customize and edit the algorithm flowchart template. EdrawMax gives you various unique diagramming tools that help you edit the template any way you want. You can change the color and the font liner of the template. You can edit the layout and include the list of requirements, measure dimensions, and render features using algorithm flowchart symbols from the EdrawMax symbol library. EdrawMax features a comprehensive library with 26000+ professional symbols that you can use for free with an easy drag and drop .
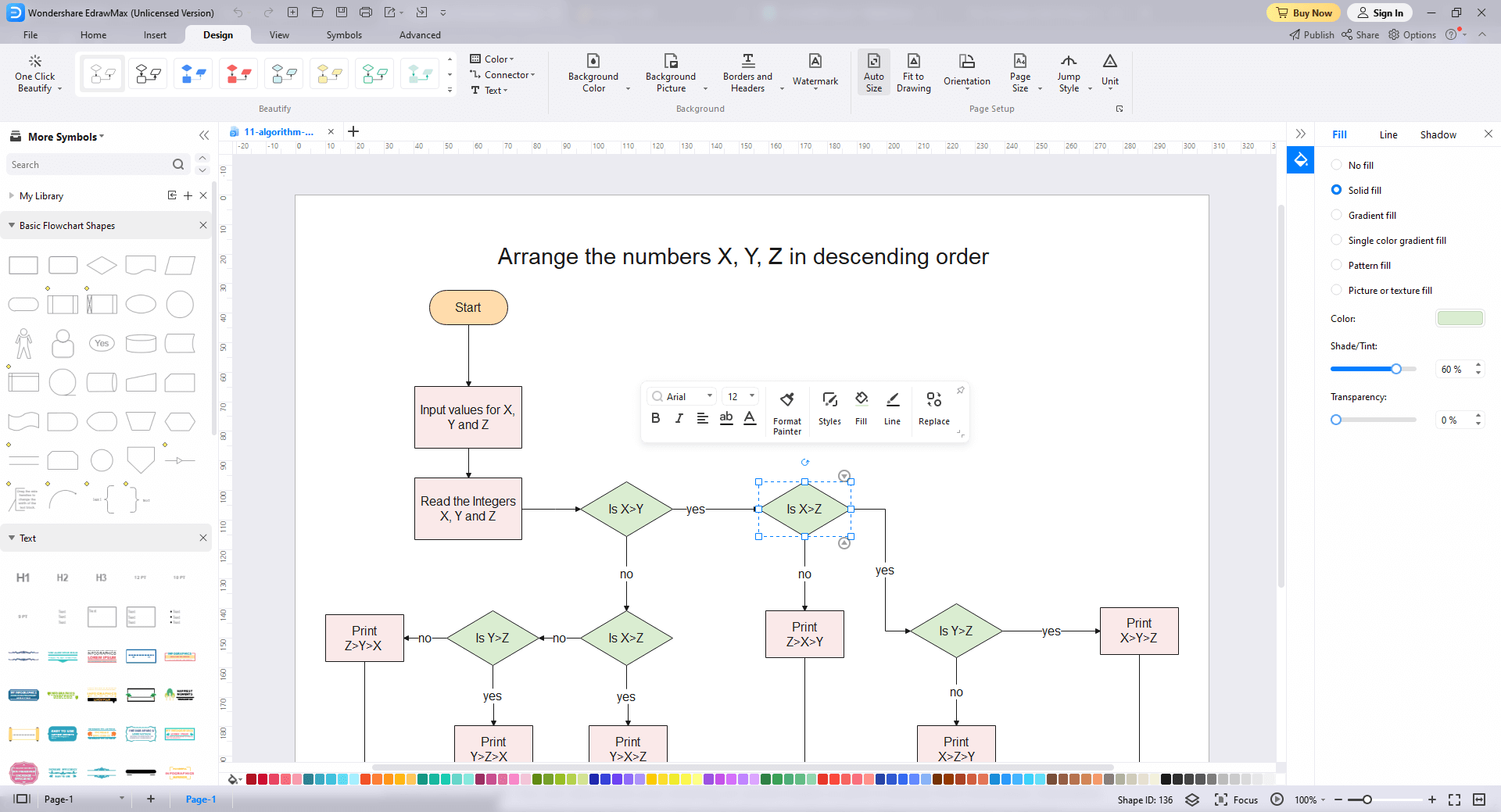
Step5 Save or Export
After you customize the algorithm flowchart template and complete your algorithm flowchart, the next step is to save or export your drawing. EdrawMax supports various document formats, which means you can export your drawing in any format you want, such as Microsoft Office, pdf, graphics, HTML, Visio, and many others. Export your drawing by going to the ' File ' option in the top menu bar and clicking on ' export '. After that, select the document format and click ' ok '. You can also share your drawing on social media or print it directly from EdrawMax. EdrawMax also gives you a presentation mode that you can use to show your drawing to others or your team members.
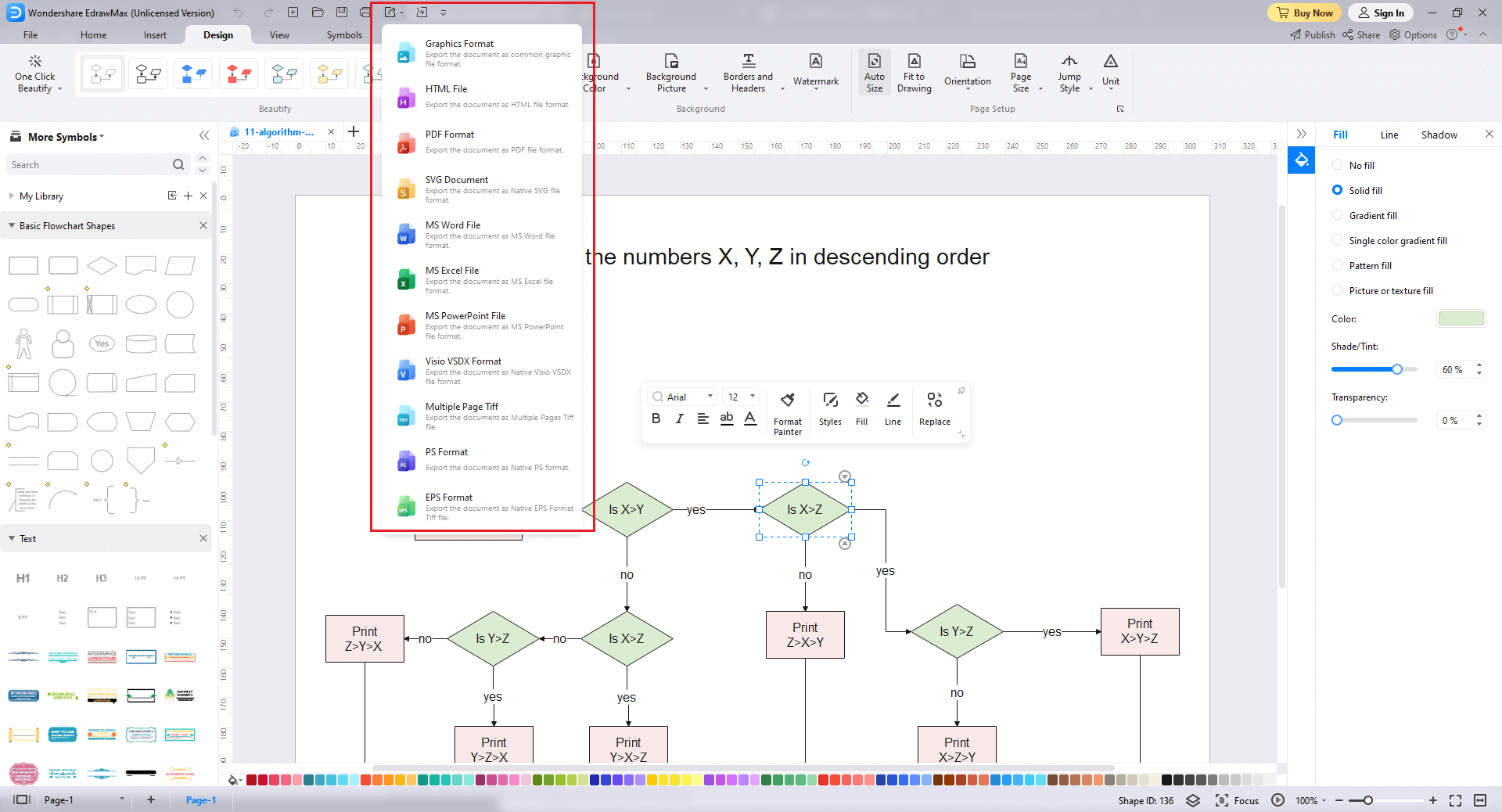
4. Free Algorithm Flowchart Software
EdrawMax is the best free algorithm flowchart software that includes an extensive collection of free templates you can download and customize to fit your needs. How can it help yourself to develop a better strategy? For instance, EdrawMax can help you improve your competitive edge by making a algorithm flowchart. You can draw with shapes, arrows, and lines. Just insert the elements of your diagrams and connect them. There is no limit on creating the number of diagrams you can create.
- EdrawMax offers easily editable templates with various options for displaying relationships and emotions.
- Professionals prefer EdrawMax because of its simple user interface and excellent team collaboration features.
- You can easily share the algorithm flowchart with others using the built-in option.
- Its user-friendly dashboard and simple drag-and-drop feature make it the best free algorithm flowchart software for both beginners and professionals.
- It can export and import files into various formats, including MS Office, Graphics, PDF, HTML, Visio, and others.
- All of your algorithm flowcharts are encrypted for maximum security. Hence, you do not have to be concerned about losing your data.
5. Final Thoughts
Flowcharts are a significant way of understanding different processes and their requirements. The graphical representation will aid you in linking different elements and decisions.
EdrawMax is an impressive software for quickly drawing professional flowcharts and diagrams. The wide array of tools and symbols make everything a breeze. You can easily craft the simplest to the most complex diagrams in no time at all. Give Edraw Max a shot and create professional-grade drawings effortlessly.
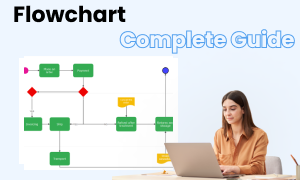
Flowchart Complete Guide
Check this complete guide to know everything about flowchart, like flowchart types, flowchart symbols, and how to make a flowchart.
You May Also Like
Evacuation plan examples & templates, uml class diagram examples, seating chart examples & templates, plumbing piping plan examples & templates, gantt chart examples.
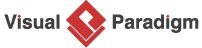
- Demo Videos
- Interactive Product Tours
- Request Demo
Flowchart Tutorial (with Symbols, Guide and Examples)
A flowchart is simply a graphical representation of steps. It shows steps in sequential order and is widely used in presenting the flow of algorithms, workflow or processes. Typically, a flowchart shows the steps as boxes of various kinds, and their order by connecting them with arrows.
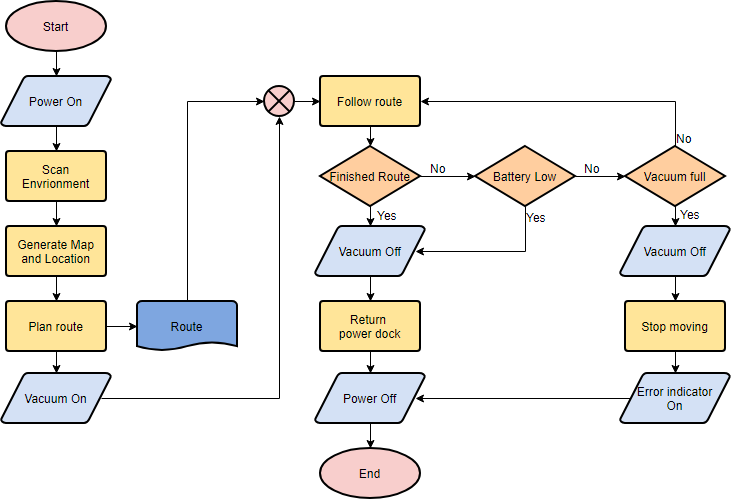
What is a Flowchart?
A flowchart is a graphical representations of steps. It was originated from computer science as a tool for representing algorithms and programming logic but had extended to use in all other kinds of processes. Nowadays, flowcharts play an extremely important role in displaying information and assisting reasoning. They help us visualize complex processes, or make explicit the structure of problems and tasks. A flowchart can also be used to define a process or project to be implemented.
Flowchart Symbols
Different flowchart shapes have different conventional meanings. The meanings of some of the more common shapes are as follows:
The terminator symbol represents the starting or ending point of the system.
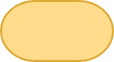
A box indicates some particular operation.
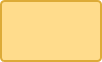
This represents a printout, such as a document or a report.
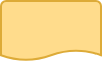
A diamond represents a decision or branching point. Lines coming out from the diamond indicates different possible situations, leading to different sub-processes.

It represents information entering or leaving the system. An input might be an order from a customer. Output can be a product to be delivered.
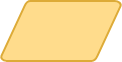
On-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on the same page.

Off-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on a different page.

Delay or Bottleneck
Identifies a delay or a bottleneck.
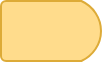
Lines represent the flow of the sequence and direction of a process.

When to Draw Flowchart?
Using a flowchart has a variety of benefits:
- It helps to clarify complex processes.
- It identifies steps that do not add value to the internal or external customer, including delays; needless storage and transportation; unnecessary work, duplication, and added expense; breakdowns in communication.
- It helps team members gain a shared understanding of the process and use this knowledge to collect data, identify problems, focus discussions, and identify resources.
- It serves as a basis for designing new processes.
Flowchart examples
Here are several flowchart examples. See how you can apply a flowchart practically.
Flowchart Example – Medical Service
This is a hospital flowchart example that shows how clinical cases shall be processed. This flowchart uses decision shapes intensively in representing alternative flows.
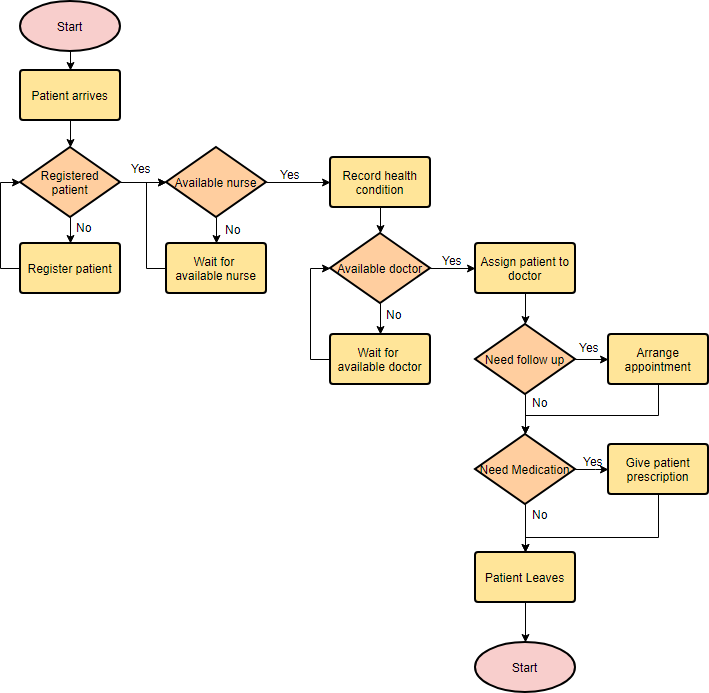
Flowchart Example – Simple Algorithms
A flowchart can also be used in visualizing algorithms, regardless of its complexity. Here is an example that shows how flowchart can be used in showing a simple summation process.
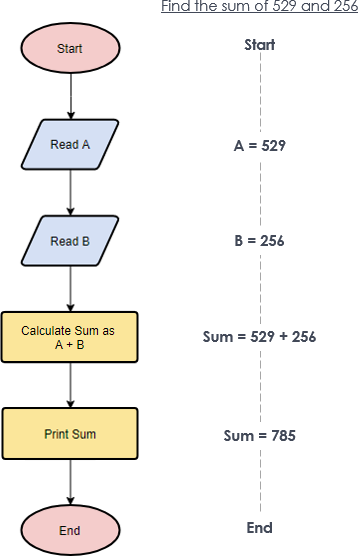
Flowchart Example – Calculate Profit and Loss
The flowchart example below shows how profit and loss can be calculated.
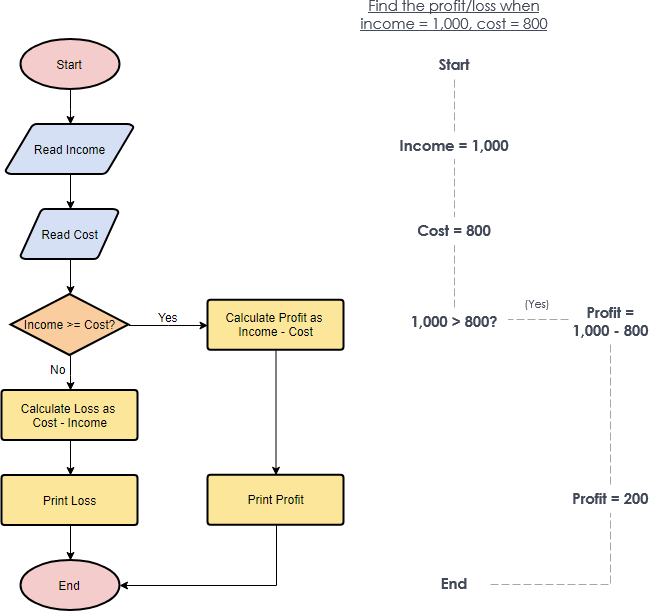
Creating a Flowchart in Visual Paradigm
Let’s see how to draw a flowchart in Visual Paradigm. We will use a very simple flowchart example here. You may expand the example when finished this tutorial.
- Select Diagram > New from the main menu.
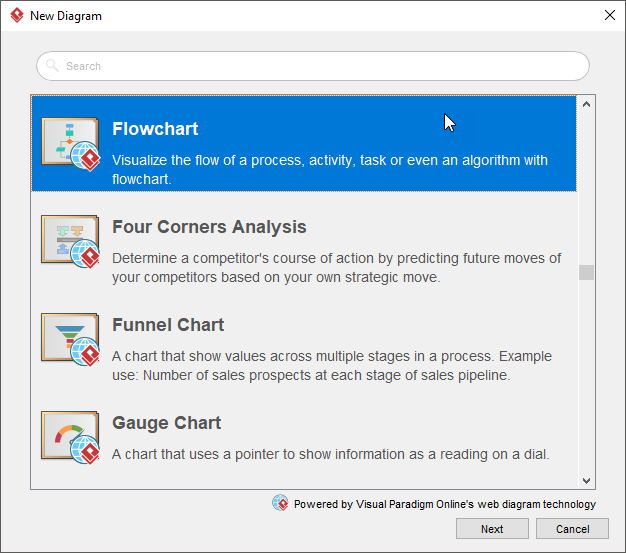
- Enter the name of the flowchart and click OK .
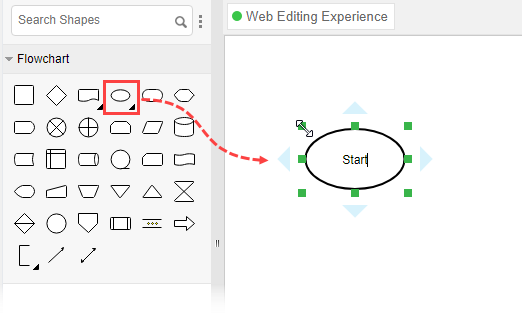
- Enter Add items to Cart as the name of the process.

Turn every software project into a successful one.
We use cookies to offer you a better experience. By visiting our website, you agree to the use of cookies as described in our Cookie Policy .
© 2024 by Visual Paradigm. All rights reserved.
- Privacy statement
- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Difference between Linear and Non-linear Data Structures
- Comparison between Adjacency List and Adjacency Matrix representation of Graph
- Difference between Brute Force and Dynamic Programming
- Difference between data type and data structure
- How to Write DSA Articles on GeeksforGeeks?
- Introduction to Linear Data Structures
- Self Referential Structures
- Introduction to Built-in Data Structures in C#
- UGC-NET | UGC NET CS 2018 July - II | Question 70
- Inbuild Data Structures in Java
- Insert a Node at Front/Beginning of a Linked List
- Insert Node at the End of a Linked List
- Top 50 Most Asked Google Tricky Interview Questions
- Data Structure Alignment : How data is arranged and accessed in Computer Memory?
- How is Blockchain Different From a Linked List?
- Difference between Singly linked list and Doubly linked list
- Difference between Stack and Tree
- Difference between Stack and Queue Data Structures
- Time complexities of different data structures
Difference Between Algorithm and Flowchart
What is an algorithm .
The word Algorithm means “a process or set of rules to be followed in calculations or other problem-solving operations”. Therefore Algorithm refers to a set of rules/instructions that step-by-step define how a work is to be executed in order to get the expected results. Let’s take a look at an example for a better understanding. As a programmer, we are all aware of the Linear Search program. ( Linear Search )
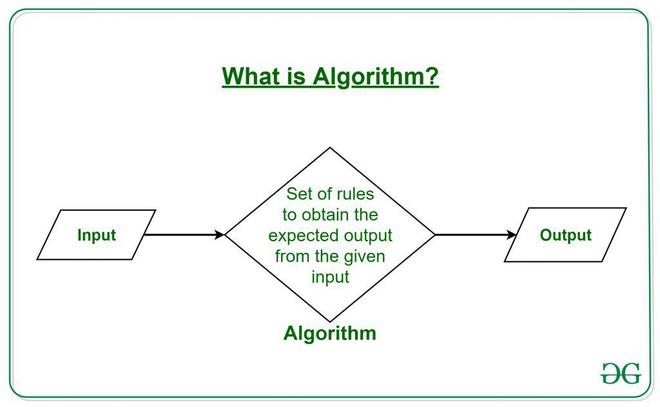
Algorithm of linear search:
Start from the leftmost element of arr[] and one by one compare x with each element of arr[]. If x matches with an element, return the index. If x doesn’t match with any of elements, return -1.
What is a Flowchart ?

Difference between algorithm and flow chart:
Please login to comment..., similar reads.
- Difference Between
- What are Tiktok AI Avatars?
- Poe Introduces A Price-per-message Revenue Model For AI Bot Creators
- Truecaller For Web Now Available For Android Users In India
- Google Introduces New AI-powered Vids App
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This is CS50x 2024. 🎉 Curious how your 2023 work counts toward the 2024 course? See our FAQs if you started in 2023 or earlier. Interested in a verified certificate, a professional certificate, or transfer credit and accreditation ?
This is CS50, Harvard University’s introduction to the intellectual enterprises of computer science and the art of programming, for concentrators and non-concentrators alike, with or without prior programming experience. (Two thirds of CS50 students have never taken CS before.) This course teaches you how to solve problems, both with and without code, with an emphasis on correctness, design, and style. Topics include computational thinking, abstraction, algorithms, data structures, and computer science more generally. Problem sets inspired by the arts, humanities, social sciences, and sciences. More than teach you how to program in one language, this course teaches you how to program fundamentally and how to teach yourself new languages ultimately. The course starts with a traditional but omnipresent language called C that underlies today’s newer languages, via which you’ll learn not only about functions, variables, conditionals, loops, and more, but also about how computers themselves work underneath the hood, memory and all. The course then transitions to Python, a higher-level language that you’ll understand all the more because of C. Toward term’s end, the course introduces SQL, via which you can store data in databases, along with HTML, CSS, and JavaScript, via which you can create web and mobile apps alike. Course culminates in a final project.
How to Take this Course
Even if you are not a student at Harvard, you are welcome to “take” this course for free via this OpenCourseWare by working your way through the course’s eleven weeks of material. For each week, follow this workflow:
And then submit the course’s final project .
If you’d like to submit the course’s problem sets and final project for feedback, be sure to create an edX account , if you haven’t already. Ask questions along the way via any of the course’s communities !
- If interested in a verified certificate from edX , enroll at cs50.edx.org instead.
- in web development, enroll at cs50.edx.org/programs/web instead.
- in artificial intelligence, enroll at cs50.edx.org/programs/ai instead.
- in Python programming, enroll at cs50.edx.org/programs/python instead.
- in game development, enroll at cs50.edx.org/programs/games instead.
- If interested in transfer credit and accreditation from Harvard Extension School , register at web.dce.harvard.edu/extension/csci/e/50 instead.
- If interested in transfer credit and accreditation from Harvard Summer School , register at web.dce.harvard.edu/summer/csci/s/50 instead.
How to Teach this Course
If you are a teacher, you are welcome to adopt or adapt these materials for your own course, per the license .
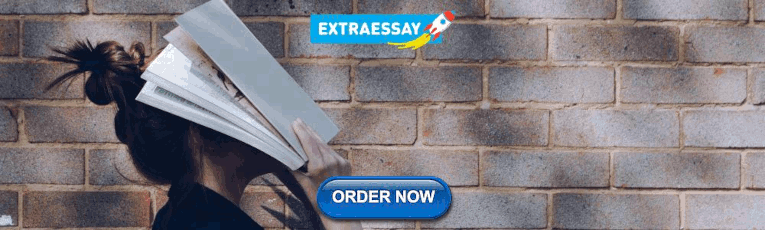
IMAGES
VIDEO
COMMENTS
Program. An algorithm is defined as sequence of steps to solve a problem (task). A flowchart is pictorial (graphical) representation of an algorithm. Set of instructions. Instruction is a command to the computer to do some task. Algorithm can also be defined as a plan to solve a problem and represents its logic. A picture is worth of 1000 words.
By looking at a flow chart one can understand the operations and sequence of operations performed in a system. This is why flowchart is often considered as a blueprint of a design used for solving a specific problem. A flowchart is defined as a symbolic or a graphical representation of an algorithm that uses different standard symbols.
Introduction to Flowcharts. It is basically a diagrammatic representation of an algorithm. Furthermore, it uses various symbols and arrows to describe the beginning, ending, and flow of the program. Moreover, the programmers use it to depicting the flow of data and instructions while problem-solving. Flowcharting is the process of drawing a ...
The algorithm and flowchart are two types of tools to explain the process of a program. This page extends the differences between an algorithm and a flowchart,and how to create your own algorithm and ... Writing a logical step-by-step method to solve the problem is called the algorithm. In other words, an algorithm is a procedure for solving ...
Explain the role of each. 1.3: Activity 3 - Using pseudo-codes and flowcharts to represent algorithms is shared under a CC BY-SA license and was authored, remixed, and/or curated by LibreTexts. The student will learn how to design an algorithm using either a pseudo code or flowchart. Pseudo code is a mixture of English like statements, some ...
To perform a cause-and-effect analysis, follow these steps. 1. Start with a problem statement. The problem statement is usually placed in a box or another shape at the far right of your page. Draw a horizontal line, called a "spine" or "backbone," along the center of the page pointing to your problem statement. 2.
What is a Flowchart? Flowchart is a graphical representation of an algorithm. Programmers often use it as a program-planning tool to solve a problem. It makes use of symbols which are connected among them to indicate the flow of information and processing. The process of drawing a flowchart for an algorithm is known as "flowcharting".
1.1: Activity 1 - Introduction to Algorithms and Problem Solving. In this learning activity section, the learner will be introduced to algorithms and how to write algorithms to solve tasks faced by learners or everyday problems. Examples of the algorithm are also provided with a specific application to everyday problems that the learner is ...
In this article, we will take a real-world problem and attempt to design an algorithm step by step to best solve it using pseudocode and flowcharts. Password validator The problem. Passwords are everywhere, and we create them all the time to access a great array of services. However, it can sometimes be helpful to guide users to make stronger ...
Examples of flowcharts in programming. 1. Add two numbers entered by the user. Flowchart to add two numbers. 2. Find the largest among three different numbers entered by the user. Flowchart to find the largest among three numbers. 3. Find all the roots of a quadratic equation ax2+bx+c=0.
Algorithms An algorithm is A step-by-step list of instructions for solving a problem. The solution must be determined in a finite amount of time. Algorithms can be expressed in many kinds of notations (e.g., natural language, pseudocode, flowcharts, etc.) Flowcharts A flowchart is one way to represent an algorithm and uses the following symbols.
ALGORITHM AND FLOW CHART | Lecture 1 2013 Amir yasseen Mahdi | 3 Example 2: One Monday morning, a student is ready to go to school but yet he/she has not picked up those books and copies which are required as per timetable. So here picking up books and copies as per timetable is a kind of problem solving.
This video is Part - 1 of Algorithms, Flowcharts, Introduction to Problem Solving Algorithm and Flowchart for Beginners , Introduction to Problem Solving, Al...
Let's try more such Flowchart and pseudocode based problems. Remember, this is the first step to problem solving!! Problem Statement: Find the largest among three different numbers entered by the user. (You can skip to check these steps and try on your own) Step 1: Mark the start of the algorithm. Step 2: Declare a,b,c the three numbers
High-level flowcharts play a key role in this approach to problem solving. They facilitate planning, understanding and decomposing the problem, communicating ideas in an early stage, step-wise implementation and evaluating and reflecting on the solution (and approach) as a whole.
A method of representing the step-by-step logical procedure for solving a problem. Flowchart is diagrammatic representation of an algorithm. It is constructed using different types of boxes and symbols. 2. It contains step-by-step English descriptions, each step representing a particular operation leading to solution of problem.
Algorithms. 1. Introduction. In this tutorial, we'll study how to represent relevant programming structures into a flowchart, thus exploring how to map an algorithm to a flowchart and vice-versa. First, we'll understand why using pseudocode and flowcharts to design an algorithm before actually implementing it with a specific programming ...
Problem-Solving Flowcharts is a graphical representation used to break down problem or process into smaller, manageable parts, identify the root causes and outline a step-by-step solution. It helps in visually organizing information and showing the relationships between various parts of the problem. This type of flowcharts consists of different ...
Welcome to the world of problem-solving with Algorithms and Flowcharts! These two essential tools are like a roadmap for computers, guiding them in making decisions and solving puzzles step by step. In this article, we'll explore what Algorithms and Flowcharts are all about and how they help simplify complex tasks, making them accessible to ...
Algorithms and flowcharts are two different ways of presenting the process of solving a problem. Algorithms consist of steps for solving a particular problem, while in flowcharts, those steps are usually displayed in shapes and process boxes with arrows.
In the New Diagram window, select Flowchart and click Next. You can start from an empty diagram or start from a flowchart template or flowchart example provided. Let's start from a blank diagram. Select Blank and click Next. Enter the name of the flowchart and click OK. Let's start by creating a Start symbol.
Flowchart. 1. An algorithm is a step-by-step procedure to solve a problem. A flowchart is a diagram created with different shapes to show the flow of data. 2. The algorithm is complex to understand. A flowchart is easy to understand. 3. In the algorithm, plain text is used.
This course teaches you how to solve problems, both with and without code, with an emphasis on correctness, design, and style. Topics include computational thinking, abstraction, algorithms, data structures, and computer science more generally. Problem sets inspired by the arts, humanities, social sciences, and sciences.